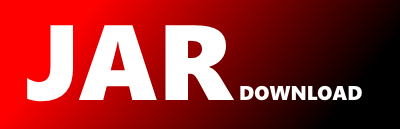
software.amazon.awssdk.services.elasticache.DefaultElastiCacheClient Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.elasticache.internal.ElastiCacheServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.elasticache.model.AddTagsToResourceRequest;
import software.amazon.awssdk.services.elasticache.model.AddTagsToResourceResponse;
import software.amazon.awssdk.services.elasticache.model.ApiCallRateForCustomerExceededException;
import software.amazon.awssdk.services.elasticache.model.AuthorizationAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.AuthorizationNotFoundException;
import software.amazon.awssdk.services.elasticache.model.AuthorizeCacheSecurityGroupIngressRequest;
import software.amazon.awssdk.services.elasticache.model.AuthorizeCacheSecurityGroupIngressResponse;
import software.amazon.awssdk.services.elasticache.model.BatchApplyUpdateActionRequest;
import software.amazon.awssdk.services.elasticache.model.BatchApplyUpdateActionResponse;
import software.amazon.awssdk.services.elasticache.model.BatchStopUpdateActionRequest;
import software.amazon.awssdk.services.elasticache.model.BatchStopUpdateActionResponse;
import software.amazon.awssdk.services.elasticache.model.CacheClusterAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.CacheClusterNotFoundException;
import software.amazon.awssdk.services.elasticache.model.CacheParameterGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.CacheParameterGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.CacheParameterGroupQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.CacheSecurityGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.CacheSecurityGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.CacheSecurityGroupQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.CacheSubnetGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.CacheSubnetGroupInUseException;
import software.amazon.awssdk.services.elasticache.model.CacheSubnetGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.CacheSubnetGroupQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.CacheSubnetQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.ClusterQuotaForCustomerExceededException;
import software.amazon.awssdk.services.elasticache.model.CompleteMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.CompleteMigrationResponse;
import software.amazon.awssdk.services.elasticache.model.CopyServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CopyServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CopySnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CopySnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSecurityGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSecurityGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CreateSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateUserRequest;
import software.amazon.awssdk.services.elasticache.model.CreateUserResponse;
import software.amazon.awssdk.services.elasticache.model.DecreaseNodeGroupsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DecreaseNodeGroupsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountRequest;
import software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountResponse;
import software.amazon.awssdk.services.elasticache.model.DefaultUserAssociatedToUserGroupException;
import software.amazon.awssdk.services.elasticache.model.DefaultUserRequiredException;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSecurityGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSecurityGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteUserRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteUserResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeEventsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUsersResponse;
import software.amazon.awssdk.services.elasticache.model.DisassociateGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DisassociateGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DuplicateUserNameException;
import software.amazon.awssdk.services.elasticache.model.ElastiCacheException;
import software.amazon.awssdk.services.elasticache.model.ExportServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.ExportServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.FailoverGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.FailoverGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.GlobalReplicationGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.GlobalReplicationGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.IncreaseNodeGroupsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.IncreaseNodeGroupsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.IncreaseReplicaCountRequest;
import software.amazon.awssdk.services.elasticache.model.IncreaseReplicaCountResponse;
import software.amazon.awssdk.services.elasticache.model.InsufficientCacheClusterCapacityException;
import software.amazon.awssdk.services.elasticache.model.InvalidArnException;
import software.amazon.awssdk.services.elasticache.model.InvalidCacheClusterStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidCacheParameterGroupStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidCacheSecurityGroupStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidCredentialsException;
import software.amazon.awssdk.services.elasticache.model.InvalidGlobalReplicationGroupStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidKmsKeyException;
import software.amazon.awssdk.services.elasticache.model.InvalidParameterCombinationException;
import software.amazon.awssdk.services.elasticache.model.InvalidParameterValueException;
import software.amazon.awssdk.services.elasticache.model.InvalidReplicationGroupStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidServerlessCacheSnapshotStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidServerlessCacheStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidSnapshotStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidSubnetException;
import software.amazon.awssdk.services.elasticache.model.InvalidUserGroupStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidUserStateException;
import software.amazon.awssdk.services.elasticache.model.InvalidVpcNetworkStateException;
import software.amazon.awssdk.services.elasticache.model.ListAllowedNodeTypeModificationsRequest;
import software.amazon.awssdk.services.elasticache.model.ListAllowedNodeTypeModificationsResponse;
import software.amazon.awssdk.services.elasticache.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.elasticache.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyUserRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyUserResponse;
import software.amazon.awssdk.services.elasticache.model.NoOperationException;
import software.amazon.awssdk.services.elasticache.model.NodeGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.NodeGroupsPerReplicationGroupQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.NodeQuotaForClusterExceededException;
import software.amazon.awssdk.services.elasticache.model.NodeQuotaForCustomerExceededException;
import software.amazon.awssdk.services.elasticache.model.PurchaseReservedCacheNodesOfferingRequest;
import software.amazon.awssdk.services.elasticache.model.PurchaseReservedCacheNodesOfferingResponse;
import software.amazon.awssdk.services.elasticache.model.RebalanceSlotsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.RebalanceSlotsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.RebootCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.RebootCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.RemoveTagsFromResourceRequest;
import software.amazon.awssdk.services.elasticache.model.RemoveTagsFromResourceResponse;
import software.amazon.awssdk.services.elasticache.model.ReplicationGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.ReplicationGroupAlreadyUnderMigrationException;
import software.amazon.awssdk.services.elasticache.model.ReplicationGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ReplicationGroupNotUnderMigrationException;
import software.amazon.awssdk.services.elasticache.model.ReservedCacheNodeAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.ReservedCacheNodeNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ReservedCacheNodeQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.ReservedCacheNodesOfferingNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ResetCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ResetCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.RevokeCacheSecurityGroupIngressRequest;
import software.amazon.awssdk.services.elasticache.model.RevokeCacheSecurityGroupIngressResponse;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheQuotaForCustomerExceededException;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheSnapshotAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheSnapshotNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ServerlessCacheSnapshotQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.ServiceLinkedRoleNotFoundException;
import software.amazon.awssdk.services.elasticache.model.ServiceUpdateNotFoundException;
import software.amazon.awssdk.services.elasticache.model.SnapshotAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.SnapshotFeatureNotSupportedException;
import software.amazon.awssdk.services.elasticache.model.SnapshotNotFoundException;
import software.amazon.awssdk.services.elasticache.model.SnapshotQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.StartMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.StartMigrationResponse;
import software.amazon.awssdk.services.elasticache.model.SubnetInUseException;
import software.amazon.awssdk.services.elasticache.model.SubnetNotAllowedException;
import software.amazon.awssdk.services.elasticache.model.TagNotFoundException;
import software.amazon.awssdk.services.elasticache.model.TagQuotaPerResourceExceededException;
import software.amazon.awssdk.services.elasticache.model.TestFailoverNotAvailableException;
import software.amazon.awssdk.services.elasticache.model.TestFailoverRequest;
import software.amazon.awssdk.services.elasticache.model.TestFailoverResponse;
import software.amazon.awssdk.services.elasticache.model.TestMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.TestMigrationResponse;
import software.amazon.awssdk.services.elasticache.model.UserAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.UserGroupAlreadyExistsException;
import software.amazon.awssdk.services.elasticache.model.UserGroupNotFoundException;
import software.amazon.awssdk.services.elasticache.model.UserGroupQuotaExceededException;
import software.amazon.awssdk.services.elasticache.model.UserNotFoundException;
import software.amazon.awssdk.services.elasticache.model.UserQuotaExceededException;
import software.amazon.awssdk.services.elasticache.transform.AddTagsToResourceRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.AuthorizeCacheSecurityGroupIngressRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.BatchApplyUpdateActionRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.BatchStopUpdateActionRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CompleteMigrationRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CopyServerlessCacheSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CopySnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateCacheClusterRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateCacheParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateCacheSecurityGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateCacheSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateServerlessCacheRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateServerlessCacheSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateUserGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DecreaseNodeGroupsInGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DecreaseReplicaCountRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteCacheClusterRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteCacheParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteCacheSecurityGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteCacheSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteServerlessCacheRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteServerlessCacheSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteUserGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheClustersRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheEngineVersionsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheParameterGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheParametersRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheSecurityGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeCacheSubnetGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeEngineDefaultParametersRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeEventsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeGlobalReplicationGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeReplicationGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeReservedCacheNodesOfferingsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeReservedCacheNodesRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeServerlessCacheSnapshotsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeServerlessCachesRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeServiceUpdatesRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeSnapshotsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeUpdateActionsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeUserGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DescribeUsersRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.DisassociateGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ExportServerlessCacheSnapshotRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.FailoverGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.IncreaseNodeGroupsInGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.IncreaseReplicaCountRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ListAllowedNodeTypeModificationsRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyCacheClusterRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyCacheParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyCacheSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyReplicationGroupShardConfigurationRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyServerlessCacheRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyUserGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ModifyUserRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.PurchaseReservedCacheNodesOfferingRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.RebalanceSlotsInGlobalReplicationGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.RebootCacheClusterRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.RemoveTagsFromResourceRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.ResetCacheParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.RevokeCacheSecurityGroupIngressRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.StartMigrationRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.TestFailoverRequestMarshaller;
import software.amazon.awssdk.services.elasticache.transform.TestMigrationRequestMarshaller;
import software.amazon.awssdk.services.elasticache.waiters.ElastiCacheWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link ElastiCacheClient}.
