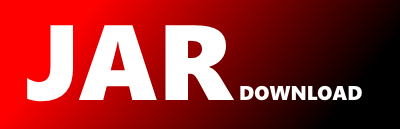
software.amazon.awssdk.services.elasticache.model.Snapshot Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a copy of an entire Redis OSS cluster as of the time when the snapshot was taken.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Snapshot implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SNAPSHOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotName").getter(getter(Snapshot::snapshotName)).setter(setter(Builder::snapshotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotName").build()).build();
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(Snapshot::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField REPLICATION_GROUP_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationGroupDescription")
.getter(getter(Snapshot::replicationGroupDescription))
.setter(setter(Builder::replicationGroupDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupDescription")
.build()).build();
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheClusterId").getter(getter(Snapshot::cacheClusterId)).setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField SNAPSHOT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotStatus").getter(getter(Snapshot::snapshotStatus)).setter(setter(Builder::snapshotStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotStatus").build()).build();
private static final SdkField SNAPSHOT_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotSource").getter(getter(Snapshot::snapshotSource)).setter(setter(Builder::snapshotSource))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotSource").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheNodeType").getter(getter(Snapshot::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(Snapshot::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(Snapshot::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField NUM_CACHE_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumCacheNodes").getter(getter(Snapshot::numCacheNodes)).setter(setter(Builder::numCacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheNodes").build()).build();
private static final SdkField PREFERRED_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredAvailabilityZone").getter(getter(Snapshot::preferredAvailabilityZone))
.setter(setter(Builder::preferredAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZone").build())
.build();
private static final SdkField PREFERRED_OUTPOST_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredOutpostArn").getter(getter(Snapshot::preferredOutpostArn))
.setter(setter(Builder::preferredOutpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredOutpostArn").build())
.build();
private static final SdkField CACHE_CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CacheClusterCreateTime").getter(getter(Snapshot::cacheClusterCreateTime))
.setter(setter(Builder::cacheClusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterCreateTime").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(Snapshot::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TopicArn").getter(getter(Snapshot::topicArn)).setter(setter(Builder::topicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TopicArn").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(Snapshot::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField CACHE_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheParameterGroupName").getter(getter(Snapshot::cacheParameterGroupName))
.setter(setter(Builder::cacheParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroupName").build())
.build();
private static final SdkField CACHE_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CacheSubnetGroupName").getter(getter(Snapshot::cacheSubnetGroupName))
.setter(setter(Builder::cacheSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSubnetGroupName").build())
.build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(Snapshot::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(Snapshot::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SnapshotRetentionLimit").getter(getter(Snapshot::snapshotRetentionLimit))
.setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWindow").getter(getter(Snapshot::snapshotWindow)).setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField NUM_NODE_GROUPS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumNodeGroups").getter(getter(Snapshot::numNodeGroups)).setter(setter(Builder::numNodeGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumNodeGroups").build()).build();
private static final SdkField AUTOMATIC_FAILOVER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutomaticFailover").getter(getter(Snapshot::automaticFailoverAsString))
.setter(setter(Builder::automaticFailover))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailover").build()).build();
private static final SdkField> NODE_SNAPSHOTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NodeSnapshots")
.getter(getter(Snapshot::nodeSnapshots))
.setter(setter(Builder::nodeSnapshots))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeSnapshots").build(),
ListTrait
.builder()
.memberLocationName("NodeSnapshot")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NodeSnapshot::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeSnapshot").build()).build()).build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(Snapshot::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(Snapshot::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField DATA_TIERING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataTiering").getter(getter(Snapshot::dataTieringAsString)).setter(setter(Builder::dataTiering))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataTiering").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SNAPSHOT_NAME_FIELD,
