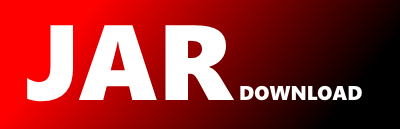
software.amazon.awssdk.services.elasticache.model.ReplicationGroup Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains all of the attributes of a specific Redis OSS replication group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationGroup implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(ReplicationGroup::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ReplicationGroup::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField GLOBAL_REPLICATION_GROUP_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("GlobalReplicationGroupInfo")
.getter(getter(ReplicationGroup::globalReplicationGroupInfo))
.setter(setter(Builder::globalReplicationGroupInfo))
.constructor(GlobalReplicationGroupInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GlobalReplicationGroupInfo").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ReplicationGroup::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PENDING_MODIFIED_VALUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PendingModifiedValues")
.getter(getter(ReplicationGroup::pendingModifiedValues)).setter(setter(Builder::pendingModifiedValues))
.constructor(ReplicationGroupPendingModifiedValues::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingModifiedValues").build())
.build();
private static final SdkField> MEMBER_CLUSTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MemberClusters")
.getter(getter(ReplicationGroup::memberClusters))
.setter(setter(Builder::memberClusters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberClusters").build(),
ListTrait
.builder()
.memberLocationName("ClusterId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ClusterId").build()).build()).build()).build();
private static final SdkField> NODE_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NodeGroups")
.getter(getter(ReplicationGroup::nodeGroups))
.setter(setter(Builder::nodeGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroups").build(),
ListTrait
.builder()
.memberLocationName("NodeGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NodeGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroup").build()).build()).build()).build();
private static final SdkField SNAPSHOTTING_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshottingClusterId").getter(getter(ReplicationGroup::snapshottingClusterId))
.setter(setter(Builder::snapshottingClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshottingClusterId").build())
.build();
private static final SdkField AUTOMATIC_FAILOVER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutomaticFailover").getter(getter(ReplicationGroup::automaticFailoverAsString))
.setter(setter(Builder::automaticFailover))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailover").build()).build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MultiAZ").getter(getter(ReplicationGroup::multiAZAsString)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField CONFIGURATION_ENDPOINT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ConfigurationEndpoint").getter(getter(ReplicationGroup::configurationEndpoint))
.setter(setter(Builder::configurationEndpoint)).constructor(Endpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationEndpoint").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(ReplicationGroup::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(ReplicationGroup::snapshotWindow))
.setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField CLUSTER_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ClusterEnabled").getter(getter(ReplicationGroup::clusterEnabled))
.setter(setter(Builder::clusterEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterEnabled").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(ReplicationGroup::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField AUTH_TOKEN_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AuthTokenEnabled").getter(getter(ReplicationGroup::authTokenEnabled))
.setter(setter(Builder::authTokenEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenEnabled").build()).build();
private static final SdkField AUTH_TOKEN_LAST_MODIFIED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).memberName("AuthTokenLastModifiedDate")
.getter(getter(ReplicationGroup::authTokenLastModifiedDate)).setter(setter(Builder::authTokenLastModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenLastModifiedDate").build())
.build();
private static final SdkField TRANSIT_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("TransitEncryptionEnabled").getter(getter(ReplicationGroup::transitEncryptionEnabled))
.setter(setter(Builder::transitEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionEnabled").build())
.build();
private static final SdkField AT_REST_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AtRestEncryptionEnabled").getter(getter(ReplicationGroup::atRestEncryptionEnabled))