*
* @see ElastiCacheClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultElastiCacheClient implements ElastiCacheClient {
private static final Logger log = Logger.loggerFor(DefaultElastiCacheClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final SyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultElastiCacheClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
}
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* For example, you can use cost-allocation tags to your ElastiCache resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services.
*
*
* For more information, see Using
* Cost Allocation Tags in Amazon ElastiCache in the ElastiCache User Guide.
*
*
* @param addTagsToResourceRequest
* Represents the input of an AddTagsToResource operation.
* @return Result of the AddTagsToResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidArnException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.AddTagsToResource
* @see AWS
* API Documentation
*/
@Override
public AddTagsToResourceResponse addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest)
throws CacheClusterNotFoundException, CacheParameterGroupNotFoundException, CacheSecurityGroupNotFoundException,
CacheSubnetGroupNotFoundException, InvalidReplicationGroupStateException, ReplicationGroupNotFoundException,
ReservedCacheNodeNotFoundException, SnapshotNotFoundException, UserNotFoundException, UserGroupNotFoundException,
ServerlessCacheNotFoundException, InvalidServerlessCacheStateException, ServerlessCacheSnapshotNotFoundException,
InvalidServerlessCacheSnapshotStateException, TagQuotaPerResourceExceededException, InvalidArnException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddTagsToResourceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addTagsToResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsToResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTagsToResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddTagsToResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addTagsToResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddTagsToResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Allows network ingress to a cache security group. Applications using ElastiCache must be running on Amazon EC2,
* and Amazon EC2 security groups are used as the authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one region to an ElastiCache cluster in another
* region.
*
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* Represents the input of an AuthorizeCacheSecurityGroupIngress operation.
* @return Result of the AuthorizeCacheSecurityGroupIngress operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws AuthorizationAlreadyExistsException
* The specified Amazon EC2 security group is already authorized for the specified cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.AuthorizeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
@Override
public AuthorizeCacheSecurityGroupIngressResponse authorizeCacheSecurityGroupIngress(
AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest)
throws CacheSecurityGroupNotFoundException, InvalidCacheSecurityGroupStateException,
AuthorizationAlreadyExistsException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AuthorizeCacheSecurityGroupIngressResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeCacheSecurityGroupIngressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
authorizeCacheSecurityGroupIngressRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeCacheSecurityGroupIngress");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeCacheSecurityGroupIngress").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(authorizeCacheSecurityGroupIngressRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AuthorizeCacheSecurityGroupIngressRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Apply the service update. For more information on service updates and applying them, see Applying Service
* Updates.
*
*
* @param batchApplyUpdateActionRequest
* @return Result of the BatchApplyUpdateAction operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.BatchApplyUpdateAction
* @see AWS API Documentation
*/
@Override
public BatchApplyUpdateActionResponse batchApplyUpdateAction(BatchApplyUpdateActionRequest batchApplyUpdateActionRequest)
throws ServiceUpdateNotFoundException, InvalidParameterValueException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(BatchApplyUpdateActionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchApplyUpdateActionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchApplyUpdateActionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchApplyUpdateAction");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchApplyUpdateAction").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchApplyUpdateActionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchApplyUpdateActionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Stop the service update. For more information on service updates and stopping them, see Stopping
* Service Updates.
