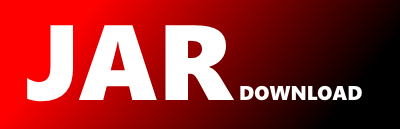
software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import javax.annotation.Generated;
import software.amazon.awssdk.AmazonWebServiceRequest;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a DescribeSnapshotsMessage
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class DescribeSnapshotsRequest extends AmazonWebServiceRequest implements
ToCopyableBuilder {
private final String replicationGroupId;
private final String cacheClusterId;
private final String snapshotName;
private final String snapshotSource;
private final String marker;
private final Integer maxRecords;
private final Boolean showNodeGroupConfig;
private DescribeSnapshotsRequest(BuilderImpl builder) {
this.replicationGroupId = builder.replicationGroupId;
this.cacheClusterId = builder.cacheClusterId;
this.snapshotName = builder.snapshotName;
this.snapshotSource = builder.snapshotSource;
this.marker = builder.marker;
this.maxRecords = builder.maxRecords;
this.showNodeGroupConfig = builder.showNodeGroupConfig;
}
/**
*
* A user-supplied replication group identifier. If this parameter is specified, only snapshots associated with that
* specific replication group are described.
*
*
* @return A user-supplied replication group identifier. If this parameter is specified, only snapshots associated
* with that specific replication group are described.
*/
public String replicationGroupId() {
return replicationGroupId;
}
/**
*
* A user-supplied cluster identifier. If this parameter is specified, only snapshots associated with that specific
* cache cluster are described.
*
*
* @return A user-supplied cluster identifier. If this parameter is specified, only snapshots associated with that
* specific cache cluster are described.
*/
public String cacheClusterId() {
return cacheClusterId;
}
/**
*
* A user-supplied name of the snapshot. If this parameter is specified, only this snapshot are described.
*
*
* @return A user-supplied name of the snapshot. If this parameter is specified, only this snapshot are described.
*/
public String snapshotName() {
return snapshotName;
}
/**
*
* If set to system
, the output shows snapshots that were automatically created by ElastiCache. If set
* to user
the output shows snapshots that were manually created. If omitted, the output shows both
* automatically and manually created snapshots.
*
*
* @return If set to system
, the output shows snapshots that were automatically created by ElastiCache.
* If set to user
the output shows snapshots that were manually created. If omitted, the output
* shows both automatically and manually created snapshots.
*/
public String snapshotSource() {
return snapshotSource;
}
/**
*
* An optional marker returned from a prior request. Use this marker for pagination of results from this operation.
* If this parameter is specified, the response includes only records beyond the marker, up to the value specified
* by MaxRecords
.
*
*
* @return An optional marker returned from a prior request. Use this marker for pagination of results from this
* operation. If this parameter is specified, the response includes only records beyond the marker, up to
* the value specified by MaxRecords
.
*/
public String marker() {
return marker;
}
/**
*
* The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can be
* retrieved.
*
*
* Default: 50
*
*
* Constraints: minimum 20; maximum 50.
*
*
* @return The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can be
* retrieved.
*
* Default: 50
*
*
* Constraints: minimum 20; maximum 50.
*/
public Integer maxRecords() {
return maxRecords;
}
/**
*
* A Boolean value which if true, the node group (shard) configuration is included in the snapshot description.
*
*
* @return A Boolean value which if true, the node group (shard) configuration is included in the snapshot
* description.
*/
public Boolean showNodeGroupConfig() {
return showNodeGroupConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((replicationGroupId() == null) ? 0 : replicationGroupId().hashCode());
hashCode = 31 * hashCode + ((cacheClusterId() == null) ? 0 : cacheClusterId().hashCode());
hashCode = 31 * hashCode + ((snapshotName() == null) ? 0 : snapshotName().hashCode());
hashCode = 31 * hashCode + ((snapshotSource() == null) ? 0 : snapshotSource().hashCode());
hashCode = 31 * hashCode + ((marker() == null) ? 0 : marker().hashCode());
hashCode = 31 * hashCode + ((maxRecords() == null) ? 0 : maxRecords().hashCode());
hashCode = 31 * hashCode + ((showNodeGroupConfig() == null) ? 0 : showNodeGroupConfig().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeSnapshotsRequest)) {
return false;
}
DescribeSnapshotsRequest other = (DescribeSnapshotsRequest) obj;
if (other.replicationGroupId() == null ^ this.replicationGroupId() == null) {
return false;
}
if (other.replicationGroupId() != null && !other.replicationGroupId().equals(this.replicationGroupId())) {
return false;
}
if (other.cacheClusterId() == null ^ this.cacheClusterId() == null) {
return false;
}
if (other.cacheClusterId() != null && !other.cacheClusterId().equals(this.cacheClusterId())) {
return false;
}
if (other.snapshotName() == null ^ this.snapshotName() == null) {
return false;
}
if (other.snapshotName() != null && !other.snapshotName().equals(this.snapshotName())) {
return false;
}
if (other.snapshotSource() == null ^ this.snapshotSource() == null) {
return false;
}
if (other.snapshotSource() != null && !other.snapshotSource().equals(this.snapshotSource())) {
return false;
}
if (other.marker() == null ^ this.marker() == null) {
return false;
}
if (other.marker() != null && !other.marker().equals(this.marker())) {
return false;
}
if (other.maxRecords() == null ^ this.maxRecords() == null) {
return false;
}
if (other.maxRecords() != null && !other.maxRecords().equals(this.maxRecords())) {
return false;
}
if (other.showNodeGroupConfig() == null ^ this.showNodeGroupConfig() == null) {
return false;
}
if (other.showNodeGroupConfig() != null && !other.showNodeGroupConfig().equals(this.showNodeGroupConfig())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (replicationGroupId() != null) {
sb.append("ReplicationGroupId: ").append(replicationGroupId()).append(",");
}
if (cacheClusterId() != null) {
sb.append("CacheClusterId: ").append(cacheClusterId()).append(",");
}
if (snapshotName() != null) {
sb.append("SnapshotName: ").append(snapshotName()).append(",");
}
if (snapshotSource() != null) {
sb.append("SnapshotSource: ").append(snapshotSource()).append(",");
}
if (marker() != null) {
sb.append("Marker: ").append(marker()).append(",");
}
if (maxRecords() != null) {
sb.append("MaxRecords: ").append(maxRecords()).append(",");
}
if (showNodeGroupConfig() != null) {
sb.append("ShowNodeGroupConfig: ").append(showNodeGroupConfig()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
*
* A user-supplied replication group identifier. If this parameter is specified, only snapshots associated with
* that specific replication group are described.
