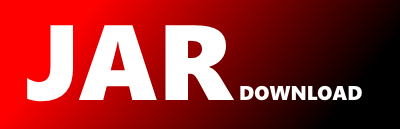
software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a ModifyCacheCluster
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyCacheClusterRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheClusterId").getter(getter(ModifyCacheClusterRequest::cacheClusterId))
.setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField NUM_CACHE_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumCacheNodes").getter(getter(ModifyCacheClusterRequest::numCacheNodes))
.setter(setter(Builder::numCacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheNodes").build()).build();
private static final SdkField> CACHE_NODE_IDS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheNodeIdsToRemove")
.getter(getter(ModifyCacheClusterRequest::cacheNodeIdsToRemove))
.setter(setter(Builder::cacheNodeIdsToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeIdsToRemove").build(),
ListTrait
.builder()
.memberLocationName("CacheNodeId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheNodeId").build()).build()).build()).build();
private static final SdkField AZ_MODE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("AZMode")
.getter(getter(ModifyCacheClusterRequest::azModeAsString)).setter(setter(Builder::azMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AZMode").build()).build();
private static final SdkField> NEW_AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NewAvailabilityZones")
.getter(getter(ModifyCacheClusterRequest::newAvailabilityZones))
.setter(setter(Builder::newAvailabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewAvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName("PreferredAvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PreferredAvailabilityZone").build()).build()).build()).build();
private static final SdkField> CACHE_SECURITY_GROUP_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheSecurityGroupNames")
.getter(getter(ModifyCacheClusterRequest::cacheSecurityGroupNames))
.setter(setter(Builder::cacheSecurityGroupNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroupNames").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroupName").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(ModifyCacheClusterRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("SecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SecurityGroupId").build()).build()).build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyCacheClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField NOTIFICATION_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotificationTopicArn").getter(getter(ModifyCacheClusterRequest::notificationTopicArn))
.setter(setter(Builder::notificationTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicArn").build())
.build();
private static final SdkField CACHE_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheParameterGroupName").getter(getter(ModifyCacheClusterRequest::cacheParameterGroupName))
.setter(setter(Builder::cacheParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroupName").build())
.build();
private static final SdkField NOTIFICATION_TOPIC_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotificationTopicStatus").getter(getter(ModifyCacheClusterRequest::notificationTopicStatus))
.setter(setter(Builder::notificationTopicStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicStatus").build())
.build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyCacheClusterRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ModifyCacheClusterRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(ModifyCacheClusterRequest::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(ModifyCacheClusterRequest::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(ModifyCacheClusterRequest::snapshotWindow))
.setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(ModifyCacheClusterRequest::cacheNodeType))
.setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField AUTH_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthToken").getter(getter(ModifyCacheClusterRequest::authToken)).setter(setter(Builder::authToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthToken").build()).build();
private static final SdkField AUTH_TOKEN_UPDATE_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthTokenUpdateStrategy").getter(getter(ModifyCacheClusterRequest::authTokenUpdateStrategyAsString))
.setter(setter(Builder::authTokenUpdateStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenUpdateStrategy").build())
.build();
private static final SdkField> LOG_DELIVERY_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LogDeliveryConfigurations")
.getter(getter(ModifyCacheClusterRequest::logDeliveryConfigurations))
.setter(setter(Builder::logDeliveryConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDeliveryConfigurations").build(),
ListTrait
.builder()
.memberLocationName("LogDeliveryConfigurationRequest")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LogDeliveryConfigurationRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LogDeliveryConfigurationRequest").build()).build()).build())
.build();
private static final SdkField IP_DISCOVERY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpDiscovery").getter(getter(ModifyCacheClusterRequest::ipDiscoveryAsString))
.setter(setter(Builder::ipDiscovery))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpDiscovery").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CACHE_CLUSTER_ID_FIELD,
NUM_CACHE_NODES_FIELD, CACHE_NODE_IDS_TO_REMOVE_FIELD, AZ_MODE_FIELD, NEW_AVAILABILITY_ZONES_FIELD,
CACHE_SECURITY_GROUP_NAMES_FIELD, SECURITY_GROUP_IDS_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD,
NOTIFICATION_TOPIC_ARN_FIELD, CACHE_PARAMETER_GROUP_NAME_FIELD, NOTIFICATION_TOPIC_STATUS_FIELD,
APPLY_IMMEDIATELY_FIELD, ENGINE_VERSION_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD,
SNAPSHOT_WINDOW_FIELD, CACHE_NODE_TYPE_FIELD, AUTH_TOKEN_FIELD, AUTH_TOKEN_UPDATE_STRATEGY_FIELD,
LOG_DELIVERY_CONFIGURATIONS_FIELD, IP_DISCOVERY_FIELD));
private final String cacheClusterId;
private final Integer numCacheNodes;
private final List cacheNodeIdsToRemove;
private final String azMode;
private final List newAvailabilityZones;
private final List cacheSecurityGroupNames;
private final List securityGroupIds;
private final String preferredMaintenanceWindow;
private final String notificationTopicArn;
private final String cacheParameterGroupName;
private final String notificationTopicStatus;
private final Boolean applyImmediately;
private final String engineVersion;
private final Boolean autoMinorVersionUpgrade;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final String cacheNodeType;
private final String authToken;
private final String authTokenUpdateStrategy;
private final List logDeliveryConfigurations;
private final String ipDiscovery;
private ModifyCacheClusterRequest(BuilderImpl builder) {
super(builder);
this.cacheClusterId = builder.cacheClusterId;
this.numCacheNodes = builder.numCacheNodes;
this.cacheNodeIdsToRemove = builder.cacheNodeIdsToRemove;
this.azMode = builder.azMode;
this.newAvailabilityZones = builder.newAvailabilityZones;
this.cacheSecurityGroupNames = builder.cacheSecurityGroupNames;
this.securityGroupIds = builder.securityGroupIds;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.notificationTopicArn = builder.notificationTopicArn;
this.cacheParameterGroupName = builder.cacheParameterGroupName;
this.notificationTopicStatus = builder.notificationTopicStatus;
this.applyImmediately = builder.applyImmediately;
this.engineVersion = builder.engineVersion;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.cacheNodeType = builder.cacheNodeType;
this.authToken = builder.authToken;
this.authTokenUpdateStrategy = builder.authTokenUpdateStrategy;
this.logDeliveryConfigurations = builder.logDeliveryConfigurations;
this.ipDiscovery = builder.ipDiscovery;
}
/**
*
* The cluster identifier. This value is stored as a lowercase string.
*
*
* @return The cluster identifier. This value is stored as a lowercase string.
*/
public final String cacheClusterId() {
return cacheClusterId;
}
/**
*
* The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is greater
* than the sum of the number of current cache nodes and the number of cache nodes pending creation (which may be
* zero), more nodes are added. If the value is less than the number of existing cache nodes, nodes are removed. If
* the value is equal to the number of current cache nodes, any pending add or remove requests are canceled.
*
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide the IDs
* of the specific cache nodes to remove.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be between 1
* and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window, whether by
* adding or removing nodes in accordance with the scale out architecture, is not queued. The customer's latest
* request to add or remove nodes to the cluster overrides any previous pending operations to modify the number of
* cache nodes in the cluster. For example, a request to remove 2 nodes would override a previous pending operation
* to remove 3 nodes. Similarly, a request to add 2 nodes would override a previous pending operation to remove 3
* nodes and vice versa. As Memcached cache nodes may now be provisioned in different Availability Zones with
* flexible cache node placement, a request to add nodes does not automatically override a previous pending
* operation to add nodes. The customer can modify the previous pending operation to add more nodes or explicitly
* cancel the pending request and retry the new request. To cancel pending operations to modify the number of cache
* nodes in a cluster, use the ModifyCacheCluster
request and set NumCacheNodes
equal to
* the number of cache nodes currently in the cluster.
*
*
*
* @return The number of cache nodes that the cluster should have. If the value for NumCacheNodes
is
* greater than the sum of the number of current cache nodes and the number of cache nodes pending creation
* (which may be zero), more nodes are added. If the value is less than the number of existing cache nodes,
* nodes are removed. If the value is equal to the number of current cache nodes, any pending add or remove
* requests are canceled.
*
* If you are removing cache nodes, you must use the CacheNodeIdsToRemove
parameter to provide
* the IDs of the specific cache nodes to remove.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*
*
*
* Adding or removing Memcached cache nodes can be applied immediately or as a pending operation (see
* ApplyImmediately
).
*
*
* A pending operation to modify the number of cache nodes in a cluster during its maintenance window,
* whether by adding or removing nodes in accordance with the scale out architecture, is not queued. The
* customer's latest request to add or remove nodes to the cluster overrides any previous pending operations
* to modify the number of cache nodes in the cluster. For example, a request to remove 2 nodes would
* override a previous pending operation to remove 3 nodes. Similarly, a request to add 2 nodes would
* override a previous pending operation to remove 3 nodes and vice versa. As Memcached cache nodes may now
* be provisioned in different Availability Zones with flexible cache node placement, a request to add nodes
* does not automatically override a previous pending operation to add nodes. The customer can modify the
* previous pending operation to add more nodes or explicitly cancel the pending request and retry the new
* request. To cancel pending operations to modify the number of cache nodes in a cluster, use the
* ModifyCacheCluster
request and set NumCacheNodes
equal to the number of cache
* nodes currently in the cluster.
*
*/
public final Integer numCacheNodes() {
return numCacheNodes;
}
/**
* For responses, this returns true if the service returned a value for the CacheNodeIdsToRemove property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCacheNodeIdsToRemove() {
return cacheNodeIdsToRemove != null && !(cacheNodeIdsToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This parameter is
* only valid when NumCacheNodes
is less than the existing number of cache nodes. The number of cache
* node IDs supplied in this parameter must match the difference between the existing number of cache nodes in the
* cluster or pending cache nodes, whichever is greater, and the value of NumCacheNodes
in the request.
*
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in this
* ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCacheNodeIdsToRemove} method.
*
*
* @return A list of cache node IDs to be removed. A node ID is a numeric identifier (0001, 0002, etc.). This
* parameter is only valid when NumCacheNodes
is less than the existing number of cache nodes.
* The number of cache node IDs supplied in this parameter must match the difference between the existing
* number of cache nodes in the cluster or pending cache nodes, whichever is greater, and the value of
* NumCacheNodes
in the request.
*
* For example: If you have 3 active cache nodes, 7 pending cache nodes, and the number of cache nodes in
* this ModifyCacheCluster
call is 5, you must list 2 (7 - 5) cache node IDs to remove.
*/
public final List cacheNodeIdsToRemove() {
return cacheNodeIdsToRemove;
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #azMode} will
* return {@link AZMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #azModeAsString}.
*
*
* @return Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone
* or created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @see AZMode
*/
public final AZMode azMode() {
return AZMode.fromValue(azMode);
}
/**
*
* Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone or
* created across multiple Availability Zones.
*
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their current
* Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #azMode} will
* return {@link AZMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #azModeAsString}.
*
*
* @return Specifies whether the new nodes in this Memcached cluster are all created in a single Availability Zone
* or created across multiple Availability Zones.
*
* Valid values: single-az
| cross-az
.
*
*
* This option is only supported for Memcached clusters.
*
*
*
* You cannot specify single-az
if the Memcached cluster already has cache nodes in different
* Availability Zones. If cross-az
is specified, existing Memcached nodes remain in their
* current Availability Zone.
*
*
* Only newly created nodes are located in different Availability Zones.
*
* @see AZMode
*/
public final String azModeAsString() {
return azMode;
}
/**
* For responses, this returns true if the service returned a value for the NewAvailabilityZones property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNewAvailabilityZones() {
return newAvailabilityZones != null && !(newAvailabilityZones instanceof SdkAutoConstructList);
}
/**
*
*
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of the number
* of active cache nodes and the number of cache nodes pending creation (which may be zero). The number of
* Availability Zones supplied in this list must match the cache nodes being added in this request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
(3 + 2)
* and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and want to
* add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an Availability Zone
* for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to cancel all
* pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any nodes
* pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability Zone. Only
* newly created nodes can be located in different Availability Zones. For guidance on how to move existing
* Memcached nodes to different Availability Zones, see the Availability Zone Considerations section of Cache Node
* Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNewAvailabilityZones} method.
*
*
* @return
* This option is only supported on Memcached clusters.
*
*
*
* The list of Availability Zones where the new Memcached cache nodes are created.
*
*
* This parameter is only valid when NumCacheNodes
in the request is greater than the sum of
* the number of active cache nodes and the number of cache nodes pending creation (which may be zero). The
* number of Availability Zones supplied in this list must match the cache nodes being added in this
* request.
*
*
* Scenarios:
*
*
* -
*
* Scenario 1: You have 3 active nodes and wish to add 2 nodes. Specify NumCacheNodes=5
* (3 + 2) and optionally specify two Availability Zones for the two new nodes.
*
*
* -
*
* Scenario 2: You have 3 active nodes and 2 nodes pending creation (from the scenario 1 call) and
* want to add 1 more node. Specify NumCacheNodes=6
((3 + 2) + 1) and optionally specify an
* Availability Zone for the new node.
*
*
* -
*
* Scenario 3: You want to cancel all pending operations. Specify NumCacheNodes=3
to
* cancel all pending operations.
*
*
*
*
* The Availability Zone placement of nodes pending creation cannot be modified. If you wish to cancel any
* nodes pending creation, add 0 nodes by setting NumCacheNodes
to the number of current nodes.
*
*
* If cross-az
is specified, existing Memcached nodes remain in their current Availability
* Zone. Only newly created nodes can be located in different Availability Zones. For guidance on how to
* move existing Memcached nodes to different Availability Zones, see the Availability Zone
* Considerations section of Cache
* Node Considerations for Memcached.
*
*
* Impact of new add/remove requests upon pending requests
*
*
* -
*
* Scenario-1
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-2
*
*
* -
*
* Pending Action: Delete
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create, pending or immediate, replaces the pending delete.
*
*
*
*
* -
*
* Scenario-3
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Delete
*
*
* -
*
* Result: The new delete, pending or immediate, replaces the pending create.
*
*
*
*
* -
*
* Scenario-4
*
*
* -
*
* Pending Action: Create
*
*
* -
*
* New Request: Create
*
*
* -
*
* Result: The new create is added to the pending create.
*
*
*
* Important: If the new create request is Apply Immediately - Yes, all creates are performed
* immediately. If the new create request is Apply Immediately - No, all creates are pending.
*
*
*
*
*/
public final List newAvailabilityZones() {
return newAvailabilityZones;
}
/**
* For responses, this returns true if the service returned a value for the CacheSecurityGroupNames property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCacheSecurityGroupNames() {
return cacheSecurityGroupNames != null && !(cacheSecurityGroupNames instanceof SdkAutoConstructList);
}
/**
*
* A list of cache security group names to authorize on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCacheSecurityGroupNames} method.
*
*
* @return A list of cache security group names to authorize on this cluster. This change is asynchronously applied
* as soon as possible.
*
* You can use this parameter only with clusters that are created outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*
*
* Constraints: Must contain no more than 255 alphanumeric characters. Must not be "Default".
*/
public final List cacheSecurityGroupNames() {
return cacheSecurityGroupNames;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* Specifies the VPC Security Groups associated with the cluster.
*
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return Specifies the VPC Security Groups associated with the cluster.
*
* This parameter can be used only with clusters that are created in an Amazon Virtual Private Cloud (Amazon
* VPC).
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
*
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications are sent.
*
* The Amazon SNS topic owner must be same as the cluster owner.
*
*/
public final String notificationTopicArn() {
return notificationTopicArn;
}
/**
*
* The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as soon as
* possible for parameters when the ApplyImmediately
parameter is specified as true
for
* this request.
