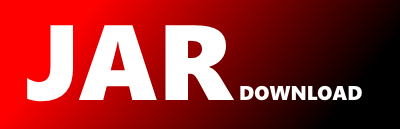
software.amazon.awssdk.services.eks.model.VpcConfigRequest Maven / Gradle / Ivy
Show all versions of eks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eks.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing the VPC configuration to use for an Amazon EKS cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VpcConfigRequest implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> SUBNET_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("subnetIds")
.getter(getter(VpcConfigRequest::subnetIds))
.setter(setter(Builder::subnetIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("subnetIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("securityGroupIds")
.getter(getter(VpcConfigRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("securityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENDPOINT_PUBLIC_ACCESS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("endpointPublicAccess").getter(getter(VpcConfigRequest::endpointPublicAccess))
.setter(setter(Builder::endpointPublicAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpointPublicAccess").build())
.build();
private static final SdkField ENDPOINT_PRIVATE_ACCESS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("endpointPrivateAccess").getter(getter(VpcConfigRequest::endpointPrivateAccess))
.setter(setter(Builder::endpointPrivateAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpointPrivateAccess").build())
.build();
private static final SdkField> PUBLIC_ACCESS_CIDRS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("publicAccessCidrs")
.getter(getter(VpcConfigRequest::publicAccessCidrs))
.setter(setter(Builder::publicAccessCidrs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("publicAccessCidrs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SUBNET_IDS_FIELD,
SECURITY_GROUP_IDS_FIELD, ENDPOINT_PUBLIC_ACCESS_FIELD, ENDPOINT_PRIVATE_ACCESS_FIELD, PUBLIC_ACCESS_CIDRS_FIELD));
private static final long serialVersionUID = 1L;
private final List subnetIds;
private final List securityGroupIds;
private final Boolean endpointPublicAccess;
private final Boolean endpointPrivateAccess;
private final List publicAccessCidrs;
private VpcConfigRequest(BuilderImpl builder) {
this.subnetIds = builder.subnetIds;
this.securityGroupIds = builder.securityGroupIds;
this.endpointPublicAccess = builder.endpointPublicAccess;
this.endpointPrivateAccess = builder.endpointPrivateAccess;
this.publicAccessCidrs = builder.publicAccessCidrs;
}
/**
* For responses, this returns true if the service returned a value for the SubnetIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSubnetIds() {
return subnetIds != null && !(subnetIds instanceof SdkAutoConstructList);
}
/**
*
* Specify subnets for your Amazon EKS nodes. Amazon EKS creates cross-account elastic network interfaces in these
* subnets to allow communication between your nodes and the Kubernetes control plane.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSubnetIds} method.
*
*
* @return Specify subnets for your Amazon EKS nodes. Amazon EKS creates cross-account elastic network interfaces in
* these subnets to allow communication between your nodes and the Kubernetes control plane.
*/
public final List subnetIds() {
return subnetIds;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* Specify one or more security groups for the cross-account elastic network interfaces that Amazon EKS creates to
* use that allow communication between your nodes and the Kubernetes control plane. If you don't specify any
* security groups, then familiarize yourself with the difference between Amazon EKS defaults for clusters deployed
* with Kubernetes:
*
*
* -
*
* 1.14 Amazon EKS platform version eks.2
and earlier
*
*
* -
*
* 1.14 Amazon EKS platform version eks.3
and later
*
*
*
*
* For more information, see Amazon
* EKS security group considerations in the Amazon EKS User Guide .
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return Specify one or more security groups for the cross-account elastic network interfaces that Amazon EKS
* creates to use that allow communication between your nodes and the Kubernetes control plane. If you don't
* specify any security groups, then familiarize yourself with the difference between Amazon EKS defaults
* for clusters deployed with Kubernetes:
*
* -
*
* 1.14 Amazon EKS platform version eks.2
and earlier
*
*
* -
*
* 1.14 Amazon EKS platform version eks.3
and later
*
*
*
*
* For more information, see Amazon EKS security group
* considerations in the Amazon EKS User Guide .
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
*
* Set this value to false
to disable public access to your cluster's Kubernetes API server endpoint.