*
*
* @param replicationGroupId
* A user-supplied replication group identifier. If this parameter is specified, only snapshots
* associated with that specific replication group are described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationGroupId(String replicationGroupId);
/**
*
* A user-supplied cluster identifier. If this parameter is specified, only snapshots associated with that
* specific cache cluster are described.
*
*
* @param cacheClusterId
* A user-supplied cluster identifier. If this parameter is specified, only snapshots associated with
* that specific cache cluster are described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheClusterId(String cacheClusterId);
/**
*
* A user-supplied name of the snapshot. If this parameter is specified, only this snapshot are described.
*
*
* @param snapshotName
* A user-supplied name of the snapshot. If this parameter is specified, only this snapshot are
* described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotName(String snapshotName);
/**
*
* If set to system
, the output shows snapshots that were automatically created by ElastiCache. If
* set to user
the output shows snapshots that were manually created. If omitted, the output shows
* both automatically and manually created snapshots.
*
*
* @param snapshotSource
* If set to system
, the output shows snapshots that were automatically created by
* ElastiCache. If set to user
the output shows snapshots that were manually created. If
* omitted, the output shows both automatically and manually created snapshots.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotSource(String snapshotSource);
/**
*
* An optional marker returned from a prior request. Use this marker for pagination of results from this
* operation. If this parameter is specified, the response includes only records beyond the marker, up to the
* value specified by MaxRecords
.
*
*
* @param marker
* An optional marker returned from a prior request. Use this marker for pagination of results from this
* operation. If this parameter is specified, the response includes only records beyond the marker, up to
* the value specified by MaxRecords
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder marker(String marker);
/**
*
* The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can be
* retrieved.
*
*
* Default: 50
*
*
* Constraints: minimum 20; maximum 50.
*
*
* @param maxRecords
* The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can
* be retrieved.
*
* Default: 50
*
*
* Constraints: minimum 20; maximum 50.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxRecords(Integer maxRecords);
/**
*
* A Boolean value which if true, the node group (shard) configuration is included in the snapshot description.
*
*
* @param showNodeGroupConfig
* A Boolean value which if true, the node group (shard) configuration is included in the snapshot
* description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder showNodeGroupConfig(Boolean showNodeGroupConfig);
}
private static final class BuilderImpl implements Builder {
private String replicationGroupId;
private String cacheClusterId;
private String snapshotName;
private String snapshotSource;
private String marker;
private Integer maxRecords;
private Boolean showNodeGroupConfig;
private BuilderImpl() {
}
private BuilderImpl(DescribeSnapshotsRequest model) {
setReplicationGroupId(model.replicationGroupId);
setCacheClusterId(model.cacheClusterId);
setSnapshotName(model.snapshotName);
setSnapshotSource(model.snapshotSource);
setMarker(model.marker);
setMaxRecords(model.maxRecords);
setShowNodeGroupConfig(model.showNodeGroupConfig);
}
public final String getReplicationGroupId() {
return replicationGroupId;
}
@Override
public final Builder replicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
return this;
}
public final void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
public final String getCacheClusterId() {
return cacheClusterId;
}
@Override
public final Builder cacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
return this;
}
public final void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
public final String getSnapshotName() {
return snapshotName;
}
@Override
public final Builder snapshotName(String snapshotName) {
this.snapshotName = snapshotName;
return this;
}
public final void setSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
}
public final String getSnapshotSource() {
return snapshotSource;
}
@Override
public final Builder snapshotSource(String snapshotSource) {
this.snapshotSource = snapshotSource;
return this;
}
public final void setSnapshotSource(String snapshotSource) {
this.snapshotSource = snapshotSource;
}
public final String getMarker() {
return marker;
}
@Override
public final Builder marker(String marker) {
this.marker = marker;
return this;
}
public final void setMarker(String marker) {
this.marker = marker;
}
public final Integer getMaxRecords() {
return maxRecords;
}
@Override
public final Builder maxRecords(Integer maxRecords) {
this.maxRecords = maxRecords;
return this;
}
public final void setMaxRecords(Integer maxRecords) {
this.maxRecords = maxRecords;
}
public final Boolean getShowNodeGroupConfig() {
return showNodeGroupConfig;
}
@Override
public final Builder showNodeGroupConfig(Boolean showNodeGroupConfig) {
this.showNodeGroupConfig = showNodeGroupConfig;
return this;
}
public final void setShowNodeGroupConfig(Boolean showNodeGroupConfig) {
this.showNodeGroupConfig = showNodeGroupConfig;
}
@Override
public DescribeSnapshotsRequest build() {
return new DescribeSnapshotsRequest(this);
}
}
}