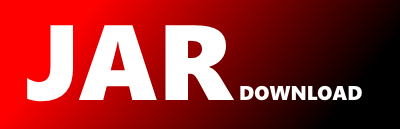
software.amazon.awssdk.services.eks.EksClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eks;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.eks.model.AccessDeniedException;
import software.amazon.awssdk.services.eks.model.AssociateAccessPolicyRequest;
import software.amazon.awssdk.services.eks.model.AssociateAccessPolicyResponse;
import software.amazon.awssdk.services.eks.model.AssociateEncryptionConfigRequest;
import software.amazon.awssdk.services.eks.model.AssociateEncryptionConfigResponse;
import software.amazon.awssdk.services.eks.model.AssociateIdentityProviderConfigRequest;
import software.amazon.awssdk.services.eks.model.AssociateIdentityProviderConfigResponse;
import software.amazon.awssdk.services.eks.model.BadRequestException;
import software.amazon.awssdk.services.eks.model.ClientException;
import software.amazon.awssdk.services.eks.model.CreateAccessEntryRequest;
import software.amazon.awssdk.services.eks.model.CreateAccessEntryResponse;
import software.amazon.awssdk.services.eks.model.CreateAddonRequest;
import software.amazon.awssdk.services.eks.model.CreateAddonResponse;
import software.amazon.awssdk.services.eks.model.CreateClusterRequest;
import software.amazon.awssdk.services.eks.model.CreateClusterResponse;
import software.amazon.awssdk.services.eks.model.CreateEksAnywhereSubscriptionRequest;
import software.amazon.awssdk.services.eks.model.CreateEksAnywhereSubscriptionResponse;
import software.amazon.awssdk.services.eks.model.CreateFargateProfileRequest;
import software.amazon.awssdk.services.eks.model.CreateFargateProfileResponse;
import software.amazon.awssdk.services.eks.model.CreateNodegroupRequest;
import software.amazon.awssdk.services.eks.model.CreateNodegroupResponse;
import software.amazon.awssdk.services.eks.model.CreatePodIdentityAssociationRequest;
import software.amazon.awssdk.services.eks.model.CreatePodIdentityAssociationResponse;
import software.amazon.awssdk.services.eks.model.DeleteAccessEntryRequest;
import software.amazon.awssdk.services.eks.model.DeleteAccessEntryResponse;
import software.amazon.awssdk.services.eks.model.DeleteAddonRequest;
import software.amazon.awssdk.services.eks.model.DeleteAddonResponse;
import software.amazon.awssdk.services.eks.model.DeleteClusterRequest;
import software.amazon.awssdk.services.eks.model.DeleteClusterResponse;
import software.amazon.awssdk.services.eks.model.DeleteEksAnywhereSubscriptionRequest;
import software.amazon.awssdk.services.eks.model.DeleteEksAnywhereSubscriptionResponse;
import software.amazon.awssdk.services.eks.model.DeleteFargateProfileRequest;
import software.amazon.awssdk.services.eks.model.DeleteFargateProfileResponse;
import software.amazon.awssdk.services.eks.model.DeleteNodegroupRequest;
import software.amazon.awssdk.services.eks.model.DeleteNodegroupResponse;
import software.amazon.awssdk.services.eks.model.DeletePodIdentityAssociationRequest;
import software.amazon.awssdk.services.eks.model.DeletePodIdentityAssociationResponse;
import software.amazon.awssdk.services.eks.model.DeregisterClusterRequest;
import software.amazon.awssdk.services.eks.model.DeregisterClusterResponse;
import software.amazon.awssdk.services.eks.model.DescribeAccessEntryRequest;
import software.amazon.awssdk.services.eks.model.DescribeAccessEntryResponse;
import software.amazon.awssdk.services.eks.model.DescribeAddonConfigurationRequest;
import software.amazon.awssdk.services.eks.model.DescribeAddonConfigurationResponse;
import software.amazon.awssdk.services.eks.model.DescribeAddonRequest;
import software.amazon.awssdk.services.eks.model.DescribeAddonResponse;
import software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest;
import software.amazon.awssdk.services.eks.model.DescribeAddonVersionsResponse;
import software.amazon.awssdk.services.eks.model.DescribeClusterRequest;
import software.amazon.awssdk.services.eks.model.DescribeClusterResponse;
import software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest;
import software.amazon.awssdk.services.eks.model.DescribeClusterVersionsResponse;
import software.amazon.awssdk.services.eks.model.DescribeEksAnywhereSubscriptionRequest;
import software.amazon.awssdk.services.eks.model.DescribeEksAnywhereSubscriptionResponse;
import software.amazon.awssdk.services.eks.model.DescribeFargateProfileRequest;
import software.amazon.awssdk.services.eks.model.DescribeFargateProfileResponse;
import software.amazon.awssdk.services.eks.model.DescribeIdentityProviderConfigRequest;
import software.amazon.awssdk.services.eks.model.DescribeIdentityProviderConfigResponse;
import software.amazon.awssdk.services.eks.model.DescribeInsightRequest;
import software.amazon.awssdk.services.eks.model.DescribeInsightResponse;
import software.amazon.awssdk.services.eks.model.DescribeNodegroupRequest;
import software.amazon.awssdk.services.eks.model.DescribeNodegroupResponse;
import software.amazon.awssdk.services.eks.model.DescribePodIdentityAssociationRequest;
import software.amazon.awssdk.services.eks.model.DescribePodIdentityAssociationResponse;
import software.amazon.awssdk.services.eks.model.DescribeUpdateRequest;
import software.amazon.awssdk.services.eks.model.DescribeUpdateResponse;
import software.amazon.awssdk.services.eks.model.DisassociateAccessPolicyRequest;
import software.amazon.awssdk.services.eks.model.DisassociateAccessPolicyResponse;
import software.amazon.awssdk.services.eks.model.DisassociateIdentityProviderConfigRequest;
import software.amazon.awssdk.services.eks.model.DisassociateIdentityProviderConfigResponse;
import software.amazon.awssdk.services.eks.model.EksException;
import software.amazon.awssdk.services.eks.model.InvalidParameterException;
import software.amazon.awssdk.services.eks.model.InvalidRequestException;
import software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest;
import software.amazon.awssdk.services.eks.model.ListAccessEntriesResponse;
import software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest;
import software.amazon.awssdk.services.eks.model.ListAccessPoliciesResponse;
import software.amazon.awssdk.services.eks.model.ListAddonsRequest;
import software.amazon.awssdk.services.eks.model.ListAddonsResponse;
import software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest;
import software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesResponse;
import software.amazon.awssdk.services.eks.model.ListClustersRequest;
import software.amazon.awssdk.services.eks.model.ListClustersResponse;
import software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest;
import software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsResponse;
import software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest;
import software.amazon.awssdk.services.eks.model.ListFargateProfilesResponse;
import software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest;
import software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsResponse;
import software.amazon.awssdk.services.eks.model.ListInsightsRequest;
import software.amazon.awssdk.services.eks.model.ListInsightsResponse;
import software.amazon.awssdk.services.eks.model.ListNodegroupsRequest;
import software.amazon.awssdk.services.eks.model.ListNodegroupsResponse;
import software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest;
import software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsResponse;
import software.amazon.awssdk.services.eks.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.eks.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.eks.model.ListUpdatesRequest;
import software.amazon.awssdk.services.eks.model.ListUpdatesResponse;
import software.amazon.awssdk.services.eks.model.NotFoundException;
import software.amazon.awssdk.services.eks.model.RegisterClusterRequest;
import software.amazon.awssdk.services.eks.model.RegisterClusterResponse;
import software.amazon.awssdk.services.eks.model.ResourceInUseException;
import software.amazon.awssdk.services.eks.model.ResourceLimitExceededException;
import software.amazon.awssdk.services.eks.model.ResourceNotFoundException;
import software.amazon.awssdk.services.eks.model.ResourcePropagationDelayException;
import software.amazon.awssdk.services.eks.model.ServerException;
import software.amazon.awssdk.services.eks.model.ServiceUnavailableException;
import software.amazon.awssdk.services.eks.model.TagResourceRequest;
import software.amazon.awssdk.services.eks.model.TagResourceResponse;
import software.amazon.awssdk.services.eks.model.UnsupportedAvailabilityZoneException;
import software.amazon.awssdk.services.eks.model.UntagResourceRequest;
import software.amazon.awssdk.services.eks.model.UntagResourceResponse;
import software.amazon.awssdk.services.eks.model.UpdateAccessEntryRequest;
import software.amazon.awssdk.services.eks.model.UpdateAccessEntryResponse;
import software.amazon.awssdk.services.eks.model.UpdateAddonRequest;
import software.amazon.awssdk.services.eks.model.UpdateAddonResponse;
import software.amazon.awssdk.services.eks.model.UpdateClusterConfigRequest;
import software.amazon.awssdk.services.eks.model.UpdateClusterConfigResponse;
import software.amazon.awssdk.services.eks.model.UpdateClusterVersionRequest;
import software.amazon.awssdk.services.eks.model.UpdateClusterVersionResponse;
import software.amazon.awssdk.services.eks.model.UpdateEksAnywhereSubscriptionRequest;
import software.amazon.awssdk.services.eks.model.UpdateEksAnywhereSubscriptionResponse;
import software.amazon.awssdk.services.eks.model.UpdateNodegroupConfigRequest;
import software.amazon.awssdk.services.eks.model.UpdateNodegroupConfigResponse;
import software.amazon.awssdk.services.eks.model.UpdateNodegroupVersionRequest;
import software.amazon.awssdk.services.eks.model.UpdateNodegroupVersionResponse;
import software.amazon.awssdk.services.eks.model.UpdatePodIdentityAssociationRequest;
import software.amazon.awssdk.services.eks.model.UpdatePodIdentityAssociationResponse;
import software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable;
import software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable;
import software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable;
import software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable;
import software.amazon.awssdk.services.eks.paginators.ListAddonsIterable;
import software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable;
import software.amazon.awssdk.services.eks.paginators.ListClustersIterable;
import software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable;
import software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable;
import software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable;
import software.amazon.awssdk.services.eks.paginators.ListInsightsIterable;
import software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable;
import software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable;
import software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable;
import software.amazon.awssdk.services.eks.waiters.EksWaiter;
/**
* Service client for accessing Amazon EKS. This can be created using the static {@link #builder()} method.
*
*
* Amazon Elastic Kubernetes Service (Amazon EKS) is a managed service that makes it easy for you to run Kubernetes on
* Amazon Web Services without needing to setup or maintain your own Kubernetes control plane. Kubernetes is an
* open-source system for automating the deployment, scaling, and management of containerized applications.
*
*
* Amazon EKS runs up-to-date versions of the open-source Kubernetes software, so you can use all the existing plugins
* and tooling from the Kubernetes community. Applications running on Amazon EKS are fully compatible with applications
* running on any standard Kubernetes environment, whether running in on-premises data centers or public clouds. This
* means that you can easily migrate any standard Kubernetes application to Amazon EKS without any code modification
* required.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EksClient extends AwsClient {
String SERVICE_NAME = "eks";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "eks";
/**
*
* Associates an access policy and its scope to an access entry. For more information about associating access
* policies, see Associating and
* disassociating access policies to and from access entries in the Amazon EKS User Guide.
*
*
* @param associateAccessPolicyRequest
* @return Result of the AssociateAccessPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateAccessPolicy
* @see AWS API
* Documentation
*/
default AssociateAccessPolicyResponse associateAccessPolicy(AssociateAccessPolicyRequest associateAccessPolicyRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Associates an access policy and its scope to an access entry. For more information about associating access
* policies, see Associating and
* disassociating access policies to and from access entries in the Amazon EKS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateAccessPolicyRequest.Builder} avoiding the
* need to create one manually via {@link AssociateAccessPolicyRequest#builder()}
*
*
* @param associateAccessPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.AssociateAccessPolicyRequest.Builder} to create a
* request.
* @return Result of the AssociateAccessPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateAccessPolicy
* @see AWS API
* Documentation
*/
default AssociateAccessPolicyResponse associateAccessPolicy(
Consumer associateAccessPolicyRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return associateAccessPolicy(AssociateAccessPolicyRequest.builder().applyMutation(associateAccessPolicyRequest).build());
}
/**
*
* Associates an encryption configuration to an existing cluster.
*
*
* Use this API to enable encryption on existing clusters that don't already have encryption enabled. This allows
* you to implement a defense-in-depth security strategy without migrating applications to new Amazon EKS clusters.
*
*
* @param associateEncryptionConfigRequest
* @return Result of the AssociateEncryptionConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateEncryptionConfig
* @see AWS
* API Documentation
*/
default AssociateEncryptionConfigResponse associateEncryptionConfig(
AssociateEncryptionConfigRequest associateEncryptionConfigRequest) throws InvalidParameterException, ClientException,
ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Associates an encryption configuration to an existing cluster.
*
*
* Use this API to enable encryption on existing clusters that don't already have encryption enabled. This allows
* you to implement a defense-in-depth security strategy without migrating applications to new Amazon EKS clusters.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateEncryptionConfigRequest.Builder} avoiding
* the need to create one manually via {@link AssociateEncryptionConfigRequest#builder()}
*
*
* @param associateEncryptionConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.AssociateEncryptionConfigRequest.Builder} to create a
* request.
* @return Result of the AssociateEncryptionConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateEncryptionConfig
* @see AWS
* API Documentation
*/
default AssociateEncryptionConfigResponse associateEncryptionConfig(
Consumer associateEncryptionConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return associateEncryptionConfig(AssociateEncryptionConfigRequest.builder()
.applyMutation(associateEncryptionConfigRequest).build());
}
/**
*
* Associates an identity provider configuration to a cluster.
*
*
* If you want to authenticate identities using an identity provider, you can create an identity provider
* configuration and associate it to your cluster. After configuring authentication to your cluster you can create
* Kubernetes Role
and ClusterRole
objects, assign permissions to them, and then bind them
* to the identities using Kubernetes RoleBinding
and ClusterRoleBinding
objects. For more
* information see Using RBAC
* Authorization in the Kubernetes documentation.
*
*
* @param associateIdentityProviderConfigRequest
* @return Result of the AssociateIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateIdentityProviderConfig
* @see AWS API Documentation
*/
default AssociateIdentityProviderConfigResponse associateIdentityProviderConfig(
AssociateIdentityProviderConfigRequest associateIdentityProviderConfigRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Associates an identity provider configuration to a cluster.
