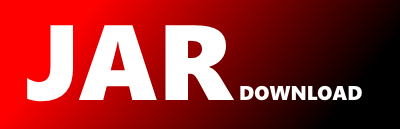
software.amazon.awssdk.services.elasticache.model.CacheCluster Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains all of the attributes of a specific cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CacheCluster implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheClusterId").getter(getter(CacheCluster::cacheClusterId)).setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField CONFIGURATION_ENDPOINT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ConfigurationEndpoint").getter(getter(CacheCluster::configurationEndpoint))
.setter(setter(Builder::configurationEndpoint)).constructor(Endpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationEndpoint").build())
.build();
private static final SdkField CLIENT_DOWNLOAD_LANDING_PAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientDownloadLandingPage").getter(getter(CacheCluster::clientDownloadLandingPage))
.setter(setter(Builder::clientDownloadLandingPage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientDownloadLandingPage").build())
.build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(CacheCluster::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(CacheCluster::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(CacheCluster::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField CACHE_CLUSTER_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheClusterStatus").getter(getter(CacheCluster::cacheClusterStatus))
.setter(setter(Builder::cacheClusterStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterStatus").build())
.build();
private static final SdkField NUM_CACHE_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumCacheNodes").getter(getter(CacheCluster::numCacheNodes)).setter(setter(Builder::numCacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheNodes").build()).build();
private static final SdkField PREFERRED_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredAvailabilityZone").getter(getter(CacheCluster::preferredAvailabilityZone))
.setter(setter(Builder::preferredAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZone").build())
.build();
private static final SdkField PREFERRED_OUTPOST_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredOutpostArn").getter(getter(CacheCluster::preferredOutpostArn))
.setter(setter(Builder::preferredOutpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredOutpostArn").build())
.build();
private static final SdkField CACHE_CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CacheClusterCreateTime").getter(getter(CacheCluster::cacheClusterCreateTime))
.setter(setter(Builder::cacheClusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterCreateTime").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(CacheCluster::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField PENDING_MODIFIED_VALUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PendingModifiedValues")
.getter(getter(CacheCluster::pendingModifiedValues)).setter(setter(Builder::pendingModifiedValues))
.constructor(PendingModifiedValues::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingModifiedValues").build())
.build();
private static final SdkField NOTIFICATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("NotificationConfiguration")
.getter(getter(CacheCluster::notificationConfiguration)).setter(setter(Builder::notificationConfiguration))
.constructor(NotificationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationConfiguration").build())
.build();
private static final SdkField> CACHE_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheSecurityGroups")
.getter(getter(CacheCluster::cacheSecurityGroups))
.setter(setter(Builder::cacheSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CacheSecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroup").build()).build()).build()).build();
private static final SdkField CACHE_PARAMETER_GROUP_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CacheParameterGroup")
.getter(getter(CacheCluster::cacheParameterGroup)).setter(setter(Builder::cacheParameterGroup))
.constructor(CacheParameterGroupStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroup").build())
.build();
private static final SdkField CACHE_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheSubnetGroupName").getter(getter(CacheCluster::cacheSubnetGroupName))
.setter(setter(Builder::cacheSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSubnetGroupName").build())
.build();
private static final SdkField> CACHE_NODES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CacheNodes")
.getter(getter(CacheCluster::cacheNodes))
.setter(setter(Builder::cacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodes").build(),
ListTrait
.builder()
.memberLocationName("CacheNode")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CacheNode::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheNode").build()).build()).build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(CacheCluster::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroups")
.getter(getter(CacheCluster::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(CacheCluster::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(CacheCluster::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(CacheCluster::snapshotWindow)).setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField AUTH_TOKEN_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AuthTokenEnabled").getter(getter(CacheCluster::authTokenEnabled))
.setter(setter(Builder::authTokenEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenEnabled").build()).build();
private static final SdkField AUTH_TOKEN_LAST_MODIFIED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).memberName("AuthTokenLastModifiedDate")
.getter(getter(CacheCluster::authTokenLastModifiedDate)).setter(setter(Builder::authTokenLastModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenLastModifiedDate").build())
.build();
private static final SdkField TRANSIT_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("TransitEncryptionEnabled").getter(getter(CacheCluster::transitEncryptionEnabled))
.setter(setter(Builder::transitEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionEnabled").build())
.build();
private static final SdkField AT_REST_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AtRestEncryptionEnabled").getter(getter(CacheCluster::atRestEncryptionEnabled))
.setter(setter(Builder::atRestEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AtRestEncryptionEnabled").build())
.build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(CacheCluster::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField REPLICATION_GROUP_LOG_DELIVERY_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ReplicationGroupLogDeliveryEnabled")
.getter(getter(CacheCluster::replicationGroupLogDeliveryEnabled))
.setter(setter(Builder::replicationGroupLogDeliveryEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupLogDeliveryEnabled")
.build()).build();
private static final SdkField> LOG_DELIVERY_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LogDeliveryConfigurations")
.getter(getter(CacheCluster::logDeliveryConfigurations))
.setter(setter(Builder::logDeliveryConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDeliveryConfigurations").build(),
ListTrait
.builder()
.memberLocationName("LogDeliveryConfiguration")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LogDeliveryConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LogDeliveryConfiguration").build()).build()).build()).build();
private static final SdkField NETWORK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetworkType").getter(getter(CacheCluster::networkTypeAsString)).setter(setter(Builder::networkType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkType").build()).build();
private static final SdkField IP_DISCOVERY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpDiscovery").getter(getter(CacheCluster::ipDiscoveryAsString)).setter(setter(Builder::ipDiscovery))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpDiscovery").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TransitEncryptionMode").getter(getter(CacheCluster::transitEncryptionModeAsString))
.setter(setter(Builder::transitEncryptionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionMode").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CACHE_CLUSTER_ID_FIELD,
CONFIGURATION_ENDPOINT_FIELD, CLIENT_DOWNLOAD_LANDING_PAGE_FIELD, CACHE_NODE_TYPE_FIELD, ENGINE_FIELD,
ENGINE_VERSION_FIELD, CACHE_CLUSTER_STATUS_FIELD, NUM_CACHE_NODES_FIELD, PREFERRED_AVAILABILITY_ZONE_FIELD,
PREFERRED_OUTPOST_ARN_FIELD, CACHE_CLUSTER_CREATE_TIME_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD,
PENDING_MODIFIED_VALUES_FIELD, NOTIFICATION_CONFIGURATION_FIELD, CACHE_SECURITY_GROUPS_FIELD,
CACHE_PARAMETER_GROUP_FIELD, CACHE_SUBNET_GROUP_NAME_FIELD, CACHE_NODES_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD,
SECURITY_GROUPS_FIELD, REPLICATION_GROUP_ID_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD, SNAPSHOT_WINDOW_FIELD,
AUTH_TOKEN_ENABLED_FIELD, AUTH_TOKEN_LAST_MODIFIED_DATE_FIELD, TRANSIT_ENCRYPTION_ENABLED_FIELD,
AT_REST_ENCRYPTION_ENABLED_FIELD, ARN_FIELD, REPLICATION_GROUP_LOG_DELIVERY_ENABLED_FIELD,
LOG_DELIVERY_CONFIGURATIONS_FIELD, NETWORK_TYPE_FIELD, IP_DISCOVERY_FIELD, TRANSIT_ENCRYPTION_MODE_FIELD));
private static final long serialVersionUID = 1L;
private final String cacheClusterId;
private final Endpoint configurationEndpoint;
private final String clientDownloadLandingPage;
private final String cacheNodeType;
private final String engine;
private final String engineVersion;
private final String cacheClusterStatus;
private final Integer numCacheNodes;
private final String preferredAvailabilityZone;
private final String preferredOutpostArn;
private final Instant cacheClusterCreateTime;
private final String preferredMaintenanceWindow;
private final PendingModifiedValues pendingModifiedValues;
private final NotificationConfiguration notificationConfiguration;
private final List cacheSecurityGroups;
private final CacheParameterGroupStatus cacheParameterGroup;
private final String cacheSubnetGroupName;
private final List cacheNodes;
private final Boolean autoMinorVersionUpgrade;
private final List securityGroups;
private final String replicationGroupId;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final Boolean authTokenEnabled;
private final Instant authTokenLastModifiedDate;
private final Boolean transitEncryptionEnabled;
private final Boolean atRestEncryptionEnabled;
private final String arn;
private final Boolean replicationGroupLogDeliveryEnabled;
private final List logDeliveryConfigurations;
private final String networkType;
private final String ipDiscovery;
private final String transitEncryptionMode;
private CacheCluster(BuilderImpl builder) {
this.cacheClusterId = builder.cacheClusterId;
this.configurationEndpoint = builder.configurationEndpoint;
this.clientDownloadLandingPage = builder.clientDownloadLandingPage;
this.cacheNodeType = builder.cacheNodeType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.cacheClusterStatus = builder.cacheClusterStatus;
this.numCacheNodes = builder.numCacheNodes;
this.preferredAvailabilityZone = builder.preferredAvailabilityZone;
this.preferredOutpostArn = builder.preferredOutpostArn;
this.cacheClusterCreateTime = builder.cacheClusterCreateTime;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.pendingModifiedValues = builder.pendingModifiedValues;
this.notificationConfiguration = builder.notificationConfiguration;
this.cacheSecurityGroups = builder.cacheSecurityGroups;
this.cacheParameterGroup = builder.cacheParameterGroup;
this.cacheSubnetGroupName = builder.cacheSubnetGroupName;
this.cacheNodes = builder.cacheNodes;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.securityGroups = builder.securityGroups;
this.replicationGroupId = builder.replicationGroupId;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.authTokenEnabled = builder.authTokenEnabled;
this.authTokenLastModifiedDate = builder.authTokenLastModifiedDate;
this.transitEncryptionEnabled = builder.transitEncryptionEnabled;
this.atRestEncryptionEnabled = builder.atRestEncryptionEnabled;
this.arn = builder.arn;
this.replicationGroupLogDeliveryEnabled = builder.replicationGroupLogDeliveryEnabled;
this.logDeliveryConfigurations = builder.logDeliveryConfigurations;
this.networkType = builder.networkType;
this.ipDiscovery = builder.ipDiscovery;
this.transitEncryptionMode = builder.transitEncryptionMode;
}
/**
*
* The user-supplied identifier of the cluster. This identifier is a unique key that identifies a cluster.
*
*
* @return The user-supplied identifier of the cluster. This identifier is a unique key that identifies a cluster.
*/
public final String cacheClusterId() {
return cacheClusterId;
}
/**
*
* Represents a Memcached cluster endpoint which can be used by an application to connect to any node in the
* cluster. The configuration endpoint will always have .cfg
in it.
*
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
*
*
* @return Represents a Memcached cluster endpoint which can be used by an application to connect to any node in the
* cluster. The configuration endpoint will always have .cfg
in it.
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
*/
public final Endpoint configurationEndpoint() {
return configurationEndpoint;
}
/**
*
* The URL of the web page where you can download the latest ElastiCache client library.
*
*
* @return The URL of the web page where you can download the latest ElastiCache client library.
*/
public final String clientDownloadLandingPage() {
return clientDownloadLandingPage;
}
/**
*
* The name of the compute and memory capacity node type for the cluster.
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*
* @return The name of the compute and memory capacity node type for the cluster.
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The name of the cache engine (memcached
or redis
) to be used for this cluster.
*
*
* @return The name of the cache engine (memcached
or redis
) to be used for this cluster.
*/
public final String engine() {
return engine;
}
/**
*
* The version of the cache engine that is used in this cluster.
*
*
* @return The version of the cache engine that is used in this cluster.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The current state of this cluster, one of the following values: available
, creating
,
* deleted
, deleting
, incompatible-network
, modifying
,
* rebooting cluster nodes
, restore-failed
, or snapshotting
.
*
*
* @return The current state of this cluster, one of the following values: available
,
* creating
, deleted
, deleting
, incompatible-network
,
* modifying
, rebooting cluster nodes
, restore-failed
, or
* snapshotting
.
*/
public final String cacheClusterStatus() {
return cacheClusterStatus;
}
/**
*
* The number of cache nodes in the cluster.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* @return The number of cache nodes in the cluster.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*/
public final Integer numCacheNodes() {
return numCacheNodes;
}
/**
*
* The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are located in
* different Availability Zones.
*
*
* @return The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are
* located in different Availability Zones.
*/
public final String preferredAvailabilityZone() {
return preferredAvailabilityZone;
}
/**
*
* The outpost ARN in which the cache cluster is created.
*
*
* @return The outpost ARN in which the cache cluster is created.
*/
public final String preferredOutpostArn() {
return preferredOutpostArn;
}
/**
*
* The date and time when the cluster was created.
*
*
* @return The date and time when the cluster was created.
*/
public final Instant cacheClusterCreateTime() {
return cacheClusterCreateTime;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
* Returns the value of the PendingModifiedValues property for this object.
*
* @return The value of the PendingModifiedValues property for this object.
*/
public final PendingModifiedValues pendingModifiedValues() {
return pendingModifiedValues;
}
/**
*
* Describes a notification topic and its status. Notification topics are used for publishing ElastiCache events to
* subscribers using Amazon Simple Notification Service (SNS).
*
*
* @return Describes a notification topic and its status. Notification topics are used for publishing ElastiCache
* events to subscribers using Amazon Simple Notification Service (SNS).
*/
public final NotificationConfiguration notificationConfiguration() {
return notificationConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the CacheSecurityGroups property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCacheSecurityGroups() {
return cacheSecurityGroups != null && !(cacheSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of cache security group elements, composed of name and status sub-elements.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCacheSecurityGroups} method.
*
*
* @return A list of cache security group elements, composed of name and status sub-elements.
*/
public final List cacheSecurityGroups() {
return cacheSecurityGroups;
}
/**
*
* Status of the cache parameter group.
*
*
* @return Status of the cache parameter group.
*/
public final CacheParameterGroupStatus cacheParameterGroup() {
return cacheParameterGroup;
}
/**
*
* The name of the cache subnet group associated with the cluster.
*
*
* @return The name of the cache subnet group associated with the cluster.
*/
public final String cacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
* For responses, this returns true if the service returned a value for the CacheNodes property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasCacheNodes() {
return cacheNodes != null && !(cacheNodes instanceof SdkAutoConstructList);
}
/**
*
* A list of cache nodes that are members of the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCacheNodes} method.
*
*
* @return A list of cache nodes that are members of the cluster.
*/
public final List cacheNodes() {
return cacheNodes;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroups() {
return securityGroups != null && !(securityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of VPC Security Groups associated with the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroups} method.
*
*
* @return A list of VPC Security Groups associated with the cluster.
*/
public final List securityGroups() {
return securityGroups;
}
/**
*
* The replication group to which this cluster belongs. If this field is empty, the cluster is not associated with
* any replication group.
*
*
* @return The replication group to which this cluster belongs. If this field is empty, the cluster is not
* associated with any replication group.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* Example: 05:00-09:00
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your
* cluster.
*
* Example: 05:00-09:00
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A flag that enables using an AuthToken
(password) when issuing Redis OSS commands.
*
*
* Default: false
*
*
* @return A flag that enables using an AuthToken
(password) when issuing Redis OSS commands.
*
* Default: false
*/
public final Boolean authTokenEnabled() {
return authTokenEnabled;
}
/**
*
* The date the auth token was last modified
*
*
* @return The date the auth token was last modified
*/
public final Instant authTokenLastModifiedDate() {
return authTokenLastModifiedDate;
}
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables in-transit encryption when set to true
.
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS
* version 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public final Boolean transitEncryptionEnabled() {
return transitEncryptionEnabled;
}
/**
*
* A flag that enables encryption at-rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To enable
* at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to true
when you
* create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables encryption at-rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To
* enable at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS
* version 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public final Boolean atRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
/**
*
* The ARN (Amazon Resource Name) of the cache cluster.
*
*
* @return The ARN (Amazon Resource Name) of the cache cluster.
*/
public final String arn() {
return arn;
}
/**
*
* A boolean value indicating whether log delivery is enabled for the replication group.
*
*
* @return A boolean value indicating whether log delivery is enabled for the replication group.
*/
public final Boolean replicationGroupLogDeliveryEnabled() {
return replicationGroupLogDeliveryEnabled;
}
/**
* For responses, this returns true if the service returned a value for the LogDeliveryConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogDeliveryConfigurations() {
return logDeliveryConfigurations != null && !(logDeliveryConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Returns the destination, format and type of the logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogDeliveryConfigurations} method.
*
*
* @return Returns the destination, format and type of the logs.
*/
public final List logDeliveryConfigurations() {
return logDeliveryConfigurations;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public final NetworkType networkType() {
return NetworkType.fromValue(networkType);
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public final String networkTypeAsString() {
return networkType;
}
/**
*
* The network type associated with the cluster, either ipv4
| ipv6
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on
* the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type associated with the cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public final IpDiscovery ipDiscovery() {
return IpDiscovery.fromValue(ipDiscovery);
}
/**
*
* The network type associated with the cluster, either ipv4
| ipv6
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on
* the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type associated with the cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public final String ipDiscoveryAsString() {
return ipDiscovery;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public final TransitEncryptionMode transitEncryptionMode() {
return TransitEncryptionMode.fromValue(transitEncryptionMode);
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public final String transitEncryptionModeAsString() {
return transitEncryptionMode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(configurationEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(clientDownloadLandingPage());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterStatus());
hashCode = 31 * hashCode + Objects.hashCode(numCacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(preferredAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(preferredOutpostArn());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(pendingModifiedValues());
hashCode = 31 * hashCode + Objects.hashCode(notificationConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasCacheSecurityGroups() ? cacheSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroup());
hashCode = 31 * hashCode + Objects.hashCode(cacheSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(hasCacheNodes() ? cacheNodes() : null);
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroups() ? securityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(authTokenEnabled());
hashCode = 31 * hashCode + Objects.hashCode(authTokenLastModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(atRestEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupLogDeliveryEnabled());
hashCode = 31 * hashCode + Objects.hashCode(hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(networkTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipDiscoveryAsString());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionModeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CacheCluster)) {
return false;
}
CacheCluster other = (CacheCluster) obj;
return Objects.equals(cacheClusterId(), other.cacheClusterId())
&& Objects.equals(configurationEndpoint(), other.configurationEndpoint())
&& Objects.equals(clientDownloadLandingPage(), other.clientDownloadLandingPage())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(cacheClusterStatus(), other.cacheClusterStatus())
&& Objects.equals(numCacheNodes(), other.numCacheNodes())
&& Objects.equals(preferredAvailabilityZone(), other.preferredAvailabilityZone())
&& Objects.equals(preferredOutpostArn(), other.preferredOutpostArn())
&& Objects.equals(cacheClusterCreateTime(), other.cacheClusterCreateTime())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(pendingModifiedValues(), other.pendingModifiedValues())
&& Objects.equals(notificationConfiguration(), other.notificationConfiguration())
&& hasCacheSecurityGroups() == other.hasCacheSecurityGroups()
&& Objects.equals(cacheSecurityGroups(), other.cacheSecurityGroups())
&& Objects.equals(cacheParameterGroup(), other.cacheParameterGroup())
&& Objects.equals(cacheSubnetGroupName(), other.cacheSubnetGroupName())
&& hasCacheNodes() == other.hasCacheNodes() && Objects.equals(cacheNodes(), other.cacheNodes())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& hasSecurityGroups() == other.hasSecurityGroups() && Objects.equals(securityGroups(), other.securityGroups())
&& Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(authTokenEnabled(), other.authTokenEnabled())
&& Objects.equals(authTokenLastModifiedDate(), other.authTokenLastModifiedDate())
&& Objects.equals(transitEncryptionEnabled(), other.transitEncryptionEnabled())
&& Objects.equals(atRestEncryptionEnabled(), other.atRestEncryptionEnabled())
&& Objects.equals(arn(), other.arn())