*
*
* @param batchStopUpdateActionRequest
* @return Result of the BatchStopUpdateAction operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.BatchStopUpdateAction
* @see AWS API Documentation
*/
@Override
public BatchStopUpdateActionResponse batchStopUpdateAction(BatchStopUpdateActionRequest batchStopUpdateActionRequest)
throws ServiceUpdateNotFoundException, InvalidParameterValueException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(BatchStopUpdateActionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchStopUpdateActionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchStopUpdateActionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchStopUpdateAction");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchStopUpdateAction").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchStopUpdateActionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchStopUpdateActionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Complete the migration of data.
*
*
* @param completeMigrationRequest
* @return Result of the CompleteMigration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotUnderMigrationException
* The designated replication group is not available for data migration.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CompleteMigration
* @see AWS
* API Documentation
*/
@Override
public CompleteMigrationResponse completeMigration(CompleteMigrationRequest completeMigrationRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException,
ReplicationGroupNotUnderMigrationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CompleteMigrationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(completeMigrationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, completeMigrationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CompleteMigration");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CompleteMigration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(completeMigrationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CompleteMigrationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a copy of an existing serverless cache’s snapshot. Available for Valkey, Redis OSS and Serverless
* Memcached only.
*
*
* @param copyServerlessCacheSnapshotRequest
* @return Result of the CopyServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Valkey, Redis OSS and Serverless
* Memcached only.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws ServerlessCacheSnapshotQuotaExceededException
* The number of serverless cache snapshots exceeds the customer snapshot quota. Available for Valkey, Redis
* OSS and Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CopyServerlessCacheSnapshot
* @see AWS API Documentation
*/
@Override
public CopyServerlessCacheSnapshotResponse copyServerlessCacheSnapshot(
CopyServerlessCacheSnapshotRequest copyServerlessCacheSnapshotRequest)
throws ServerlessCacheSnapshotAlreadyExistsException, ServerlessCacheSnapshotNotFoundException,
ServerlessCacheSnapshotQuotaExceededException, InvalidServerlessCacheSnapshotStateException,
ServiceLinkedRoleNotFoundException, TagQuotaPerResourceExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CopyServerlessCacheSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(copyServerlessCacheSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyServerlessCacheSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyServerlessCacheSnapshot");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CopyServerlessCacheSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(copyServerlessCacheSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CopyServerlessCacheSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Makes a copy of an existing snapshot.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* Users or groups that have permissions to use the CopySnapshot
operation can create their own Amazon
* S3 buckets and copy snapshots to it. To control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot
operation. For more information about using IAM to control the
* use of ElastiCache operations, see Exporting Snapshots and
* Authentication & Access
* Control.
*
*
*
* You could receive the following error messages.
*
*
* Error Messages
*
*
* -
*
* Error Message: The S3 bucket %s is outside of the region.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s does not exist.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s is not owned by the authenticated user.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The authenticated user does not have sufficient permissions to perform the desired
* activity.
*
*
* Solution: Contact your system administrator to get the needed permissions.
*
*
* -
*
* Error Message: The S3 bucket %s already contains an object with key %s.
*
*
* Solution: Give the TargetSnapshotName
a new and unique value. If exporting a snapshot, you
* could alternatively create a new Amazon S3 bucket and use this same value for TargetSnapshotName
.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ permissions %s on the S3 Bucket.
*
*
* Solution: Add List and Read permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted WRITE permissions %s on the S3 Bucket.
*
*
* Solution: Add Upload/Delete permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ_ACP permissions %s on the S3 Bucket.
*
*
* Solution: Add View Permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
*
*
* @param copySnapshotRequest
* Represents the input of a CopySnapshotMessage
operation.
* @return Result of the CopySnapshot operation returned by the service.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidSnapshotStateException
* The current state of the snapshot does not allow the requested operation to occur.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CopySnapshot
* @see AWS API
* Documentation
*/
@Override
public CopySnapshotResponse copySnapshot(CopySnapshotRequest copySnapshotRequest) throws SnapshotAlreadyExistsException,
SnapshotNotFoundException, SnapshotQuotaExceededException, InvalidSnapshotStateException,
TagQuotaPerResourceExceededException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CopySnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(copySnapshotRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copySnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopySnapshot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CopySnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(copySnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CopySnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant cache engine software, either
* Memcached, Valkey or Redis OSS.
