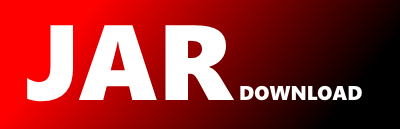
software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a CreateReplicationGroup
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateReplicationGroupRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(CreateReplicationGroupRequest::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField REPLICATION_GROUP_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationGroupDescription")
.getter(getter(CreateReplicationGroupRequest::replicationGroupDescription))
.setter(setter(Builder::replicationGroupDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupDescription")
.build()).build();
private static final SdkField GLOBAL_REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GlobalReplicationGroupId").getter(getter(CreateReplicationGroupRequest::globalReplicationGroupId))
.setter(setter(Builder::globalReplicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GlobalReplicationGroupId").build())
.build();
private static final SdkField PRIMARY_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PrimaryClusterId").getter(getter(CreateReplicationGroupRequest::primaryClusterId))
.setter(setter(Builder::primaryClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrimaryClusterId").build()).build();
private static final SdkField AUTOMATIC_FAILOVER_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutomaticFailoverEnabled").getter(getter(CreateReplicationGroupRequest::automaticFailoverEnabled))
.setter(setter(Builder::automaticFailoverEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailoverEnabled").build())
.build();
private static final SdkField MULTI_AZ_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZEnabled").getter(getter(CreateReplicationGroupRequest::multiAZEnabled))
.setter(setter(Builder::multiAZEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZEnabled").build()).build();
private static final SdkField NUM_CACHE_CLUSTERS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumCacheClusters").getter(getter(CreateReplicationGroupRequest::numCacheClusters))
.setter(setter(Builder::numCacheClusters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheClusters").build()).build();
private static final SdkField> PREFERRED_CACHE_CLUSTER_A_ZS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PreferredCacheClusterAZs")
.getter(getter(CreateReplicationGroupRequest::preferredCacheClusterAZs))
.setter(setter(Builder::preferredCacheClusterAZs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredCacheClusterAZs").build(),
ListTrait
.builder()
.memberLocationName("AvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AvailabilityZone").build()).build()).build()).build();
private static final SdkField NUM_NODE_GROUPS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumNodeGroups").getter(getter(CreateReplicationGroupRequest::numNodeGroups))
.setter(setter(Builder::numNodeGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumNodeGroups").build()).build();
private static final SdkField REPLICAS_PER_NODE_GROUP_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ReplicasPerNodeGroup").getter(getter(CreateReplicationGroupRequest::replicasPerNodeGroup))
.setter(setter(Builder::replicasPerNodeGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicasPerNodeGroup").build())
.build();
private static final SdkField> NODE_GROUP_CONFIGURATION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NodeGroupConfiguration")
.getter(getter(CreateReplicationGroupRequest::nodeGroupConfiguration))
.setter(setter(Builder::nodeGroupConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupConfiguration").build(),
ListTrait
.builder()
.memberLocationName("NodeGroupConfiguration")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NodeGroupConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroupConfiguration").build()).build()).build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(CreateReplicationGroupRequest::cacheNodeType))
.setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(CreateReplicationGroupRequest::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(CreateReplicationGroupRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField CACHE_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheParameterGroupName").getter(getter(CreateReplicationGroupRequest::cacheParameterGroupName))
.setter(setter(Builder::cacheParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroupName").build())
.build();
private static final SdkField CACHE_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheSubnetGroupName").getter(getter(CreateReplicationGroupRequest::cacheSubnetGroupName))
.setter(setter(Builder::cacheSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSubnetGroupName").build())
.build();
private static final SdkField> CACHE_SECURITY_GROUP_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheSecurityGroupNames")
.getter(getter(CreateReplicationGroupRequest::cacheSecurityGroupNames))
.setter(setter(Builder::cacheSecurityGroupNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroupNames").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroupName").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(CreateReplicationGroupRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("SecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SecurityGroupId").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateReplicationGroupRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final SdkField> SNAPSHOT_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SnapshotArns")
.getter(getter(CreateReplicationGroupRequest::snapshotArns))
.setter(setter(Builder::snapshotArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotArns").build(),
ListTrait
.builder()
.memberLocationName("SnapshotArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SnapshotArn").build()).build()).build()).build();
private static final SdkField SNAPSHOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotName").getter(getter(CreateReplicationGroupRequest::snapshotName))
.setter(setter(Builder::snapshotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotName").build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(CreateReplicationGroupRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(CreateReplicationGroupRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField NOTIFICATION_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotificationTopicArn").getter(getter(CreateReplicationGroupRequest::notificationTopicArn))