&& Objects.equals(replicationGroupLogDeliveryEnabled(), other.replicationGroupLogDeliveryEnabled())
&& hasLogDeliveryConfigurations() == other.hasLogDeliveryConfigurations()
&& Objects.equals(logDeliveryConfigurations(), other.logDeliveryConfigurations())
&& Objects.equals(networkTypeAsString(), other.networkTypeAsString())
&& Objects.equals(ipDiscoveryAsString(), other.ipDiscoveryAsString())
&& Objects.equals(transitEncryptionModeAsString(), other.transitEncryptionModeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CacheCluster").add("CacheClusterId", cacheClusterId())
.add("ConfigurationEndpoint", configurationEndpoint())
.add("ClientDownloadLandingPage", clientDownloadLandingPage()).add("CacheNodeType", cacheNodeType())
.add("Engine", engine()).add("EngineVersion", engineVersion()).add("CacheClusterStatus", cacheClusterStatus())
.add("NumCacheNodes", numCacheNodes()).add("PreferredAvailabilityZone", preferredAvailabilityZone())
.add("PreferredOutpostArn", preferredOutpostArn()).add("CacheClusterCreateTime", cacheClusterCreateTime())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("PendingModifiedValues", pendingModifiedValues())
.add("NotificationConfiguration", notificationConfiguration())
.add("CacheSecurityGroups", hasCacheSecurityGroups() ? cacheSecurityGroups() : null)
.add("CacheParameterGroup", cacheParameterGroup()).add("CacheSubnetGroupName", cacheSubnetGroupName())
.add("CacheNodes", hasCacheNodes() ? cacheNodes() : null)
.add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SecurityGroups", hasSecurityGroups() ? securityGroups() : null)
.add("ReplicationGroupId", replicationGroupId()).add("SnapshotRetentionLimit", snapshotRetentionLimit())
.add("SnapshotWindow", snapshotWindow()).add("AuthTokenEnabled", authTokenEnabled())
.add("AuthTokenLastModifiedDate", authTokenLastModifiedDate())
.add("TransitEncryptionEnabled", transitEncryptionEnabled())
.add("AtRestEncryptionEnabled", atRestEncryptionEnabled()).add("ARN", arn())
.add("ReplicationGroupLogDeliveryEnabled", replicationGroupLogDeliveryEnabled())
.add("LogDeliveryConfigurations", hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null)
.add("NetworkType", networkTypeAsString()).add("IpDiscovery", ipDiscoveryAsString())
.add("TransitEncryptionMode", transitEncryptionModeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "ConfigurationEndpoint":
return Optional.ofNullable(clazz.cast(configurationEndpoint()));
case "ClientDownloadLandingPage":
return Optional.ofNullable(clazz.cast(clientDownloadLandingPage()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "CacheClusterStatus":
return Optional.ofNullable(clazz.cast(cacheClusterStatus()));
case "NumCacheNodes":
return Optional.ofNullable(clazz.cast(numCacheNodes()));
case "PreferredAvailabilityZone":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZone()));
case "PreferredOutpostArn":
return Optional.ofNullable(clazz.cast(preferredOutpostArn()));
case "CacheClusterCreateTime":
return Optional.ofNullable(clazz.cast(cacheClusterCreateTime()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "PendingModifiedValues":
return Optional.ofNullable(clazz.cast(pendingModifiedValues()));
case "NotificationConfiguration":
return Optional.ofNullable(clazz.cast(notificationConfiguration()));
case "CacheSecurityGroups":
return Optional.ofNullable(clazz.cast(cacheSecurityGroups()));
case "CacheParameterGroup":
return Optional.ofNullable(clazz.cast(cacheParameterGroup()));
case "CacheSubnetGroupName":
return Optional.ofNullable(clazz.cast(cacheSubnetGroupName()));
case "CacheNodes":
return Optional.ofNullable(clazz.cast(cacheNodes()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "AuthTokenEnabled":
return Optional.ofNullable(clazz.cast(authTokenEnabled()));
case "AuthTokenLastModifiedDate":
return Optional.ofNullable(clazz.cast(authTokenLastModifiedDate()));
case "TransitEncryptionEnabled":
return Optional.ofNullable(clazz.cast(transitEncryptionEnabled()));
case "AtRestEncryptionEnabled":
return Optional.ofNullable(clazz.cast(atRestEncryptionEnabled()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "ReplicationGroupLogDeliveryEnabled":
return Optional.ofNullable(clazz.cast(replicationGroupLogDeliveryEnabled()));
case "LogDeliveryConfigurations":
return Optional.ofNullable(clazz.cast(logDeliveryConfigurations()));
case "NetworkType":
return Optional.ofNullable(clazz.cast(networkTypeAsString()));
case "IpDiscovery":
return Optional.ofNullable(clazz.cast(ipDiscoveryAsString()));
case "TransitEncryptionMode":
return Optional.ofNullable(clazz.cast(transitEncryptionModeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function