REPLICATION_GROUP_ID_FIELD, REPLICATION_GROUP_DESCRIPTION_FIELD, CACHE_CLUSTER_ID_FIELD, SNAPSHOT_STATUS_FIELD,
SNAPSHOT_SOURCE_FIELD, CACHE_NODE_TYPE_FIELD, ENGINE_FIELD, ENGINE_VERSION_FIELD, NUM_CACHE_NODES_FIELD,
PREFERRED_AVAILABILITY_ZONE_FIELD, PREFERRED_OUTPOST_ARN_FIELD, CACHE_CLUSTER_CREATE_TIME_FIELD,
PREFERRED_MAINTENANCE_WINDOW_FIELD, TOPIC_ARN_FIELD, PORT_FIELD, CACHE_PARAMETER_GROUP_NAME_FIELD,
CACHE_SUBNET_GROUP_NAME_FIELD, VPC_ID_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD,
SNAPSHOT_WINDOW_FIELD, NUM_NODE_GROUPS_FIELD, AUTOMATIC_FAILOVER_FIELD, NODE_SNAPSHOTS_FIELD, KMS_KEY_ID_FIELD,
ARN_FIELD, DATA_TIERING_FIELD));
private static final long serialVersionUID = 1L;
private final String snapshotName;
private final String replicationGroupId;
private final String replicationGroupDescription;
private final String cacheClusterId;
private final String snapshotStatus;
private final String snapshotSource;
private final String cacheNodeType;
private final String engine;
private final String engineVersion;
private final Integer numCacheNodes;
private final String preferredAvailabilityZone;
private final String preferredOutpostArn;
private final Instant cacheClusterCreateTime;
private final String preferredMaintenanceWindow;
private final String topicArn;
private final Integer port;
private final String cacheParameterGroupName;
private final String cacheSubnetGroupName;
private final String vpcId;
private final Boolean autoMinorVersionUpgrade;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final Integer numNodeGroups;
private final String automaticFailover;
private final List nodeSnapshots;
private final String kmsKeyId;
private final String arn;
private final String dataTiering;
private Snapshot(BuilderImpl builder) {
this.snapshotName = builder.snapshotName;
this.replicationGroupId = builder.replicationGroupId;
this.replicationGroupDescription = builder.replicationGroupDescription;
this.cacheClusterId = builder.cacheClusterId;
this.snapshotStatus = builder.snapshotStatus;
this.snapshotSource = builder.snapshotSource;
this.cacheNodeType = builder.cacheNodeType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.numCacheNodes = builder.numCacheNodes;
this.preferredAvailabilityZone = builder.preferredAvailabilityZone;
this.preferredOutpostArn = builder.preferredOutpostArn;
this.cacheClusterCreateTime = builder.cacheClusterCreateTime;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.topicArn = builder.topicArn;
this.port = builder.port;
this.cacheParameterGroupName = builder.cacheParameterGroupName;
this.cacheSubnetGroupName = builder.cacheSubnetGroupName;
this.vpcId = builder.vpcId;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.numNodeGroups = builder.numNodeGroups;
this.automaticFailover = builder.automaticFailover;
this.nodeSnapshots = builder.nodeSnapshots;
this.kmsKeyId = builder.kmsKeyId;
this.arn = builder.arn;
this.dataTiering = builder.dataTiering;
}
/**
*
* The name of a snapshot. For an automatic snapshot, the name is system-generated. For a manual snapshot, this is
* the user-provided name.
*
*
* @return The name of a snapshot. For an automatic snapshot, the name is system-generated. For a manual snapshot,
* this is the user-provided name.
*/
public final String snapshotName() {
return snapshotName;
}
/**
*
* The unique identifier of the source replication group.
*
*
* @return The unique identifier of the source replication group.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* A description of the source replication group.
*
*
* @return A description of the source replication group.
*/
public final String replicationGroupDescription() {
return replicationGroupDescription;
}
/**
*
* The user-supplied identifier of the source cluster.
*
*
* @return The user-supplied identifier of the source cluster.
*/
public final String cacheClusterId() {
return cacheClusterId;
}
/**
*
* The status of the snapshot. Valid values: creating
| available
| restoring
* | copying
| deleting
.
*
*
* @return The status of the snapshot. Valid values: creating
| available
|
* restoring
| copying
| deleting
.
*/
public final String snapshotStatus() {
return snapshotStatus;
}
/**
*
* Indicates whether the snapshot is from an automatic backup (automated
) or was created manually (
* manual
).
*
*
* @return Indicates whether the snapshot is from an automatic backup (automated
) or was created
* manually (manual
).
*/
public final String snapshotSource() {
return snapshotSource;
}
/**
*
* The name of the compute and memory capacity node type for the source cluster.
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
, cache.m6g.2xlarge
,
* cache.m6g.4xlarge
, cache.m6g.8xlarge
, cache.m6g.12xlarge
,
* cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
, cache.m5.2xlarge
,
* cache.m5.4xlarge
, cache.m5.12xlarge
, cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine version
* 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
, cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
, cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine version
* 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
, cache.r6g.2xlarge
,
* cache.r6g.4xlarge
, cache.r6g.8xlarge
, cache.r6g.12xlarge
,
* cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
, cache.r5.2xlarge
,
* cache.r5.4xlarge
, cache.r5.12xlarge
, cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters is not
* supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported on Redis
* OSS version 2.8.22 and later.