.setter(setter(Builder::notificationTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicArn").build())
.build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(CreateReplicationGroupRequest::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(CreateReplicationGroupRequest::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(CreateReplicationGroupRequest::snapshotWindow))
.setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField AUTH_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthToken").getter(getter(CreateReplicationGroupRequest::authToken)).setter(setter(Builder::authToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthToken").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("TransitEncryptionEnabled").getter(getter(CreateReplicationGroupRequest::transitEncryptionEnabled))
.setter(setter(Builder::transitEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionEnabled").build())
.build();
private static final SdkField AT_REST_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AtRestEncryptionEnabled").getter(getter(CreateReplicationGroupRequest::atRestEncryptionEnabled))
.setter(setter(Builder::atRestEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AtRestEncryptionEnabled").build())
.build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(CreateReplicationGroupRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField> USER_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroupIds")
.getter(getter(CreateReplicationGroupRequest::userGroupIds))
.setter(setter(Builder::userGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOG_DELIVERY_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LogDeliveryConfigurations")
.getter(getter(CreateReplicationGroupRequest::logDeliveryConfigurations))
.setter(setter(Builder::logDeliveryConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDeliveryConfigurations").build(),
ListTrait
.builder()
.memberLocationName("LogDeliveryConfigurationRequest")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LogDeliveryConfigurationRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LogDeliveryConfigurationRequest").build()).build()).build())
.build();
private static final SdkField DATA_TIERING_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DataTieringEnabled").getter(getter(CreateReplicationGroupRequest::dataTieringEnabled))
.setter(setter(Builder::dataTieringEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataTieringEnabled").build())
.build();
private static final SdkField NETWORK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetworkType").getter(getter(CreateReplicationGroupRequest::networkTypeAsString))
.setter(setter(Builder::networkType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkType").build()).build();
private static final SdkField IP_DISCOVERY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpDiscovery").getter(getter(CreateReplicationGroupRequest::ipDiscoveryAsString))
.setter(setter(Builder::ipDiscovery))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpDiscovery").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TransitEncryptionMode").getter(getter(CreateReplicationGroupRequest::transitEncryptionModeAsString))
.setter(setter(Builder::transitEncryptionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionMode").build())
.build();
private static final SdkField CLUSTER_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterMode").getter(getter(CreateReplicationGroupRequest::clusterModeAsString))
.setter(setter(Builder::clusterMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterMode").build()).build();
private static final SdkField SERVERLESS_CACHE_SNAPSHOT_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ServerlessCacheSnapshotName")
.getter(getter(CreateReplicationGroupRequest::serverlessCacheSnapshotName))
.setter(setter(Builder::serverlessCacheSnapshotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerlessCacheSnapshotName")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
REPLICATION_GROUP_DESCRIPTION_FIELD, GLOBAL_REPLICATION_GROUP_ID_FIELD, PRIMARY_CLUSTER_ID_FIELD,
AUTOMATIC_FAILOVER_ENABLED_FIELD, MULTI_AZ_ENABLED_FIELD, NUM_CACHE_CLUSTERS_FIELD,
PREFERRED_CACHE_CLUSTER_A_ZS_FIELD, NUM_NODE_GROUPS_FIELD, REPLICAS_PER_NODE_GROUP_FIELD,
NODE_GROUP_CONFIGURATION_FIELD, CACHE_NODE_TYPE_FIELD, ENGINE_FIELD, ENGINE_VERSION_FIELD,
CACHE_PARAMETER_GROUP_NAME_FIELD, CACHE_SUBNET_GROUP_NAME_FIELD, CACHE_SECURITY_GROUP_NAMES_FIELD,
SECURITY_GROUP_IDS_FIELD, TAGS_FIELD, SNAPSHOT_ARNS_FIELD, SNAPSHOT_NAME_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD,
PORT_FIELD, NOTIFICATION_TOPIC_ARN_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD,
SNAPSHOT_WINDOW_FIELD, AUTH_TOKEN_FIELD, TRANSIT_ENCRYPTION_ENABLED_FIELD, AT_REST_ENCRYPTION_ENABLED_FIELD,
KMS_KEY_ID_FIELD, USER_GROUP_IDS_FIELD, LOG_DELIVERY_CONFIGURATIONS_FIELD, DATA_TIERING_ENABLED_FIELD,
NETWORK_TYPE_FIELD, IP_DISCOVERY_FIELD, TRANSIT_ENCRYPTION_MODE_FIELD, CLUSTER_MODE_FIELD,
SERVERLESS_CACHE_SNAPSHOT_NAME_FIELD));
private final String replicationGroupId;
private final String replicationGroupDescription;
private final String globalReplicationGroupId;
private final String primaryClusterId;
private final Boolean automaticFailoverEnabled;
private final Boolean multiAZEnabled;
private final Integer numCacheClusters;
private final List preferredCacheClusterAZs;
private final Integer numNodeGroups;
private final Integer replicasPerNodeGroup;
private final List nodeGroupConfiguration;
private final String cacheNodeType;
private final String engine;
private final String engineVersion;
private final String cacheParameterGroupName;
private final String cacheSubnetGroupName;
private final List cacheSecurityGroupNames;
private final List securityGroupIds;
private final List tags;
private final List snapshotArns;
private final String snapshotName;
private final String preferredMaintenanceWindow;
private final Integer port;
private final String notificationTopicArn;
private final Boolean autoMinorVersionUpgrade;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final String authToken;