.setter(setter(Builder::atRestEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AtRestEncryptionEnabled").build())
.build();
private static final SdkField> MEMBER_CLUSTERS_OUTPOST_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MemberClustersOutpostArns")
.getter(getter(ReplicationGroup::memberClustersOutpostArns))
.setter(setter(Builder::memberClustersOutpostArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberClustersOutpostArns").build(),
ListTrait
.builder()
.memberLocationName("ReplicationGroupOutpostArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReplicationGroupOutpostArn").build()).build()).build())
.build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(ReplicationGroup::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(ReplicationGroup::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField> USER_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserGroupIds")
.getter(getter(ReplicationGroup::userGroupIds))
.setter(setter(Builder::userGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOG_DELIVERY_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LogDeliveryConfigurations")
.getter(getter(ReplicationGroup::logDeliveryConfigurations))
.setter(setter(Builder::logDeliveryConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDeliveryConfigurations").build(),
ListTrait
.builder()
.memberLocationName("LogDeliveryConfiguration")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LogDeliveryConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LogDeliveryConfiguration").build()).build()).build()).build();
private static final SdkField REPLICATION_GROUP_CREATE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ReplicationGroupCreateTime")
.getter(getter(ReplicationGroup::replicationGroupCreateTime))
.setter(setter(Builder::replicationGroupCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupCreateTime").build())
.build();
private static final SdkField DATA_TIERING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataTiering").getter(getter(ReplicationGroup::dataTieringAsString)).setter(setter(Builder::dataTiering))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataTiering").build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(ReplicationGroup::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField NETWORK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetworkType").getter(getter(ReplicationGroup::networkTypeAsString)).setter(setter(Builder::networkType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkType").build()).build();
private static final SdkField IP_DISCOVERY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpDiscovery").getter(getter(ReplicationGroup::ipDiscoveryAsString)).setter(setter(Builder::ipDiscovery))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpDiscovery").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TransitEncryptionMode").getter(getter(ReplicationGroup::transitEncryptionModeAsString))
.setter(setter(Builder::transitEncryptionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionMode").build())
.build();
private static final SdkField CLUSTER_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterMode").getter(getter(ReplicationGroup::clusterModeAsString)).setter(setter(Builder::clusterMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterMode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
DESCRIPTION_FIELD, GLOBAL_REPLICATION_GROUP_INFO_FIELD, STATUS_FIELD, PENDING_MODIFIED_VALUES_FIELD,
MEMBER_CLUSTERS_FIELD, NODE_GROUPS_FIELD, SNAPSHOTTING_CLUSTER_ID_FIELD, AUTOMATIC_FAILOVER_FIELD, MULTI_AZ_FIELD,
CONFIGURATION_ENDPOINT_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD, SNAPSHOT_WINDOW_FIELD, CLUSTER_ENABLED_FIELD,
CACHE_NODE_TYPE_FIELD, AUTH_TOKEN_ENABLED_FIELD, AUTH_TOKEN_LAST_MODIFIED_DATE_FIELD,
TRANSIT_ENCRYPTION_ENABLED_FIELD, AT_REST_ENCRYPTION_ENABLED_FIELD, MEMBER_CLUSTERS_OUTPOST_ARNS_FIELD,
KMS_KEY_ID_FIELD, ARN_FIELD, USER_GROUP_IDS_FIELD, LOG_DELIVERY_CONFIGURATIONS_FIELD,
REPLICATION_GROUP_CREATE_TIME_FIELD, DATA_TIERING_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, NETWORK_TYPE_FIELD,
IP_DISCOVERY_FIELD, TRANSIT_ENCRYPTION_MODE_FIELD, CLUSTER_MODE_FIELD));
private static final long serialVersionUID = 1L;
private final String replicationGroupId;
private final String description;
private final GlobalReplicationGroupInfo globalReplicationGroupInfo;
private final String status;
private final ReplicationGroupPendingModifiedValues pendingModifiedValues;
private final List memberClusters;
private final List nodeGroups;
private final String snapshottingClusterId;
private final String automaticFailover;
private final String multiAZ;
private final Endpoint configurationEndpoint;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final Boolean clusterEnabled;
private final String cacheNodeType;
private final Boolean authTokenEnabled;
private final Instant authTokenLastModifiedDate;
private final Boolean transitEncryptionEnabled;
private final Boolean atRestEncryptionEnabled;
private final List memberClustersOutpostArns;
private final String kmsKeyId;
private final String arn;
private final List userGroupIds;
private final List logDeliveryConfigurations;
private final Instant replicationGroupCreateTime;
private final String dataTiering;
private final Boolean autoMinorVersionUpgrade;
private final String networkType;
private final String ipDiscovery;
private final String transitEncryptionMode;
private final String clusterMode;
private ReplicationGroup(BuilderImpl builder) {
this.replicationGroupId = builder.replicationGroupId;
this.description = builder.description;
this.globalReplicationGroupInfo = builder.globalReplicationGroupInfo;
this.status = builder.status;
this.pendingModifiedValues = builder.pendingModifiedValues;
this.memberClusters = builder.memberClusters;
this.nodeGroups = builder.nodeGroups;
this.snapshottingClusterId = builder.snapshottingClusterId;
this.automaticFailover = builder.automaticFailover;
this.multiAZ = builder.multiAZ;
this.configurationEndpoint = builder.configurationEndpoint;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.clusterEnabled = builder.clusterEnabled;
this.cacheNodeType = builder.cacheNodeType;
this.authTokenEnabled = builder.authTokenEnabled;
this.authTokenLastModifiedDate = builder.authTokenLastModifiedDate;
this.transitEncryptionEnabled = builder.transitEncryptionEnabled;
this.atRestEncryptionEnabled = builder.atRestEncryptionEnabled;
this.memberClustersOutpostArns = builder.memberClustersOutpostArns;
this.kmsKeyId = builder.kmsKeyId;
this.arn = builder.arn;
this.userGroupIds = builder.userGroupIds;
this.logDeliveryConfigurations = builder.logDeliveryConfigurations;
this.replicationGroupCreateTime = builder.replicationGroupCreateTime;
this.dataTiering = builder.dataTiering;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.networkType = builder.networkType;
this.ipDiscovery = builder.ipDiscovery;
this.transitEncryptionMode = builder.transitEncryptionMode;
this.clusterMode = builder.clusterMode;
}
/**
*
* The identifier for the replication group.
*
*
* @return The identifier for the replication group.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The user supplied description of the replication group.
*
*
* @return The user supplied description of the replication group.
*/
public final String description() {
return description;
}
/**
*
* The name of the Global datastore and role of this replication group in the Global datastore.
*
*
* @return The name of the Global datastore and role of this replication group in the Global datastore.
*/
public final GlobalReplicationGroupInfo globalReplicationGroupInfo() {
return globalReplicationGroupInfo;
}
/**
*
* The current state of this replication group - creating
, available
,
* modifying
, deleting
, create-failed
, snapshotting
.
*
*
* @return The current state of this replication group - creating
, available
,
* modifying
, deleting
, create-failed
, snapshotting
.
*/
public final String status() {
return status;
}
/**
*
* A group of settings to be applied to the replication group, either immediately or during the next maintenance
* window.
*
*
* @return A group of settings to be applied to the replication group, either immediately or during the next
* maintenance window.
*/
public final ReplicationGroupPendingModifiedValues pendingModifiedValues() {
return pendingModifiedValues;
}
/**
* For responses, this returns true if the service returned a value for the MemberClusters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasMemberClusters() {
return memberClusters != null && !(memberClusters instanceof SdkAutoConstructList);
}
/**
*
* The names of all the cache clusters that are part of this replication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMemberClusters} method.
*
*
* @return The names of all the cache clusters that are part of this replication group.
*/
public final List memberClusters() {
return memberClusters;
}
/**
* For responses, this returns true if the service returned a value for the NodeGroups property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasNodeGroups() {
return nodeGroups != null && !(nodeGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of node groups in this replication group. For Redis OSS (cluster mode disabled) replication groups, this
* is a single-element list. For Redis OSS (cluster mode enabled) replication groups, the list contains an entry for
* each node group (shard).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNodeGroups} method.