*
*
*
*
* @return The name of the compute and memory capacity node type for the source cluster.
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* M7g node types: cache.m7g.large
, cache.m7g.xlarge
,
* cache.m7g.2xlarge
, cache.m7g.4xlarge
, cache.m7g.8xlarge
,
* cache.m7g.12xlarge
, cache.m7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* M6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.m6g.large
, cache.m6g.xlarge
,
* cache.m6g.2xlarge
, cache.m6g.4xlarge
, cache.m6g.8xlarge
,
* cache.m6g.12xlarge
, cache.m6g.16xlarge
*
*
* M5 node types: cache.m5.large
, cache.m5.xlarge
,
* cache.m5.2xlarge
, cache.m5.4xlarge
, cache.m5.12xlarge
,
* cache.m5.24xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* T4g node types (available only for Redis OSS engine version 5.0.6 onward and Memcached engine
* version 1.5.16 onward): cache.t4g.micro
, cache.t4g.small
,
* cache.t4g.medium
*
*
* T3 node types: cache.t3.micro
, cache.t3.small
,
* cache.t3.medium
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R7g node types: cache.r7g.large
, cache.r7g.xlarge
,
* cache.r7g.2xlarge
, cache.r7g.4xlarge
, cache.r7g.8xlarge
,
* cache.r7g.12xlarge
, cache.r7g.16xlarge
*
*
*
* For region availability, see Supported Node Types
*
*
*
* R6g node types (available only for Redis OSS engine version 5.0.6 onward and for Memcached engine
* version 1.5.16 onward): cache.r6g.large
, cache.r6g.xlarge
,
* cache.r6g.2xlarge
, cache.r6g.4xlarge
, cache.r6g.8xlarge
,
* cache.r6g.12xlarge
, cache.r6g.16xlarge
*
*
* R5 node types: cache.r5.large
, cache.r5.xlarge
,
* cache.r5.2xlarge
, cache.r5.4xlarge
, cache.r5.12xlarge
,
* cache.r5.24xlarge
*
*
* R4 node types: cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended. Existing clusters are still supported but creation of new clusters
* is not supported for these types.)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
*
*
*
*
* Additional node type info
*
*
* -
*
* All current generation instance types are created in Amazon VPC by default.
*
*
* -
*
* Redis OSS append-only files (AOF) are not supported for T1 or T2 instances.
*
*
* -
*
* Redis OSS Multi-AZ with automatic failover is not supported on T1 instances.
*
*
* -
*
* Redis OSS configuration variables appendonly
and appendfsync
are not supported
* on Redis OSS version 2.8.22 and later.
*
*
*/
public final String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The name of the cache engine (memcached
or redis
) used by the source cluster.
*
*
* @return The name of the cache engine (memcached
or redis
) used by the source cluster.
*/
public final String engine() {
return engine;
}
/**
*
* The version of the cache engine version that is used by the source cluster.
*
*
* @return The version of the cache engine version that is used by the source cluster.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The number of cache nodes in the source cluster.
*
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 40.
*
*
* @return The number of cache nodes in the source cluster.
*
* For clusters running Redis OSS, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 40.
*/
public final Integer numCacheNodes() {
return numCacheNodes;
}
/**
*
* The name of the Availability Zone in which the source cluster is located.
*
*
* @return The name of the Availability Zone in which the source cluster is located.
*/
public final String preferredAvailabilityZone() {
return preferredAvailabilityZone;
}
/**
*
* The ARN (Amazon Resource Name) of the preferred outpost.
*
*
* @return The ARN (Amazon Resource Name) of the preferred outpost.
*/
public final String preferredOutpostArn() {
return preferredOutpostArn;
}
/**
*
* The date and time when the source cluster was created.
*
*
* @return The date and time when the source cluster was created.
*/
public final Instant cacheClusterCreateTime() {
return cacheClusterCreateTime;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The Amazon Resource Name (ARN) for the topic used by the source cluster for publishing notifications.
*
*
* @return The Amazon Resource Name (ARN) for the topic used by the source cluster for publishing notifications.
*/
public final String topicArn() {
return topicArn;
}
/**
*
* The port number used by each cache nodes in the source cluster.