*
*
* This operation is not supported for Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
* @param createCacheClusterRequest
* Represents the input of a CreateCacheCluster operation.
* @return Result of the CreateCacheCluster operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws CacheClusterAlreadyExistsException
* You already have a cluster with the given identifier.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateCacheCluster
* @see AWS API Documentation
*/
@Override
public CreateCacheClusterResponse createCacheCluster(CreateCacheClusterRequest createCacheClusterRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, CacheClusterAlreadyExistsException,
InsufficientCacheClusterCapacityException, CacheSecurityGroupNotFoundException, CacheSubnetGroupNotFoundException,
ClusterQuotaForCustomerExceededException, NodeQuotaForClusterExceededException,
NodeQuotaForCustomerExceededException, CacheParameterGroupNotFoundException, InvalidVpcNetworkStateException,
TagQuotaPerResourceExceededException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCacheClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCacheClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCacheClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCacheCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateCacheCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createCacheClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCacheClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new Amazon ElastiCache cache parameter group. An ElastiCache cache parameter group is a collection of
* parameters and their values that are applied to all of the nodes in any cluster or replication group using the
* CacheParameterGroup.
*
*
* A newly created CacheParameterGroup is an exact duplicate of the default parameter group for the
* CacheParameterGroupFamily. To customize the newly created CacheParameterGroup you can change the values of
* specific parameters. For more information, see:
*
*
* -
*
*
* ModifyCacheParameterGroup in the ElastiCache API Reference.
*
*
* -
*
* Parameters and Parameter
* Groups in the ElastiCache User Guide.
*
*
*
*
* @param createCacheParameterGroupRequest
* Represents the input of a CreateCacheParameterGroup
operation.
* @return Result of the CreateCacheParameterGroup operation returned by the service.
* @throws CacheParameterGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of cache security groups.
* @throws CacheParameterGroupAlreadyExistsException
* A cache parameter group with the requested name already exists.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateCacheParameterGroup
* @see AWS API Documentation
*/
@Override
public CreateCacheParameterGroupResponse createCacheParameterGroup(
CreateCacheParameterGroupRequest createCacheParameterGroupRequest) throws CacheParameterGroupQuotaExceededException,
CacheParameterGroupAlreadyExistsException, InvalidCacheParameterGroupStateException,
TagQuotaPerResourceExceededException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCacheParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCacheParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCacheParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCacheParameterGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCacheParameterGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createCacheParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCacheParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new cache security group. Use a cache security group to control access to one or more clusters.
*
*
* Cache security groups are only used when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC). If you are creating a cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
* @param createCacheSecurityGroupRequest
* Represents the input of a CreateCacheSecurityGroup
operation.
* @return Result of the CreateCacheSecurityGroup operation returned by the service.
* @throws CacheSecurityGroupAlreadyExistsException
* A cache security group with the specified name already exists.
* @throws CacheSecurityGroupQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of cache security groups.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateCacheSecurityGroup
* @see AWS API Documentation
*/
@Override
public CreateCacheSecurityGroupResponse createCacheSecurityGroup(
CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest) throws CacheSecurityGroupAlreadyExistsException,
CacheSecurityGroupQuotaExceededException, TagQuotaPerResourceExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCacheSecurityGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCacheSecurityGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCacheSecurityGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCacheSecurityGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCacheSecurityGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createCacheSecurityGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCacheSecurityGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new cache subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createCacheSubnetGroupRequest
* Represents the input of a CreateCacheSubnetGroup
operation.
* @return Result of the CreateCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupAlreadyExistsException
* The requested cache subnet group name is already in use by an existing cache subnet group.
* @throws CacheSubnetGroupQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of cache subnet groups.