*
*
* If you want to authenticate identities using an identity provider, you can create an identity provider
* configuration and associate it to your cluster. After configuring authentication to your cluster you can create
* Kubernetes Role
and ClusterRole
objects, assign permissions to them, and then bind them
* to the identities using Kubernetes RoleBinding
and ClusterRoleBinding
objects. For more
* information see Using RBAC
* Authorization in the Kubernetes documentation.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateIdentityProviderConfigRequest.Builder}
* avoiding the need to create one manually via {@link AssociateIdentityProviderConfigRequest#builder()}
*
*
* @param associateIdentityProviderConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.AssociateIdentityProviderConfigRequest.Builder} to create
* a request.
* @return Result of the AssociateIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.AssociateIdentityProviderConfig
* @see AWS API Documentation
*/
default AssociateIdentityProviderConfigResponse associateIdentityProviderConfig(
Consumer associateIdentityProviderConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return associateIdentityProviderConfig(AssociateIdentityProviderConfigRequest.builder()
.applyMutation(associateIdentityProviderConfigRequest).build());
}
/**
*
* Creates an access entry.
*
*
* An access entry allows an IAM principal to access your cluster. Access entries can replace the need to maintain
* entries in the aws-auth
ConfigMap
for authentication. You have the following options
* for authorizing an IAM principal to access Kubernetes objects on your cluster: Kubernetes role-based access
* control (RBAC), Amazon EKS, or both. Kubernetes RBAC authorization requires you to create and manage Kubernetes
* Role
, ClusterRole
, RoleBinding
, and ClusterRoleBinding
* objects, in addition to managing access entries. If you use Amazon EKS authorization exclusively, you don't need
* to create and manage Kubernetes Role
, ClusterRole
, RoleBinding
, and
* ClusterRoleBinding
objects.
*
*
* For more information about access entries, see Access entries in the Amazon
* EKS User Guide.
*
*
* @param createAccessEntryRequest
* @return Result of the CreateAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateAccessEntry
* @see AWS API
* Documentation
*/
default CreateAccessEntryResponse createAccessEntry(CreateAccessEntryRequest createAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
ResourceLimitExceededException, ResourceInUseException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an access entry.
*
*
* An access entry allows an IAM principal to access your cluster. Access entries can replace the need to maintain
* entries in the aws-auth
ConfigMap
for authentication. You have the following options
* for authorizing an IAM principal to access Kubernetes objects on your cluster: Kubernetes role-based access
* control (RBAC), Amazon EKS, or both. Kubernetes RBAC authorization requires you to create and manage Kubernetes
* Role
, ClusterRole
, RoleBinding
, and ClusterRoleBinding
* objects, in addition to managing access entries. If you use Amazon EKS authorization exclusively, you don't need
* to create and manage Kubernetes Role
, ClusterRole
, RoleBinding
, and
* ClusterRoleBinding
objects.
*
*
* For more information about access entries, see Access entries in the Amazon
* EKS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAccessEntryRequest.Builder} avoiding the need
* to create one manually via {@link CreateAccessEntryRequest#builder()}
*
*
* @param createAccessEntryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateAccessEntryRequest.Builder} to create a request.
* @return Result of the CreateAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateAccessEntry
* @see AWS API
* Documentation
*/
default CreateAccessEntryResponse createAccessEntry(Consumer createAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
ResourceLimitExceededException, ResourceInUseException, AwsServiceException, SdkClientException, EksException {
return createAccessEntry(CreateAccessEntryRequest.builder().applyMutation(createAccessEntryRequest).build());
}
/**
*
* Creates an Amazon EKS add-on.
*
*
* Amazon EKS add-ons help to automate the provisioning and lifecycle management of common operational software for
* Amazon EKS clusters. For more information, see Amazon EKS add-ons in the Amazon
* EKS User Guide.
*
*
* @param createAddonRequest
* @return Result of the CreateAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateAddon
* @see AWS API
* Documentation
*/
default CreateAddonResponse createAddon(CreateAddonRequest createAddonRequest) throws InvalidParameterException,
InvalidRequestException, ResourceNotFoundException, ResourceInUseException, ClientException, ServerException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Amazon EKS add-on.
*
*
* Amazon EKS add-ons help to automate the provisioning and lifecycle management of common operational software for
* Amazon EKS clusters. For more information, see Amazon EKS add-ons in the Amazon
* EKS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAddonRequest.Builder} avoiding the need to
* create one manually via {@link CreateAddonRequest#builder()}
*
*
* @param createAddonRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateAddonRequest.Builder} to create a request.
* @return Result of the CreateAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateAddon
* @see AWS API
* Documentation
*/
default CreateAddonResponse createAddon(Consumer createAddonRequest)
throws InvalidParameterException, InvalidRequestException, ResourceNotFoundException, ResourceInUseException,
ClientException, ServerException, AwsServiceException, SdkClientException, EksException {
return createAddon(CreateAddonRequest.builder().applyMutation(createAddonRequest).build());
}
/**
*
* Creates an Amazon EKS control plane.
*
*
* The Amazon EKS control plane consists of control plane instances that run the Kubernetes software, such as
* etcd
and the API server. The control plane runs in an account managed by Amazon Web Services, and
* the Kubernetes API is exposed by the Amazon EKS API server endpoint. Each Amazon EKS cluster control plane is
* single tenant and unique. It runs on its own set of Amazon EC2 instances.
*
*
* The cluster control plane is provisioned across multiple Availability Zones and fronted by an Elastic Load
* Balancing Network Load Balancer. Amazon EKS also provisions elastic network interfaces in your VPC subnets to
* provide connectivity from the control plane instances to the nodes (for example, to support
* kubectl exec
, logs
, and proxy
data flows).
*
*
* Amazon EKS nodes run in your Amazon Web Services account and connect to your cluster's control plane over the
* Kubernetes API server endpoint and a certificate file that is created for your cluster.
*
*
* You can use the endpointPublicAccess
and endpointPrivateAccess
parameters to enable or
* disable public and private access to your cluster's Kubernetes API server endpoint. By default, public access is
* enabled, and private access is disabled. For more information, see Amazon EKS Cluster Endpoint Access
* Control in the Amazon EKS User Guide .
*
*
* You can use the logging
parameter to enable or disable exporting the Kubernetes control plane logs
* for your cluster to CloudWatch Logs. By default, cluster control plane logs aren't exported to CloudWatch Logs.
* For more information, see Amazon EKS Cluster Control Plane
* Logs in the Amazon EKS User Guide .
*
*
*
* CloudWatch Logs ingestion, archive storage, and data scanning rates apply to exported control plane logs. For
* more information, see CloudWatch Pricing.
*
*
*
* In most cases, it takes several minutes to create a cluster. After you create an Amazon EKS cluster, you must
* configure your Kubernetes tooling to communicate with the API server and launch nodes into your cluster. For more
* information, see Allowing users to
* access your cluster and Launching Amazon EKS nodes in the
* Amazon EKS User Guide.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws UnsupportedAvailabilityZoneException
* At least one of your specified cluster subnets is in an Availability Zone that does not support Amazon
* EKS. The exception output specifies the supported Availability Zones for your account, from which you can
* choose subnets for your cluster.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateCluster
* @see AWS API
* Documentation
*/
default CreateClusterResponse createCluster(CreateClusterRequest createClusterRequest) throws ResourceInUseException,
ResourceLimitExceededException, InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, UnsupportedAvailabilityZoneException, AwsServiceException, SdkClientException,
EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Amazon EKS control plane.
*
*
* The Amazon EKS control plane consists of control plane instances that run the Kubernetes software, such as
* etcd
and the API server. The control plane runs in an account managed by Amazon Web Services, and
* the Kubernetes API is exposed by the Amazon EKS API server endpoint. Each Amazon EKS cluster control plane is
* single tenant and unique. It runs on its own set of Amazon EC2 instances.
*
*
* The cluster control plane is provisioned across multiple Availability Zones and fronted by an Elastic Load
* Balancing Network Load Balancer. Amazon EKS also provisions elastic network interfaces in your VPC subnets to
* provide connectivity from the control plane instances to the nodes (for example, to support
* kubectl exec
, logs
, and proxy
data flows).
*
*
* Amazon EKS nodes run in your Amazon Web Services account and connect to your cluster's control plane over the
* Kubernetes API server endpoint and a certificate file that is created for your cluster.
*
*
* You can use the endpointPublicAccess
and endpointPrivateAccess
parameters to enable or
* disable public and private access to your cluster's Kubernetes API server endpoint. By default, public access is
* enabled, and private access is disabled. For more information, see Amazon EKS Cluster Endpoint Access
* Control in the Amazon EKS User Guide .
*
*
* You can use the logging
parameter to enable or disable exporting the Kubernetes control plane logs
* for your cluster to CloudWatch Logs. By default, cluster control plane logs aren't exported to CloudWatch Logs.
* For more information, see Amazon EKS Cluster Control Plane
* Logs in the Amazon EKS User Guide .
*
*
*
* CloudWatch Logs ingestion, archive storage, and data scanning rates apply to exported control plane logs. For
* more information, see CloudWatch Pricing.
*
*
*
* In most cases, it takes several minutes to create a cluster. After you create an Amazon EKS cluster, you must
* configure your Kubernetes tooling to communicate with the API server and launch nodes into your cluster. For more
* information, see Allowing users to
* access your cluster and Launching Amazon EKS nodes in the
* Amazon EKS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateClusterRequest.Builder} avoiding the need to
* create one manually via {@link CreateClusterRequest#builder()}
*
*
* @param createClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateClusterRequest.Builder} to create a request.
* @return Result of the CreateCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws UnsupportedAvailabilityZoneException
* At least one of your specified cluster subnets is in an Availability Zone that does not support Amazon
* EKS. The exception output specifies the supported Availability Zones for your account, from which you can
* choose subnets for your cluster.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateCluster
* @see AWS API
* Documentation
*/
default CreateClusterResponse createCluster(Consumer createClusterRequest)
throws ResourceInUseException, ResourceLimitExceededException, InvalidParameterException, ClientException,
ServerException, ServiceUnavailableException, UnsupportedAvailabilityZoneException, AwsServiceException,
SdkClientException, EksException {
return createCluster(CreateClusterRequest.builder().applyMutation(createClusterRequest).build());
}
/**
*
* Creates an EKS Anywhere subscription. When a subscription is created, it is a contract agreement for the length
* of the term specified in the request. Licenses that are used to validate support are provisioned in Amazon Web
* Services License Manager and the caller account is granted access to EKS Anywhere Curated Packages.
*
*
* @param createEksAnywhereSubscriptionRequest
* @return Result of the CreateEksAnywhereSubscription operation returned by the service.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateEksAnywhereSubscription
* @see AWS API Documentation
*/
default CreateEksAnywhereSubscriptionResponse createEksAnywhereSubscription(
CreateEksAnywhereSubscriptionRequest createEksAnywhereSubscriptionRequest) throws ResourceLimitExceededException,
InvalidParameterException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an EKS Anywhere subscription. When a subscription is created, it is a contract agreement for the length
* of the term specified in the request. Licenses that are used to validate support are provisioned in Amazon Web
* Services License Manager and the caller account is granted access to EKS Anywhere Curated Packages.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEksAnywhereSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link CreateEksAnywhereSubscriptionRequest#builder()}
*
*
* @param createEksAnywhereSubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateEksAnywhereSubscriptionRequest.Builder} to create a
* request.
* @return Result of the CreateEksAnywhereSubscription operation returned by the service.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateEksAnywhereSubscription
* @see AWS API Documentation
*/
default CreateEksAnywhereSubscriptionResponse createEksAnywhereSubscription(
Consumer createEksAnywhereSubscriptionRequest)
throws ResourceLimitExceededException, InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return createEksAnywhereSubscription(CreateEksAnywhereSubscriptionRequest.builder()
.applyMutation(createEksAnywhereSubscriptionRequest).build());
}
/**
*
* Creates an Fargate profile for your Amazon EKS cluster. You must have at least one Fargate profile in a cluster
* to be able to run pods on Fargate.
*
*
* The Fargate profile allows an administrator to declare which pods run on Fargate and specify which pods run on
* which Fargate profile. This declaration is done through the profile’s selectors. Each profile can have up to five
* selectors that contain a namespace and labels. A namespace is required for every selector. The label field
* consists of multiple optional key-value pairs. Pods that match the selectors are scheduled on Fargate. If a
* to-be-scheduled pod matches any of the selectors in the Fargate profile, then that pod is run on Fargate.
*
*
* When you create a Fargate profile, you must specify a pod execution role to use with the pods that are scheduled
* with the profile. This role is added to the cluster's Kubernetes Role Based Access Control (RBAC) for
* authorization so that the kubelet
that is running on the Fargate infrastructure can register with
* your Amazon EKS cluster so that it can appear in your cluster as a node. The pod execution role also provides IAM
* permissions to the Fargate infrastructure to allow read access to Amazon ECR image repositories. For more
* information, see Pod Execution
* Role in the Amazon EKS User Guide.
*
*
* Fargate profiles are immutable. However, you can create a new updated profile to replace an existing profile and
* then delete the original after the updated profile has finished creating.
*
*
* If any Fargate profiles in a cluster are in the DELETING
status, you must wait for that Fargate
* profile to finish deleting before you can create any other profiles in that cluster.
*
*
* For more information, see Fargate
* profile in the Amazon EKS User Guide.
*
*
* @param createFargateProfileRequest
* @return Result of the CreateFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws UnsupportedAvailabilityZoneException
* At least one of your specified cluster subnets is in an Availability Zone that does not support Amazon
* EKS. The exception output specifies the supported Availability Zones for your account, from which you can
* choose subnets for your cluster.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateFargateProfile
* @see AWS API
* Documentation
*/
default CreateFargateProfileResponse createFargateProfile(CreateFargateProfileRequest createFargateProfileRequest)
throws InvalidParameterException, InvalidRequestException, ClientException, ServerException,
ResourceLimitExceededException, UnsupportedAvailabilityZoneException, AwsServiceException, SdkClientException,
EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Fargate profile for your Amazon EKS cluster. You must have at least one Fargate profile in a cluster
* to be able to run pods on Fargate.
*
*
* The Fargate profile allows an administrator to declare which pods run on Fargate and specify which pods run on
* which Fargate profile. This declaration is done through the profile’s selectors. Each profile can have up to five
* selectors that contain a namespace and labels. A namespace is required for every selector. The label field
* consists of multiple optional key-value pairs. Pods that match the selectors are scheduled on Fargate. If a
* to-be-scheduled pod matches any of the selectors in the Fargate profile, then that pod is run on Fargate.
*
*
* When you create a Fargate profile, you must specify a pod execution role to use with the pods that are scheduled
* with the profile. This role is added to the cluster's Kubernetes Role Based Access Control (RBAC) for
* authorization so that the kubelet
that is running on the Fargate infrastructure can register with
* your Amazon EKS cluster so that it can appear in your cluster as a node. The pod execution role also provides IAM
* permissions to the Fargate infrastructure to allow read access to Amazon ECR image repositories. For more
* information, see Pod Execution
* Role in the Amazon EKS User Guide.
*
*
* Fargate profiles are immutable. However, you can create a new updated profile to replace an existing profile and
* then delete the original after the updated profile has finished creating.
*
*
* If any Fargate profiles in a cluster are in the DELETING
status, you must wait for that Fargate
* profile to finish deleting before you can create any other profiles in that cluster.
*
*
* For more information, see Fargate
* profile in the Amazon EKS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateFargateProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateFargateProfileRequest#builder()}
*
*
* @param createFargateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateFargateProfileRequest.Builder} to create a request.
* @return Result of the CreateFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws UnsupportedAvailabilityZoneException
* At least one of your specified cluster subnets is in an Availability Zone that does not support Amazon
* EKS. The exception output specifies the supported Availability Zones for your account, from which you can
* choose subnets for your cluster.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateFargateProfile
* @see AWS API
* Documentation
*/
default CreateFargateProfileResponse createFargateProfile(
Consumer createFargateProfileRequest) throws InvalidParameterException,
InvalidRequestException, ClientException, ServerException, ResourceLimitExceededException,
UnsupportedAvailabilityZoneException, AwsServiceException, SdkClientException, EksException {
return createFargateProfile(CreateFargateProfileRequest.builder().applyMutation(createFargateProfileRequest).build());
}
/**
*
* Creates a managed node group for an Amazon EKS cluster.
*
*
* You can only create a node group for your cluster that is equal to the current Kubernetes version for the
* cluster. All node groups are created with the latest AMI release version for the respective minor Kubernetes
* version of the cluster, unless you deploy a custom AMI using a launch template. For more information about using
* launch templates, see Customizing managed nodes with
* launch templates.
*
*
* An Amazon EKS managed node group is an Amazon EC2 Auto Scaling group and associated Amazon EC2 instances that are
* managed by Amazon Web Services for an Amazon EKS cluster. For more information, see Managed node groups in the
* Amazon EKS User Guide.
*
*
*
* Windows AMI types are only supported for commercial Amazon Web Services Regions that support Windows on Amazon
* EKS.
*
*
*
* @param createNodegroupRequest
* @return Result of the CreateNodegroup operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateNodegroup
* @see AWS API
* Documentation
*/
default CreateNodegroupResponse createNodegroup(CreateNodegroupRequest createNodegroupRequest) throws ResourceInUseException,
ResourceLimitExceededException, InvalidRequestException, InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a managed node group for an Amazon EKS cluster.
*
*
* You can only create a node group for your cluster that is equal to the current Kubernetes version for the
* cluster. All node groups are created with the latest AMI release version for the respective minor Kubernetes
* version of the cluster, unless you deploy a custom AMI using a launch template. For more information about using
* launch templates, see Customizing managed nodes with
* launch templates.
*
*
* An Amazon EKS managed node group is an Amazon EC2 Auto Scaling group and associated Amazon EC2 instances that are
* managed by Amazon Web Services for an Amazon EKS cluster. For more information, see Managed node groups in the
* Amazon EKS User Guide.
*
*
*
* Windows AMI types are only supported for commercial Amazon Web Services Regions that support Windows on Amazon
* EKS.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNodegroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateNodegroupRequest#builder()}
*
*
* @param createNodegroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreateNodegroupRequest.Builder} to create a request.
* @return Result of the CreateNodegroup operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreateNodegroup
* @see AWS API
* Documentation
*/
default CreateNodegroupResponse createNodegroup(Consumer createNodegroupRequest)
throws ResourceInUseException, ResourceLimitExceededException, InvalidRequestException, InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return createNodegroup(CreateNodegroupRequest.builder().applyMutation(createNodegroupRequest).build());
}
/**
*
* Creates an EKS Pod Identity association between a service account in an Amazon EKS cluster and an IAM role with
* EKS Pod Identity. Use EKS Pod Identity to give temporary IAM credentials to pods and the credentials are
* rotated automatically.
*
*
* Amazon EKS Pod Identity associations provide the ability to manage credentials for your applications, similar to
* the way that Amazon EC2 instance profiles provide credentials to Amazon EC2 instances.
*
*
* If a pod uses a service account that has an association, Amazon EKS sets environment variables in the containers
* of the pod. The environment variables configure the Amazon Web Services SDKs, including the Command Line
* Interface, to use the EKS Pod Identity credentials.
*
*
* Pod Identity is a simpler method than IAM roles for service accounts, as this method doesn't use OIDC
* identity providers. Additionally, you can configure a role for Pod Identity once, and reuse it across clusters.
*
*
* @param createPodIdentityAssociationRequest
* @return Result of the CreatePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreatePodIdentityAssociation
* @see AWS API Documentation
*/
default CreatePodIdentityAssociationResponse createPodIdentityAssociation(
CreatePodIdentityAssociationRequest createPodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, ResourceLimitExceededException,
ResourceInUseException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an EKS Pod Identity association between a service account in an Amazon EKS cluster and an IAM role with
* EKS Pod Identity. Use EKS Pod Identity to give temporary IAM credentials to pods and the credentials are
* rotated automatically.
*
*
* Amazon EKS Pod Identity associations provide the ability to manage credentials for your applications, similar to
* the way that Amazon EC2 instance profiles provide credentials to Amazon EC2 instances.
*
*
* If a pod uses a service account that has an association, Amazon EKS sets environment variables in the containers
* of the pod. The environment variables configure the Amazon Web Services SDKs, including the Command Line
* Interface, to use the EKS Pod Identity credentials.
*
*
* Pod Identity is a simpler method than IAM roles for service accounts, as this method doesn't use OIDC
* identity providers. Additionally, you can configure a role for Pod Identity once, and reuse it across clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePodIdentityAssociationRequest.Builder}
* avoiding the need to create one manually via {@link CreatePodIdentityAssociationRequest#builder()}
*
*
* @param createPodIdentityAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.CreatePodIdentityAssociationRequest.Builder} to create a
* request.
* @return Result of the CreatePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.CreatePodIdentityAssociation
* @see AWS API Documentation
*/
default CreatePodIdentityAssociationResponse createPodIdentityAssociation(
Consumer createPodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, ResourceLimitExceededException,
ResourceInUseException, AwsServiceException, SdkClientException, EksException {
return createPodIdentityAssociation(CreatePodIdentityAssociationRequest.builder()
.applyMutation(createPodIdentityAssociationRequest).build());
}
/**
*
* Deletes an access entry.
*
*
* Deleting an access entry of a type other than Standard
can cause your cluster to function
* improperly. If you delete an access entry in error, you can recreate it.
*
*
* @param deleteAccessEntryRequest
* @return Result of the DeleteAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteAccessEntry
* @see AWS API
* Documentation
*/
default DeleteAccessEntryResponse deleteAccessEntry(DeleteAccessEntryRequest deleteAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException,
EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an access entry.
*
*
* Deleting an access entry of a type other than Standard
can cause your cluster to function
* improperly. If you delete an access entry in error, you can recreate it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccessEntryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAccessEntryRequest#builder()}
*
*
* @param deleteAccessEntryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteAccessEntryRequest.Builder} to create a request.
* @return Result of the DeleteAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteAccessEntry
* @see AWS API
* Documentation
*/
default DeleteAccessEntryResponse deleteAccessEntry(Consumer deleteAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException,
EksException {
return deleteAccessEntry(DeleteAccessEntryRequest.builder().applyMutation(deleteAccessEntryRequest).build());
}
/**
*
* Deletes an Amazon EKS add-on.
*
*
* When you remove an add-on, it's deleted from the cluster. You can always manually start an add-on on the cluster
* using the Kubernetes API.
*
*
* @param deleteAddonRequest
* @return Result of the DeleteAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteAddon
* @see AWS API
* Documentation
*/
default DeleteAddonResponse deleteAddon(DeleteAddonRequest deleteAddonRequest) throws InvalidParameterException,
InvalidRequestException, ResourceNotFoundException, ClientException, ServerException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon EKS add-on.
*
*
* When you remove an add-on, it's deleted from the cluster. You can always manually start an add-on on the cluster
* using the Kubernetes API.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAddonRequest.Builder} avoiding the need to
* create one manually via {@link DeleteAddonRequest#builder()}
*
*
* @param deleteAddonRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteAddonRequest.Builder} to create a request.
* @return Result of the DeleteAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteAddon
* @see AWS API
* Documentation
*/
default DeleteAddonResponse deleteAddon(Consumer deleteAddonRequest)
throws InvalidParameterException, InvalidRequestException, ResourceNotFoundException, ClientException,
ServerException, AwsServiceException, SdkClientException, EksException {
return deleteAddon(DeleteAddonRequest.builder().applyMutation(deleteAddonRequest).build());
}
/**
*
* Deletes an Amazon EKS cluster control plane.
*
*
* If you have active services in your cluster that are associated with a load balancer, you must delete those
* services before deleting the cluster so that the load balancers are deleted properly. Otherwise, you can have
* orphaned resources in your VPC that prevent you from being able to delete the VPC. For more information, see Deleting a cluster in the
* Amazon EKS User Guide.
*
*
* If you have managed node groups or Fargate profiles attached to the cluster, you must delete them first. For more
* information, see DeleteNodgroup
and DeleteFargateProfile
.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteCluster
* @see AWS API
* Documentation
*/
default DeleteClusterResponse deleteCluster(DeleteClusterRequest deleteClusterRequest) throws ResourceInUseException,
ResourceNotFoundException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon EKS cluster control plane.
*
*
* If you have active services in your cluster that are associated with a load balancer, you must delete those
* services before deleting the cluster so that the load balancers are deleted properly. Otherwise, you can have
* orphaned resources in your VPC that prevent you from being able to delete the VPC. For more information, see Deleting a cluster in the
* Amazon EKS User Guide.
*
*
* If you have managed node groups or Fargate profiles attached to the cluster, you must delete them first. For more
* information, see DeleteNodgroup
and DeleteFargateProfile
.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteClusterRequest.Builder} avoiding the need to
* create one manually via {@link DeleteClusterRequest#builder()}
*
*
* @param deleteClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteClusterRequest.Builder} to create a request.
* @return Result of the DeleteCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteCluster
* @see AWS API
* Documentation
*/
default DeleteClusterResponse deleteCluster(Consumer deleteClusterRequest)
throws ResourceInUseException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return deleteCluster(DeleteClusterRequest.builder().applyMutation(deleteClusterRequest).build());
}
/**
*
* Deletes an expired or inactive subscription. Deleting inactive subscriptions removes them from the Amazon Web
* Services Management Console view and from list/describe API responses. Subscriptions can only be cancelled within
* 7 days of creation and are cancelled by creating a ticket in the Amazon Web Services Support Center.
*
*
* @param deleteEksAnywhereSubscriptionRequest
* @return Result of the DeleteEksAnywhereSubscription operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteEksAnywhereSubscription
* @see AWS API Documentation
*/
default DeleteEksAnywhereSubscriptionResponse deleteEksAnywhereSubscription(
DeleteEksAnywhereSubscriptionRequest deleteEksAnywhereSubscriptionRequest) throws ResourceNotFoundException,
ClientException, InvalidRequestException, ServerException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an expired or inactive subscription. Deleting inactive subscriptions removes them from the Amazon Web
* Services Management Console view and from list/describe API responses. Subscriptions can only be cancelled within
* 7 days of creation and are cancelled by creating a ticket in the Amazon Web Services Support Center.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEksAnywhereSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteEksAnywhereSubscriptionRequest#builder()}
*
*
* @param deleteEksAnywhereSubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteEksAnywhereSubscriptionRequest.Builder} to create a
* request.
* @return Result of the DeleteEksAnywhereSubscription operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteEksAnywhereSubscription
* @see AWS API Documentation
*/
default DeleteEksAnywhereSubscriptionResponse deleteEksAnywhereSubscription(
Consumer deleteEksAnywhereSubscriptionRequest)
throws ResourceNotFoundException, ClientException, InvalidRequestException, ServerException, AwsServiceException,
SdkClientException, EksException {
return deleteEksAnywhereSubscription(DeleteEksAnywhereSubscriptionRequest.builder()
.applyMutation(deleteEksAnywhereSubscriptionRequest).build());
}
/**
*
* Deletes an Fargate profile.
*
*
* When you delete a Fargate profile, any Pod
running on Fargate that was created with the profile is
* deleted. If the Pod
matches another Fargate profile, then it is scheduled on Fargate with that
* profile. If it no longer matches any Fargate profiles, then it's not scheduled on Fargate and may remain in a
* pending state.
*
*
* Only one Fargate profile in a cluster can be in the DELETING
status at a time. You must wait for a
* Fargate profile to finish deleting before you can delete any other profiles in that cluster.
*
*
* @param deleteFargateProfileRequest
* @return Result of the DeleteFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteFargateProfile
* @see AWS API
* Documentation
*/
default DeleteFargateProfileResponse deleteFargateProfile(DeleteFargateProfileRequest deleteFargateProfileRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Fargate profile.
*
*
* When you delete a Fargate profile, any Pod
running on Fargate that was created with the profile is
* deleted. If the Pod
matches another Fargate profile, then it is scheduled on Fargate with that
* profile. If it no longer matches any Fargate profiles, then it's not scheduled on Fargate and may remain in a
* pending state.
*
*
* Only one Fargate profile in a cluster can be in the DELETING
status at a time. You must wait for a
* Fargate profile to finish deleting before you can delete any other profiles in that cluster.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFargateProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteFargateProfileRequest#builder()}
*
*
* @param deleteFargateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteFargateProfileRequest.Builder} to create a request.
* @return Result of the DeleteFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteFargateProfile
* @see AWS API
* Documentation
*/
default DeleteFargateProfileResponse deleteFargateProfile(
Consumer deleteFargateProfileRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return deleteFargateProfile(DeleteFargateProfileRequest.builder().applyMutation(deleteFargateProfileRequest).build());
}
/**
*
* Deletes a managed node group.
*
*
* @param deleteNodegroupRequest
* @return Result of the DeleteNodegroup operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteNodegroup
* @see AWS API
* Documentation
*/
default DeleteNodegroupResponse deleteNodegroup(DeleteNodegroupRequest deleteNodegroupRequest) throws ResourceInUseException,
ResourceNotFoundException, InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a managed node group.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNodegroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteNodegroupRequest#builder()}
*
*
* @param deleteNodegroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeleteNodegroupRequest.Builder} to create a request.
* @return Result of the DeleteNodegroup operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeleteNodegroup
* @see AWS API
* Documentation
*/
default DeleteNodegroupResponse deleteNodegroup(Consumer deleteNodegroupRequest)
throws ResourceInUseException, ResourceNotFoundException, InvalidParameterException, ClientException,
ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return deleteNodegroup(DeleteNodegroupRequest.builder().applyMutation(deleteNodegroupRequest).build());
}
/**
*
* Deletes a EKS Pod Identity association.
*
*
* The temporary Amazon Web Services credentials from the previous IAM role session might still be valid until the
* session expiry. If you need to immediately revoke the temporary session credentials, then go to the role in the
* IAM console.
*
*
* @param deletePodIdentityAssociationRequest
* @return Result of the DeletePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeletePodIdentityAssociation
* @see AWS API Documentation
*/
default DeletePodIdentityAssociationResponse deletePodIdentityAssociation(
DeletePodIdentityAssociationRequest deletePodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a EKS Pod Identity association.
*
*
* The temporary Amazon Web Services credentials from the previous IAM role session might still be valid until the
* session expiry. If you need to immediately revoke the temporary session credentials, then go to the role in the
* IAM console.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePodIdentityAssociationRequest.Builder}
* avoiding the need to create one manually via {@link DeletePodIdentityAssociationRequest#builder()}
*
*
* @param deletePodIdentityAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeletePodIdentityAssociationRequest.Builder} to create a
* request.
* @return Result of the DeletePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeletePodIdentityAssociation
* @see AWS API Documentation
*/
default DeletePodIdentityAssociationResponse deletePodIdentityAssociation(
Consumer deletePodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return deletePodIdentityAssociation(DeletePodIdentityAssociationRequest.builder()
.applyMutation(deletePodIdentityAssociationRequest).build());
}
/**
*
* Deregisters a connected cluster to remove it from the Amazon EKS control plane.
*
*
* A connected cluster is a Kubernetes cluster that you've connected to your control plane using the Amazon EKS Connector.
*
*
* @param deregisterClusterRequest
* @return Result of the DeregisterCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The IAM
* principal making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access management in the IAM
* User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeregisterCluster
* @see AWS API
* Documentation
*/
default DeregisterClusterResponse deregisterCluster(DeregisterClusterRequest deregisterClusterRequest)
throws ResourceInUseException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AccessDeniedException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a connected cluster to remove it from the Amazon EKS control plane.
*
*
* A connected cluster is a Kubernetes cluster that you've connected to your control plane using the Amazon EKS Connector.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterClusterRequest.Builder} avoiding the need
* to create one manually via {@link DeregisterClusterRequest#builder()}
*
*
* @param deregisterClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DeregisterClusterRequest.Builder} to create a request.
* @return Result of the DeregisterCluster operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The IAM
* principal making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access management in the IAM
* User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DeregisterCluster
* @see AWS API
* Documentation
*/
default DeregisterClusterResponse deregisterCluster(Consumer deregisterClusterRequest)
throws ResourceInUseException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AccessDeniedException, AwsServiceException, SdkClientException, EksException {
return deregisterCluster(DeregisterClusterRequest.builder().applyMutation(deregisterClusterRequest).build());
}
/**
*
* Describes an access entry.
*
*
* @param describeAccessEntryRequest
* @return Result of the DescribeAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAccessEntry
* @see AWS API
* Documentation
*/
default DescribeAccessEntryResponse describeAccessEntry(DescribeAccessEntryRequest describeAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException,
EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an access entry.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccessEntryRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAccessEntryRequest#builder()}
*
*
* @param describeAccessEntryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeAccessEntryRequest.Builder} to create a request.
* @return Result of the DescribeAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAccessEntry
* @see AWS API
* Documentation
*/
default DescribeAccessEntryResponse describeAccessEntry(
Consumer describeAccessEntryRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return describeAccessEntry(DescribeAccessEntryRequest.builder().applyMutation(describeAccessEntryRequest).build());
}
/**
*
* Describes an Amazon EKS add-on.
*
*
* @param describeAddonRequest
* @return Result of the DescribeAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddon
* @see AWS API
* Documentation
*/
default DescribeAddonResponse describeAddon(DescribeAddonRequest describeAddonRequest) throws InvalidParameterException,
InvalidRequestException, ResourceNotFoundException, ClientException, ServerException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an Amazon EKS add-on.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAddonRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAddonRequest#builder()}
*
*
* @param describeAddonRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeAddonRequest.Builder} to create a request.
* @return Result of the DescribeAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddon
* @see AWS API
* Documentation
*/
default DescribeAddonResponse describeAddon(Consumer describeAddonRequest)
throws InvalidParameterException, InvalidRequestException, ResourceNotFoundException, ClientException,
ServerException, AwsServiceException, SdkClientException, EksException {
return describeAddon(DescribeAddonRequest.builder().applyMutation(describeAddonRequest).build());
}
/**
*
* Returns configuration options.
*
*
* @param describeAddonConfigurationRequest
* @return Result of the DescribeAddonConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonConfiguration
* @see AWS API Documentation
*/
default DescribeAddonConfigurationResponse describeAddonConfiguration(
DescribeAddonConfigurationRequest describeAddonConfigurationRequest) throws ServerException,
ResourceNotFoundException, InvalidParameterException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns configuration options.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAddonConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link DescribeAddonConfigurationRequest#builder()}
*
*
* @param describeAddonConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeAddonConfigurationRequest.Builder} to create a
* request.
* @return Result of the DescribeAddonConfiguration operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonConfiguration
* @see AWS API Documentation
*/
default DescribeAddonConfigurationResponse describeAddonConfiguration(
Consumer describeAddonConfigurationRequest) throws ServerException,
ResourceNotFoundException, InvalidParameterException, AwsServiceException, SdkClientException, EksException {
return describeAddonConfiguration(DescribeAddonConfigurationRequest.builder()
.applyMutation(describeAddonConfigurationRequest).build());
}
/**
*
* Describes the versions for an add-on.
*
*
* Information such as the Kubernetes versions that you can use the add-on with, the owner
,
* publisher
, and the type
of the add-on are returned.
*
*
* @param describeAddonVersionsRequest
* @return Result of the DescribeAddonVersions operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonVersions
* @see AWS API
* Documentation
*/
default DescribeAddonVersionsResponse describeAddonVersions(DescribeAddonVersionsRequest describeAddonVersionsRequest)
throws ServerException, ResourceNotFoundException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the versions for an add-on.
*
*
* Information such as the Kubernetes versions that you can use the add-on with, the owner
,
* publisher
, and the type
of the add-on are returned.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAddonVersionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAddonVersionsRequest#builder()}
*
*
* @param describeAddonVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest.Builder} to create a
* request.
* @return Result of the DescribeAddonVersions operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonVersions
* @see AWS API
* Documentation
*/
default DescribeAddonVersionsResponse describeAddonVersions(
Consumer describeAddonVersionsRequest) throws ServerException,
ResourceNotFoundException, InvalidParameterException, AwsServiceException, SdkClientException, EksException {
return describeAddonVersions(DescribeAddonVersionsRequest.builder().applyMutation(describeAddonVersionsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeAddonVersions(software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client.describeAddonVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client
* .describeAddonVersionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.DescribeAddonVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client.describeAddonVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAddonVersions(software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest)}
* operation.
*
*
* @param describeAddonVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonVersions
* @see AWS API
* Documentation
*/
default DescribeAddonVersionsIterable describeAddonVersionsPaginator(DescribeAddonVersionsRequest describeAddonVersionsRequest)
throws ServerException, ResourceNotFoundException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return new DescribeAddonVersionsIterable(this, describeAddonVersionsRequest);
}
/**
*
* This is a variant of
* {@link #describeAddonVersions(software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client.describeAddonVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client
* .describeAddonVersionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.DescribeAddonVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeAddonVersionsIterable responses = client.describeAddonVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAddonVersions(software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAddonVersionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAddonVersionsRequest#builder()}
*
*
* @param describeAddonVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeAddonVersionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeAddonVersions
* @see AWS API
* Documentation
*/
default DescribeAddonVersionsIterable describeAddonVersionsPaginator(
Consumer describeAddonVersionsRequest) throws ServerException,
ResourceNotFoundException, InvalidParameterException, AwsServiceException, SdkClientException, EksException {
return describeAddonVersionsPaginator(DescribeAddonVersionsRequest.builder().applyMutation(describeAddonVersionsRequest)
.build());
}
/**
*
* Describes an Amazon EKS cluster.
*
*
* The API server endpoint and certificate authority data returned by this operation are required for
* kubelet
and kubectl
to communicate with your Kubernetes API server. For more
* information, see Creating or
* updating a kubeconfig
file for an Amazon EKS cluster.
*
*
*
* The API server endpoint and certificate authority data aren't available until the cluster reaches the
* ACTIVE
state.
*
*
*
* @param describeClusterRequest
* @return Result of the DescribeCluster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeCluster
* @see AWS API
* Documentation
*/
default DescribeClusterResponse describeCluster(DescribeClusterRequest describeClusterRequest)
throws ResourceNotFoundException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an Amazon EKS cluster.
*
*
* The API server endpoint and certificate authority data returned by this operation are required for
* kubelet
and kubectl
to communicate with your Kubernetes API server. For more
* information, see Creating or
* updating a kubeconfig
file for an Amazon EKS cluster.
*
*
*
* The API server endpoint and certificate authority data aren't available until the cluster reaches the
* ACTIVE
state.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClusterRequest.Builder} avoiding the need
* to create one manually via {@link DescribeClusterRequest#builder()}
*
*
* @param describeClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeClusterRequest.Builder} to create a request.
* @return Result of the DescribeCluster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeCluster
* @see AWS API
* Documentation
*/
default DescribeClusterResponse describeCluster(Consumer describeClusterRequest)
throws ResourceNotFoundException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return describeCluster(DescribeClusterRequest.builder().applyMutation(describeClusterRequest).build());
}
/**
*
* Lists available Kubernetes versions for Amazon EKS clusters.
*
*
* @param describeClusterVersionsRequest
* @return Result of the DescribeClusterVersions operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeClusterVersions
* @see AWS
* API Documentation
*/
default DescribeClusterVersionsResponse describeClusterVersions(DescribeClusterVersionsRequest describeClusterVersionsRequest)
throws ServerException, InvalidParameterException, InvalidRequestException, AwsServiceException, SdkClientException,
EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists available Kubernetes versions for Amazon EKS clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClusterVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeClusterVersionsRequest#builder()}
*
*
* @param describeClusterVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest.Builder} to create a
* request.
* @return Result of the DescribeClusterVersions operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeClusterVersions
* @see AWS
* API Documentation
*/
default DescribeClusterVersionsResponse describeClusterVersions(
Consumer describeClusterVersionsRequest) throws ServerException,
InvalidParameterException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return describeClusterVersions(DescribeClusterVersionsRequest.builder().applyMutation(describeClusterVersionsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #describeClusterVersions(software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client.describeClusterVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client
* .describeClusterVersionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.DescribeClusterVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client.describeClusterVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeClusterVersions(software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest)}
* operation.
*
*
* @param describeClusterVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeClusterVersions
* @see AWS
* API Documentation
*/
default DescribeClusterVersionsIterable describeClusterVersionsPaginator(
DescribeClusterVersionsRequest describeClusterVersionsRequest) throws ServerException, InvalidParameterException,
InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return new DescribeClusterVersionsIterable(this, describeClusterVersionsRequest);
}
/**
*
* This is a variant of
* {@link #describeClusterVersions(software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client.describeClusterVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client
* .describeClusterVersionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.DescribeClusterVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.DescribeClusterVersionsIterable responses = client.describeClusterVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeClusterVersions(software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClusterVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeClusterVersionsRequest#builder()}
*
*
* @param describeClusterVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeClusterVersionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeClusterVersions
* @see AWS
* API Documentation
*/
default DescribeClusterVersionsIterable describeClusterVersionsPaginator(
Consumer describeClusterVersionsRequest) throws ServerException,
InvalidParameterException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return describeClusterVersionsPaginator(DescribeClusterVersionsRequest.builder()
.applyMutation(describeClusterVersionsRequest).build());
}
/**
*
* Returns descriptive information about a subscription.
*
*
* @param describeEksAnywhereSubscriptionRequest
* @return Result of the DescribeEksAnywhereSubscription operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeEksAnywhereSubscription
* @see AWS API Documentation
*/
default DescribeEksAnywhereSubscriptionResponse describeEksAnywhereSubscription(
DescribeEksAnywhereSubscriptionRequest describeEksAnywhereSubscriptionRequest) throws ResourceNotFoundException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns descriptive information about a subscription.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEksAnywhereSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEksAnywhereSubscriptionRequest#builder()}
*
*
* @param describeEksAnywhereSubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeEksAnywhereSubscriptionRequest.Builder} to create
* a request.
* @return Result of the DescribeEksAnywhereSubscription operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeEksAnywhereSubscription
* @see AWS API Documentation
*/
default DescribeEksAnywhereSubscriptionResponse describeEksAnywhereSubscription(
Consumer describeEksAnywhereSubscriptionRequest)
throws ResourceNotFoundException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return describeEksAnywhereSubscription(DescribeEksAnywhereSubscriptionRequest.builder()
.applyMutation(describeEksAnywhereSubscriptionRequest).build());
}
/**
*
* Describes an Fargate profile.
*
*
* @param describeFargateProfileRequest
* @return Result of the DescribeFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeFargateProfile
* @see AWS
* API Documentation
*/
default DescribeFargateProfileResponse describeFargateProfile(DescribeFargateProfileRequest describeFargateProfileRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an Fargate profile.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeFargateProfileRequest.Builder} avoiding the
* need to create one manually via {@link DescribeFargateProfileRequest#builder()}
*
*
* @param describeFargateProfileRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeFargateProfileRequest.Builder} to create a
* request.
