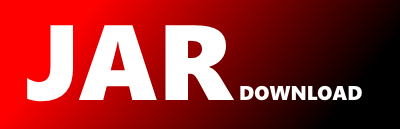
software.amazon.awssdk.services.elasticache.model.ReservedCacheNode Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the output of a PurchaseReservedCacheNodesOffering
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class ReservedCacheNode implements ToCopyableBuilder {
private final String reservedCacheNodeId;
private final String reservedCacheNodesOfferingId;
private final String cacheNodeType;
private final Instant startTime;
private final Integer duration;
private final Double fixedPrice;
private final Double usagePrice;
private final Integer cacheNodeCount;
private final String productDescription;
private final String offeringType;
private final String state;
private final List recurringCharges;
private ReservedCacheNode(BuilderImpl builder) {
this.reservedCacheNodeId = builder.reservedCacheNodeId;
this.reservedCacheNodesOfferingId = builder.reservedCacheNodesOfferingId;
this.cacheNodeType = builder.cacheNodeType;
this.startTime = builder.startTime;
this.duration = builder.duration;
this.fixedPrice = builder.fixedPrice;
this.usagePrice = builder.usagePrice;
this.cacheNodeCount = builder.cacheNodeCount;
this.productDescription = builder.productDescription;
this.offeringType = builder.offeringType;
this.state = builder.state;
this.recurringCharges = builder.recurringCharges;
}
/**
*
* The unique identifier for the reservation.
*
*
* @return The unique identifier for the reservation.
*/
public String reservedCacheNodeId() {
return reservedCacheNodeId;
}
/**
*
* The offering identifier.
*
*
* @return The offering identifier.
*/
public String reservedCacheNodesOfferingId() {
return reservedCacheNodesOfferingId;
}
/**
*
* The cache node type for the reserved cache nodes.
*
*
* Valid node types are as follows:
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation: cache.t2.micro
, cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
, cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: cache.t1.micro
, cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized: cache.c1.xlarge
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* -
*
* Previous generation: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis backup/restore is not supported for Redis (cluster mode disabled) T1 and T2 instances. Backup/restore is
* supported on Redis (cluster mode enabled) T2 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see Amazon ElastiCache Product Features and Details and either
* Cache Node Type-Specific Parameters for Memcached or Cache Node Type-Specific Parameters for Redis.
*
*
* @return The cache node type for the reserved cache nodes.
*
* Valid node types are as follows:
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
, cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
, cache.m4.large
,
* cache.m4.xlarge
, cache.m4.2xlarge
, cache.m4.4xlarge
,
* cache.m4.10xlarge
*
*
* -
*
* Previous generation: cache.t1.micro
, cache.m1.small
,
* cache.m1.medium
, cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized: cache.c1.xlarge
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* -
*
* Previous generation: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis backup/restore is not supported for Redis (cluster mode disabled) T1 and T2 instances.
* Backup/restore is supported on Redis (cluster mode enabled) T2 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see Amazon ElastiCache Product Features and Details and
* either Cache Node Type-Specific Parameters for Memcached or Cache Node Type-Specific Parameters for Redis.
*/
public String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The time the reservation started.
*
*
* @return The time the reservation started.
*/
public Instant startTime() {
return startTime;
}
/**
*
* The duration of the reservation in seconds.
*
*
* @return The duration of the reservation in seconds.
*/
public Integer duration() {
return duration;
}
/**
*
* The fixed price charged for this reserved cache node.
*
*
* @return The fixed price charged for this reserved cache node.
*/
public Double fixedPrice() {
return fixedPrice;
}
/**
*
* The hourly price charged for this reserved cache node.
*
*
* @return The hourly price charged for this reserved cache node.
*/
public Double usagePrice() {
return usagePrice;
}
/**
*
* The number of cache nodes that have been reserved.
*
*
* @return The number of cache nodes that have been reserved.
*/
public Integer cacheNodeCount() {
return cacheNodeCount;
}
/**
*
* The description of the reserved cache node.
*
*
* @return The description of the reserved cache node.
*/
public String productDescription() {
return productDescription;
}
/**
*
* The offering type of this reserved cache node.
*
*
* @return The offering type of this reserved cache node.
*/
public String offeringType() {
return offeringType;
}
/**
*
* The state of the reserved cache node.
*
*
* @return The state of the reserved cache node.
*/
public String state() {
return state;
}
/**
*
* The recurring price charged to run this reserved cache node.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The recurring price charged to run this reserved cache node.
*/
public List recurringCharges() {
return recurringCharges;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((reservedCacheNodeId() == null) ? 0 : reservedCacheNodeId().hashCode());
hashCode = 31 * hashCode + ((reservedCacheNodesOfferingId() == null) ? 0 : reservedCacheNodesOfferingId().hashCode());
hashCode = 31 * hashCode + ((cacheNodeType() == null) ? 0 : cacheNodeType().hashCode());
hashCode = 31 * hashCode + ((startTime() == null) ? 0 : startTime().hashCode());
hashCode = 31 * hashCode + ((duration() == null) ? 0 : duration().hashCode());
hashCode = 31 * hashCode + ((fixedPrice() == null) ? 0 : fixedPrice().hashCode());
hashCode = 31 * hashCode + ((usagePrice() == null) ? 0 : usagePrice().hashCode());
hashCode = 31 * hashCode + ((cacheNodeCount() == null) ? 0 : cacheNodeCount().hashCode());
hashCode = 31 * hashCode + ((productDescription() == null) ? 0 : productDescription().hashCode());
hashCode = 31 * hashCode + ((offeringType() == null) ? 0 : offeringType().hashCode());
hashCode = 31 * hashCode + ((state() == null) ? 0 : state().hashCode());
hashCode = 31 * hashCode + ((recurringCharges() == null) ? 0 : recurringCharges().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReservedCacheNode)) {
return false;
}
ReservedCacheNode other = (ReservedCacheNode) obj;
if (other.reservedCacheNodeId() == null ^ this.reservedCacheNodeId() == null) {
return false;
}
if (other.reservedCacheNodeId() != null && !other.reservedCacheNodeId().equals(this.reservedCacheNodeId())) {
return false;
}
if (other.reservedCacheNodesOfferingId() == null ^ this.reservedCacheNodesOfferingId() == null) {
return false;
}
if (other.reservedCacheNodesOfferingId() != null
&& !other.reservedCacheNodesOfferingId().equals(this.reservedCacheNodesOfferingId())) {
return false;
}
if (other.cacheNodeType() == null ^ this.cacheNodeType() == null) {
return false;
}
if (other.cacheNodeType() != null && !other.cacheNodeType().equals(this.cacheNodeType())) {
return false;
}
if (other.startTime() == null ^ this.startTime() == null) {
return false;
}
if (other.startTime() != null && !other.startTime().equals(this.startTime())) {
return false;
}
if (other.duration() == null ^ this.duration() == null) {
return false;
}
if (other.duration() != null && !other.duration().equals(this.duration())) {
return false;
}
if (other.fixedPrice() == null ^ this.fixedPrice() == null) {
return false;
}
if (other.fixedPrice() != null && !other.fixedPrice().equals(this.fixedPrice())) {
return false;
}
if (other.usagePrice() == null ^ this.usagePrice() == null) {
return false;
}
if (other.usagePrice() != null && !other.usagePrice().equals(this.usagePrice())) {
return false;
}
if (other.cacheNodeCount() == null ^ this.cacheNodeCount() == null) {
return false;
}
if (other.cacheNodeCount() != null && !other.cacheNodeCount().equals(this.cacheNodeCount())) {
return false;
}
if (other.productDescription() == null ^ this.productDescription() == null) {
return false;
}
if (other.productDescription() != null && !other.productDescription().equals(this.productDescription())) {
return false;
}
if (other.offeringType() == null ^ this.offeringType() == null) {
return false;
}
if (other.offeringType() != null && !other.offeringType().equals(this.offeringType())) {
return false;
}
if (other.state() == null ^ this.state() == null) {
return false;
}
if (other.state() != null && !other.state().equals(this.state())) {
return false;
}
if (other.recurringCharges() == null ^ this.recurringCharges() == null) {
return false;
}
if (other.recurringCharges() != null && !other.recurringCharges().equals(this.recurringCharges())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (reservedCacheNodeId() != null) {
sb.append("ReservedCacheNodeId: ").append(reservedCacheNodeId()).append(",");
}
if (reservedCacheNodesOfferingId() != null) {
sb.append("ReservedCacheNodesOfferingId: ").append(reservedCacheNodesOfferingId()).append(",");
}
if (cacheNodeType() != null) {
sb.append("CacheNodeType: ").append(cacheNodeType()).append(",");
}
if (startTime() != null) {
sb.append("StartTime: ").append(startTime()).append(",");
}
if (duration() != null) {
sb.append("Duration: ").append(duration()).append(",");
}
if (fixedPrice() != null) {
sb.append("FixedPrice: ").append(fixedPrice()).append(",");
}
if (usagePrice() != null) {
sb.append("UsagePrice: ").append(usagePrice()).append(",");
}
if (cacheNodeCount() != null) {
sb.append("CacheNodeCount: ").append(cacheNodeCount()).append(",");
}
if (productDescription() != null) {
sb.append("ProductDescription: ").append(productDescription()).append(",");
}
if (offeringType() != null) {
sb.append("OfferingType: ").append(offeringType()).append(",");
}
if (state() != null) {
sb.append("State: ").append(state()).append(",");
}
if (recurringCharges() != null) {
sb.append("RecurringCharges: ").append(recurringCharges()).append(",");
}
sb.append("}");
return sb.toString();
}
public interface Builder extends CopyableBuilder {
/**
*
* The unique identifier for the reservation.
*
*
* @param reservedCacheNodeId
* The unique identifier for the reservation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reservedCacheNodeId(String reservedCacheNodeId);
/**
*
* The offering identifier.
*
*
* @param reservedCacheNodesOfferingId
* The offering identifier.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reservedCacheNodesOfferingId(String reservedCacheNodesOfferingId);
/**
*
* The cache node type for the reserved cache nodes.
*
*
* Valid node types are as follows:
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation: cache.t2.micro
, cache.t2.small
, cache.t2.medium
,
* cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
, cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: cache.t1.micro
, cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized: cache.c1.xlarge
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* -
*
* Previous generation: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis backup/restore is not supported for Redis (cluster mode disabled) T1 and T2 instances. Backup/restore
* is supported on Redis (cluster mode enabled) T2 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see Amazon ElastiCache Product Features and Details and
* either Cache Node Type-Specific Parameters for Memcached or Cache Node Type-Specific Parameters for Redis.
*
*
* @param cacheNodeType
* The cache node type for the reserved cache nodes.
*
* Valid node types are as follows:
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
, cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
, cache.m4.large
,
* cache.m4.xlarge
, cache.m4.2xlarge
, cache.m4.4xlarge
,
* cache.m4.10xlarge
*
*
* -
*
* Previous generation: cache.t1.micro
, cache.m1.small
,
* cache.m1.medium
, cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized: cache.c1.xlarge
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* -
*
* Previous generation: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis backup/restore is not supported for Redis (cluster mode disabled) T1 and T2 instances.
* Backup/restore is supported on Redis (cluster mode enabled) T2 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see Amazon ElastiCache Product Features and Details
* and either Cache Node Type-Specific Parameters for Memcached or Cache Node Type-Specific Parameters for Redis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodeType(String cacheNodeType);
/**
*
* The time the reservation started.
*
*
* @param startTime
* The time the reservation started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startTime(Instant startTime);
/**
*
* The duration of the reservation in seconds.
*
*
* @param duration
* The duration of the reservation in seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder duration(Integer duration);
/**
*
* The fixed price charged for this reserved cache node.
*
*
* @param fixedPrice
* The fixed price charged for this reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fixedPrice(Double fixedPrice);
/**
*
* The hourly price charged for this reserved cache node.
*
*
* @param usagePrice
* The hourly price charged for this reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder usagePrice(Double usagePrice);
/**
*
* The number of cache nodes that have been reserved.
*
*
* @param cacheNodeCount
* The number of cache nodes that have been reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodeCount(Integer cacheNodeCount);
/**
*
* The description of the reserved cache node.
*
*
* @param productDescription
* The description of the reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder productDescription(String productDescription);
/**
*
* The offering type of this reserved cache node.
*
*
* @param offeringType
* The offering type of this reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder offeringType(String offeringType);
/**
*
* The state of the reserved cache node.
*
*
* @param state
* The state of the reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder state(String state);
/**
*
* The recurring price charged to run this reserved cache node.
*
*
* @param recurringCharges
* The recurring price charged to run this reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recurringCharges(Collection recurringCharges);
/**
*
* The recurring price charged to run this reserved cache node.