private final Boolean transitEncryptionEnabled;
private final Boolean atRestEncryptionEnabled;
private final String kmsKeyId;
private final List userGroupIds;
private final List logDeliveryConfigurations;
private final Boolean dataTieringEnabled;
private final String networkType;
private final String ipDiscovery;
private final String transitEncryptionMode;
private final String clusterMode;
private final String serverlessCacheSnapshotName;
private CreateReplicationGroupRequest(BuilderImpl builder) {
super(builder);
this.replicationGroupId = builder.replicationGroupId;
this.replicationGroupDescription = builder.replicationGroupDescription;
this.globalReplicationGroupId = builder.globalReplicationGroupId;
this.primaryClusterId = builder.primaryClusterId;
this.automaticFailoverEnabled = builder.automaticFailoverEnabled;
this.multiAZEnabled = builder.multiAZEnabled;
this.numCacheClusters = builder.numCacheClusters;
this.preferredCacheClusterAZs = builder.preferredCacheClusterAZs;
this.numNodeGroups = builder.numNodeGroups;
this.replicasPerNodeGroup = builder.replicasPerNodeGroup;
this.nodeGroupConfiguration = builder.nodeGroupConfiguration;
this.cacheNodeType = builder.cacheNodeType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.cacheParameterGroupName = builder.cacheParameterGroupName;
this.cacheSubnetGroupName = builder.cacheSubnetGroupName;
this.cacheSecurityGroupNames = builder.cacheSecurityGroupNames;
this.securityGroupIds = builder.securityGroupIds;
this.tags = builder.tags;
this.snapshotArns = builder.snapshotArns;
this.snapshotName = builder.snapshotName;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.port = builder.port;
this.notificationTopicArn = builder.notificationTopicArn;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.authToken = builder.authToken;
this.transitEncryptionEnabled = builder.transitEncryptionEnabled;
this.atRestEncryptionEnabled = builder.atRestEncryptionEnabled;
this.kmsKeyId = builder.kmsKeyId;
this.userGroupIds = builder.userGroupIds;
this.logDeliveryConfigurations = builder.logDeliveryConfigurations;
this.dataTieringEnabled = builder.dataTieringEnabled;
this.networkType = builder.networkType;
this.ipDiscovery = builder.ipDiscovery;
this.transitEncryptionMode = builder.transitEncryptionMode;
this.clusterMode = builder.clusterMode;
this.serverlessCacheSnapshotName = builder.serverlessCacheSnapshotName;
}
/**
*
* The replication group identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 40 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The replication group identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 40 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* A user-created description for the replication group.
*
*
* @return A user-created description for the replication group.
*/
public final String replicationGroupDescription() {
return replicationGroupDescription;
}
/**
*
* The name of the Global datastore
*
*
* @return The name of the Global datastore
*/
public final String globalReplicationGroupId() {
return globalReplicationGroupId;
}
/**
*
* The identifier of the cluster that serves as the primary for this replication group. This cluster must already
* exist and have a status of available
.
*
*
* This parameter is not required if NumCacheClusters
, NumNodeGroups
, or
* ReplicasPerNodeGroup
is specified.
*
*
* @return The identifier of the cluster that serves as the primary for this replication group. This cluster must
* already exist and have a status of available
.
*
* This parameter is not required if NumCacheClusters
, NumNodeGroups
, or
* ReplicasPerNodeGroup
is specified.
*/
public final String primaryClusterId() {
return primaryClusterId;
}
/**
*
* Specifies whether a read-only replica is automatically promoted to read/write primary if the existing primary
* fails.
*
*
* AutomaticFailoverEnabled
must be enabled for Redis (cluster mode enabled) replication groups.
*
*
* Default: false
*
*
* @return Specifies whether a read-only replica is automatically promoted to read/write primary if the existing
* primary fails.
*
* AutomaticFailoverEnabled
must be enabled for Redis (cluster mode enabled) replication
* groups.
*
*
* Default: false
*/
public final Boolean automaticFailoverEnabled() {
return automaticFailoverEnabled;
}
/**
*
* A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ.
*
*
* @return A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ.
*/
public final Boolean multiAZEnabled() {
return multiAZEnabled;
}
/**
*
* The number of clusters this replication group initially has.
*
*
* This parameter is not used if there is more than one node group (shard). You should use
* ReplicasPerNodeGroup
instead.
*
*
* If AutomaticFailoverEnabled
is true
, the value of this parameter must be at least 2. If
* AutomaticFailoverEnabled
is false
you can omit this parameter (it will default to 1),
* or you can explicitly set it to a value between 2 and 6.
*
*
* The maximum permitted value for NumCacheClusters
is 6 (1 primary plus 5 replicas).
*
*
* @return The number of clusters this replication group initially has.
*
* This parameter is not used if there is more than one node group (shard). You should use
* ReplicasPerNodeGroup
instead.
*
*
* If AutomaticFailoverEnabled
is true
, the value of this parameter must be at
* least 2. If AutomaticFailoverEnabled
is false
you can omit this parameter (it
* will default to 1), or you can explicitly set it to a value between 2 and 6.
*
*
* The maximum permitted value for NumCacheClusters
is 6 (1 primary plus 5 replicas).
*/
public final Integer numCacheClusters() {
return numCacheClusters;
}
/**
* For responses, this returns true if the service returned a value for the PreferredCacheClusterAZs property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasPreferredCacheClusterAZs() {
return preferredCacheClusterAZs != null && !(preferredCacheClusterAZs instanceof SdkAutoConstructList);
}
/**
*
* A list of EC2 Availability Zones in which the replication group's clusters are created. The order of the
* Availability Zones in the list is the order in which clusters are allocated. The primary cluster is created in
* the first AZ in the list.
*
*
* This parameter is not used if there is more than one node group (shard). You should use
* NodeGroupConfiguration
instead.
