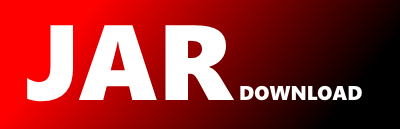
software.amazon.awssdk.services.elasticache.model.ReplicationGroupPendingModifiedValues Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The settings to be applied to the Redis replication group, either immediately or during the next maintenance window.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationGroupPendingModifiedValues implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PRIMARY_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PrimaryClusterId").getter(getter(ReplicationGroupPendingModifiedValues::primaryClusterId))
.setter(setter(Builder::primaryClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrimaryClusterId").build()).build();
private static final SdkField AUTOMATIC_FAILOVER_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutomaticFailoverStatus")
.getter(getter(ReplicationGroupPendingModifiedValues::automaticFailoverStatusAsString))
.setter(setter(Builder::automaticFailoverStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailoverStatus").build())
.build();
private static final SdkField RESHARDING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Resharding")
.getter(getter(ReplicationGroupPendingModifiedValues::resharding)).setter(setter(Builder::resharding))
.constructor(ReshardingStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Resharding").build()).build();
private static final SdkField AUTH_TOKEN_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthTokenStatus").getter(getter(ReplicationGroupPendingModifiedValues::authTokenStatusAsString))
.setter(setter(Builder::authTokenStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenStatus").build()).build();
private static final SdkField USER_GROUPS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("UserGroups")
.getter(getter(ReplicationGroupPendingModifiedValues::userGroups)).setter(setter(Builder::userGroups))
.constructor(UserGroupsUpdateStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroups").build()).build();
private static final SdkField> LOG_DELIVERY_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LogDeliveryConfigurations")
.getter(getter(ReplicationGroupPendingModifiedValues::logDeliveryConfigurations))
.setter(setter(Builder::logDeliveryConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDeliveryConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PendingLogDeliveryConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PRIMARY_CLUSTER_ID_FIELD,
AUTOMATIC_FAILOVER_STATUS_FIELD, RESHARDING_FIELD, AUTH_TOKEN_STATUS_FIELD, USER_GROUPS_FIELD,
LOG_DELIVERY_CONFIGURATIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String primaryClusterId;
private final String automaticFailoverStatus;
private final ReshardingStatus resharding;
private final String authTokenStatus;
private final UserGroupsUpdateStatus userGroups;
private final List logDeliveryConfigurations;
private ReplicationGroupPendingModifiedValues(BuilderImpl builder) {
this.primaryClusterId = builder.primaryClusterId;
this.automaticFailoverStatus = builder.automaticFailoverStatus;
this.resharding = builder.resharding;
this.authTokenStatus = builder.authTokenStatus;
this.userGroups = builder.userGroups;
this.logDeliveryConfigurations = builder.logDeliveryConfigurations;
}
/**
*
* The primary cluster ID that is applied immediately (if --apply-immediately
was specified), or during
* the next maintenance window.
*
*
* @return The primary cluster ID that is applied immediately (if --apply-immediately
was specified),
* or during the next maintenance window.
*/
public final String primaryClusterId() {
return primaryClusterId;
}
/**
*
* Indicates the status of automatic failover for this Redis replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #automaticFailoverStatus} will return {@link PendingAutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #automaticFailoverStatusAsString}.
*
*
* @return Indicates the status of automatic failover for this Redis replication group.
* @see PendingAutomaticFailoverStatus
*/
public final PendingAutomaticFailoverStatus automaticFailoverStatus() {
return PendingAutomaticFailoverStatus.fromValue(automaticFailoverStatus);
}
/**
*
* Indicates the status of automatic failover for this Redis replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #automaticFailoverStatus} will return {@link PendingAutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #automaticFailoverStatusAsString}.
*
*
* @return Indicates the status of automatic failover for this Redis replication group.
* @see PendingAutomaticFailoverStatus
*/
public final String automaticFailoverStatusAsString() {
return automaticFailoverStatus;
}
/**
*
* The status of an online resharding operation.
*
*
* @return The status of an online resharding operation.
*/
public final ReshardingStatus resharding() {
return resharding;
}
/**
*
* The auth token status
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authTokenStatus}
* will return {@link AuthTokenUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #authTokenStatusAsString}.
*
*
* @return The auth token status
* @see AuthTokenUpdateStatus
*/
public final AuthTokenUpdateStatus authTokenStatus() {
return AuthTokenUpdateStatus.fromValue(authTokenStatus);
}
/**
*
* The auth token status
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authTokenStatus}
* will return {@link AuthTokenUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #authTokenStatusAsString}.
*
*
* @return The auth token status
* @see AuthTokenUpdateStatus
*/
public final String authTokenStatusAsString() {
return authTokenStatus;
}
/**
*
* The user group being modified.
*
*
* @return The user group being modified.
*/
public final UserGroupsUpdateStatus userGroups() {
return userGroups;
}
/**
* For responses, this returns true if the service returned a value for the LogDeliveryConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogDeliveryConfigurations() {
return logDeliveryConfigurations != null && !(logDeliveryConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The log delivery configurations being modified
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogDeliveryConfigurations} method.
*
*
* @return The log delivery configurations being modified
*/
public final List logDeliveryConfigurations() {
return logDeliveryConfigurations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(primaryClusterId());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resharding());
hashCode = 31 * hashCode + Objects.hashCode(authTokenStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(userGroups());
hashCode = 31 * hashCode + Objects.hashCode(hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationGroupPendingModifiedValues)) {
return false;
}
ReplicationGroupPendingModifiedValues other = (ReplicationGroupPendingModifiedValues) obj;
return Objects.equals(primaryClusterId(), other.primaryClusterId())
&& Objects.equals(automaticFailoverStatusAsString(), other.automaticFailoverStatusAsString())
&& Objects.equals(resharding(), other.resharding())
&& Objects.equals(authTokenStatusAsString(), other.authTokenStatusAsString())
&& Objects.equals(userGroups(), other.userGroups())
&& hasLogDeliveryConfigurations() == other.hasLogDeliveryConfigurations()
&& Objects.equals(logDeliveryConfigurations(), other.logDeliveryConfigurations());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReplicationGroupPendingModifiedValues").add("PrimaryClusterId", primaryClusterId())
.add("AutomaticFailoverStatus", automaticFailoverStatusAsString()).add("Resharding", resharding())
.add("AuthTokenStatus", authTokenStatusAsString()).add("UserGroups", userGroups())
.add("LogDeliveryConfigurations", hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PrimaryClusterId":
return Optional.ofNullable(clazz.cast(primaryClusterId()));
case "AutomaticFailoverStatus":
return Optional.ofNullable(clazz.cast(automaticFailoverStatusAsString()));
case "Resharding":
return Optional.ofNullable(clazz.cast(resharding()));
case "AuthTokenStatus":
return Optional.ofNullable(clazz.cast(authTokenStatusAsString()));
case "UserGroups":
return Optional.ofNullable(clazz.cast(userGroups()));
case "LogDeliveryConfigurations":
return Optional.ofNullable(clazz.cast(logDeliveryConfigurations()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function