*
*
* @param recurringCharges
* The recurring price charged to run this reserved cache node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recurringCharges(RecurringCharge... recurringCharges);
}
private static final class BuilderImpl implements Builder {
private String reservedCacheNodeId;
private String reservedCacheNodesOfferingId;
private String cacheNodeType;
private Instant startTime;
private Integer duration;
private Double fixedPrice;
private Double usagePrice;
private Integer cacheNodeCount;
private String productDescription;
private String offeringType;
private String state;
private List recurringCharges;
private BuilderImpl() {
}
private BuilderImpl(ReservedCacheNode model) {
setReservedCacheNodeId(model.reservedCacheNodeId);
setReservedCacheNodesOfferingId(model.reservedCacheNodesOfferingId);
setCacheNodeType(model.cacheNodeType);
setStartTime(model.startTime);
setDuration(model.duration);
setFixedPrice(model.fixedPrice);
setUsagePrice(model.usagePrice);
setCacheNodeCount(model.cacheNodeCount);
setProductDescription(model.productDescription);
setOfferingType(model.offeringType);
setState(model.state);
setRecurringCharges(model.recurringCharges);
}
public final String getReservedCacheNodeId() {
return reservedCacheNodeId;
}
@Override
public final Builder reservedCacheNodeId(String reservedCacheNodeId) {
this.reservedCacheNodeId = reservedCacheNodeId;
return this;
}
public final void setReservedCacheNodeId(String reservedCacheNodeId) {
this.reservedCacheNodeId = reservedCacheNodeId;
}
public final String getReservedCacheNodesOfferingId() {
return reservedCacheNodesOfferingId;
}
@Override
public final Builder reservedCacheNodesOfferingId(String reservedCacheNodesOfferingId) {
this.reservedCacheNodesOfferingId = reservedCacheNodesOfferingId;
return this;
}
public final void setReservedCacheNodesOfferingId(String reservedCacheNodesOfferingId) {
this.reservedCacheNodesOfferingId = reservedCacheNodesOfferingId;
}
public final String getCacheNodeType() {
return cacheNodeType;
}
@Override
public final Builder cacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
return this;
}
public final void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
public final Instant getStartTime() {
return startTime;
}
@Override
public final Builder startTime(Instant startTime) {
this.startTime = startTime;
return this;
}
public final void setStartTime(Instant startTime) {
this.startTime = startTime;
}
public final Integer getDuration() {
return duration;
}
@Override
public final Builder duration(Integer duration) {
this.duration = duration;
return this;
}
public final void setDuration(Integer duration) {
this.duration = duration;
}
public final Double getFixedPrice() {
return fixedPrice;
}
@Override
public final Builder fixedPrice(Double fixedPrice) {
this.fixedPrice = fixedPrice;
return this;
}
public final void setFixedPrice(Double fixedPrice) {
this.fixedPrice = fixedPrice;
}
public final Double getUsagePrice() {
return usagePrice;
}
@Override
public final Builder usagePrice(Double usagePrice) {
this.usagePrice = usagePrice;
return this;
}
public final void setUsagePrice(Double usagePrice) {
this.usagePrice = usagePrice;
}
public final Integer getCacheNodeCount() {
return cacheNodeCount;
}
@Override
public final Builder cacheNodeCount(Integer cacheNodeCount) {
this.cacheNodeCount = cacheNodeCount;
return this;
}
public final void setCacheNodeCount(Integer cacheNodeCount) {
this.cacheNodeCount = cacheNodeCount;
}
public final String getProductDescription() {
return productDescription;
}
@Override
public final Builder productDescription(String productDescription) {
this.productDescription = productDescription;
return this;
}
public final void setProductDescription(String productDescription) {
this.productDescription = productDescription;
}
public final String getOfferingType() {
return offeringType;
}
@Override
public final Builder offeringType(String offeringType) {
this.offeringType = offeringType;
return this;
}
public final void setOfferingType(String offeringType) {
this.offeringType = offeringType;
}
public final String getState() {
return state;
}
@Override
public final Builder state(String state) {
this.state = state;
return this;
}
public final void setState(String state) {
this.state = state;
}
public final Collection getRecurringCharges() {
return recurringCharges;
}
@Override
public final Builder recurringCharges(Collection recurringCharges) {
this.recurringCharges = RecurringChargeListCopier.copy(recurringCharges);
return this;
}
@Override
@SafeVarargs
public final Builder recurringCharges(RecurringCharge... recurringCharges) {
recurringCharges(Arrays.asList(recurringCharges));
return this;
}
public final void setRecurringCharges(Collection recurringCharges) {
this.recurringCharges = RecurringChargeListCopier.copy(recurringCharges);
}
@Override
public ReservedCacheNode build() {
return new ReservedCacheNode(this);
}
}
}