*
*
*
* If you are creating your replication group in an Amazon VPC (recommended), you can only locate clusters in
* Availability Zones associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheClusters
.
*
*
*
* Default: system chosen Availability Zones.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPreferredCacheClusterAZs} method.
*
*
* @return A list of EC2 Availability Zones in which the replication group's clusters are created. The order of the
* Availability Zones in the list is the order in which clusters are allocated. The primary cluster is
* created in the first AZ in the list.
*
* This parameter is not used if there is more than one node group (shard). You should use
* NodeGroupConfiguration
instead.
*
*
*
* If you are creating your replication group in an Amazon VPC (recommended), you can only locate clusters
* in Availability Zones associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheClusters
.
*
*
*
* Default: system chosen Availability Zones.
*/
public final List preferredCacheClusterAZs() {
return preferredCacheClusterAZs;
}
/**
*
* An optional parameter that specifies the number of node groups (shards) for this Redis (cluster mode enabled)
* replication group. For Redis (cluster mode disabled) either omit this parameter or set it to 1.
*
*
* Default: 1
*
*
* @return An optional parameter that specifies the number of node groups (shards) for this Redis (cluster mode
* enabled) replication group. For Redis (cluster mode disabled) either omit this parameter or set it to
* 1.
*
* Default: 1
*/
public final Integer numNodeGroups() {
return numNodeGroups;
}
/**
*
* An optional parameter that specifies the number of replica nodes in each node group (shard). Valid values are 0
* to 5.
*
*
* @return An optional parameter that specifies the number of replica nodes in each node group (shard). Valid values
* are 0 to 5.
*/
public final Integer replicasPerNodeGroup() {
return replicasPerNodeGroup;
}
/**
* For responses, this returns true if the service returned a value for the NodeGroupConfiguration property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasNodeGroupConfiguration() {
return nodeGroupConfiguration != null && !(nodeGroupConfiguration instanceof SdkAutoConstructList);
}
/**
*
* A list of node group (shard) configuration options. Each node group (shard) configuration has the following
* members: PrimaryAvailabilityZone
, ReplicaAvailabilityZones
, ReplicaCount
,
* and Slots
.
*
*
* If you're creating a Redis (cluster mode disabled) or a Redis (cluster mode enabled) replication group, you can
* use this parameter to individually configure each node group (shard), or you can omit this parameter. However, it
* is required when seeding a Redis (cluster mode enabled) cluster from a S3 rdb file. You must configure each node
* group (shard) using this parameter because you must specify the slots for each node group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNodeGroupConfiguration} method.
*
*
* @return A list of node group (shard) configuration options. Each node group (shard) configuration has the
* following members: PrimaryAvailabilityZone
, ReplicaAvailabilityZones
,
* ReplicaCount
, and Slots
.
*
* If you're creating a Redis (cluster mode disabled) or a Redis (cluster mode enabled) replication group,
* you can use this parameter to individually configure each node group (shard), or you can omit this
* parameter. However, it is required when seeding a Redis (cluster mode enabled) cluster from a S3 rdb
* file. You must configure each node group (shard) using this parameter because you must specify the slots
* for each node group.
*/
public final List nodeGroupConfiguration() {
return nodeGroupConfiguration;
}
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis engine version 5.0.6 onward and Memcached engine version 1.5.16
* onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis configuration variables appendonly
and appendfsync
are not supported on Redis
* version 2.8.22 and later.
*
*
*
*
* @return The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis configuration variables appendonly
and appendfsync
are not supported on
* Redis version 2.8.22 and later.
*
*
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The name of the cache engine to be used for the clusters in this replication group. The value must be set to
* Redis
.
*
*
* @return The name of the cache engine to be used for the clusters in this replication group. The value must be set
* to Redis
.
*/
public final String engine() {
return engine;
}
/**
*
* The version number of the cache engine to be used for the clusters in this replication group. To view the
* supported cache engine versions, use the DescribeCacheEngineVersions
operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting
* a Cache Engine and Version) in the ElastiCache User Guide, but you cannot downgrade to an earlier
* engine version. If you want to use an earlier engine version, you must delete the existing cluster or replication
* group and create it anew with the earlier engine version.
*
*
* @return The version number of the cache engine to be used for the clusters in this replication group. To view the
* supported cache engine versions, use the DescribeCacheEngineVersions
operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version) in the ElastiCache User Guide, but you cannot downgrade
* to an earlier engine version. If you want to use an earlier engine version, you must delete the existing
* cluster or replication group and create it anew with the earlier engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The name of the parameter group to associate with this replication group. If this argument is omitted, the
* default cache parameter group for the specified engine is used.
*
*
* If you are running Redis version 3.2.4 or later, only one node group (shard), and want to use a default parameter
* group, we recommend that you specify the parameter group by name.
*
*
* -
*
* To create a Redis (cluster mode disabled) replication group, use
* CacheParameterGroupName=default.redis3.2
.
*
*
* -
*
* To create a Redis (cluster mode enabled) replication group, use
* CacheParameterGroupName=default.redis3.2.cluster.on
.
*
*
*
*
* @return The name of the parameter group to associate with this replication group. If this argument is omitted,
* the default cache parameter group for the specified engine is used.
*
* If you are running Redis version 3.2.4 or later, only one node group (shard), and want to use a default
* parameter group, we recommend that you specify the parameter group by name.
