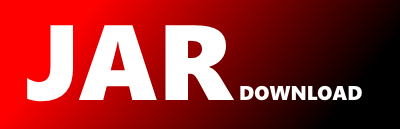
software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a ModifyReplicationGroups
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyReplicationGroupRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(ModifyReplicationGroupRequest::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField REPLICATION_GROUP_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationGroupDescription")
.getter(getter(ModifyReplicationGroupRequest::replicationGroupDescription))
.setter(setter(Builder::replicationGroupDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupDescription")
.build()).build();
private static final SdkField PRIMARY_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PrimaryClusterId").getter(getter(ModifyReplicationGroupRequest::primaryClusterId))
.setter(setter(Builder::primaryClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrimaryClusterId").build()).build();
private static final SdkField SNAPSHOTTING_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshottingClusterId").getter(getter(ModifyReplicationGroupRequest::snapshottingClusterId))
.setter(setter(Builder::snapshottingClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshottingClusterId").build())
.build();
private static final SdkField AUTOMATIC_FAILOVER_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutomaticFailoverEnabled").getter(getter(ModifyReplicationGroupRequest::automaticFailoverEnabled))
.setter(setter(Builder::automaticFailoverEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailoverEnabled").build())
.build();
private static final SdkField MULTI_AZ_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZEnabled").getter(getter(ModifyReplicationGroupRequest::multiAZEnabled))
.setter(setter(Builder::multiAZEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZEnabled").build()).build();
private static final SdkField NODE_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeGroupId").getter(getter(ModifyReplicationGroupRequest::nodeGroupId))
.setter(setter(Builder::nodeGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupId").build()).build();
private static final SdkField> CACHE_SECURITY_GROUP_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheSecurityGroupNames")
.getter(getter(ModifyReplicationGroupRequest::cacheSecurityGroupNames))
.setter(setter(Builder::cacheSecurityGroupNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroupNames").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroupName").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(ModifyReplicationGroupRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("SecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SecurityGroupId").build()).build()).build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyReplicationGroupRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField NOTIFICATION_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotificationTopicArn").getter(getter(ModifyReplicationGroupRequest::notificationTopicArn))
.setter(setter(Builder::notificationTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicArn").build())
.build();
private static final SdkField CACHE_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheParameterGroupName").getter(getter(ModifyReplicationGroupRequest::cacheParameterGroupName))
.setter(setter(Builder::cacheParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroupName").build())
.build();
private static final SdkField NOTIFICATION_TOPIC_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotificationTopicStatus").getter(getter(ModifyReplicationGroupRequest::notificationTopicStatus))
.setter(setter(Builder::notificationTopicStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicStatus").build())
.build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyReplicationGroupRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ModifyReplicationGroupRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(ModifyReplicationGroupRequest::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(ModifyReplicationGroupRequest::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(ModifyReplicationGroupRequest::snapshotWindow))
.setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(ModifyReplicationGroupRequest::cacheNodeType))
.setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField AUTH_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthToken").getter(getter(ModifyReplicationGroupRequest::authToken)).setter(setter(Builder::authToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthToken").build()).build();
private static final SdkField AUTH_TOKEN_UPDATE_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthTokenUpdateStrategy").getter(getter(ModifyReplicationGroupRequest::authTokenUpdateStrategyAsString))
.setter(setter(Builder::authTokenUpdateStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenUpdateStrategy").build())