*
*
* @return The name of the cache parameter group to apply to this cluster. This change is asynchronously applied as
* soon as possible for parameters when the ApplyImmediately
parameter is specified as
* true
for this request.
*/
public final String cacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
*
* The status of the Amazon SNS notification topic. Notifications are sent only if the status is active
* .
*
*
* Valid values: active
| inactive
*
*
* @return The status of the Amazon SNS notification topic. Notifications are sent only if the status is
* active
.
*
* Valid values: active
| inactive
*/
public final String notificationTopicStatus() {
return notificationTopicStatus;
}
/**
*
* If true
, this parameter causes the modifications in this request and any pending modifications to be
* applied, asynchronously and as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the cluster.
*
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next failure
* reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*
*
* @return If true
, this parameter causes the modifications in this request and any pending
* modifications to be applied, asynchronously and as soon as possible, regardless of the
* PreferredMaintenanceWindow
setting for the cluster.
*
* If false
, changes to the cluster are applied on the next maintenance reboot, or the next
* failure reboot, whichever occurs first.
*
*
*
* If you perform a ModifyCacheCluster
before a pending modification is applied, the pending
* modification is replaced by the newer modification.
*
*
*
* Valid values: true
| false
*
*
* Default: false
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
/**
*
* The upgraded version of the cache engine to be run on the cache nodes.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster and create it anew with the earlier engine version.
*
*
* @return The upgraded version of the cache engine to be run on the cache nodes.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster and create it anew with the
* earlier engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* If you are running Redis engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis engine version 6.0 or later, set this parameter to yes if you want to opt-in to
* the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A valid cache node type that you want to scale this cluster up to.
*
*
* @return A valid cache node type that you want to scale this cluster up to.
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* Reserved parameter. The password used to access a password protected server. This parameter must be specified
* with the auth-token-update
parameter. Password constraints:
*
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server. This parameter must be
* specified with the auth-token-update
parameter. Password constraints:
*
* -
*
* Must be only printable ASCII characters
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@', '%'
*
*
*
*
* For more information, see AUTH password at AUTH.
*/
public final String authToken() {
return authToken;
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with Redis
* AUTH
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authTokenUpdateStrategy} will return {@link AuthTokenUpdateStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #authTokenUpdateStrategyAsString}.
*
*
* @return Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with
* Redis AUTH
* @see AuthTokenUpdateStrategyType
*/
public final AuthTokenUpdateStrategyType authTokenUpdateStrategy() {
return AuthTokenUpdateStrategyType.fromValue(authTokenUpdateStrategy);
}
/**
*
* Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with Redis
* AUTH
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authTokenUpdateStrategy} will return {@link AuthTokenUpdateStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #authTokenUpdateStrategyAsString}.
*
*
* @return Specifies the strategy to use to update the AUTH token. This parameter must be specified with the
* auth-token
parameter. Possible values:
*
* -
*
* Rotate
*
*
* -
*
* Set
*
*
*
*
* For more information, see Authenticating Users with
* Redis AUTH
* @see AuthTokenUpdateStrategyType
*/
public final String authTokenUpdateStrategyAsString() {
return authTokenUpdateStrategy;
}
/**
* For responses, this returns true if the service returned a value for the LogDeliveryConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogDeliveryConfigurations() {
return logDeliveryConfigurations != null && !(logDeliveryConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogDeliveryConfigurations} method.
*
*
* @return Specifies the destination, format and type of the logs.