*
*
* @return A list of node groups in this replication group. For Redis OSS (cluster mode disabled) replication
* groups, this is a single-element list. For Redis OSS (cluster mode enabled) replication groups, the list
* contains an entry for each node group (shard).
*/
public final List nodeGroups() {
return nodeGroups;
}
/**
*
* The cluster ID that is used as the daily snapshot source for the replication group.
*
*
* @return The cluster ID that is used as the daily snapshot source for the replication group.
*/
public final String snapshottingClusterId() {
return snapshottingClusterId;
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of automatic failover for this Redis OSS replication group.
* @see AutomaticFailoverStatus
*/
public final AutomaticFailoverStatus automaticFailover() {
return AutomaticFailoverStatus.fromValue(automaticFailover);
}
/**
*
* Indicates the status of automatic failover for this Redis OSS replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of automatic failover for this Redis OSS replication group.
* @see AutomaticFailoverStatus
*/
public final String automaticFailoverAsString() {
return automaticFailover;
}
/**
*
* A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #multiAZ} will
* return {@link MultiAZStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #multiAZAsString}.
*
*
* @return A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ
* @see MultiAZStatus
*/
public final MultiAZStatus multiAZ() {
return MultiAZStatus.fromValue(multiAZ);
}
/**
*
* A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #multiAZ} will
* return {@link MultiAZStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #multiAZAsString}.
*
*
* @return A flag indicating if you have Multi-AZ enabled to enhance fault tolerance. For more information, see Minimizing Downtime:
* Multi-AZ
* @see MultiAZStatus
*/
public final String multiAZAsString() {
return multiAZ;
}
/**
*
* The configuration endpoint for this replication group. Use the configuration endpoint to connect to this
* replication group.
*
*
* @return The configuration endpoint for this replication group. Use the configuration endpoint to connect to this
* replication group.
*/
public final Endpoint configurationEndpoint() {
return configurationEndpoint;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A flag indicating whether or not this replication group is cluster enabled; i.e., whether its data can be
* partitioned across multiple shards (API/CLI: node groups).
*
*
* Valid values: true
| false
*
*
* @return A flag indicating whether or not this replication group is cluster enabled; i.e., whether its data can be
* partitioned across multiple shards (API/CLI: node groups).
*
* Valid values: true
| false
*/
public final Boolean clusterEnabled() {
return clusterEnabled;
}
/**
*
* The name of the compute and memory capacity node type for each node in the replication group.
*
*
* @return The name of the compute and memory capacity node type for each node in the replication group.
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* A flag that enables using an AuthToken
(password) when issuing Redis OSS commands.
*
*
* Default: false
*
*
* @return A flag that enables using an AuthToken
(password) when issuing Redis OSS commands.
*
* Default: false
*/
public final Boolean authTokenEnabled() {
return authTokenEnabled;
}
/**
*
* The date the auth token was last modified
*
*
* @return The date the auth token was last modified
*/
public final Instant authTokenLastModifiedDate() {
return authTokenLastModifiedDate;
}
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables in-transit encryption when set to true
.
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS
* version 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public final Boolean transitEncryptionEnabled() {
return transitEncryptionEnabled;
}
/**
*
* A flag that enables encryption at-rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To enable
* encryption at-rest on a cluster you must set AtRestEncryptionEnabled
to true
when you
* create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables encryption at-rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To
* enable encryption at-rest on a cluster you must set AtRestEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using Redis OSS
* version 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public final Boolean atRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
/**
* For responses, this returns true if the service returned a value for the MemberClustersOutpostArns property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasMemberClustersOutpostArns() {
return memberClustersOutpostArns != null && !(memberClustersOutpostArns instanceof SdkAutoConstructList);
}
/**
*
* The outpost ARNs of the replication group's member clusters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMemberClustersOutpostArns} method.
*
*
* @return The outpost ARNs of the replication group's member clusters.
*/
public final List memberClustersOutpostArns() {
return memberClustersOutpostArns;
}
/**
*
* The ID of the KMS key used to encrypt the disk in the cluster.
*
*
* @return The ID of the KMS key used to encrypt the disk in the cluster.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The ARN (Amazon Resource Name) of the replication group.
*
*
* @return The ARN (Amazon Resource Name) of the replication group.
*/
public final String arn() {
return arn;
}
/**
* For responses, this returns true if the service returned a value for the UserGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasUserGroupIds() {
return userGroupIds != null && !(userGroupIds instanceof SdkAutoConstructList);
}
/**
*
* The ID of the user group associated to the replication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserGroupIds} method.
*
*
* @return The ID of the user group associated to the replication group.
*/
public final List userGroupIds() {
return userGroupIds;
}
/**
* For responses, this returns true if the service returned a value for the LogDeliveryConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLogDeliveryConfigurations() {
return logDeliveryConfigurations != null && !(logDeliveryConfigurations instanceof SdkAutoConstructList);
}
/**
*
* Returns the destination, format and type of the logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLogDeliveryConfigurations} method.
*
*
* @return Returns the destination, format and type of the logs.
*/
public final List logDeliveryConfigurations() {
return logDeliveryConfigurations;
}
/**
*
* The date and time when the cluster was created.
*
*
* @return The date and time when the cluster was created.
*/
public final Instant replicationGroupCreateTime() {
return replicationGroupCreateTime;
}
/**
*
* Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type. This
* parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataTiering} will
* return {@link DataTieringStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTieringAsString}.
*
*
* @return Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type.
* This parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
* @see DataTieringStatus
*/
public final DataTieringStatus dataTiering() {
return DataTieringStatus.fromValue(dataTiering);
}
/**
*
* Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type. This
* parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataTiering} will
* return {@link DataTieringStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTieringAsString}.
*
*
* @return Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type.
* This parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
* @see DataTieringStatus
*/
public final String dataTieringAsString() {
return dataTiering;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in
* to the next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public final NetworkType networkType() {
return NetworkType.fromValue(networkType);
}
/**
*
* Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for workloads
* using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkType} will
* return {@link NetworkType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkTypeAsString}.
*
*
* @return Must be either ipv4
| ipv6
| dual_stack
. IPv6 is supported for
* workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all instances
* built on the Nitro system.
* @see NetworkType
*/
public final String networkTypeAsString() {
return networkType;
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public final IpDiscovery ipDiscovery() {
return IpDiscovery.fromValue(ipDiscovery);
}
/**
*
* The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6 is
* supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on all
* instances built on the Nitro system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipDiscovery} will
* return {@link IpDiscovery#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipDiscoveryAsString}.
*
*
* @return The network type you choose when modifying a cluster, either ipv4
| ipv6
. IPv6
* is supported for workloads using Redis OSS engine version 6.2 onward or Memcached engine version 1.6.6 on
* all instances built on the Nitro system.
* @see IpDiscovery
*/
public final String ipDiscoveryAsString() {
return ipDiscovery;
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public final TransitEncryptionMode transitEncryptionMode() {
return TransitEncryptionMode.fromValue(transitEncryptionMode);
}
/**
*
* A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #transitEncryptionMode} will return {@link TransitEncryptionMode#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #transitEncryptionModeAsString}.
*
*
* @return A setting that allows you to migrate your clients to use in-transit encryption, with no downtime.
* @see TransitEncryptionMode
*/
public final String transitEncryptionModeAsString() {
return transitEncryptionMode;
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterMode} will
* return {@link ClusterMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterModeAsString}.
*
*
* @return Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public final ClusterMode clusterMode() {
return ClusterMode.fromValue(clusterMode);
}
/**
*
* Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode to
* Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled and cluster
* mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can then complete cluster
* mode configuration and set the cluster mode to Enabled.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterMode} will
* return {@link ClusterMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterModeAsString}.
*
*
* @return Enabled or Disabled. To modify cluster mode from Disabled to Enabled, you must first set the cluster mode
* to Compatible. Compatible mode allows your Redis OSS clients to connect using both cluster mode enabled
* and cluster mode disabled. After you migrate all Redis OSS clients to use cluster mode enabled, you can
* then complete cluster mode configuration and set the cluster mode to Enabled.
* @see ClusterMode
*/
public final String clusterModeAsString() {
return clusterMode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(globalReplicationGroupInfo());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(pendingModifiedValues());
hashCode = 31 * hashCode + Objects.hashCode(hasMemberClusters() ? memberClusters() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNodeGroups() ? nodeGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(snapshottingClusterId());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverAsString());
hashCode = 31 * hashCode + Objects.hashCode(multiAZAsString());
hashCode = 31 * hashCode + Objects.hashCode(configurationEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(clusterEnabled());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(authTokenEnabled());
hashCode = 31 * hashCode + Objects.hashCode(authTokenLastModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(atRestEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(hasMemberClustersOutpostArns() ? memberClustersOutpostArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(hasUserGroupIds() ? userGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(dataTieringAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(networkTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipDiscoveryAsString());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(clusterModeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationGroup)) {
return false;
}
ReplicationGroup other = (ReplicationGroup) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(description(), other.description())
&& Objects.equals(globalReplicationGroupInfo(), other.globalReplicationGroupInfo())
&& Objects.equals(status(), other.status())
&& Objects.equals(pendingModifiedValues(), other.pendingModifiedValues())
&& hasMemberClusters() == other.hasMemberClusters() && Objects.equals(memberClusters(), other.memberClusters())
&& hasNodeGroups() == other.hasNodeGroups() && Objects.equals(nodeGroups(), other.nodeGroups())
&& Objects.equals(snapshottingClusterId(), other.snapshottingClusterId())
&& Objects.equals(automaticFailoverAsString(), other.automaticFailoverAsString())
&& Objects.equals(multiAZAsString(), other.multiAZAsString())
&& Objects.equals(configurationEndpoint(), other.configurationEndpoint())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(clusterEnabled(), other.clusterEnabled())
&& Objects.equals(cacheNodeType(), other.cacheNodeType())
&& Objects.equals(authTokenEnabled(), other.authTokenEnabled())
&& Objects.equals(authTokenLastModifiedDate(), other.authTokenLastModifiedDate())
&& Objects.equals(transitEncryptionEnabled(), other.transitEncryptionEnabled())
&& Objects.equals(atRestEncryptionEnabled(), other.atRestEncryptionEnabled())
&& hasMemberClustersOutpostArns() == other.hasMemberClustersOutpostArns()
&& Objects.equals(memberClustersOutpostArns(), other.memberClustersOutpostArns())
&& Objects.equals(kmsKeyId(), other.kmsKeyId()) && Objects.equals(arn(), other.arn())
&& hasUserGroupIds() == other.hasUserGroupIds() && Objects.equals(userGroupIds(), other.userGroupIds())
&& hasLogDeliveryConfigurations() == other.hasLogDeliveryConfigurations()
&& Objects.equals(logDeliveryConfigurations(), other.logDeliveryConfigurations())
&& Objects.equals(replicationGroupCreateTime(), other.replicationGroupCreateTime())
&& Objects.equals(dataTieringAsString(), other.dataTieringAsString())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(networkTypeAsString(), other.networkTypeAsString())
&& Objects.equals(ipDiscoveryAsString(), other.ipDiscoveryAsString())
&& Objects.equals(transitEncryptionModeAsString(), other.transitEncryptionModeAsString())