* @throws CacheSubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a cache subnet
* group.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws SubnetNotAllowedException
* At least one subnet ID does not match the other subnet IDs. This mismatch typically occurs when a user
* sets one subnet ID to a regional Availability Zone and a different one to an outpost. Or when a user sets
* the subnet ID to an Outpost when not subscribed on this service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateCacheSubnetGroup
* @see AWS API Documentation
*/
@Override
public CreateCacheSubnetGroupResponse createCacheSubnetGroup(CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest)
throws CacheSubnetGroupAlreadyExistsException, CacheSubnetGroupQuotaExceededException,
CacheSubnetQuotaExceededException, TagQuotaPerResourceExceededException, InvalidSubnetException,
SubnetNotAllowedException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCacheSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCacheSubnetGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCacheSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCacheSubnetGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCacheSubnetGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createCacheSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCacheSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Global Datastore offers fully managed, fast, reliable and secure cross-region replication. Using Global Datastore
* with Valkey or Redis OSS, you can create cross-region read replica clusters for ElastiCache to enable low-latency
* reads and disaster recovery across regions. For more information, see Replication Across
* Regions Using Global Datastore.
*
*
* -
*
* The GlobalReplicationGroupIdSuffix is the name of the Global datastore.
*
*
* -
*
* The PrimaryReplicationGroupId represents the name of the primary cluster that accepts writes and will
* replicate updates to the secondary cluster.
*
*
*
*
* @param createGlobalReplicationGroupRequest
* @return Result of the CreateGlobalReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws GlobalReplicationGroupAlreadyExistsException
* The Global datastore name already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public CreateGlobalReplicationGroupResponse createGlobalReplicationGroup(
CreateGlobalReplicationGroupRequest createGlobalReplicationGroupRequest) throws ReplicationGroupNotFoundException,
InvalidReplicationGroupStateException, GlobalReplicationGroupAlreadyExistsException,
ServiceLinkedRoleNotFoundException, InvalidParameterValueException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createGlobalReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a Valkey or Redis OSS (cluster mode disabled) or a Valkey or Redis OSS (cluster mode enabled) replication
* group.
*
*
* This API can be used to create a standalone regional replication group or a secondary replication group
* associated with a Global datastore.
*
*
* A Valkey or Redis OSS (cluster mode disabled) replication group is a collection of nodes, where one of the nodes
* is a read/write primary and the others are read-only replicas. Writes to the primary are asynchronously
* propagated to the replicas.
*
*
* A Valkey or Redis OSS cluster-mode enabled cluster is comprised of from 1 to 90 shards (API/CLI: node groups).
* Each shard has a primary node and up to 5 read-only replica nodes. The configuration can range from 90 shards and
* 0 replicas to 15 shards and 5 replicas, which is the maximum number or replicas allowed.
*
*
* The node or shard limit can be increased to a maximum of 500 per cluster if the Valkey or Redis OSS engine
* version is 5.0.6 or higher. For example, you can choose to configure a 500 node cluster that ranges between 83
* shards (one primary and 5 replicas per shard) and 500 shards (single primary and no replicas). Make sure there
* are enough available IP addresses to accommodate the increase. Common pitfalls include the subnets in the subnet
* group have too small a CIDR range or the subnets are shared and heavily used by other clusters. For more
* information, see Creating a Subnet
* Group. For versions below 5.0.6, the limit is 250 per cluster.
*
*
* To request a limit increase, see Amazon Service Limits and choose
* the limit type Nodes per cluster per instance type.
*
*
* When a Valkey or Redis OSS (cluster mode disabled) replication group has been successfully created, you can add
* one or more read replicas to it, up to a total of 5 read replicas. If you need to increase or decrease the number
* of node groups (console: shards), you can use scaling. For more information, see Scaling self-designed clusters in
* the ElastiCache User Guide.
*
*
*
* This operation is valid for Valkey and Redis OSS only.
*
*
*
* @param createReplicationGroupRequest
* Represents the input of a CreateReplicationGroup
operation.
* @return Result of the CreateReplicationGroup operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws ReplicationGroupAlreadyExistsException
* The specified replication group already exists.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateReplicationGroup
* @see AWS API Documentation
*/
@Override
public CreateReplicationGroupResponse createReplicationGroup(CreateReplicationGroupRequest createReplicationGroupRequest)
throws CacheClusterNotFoundException, InvalidCacheClusterStateException, ReplicationGroupAlreadyExistsException,
InvalidUserGroupStateException, UserGroupNotFoundException, InsufficientCacheClusterCapacityException,
CacheSecurityGroupNotFoundException, CacheSubnetGroupNotFoundException, ClusterQuotaForCustomerExceededException,
NodeQuotaForClusterExceededException, NodeQuotaForCustomerExceededException, CacheParameterGroupNotFoundException,
InvalidVpcNetworkStateException, TagQuotaPerResourceExceededException,
NodeGroupsPerReplicationGroupQuotaExceededException, GlobalReplicationGroupNotFoundException,
InvalidGlobalReplicationGroupStateException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a serverless cache.