*
*
* -
*
* To create a Redis (cluster mode disabled) replication group, use
* CacheParameterGroupName=default.redis3.2
.
*
*
* -
*
* To create a Redis (cluster mode enabled) replication group, use
* CacheParameterGroupName=default.redis3.2.cluster.on
.
*
*
*/
public final String cacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
*
* The name of the cache subnet group to be used for the replication group.
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @return The name of the cache subnet group to be used for the replication group.
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*/
public final String cacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
* For responses, this returns true if the service returned a value for the CacheSecurityGroupNames property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCacheSecurityGroupNames() {
return cacheSecurityGroupNames != null && !(cacheSecurityGroupNames instanceof SdkAutoConstructList);
}
/**
*
* A list of cache security group names to associate with this replication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCacheSecurityGroupNames} method.
*
*
* @return A list of cache security group names to associate with this replication group.
*/
public final List cacheSecurityGroupNames() {
return cacheSecurityGroupNames;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* One or more Amazon VPC security groups associated with this replication group.
*
*
* Use this parameter only when you are creating a replication group in an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return One or more Amazon VPC security groups associated with this replication group.
*
* Use this parameter only when you are creating a replication group in an Amazon Virtual Private Cloud
* (Amazon VPC).
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags to be added to this resource. Tags are comma-separated key,value pairs (e.g. Key=
* myKey
, Value=myKeyValue
. You can include multiple tags as shown following: Key=
* myKey
, Value=myKeyValue
Key=mySecondKey
, Value=
* mySecondKeyValue
. Tags on replication groups will be replicated to all nodes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags to be added to this resource. Tags are comma-separated key,value pairs (e.g. Key=
* myKey
, Value=myKeyValue
. You can include multiple tags as shown following: Key=
* myKey
, Value=myKeyValue
Key=mySecondKey
, Value=
* mySecondKeyValue
. Tags on replication groups will be replicated to all nodes.
*/
public final List tags() {
return tags;
}
/**
* For responses, this returns true if the service returned a value for the SnapshotArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSnapshotArns() {
return snapshotArns != null && !(snapshotArns instanceof SdkAutoConstructList);
}
/**
*
* A list of Amazon Resource Names (ARN) that uniquely identify the Redis RDB snapshot files stored in Amazon S3.
* The snapshot files are used to populate the new replication group. The Amazon S3 object name in the ARN cannot
* contain any commas. The new replication group will have the number of node groups (console: shards) specified by
* the parameter NumNodeGroups or the number of node groups configured by NodeGroupConfiguration
* regardless of the number of ARNs specified here.
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSnapshotArns} method.
*
*
* @return A list of Amazon Resource Names (ARN) that uniquely identify the Redis RDB snapshot files stored in
* Amazon S3. The snapshot files are used to populate the new replication group. The Amazon S3 object name
* in the ARN cannot contain any commas. The new replication group will have the number of node groups
* (console: shards) specified by the parameter NumNodeGroups or the number of node groups configured
* by NodeGroupConfiguration regardless of the number of ARNs specified here.
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public final List snapshotArns() {
return snapshotArns;
}
/**
*
* The name of a snapshot from which to restore data into the new replication group. The snapshot status changes to
* restoring
while the new replication group is being created.
*
*
* @return The name of a snapshot from which to restore data into the new replication group. The snapshot status
* changes to restoring
while the new replication group is being created.
*/
public final String snapshotName() {
return snapshotName;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The port number on which each member of the replication group accepts connections.
*
*
* @return The port number on which each member of the replication group accepts connections.
*/
public final Integer port() {
return port;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*/
public final String notificationTopicArn() {
return notificationTopicArn;
}
/**
*
* If you are running Redis engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis engine version 6.0 or later, set this parameter to yes if you want to opt-in to
* the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days before being
* deleted.
*
*
* Default: 0 (i.e., automatic backups are disabled for this cluster).
*
*
* @return The number of days for which ElastiCache retains automatic snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5
* days before being deleted.
*
* Default: 0 (i.e., automatic backups are disabled for this cluster).
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* AuthToken
can be specified only on replication groups where TransitEncryptionEnabled
is
* true
.
*
*
*
* For HIPAA compliance, you must specify TransitEncryptionEnabled
as true
, an
* AuthToken
, and a CacheSubnetGroup
.
*
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable special
* characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server.
*
* AuthToken
can be specified only on replication groups where
* TransitEncryptionEnabled
is true
.
*
*
*
* For HIPAA compliance, you must specify TransitEncryptionEnabled
as true
, an
* AuthToken
, and a CacheSubnetGroup
.
*
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* The only permitted printable special characters are !, &, #, $, ^, <, >, and -. Other printable
* special characters cannot be used in the AUTH token.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*/
public final String authToken() {
return authToken;
}
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* This parameter is valid only if the Engine
parameter is redis
, the
* EngineVersion
parameter is 3.2.6
, 4.x
or later, and the cluster is being
* created in an Amazon VPC.
*
*
* If you enable in-transit encryption, you must also specify a value for CacheSubnetGroup
.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
*
* For HIPAA compliance, you must specify TransitEncryptionEnabled
as true
, an
* AuthToken
, and a CacheSubnetGroup
.