.build();
private static final SdkField> USER_GROUP_IDS_TO_ADD_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroupIdsToAdd")
.getter(getter(ModifyReplicationGroupRequest::userGroupIdsToAdd))
.setter(setter(Builder::userGroupIdsToAdd))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroupIdsToAdd").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> USER_GROUP_IDS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroupIdsToRemove")
.getter(getter(ModifyReplicationGroupRequest::userGroupIdsToRemove))
.setter(setter(Builder::userGroupIdsToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroupIdsToRemove").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REMOVE_USER_GROUPS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RemoveUserGroups").getter(getter(ModifyReplicationGroupRequest::removeUserGroups))
.setter(setter(Builder::removeUserGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemoveUserGroups").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
REPLICATION_GROUP_DESCRIPTION_FIELD, PRIMARY_CLUSTER_ID_FIELD, SNAPSHOTTING_CLUSTER_ID_FIELD,
AUTOMATIC_FAILOVER_ENABLED_FIELD, MULTI_AZ_ENABLED_FIELD, NODE_GROUP_ID_FIELD, CACHE_SECURITY_GROUP_NAMES_FIELD,
SECURITY_GROUP_IDS_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, NOTIFICATION_TOPIC_ARN_FIELD,
CACHE_PARAMETER_GROUP_NAME_FIELD, NOTIFICATION_TOPIC_STATUS_FIELD, APPLY_IMMEDIATELY_FIELD, ENGINE_VERSION_FIELD,
AUTO_MINOR_VERSION_UPGRADE_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD, SNAPSHOT_WINDOW_FIELD, CACHE_NODE_TYPE_FIELD,
AUTH_TOKEN_FIELD, AUTH_TOKEN_UPDATE_STRATEGY_FIELD, USER_GROUP_IDS_TO_ADD_FIELD, USER_GROUP_IDS_TO_REMOVE_FIELD,
REMOVE_USER_GROUPS_FIELD));
private final String replicationGroupId;
private final String replicationGroupDescription;
private final String primaryClusterId;
private final String snapshottingClusterId;
private final Boolean automaticFailoverEnabled;
private final Boolean multiAZEnabled;
private final String nodeGroupId;
private final List cacheSecurityGroupNames;
private final List securityGroupIds;
private final String preferredMaintenanceWindow;
private final String notificationTopicArn;
private final String cacheParameterGroupName;
private final String notificationTopicStatus;
private final Boolean applyImmediately;
private final String engineVersion;
private final Boolean autoMinorVersionUpgrade;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final String cacheNodeType;
private final String authToken;
private final String authTokenUpdateStrategy;
private final List userGroupIdsToAdd;
private final List userGroupIdsToRemove;
private final Boolean removeUserGroups;
private ModifyReplicationGroupRequest(BuilderImpl builder) {
super(builder);
this.replicationGroupId = builder.replicationGroupId;
this.replicationGroupDescription = builder.replicationGroupDescription;
this.primaryClusterId = builder.primaryClusterId;
this.snapshottingClusterId = builder.snapshottingClusterId;
this.automaticFailoverEnabled = builder.automaticFailoverEnabled;
this.multiAZEnabled = builder.multiAZEnabled;
this.nodeGroupId = builder.nodeGroupId;
this.cacheSecurityGroupNames = builder.cacheSecurityGroupNames;
this.securityGroupIds = builder.securityGroupIds;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.notificationTopicArn = builder.notificationTopicArn;
this.cacheParameterGroupName = builder.cacheParameterGroupName;
this.notificationTopicStatus = builder.notificationTopicStatus;
this.applyImmediately = builder.applyImmediately;
this.engineVersion = builder.engineVersion;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.cacheNodeType = builder.cacheNodeType;
this.authToken = builder.authToken;
this.authTokenUpdateStrategy = builder.authTokenUpdateStrategy;
this.userGroupIdsToAdd = builder.userGroupIdsToAdd;
this.userGroupIdsToRemove = builder.userGroupIdsToRemove;
this.removeUserGroups = builder.removeUserGroups;
}
/**
*
* The identifier of the replication group to modify.
*
*
* @return The identifier of the replication group to modify.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* A description for the replication group. Maximum length is 255 characters.
*
*
* @return A description for the replication group. Maximum length is 255 characters.
*/
public final String replicationGroupDescription() {
return replicationGroupDescription;
}
/**
*
* For replication groups with a single primary, if this parameter is specified, ElastiCache promotes the specified
* cluster in the specified replication group to the primary role. The nodes of all other clusters in the
* replication group are read replicas.
*
*
* @return For replication groups with a single primary, if this parameter is specified, ElastiCache promotes the
* specified cluster in the specified replication group to the primary role. The nodes of all other clusters
* in the replication group are read replicas.
