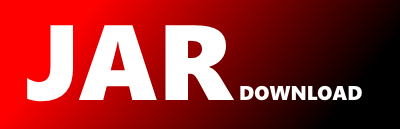
software.amazon.awssdk.services.elasticache.model.ServerlessCacheSnapshot Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The resource representing a serverless cache snapshot. Available for Redis OSS and Serverless Memcached only.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServerlessCacheSnapshot implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SERVERLESS_CACHE_SNAPSHOT_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ServerlessCacheSnapshotName")
.getter(getter(ServerlessCacheSnapshot::serverlessCacheSnapshotName))
.setter(setter(Builder::serverlessCacheSnapshotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerlessCacheSnapshotName")
.build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(ServerlessCacheSnapshot::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(ServerlessCacheSnapshot::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField SNAPSHOT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotType").getter(getter(ServerlessCacheSnapshot::snapshotType))
.setter(setter(Builder::snapshotType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotType").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ServerlessCacheSnapshot::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTime").getter(getter(ServerlessCacheSnapshot::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTime").build()).build();
private static final SdkField EXPIRY_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ExpiryTime").getter(getter(ServerlessCacheSnapshot::expiryTime)).setter(setter(Builder::expiryTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpiryTime").build()).build();
private static final SdkField BYTES_USED_FOR_CACHE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BytesUsedForCache").getter(getter(ServerlessCacheSnapshot::bytesUsedForCache))
.setter(setter(Builder::bytesUsedForCache))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BytesUsedForCache").build()).build();
private static final SdkField SERVERLESS_CACHE_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ServerlessCacheConfiguration")
.getter(getter(ServerlessCacheSnapshot::serverlessCacheConfiguration))
.setter(setter(Builder::serverlessCacheConfiguration))
.constructor(ServerlessCacheConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerlessCacheConfiguration")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
SERVERLESS_CACHE_SNAPSHOT_NAME_FIELD, ARN_FIELD, KMS_KEY_ID_FIELD, SNAPSHOT_TYPE_FIELD, STATUS_FIELD,
CREATE_TIME_FIELD, EXPIRY_TIME_FIELD, BYTES_USED_FOR_CACHE_FIELD, SERVERLESS_CACHE_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String serverlessCacheSnapshotName;
private final String arn;
private final String kmsKeyId;
private final String snapshotType;
private final String status;
private final Instant createTime;
private final Instant expiryTime;
private final String bytesUsedForCache;
private final ServerlessCacheConfiguration serverlessCacheConfiguration;
private ServerlessCacheSnapshot(BuilderImpl builder) {
this.serverlessCacheSnapshotName = builder.serverlessCacheSnapshotName;
this.arn = builder.arn;
this.kmsKeyId = builder.kmsKeyId;
this.snapshotType = builder.snapshotType;
this.status = builder.status;
this.createTime = builder.createTime;
this.expiryTime = builder.expiryTime;
this.bytesUsedForCache = builder.bytesUsedForCache;
this.serverlessCacheConfiguration = builder.serverlessCacheConfiguration;
}
/**
*
* The identifier of a serverless cache snapshot. Available for Redis OSS and Serverless Memcached only.
*
*
* @return The identifier of a serverless cache snapshot. Available for Redis OSS and Serverless Memcached only.
*/
public final String serverlessCacheSnapshotName() {
return serverlessCacheSnapshotName;
}
/**
*
* The Amazon Resource Name (ARN) of a serverless cache snapshot. Available for Redis OSS and Serverless Memcached
* only.
*
*
* @return The Amazon Resource Name (ARN) of a serverless cache snapshot. Available for Redis OSS and Serverless
* Memcached only.
*/
public final String arn() {
return arn;
}
/**
*
* The ID of the Amazon Web Services Key Management Service (KMS) key of a serverless cache snapshot. Available for
* Redis OSS and Serverless Memcached only.
*
*
* @return The ID of the Amazon Web Services Key Management Service (KMS) key of a serverless cache snapshot.
* Available for Redis OSS and Serverless Memcached only.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The type of snapshot of serverless cache. Available for Redis OSS and Serverless Memcached only.
*
*
* @return The type of snapshot of serverless cache. Available for Redis OSS and Serverless Memcached only.
*/
public final String snapshotType() {
return snapshotType;
}
/**
*
* The current status of the serverless cache. Available for Redis OSS and Serverless Memcached only.
*
*
* @return The current status of the serverless cache. Available for Redis OSS and Serverless Memcached only.
*/
public final String status() {
return status;
}
/**
*
* The date and time that the source serverless cache's metadata and cache data set was obtained for the snapshot.
* Available for Redis OSS and Serverless Memcached only.
*
*
* @return The date and time that the source serverless cache's metadata and cache data set was obtained for the
* snapshot. Available for Redis OSS and Serverless Memcached only.
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The time that the serverless cache snapshot will expire. Available for Redis OSS and Serverless Memcached only.
*
*
* @return The time that the serverless cache snapshot will expire. Available for Redis OSS and Serverless Memcached
* only.
*/
public final Instant expiryTime() {
return expiryTime;
}
/**
*
* The total size of a serverless cache snapshot, in bytes. Available for Redis OSS and Serverless Memcached only.
*
*
* @return The total size of a serverless cache snapshot, in bytes. Available for Redis OSS and Serverless Memcached
* only.
*/
public final String bytesUsedForCache() {
return bytesUsedForCache;
}
/**
*
* The configuration of the serverless cache, at the time the snapshot was taken. Available for Redis OSS and
* Serverless Memcached only.
*
*
* @return The configuration of the serverless cache, at the time the snapshot was taken. Available for Redis OSS
* and Serverless Memcached only.
*/
public final ServerlessCacheConfiguration serverlessCacheConfiguration() {
return serverlessCacheConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serverlessCacheSnapshotName());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(snapshotType());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(expiryTime());
hashCode = 31 * hashCode + Objects.hashCode(bytesUsedForCache());
hashCode = 31 * hashCode + Objects.hashCode(serverlessCacheConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServerlessCacheSnapshot)) {
return false;
}
ServerlessCacheSnapshot other = (ServerlessCacheSnapshot) obj;
return Objects.equals(serverlessCacheSnapshotName(), other.serverlessCacheSnapshotName())
&& Objects.equals(arn(), other.arn()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(snapshotType(), other.snapshotType()) && Objects.equals(status(), other.status())
&& Objects.equals(createTime(), other.createTime()) && Objects.equals(expiryTime(), other.expiryTime())
&& Objects.equals(bytesUsedForCache(), other.bytesUsedForCache())
&& Objects.equals(serverlessCacheConfiguration(), other.serverlessCacheConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServerlessCacheSnapshot").add("ServerlessCacheSnapshotName", serverlessCacheSnapshotName())
.add("ARN", arn()).add("KmsKeyId", kmsKeyId()).add("SnapshotType", snapshotType()).add("Status", status())
.add("CreateTime", createTime()).add("ExpiryTime", expiryTime()).add("BytesUsedForCache", bytesUsedForCache())
.add("ServerlessCacheConfiguration", serverlessCacheConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServerlessCacheSnapshotName":
return Optional.ofNullable(clazz.cast(serverlessCacheSnapshotName()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "SnapshotType":
return Optional.ofNullable(clazz.cast(snapshotType()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "CreateTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "ExpiryTime":
return Optional.ofNullable(clazz.cast(expiryTime()));
case "BytesUsedForCache":
return Optional.ofNullable(clazz.cast(bytesUsedForCache()));
case "ServerlessCacheConfiguration":
return Optional.ofNullable(clazz.cast(serverlessCacheConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function