*/
public final List logDeliveryConfigurations() {
return logDeliveryConfigurations;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
* @see IpDiscovery
*/
public final IpDiscovery ipDiscovery() {
return IpDiscovery.fromValue(ipDiscovery);
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
* @see IpDiscovery
*/
public final String ipDiscoveryAsString() {
return ipDiscovery;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(numCacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(hasCacheNodeIdsToRemove() ? cacheNodeIdsToRemove() : null);
hashCode = 31 * hashCode + Objects.hashCode(azModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasNewAvailabilityZones() ? newAvailabilityZones() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicStatus());
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(authToken());
hashCode = 31 * hashCode + Objects.hashCode(authTokenUpdateStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(ipDiscoveryAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyCacheClusterRequest)) {
return false;
}
ModifyCacheClusterRequest other = (ModifyCacheClusterRequest) obj;
return Objects.equals(cacheClusterId(), other.cacheClusterId()) && Objects.equals(numCacheNodes(), other.numCacheNodes())
&& hasCacheNodeIdsToRemove() == other.hasCacheNodeIdsToRemove()
&& Objects.equals(cacheNodeIdsToRemove(), other.cacheNodeIdsToRemove())
&& Objects.equals(azModeAsString(), other.azModeAsString())
&& hasNewAvailabilityZones() == other.hasNewAvailabilityZones()
&& Objects.equals(newAvailabilityZones(), other.newAvailabilityZones())
&& hasCacheSecurityGroupNames() == other.hasCacheSecurityGroupNames()
&& Objects.equals(cacheSecurityGroupNames(), other.cacheSecurityGroupNames())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(notificationTopicArn(), other.notificationTopicArn())
&& Objects.equals(cacheParameterGroupName(), other.cacheParameterGroupName())
&& Objects.equals(notificationTopicStatus(), other.notificationTopicStatus())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(authToken(), other.authToken())
&& Objects.equals(authTokenUpdateStrategyAsString(), other.authTokenUpdateStrategyAsString())
&& hasLogDeliveryConfigurations() == other.hasLogDeliveryConfigurations()
&& Objects.equals(logDeliveryConfigurations(), other.logDeliveryConfigurations())
&& Objects.equals(ipDiscoveryAsString(), other.ipDiscoveryAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyCacheClusterRequest").add("CacheClusterId", cacheClusterId())
.add("NumCacheNodes", numCacheNodes())
.add("CacheNodeIdsToRemove", hasCacheNodeIdsToRemove() ? cacheNodeIdsToRemove() : null)
.add("AZMode", azModeAsString())
.add("NewAvailabilityZones", hasNewAvailabilityZones() ? newAvailabilityZones() : null)
.add("CacheSecurityGroupNames", hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null)
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("NotificationTopicArn", notificationTopicArn()).add("CacheParameterGroupName", cacheParameterGroupName())
.add("NotificationTopicStatus", notificationTopicStatus()).add("ApplyImmediately", applyImmediately())
.add("EngineVersion", engineVersion()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("CacheNodeType", cacheNodeType()).add("AuthToken", authToken())
.add("AuthTokenUpdateStrategy", authTokenUpdateStrategyAsString())
.add("LogDeliveryConfigurations", hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null)
.add("IpDiscovery", ipDiscoveryAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "NumCacheNodes":
return Optional.ofNullable(clazz.cast(numCacheNodes()));
case "CacheNodeIdsToRemove":
return Optional.ofNullable(clazz.cast(cacheNodeIdsToRemove()));
case "AZMode":
return Optional.ofNullable(clazz.cast(azModeAsString()));
case "NewAvailabilityZones":
return Optional.ofNullable(clazz.cast(newAvailabilityZones()));
case "CacheSecurityGroupNames":
return Optional.ofNullable(clazz.cast(cacheSecurityGroupNames()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "NotificationTopicArn":
return Optional.ofNullable(clazz.cast(notificationTopicArn()));
case "CacheParameterGroupName":
return Optional.ofNullable(clazz.cast(cacheParameterGroupName()));
case "NotificationTopicStatus":
return Optional.ofNullable(clazz.cast(notificationTopicStatus()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "AuthToken":
return Optional.ofNullable(clazz.cast(authToken()));
case "AuthTokenUpdateStrategy":
return Optional.ofNullable(clazz.cast(authTokenUpdateStrategyAsString()));
case "LogDeliveryConfigurations":
return Optional.ofNullable(clazz.cast(logDeliveryConfigurations()));
case "IpDiscovery":
return Optional.ofNullable(clazz.cast(ipDiscoveryAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function