* If you disable public access, your cluster's Kubernetes API server can only receive requests from within the
* cluster VPC. The default value for this parameter is true
, which enables public access for your
* Kubernetes API server. For more information, see Amazon EKS cluster endpoint access
* control in the Amazon EKS User Guide .
*
*
* @return Set this value to false
to disable public access to your cluster's Kubernetes API server
* endpoint. If you disable public access, your cluster's Kubernetes API server can only receive requests
* from within the cluster VPC. The default value for this parameter is true
, which enables
* public access for your Kubernetes API server. For more information, see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*/
public final Boolean endpointPublicAccess() {
return endpointPublicAccess;
}
/**
*
* Set this value to true
to enable private access for your cluster's Kubernetes API server endpoint.
* If you enable private access, Kubernetes API requests from within your cluster's VPC use the private VPC
* endpoint. The default value for this parameter is false
, which disables private access for your
* Kubernetes API server. If you disable private access and you have nodes or Fargate pods in the cluster, then
* ensure that publicAccessCidrs
includes the necessary CIDR blocks for communication with the nodes or
* Fargate pods. For more information, see Amazon EKS cluster endpoint access
* control in the Amazon EKS User Guide .
*
*
* @return Set this value to true
to enable private access for your cluster's Kubernetes API server
* endpoint. If you enable private access, Kubernetes API requests from within your cluster's VPC use the
* private VPC endpoint. The default value for this parameter is false
, which disables private
* access for your Kubernetes API server. If you disable private access and you have nodes or Fargate pods
* in the cluster, then ensure that publicAccessCidrs
includes the necessary CIDR blocks for
* communication with the nodes or Fargate pods. For more information, see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*/
public final Boolean endpointPrivateAccess() {
return endpointPrivateAccess;
}
/**
* For responses, this returns true if the service returned a value for the PublicAccessCidrs property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPublicAccessCidrs() {
return publicAccessCidrs != null && !(publicAccessCidrs instanceof SdkAutoConstructList);
}
/**
*
* The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint. Communication to
* the endpoint from addresses outside of the CIDR blocks that you specify is denied. The default value is
* 0.0.0.0/0
. If you've disabled private endpoint access and you have nodes or Fargate pods in the
* cluster, then ensure that you specify the necessary CIDR blocks. For more information, see Amazon EKS cluster endpoint access
* control in the Amazon EKS User Guide .
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPublicAccessCidrs} method.
*
*
* @return The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.
* Communication to the endpoint from addresses outside of the CIDR blocks that you specify is denied. The
* default value is 0.0.0.0/0
. If you've disabled private endpoint access and you have nodes or
* Fargate pods in the cluster, then ensure that you specify the necessary CIDR blocks. For more
* information, see Amazon
* EKS cluster endpoint access control in the Amazon EKS User Guide .
*/
public final List publicAccessCidrs() {
return publicAccessCidrs;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasSubnetIds() ? subnetIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(endpointPublicAccess());
hashCode = 31 * hashCode + Objects.hashCode(endpointPrivateAccess());
hashCode = 31 * hashCode + Objects.hashCode(hasPublicAccessCidrs() ? publicAccessCidrs() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VpcConfigRequest)) {
return false;
}
VpcConfigRequest other = (VpcConfigRequest) obj;
return hasSubnetIds() == other.hasSubnetIds() && Objects.equals(subnetIds(), other.subnetIds())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(endpointPublicAccess(), other.endpointPublicAccess())
&& Objects.equals(endpointPrivateAccess(), other.endpointPrivateAccess())
&& hasPublicAccessCidrs() == other.hasPublicAccessCidrs()
&& Objects.equals(publicAccessCidrs(), other.publicAccessCidrs());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VpcConfigRequest").add("SubnetIds", hasSubnetIds() ? subnetIds() : null)
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("EndpointPublicAccess", endpointPublicAccess()).add("EndpointPrivateAccess", endpointPrivateAccess())
.add("PublicAccessCidrs", hasPublicAccessCidrs() ? publicAccessCidrs() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "subnetIds":
return Optional.ofNullable(clazz.cast(subnetIds()));
case "securityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "endpointPublicAccess":
return Optional.ofNullable(clazz.cast(endpointPublicAccess()));
case "endpointPrivateAccess":
return Optional.ofNullable(clazz.cast(endpointPrivateAccess()));
case "publicAccessCidrs":
return Optional.ofNullable(clazz.cast(publicAccessCidrs()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* 1.14 Amazon EKS platform version eks.2
and earlier
*
*
* -
*
* 1.14 Amazon EKS platform version eks.3
and later
*
*
*
*
* For more information, see Amazon EKS security group
* considerations in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroupIds(Collection securityGroupIds);
/**
*
* Specify one or more security groups for the cross-account elastic network interfaces that Amazon EKS creates
* to use that allow communication between your nodes and the Kubernetes control plane. If you don't specify any
* security groups, then familiarize yourself with the difference between Amazon EKS defaults for clusters
* deployed with Kubernetes:
*
*
* -
*
* 1.14 Amazon EKS platform version eks.2
and earlier
*
*
* -
*
* 1.14 Amazon EKS platform version eks.3
and later
*
*
*
*
* For more information, see Amazon EKS security group
* considerations in the Amazon EKS User Guide .
*
*
* @param securityGroupIds
* Specify one or more security groups for the cross-account elastic network interfaces that Amazon EKS
* creates to use that allow communication between your nodes and the Kubernetes control plane. If you
* don't specify any security groups, then familiarize yourself with the difference between Amazon EKS
* defaults for clusters deployed with Kubernetes:
*
* -
*
* 1.14 Amazon EKS platform version eks.2
and earlier
*
*
* -
*
* 1.14 Amazon EKS platform version eks.3
and later
*
*
*
*
* For more information, see Amazon EKS security group
* considerations in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroupIds(String... securityGroupIds);
/**
*
* Set this value to false
to disable public access to your cluster's Kubernetes API server
* endpoint. If you disable public access, your cluster's Kubernetes API server can only receive requests from
* within the cluster VPC. The default value for this parameter is true
, which enables public
* access for your Kubernetes API server. For more information, see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*
*
* @param endpointPublicAccess
* Set this value to false
to disable public access to your cluster's Kubernetes API server
* endpoint. If you disable public access, your cluster's Kubernetes API server can only receive requests
* from within the cluster VPC. The default value for this parameter is true
, which enables
* public access for your Kubernetes API server. For more information, see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endpointPublicAccess(Boolean endpointPublicAccess);
/**
*
* Set this value to true
to enable private access for your cluster's Kubernetes API server
* endpoint. If you enable private access, Kubernetes API requests from within your cluster's VPC use the
* private VPC endpoint. The default value for this parameter is false
, which disables private
* access for your Kubernetes API server. If you disable private access and you have nodes or Fargate pods in
* the cluster, then ensure that publicAccessCidrs
includes the necessary CIDR blocks for
* communication with the nodes or Fargate pods. For more information, see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*
*
* @param endpointPrivateAccess
* Set this value to true
to enable private access for your cluster's Kubernetes API server
* endpoint. If you enable private access, Kubernetes API requests from within your cluster's VPC use the
* private VPC endpoint. The default value for this parameter is false
, which disables
* private access for your Kubernetes API server. If you disable private access and you have nodes or
* Fargate pods in the cluster, then ensure that publicAccessCidrs
includes the necessary
* CIDR blocks for communication with the nodes or Fargate pods. For more information, see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endpointPrivateAccess(Boolean endpointPrivateAccess);
/**
*
* The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.
* Communication to the endpoint from addresses outside of the CIDR blocks that you specify is denied. The
* default value is 0.0.0.0/0
. If you've disabled private endpoint access and you have nodes or
* Fargate pods in the cluster, then ensure that you specify the necessary CIDR blocks. For more information,
* see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
*
*
* @param publicAccessCidrs
* The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.
* Communication to the endpoint from addresses outside of the CIDR blocks that you specify is denied.
* The default value is 0.0.0.0/0
. If you've disabled private endpoint access and you have
* nodes or Fargate pods in the cluster, then ensure that you specify the necessary CIDR blocks. For more
* information, see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder publicAccessCidrs(Collection publicAccessCidrs);
/**
*
* The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.