*
*
* @return The port number used by each cache nodes in the source cluster.
*/
public final Integer port() {
return port;
}
/**
*
* The cache parameter group that is associated with the source cluster.
*
*
* @return The cache parameter group that is associated with the source cluster.
*/
public final String cacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
*
* The name of the cache subnet group associated with the source cluster.
*
*
* @return The name of the cache subnet group associated with the source cluster.
*/
public final String cacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
*
* The Amazon Virtual Private Cloud identifier (VPC ID) of the cache subnet group for the source cluster.
*
*
* @return The Amazon Virtual Private Cloud identifier (VPC ID) of the cache subnet group for the source cluster.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to opt-in to the
* next auto minor version upgrade campaign. This parameter is disabled for previous versions.
*
*
* @return If you are running Redis OSS engine version 6.0 or later, set this parameter to yes if you want to
* opt-in to the next auto minor version upgrade campaign. This parameter is disabled for previous
* versions.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* For an automatic snapshot, the number of days for which ElastiCache retains the snapshot before deleting it.
*
*
* For manual snapshots, this field reflects the SnapshotRetentionLimit
for the source cluster when the
* snapshot was created. This field is otherwise ignored: Manual snapshots do not expire, and can only be deleted
* using the DeleteSnapshot
operation.
*
*
* Important If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*
* @return For an automatic snapshot, the number of days for which ElastiCache retains the snapshot before deleting
* it.
*
* For manual snapshots, this field reflects the SnapshotRetentionLimit
for the source cluster
* when the snapshot was created. This field is otherwise ignored: Manual snapshots do not expire, and can
* only be deleted using the DeleteSnapshot
operation.
*
*
* Important If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*/
public final Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range during which ElastiCache takes daily snapshots of the source cluster.
*
*
* @return The daily time range during which ElastiCache takes daily snapshots of the source cluster.
*/
public final String snapshotWindow() {
return snapshotWindow;
}
/**
*
* The number of node groups (shards) in this snapshot. When restoring from a snapshot, the number of node groups
* (shards) in the snapshot and in the restored replication group must be the same.
*
*
* @return The number of node groups (shards) in this snapshot. When restoring from a snapshot, the number of node
* groups (shards) in the snapshot and in the restored replication group must be the same.
*/
public final Integer numNodeGroups() {
return numNodeGroups;
}
/**
*
* Indicates the status of automatic failover for the source Redis OSS replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of automatic failover for the source Redis OSS replication group.
* @see AutomaticFailoverStatus
*/
public final AutomaticFailoverStatus automaticFailover() {
return AutomaticFailoverStatus.fromValue(automaticFailover);
}
/**
*
* Indicates the status of automatic failover for the source Redis OSS replication group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of automatic failover for the source Redis OSS replication group.
* @see AutomaticFailoverStatus
*/
public final String automaticFailoverAsString() {
return automaticFailover;
}
/**
* For responses, this returns true if the service returned a value for the NodeSnapshots property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNodeSnapshots() {
return nodeSnapshots != null && !(nodeSnapshots instanceof SdkAutoConstructList);
}
/**
*
* A list of the cache nodes in the source cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNodeSnapshots} method.
*
*
* @return A list of the cache nodes in the source cluster.
*/
public final List nodeSnapshots() {
return nodeSnapshots;
}
/**
*
* The ID of the KMS key used to encrypt the snapshot.
*
*
* @return The ID of the KMS key used to encrypt the snapshot.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The ARN (Amazon Resource Name) of the snapshot.
*
*
* @return The ARN (Amazon Resource Name) of the snapshot.
*/
public final String arn() {
return arn;
}
/**
*
* Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type. This
* parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataTiering} will
* return {@link DataTieringStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTieringAsString}.
*
*
* @return Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type.
* This parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
* @see DataTieringStatus
*/
public final DataTieringStatus dataTiering() {
return DataTieringStatus.fromValue(dataTiering);
}
/**
*
* Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type. This
* parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataTiering} will
* return {@link DataTieringStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTieringAsString}.
*
*
* @return Enables data tiering. Data tiering is only supported for replication groups using the r6gd node type.
* This parameter must be set to true when using r6gd nodes. For more information, see Data tiering.