&& Objects.equals(clusterModeAsString(), other.clusterModeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReplicationGroup").add("ReplicationGroupId", replicationGroupId())
.add("Description", description()).add("GlobalReplicationGroupInfo", globalReplicationGroupInfo())
.add("Status", status()).add("PendingModifiedValues", pendingModifiedValues())
.add("MemberClusters", hasMemberClusters() ? memberClusters() : null)
.add("NodeGroups", hasNodeGroups() ? nodeGroups() : null).add("SnapshottingClusterId", snapshottingClusterId())
.add("AutomaticFailover", automaticFailoverAsString()).add("MultiAZ", multiAZAsString())
.add("ConfigurationEndpoint", configurationEndpoint()).add("SnapshotRetentionLimit", snapshotRetentionLimit())
.add("SnapshotWindow", snapshotWindow()).add("ClusterEnabled", clusterEnabled())
.add("CacheNodeType", cacheNodeType()).add("AuthTokenEnabled", authTokenEnabled())
.add("AuthTokenLastModifiedDate", authTokenLastModifiedDate())
.add("TransitEncryptionEnabled", transitEncryptionEnabled())
.add("AtRestEncryptionEnabled", atRestEncryptionEnabled())
.add("MemberClustersOutpostArns", hasMemberClustersOutpostArns() ? memberClustersOutpostArns() : null)
.add("KmsKeyId", kmsKeyId()).add("ARN", arn()).add("UserGroupIds", hasUserGroupIds() ? userGroupIds() : null)
.add("LogDeliveryConfigurations", hasLogDeliveryConfigurations() ? logDeliveryConfigurations() : null)
.add("ReplicationGroupCreateTime", replicationGroupCreateTime()).add("DataTiering", dataTieringAsString())
.add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade()).add("NetworkType", networkTypeAsString())
.add("IpDiscovery", ipDiscoveryAsString()).add("TransitEncryptionMode", transitEncryptionModeAsString())
.add("ClusterMode", clusterModeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "GlobalReplicationGroupInfo":
return Optional.ofNullable(clazz.cast(globalReplicationGroupInfo()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "PendingModifiedValues":
return Optional.ofNullable(clazz.cast(pendingModifiedValues()));
case "MemberClusters":
return Optional.ofNullable(clazz.cast(memberClusters()));
case "NodeGroups":
return Optional.ofNullable(clazz.cast(nodeGroups()));
case "SnapshottingClusterId":
return Optional.ofNullable(clazz.cast(snapshottingClusterId()));
case "AutomaticFailover":
return Optional.ofNullable(clazz.cast(automaticFailoverAsString()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZAsString()));
case "ConfigurationEndpoint":
return Optional.ofNullable(clazz.cast(configurationEndpoint()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "ClusterEnabled":
return Optional.ofNullable(clazz.cast(clusterEnabled()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "AuthTokenEnabled":
return Optional.ofNullable(clazz.cast(authTokenEnabled()));
case "AuthTokenLastModifiedDate":
return Optional.ofNullable(clazz.cast(authTokenLastModifiedDate()));
case "TransitEncryptionEnabled":
return Optional.ofNullable(clazz.cast(transitEncryptionEnabled()));
case "AtRestEncryptionEnabled":
return Optional.ofNullable(clazz.cast(atRestEncryptionEnabled()));
case "MemberClustersOutpostArns":
return Optional.ofNullable(clazz.cast(memberClustersOutpostArns()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "UserGroupIds":
return Optional.ofNullable(clazz.cast(userGroupIds()));
case "LogDeliveryConfigurations":
return Optional.ofNullable(clazz.cast(logDeliveryConfigurations()));
case "ReplicationGroupCreateTime":
return Optional.ofNullable(clazz.cast(replicationGroupCreateTime()));
case "DataTiering":
return Optional.ofNullable(clazz.cast(dataTieringAsString()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "NetworkType":
return Optional.ofNullable(clazz.cast(networkTypeAsString()));
case "IpDiscovery":
return Optional.ofNullable(clazz.cast(ipDiscoveryAsString()));
case "TransitEncryptionMode":
return Optional.ofNullable(clazz.cast(transitEncryptionModeAsString()));
case "ClusterMode":
return Optional.ofNullable(clazz.cast(clusterModeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function