* Communication to the endpoint from addresses outside of the CIDR blocks that you specify is denied. The
* default value is 0.0.0.0/0
. If you've disabled private endpoint access and you have nodes or
* Fargate pods in the cluster, then ensure that you specify the necessary CIDR blocks. For more information,
* see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
*
*
* @param publicAccessCidrs
* The CIDR blocks that are allowed access to your cluster's public Kubernetes API server endpoint.
* Communication to the endpoint from addresses outside of the CIDR blocks that you specify is denied.
* The default value is 0.0.0.0/0
. If you've disabled private endpoint access and you have
* nodes or Fargate pods in the cluster, then ensure that you specify the necessary CIDR blocks. For more
* information, see Amazon EKS cluster
* endpoint access control in the Amazon EKS User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder publicAccessCidrs(String... publicAccessCidrs);
}
static final class BuilderImpl implements Builder {
private List subnetIds = DefaultSdkAutoConstructList.getInstance();
private List securityGroupIds = DefaultSdkAutoConstructList.getInstance();
private Boolean endpointPublicAccess;
private Boolean endpointPrivateAccess;
private List publicAccessCidrs = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(VpcConfigRequest model) {
subnetIds(model.subnetIds);
securityGroupIds(model.securityGroupIds);
endpointPublicAccess(model.endpointPublicAccess);
endpointPrivateAccess(model.endpointPrivateAccess);
publicAccessCidrs(model.publicAccessCidrs);
}
public final Collection getSubnetIds() {
if (subnetIds instanceof SdkAutoConstructList) {
return null;
}
return subnetIds;
}
public final void setSubnetIds(Collection subnetIds) {
this.subnetIds = StringListCopier.copy(subnetIds);
}
@Override
public final Builder subnetIds(Collection subnetIds) {
this.subnetIds = StringListCopier.copy(subnetIds);
return this;
}
@Override
@SafeVarargs
public final Builder subnetIds(String... subnetIds) {
subnetIds(Arrays.asList(subnetIds));
return this;
}
public final Collection getSecurityGroupIds() {
if (securityGroupIds instanceof SdkAutoConstructList) {
return null;
}
return securityGroupIds;
}
public final void setSecurityGroupIds(Collection securityGroupIds) {
this.securityGroupIds = StringListCopier.copy(securityGroupIds);
}
@Override
public final Builder securityGroupIds(Collection securityGroupIds) {
this.securityGroupIds = StringListCopier.copy(securityGroupIds);
return this;
}
@Override
@SafeVarargs
public final Builder securityGroupIds(String... securityGroupIds) {
securityGroupIds(Arrays.asList(securityGroupIds));
return this;
}
public final Boolean getEndpointPublicAccess() {
return endpointPublicAccess;
}
public final void setEndpointPublicAccess(Boolean endpointPublicAccess) {
this.endpointPublicAccess = endpointPublicAccess;
}
@Override
public final Builder endpointPublicAccess(Boolean endpointPublicAccess) {
this.endpointPublicAccess = endpointPublicAccess;
return this;
}
public final Boolean getEndpointPrivateAccess() {
return endpointPrivateAccess;
}
public final void setEndpointPrivateAccess(Boolean endpointPrivateAccess) {
this.endpointPrivateAccess = endpointPrivateAccess;
}
@Override
public final Builder endpointPrivateAccess(Boolean endpointPrivateAccess) {
this.endpointPrivateAccess = endpointPrivateAccess;
return this;
}
public final Collection getPublicAccessCidrs() {
if (publicAccessCidrs instanceof SdkAutoConstructList) {
return null;
}
return publicAccessCidrs;
}
public final void setPublicAccessCidrs(Collection publicAccessCidrs) {
this.publicAccessCidrs = StringListCopier.copy(publicAccessCidrs);
}
@Override
public final Builder publicAccessCidrs(Collection publicAccessCidrs) {
this.publicAccessCidrs = StringListCopier.copy(publicAccessCidrs);
return this;
}
@Override
@SafeVarargs
public final Builder publicAccessCidrs(String... publicAccessCidrs) {
publicAccessCidrs(Arrays.asList(publicAccessCidrs));
return this;
}
@Override
public VpcConfigRequest build() {
return new VpcConfigRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}