* @see DataTieringStatus
*/
public final String dataTieringAsString() {
return dataTiering;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(snapshotName());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupDescription());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(snapshotStatus());
hashCode = 31 * hashCode + Objects.hashCode(snapshotSource());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(numCacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(preferredAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(preferredOutpostArn());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(topicArn());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cacheSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(numNodeGroups());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasNodeSnapshots() ? nodeSnapshots() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(dataTieringAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Snapshot)) {
return false;
}
Snapshot other = (Snapshot) obj;
return Objects.equals(snapshotName(), other.snapshotName())
&& Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(replicationGroupDescription(), other.replicationGroupDescription())
&& Objects.equals(cacheClusterId(), other.cacheClusterId())
&& Objects.equals(snapshotStatus(), other.snapshotStatus())
&& Objects.equals(snapshotSource(), other.snapshotSource())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(numCacheNodes(), other.numCacheNodes())
&& Objects.equals(preferredAvailabilityZone(), other.preferredAvailabilityZone())
&& Objects.equals(preferredOutpostArn(), other.preferredOutpostArn())
&& Objects.equals(cacheClusterCreateTime(), other.cacheClusterCreateTime())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(topicArn(), other.topicArn()) && Objects.equals(port(), other.port())
&& Objects.equals(cacheParameterGroupName(), other.cacheParameterGroupName())
&& Objects.equals(cacheSubnetGroupName(), other.cacheSubnetGroupName()) && Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(numNodeGroups(), other.numNodeGroups())
&& Objects.equals(automaticFailoverAsString(), other.automaticFailoverAsString())
&& hasNodeSnapshots() == other.hasNodeSnapshots() && Objects.equals(nodeSnapshots(), other.nodeSnapshots())
&& Objects.equals(kmsKeyId(), other.kmsKeyId()) && Objects.equals(arn(), other.arn())
&& Objects.equals(dataTieringAsString(), other.dataTieringAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Snapshot").add("SnapshotName", snapshotName()).add("ReplicationGroupId", replicationGroupId())
.add("ReplicationGroupDescription", replicationGroupDescription()).add("CacheClusterId", cacheClusterId())
.add("SnapshotStatus", snapshotStatus()).add("SnapshotSource", snapshotSource())
.add("CacheNodeType", cacheNodeType()).add("Engine", engine()).add("EngineVersion", engineVersion())
.add("NumCacheNodes", numCacheNodes()).add("PreferredAvailabilityZone", preferredAvailabilityZone())
.add("PreferredOutpostArn", preferredOutpostArn()).add("CacheClusterCreateTime", cacheClusterCreateTime())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("TopicArn", topicArn()).add("Port", port())
.add("CacheParameterGroupName", cacheParameterGroupName()).add("CacheSubnetGroupName", cacheSubnetGroupName())
.add("VpcId", vpcId()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("NumNodeGroups", numNodeGroups()).add("AutomaticFailover", automaticFailoverAsString())
.add("NodeSnapshots", hasNodeSnapshots() ? nodeSnapshots() : null).add("KmsKeyId", kmsKeyId()).add("ARN", arn())
.add("DataTiering", dataTieringAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SnapshotName":
return Optional.ofNullable(clazz.cast(snapshotName()));
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "ReplicationGroupDescription":
return Optional.ofNullable(clazz.cast(replicationGroupDescription()));
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "SnapshotStatus":
return Optional.ofNullable(clazz.cast(snapshotStatus()));
case "SnapshotSource":
return Optional.ofNullable(clazz.cast(snapshotSource()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "NumCacheNodes":
return Optional.ofNullable(clazz.cast(numCacheNodes()));
case "PreferredAvailabilityZone":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZone()));
case "PreferredOutpostArn":
return Optional.ofNullable(clazz.cast(preferredOutpostArn()));
case "CacheClusterCreateTime":
return Optional.ofNullable(clazz.cast(cacheClusterCreateTime()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "TopicArn":
return Optional.ofNullable(clazz.cast(topicArn()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "CacheParameterGroupName":
return Optional.ofNullable(clazz.cast(cacheParameterGroupName()));
case "CacheSubnetGroupName":
return Optional.ofNullable(clazz.cast(cacheSubnetGroupName()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "NumNodeGroups":
return Optional.ofNullable(clazz.cast(numNodeGroups()));
case "AutomaticFailover":
return Optional.ofNullable(clazz.cast(automaticFailoverAsString()));
case "NodeSnapshots":
return Optional.ofNullable(clazz.cast(nodeSnapshots()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "DataTiering":
return Optional.ofNullable(clazz.cast(dataTieringAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function