*
*
*
* @return A flag that enables in-transit encryption when set to true
.
*
* This parameter is valid only if the Engine
parameter is redis
, the
* EngineVersion
parameter is 3.2.6
, 4.x
or later, and the cluster is
* being created in an Amazon VPC.
*
*
* If you enable in-transit encryption, you must also specify a value for CacheSubnetGroup
.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
*
* For HIPAA compliance, you must specify TransitEncryptionEnabled
as true
, an
* AuthToken
, and a CacheSubnetGroup
.
*
*/
public final Boolean transitEncryptionEnabled() {
return transitEncryptionEnabled;
}
/**
*
* A flag that enables encryption at rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the replication group is created. To
* enable encryption at rest on a replication group you must set AtRestEncryptionEnabled
to
* true
when you create the replication group.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables encryption at rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the replication group is
* created. To enable encryption at rest on a replication group you must set
* AtRestEncryptionEnabled
to true
when you create the replication group.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public final Boolean atRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
/**
*
* The ID of the KMS key used to encrypt the disk in the cluster.
*
*
* @return The ID of the KMS key used to encrypt the disk in the cluster.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
* For responses, this returns true if the service returned a value for the UserGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasUserGroupIds() {
return userGroupIds != null && !(userGroupIds instanceof SdkAutoConstructList);
}
/**
*
* The user group to associate with the replication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserGroupIds} method.
*
*
* @return The user group to associate with the replication group.
*/
public final List userGroupIds() {
return userGroupIds;
}
/**
* For responses, this returns true if the service returned a value for the LogDeliveryConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogDeliveryConfigurations() {
return logDeliveryConfigurations != null && !(logDeliveryConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Specifies the destination, format and type of the logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogDeliveryConfigurations} method.
*
*
* @return Specifies the destination, format and type of the logs.
*/
public final List logDeliveryConfigurations() {
return logDeliveryConfigurations;
}
/**
*
* Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type. This
* parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*
*
* @return Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type.
* This parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*/
public final Boolean dataTieringEnabled() {
return dataTieringEnabled;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built
* on the Nitro system.
* @see NetworkType
*/
public final NetworkType networkType() {
return NetworkType.fromValue(networkType);
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built
* on the Nitro system.
* @see NetworkType
*/
public final String networkTypeAsString() {
return networkType;
}
/**
*
* The network type you choose when creating a replication group, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when creating a replication group, either ipv4
|
* ipv6
. IPv6 is supported for workloads using Redis engine version 6.2 onward or Memcached
* engine version 1.6.6 on all instances built on the Nitro
* system.
* @see IpDiscovery
*/
public final IpDiscovery ipDiscovery() {
return IpDiscovery.fromValue(ipDiscovery);
}
/**
*
* The network type you choose when creating a replication group, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when creating a replication group, either ipv4
|
* ipv6
. IPv6 is supported for workloads using Redis engine version 6.2 onward or Memcached
* engine version 1.6.6 on all instances built on the Nitro
* system.
* @see IpDiscovery
*/
public final String ipDiscoveryAsString() {
return ipDiscovery;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* When setting TransitEncryptionEnabled
to true
, you can set your
* TransitEncryptionMode
to preferred
in the same request, to allow both encrypted and
* unencrypted connections at the same time. Once you migrate all your Redis clients to use encrypted connections
* you can modify the value to required
to allow encrypted connections only.
*
*
* Setting TransitEncryptionMode
to required
is a two-step process that requires you to
* first set the TransitEncryptionMode
to preferred
, after that you can set
* TransitEncryptionMode
to required
.
*
*
* This process will not trigger the replacement of the replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
* When setting TransitEncryptionEnabled
to true
, you can set your
* TransitEncryptionMode
to preferred
in the same request, to allow both encrypted
* and unencrypted connections at the same time. Once you migrate all your Redis clients to use encrypted
* connections you can modify the value to required
to allow encrypted connections only.
*
*
* Setting TransitEncryptionMode
to required
is a two-step process that requires
* you to first set the TransitEncryptionMode
to preferred
, after that you can set
* TransitEncryptionMode
to required
.
*
*
* This process will not trigger the replacement of the replication group.
* @see TransitEncryptionMode
*/
public final TransitEncryptionMode transitEncryptionMode() {
return TransitEncryptionMode.fromValue(transitEncryptionMode);
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* When setting TransitEncryptionEnabled
to true
, you can set your
* TransitEncryptionMode
to preferred
in the same request, to allow both encrypted and
* unencrypted connections at the same time. Once you migrate all your Redis clients to use encrypted connections
* you can modify the value to required
to allow encrypted connections only.
*
*
* Setting TransitEncryptionMode
to required
is a two-step process that requires you to
* first set the TransitEncryptionMode
to preferred
, after that you can set
* TransitEncryptionMode
to required
.
*
*
* This process will not trigger the replacement of the replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
* When setting TransitEncryptionEnabled
to true
, you can set your
* TransitEncryptionMode
to preferred
in the same request, to allow both encrypted
* and unencrypted connections at the same time. Once you migrate all your Redis clients to use encrypted
* connections you can modify the value to required
to allow encrypted connections only.
*
*
* Setting TransitEncryptionMode
to required
is a two-step process that requires
* you to first set the TransitEncryptionMode
to preferred
, after that you can set
* TransitEncryptionMode
to required
.