* @return Result of the DescribeFargateProfile operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeFargateProfile
* @see AWS
* API Documentation
*/
default DescribeFargateProfileResponse describeFargateProfile(
Consumer describeFargateProfileRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return describeFargateProfile(DescribeFargateProfileRequest.builder().applyMutation(describeFargateProfileRequest)
.build());
}
/**
*
* Describes an identity provider configuration.
*
*
* @param describeIdentityProviderConfigRequest
* @return Result of the DescribeIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeIdentityProviderConfig
* @see AWS API Documentation
*/
default DescribeIdentityProviderConfigResponse describeIdentityProviderConfig(
DescribeIdentityProviderConfigRequest describeIdentityProviderConfigRequest) throws InvalidParameterException,
ResourceNotFoundException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an identity provider configuration.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeIdentityProviderConfigRequest.Builder}
* avoiding the need to create one manually via {@link DescribeIdentityProviderConfigRequest#builder()}
*
*
* @param describeIdentityProviderConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeIdentityProviderConfigRequest.Builder} to create
* a request.
* @return Result of the DescribeIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeIdentityProviderConfig
* @see AWS API Documentation
*/
default DescribeIdentityProviderConfigResponse describeIdentityProviderConfig(
Consumer describeIdentityProviderConfigRequest)
throws InvalidParameterException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return describeIdentityProviderConfig(DescribeIdentityProviderConfigRequest.builder()
.applyMutation(describeIdentityProviderConfigRequest).build());
}
/**
*
* Returns details about an insight that you specify using its ID.
*
*
* @param describeInsightRequest
* @return Result of the DescribeInsight operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeInsight
* @see AWS API
* Documentation
*/
default DescribeInsightResponse describeInsight(DescribeInsightRequest describeInsightRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns details about an insight that you specify using its ID.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInsightRequest.Builder} avoiding the need
* to create one manually via {@link DescribeInsightRequest#builder()}
*
*
* @param describeInsightRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeInsightRequest.Builder} to create a request.
* @return Result of the DescribeInsight operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeInsight
* @see AWS API
* Documentation
*/
default DescribeInsightResponse describeInsight(Consumer describeInsightRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return describeInsight(DescribeInsightRequest.builder().applyMutation(describeInsightRequest).build());
}
/**
*
* Describes a managed node group.
*
*
* @param describeNodegroupRequest
* @return Result of the DescribeNodegroup operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeNodegroup
* @see AWS API
* Documentation
*/
default DescribeNodegroupResponse describeNodegroup(DescribeNodegroupRequest describeNodegroupRequest)
throws InvalidParameterException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes a managed node group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeNodegroupRequest.Builder} avoiding the need
* to create one manually via {@link DescribeNodegroupRequest#builder()}
*
*
* @param describeNodegroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeNodegroupRequest.Builder} to create a request.
* @return Result of the DescribeNodegroup operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeNodegroup
* @see AWS API
* Documentation
*/
default DescribeNodegroupResponse describeNodegroup(Consumer describeNodegroupRequest)
throws InvalidParameterException, ResourceNotFoundException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return describeNodegroup(DescribeNodegroupRequest.builder().applyMutation(describeNodegroupRequest).build());
}
/**
*
* Returns descriptive information about an EKS Pod Identity association.
*
*
* This action requires the ID of the association. You can get the ID from the response to the
* CreatePodIdentityAssocation
for newly created associations. Or, you can list the IDs for
* associations with ListPodIdentityAssociations
and filter the list by namespace or service account.
*
*
* @param describePodIdentityAssociationRequest
* @return Result of the DescribePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribePodIdentityAssociation
* @see AWS API Documentation
*/
default DescribePodIdentityAssociationResponse describePodIdentityAssociation(
DescribePodIdentityAssociationRequest describePodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns descriptive information about an EKS Pod Identity association.
*
*
* This action requires the ID of the association. You can get the ID from the response to the
* CreatePodIdentityAssocation
for newly created associations. Or, you can list the IDs for
* associations with ListPodIdentityAssociations
and filter the list by namespace or service account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePodIdentityAssociationRequest.Builder}
* avoiding the need to create one manually via {@link DescribePodIdentityAssociationRequest#builder()}
*
*
* @param describePodIdentityAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribePodIdentityAssociationRequest.Builder} to create
* a request.
* @return Result of the DescribePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribePodIdentityAssociation
* @see AWS API Documentation
*/
default DescribePodIdentityAssociationResponse describePodIdentityAssociation(
Consumer describePodIdentityAssociationRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return describePodIdentityAssociation(DescribePodIdentityAssociationRequest.builder()
.applyMutation(describePodIdentityAssociationRequest).build());
}
/**
*
* Describes an update to an Amazon EKS resource.
*
*
* When the status of the update is Succeeded
, the update is complete. If an update fails, the status
* is Failed
, and an error detail explains the reason for the failure.
*
*
* @param describeUpdateRequest
* Describes an update request.
* @return Result of the DescribeUpdate operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeUpdate
* @see AWS API
* Documentation
*/
default DescribeUpdateResponse describeUpdate(DescribeUpdateRequest describeUpdateRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an update to an Amazon EKS resource.
*
*
* When the status of the update is Succeeded
, the update is complete. If an update fails, the status
* is Failed
, and an error detail explains the reason for the failure.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUpdateRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUpdateRequest#builder()}
*
*
* @param describeUpdateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DescribeUpdateRequest.Builder} to create a request.
* Describes an update request.
* @return Result of the DescribeUpdate operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DescribeUpdate
* @see AWS API
* Documentation
*/
default DescribeUpdateResponse describeUpdate(Consumer describeUpdateRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
return describeUpdate(DescribeUpdateRequest.builder().applyMutation(describeUpdateRequest).build());
}
/**
*
* Disassociates an access policy from an access entry.
*
*
* @param disassociateAccessPolicyRequest
* @return Result of the DisassociateAccessPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DisassociateAccessPolicy
* @see AWS
* API Documentation
*/
default DisassociateAccessPolicyResponse disassociateAccessPolicy(
DisassociateAccessPolicyRequest disassociateAccessPolicyRequest) throws ServerException, ResourceNotFoundException,
InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an access policy from an access entry.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateAccessPolicyRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateAccessPolicyRequest#builder()}
*
*
* @param disassociateAccessPolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DisassociateAccessPolicyRequest.Builder} to create a
* request.
* @return Result of the DisassociateAccessPolicy operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DisassociateAccessPolicy
* @see AWS
* API Documentation
*/
default DisassociateAccessPolicyResponse disassociateAccessPolicy(
Consumer disassociateAccessPolicyRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return disassociateAccessPolicy(DisassociateAccessPolicyRequest.builder().applyMutation(disassociateAccessPolicyRequest)
.build());
}
/**
*
* Disassociates an identity provider configuration from a cluster.
*
*
* If you disassociate an identity provider from your cluster, users included in the provider can no longer access
* the cluster. However, you can still access the cluster with IAM principals.
*
*
* @param disassociateIdentityProviderConfigRequest
* @return Result of the DisassociateIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DisassociateIdentityProviderConfig
* @see AWS API Documentation
*/
default DisassociateIdentityProviderConfigResponse disassociateIdentityProviderConfig(
DisassociateIdentityProviderConfigRequest disassociateIdentityProviderConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an identity provider configuration from a cluster.
*
*
* If you disassociate an identity provider from your cluster, users included in the provider can no longer access
* the cluster. However, you can still access the cluster with IAM principals.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateIdentityProviderConfigRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateIdentityProviderConfigRequest#builder()}
*
*
* @param disassociateIdentityProviderConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.DisassociateIdentityProviderConfigRequest.Builder} to
* create a request.
* @return Result of the DisassociateIdentityProviderConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.DisassociateIdentityProviderConfig
* @see AWS API Documentation
*/
default DisassociateIdentityProviderConfigResponse disassociateIdentityProviderConfig(
Consumer disassociateIdentityProviderConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return disassociateIdentityProviderConfig(DisassociateIdentityProviderConfigRequest.builder()
.applyMutation(disassociateIdentityProviderConfigRequest).build());
}
/**
*
* Lists the access entries for your cluster.
*
*
* @param listAccessEntriesRequest
* @return Result of the ListAccessEntries operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessEntries
* @see AWS API
* Documentation
*/
default ListAccessEntriesResponse listAccessEntries(ListAccessEntriesRequest listAccessEntriesRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the access entries for your cluster.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessEntriesRequest.Builder} avoiding the need
* to create one manually via {@link ListAccessEntriesRequest#builder()}
*
*
* @param listAccessEntriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest.Builder} to create a request.
* @return Result of the ListAccessEntries operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessEntries
* @see AWS API
* Documentation
*/
default ListAccessEntriesResponse listAccessEntries(Consumer listAccessEntriesRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return listAccessEntries(ListAccessEntriesRequest.builder().applyMutation(listAccessEntriesRequest).build());
}
/**
*
* This is a variant of
* {@link #listAccessEntries(software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client.listAccessEntriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client
* .listAccessEntriesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAccessEntriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client.listAccessEntriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessEntries(software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest)} operation.
*
*
* @param listAccessEntriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessEntries
* @see AWS API
* Documentation
*/
default ListAccessEntriesIterable listAccessEntriesPaginator(ListAccessEntriesRequest listAccessEntriesRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return new ListAccessEntriesIterable(this, listAccessEntriesRequest);
}
/**
*
* This is a variant of
* {@link #listAccessEntries(software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client.listAccessEntriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client
* .listAccessEntriesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAccessEntriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessEntriesIterable responses = client.listAccessEntriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessEntries(software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessEntriesRequest.Builder} avoiding the need
* to create one manually via {@link ListAccessEntriesRequest#builder()}
*
*
* @param listAccessEntriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAccessEntriesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessEntries
* @see AWS API
* Documentation
*/
default ListAccessEntriesIterable listAccessEntriesPaginator(
Consumer listAccessEntriesRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return listAccessEntriesPaginator(ListAccessEntriesRequest.builder().applyMutation(listAccessEntriesRequest).build());
}
/**
*
* Lists the available access policies.
*
*
* @param listAccessPoliciesRequest
* @return Result of the ListAccessPolicies operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessPolicies
* @see AWS API
* Documentation
*/
default ListAccessPoliciesResponse listAccessPolicies(ListAccessPoliciesRequest listAccessPoliciesRequest)
throws ServerException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available access policies.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListAccessPoliciesRequest#builder()}
*
*
* @param listAccessPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest.Builder} to create a request.
* @return Result of the ListAccessPolicies operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessPolicies
* @see AWS API
* Documentation
*/
default ListAccessPoliciesResponse listAccessPolicies(Consumer listAccessPoliciesRequest)
throws ServerException, AwsServiceException, SdkClientException, EksException {
return listAccessPolicies(ListAccessPoliciesRequest.builder().applyMutation(listAccessPoliciesRequest).build());
}
/**
*
* This is a variant of
* {@link #listAccessPolicies(software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client.listAccessPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client
* .listAccessPoliciesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAccessPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client.listAccessPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPolicies(software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest)} operation.
*
*
* @param listAccessPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessPolicies
* @see AWS API
* Documentation
*/
default ListAccessPoliciesIterable listAccessPoliciesPaginator(ListAccessPoliciesRequest listAccessPoliciesRequest)
throws ServerException, AwsServiceException, SdkClientException, EksException {
return new ListAccessPoliciesIterable(this, listAccessPoliciesRequest);
}
/**
*
* This is a variant of
* {@link #listAccessPolicies(software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client.listAccessPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client
* .listAccessPoliciesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAccessPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAccessPoliciesIterable responses = client.listAccessPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccessPolicies(software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccessPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListAccessPoliciesRequest#builder()}
*
*
* @param listAccessPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAccessPoliciesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAccessPolicies
* @see AWS API
* Documentation
*/
default ListAccessPoliciesIterable listAccessPoliciesPaginator(
Consumer listAccessPoliciesRequest) throws ServerException, AwsServiceException,
SdkClientException, EksException {
return listAccessPoliciesPaginator(ListAccessPoliciesRequest.builder().applyMutation(listAccessPoliciesRequest).build());
}
/**
*
* Lists the installed add-ons.
*
*
* @param listAddonsRequest
* @return Result of the ListAddons operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAddons
* @see AWS API
* Documentation
*/
default ListAddonsResponse listAddons(ListAddonsRequest listAddonsRequest) throws InvalidParameterException,
InvalidRequestException, ClientException, ResourceNotFoundException, ServerException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the installed add-ons.
*
*
*
* This is a convenience which creates an instance of the {@link ListAddonsRequest.Builder} avoiding the need to
* create one manually via {@link ListAddonsRequest#builder()}
*
*
* @param listAddonsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAddonsRequest.Builder} to create a request.
* @return Result of the ListAddons operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAddons
* @see AWS API
* Documentation
*/
default ListAddonsResponse listAddons(Consumer listAddonsRequest)
throws InvalidParameterException, InvalidRequestException, ClientException, ResourceNotFoundException,
ServerException, AwsServiceException, SdkClientException, EksException {
return listAddons(ListAddonsRequest.builder().applyMutation(listAddonsRequest).build());
}
/**
*
* This is a variant of {@link #listAddons(software.amazon.awssdk.services.eks.model.ListAddonsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAddonsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAddons(software.amazon.awssdk.services.eks.model.ListAddonsRequest)} operation.
*
*
* @param listAddonsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAddons
* @see AWS API
* Documentation
*/
default ListAddonsIterable listAddonsPaginator(ListAddonsRequest listAddonsRequest) throws InvalidParameterException,
InvalidRequestException, ClientException, ResourceNotFoundException, ServerException, AwsServiceException,
SdkClientException, EksException {
return new ListAddonsIterable(this, listAddonsRequest);
}
/**
*
* This is a variant of {@link #listAddons(software.amazon.awssdk.services.eks.model.ListAddonsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAddonsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAddonsIterable responses = client.listAddonsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAddons(software.amazon.awssdk.services.eks.model.ListAddonsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAddonsRequest.Builder} avoiding the need to
* create one manually via {@link ListAddonsRequest#builder()}
*
*
* @param listAddonsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAddonsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAddons
* @see AWS API
* Documentation
*/
default ListAddonsIterable listAddonsPaginator(Consumer listAddonsRequest)
throws InvalidParameterException, InvalidRequestException, ClientException, ResourceNotFoundException,
ServerException, AwsServiceException, SdkClientException, EksException {
return listAddonsPaginator(ListAddonsRequest.builder().applyMutation(listAddonsRequest).build());
}
/**
*
* Lists the access policies associated with an access entry.
*
*
* @param listAssociatedAccessPoliciesRequest
* @return Result of the ListAssociatedAccessPolicies operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAssociatedAccessPolicies
* @see AWS API Documentation
*/
default ListAssociatedAccessPoliciesResponse listAssociatedAccessPolicies(
ListAssociatedAccessPoliciesRequest listAssociatedAccessPoliciesRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the access policies associated with an access entry.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedAccessPoliciesRequest.Builder}
* avoiding the need to create one manually via {@link ListAssociatedAccessPoliciesRequest#builder()}
*
*
* @param listAssociatedAccessPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest.Builder} to create a
* request.
* @return Result of the ListAssociatedAccessPolicies operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAssociatedAccessPolicies
* @see AWS API Documentation
*/
default ListAssociatedAccessPoliciesResponse listAssociatedAccessPolicies(
Consumer listAssociatedAccessPoliciesRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return listAssociatedAccessPolicies(ListAssociatedAccessPoliciesRequest.builder()
.applyMutation(listAssociatedAccessPoliciesRequest).build());
}
/**
*
* This is a variant of
* {@link #listAssociatedAccessPolicies(software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client.listAssociatedAccessPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client
* .listAssociatedAccessPoliciesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client.listAssociatedAccessPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAssociatedAccessPolicies(software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest)}
* operation.
*
*
* @param listAssociatedAccessPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAssociatedAccessPolicies
* @see AWS API Documentation
*/
default ListAssociatedAccessPoliciesIterable listAssociatedAccessPoliciesPaginator(
ListAssociatedAccessPoliciesRequest listAssociatedAccessPoliciesRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return new ListAssociatedAccessPoliciesIterable(this, listAssociatedAccessPoliciesRequest);
}
/**
*
* This is a variant of
* {@link #listAssociatedAccessPolicies(software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client.listAssociatedAccessPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client
* .listAssociatedAccessPoliciesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListAssociatedAccessPoliciesIterable responses = client.listAssociatedAccessPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAssociatedAccessPolicies(software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedAccessPoliciesRequest.Builder}
* avoiding the need to create one manually via {@link ListAssociatedAccessPoliciesRequest#builder()}
*
*
* @param listAssociatedAccessPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListAssociatedAccessPoliciesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListAssociatedAccessPolicies
* @see AWS API Documentation
*/
default ListAssociatedAccessPoliciesIterable listAssociatedAccessPoliciesPaginator(
Consumer listAssociatedAccessPoliciesRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return listAssociatedAccessPoliciesPaginator(ListAssociatedAccessPoliciesRequest.builder()
.applyMutation(listAssociatedAccessPoliciesRequest).build());
}
/**
*
* Lists the Amazon EKS clusters in your Amazon Web Services account in the specified Amazon Web Services Region.
*
*
* @param listClustersRequest
* @return Result of the ListClusters operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersResponse listClusters(ListClustersRequest listClustersRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Amazon EKS clusters in your Amazon Web Services account in the specified Amazon Web Services Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListClustersRequest.Builder} avoiding the need to
* create one manually via {@link ListClustersRequest#builder()}
*
*
* @param listClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListClustersRequest.Builder} to create a request.
* @return Result of the ListClusters operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersResponse listClusters(Consumer listClustersRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return listClusters(ListClustersRequest.builder().applyMutation(listClustersRequest).build());
}
/**
*
* Lists the Amazon EKS clusters in your Amazon Web Services account in the specified Amazon Web Services Region.
*
*
* @return Result of the ListClusters operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see #listClusters(ListClustersRequest)
* @see AWS API
* Documentation
*/
default ListClustersResponse listClusters() throws InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return listClusters(ListClustersRequest.builder().build());
}
/**
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see #listClustersPaginator(ListClustersRequest)
* @see AWS API
* Documentation
*/
default ListClustersIterable listClustersPaginator() throws InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return listClustersPaginator(ListClustersRequest.builder().build());
}
/**
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)} operation.
*
*
* @param listClustersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersIterable listClustersPaginator(ListClustersRequest listClustersRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return new ListClustersIterable(this, listClustersRequest);
}
/**
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListClustersIterable responses = client.listClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.eks.model.ListClustersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListClustersRequest.Builder} avoiding the need to
* create one manually via {@link ListClustersRequest#builder()}
*
*
* @param listClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListClustersRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersIterable listClustersPaginator(Consumer listClustersRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return listClustersPaginator(ListClustersRequest.builder().applyMutation(listClustersRequest).build());
}
/**
*
* Displays the full description of the subscription.
*
*
* @param listEksAnywhereSubscriptionsRequest
* @return Result of the ListEksAnywhereSubscriptions operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListEksAnywhereSubscriptions
* @see AWS API Documentation
*/
default ListEksAnywhereSubscriptionsResponse listEksAnywhereSubscriptions(
ListEksAnywhereSubscriptionsRequest listEksAnywhereSubscriptionsRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Displays the full description of the subscription.
*
*
*
* This is a convenience which creates an instance of the {@link ListEksAnywhereSubscriptionsRequest.Builder}
* avoiding the need to create one manually via {@link ListEksAnywhereSubscriptionsRequest#builder()}
*
*
* @param listEksAnywhereSubscriptionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest.Builder} to create a
* request.
* @return Result of the ListEksAnywhereSubscriptions operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListEksAnywhereSubscriptions
* @see AWS API Documentation
*/
default ListEksAnywhereSubscriptionsResponse listEksAnywhereSubscriptions(
Consumer listEksAnywhereSubscriptionsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return listEksAnywhereSubscriptions(ListEksAnywhereSubscriptionsRequest.builder()
.applyMutation(listEksAnywhereSubscriptionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listEksAnywhereSubscriptions(software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client.listEksAnywhereSubscriptionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client
* .listEksAnywhereSubscriptionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client.listEksAnywhereSubscriptionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEksAnywhereSubscriptions(software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest)}
* operation.
*
*
* @param listEksAnywhereSubscriptionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListEksAnywhereSubscriptions
* @see AWS API Documentation
*/
default ListEksAnywhereSubscriptionsIterable listEksAnywhereSubscriptionsPaginator(
ListEksAnywhereSubscriptionsRequest listEksAnywhereSubscriptionsRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, AwsServiceException, SdkClientException, EksException {
return new ListEksAnywhereSubscriptionsIterable(this, listEksAnywhereSubscriptionsRequest);
}
/**
*
* This is a variant of
* {@link #listEksAnywhereSubscriptions(software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client.listEksAnywhereSubscriptionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client
* .listEksAnywhereSubscriptionsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListEksAnywhereSubscriptionsIterable responses = client.listEksAnywhereSubscriptionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEksAnywhereSubscriptions(software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListEksAnywhereSubscriptionsRequest.Builder}
* avoiding the need to create one manually via {@link ListEksAnywhereSubscriptionsRequest#builder()}
*
*
* @param listEksAnywhereSubscriptionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListEksAnywhereSubscriptionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListEksAnywhereSubscriptions
* @see AWS API Documentation
*/
default ListEksAnywhereSubscriptionsIterable listEksAnywhereSubscriptionsPaginator(
Consumer listEksAnywhereSubscriptionsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException, AwsServiceException,
SdkClientException, EksException {
return listEksAnywhereSubscriptionsPaginator(ListEksAnywhereSubscriptionsRequest.builder()
.applyMutation(listEksAnywhereSubscriptionsRequest).build());
}
/**
*
* Lists the Fargate profiles associated with the specified cluster in your Amazon Web Services account in the
* specified Amazon Web Services Region.
*
*
* @param listFargateProfilesRequest
* @return Result of the ListFargateProfiles operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListFargateProfiles
* @see AWS API
* Documentation
*/
default ListFargateProfilesResponse listFargateProfiles(ListFargateProfilesRequest listFargateProfilesRequest)
throws InvalidParameterException, ResourceNotFoundException, ClientException, ServerException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Fargate profiles associated with the specified cluster in your Amazon Web Services account in the
* specified Amazon Web Services Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListFargateProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListFargateProfilesRequest#builder()}
*
*
* @param listFargateProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest.Builder} to create a request.
* @return Result of the ListFargateProfiles operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListFargateProfiles
* @see AWS API
* Documentation
*/
default ListFargateProfilesResponse listFargateProfiles(
Consumer listFargateProfilesRequest) throws InvalidParameterException,
ResourceNotFoundException, ClientException, ServerException, AwsServiceException, SdkClientException, EksException {
return listFargateProfiles(ListFargateProfilesRequest.builder().applyMutation(listFargateProfilesRequest).build());
}
/**
*
* This is a variant of
* {@link #listFargateProfiles(software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client.listFargateProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client
* .listFargateProfilesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListFargateProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client.listFargateProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFargateProfiles(software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest)} operation.
*
*
* @param listFargateProfilesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListFargateProfiles
* @see AWS API
* Documentation
*/
default ListFargateProfilesIterable listFargateProfilesPaginator(ListFargateProfilesRequest listFargateProfilesRequest)
throws InvalidParameterException, ResourceNotFoundException, ClientException, ServerException, AwsServiceException,
SdkClientException, EksException {
return new ListFargateProfilesIterable(this, listFargateProfilesRequest);
}
/**
*
* This is a variant of
* {@link #listFargateProfiles(software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client.listFargateProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client
* .listFargateProfilesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListFargateProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListFargateProfilesIterable responses = client.listFargateProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFargateProfiles(software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListFargateProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListFargateProfilesRequest#builder()}
*
*
* @param listFargateProfilesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListFargateProfilesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListFargateProfiles
* @see AWS API
* Documentation
*/
default ListFargateProfilesIterable listFargateProfilesPaginator(
Consumer listFargateProfilesRequest) throws InvalidParameterException,
ResourceNotFoundException, ClientException, ServerException, AwsServiceException, SdkClientException, EksException {
return listFargateProfilesPaginator(ListFargateProfilesRequest.builder().applyMutation(listFargateProfilesRequest)
.build());
}
/**
*
* Lists the identity provider configurations for your cluster.
*
*
* @param listIdentityProviderConfigsRequest
* @return Result of the ListIdentityProviderConfigs operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListIdentityProviderConfigs
* @see AWS API Documentation
*/
default ListIdentityProviderConfigsResponse listIdentityProviderConfigs(
ListIdentityProviderConfigsRequest listIdentityProviderConfigsRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the identity provider configurations for your cluster.
*
*
*
* This is a convenience which creates an instance of the {@link ListIdentityProviderConfigsRequest.Builder}
* avoiding the need to create one manually via {@link ListIdentityProviderConfigsRequest#builder()}
*
*
* @param listIdentityProviderConfigsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest.Builder} to create a
* request.
* @return Result of the ListIdentityProviderConfigs operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListIdentityProviderConfigs
* @see AWS API Documentation
*/
default ListIdentityProviderConfigsResponse listIdentityProviderConfigs(
Consumer listIdentityProviderConfigsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return listIdentityProviderConfigs(ListIdentityProviderConfigsRequest.builder()
.applyMutation(listIdentityProviderConfigsRequest).build());
}
/**
*
* This is a variant of
* {@link #listIdentityProviderConfigs(software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client.listIdentityProviderConfigsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client
* .listIdentityProviderConfigsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client.listIdentityProviderConfigsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIdentityProviderConfigs(software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest)}
* operation.
*
*
* @param listIdentityProviderConfigsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListIdentityProviderConfigs
* @see AWS API Documentation
*/
default ListIdentityProviderConfigsIterable listIdentityProviderConfigsPaginator(
ListIdentityProviderConfigsRequest listIdentityProviderConfigsRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
return new ListIdentityProviderConfigsIterable(this, listIdentityProviderConfigsRequest);
}
/**
*
* This is a variant of
* {@link #listIdentityProviderConfigs(software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client.listIdentityProviderConfigsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client
* .listIdentityProviderConfigsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListIdentityProviderConfigsIterable responses = client.listIdentityProviderConfigsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIdentityProviderConfigs(software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListIdentityProviderConfigsRequest.Builder}
* avoiding the need to create one manually via {@link ListIdentityProviderConfigsRequest#builder()}
*
*
* @param listIdentityProviderConfigsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListIdentityProviderConfigsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListIdentityProviderConfigs
* @see AWS API Documentation
*/
default ListIdentityProviderConfigsIterable listIdentityProviderConfigsPaginator(
Consumer listIdentityProviderConfigsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return listIdentityProviderConfigsPaginator(ListIdentityProviderConfigsRequest.builder()
.applyMutation(listIdentityProviderConfigsRequest).build());
}
/**
*
* Returns a list of all insights checked for against the specified cluster. You can filter which insights are
* returned by category, associated Kubernetes version, and status.
*
*
* @param listInsightsRequest
* @return Result of the ListInsights operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListInsights
* @see AWS API
* Documentation
*/
default ListInsightsResponse listInsights(ListInsightsRequest listInsightsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all insights checked for against the specified cluster. You can filter which insights are
* returned by category, associated Kubernetes version, and status.
*
*
*
* This is a convenience which creates an instance of the {@link ListInsightsRequest.Builder} avoiding the need to
* create one manually via {@link ListInsightsRequest#builder()}
*
*
* @param listInsightsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListInsightsRequest.Builder} to create a request.
* @return Result of the ListInsights operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListInsights
* @see AWS API
* Documentation
*/
default ListInsightsResponse listInsights(Consumer listInsightsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return listInsights(ListInsightsRequest.builder().applyMutation(listInsightsRequest).build());
}
/**
*
* This is a variant of {@link #listInsights(software.amazon.awssdk.services.eks.model.ListInsightsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListInsightsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInsights(software.amazon.awssdk.services.eks.model.ListInsightsRequest)} operation.
*
*
* @param listInsightsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListInsights
* @see AWS API
* Documentation
*/
default ListInsightsIterable listInsightsPaginator(ListInsightsRequest listInsightsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return new ListInsightsIterable(this, listInsightsRequest);
}
/**
*
* This is a variant of {@link #listInsights(software.amazon.awssdk.services.eks.model.ListInsightsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListInsightsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListInsightsIterable responses = client.listInsightsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInsights(software.amazon.awssdk.services.eks.model.ListInsightsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListInsightsRequest.Builder} avoiding the need to
* create one manually via {@link ListInsightsRequest#builder()}
*
*
* @param listInsightsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListInsightsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListInsights
* @see AWS API
* Documentation
*/
default ListInsightsIterable listInsightsPaginator(Consumer listInsightsRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return listInsightsPaginator(ListInsightsRequest.builder().applyMutation(listInsightsRequest).build());
}
/**
*
* Lists the managed node groups associated with the specified cluster in your Amazon Web Services account in the
* specified Amazon Web Services Region. Self-managed node groups aren't listed.
