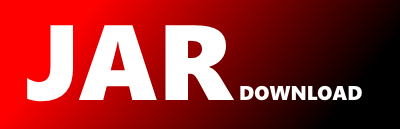
software.amazon.awssdk.services.elasticache.model.CreateUserRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateUserRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("UserId")
.getter(getter(CreateUserRequest::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserId").build()).build();
private static final SdkField USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserName").getter(getter(CreateUserRequest::userName)).setter(setter(Builder::userName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserName").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(CreateUserRequest::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField> PASSWORDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Passwords")
.getter(getter(CreateUserRequest::passwords))
.setter(setter(Builder::passwords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Passwords").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ACCESS_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccessString").getter(getter(CreateUserRequest::accessString)).setter(setter(Builder::accessString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccessString").build()).build();
private static final SdkField NO_PASSWORD_REQUIRED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("NoPasswordRequired").getter(getter(CreateUserRequest::noPasswordRequired))
.setter(setter(Builder::noPasswordRequired))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NoPasswordRequired").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateUserRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final SdkField AUTHENTICATION_MODE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AuthenticationMode")
.getter(getter(CreateUserRequest::authenticationMode)).setter(setter(Builder::authenticationMode))
.constructor(AuthenticationMode::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthenticationMode").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USER_ID_FIELD,
USER_NAME_FIELD, ENGINE_FIELD, PASSWORDS_FIELD, ACCESS_STRING_FIELD, NO_PASSWORD_REQUIRED_FIELD, TAGS_FIELD,
AUTHENTICATION_MODE_FIELD));
private final String userId;
private final String userName;
private final String engine;
private final List passwords;
private final String accessString;
private final Boolean noPasswordRequired;
private final List tags;
private final AuthenticationMode authenticationMode;
private CreateUserRequest(BuilderImpl builder) {
super(builder);
this.userId = builder.userId;
this.userName = builder.userName;
this.engine = builder.engine;
this.passwords = builder.passwords;
this.accessString = builder.accessString;
this.noPasswordRequired = builder.noPasswordRequired;
this.tags = builder.tags;
this.authenticationMode = builder.authenticationMode;
}
/**
*
* The ID of the user.
*
*
* @return The ID of the user.
*/
public final String userId() {
return userId;
}
/**
*
* The username of the user.
*
*
* @return The username of the user.
*/
public final String userName() {
return userName;
}
/**
*
* The current supported value is Redis.
*
*
* @return The current supported value is Redis.
*/
public final String engine() {
return engine;
}
/**
* For responses, this returns true if the service returned a value for the Passwords property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasPasswords() {
return passwords != null && !(passwords instanceof SdkAutoConstructList);
}
/**
*
* Passwords used for this user. You can create up to two passwords for each user.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPasswords} method.
*
*
* @return Passwords used for this user. You can create up to two passwords for each user.
*/
public final List passwords() {
return passwords;
}
/**
*
* Access permissions string used for this user.
*
*
* @return Access permissions string used for this user.
*/
public final String accessString() {
return accessString;
}
/**
*
* Indicates a password is not required for this user.
*
*
* @return Indicates a password is not required for this user.
*/
public final Boolean noPasswordRequired() {
return noPasswordRequired;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags to be added to this resource. A tag is a key-value pair. A tag key must be accompanied by a tag
* value, although null is accepted.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags to be added to this resource. A tag is a key-value pair. A tag key must be accompanied by
* a tag value, although null is accepted.
*/
public final List tags() {
return tags;
}
/**
*
* Specifies how to authenticate the user.
*
*
* @return Specifies how to authenticate the user.
*/
public final AuthenticationMode authenticationMode() {
return authenticationMode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(userName());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(hasPasswords() ? passwords() : null);
hashCode = 31 * hashCode + Objects.hashCode(accessString());
hashCode = 31 * hashCode + Objects.hashCode(noPasswordRequired());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(authenticationMode());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateUserRequest)) {
return false;
}
CreateUserRequest other = (CreateUserRequest) obj;
return Objects.equals(userId(), other.userId()) && Objects.equals(userName(), other.userName())
&& Objects.equals(engine(), other.engine()) && hasPasswords() == other.hasPasswords()
&& Objects.equals(passwords(), other.passwords()) && Objects.equals(accessString(), other.accessString())
&& Objects.equals(noPasswordRequired(), other.noPasswordRequired()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(authenticationMode(), other.authenticationMode());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateUserRequest").add("UserId", userId()).add("UserName", userName()).add("Engine", engine())
.add("Passwords", hasPasswords() ? passwords() : null).add("AccessString", accessString())
.add("NoPasswordRequired", noPasswordRequired()).add("Tags", hasTags() ? tags() : null)
.add("AuthenticationMode", authenticationMode()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UserId":
return Optional.ofNullable(clazz.cast(userId()));
case "UserName":
return Optional.ofNullable(clazz.cast(userName()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "Passwords":
return Optional.ofNullable(clazz.cast(passwords()));
case "AccessString":
return Optional.ofNullable(clazz.cast(accessString()));
case "NoPasswordRequired":
return Optional.ofNullable(clazz.cast(noPasswordRequired()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "AuthenticationMode":
return Optional.ofNullable(clazz.cast(authenticationMode()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function