*/
public final String primaryClusterId() {
return primaryClusterId;
}
/**
*
* The cluster ID that is used as the daily snapshot source for the replication group. This parameter cannot be set
* for Redis (cluster mode enabled) replication groups.
*
*
* @return The cluster ID that is used as the daily snapshot source for the replication group. This parameter cannot
* be set for Redis (cluster mode enabled) replication groups.
*/
public final String snapshottingClusterId() {
return snapshottingClusterId;
}
/**
*
* Determines whether a read replica is automatically promoted to read/write primary if the existing primary
* encounters a failure.
*
*
* Valid values: true
| false
*
*
* @return Determines whether a read replica is automatically promoted to read/write primary if the existing primary
* encounters a failure.
*
* Valid values: true
| false
*/
public final Boolean automaticFailoverEnabled() {
return automaticFailoverEnabled;
}
/**
*
* A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ.
*
*
* @return A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ.
*/
public final Boolean multiAZEnabled() {
return multiAZEnabled;
}
/**
*
* Deprecated. This parameter is not used.
*
*
* @return Deprecated. This parameter is not used.
*/
public final String nodeGroupId() {
return nodeGroupId;
}
/**
* Returns true if the CacheSecurityGroupNames property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasCacheSecurityGroupNames() {
return cacheSecurityGroupNames != null && !(cacheSecurityGroupNames instanceof SdkAutoConstructList);
}
/**
*
* A list of cache security group names to authorize for the clusters in this replication group. This change is
* asynchronously applied as soon as possible.
*
*
* This parameter can be used only with replication group containing clusters running outside of an Amazon Virtual
* Private Cloud (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be Default
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCacheSecurityGroupNames()} to see if a value was sent in this field.
*
*
* @return A list of cache security group names to authorize for the clusters in this replication group. This change
* is asynchronously applied as soon as possible.
*
* This parameter can be used only with replication group containing clusters running outside of an Amazon
* Virtual Private Cloud (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be Default
.
*/
public final List cacheSecurityGroupNames() {
return cacheSecurityGroupNames;
}
/**
* Returns true if the SecurityGroupIds property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* Specifies the VPC Security Groups associated with the clusters in the replication group.
*
*
* This parameter can be used only with replication group containing clusters running in an Amazon Virtual Private
* Cloud (Amazon VPC).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSecurityGroupIds()} to see if a value was sent in this field.
*
*
* @return Specifies the VPC Security Groups associated with the clusters in the replication group.
*
* This parameter can be used only with replication group containing clusters running in an Amazon Virtual
* Private Cloud (Amazon VPC).
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the replication group owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
* The Amazon SNS topic owner must be same as the replication group owner.
*
*/
public final String notificationTopicArn() {
return notificationTopicArn;
}
/**
*
* The name of the cache parameter group to apply to all of the clusters in this replication group. This change is
* asynchronously applied as soon as possible for parameters when the ApplyImmediately
parameter is
* specified as true
for this request.
*
*
* @return The name of the cache parameter group to apply to all of the clusters in this replication group. This
* change is asynchronously applied as soon as possible for parameters when the
* ApplyImmediately
parameter is specified as true
for this request.
*/
public final String cacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
*
* The status of the Amazon SNS notification topic for the replication group. Notifications are sent only if the
* status is active
.
*
*
* Valid values: active
| inactive
*
*
* @return The status of the Amazon SNS notification topic for the replication group. Notifications are sent only if
* the status is active
.
*
* Valid values: active
| inactive
*/
public final String notificationTopicStatus() {
return notificationTopicStatus;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the replication group.
*
*
* If false
, changes to the nodes in the replication group are applied on the next maintenance reboot,
* or the next failure reboot, whichever occurs first.
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @return If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the replication group.
*
* If false
, changes to the nodes in the replication group are applied on the next maintenance
* reboot, or the next failure reboot, whichever occurs first.
*
*
* Valid values: true
| false
*
*
* Default: false
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
/**
*
* The upgraded version of the cache engine to be run on the clusters in the replication group.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing replication group and create it anew with the earlier engine
* version.
*
*
* @return The upgraded version of the cache engine to be run on the clusters in the replication group.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing replication group and create it anew
* with the earlier engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* This parameter is currently disabled.
*
*
* @return This parameter is currently disabled.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic node group (shard) snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5
* days before being deleted.
*
*
* Important If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*
* @return The number of days for which ElastiCache retains automatic node group (shard) snapshots before deleting
* them. For example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today
* is retained for 5 days before being deleted.
*
* Important If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of the node group (shard)
* specified by SnapshottingClusterId
.
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of the node group
* (shard) specified by SnapshottingClusterId
.
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A valid cache node type that you want to scale this replication group to.
*
*
* @return A valid cache node type that you want to scale this replication group to.
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update-strategy
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server. This parameter must be
* specified with the auth-token-update-strategy
parameter. Password constraints:
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*/
public final String authToken() {
return authToken;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with Redis
* AUTH
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authTokenUpdateStrategy} will return {@link AuthTokenUpdateStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #authTokenUpdateStrategyAsString}.
*
*
* @return Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with
* Redis AUTH
* @see AuthTokenUpdateStrategyType
*/
public final AuthTokenUpdateStrategyType authTokenUpdateStrategy() {
return AuthTokenUpdateStrategyType.fromValue(authTokenUpdateStrategy);
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with Redis
* AUTH
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authTokenUpdateStrategy} will return {@link AuthTokenUpdateStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #authTokenUpdateStrategyAsString}.
*
*
* @return Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with
* Redis AUTH
* @see AuthTokenUpdateStrategyType
*/
public final String authTokenUpdateStrategyAsString() {
return authTokenUpdateStrategy;
}
/**
* Returns true if the UserGroupIdsToAdd property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasUserGroupIdsToAdd() {
return userGroupIdsToAdd != null && !(userGroupIdsToAdd instanceof SdkAutoConstructList);
}
/**
*
* A list of user group IDs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasUserGroupIdsToAdd()} to see if a value was sent in this field.
*
*
* @return A list of user group IDs.
*/
public final List userGroupIdsToAdd() {
return userGroupIdsToAdd;
}
/**
* Returns true if the UserGroupIdsToRemove property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasUserGroupIdsToRemove() {
return userGroupIdsToRemove != null && !(userGroupIdsToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of users groups to remove, meaning the users in the group no longer can access thereplication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasUserGroupIdsToRemove()} to see if a value was sent in this field.
*
*
* @return A list of users groups to remove, meaning the users in the group no longer can access thereplication
* group.
*/
public final List userGroupIdsToRemove() {
return userGroupIdsToRemove;
}
/**
*
* Removes the user groups that can access this replication group.
*
*
* @return Removes the user groups that can access this replication group.
*/
public final Boolean removeUserGroups() {
return removeUserGroups;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupDescription());
hashCode = 31 * hashCode + Objects.hashCode(primaryClusterId());
hashCode = 31 * hashCode + Objects.hashCode(snapshottingClusterId());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverEnabled());
hashCode = 31 * hashCode + Objects.hashCode(multiAZEnabled());
hashCode = 31 * hashCode + Objects.hashCode(nodeGroupId());
hashCode = 31 * hashCode + Objects.hashCode(hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicStatus());
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(authToken());