*
*
* @param listNodegroupsRequest
* @return Result of the ListNodegroups operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListNodegroups
* @see AWS API
* Documentation
*/
default ListNodegroupsResponse listNodegroups(ListNodegroupsRequest listNodegroupsRequest) throws InvalidParameterException,
ClientException, ServerException, ServiceUnavailableException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the managed node groups associated with the specified cluster in your Amazon Web Services account in the
* specified Amazon Web Services Region. Self-managed node groups aren't listed.
*
*
*
* This is a convenience which creates an instance of the {@link ListNodegroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListNodegroupsRequest#builder()}
*
*
* @param listNodegroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListNodegroupsRequest.Builder} to create a request.
* @return Result of the ListNodegroups operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListNodegroups
* @see AWS API
* Documentation
*/
default ListNodegroupsResponse listNodegroups(Consumer listNodegroupsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return listNodegroups(ListNodegroupsRequest.builder().applyMutation(listNodegroupsRequest).build());
}
/**
*
* This is a variant of {@link #listNodegroups(software.amazon.awssdk.services.eks.model.ListNodegroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListNodegroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listNodegroups(software.amazon.awssdk.services.eks.model.ListNodegroupsRequest)} operation.
*
*
* @param listNodegroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListNodegroups
* @see AWS API
* Documentation
*/
default ListNodegroupsIterable listNodegroupsPaginator(ListNodegroupsRequest listNodegroupsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return new ListNodegroupsIterable(this, listNodegroupsRequest);
}
/**
*
* This is a variant of {@link #listNodegroups(software.amazon.awssdk.services.eks.model.ListNodegroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListNodegroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListNodegroupsIterable responses = client.listNodegroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listNodegroups(software.amazon.awssdk.services.eks.model.ListNodegroupsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListNodegroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListNodegroupsRequest#builder()}
*
*
* @param listNodegroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListNodegroupsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListNodegroups
* @see AWS API
* Documentation
*/
default ListNodegroupsIterable listNodegroupsPaginator(Consumer listNodegroupsRequest)
throws InvalidParameterException, ClientException, ServerException, ServiceUnavailableException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return listNodegroupsPaginator(ListNodegroupsRequest.builder().applyMutation(listNodegroupsRequest).build());
}
/**
*
* List the EKS Pod Identity associations in a cluster. You can filter the list by the namespace that the
* association is in or the service account that the association uses.
*
*
* @param listPodIdentityAssociationsRequest
* @return Result of the ListPodIdentityAssociations operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListPodIdentityAssociations
* @see AWS API Documentation
*/
default ListPodIdentityAssociationsResponse listPodIdentityAssociations(
ListPodIdentityAssociationsRequest listPodIdentityAssociationsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* List the EKS Pod Identity associations in a cluster. You can filter the list by the namespace that the
* association is in or the service account that the association uses.
*
*
*
* This is a convenience which creates an instance of the {@link ListPodIdentityAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link ListPodIdentityAssociationsRequest#builder()}
*
*
* @param listPodIdentityAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest.Builder} to create a
* request.
* @return Result of the ListPodIdentityAssociations operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListPodIdentityAssociations
* @see AWS API Documentation
*/
default ListPodIdentityAssociationsResponse listPodIdentityAssociations(
Consumer listPodIdentityAssociationsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return listPodIdentityAssociations(ListPodIdentityAssociationsRequest.builder()
.applyMutation(listPodIdentityAssociationsRequest).build());
}
/**
*
* This is a variant of
* {@link #listPodIdentityAssociations(software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client.listPodIdentityAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client
* .listPodIdentityAssociationsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client.listPodIdentityAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPodIdentityAssociations(software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest)}
* operation.
*
*
* @param listPodIdentityAssociationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListPodIdentityAssociations
* @see AWS API Documentation
*/
default ListPodIdentityAssociationsIterable listPodIdentityAssociationsPaginator(
ListPodIdentityAssociationsRequest listPodIdentityAssociationsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return new ListPodIdentityAssociationsIterable(this, listPodIdentityAssociationsRequest);
}
/**
*
* This is a variant of
* {@link #listPodIdentityAssociations(software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client.listPodIdentityAssociationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client
* .listPodIdentityAssociationsPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListPodIdentityAssociationsIterable responses = client.listPodIdentityAssociationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPodIdentityAssociations(software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPodIdentityAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link ListPodIdentityAssociationsRequest#builder()}
*
*
* @param listPodIdentityAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListPodIdentityAssociationsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListPodIdentityAssociations
* @see AWS API Documentation
*/
default ListPodIdentityAssociationsIterable listPodIdentityAssociationsPaginator(
Consumer listPodIdentityAssociationsRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return listPodIdentityAssociationsPaginator(ListPodIdentityAssociationsRequest.builder()
.applyMutation(listPodIdentityAssociationsRequest).build());
}
/**
*
* List the tags for an Amazon EKS resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws BadRequestException, NotFoundException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an Amazon EKS resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListTagsForResourceRequest.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws BadRequestException,
NotFoundException, AwsServiceException, SdkClientException, EksException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Lists the updates associated with an Amazon EKS resource in your Amazon Web Services account, in the specified
* Amazon Web Services Region.
*
*
* @param listUpdatesRequest
* @return Result of the ListUpdates operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListUpdates
* @see AWS API
* Documentation
*/
default ListUpdatesResponse listUpdates(ListUpdatesRequest listUpdatesRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the updates associated with an Amazon EKS resource in your Amazon Web Services account, in the specified
* Amazon Web Services Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListUpdatesRequest.Builder} avoiding the need to
* create one manually via {@link ListUpdatesRequest#builder()}
*
*
* @param listUpdatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListUpdatesRequest.Builder} to create a request.
* @return Result of the ListUpdates operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListUpdates
* @see AWS API
* Documentation
*/
default ListUpdatesResponse listUpdates(Consumer listUpdatesRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
return listUpdates(ListUpdatesRequest.builder().applyMutation(listUpdatesRequest).build());
}
/**
*
* This is a variant of {@link #listUpdates(software.amazon.awssdk.services.eks.model.ListUpdatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListUpdatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUpdates(software.amazon.awssdk.services.eks.model.ListUpdatesRequest)} operation.
*
*
* @param listUpdatesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListUpdates
* @see AWS API
* Documentation
*/
default ListUpdatesIterable listUpdatesPaginator(ListUpdatesRequest listUpdatesRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, AwsServiceException, SdkClientException, EksException {
return new ListUpdatesIterable(this, listUpdatesRequest);
}
/**
*
* This is a variant of {@link #listUpdates(software.amazon.awssdk.services.eks.model.ListUpdatesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* for (software.amazon.awssdk.services.eks.model.ListUpdatesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.eks.paginators.ListUpdatesIterable responses = client.listUpdatesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUpdates(software.amazon.awssdk.services.eks.model.ListUpdatesRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListUpdatesRequest.Builder} avoiding the need to
* create one manually via {@link ListUpdatesRequest#builder()}
*
*
* @param listUpdatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.ListUpdatesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.ListUpdates
* @see AWS API
* Documentation
*/
default ListUpdatesIterable listUpdatesPaginator(Consumer listUpdatesRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException, AwsServiceException,
SdkClientException, EksException {
return listUpdatesPaginator(ListUpdatesRequest.builder().applyMutation(listUpdatesRequest).build());
}
/**
*
* Connects a Kubernetes cluster to the Amazon EKS control plane.
*
*
* Any Kubernetes cluster can be connected to the Amazon EKS control plane to view current information about the
* cluster and its nodes.
*
*
* Cluster connection requires two steps. First, send a RegisterClusterRequest
to add it to
* the Amazon EKS control plane.
*
*
* Second, a Manifest containing the activationID
and activationCode
must be applied to the
* Kubernetes cluster through it's native provider to provide visibility.
*
*
* After the manifest is updated and applied, the connected cluster is visible to the Amazon EKS control plane. If
* the manifest isn't applied within three days, the connected cluster will no longer be visible and must be
* deregistered using DeregisterCluster
.
*
*
* @param registerClusterRequest
* @return Result of the RegisterCluster operation returned by the service.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The IAM
* principal making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access management in the IAM
* User Guide.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourcePropagationDelayException
* Required resources (such as service-linked roles) were created and are still propagating. Retry later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.RegisterCluster
* @see AWS API
* Documentation
*/
default RegisterClusterResponse registerCluster(RegisterClusterRequest registerClusterRequest)
throws ResourceLimitExceededException, InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AccessDeniedException, ResourceInUseException, ResourcePropagationDelayException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Connects a Kubernetes cluster to the Amazon EKS control plane.
*
*
* Any Kubernetes cluster can be connected to the Amazon EKS control plane to view current information about the
* cluster and its nodes.
*
*
* Cluster connection requires two steps. First, send a RegisterClusterRequest
to add it to
* the Amazon EKS control plane.
*
*
* Second, a Manifest containing the activationID
and activationCode
must be applied to the
* Kubernetes cluster through it's native provider to provide visibility.
*
*
* After the manifest is updated and applied, the connected cluster is visible to the Amazon EKS control plane. If
* the manifest isn't applied within three days, the connected cluster will no longer be visible and must be
* deregistered using DeregisterCluster
.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterClusterRequest.Builder} avoiding the need
* to create one manually via {@link RegisterClusterRequest#builder()}
*
*
* @param registerClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.RegisterClusterRequest.Builder} to create a request.
* @return Result of the RegisterCluster operation returned by the service.
* @throws ResourceLimitExceededException
* You have encountered a service limit on the specified resource.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ServiceUnavailableException
* The service is unavailable. Back off and retry the operation.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The IAM
* principal making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access management in the IAM
* User Guide.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourcePropagationDelayException
* Required resources (such as service-linked roles) were created and are still propagating. Retry later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.RegisterCluster
* @see AWS API
* Documentation
*/
default RegisterClusterResponse registerCluster(Consumer registerClusterRequest)
throws ResourceLimitExceededException, InvalidParameterException, ClientException, ServerException,
ServiceUnavailableException, AccessDeniedException, ResourceInUseException, ResourcePropagationDelayException,
AwsServiceException, SdkClientException, EksException {
return registerCluster(RegisterClusterRequest.builder().applyMutation(registerClusterRequest).build());
}
/**
*
* Associates the specified tags to an Amazon EKS resource with the specified resourceArn
. If existing
* tags on a resource are not specified in the request parameters, they aren't changed. When a resource is deleted,
* the tags associated with that resource are also deleted. Tags that you create for Amazon EKS resources don't
* propagate to any other resources associated with the cluster. For example, if you tag a cluster with this
* operation, that tag doesn't automatically propagate to the subnets and nodes associated with the cluster.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws BadRequestException, NotFoundException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified tags to an Amazon EKS resource with the specified resourceArn
. If existing
* tags on a resource are not specified in the request parameters, they aren't changed. When a resource is deleted,
* the tags associated with that resource are also deleted. Tags that you create for Amazon EKS resources don't
* propagate to any other resources associated with the cluster. For example, if you tag a cluster with this
* operation, that tag doesn't automatically propagate to the subnets and nodes associated with the cluster.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest) throws BadRequestException,
NotFoundException, AwsServiceException, SdkClientException, EksException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes specified tags from an Amazon EKS resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws BadRequestException,
NotFoundException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from an Amazon EKS resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* This exception is thrown if the request contains a semantic error. The precise meaning will depend on the
* API, and will be documented in the error message.
* @throws NotFoundException
* A service resource associated with the request could not be found. Clients should not retry such
* requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws BadRequestException, NotFoundException, AwsServiceException, SdkClientException, EksException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an access entry.
*
*
* @param updateAccessEntryRequest
* @return Result of the UpdateAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateAccessEntry
* @see AWS API
* Documentation
*/
default UpdateAccessEntryResponse updateAccessEntry(UpdateAccessEntryRequest updateAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an access entry.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAccessEntryRequest.Builder} avoiding the need
* to create one manually via {@link UpdateAccessEntryRequest#builder()}
*
*
* @param updateAccessEntryRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateAccessEntryRequest.Builder} to create a request.
* @return Result of the UpdateAccessEntry operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateAccessEntry
* @see AWS API
* Documentation
*/
default UpdateAccessEntryResponse updateAccessEntry(Consumer updateAccessEntryRequest)
throws ServerException, ResourceNotFoundException, InvalidRequestException, InvalidParameterException,
AwsServiceException, SdkClientException, EksException {
return updateAccessEntry(UpdateAccessEntryRequest.builder().applyMutation(updateAccessEntryRequest).build());
}
/**
*
* Updates an Amazon EKS add-on.
*
*
* @param updateAddonRequest
* @return Result of the UpdateAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateAddon
* @see AWS API
* Documentation
*/
default UpdateAddonResponse updateAddon(UpdateAddonRequest updateAddonRequest) throws InvalidParameterException,
InvalidRequestException, ResourceNotFoundException, ResourceInUseException, ClientException, ServerException,
AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon EKS add-on.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAddonRequest.Builder} avoiding the need to
* create one manually via {@link UpdateAddonRequest#builder()}
*
*
* @param updateAddonRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateAddonRequest.Builder} to create a request.
* @return Result of the UpdateAddon operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateAddon
* @see AWS API
* Documentation
*/
default UpdateAddonResponse updateAddon(Consumer updateAddonRequest)
throws InvalidParameterException, InvalidRequestException, ResourceNotFoundException, ResourceInUseException,
ClientException, ServerException, AwsServiceException, SdkClientException, EksException {
return updateAddon(UpdateAddonRequest.builder().applyMutation(updateAddonRequest).build());
}
/**
*
* Updates an Amazon EKS cluster configuration. Your cluster continues to function during the update. The response
* output includes an update ID that you can use to track the status of your cluster update with
* DescribeUpdate
"/>.
*
*
* You can use this API operation to enable or disable exporting the Kubernetes control plane logs for your cluster
* to CloudWatch Logs. By default, cluster control plane logs aren't exported to CloudWatch Logs. For more
* information, see Amazon EKS
* Cluster control plane logs in the Amazon EKS User Guide .
*
*
*
* CloudWatch Logs ingestion, archive storage, and data scanning rates apply to exported control plane logs. For
* more information, see CloudWatch Pricing.