*
*
* This process will not trigger the replacement of the replication group.
* @see TransitEncryptionMode
*/
public final String transitEncryptionModeAsString() {
return transitEncryptionMode;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis clients to connect using both cluster mode enabled and cluster mode
* disabled. After you migrate all Redis clients to use cluster mode enabled, you can then complete cluster mode
* configuration and set the cluster mode to Enabled.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterMode} will
* return {@link ClusterMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterModeAsString}.
*
*
* @return Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis clients to connect using both cluster mode enabled and
* cluster mode disabled. After you migrate all Redis clients to use cluster mode enabled, you can then
* complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public final ClusterMode clusterMode() {
return ClusterMode.fromValue(clusterMode);
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis clients to connect using both cluster mode enabled and cluster mode
* disabled. After you migrate all Redis clients to use cluster mode enabled, you can then complete cluster mode
* configuration and set the cluster mode to Enabled.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterMode} will
* return {@link ClusterMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterModeAsString}.
*
*
* @return Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis clients to connect using both cluster mode enabled and
* cluster mode disabled. After you migrate all Redis clients to use cluster mode enabled, you can then
* complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public final String clusterModeAsString() {
return clusterMode;
}
/**
*
* The name of the snapshot used to create a replication group. Available for Redis only.
*
*
* @return The name of the snapshot used to create a replication group. Available for Redis only.
*/
public final String serverlessCacheSnapshotName() {
return serverlessCacheSnapshotName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupDescription());
hashCode = 31 * hashCode + Objects.hashCode(globalReplicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(primaryClusterId());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverEnabled());
hashCode = 31 * hashCode + Objects.hashCode(multiAZEnabled());
hashCode = 31 * hashCode + Objects.hashCode(numCacheClusters());
hashCode = 31 * hashCode + Objects.hashCode(hasPreferredCacheClusterAZs() ? preferredCacheClusterAZs() : null);
hashCode = 31 * hashCode + Objects.hashCode(numNodeGroups());
hashCode = 31 * hashCode + Objects.hashCode(replicasPerNodeGroup());
hashCode = 31 * hashCode + Objects.hashCode(hasNodeGroupConfiguration() ? nodeGroupConfiguration() : null);
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cacheSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSnapshotArns() ? snapshotArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(snapshotName());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(authToken());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(atRestEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroupIds() ? userGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(dataTieringEnabled());
hashCode = 31 * hashCode + Objects.hashCode(networkTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipDiscoveryAsString());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(clusterModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(serverlessCacheSnapshotName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateReplicationGroupRequest)) {
return false;
}
CreateReplicationGroupRequest other = (CreateReplicationGroupRequest) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(replicationGroupDescription(), other.replicationGroupDescription())
&& Objects.equals(globalReplicationGroupId(), other.globalReplicationGroupId())
&& Objects.equals(primaryClusterId(), other.primaryClusterId())
&& Objects.equals(automaticFailoverEnabled(), other.automaticFailoverEnabled())
&& Objects.equals(multiAZEnabled(), other.multiAZEnabled())
&& Objects.equals(numCacheClusters(), other.numCacheClusters())
&& hasPreferredCacheClusterAZs() == other.hasPreferredCacheClusterAZs()
&& Objects.equals(preferredCacheClusterAZs(), other.preferredCacheClusterAZs())
&& Objects.equals(numNodeGroups(), other.numNodeGroups())
&& Objects.equals(replicasPerNodeGroup(), other.replicasPerNodeGroup())
&& hasNodeGroupConfiguration() == other.hasNodeGroupConfiguration()
&& Objects.equals(nodeGroupConfiguration(), other.nodeGroupConfiguration())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(cacheParameterGroupName(), other.cacheParameterGroupName())
&& Objects.equals(cacheSubnetGroupName(), other.cacheSubnetGroupName())
&& hasCacheSecurityGroupNames() == other.hasCacheSecurityGroupNames()
&& Objects.equals(cacheSecurityGroupNames(), other.cacheSecurityGroupNames())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && hasSnapshotArns() == other.hasSnapshotArns()
&& Objects.equals(snapshotArns(), other.snapshotArns()) && Objects.equals(snapshotName(), other.snapshotName())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(port(), other.port()) && Objects.equals(notificationTopicArn(), other.notificationTopicArn())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow()) && Objects.equals(authToken(), other.authToken())
&& Objects.equals(transitEncryptionEnabled(), other.transitEncryptionEnabled())
&& Objects.equals(atRestEncryptionEnabled(), other.atRestEncryptionEnabled())
&& Objects.equals(kmsKeyId(), other.kmsKeyId()) && hasUserGroupIds() == other.hasUserGroupIds()
&& Objects.equals(userGroupIds(), other.userGroupIds())
&& hasLogDeliveryConfigurations() == other.hasLogDeliveryConfigurations()
&& Objects.equals(logDeliveryConfigurations(), other.logDeliveryConfigurations())
&& Objects.equals(dataTieringEnabled(), other.dataTieringEnabled())
&& Objects.equals(networkTypeAsString(), other.networkTypeAsString())
&& Objects.equals(ipDiscoveryAsString(), other.ipDiscoveryAsString())
&& Objects.equals(transitEncryptionModeAsString(), other.transitEncryptionModeAsString())
&& Objects.equals(clusterModeAsString(), other.clusterModeAsString())
&& Objects.equals(serverlessCacheSnapshotName(), other.serverlessCacheSnapshotName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateReplicationGroupRequest").add("ReplicationGroupId", replicationGroupId())
.add("ReplicationGroupDescription", replicationGroupDescription())
.add("GlobalReplicationGroupId", globalReplicationGroupId()).add("PrimaryClusterId", primaryClusterId())
.add("AutomaticFailoverEnabled", automaticFailoverEnabled()).add("MultiAZEnabled", multiAZEnabled())
.add("NumCacheClusters", numCacheClusters())
.add("PreferredCacheClusterAZs", hasPreferredCacheClusterAZs() ? preferredCacheClusterAZs() : null)
.add("NumNodeGroups", numNodeGroups()).add("ReplicasPerNodeGroup", replicasPerNodeGroup())
.add("NodeGroupConfiguration", hasNodeGroupConfiguration() ? nodeGroupConfiguration() : null)
.add("CacheNodeType", cacheNodeType()).add("Engine", engine()).add("EngineVersion", engineVersion())
.add("CacheParameterGroupName", cacheParameterGroupName()).add("CacheSubnetGroupName", cacheSubnetGroupName())
.add("CacheSecurityGroupNames", hasCacheSecurityGroupNames() ? cacheSecurityGroupNames() : null)
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("Tags", hasTags() ? tags() : null).add("SnapshotArns", hasSnapshotArns() ? snapshotArns() : null)
.add("SnapshotName", snapshotName()).add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("Port", port()).add("NotificationTopicArn", notificationTopicArn())
.add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("AuthToken", authToken()).add("TransitEncryptionEnabled", transitEncryptionEnabled())
.add("AtRestEncryptionEnabled", atRestEncryptionEnabled()).add("KmsKeyId", kmsKeyId())
.add("UserGroupIds", hasUserGroupIds() ? userGroupIds() : null)
.add("LogDeliveryConfigurations", hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null)
.add("DataTieringEnabled", dataTieringEnabled()).add("NetworkType", networkTypeAsString())
.add("IpDiscovery", ipDiscoveryAsString()).add("TransitEncryptionMode", transitEncryptionModeAsString())
.add("ClusterMode", clusterModeAsString()).add("ServerlessCacheSnapshotName", serverlessCacheSnapshotName())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "ReplicationGroupDescription":
return Optional.ofNullable(clazz.cast(replicationGroupDescription()));
case "GlobalReplicationGroupId":
return Optional.ofNullable(clazz.cast(globalReplicationGroupId()));
case "PrimaryClusterId":
return Optional.ofNullable(clazz.cast(primaryClusterId()));
case "AutomaticFailoverEnabled":
return Optional.ofNullable(clazz.cast(automaticFailoverEnabled()));
case "MultiAZEnabled":
return Optional.ofNullable(clazz.cast(multiAZEnabled()));
case "NumCacheClusters":
return Optional.ofNullable(clazz.cast(numCacheClusters()));
case "PreferredCacheClusterAZs":
return Optional.ofNullable(clazz.cast(preferredCacheClusterAZs()));
case "NumNodeGroups":
return Optional.ofNullable(clazz.cast(numNodeGroups()));
case "ReplicasPerNodeGroup":
return Optional.ofNullable(clazz.cast(replicasPerNodeGroup()));
case "NodeGroupConfiguration":
return Optional.ofNullable(clazz.cast(nodeGroupConfiguration()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "CacheParameterGroupName":
return Optional.ofNullable(clazz.cast(cacheParameterGroupName()));
case "CacheSubnetGroupName":
return Optional.ofNullable(clazz.cast(cacheSubnetGroupName()));
case "CacheSecurityGroupNames":
return Optional.ofNullable(clazz.cast(cacheSecurityGroupNames()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "SnapshotArns":
return Optional.ofNullable(clazz.cast(snapshotArns()));
case "SnapshotName":
return Optional.ofNullable(clazz.cast(snapshotName()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "NotificationTopicArn":
return Optional.ofNullable(clazz.cast(notificationTopicArn()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "AuthToken":
return Optional.ofNullable(clazz.cast(authToken()));
case "TransitEncryptionEnabled":
return Optional.ofNullable(clazz.cast(transitEncryptionEnabled()));
case "AtRestEncryptionEnabled":
return Optional.ofNullable(clazz.cast(atRestEncryptionEnabled()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "UserGroupIds":
return Optional.ofNullable(clazz.cast(userGroupIds()));
case "LogDeliveryConfigurations":
return Optional.ofNullable(clazz.cast(logDeliveryConfigurations()));
case "DataTieringEnabled":
return Optional.ofNullable(clazz.cast(dataTieringEnabled()));
case "NetworkType":
return Optional.ofNullable(clazz.cast(networkTypeAsString()));
case "IpDiscovery":
return Optional.ofNullable(clazz.cast(ipDiscoveryAsString()));
case "TransitEncryptionMode":
return Optional.ofNullable(clazz.cast(transitEncryptionModeAsString()));
case "ClusterMode":
return Optional.ofNullable(clazz.cast(clusterModeAsString()));
case "ServerlessCacheSnapshotName":
return Optional.ofNullable(clazz.cast(serverlessCacheSnapshotName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function