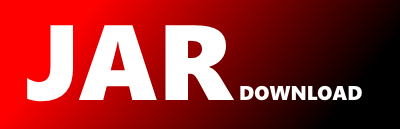
software.amazon.awssdk.services.elasticache.model.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticache Show documentation
Show all versions of elasticache Show documentation
The AWS Java SDK for Amazon ElastiCache module holds the client classes that are used for communicating
with Amazon ElastiCache Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class User implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("UserId")
.getter(getter(User::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserId").build()).build();
private static final SdkField USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserName").getter(getter(User::userName)).setter(setter(Builder::userName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserName").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(User::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(User::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ACCESS_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccessString").getter(getter(User::accessString)).setter(setter(Builder::accessString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccessString").build()).build();
private static final SdkField> USER_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroupIds")
.getter(getter(User::userGroupIds))
.setter(setter(Builder::userGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AUTHENTICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Authentication").getter(getter(User::authentication))
.setter(setter(Builder::authentication)).constructor(Authentication::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Authentication").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(User::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USER_ID_FIELD,
USER_NAME_FIELD, STATUS_FIELD, ENGINE_FIELD, ACCESS_STRING_FIELD, USER_GROUP_IDS_FIELD, AUTHENTICATION_FIELD,
ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String userId;
private final String userName;
private final String status;
private final String engine;
private final String accessString;
private final List userGroupIds;
private final Authentication authentication;
private final String arn;
private User(BuilderImpl builder) {
this.userId = builder.userId;
this.userName = builder.userName;
this.status = builder.status;
this.engine = builder.engine;
this.accessString = builder.accessString;
this.userGroupIds = builder.userGroupIds;
this.authentication = builder.authentication;
this.arn = builder.arn;
}
/**
*
* The ID of the user.
*
*
* @return The ID of the user.
*/
public final String userId() {
return userId;
}
/**
*
* The username of the user.
*
*
* @return The username of the user.
*/
public final String userName() {
return userName;
}
/**
*
* Indicates the user status. Can be "active", "modifying" or "deleting".
*
*
* @return Indicates the user status. Can be "active", "modifying" or "deleting".
*/
public final String status() {
return status;
}
/**
*
* The current supported value is Redis.
*
*
* @return The current supported value is Redis.
*/
public final String engine() {
return engine;
}
/**
*
* Access permissions string used for this user.
*
*
* @return Access permissions string used for this user.
*/
public final String accessString() {
return accessString;
}
/**
* Returns true if the UserGroupIds property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasUserGroupIds() {
return userGroupIds != null && !(userGroupIds instanceof SdkAutoConstructList);
}
/**
*
* Returns a list of the user group IDs the user belongs to.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasUserGroupIds()} to see if a value was sent in this field.
*
*
* @return Returns a list of the user group IDs the user belongs to.
*/
public final List userGroupIds() {
return userGroupIds;
}
/**
*
* Denotes whether the user requires a password to authenticate.
*
*
* @return Denotes whether the user requires a password to authenticate.
*/
public final Authentication authentication() {
return authentication;
}
/**
*
* The Amazon Resource Name (ARN) of the user.
*
*
* @return The Amazon Resource Name (ARN) of the user.
*/
public final String arn() {
return arn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(userName());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(accessString());
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroupIds() ? userGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(authentication());
hashCode = 31 * hashCode + Objects.hashCode(arn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof User)) {
return false;
}
User other = (User) obj;
return Objects.equals(userId(), other.userId()) && Objects.equals(userName(), other.userName())
&& Objects.equals(status(), other.status()) && Objects.equals(engine(), other.engine())
&& Objects.equals(accessString(), other.accessString()) && hasUserGroupIds() == other.hasUserGroupIds()
&& Objects.equals(userGroupIds(), other.userGroupIds())
&& Objects.equals(authentication(), other.authentication()) && Objects.equals(arn(), other.arn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("User").add("UserId", userId()).add("UserName", userName()).add("Status", status())
.add("Engine", engine()).add("AccessString", accessString())
.add("UserGroupIds", hasUserGroupIds() ? userGroupIds() : null).add("Authentication", authentication())
.add("ARN", arn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UserId":
return Optional.ofNullable(clazz.cast(userId()));
case "UserName":
return Optional.ofNullable(clazz.cast(userName()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "AccessString":
return Optional.ofNullable(clazz.cast(accessString()));
case "UserGroupIds":
return Optional.ofNullable(clazz.cast(userGroupIds()));
case "Authentication":
return Optional.ofNullable(clazz.cast(authentication()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy