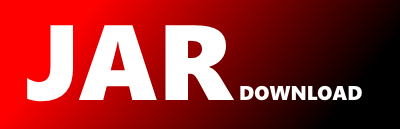
software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input for a ModifyReplicationGroupShardConfiguration
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyReplicationGroupShardConfigurationRequest extends ElastiCacheRequest
implements
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(ModifyReplicationGroupShardConfigurationRequest::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField NODE_GROUP_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NodeGroupCount").getter(getter(ModifyReplicationGroupShardConfigurationRequest::nodeGroupCount))
.setter(setter(Builder::nodeGroupCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupCount").build()).build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyReplicationGroupShardConfigurationRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField> RESHARDING_CONFIGURATION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReshardingConfiguration")
.getter(getter(ModifyReplicationGroupShardConfigurationRequest::reshardingConfiguration))
.setter(setter(Builder::reshardingConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReshardingConfiguration").build(),
ListTrait
.builder()
.memberLocationName("ReshardingConfiguration")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ReshardingConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReshardingConfiguration").build()).build()).build()).build();
private static final SdkField> NODE_GROUPS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NodeGroupsToRemove")
.getter(getter(ModifyReplicationGroupShardConfigurationRequest::nodeGroupsToRemove))
.setter(setter(Builder::nodeGroupsToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupsToRemove").build(),
ListTrait
.builder()
.memberLocationName("NodeGroupToRemove")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroupToRemove").build()).build()).build()).build();
private static final SdkField> NODE_GROUPS_TO_RETAIN_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NodeGroupsToRetain")
.getter(getter(ModifyReplicationGroupShardConfigurationRequest::nodeGroupsToRetain))
.setter(setter(Builder::nodeGroupsToRetain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupsToRetain").build(),
ListTrait
.builder()
.memberLocationName("NodeGroupToRetain")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroupToRetain").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
NODE_GROUP_COUNT_FIELD, APPLY_IMMEDIATELY_FIELD, RESHARDING_CONFIGURATION_FIELD, NODE_GROUPS_TO_REMOVE_FIELD,
NODE_GROUPS_TO_RETAIN_FIELD));
private final String replicationGroupId;
private final Integer nodeGroupCount;
private final Boolean applyImmediately;
private final List reshardingConfiguration;
private final List nodeGroupsToRemove;
private final List nodeGroupsToRetain;
private ModifyReplicationGroupShardConfigurationRequest(BuilderImpl builder) {
super(builder);
this.replicationGroupId = builder.replicationGroupId;
this.nodeGroupCount = builder.nodeGroupCount;
this.applyImmediately = builder.applyImmediately;
this.reshardingConfiguration = builder.reshardingConfiguration;
this.nodeGroupsToRemove = builder.nodeGroupsToRemove;
this.nodeGroupsToRetain = builder.nodeGroupsToRetain;
}
/**
*
* The name of the Redis (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*
*
* @return The name of the Redis (cluster mode enabled) cluster (replication group) on which the shards are to be
* configured.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The number of node groups (shards) that results from the modification of the shard configuration.
*
*
* @return The number of node groups (shards) that results from the modification of the shard configuration.
*/
public final Integer nodeGroupCount() {
return nodeGroupCount;
}
/**
*
* Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value for
* this parameter is true
.
*
*
* Value: true
*
*
* @return Indicates that the shard reconfiguration process begins immediately. At present, the only permitted value
* for this parameter is true
.
*
* Value: true
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
/**
* For responses, this returns true if the service returned a value for the ReshardingConfiguration property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasReshardingConfiguration() {
return reshardingConfiguration != null && !(reshardingConfiguration instanceof SdkAutoConstructList);
}
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReshardingConfiguration} method.
*
*
* @return Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
*/
public final List reshardingConfiguration() {
return reshardingConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the NodeGroupsToRemove property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNodeGroupsToRemove() {
return nodeGroupsToRemove != null && !(nodeGroupsToRemove instanceof SdkAutoConstructList);
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRemove
* is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
from the
* cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNodeGroupsToRemove} method.
*
*
* @return If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
*/
public final List nodeGroupsToRemove() {
return nodeGroupsToRemove;
}
/**
* For responses, this returns true if the service returned a value for the NodeGroupsToRetain property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNodeGroupsToRetain() {
return nodeGroupsToRetain != null && !(nodeGroupsToRetain instanceof SdkAutoConstructList);
}
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then either
* NodeGroupsToRemove
or NodeGroupsToRetain
is required. NodeGroupsToRetain
* is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNodeGroupsToRetain} method.
*
*
* @return If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*/
public final List nodeGroupsToRetain() {
return nodeGroupsToRetain;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(nodeGroupCount());
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(hasReshardingConfiguration() ? reshardingConfiguration() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNodeGroupsToRemove() ? nodeGroupsToRemove() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNodeGroupsToRetain() ? nodeGroupsToRetain() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyReplicationGroupShardConfigurationRequest)) {
return false;
}
ModifyReplicationGroupShardConfigurationRequest other = (ModifyReplicationGroupShardConfigurationRequest) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(nodeGroupCount(), other.nodeGroupCount())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& hasReshardingConfiguration() == other.hasReshardingConfiguration()
&& Objects.equals(reshardingConfiguration(), other.reshardingConfiguration())
&& hasNodeGroupsToRemove() == other.hasNodeGroupsToRemove()
&& Objects.equals(nodeGroupsToRemove(), other.nodeGroupsToRemove())
&& hasNodeGroupsToRetain() == other.hasNodeGroupsToRetain()
&& Objects.equals(nodeGroupsToRetain(), other.nodeGroupsToRetain());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyReplicationGroupShardConfigurationRequest")
.add("ReplicationGroupId", replicationGroupId()).add("NodeGroupCount", nodeGroupCount())
.add("ApplyImmediately", applyImmediately())
.add("ReshardingConfiguration", hasReshardingConfiguration() ? reshardingConfiguration() : null)
.add("NodeGroupsToRemove", hasNodeGroupsToRemove() ? nodeGroupsToRemove() : null)
.add("NodeGroupsToRetain", hasNodeGroupsToRetain() ? nodeGroupsToRetain() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "NodeGroupCount":
return Optional.ofNullable(clazz.cast(nodeGroupCount()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "ReshardingConfiguration":
return Optional.ofNullable(clazz.cast(reshardingConfiguration()));
case "NodeGroupsToRemove":
return Optional.ofNullable(clazz.cast(nodeGroupsToRemove()));
case "NodeGroupsToRetain":
return Optional.ofNullable(clazz.cast(nodeGroupsToRetain()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Value: true
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder applyImmediately(Boolean applyImmediately);
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* @param reshardingConfiguration
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use
* this parameter to specify the preferred availability zones of the cluster's shards. If you omit this
* parameter ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reshardingConfiguration(Collection reshardingConfiguration);
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
*
* @param reshardingConfiguration
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use
* this parameter to specify the preferred availability zones of the cluster's shards. If you omit this
* parameter ElastiCache selects availability zones for you.
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the
* current number of node groups (shards).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reshardingConfiguration(ReshardingConfiguration... reshardingConfiguration);
/**
*
* Specifies the preferred availability zones for each node group in the cluster. If the value of
* NodeGroupCount
is greater than the current number of node groups (shards), you can use this
* parameter to specify the preferred availability zones of the cluster's shards. If you omit this parameter
* ElastiCache selects availability zones for you.
*
*
* You can specify this parameter only if the value of NodeGroupCount
is greater than the current
* number of node groups (shards).
*
* This is a convenience method that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #reshardingConfiguration(List)}.
*
* @param reshardingConfiguration
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #reshardingConfiguration(List)
*/
Builder reshardingConfiguration(Consumer... reshardingConfiguration);
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
from
* the cluster.
*
*
* @param nodeGroupsToRemove
* If the value of NodeGroupCount
is less than the current number of node groups (shards),
* then either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nodeGroupsToRemove(Collection nodeGroupsToRemove);
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
from
* the cluster.
*
*
* @param nodeGroupsToRemove
* If the value of NodeGroupCount
is less than the current number of node groups (shards),
* then either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRemove
is a list of NodeGroupId
s to remove from the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups listed by NodeGroupsToRemove
* from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nodeGroupsToRemove(String... nodeGroupsToRemove);
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* @param nodeGroupsToRetain
* If the value of NodeGroupCount
is less than the current number of node groups (shards),
* then either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nodeGroupsToRetain(Collection nodeGroupsToRetain);
/**
*
* If the value of NodeGroupCount
is less than the current number of node groups (shards), then
* either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
*
*
* @param nodeGroupsToRetain
* If the value of NodeGroupCount
is less than the current number of node groups (shards),
* then either NodeGroupsToRemove
or NodeGroupsToRetain
is required.
* NodeGroupsToRetain
is a list of NodeGroupId
s to retain in the cluster.
*
* ElastiCache for Redis will attempt to remove all node groups except those listed by
* NodeGroupsToRetain
from the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nodeGroupsToRetain(String... nodeGroupsToRetain);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ElastiCacheRequest.BuilderImpl implements Builder {
private String replicationGroupId;
private Integer nodeGroupCount;
private Boolean applyImmediately;
private List reshardingConfiguration = DefaultSdkAutoConstructList.getInstance();
private List nodeGroupsToRemove = DefaultSdkAutoConstructList.getInstance();
private List nodeGroupsToRetain = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(ModifyReplicationGroupShardConfigurationRequest model) {
super(model);
replicationGroupId(model.replicationGroupId);
nodeGroupCount(model.nodeGroupCount);
applyImmediately(model.applyImmediately);
reshardingConfiguration(model.reshardingConfiguration);
nodeGroupsToRemove(model.nodeGroupsToRemove);
nodeGroupsToRetain(model.nodeGroupsToRetain);
}
public final String getReplicationGroupId() {
return replicationGroupId;
}
public final void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
@Override
public final Builder replicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
return this;
}
public final Integer getNodeGroupCount() {
return nodeGroupCount;
}
public final void setNodeGroupCount(Integer nodeGroupCount) {
this.nodeGroupCount = nodeGroupCount;
}
@Override
public final Builder nodeGroupCount(Integer nodeGroupCount) {
this.nodeGroupCount = nodeGroupCount;
return this;
}
public final Boolean getApplyImmediately() {
return applyImmediately;
}
public final void setApplyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
}
@Override
public final Builder applyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
return this;
}
public final List getReshardingConfiguration() {
List result = ReshardingConfigurationListCopier
.copyToBuilder(this.reshardingConfiguration);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setReshardingConfiguration(Collection reshardingConfiguration) {
this.reshardingConfiguration = ReshardingConfigurationListCopier.copyFromBuilder(reshardingConfiguration);
}
@Override
public final Builder reshardingConfiguration(Collection reshardingConfiguration) {
this.reshardingConfiguration = ReshardingConfigurationListCopier.copy(reshardingConfiguration);
return this;
}
@Override
@SafeVarargs
public final Builder reshardingConfiguration(ReshardingConfiguration... reshardingConfiguration) {
reshardingConfiguration(Arrays.asList(reshardingConfiguration));
return this;
}
@Override
@SafeVarargs
public final Builder reshardingConfiguration(Consumer... reshardingConfiguration) {
reshardingConfiguration(Stream.of(reshardingConfiguration)
.map(c -> ReshardingConfiguration.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Collection getNodeGroupsToRemove() {
if (nodeGroupsToRemove instanceof SdkAutoConstructList) {
return null;
}
return nodeGroupsToRemove;
}
public final void setNodeGroupsToRemove(Collection nodeGroupsToRemove) {
this.nodeGroupsToRemove = NodeGroupsToRemoveListCopier.copy(nodeGroupsToRemove);
}
@Override
public final Builder nodeGroupsToRemove(Collection nodeGroupsToRemove) {
this.nodeGroupsToRemove = NodeGroupsToRemoveListCopier.copy(nodeGroupsToRemove);
return this;
}
@Override
@SafeVarargs
public final Builder nodeGroupsToRemove(String... nodeGroupsToRemove) {
nodeGroupsToRemove(Arrays.asList(nodeGroupsToRemove));
return this;
}
public final Collection getNodeGroupsToRetain() {
if (nodeGroupsToRetain instanceof SdkAutoConstructList) {
return null;
}
return nodeGroupsToRetain;
}
public final void setNodeGroupsToRetain(Collection nodeGroupsToRetain) {
this.nodeGroupsToRetain = NodeGroupsToRetainListCopier.copy(nodeGroupsToRetain);
}
@Override
public final Builder nodeGroupsToRetain(Collection nodeGroupsToRetain) {
this.nodeGroupsToRetain = NodeGroupsToRetainListCopier.copy(nodeGroupsToRetain);
return this;
}
@Override
@SafeVarargs
public final Builder nodeGroupsToRetain(String... nodeGroupsToRetain) {
nodeGroupsToRetain(Arrays.asList(nodeGroupsToRetain));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public ModifyReplicationGroupShardConfigurationRequest build() {
return new ModifyReplicationGroupShardConfigurationRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}