*
*
*
* You can also use this API operation to enable or disable public and private access to your cluster's Kubernetes
* API server endpoint. By default, public access is enabled, and private access is disabled. For more information,
* see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*
*
* You can also use this API operation to choose different subnets and security groups for the cluster. You must
* specify at least two subnets that are in different Availability Zones. You can't change which VPC the subnets are
* from, the subnets must be in the same VPC as the subnets that the cluster was created with. For more information
* about the VPC requirements, see https
* ://docs.aws.amazon.com/eks/latest/userguide/network_reqs.html in the Amazon EKS User Guide .
*
*
* You can also use this API operation to enable or disable ARC zonal shift. If zonal shift is enabled, Amazon Web
* Services configures zonal autoshift for the cluster.
*
*
* Cluster updates are asynchronous, and they should finish within a few minutes. During an update, the cluster
* status moves to UPDATING
(this status transition is eventually consistent). When the update is
* complete (either Failed
or Successful
), the cluster status moves to Active
* .
*
*
* @param updateClusterConfigRequest
* @return Result of the UpdateClusterConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateClusterConfig
* @see AWS API
* Documentation
*/
default UpdateClusterConfigResponse updateClusterConfig(UpdateClusterConfigRequest updateClusterConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon EKS cluster configuration. Your cluster continues to function during the update. The response
* output includes an update ID that you can use to track the status of your cluster update with
* DescribeUpdate
"/>.
*
*
* You can use this API operation to enable or disable exporting the Kubernetes control plane logs for your cluster
* to CloudWatch Logs. By default, cluster control plane logs aren't exported to CloudWatch Logs. For more
* information, see Amazon EKS
* Cluster control plane logs in the Amazon EKS User Guide .
*
*
*
* CloudWatch Logs ingestion, archive storage, and data scanning rates apply to exported control plane logs. For
* more information, see CloudWatch Pricing.
*
*
*
* You can also use this API operation to enable or disable public and private access to your cluster's Kubernetes
* API server endpoint. By default, public access is enabled, and private access is disabled. For more information,
* see Amazon EKS cluster endpoint
* access control in the Amazon EKS User Guide .
*
*
* You can also use this API operation to choose different subnets and security groups for the cluster. You must
* specify at least two subnets that are in different Availability Zones. You can't change which VPC the subnets are
* from, the subnets must be in the same VPC as the subnets that the cluster was created with. For more information
* about the VPC requirements, see https
* ://docs.aws.amazon.com/eks/latest/userguide/network_reqs.html in the Amazon EKS User Guide .
*
*
* You can also use this API operation to enable or disable ARC zonal shift. If zonal shift is enabled, Amazon Web
* Services configures zonal autoshift for the cluster.
*
*
* Cluster updates are asynchronous, and they should finish within a few minutes. During an update, the cluster
* status moves to UPDATING
(this status transition is eventually consistent). When the update is
* complete (either Failed
or Successful
), the cluster status moves to Active
* .
*
*
*
* This is a convenience which creates an instance of the {@link UpdateClusterConfigRequest.Builder} avoiding the
* need to create one manually via {@link UpdateClusterConfigRequest#builder()}
*
*
* @param updateClusterConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateClusterConfigRequest.Builder} to create a request.
* @return Result of the UpdateClusterConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateClusterConfig
* @see AWS API
* Documentation
*/
default UpdateClusterConfigResponse updateClusterConfig(
Consumer updateClusterConfigRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException,
AwsServiceException, SdkClientException, EksException {
return updateClusterConfig(UpdateClusterConfigRequest.builder().applyMutation(updateClusterConfigRequest).build());
}
/**
*
* Updates an Amazon EKS cluster to the specified Kubernetes version. Your cluster continues to function during the
* update. The response output includes an update ID that you can use to track the status of your cluster update
* with the DescribeUpdate API operation.
*
*
* Cluster updates are asynchronous, and they should finish within a few minutes. During an update, the cluster
* status moves to UPDATING
(this status transition is eventually consistent). When the update is
* complete (either Failed
or Successful
), the cluster status moves to Active
* .
*
*
* If your cluster has managed node groups attached to it, all of your node groups’ Kubernetes versions must match
* the cluster’s Kubernetes version in order to update the cluster to a new Kubernetes version.
*
*
* @param updateClusterVersionRequest
* @return Result of the UpdateClusterVersion operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateClusterVersion
* @see AWS API
* Documentation
*/
default UpdateClusterVersionResponse updateClusterVersion(UpdateClusterVersionRequest updateClusterVersionRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon EKS cluster to the specified Kubernetes version. Your cluster continues to function during the
* update. The response output includes an update ID that you can use to track the status of your cluster update
* with the DescribeUpdate API operation.
*
*
* Cluster updates are asynchronous, and they should finish within a few minutes. During an update, the cluster
* status moves to UPDATING
(this status transition is eventually consistent). When the update is
* complete (either Failed
or Successful
), the cluster status moves to Active
* .
*
*
* If your cluster has managed node groups attached to it, all of your node groups’ Kubernetes versions must match
* the cluster’s Kubernetes version in order to update the cluster to a new Kubernetes version.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateClusterVersionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateClusterVersionRequest#builder()}
*
*
* @param updateClusterVersionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateClusterVersionRequest.Builder} to create a request.
* @return Result of the UpdateClusterVersion operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateClusterVersion
* @see AWS API
* Documentation
*/
default UpdateClusterVersionResponse updateClusterVersion(
Consumer updateClusterVersionRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException,
AwsServiceException, SdkClientException, EksException {
return updateClusterVersion(UpdateClusterVersionRequest.builder().applyMutation(updateClusterVersionRequest).build());
}
/**
*
* Update an EKS Anywhere Subscription. Only auto renewal and tags can be updated after subscription creation.
*
*
* @param updateEksAnywhereSubscriptionRequest
* @return Result of the UpdateEksAnywhereSubscription operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateEksAnywhereSubscription
* @see AWS API Documentation
*/
default UpdateEksAnywhereSubscriptionResponse updateEksAnywhereSubscription(
UpdateEksAnywhereSubscriptionRequest updateEksAnywhereSubscriptionRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceNotFoundException, InvalidRequestException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Update an EKS Anywhere Subscription. Only auto renewal and tags can be updated after subscription creation.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEksAnywhereSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link UpdateEksAnywhereSubscriptionRequest#builder()}
*
*
* @param updateEksAnywhereSubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateEksAnywhereSubscriptionRequest.Builder} to create a
* request.
* @return Result of the UpdateEksAnywhereSubscription operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateEksAnywhereSubscription
* @see AWS API Documentation
*/
default UpdateEksAnywhereSubscriptionResponse updateEksAnywhereSubscription(
Consumer updateEksAnywhereSubscriptionRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceNotFoundException,
InvalidRequestException, AwsServiceException, SdkClientException, EksException {
return updateEksAnywhereSubscription(UpdateEksAnywhereSubscriptionRequest.builder()
.applyMutation(updateEksAnywhereSubscriptionRequest).build());
}
/**
*
* Updates an Amazon EKS managed node group configuration. Your node group continues to function during the update.
* The response output includes an update ID that you can use to track the status of your node group update with the
* DescribeUpdate API operation. Currently you can update the Kubernetes labels for a node group or the
* scaling configuration.
*
*
* @param updateNodegroupConfigRequest
* @return Result of the UpdateNodegroupConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateNodegroupConfig
* @see AWS API
* Documentation
*/
default UpdateNodegroupConfigResponse updateNodegroupConfig(UpdateNodegroupConfigRequest updateNodegroupConfigRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon EKS managed node group configuration. Your node group continues to function during the update.
* The response output includes an update ID that you can use to track the status of your node group update with the
* DescribeUpdate API operation. Currently you can update the Kubernetes labels for a node group or the
* scaling configuration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNodegroupConfigRequest.Builder} avoiding the
* need to create one manually via {@link UpdateNodegroupConfigRequest#builder()}
*
*
* @param updateNodegroupConfigRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateNodegroupConfigRequest.Builder} to create a
* request.
* @return Result of the UpdateNodegroupConfig operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateNodegroupConfig
* @see AWS API
* Documentation
*/
default UpdateNodegroupConfigResponse updateNodegroupConfig(
Consumer updateNodegroupConfigRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException,
AwsServiceException, SdkClientException, EksException {
return updateNodegroupConfig(UpdateNodegroupConfigRequest.builder().applyMutation(updateNodegroupConfigRequest).build());
}
/**
*
* Updates the Kubernetes version or AMI version of an Amazon EKS managed node group.
*
*
* You can update a node group using a launch template only if the node group was originally deployed with a launch
* template. If you need to update a custom AMI in a node group that was deployed with a launch template, then
* update your custom AMI, specify the new ID in a new version of the launch template, and then update the node
* group to the new version of the launch template.
*
*
* If you update without a launch template, then you can update to the latest available AMI version of a node
* group's current Kubernetes version by not specifying a Kubernetes version in the request. You can update to the
* latest AMI version of your cluster's current Kubernetes version by specifying your cluster's Kubernetes version
* in the request. For information about Linux versions, see Amazon EKS optimized Amazon
* Linux AMI versions in the Amazon EKS User Guide. For information about Windows versions, see Amazon EKS optimized
* Windows AMI versions in the Amazon EKS User Guide.
*
*
* You cannot roll back a node group to an earlier Kubernetes version or AMI version.
*
*
* When a node in a managed node group is terminated due to a scaling action or update, every Pod
on
* that node is drained first. Amazon EKS attempts to drain the nodes gracefully and will fail if it is unable to do
* so. You can force
the update if Amazon EKS is unable to drain the nodes as a result of a
* Pod
disruption budget issue.
*
*
* @param updateNodegroupVersionRequest
* @return Result of the UpdateNodegroupVersion operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateNodegroupVersion
* @see AWS
* API Documentation
*/
default UpdateNodegroupVersionResponse updateNodegroupVersion(UpdateNodegroupVersionRequest updateNodegroupVersionRequest)
throws InvalidParameterException, ClientException, ServerException, ResourceInUseException,
ResourceNotFoundException, InvalidRequestException, AwsServiceException, SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the Kubernetes version or AMI version of an Amazon EKS managed node group.
*
*
* You can update a node group using a launch template only if the node group was originally deployed with a launch
* template. If you need to update a custom AMI in a node group that was deployed with a launch template, then
* update your custom AMI, specify the new ID in a new version of the launch template, and then update the node
* group to the new version of the launch template.
*
*
* If you update without a launch template, then you can update to the latest available AMI version of a node
* group's current Kubernetes version by not specifying a Kubernetes version in the request. You can update to the
* latest AMI version of your cluster's current Kubernetes version by specifying your cluster's Kubernetes version
* in the request. For information about Linux versions, see Amazon EKS optimized Amazon
* Linux AMI versions in the Amazon EKS User Guide. For information about Windows versions, see Amazon EKS optimized
* Windows AMI versions in the Amazon EKS User Guide.
*
*
* You cannot roll back a node group to an earlier Kubernetes version or AMI version.
*
*
* When a node in a managed node group is terminated due to a scaling action or update, every Pod
on
* that node is drained first. Amazon EKS attempts to drain the nodes gracefully and will fail if it is unable to do
* so. You can force
the update if Amazon EKS is unable to drain the nodes as a result of a
* Pod
disruption budget issue.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNodegroupVersionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateNodegroupVersionRequest#builder()}
*
*
* @param updateNodegroupVersionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdateNodegroupVersionRequest.Builder} to create a
* request.
* @return Result of the UpdateNodegroupVersion operation returned by the service.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws ClientException
* These errors are usually caused by a client action. Actions can include using an action or resource on
* behalf of an IAM
* principal that doesn't have permissions to use the action or resource or specifying an identifier
* that is not valid.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdateNodegroupVersion
* @see AWS
* API Documentation
*/
default UpdateNodegroupVersionResponse updateNodegroupVersion(
Consumer updateNodegroupVersionRequest) throws InvalidParameterException,
ClientException, ServerException, ResourceInUseException, ResourceNotFoundException, InvalidRequestException,
AwsServiceException, SdkClientException, EksException {
return updateNodegroupVersion(UpdateNodegroupVersionRequest.builder().applyMutation(updateNodegroupVersionRequest)
.build());
}
/**
*
* Updates a EKS Pod Identity association. Only the IAM role can be changed; an association can't be moved between
* clusters, namespaces, or service accounts. If you need to edit the namespace or service account, you need to
* delete the association and then create a new association with your desired settings.
*
*
* @param updatePodIdentityAssociationRequest
* @return Result of the UpdatePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdatePodIdentityAssociation
* @see AWS API Documentation
*/
default UpdatePodIdentityAssociationResponse updatePodIdentityAssociation(
UpdatePodIdentityAssociationRequest updatePodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a EKS Pod Identity association. Only the IAM role can be changed; an association can't be moved between
* clusters, namespaces, or service accounts. If you need to edit the namespace or service account, you need to
* delete the association and then create a new association with your desired settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePodIdentityAssociationRequest.Builder}
* avoiding the need to create one manually via {@link UpdatePodIdentityAssociationRequest#builder()}
*
*
* @param updatePodIdentityAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.eks.model.UpdatePodIdentityAssociationRequest.Builder} to create a
* request.
* @return Result of the UpdatePodIdentityAssociation operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server-side issue.
* @throws ResourceNotFoundException
* The specified resource could not be found. You can view your available clusters with
* ListClusters
. You can view your available managed node groups with
* ListNodegroups
. Amazon EKS clusters and node groups are Amazon Web Services Region specific.
* @throws InvalidRequestException
* The request is invalid given the state of the cluster. Check the state of the cluster and the associated
* operations.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available parameters for the API request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EksClient.UpdatePodIdentityAssociation
* @see AWS API Documentation
*/
default UpdatePodIdentityAssociationResponse updatePodIdentityAssociation(
Consumer updatePodIdentityAssociationRequest) throws ServerException,
ResourceNotFoundException, InvalidRequestException, InvalidParameterException, AwsServiceException,
SdkClientException, EksException {
return updatePodIdentityAssociation(UpdatePodIdentityAssociationRequest.builder()
.applyMutation(updatePodIdentityAssociationRequest).build());
}
/**
* Create an instance of {@link EksWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link EksWaiter}
*/
default EksWaiter waiter() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link EksClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EksClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EksClient}.
*/
static EksClientBuilder builder() {
return new DefaultEksClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default EksServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}