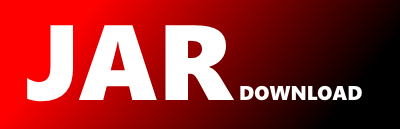
software.amazon.awssdk.services.ecs.model.Tmpfs Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The container path, mount options, and size of the tmpfs mount.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Tmpfs implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONTAINER_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Tmpfs::containerPath)).setter(setter(Builder::containerPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerPath").build()).build();
private static final SdkField SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(Tmpfs::size)).setter(setter(Builder::size))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("size").build()).build();
private static final SdkField> MOUNT_OPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(Tmpfs::mountOptions))
.setter(setter(Builder::mountOptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mountOptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_PATH_FIELD,
SIZE_FIELD, MOUNT_OPTIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String containerPath;
private final Integer size;
private final List mountOptions;
private Tmpfs(BuilderImpl builder) {
this.containerPath = builder.containerPath;
this.size = builder.size;
this.mountOptions = builder.mountOptions;
}
/**
*
* The absolute file path where the tmpfs volume is to be mounted.
*
*
* @return The absolute file path where the tmpfs volume is to be mounted.
*/
public String containerPath() {
return containerPath;
}
/**
*
* The size (in MiB) of the tmpfs volume.
*
*
* @return The size (in MiB) of the tmpfs volume.
*/
public Integer size() {
return size;
}
/**
*
* The list of tmpfs volume mount options.
*
*
* Valid values:
* "defaults" | "ro" | "rw" | "suid" | "nosuid" | "dev" | "nodev" | "exec" | "noexec" | "sync" | "async" | "dirsync" | "remount" | "mand" | "nomand" | "atime" | "noatime" | "diratime" | "nodiratime" | "bind" | "rbind" | "unbindable" | "runbindable" | "private" | "rprivate" | "shared" | "rshared" | "slave" | "rslave" | "relatime" | "norelatime" | "strictatime" | "nostrictatime" | "mode" | "uid" | "gid" | "nr_inodes" | "nr_blocks" | "mpol"
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The list of tmpfs volume mount options.
*
* Valid values:
* "defaults" | "ro" | "rw" | "suid" | "nosuid" | "dev" | "nodev" | "exec" | "noexec" | "sync" | "async" | "dirsync" | "remount" | "mand" | "nomand" | "atime" | "noatime" | "diratime" | "nodiratime" | "bind" | "rbind" | "unbindable" | "runbindable" | "private" | "rprivate" | "shared" | "rshared" | "slave" | "rslave" | "relatime" | "norelatime" | "strictatime" | "nostrictatime" | "mode" | "uid" | "gid" | "nr_inodes" | "nr_blocks" | "mpol"
*/
public List mountOptions() {
return mountOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerPath());
hashCode = 31 * hashCode + Objects.hashCode(size());
hashCode = 31 * hashCode + Objects.hashCode(mountOptions());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Tmpfs)) {
return false;
}
Tmpfs other = (Tmpfs) obj;
return Objects.equals(containerPath(), other.containerPath()) && Objects.equals(size(), other.size())
&& Objects.equals(mountOptions(), other.mountOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Tmpfs").add("ContainerPath", containerPath()).add("Size", size())
.add("MountOptions", mountOptions()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerPath":
return Optional.ofNullable(clazz.cast(containerPath()));
case "size":
return Optional.ofNullable(clazz.cast(size()));
case "mountOptions":
return Optional.ofNullable(clazz.cast(mountOptions()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Valid values:
* "defaults" | "ro" | "rw" | "suid" | "nosuid" | "dev" | "nodev" | "exec" | "noexec" | "sync" | "async" | "dirsync" | "remount" | "mand" | "nomand" | "atime" | "noatime" | "diratime" | "nodiratime" | "bind" | "rbind" | "unbindable" | "runbindable" | "private" | "rprivate" | "shared" | "rshared" | "slave" | "rslave" | "relatime" | "norelatime" | "strictatime" | "nostrictatime" | "mode" | "uid" | "gid" | "nr_inodes" | "nr_blocks" | "mpol"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mountOptions(Collection mountOptions);
/**
*
* The list of tmpfs volume mount options.
*
*
* Valid values:
* "defaults" | "ro" | "rw" | "suid" | "nosuid" | "dev" | "nodev" | "exec" | "noexec" | "sync" | "async" | "dirsync" | "remount" | "mand" | "nomand" | "atime" | "noatime" | "diratime" | "nodiratime" | "bind" | "rbind" | "unbindable" | "runbindable" | "private" | "rprivate" | "shared" | "rshared" | "slave" | "rslave" | "relatime" | "norelatime" | "strictatime" | "nostrictatime" | "mode" | "uid" | "gid" | "nr_inodes" | "nr_blocks" | "mpol"
*
*
* @param mountOptions
* The list of tmpfs volume mount options.
*
* Valid values:
* "defaults" | "ro" | "rw" | "suid" | "nosuid" | "dev" | "nodev" | "exec" | "noexec" | "sync" | "async" | "dirsync" | "remount" | "mand" | "nomand" | "atime" | "noatime" | "diratime" | "nodiratime" | "bind" | "rbind" | "unbindable" | "runbindable" | "private" | "rprivate" | "shared" | "rshared" | "slave" | "rslave" | "relatime" | "norelatime" | "strictatime" | "nostrictatime" | "mode" | "uid" | "gid" | "nr_inodes" | "nr_blocks" | "mpol"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mountOptions(String... mountOptions);
}
static final class BuilderImpl implements Builder {
private String containerPath;
private Integer size;
private List mountOptions = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(Tmpfs model) {
containerPath(model.containerPath);
size(model.size);
mountOptions(model.mountOptions);
}
public final String getContainerPath() {
return containerPath;
}
@Override
public final Builder containerPath(String containerPath) {
this.containerPath = containerPath;
return this;
}
public final void setContainerPath(String containerPath) {
this.containerPath = containerPath;
}
public final Integer getSize() {
return size;
}
@Override
public final Builder size(Integer size) {
this.size = size;
return this;
}
public final void setSize(Integer size) {
this.size = size;
}
public final Collection getMountOptions() {
return mountOptions;
}
@Override
public final Builder mountOptions(Collection mountOptions) {
this.mountOptions = StringListCopier.copy(mountOptions);
return this;
}
@Override
@SafeVarargs
public final Builder mountOptions(String... mountOptions) {
mountOptions(Arrays.asList(mountOptions));
return this;
}
public final void setMountOptions(Collection mountOptions) {
this.mountOptions = StringListCopier.copy(mountOptions);
}
@Override
public Tmpfs build() {
return new Tmpfs(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}