hashCode = 31 * hashCode + Objects.hashCode(authTokenUpdateStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroupIdsToAdd() ? userGroupIdsToAdd() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroupIdsToRemove() ? userGroupIdsToRemove() : null);
hashCode = 31 * hashCode + Objects.hashCode(removeUserGroups());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyReplicationGroupRequest)) {
return false;
}
ModifyReplicationGroupRequest other = (ModifyReplicationGroupRequest) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(replicationGroupDescription(), other.replicationGroupDescription())
&& Objects.equals(primaryClusterId(), other.primaryClusterId())
&& Objects.equals(snapshottingClusterId(), other.snapshottingClusterId())
&& Objects.equals(automaticFailoverEnabled(), other.automaticFailoverEnabled())
&& Objects.equals(multiAZEnabled(), other.multiAZEnabled()) && Objects.equals(nodeGroupId(), other.nodeGroupId())
&& hasCacheSecurityGroupNames() == other.hasCacheSecurityGroupNames()
&& Objects.equals(cacheSecurityGroupNames(), other.cacheSecurityGroupNames())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(notificationTopicArn(), other.notificationTopicArn())
&& Objects.equals(cacheParameterGroupName(), other.cacheParameterGroupName())
&& Objects.equals(notificationTopicStatus(), other.notificationTopicStatus())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(authToken(), other.authToken())
&& Objects.equals(authTokenUpdateStrategyAsString(), other.authTokenUpdateStrategyAsString())
&& hasUserGroupIdsToAdd() == other.hasUserGroupIdsToAdd()
&& Objects.equals(userGroupIdsToAdd(), other.userGroupIdsToAdd())
&& hasUserGroupIdsToRemove() == other.hasUserGroupIdsToRemove()
&& Objects.equals(userGroupIdsToRemove(), other.userGroupIdsToRemove())
&& Objects.equals(removeUserGroups(), other.removeUserGroups());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyReplicationGroupRequest").add("ReplicationGroupId", replicationGroupId())
.add("ReplicationGroupDescription", replicationGroupDescription()).add("PrimaryClusterId", primaryClusterId())
.add("SnapshottingClusterId", snapshottingClusterId())
.add("AutomaticFailoverEnabled", automaticFailoverEnabled()).add("MultiAZEnabled", multiAZEnabled())
.add("NodeGroupId", nodeGroupId())
.add("CacheSecurityGroupNames", hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null)
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("NotificationTopicArn", notificationTopicArn()).add("CacheParameterGroupName", cacheParameterGroupName())
.add("NotificationTopicStatus", notificationTopicStatus()).add("ApplyImmediately", applyImmediately())
.add("EngineVersion", engineVersion()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("CacheNodeType", cacheNodeType()).add("AuthToken", authToken())
.add("AuthTokenUpdateStrategy", authTokenUpdateStrategyAsString())
.add("UserGroupIdsToAdd", hasUserGroupIdsToAdd() ? userGroupIdsToAdd() : null)
.add("UserGroupIdsToRemove", hasUserGroupIdsToRemove() ? userGroupIdsToRemove() : null)
.add("RemoveUserGroups", removeUserGroups()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "ReplicationGroupDescription":
return Optional.ofNullable(clazz.cast(replicationGroupDescription()));
case "PrimaryClusterId":
return Optional.ofNullable(clazz.cast(primaryClusterId()));
case "SnapshottingClusterId":
return Optional.ofNullable(clazz.cast(snapshottingClusterId()));
case "AutomaticFailoverEnabled":
return Optional.ofNullable(clazz.cast(automaticFailoverEnabled()));
case "MultiAZEnabled":
return Optional.ofNullable(clazz.cast(multiAZEnabled()));
case "NodeGroupId":
return Optional.ofNullable(clazz.cast(nodeGroupId()));
case "CacheSecurityGroupNames":
return Optional.ofNullable(clazz.cast(cacheSecurityGroupNames()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "NotificationTopicArn":
return Optional.ofNullable(clazz.cast(notificationTopicArn()));
case "CacheParameterGroupName":
return Optional.ofNullable(clazz.cast(cacheParameterGroupName()));
case "NotificationTopicStatus":
return Optional.ofNullable(clazz.cast(notificationTopicStatus()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "AuthToken":
return Optional.ofNullable(clazz.cast(authToken()));
case "AuthTokenUpdateStrategy":
return Optional.ofNullable(clazz.cast(authTokenUpdateStrategyAsString()));
case "UserGroupIdsToAdd":
return Optional.ofNullable(clazz.cast(userGroupIdsToAdd()));
case "UserGroupIdsToRemove":
return Optional.ofNullable(clazz.cast(userGroupIdsToRemove()));
case "RemoveUserGroups":
return Optional.ofNullable(clazz.cast(removeUserGroups()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function