*
*
* @param createServerlessCacheRequest
* @return Result of the CreateServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheAlreadyExistsException
* A serverless cache with this name already exists.
* @throws ServerlessCacheQuotaForCustomerExceededException
* The number of serverless caches exceeds the customer quota.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateServerlessCache
* @see AWS API Documentation
*/
@Override
public CreateServerlessCacheResponse createServerlessCache(CreateServerlessCacheRequest createServerlessCacheRequest)
throws ServerlessCacheNotFoundException, InvalidServerlessCacheStateException, ServerlessCacheAlreadyExistsException,
ServerlessCacheQuotaForCustomerExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, InvalidCredentialsException, InvalidUserGroupStateException,
UserGroupNotFoundException, TagQuotaPerResourceExceededException, ServiceLinkedRoleNotFoundException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateServerlessCacheResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServerlessCacheRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServerlessCacheRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServerlessCache");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateServerlessCache").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createServerlessCacheRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateServerlessCacheRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API creates a copy of an entire ServerlessCache at a specific moment in time. Available for Valkey, Redis
* OSS and Serverless Memcached only.
*
*
* @param createServerlessCacheSnapshotRequest
* @return Result of the CreateServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Valkey, Redis OSS and Serverless
* Memcached only.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotQuotaExceededException
* The number of serverless cache snapshots exceeds the customer snapshot quota. Available for Valkey, Redis
* OSS and Serverless Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateServerlessCacheSnapshot
* @see AWS API Documentation
*/
@Override
public CreateServerlessCacheSnapshotResponse createServerlessCacheSnapshot(
CreateServerlessCacheSnapshotRequest createServerlessCacheSnapshotRequest)
throws ServerlessCacheSnapshotAlreadyExistsException, ServerlessCacheNotFoundException,
InvalidServerlessCacheStateException, ServerlessCacheSnapshotQuotaExceededException,
ServiceLinkedRoleNotFoundException, TagQuotaPerResourceExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateServerlessCacheSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServerlessCacheSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createServerlessCacheSnapshotRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateServerlessCacheSnapshot");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateServerlessCacheSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createServerlessCacheSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateServerlessCacheSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a copy of an entire cluster or replication group at a specific moment in time.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param createSnapshotRequest
* Represents the input of a CreateSnapshot
operation.
* @return Result of the CreateSnapshot operation returned by the service.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateSnapshot
* @see AWS
* API Documentation
*/
@Override
public CreateSnapshotResponse createSnapshot(CreateSnapshotRequest createSnapshotRequest)
throws SnapshotAlreadyExistsException, CacheClusterNotFoundException, ReplicationGroupNotFoundException,
InvalidCacheClusterStateException, InvalidReplicationGroupStateException, SnapshotQuotaExceededException,
SnapshotFeatureNotSupportedException, TagQuotaPerResourceExceededException, InvalidParameterCombinationException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSnapshotRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSnapshot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 and onwards: Creates a user. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws UserAlreadyExistsException
* A user with this ID already exists.
* @throws UserQuotaExceededException
* The quota of users has been exceeded.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResponse createUser(CreateUserRequest createUserRequest) throws UserAlreadyExistsException,
UserQuotaExceededException, DuplicateUserNameException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, TagQuotaPerResourceExceededException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(createUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Creates a user group. For more information, see
* Using Role Based Access
* Control (RBAC)
*
*
* @param createUserGroupRequest
* @return Result of the CreateUserGroup operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws UserGroupAlreadyExistsException
* The user group with this ID already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws DefaultUserRequiredException
* You must add default user to a user group.
* @throws UserGroupQuotaExceededException
* The number of users exceeds the user group limit.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.CreateUserGroup
* @see AWS
* API Documentation
*/
@Override
public CreateUserGroupResponse createUserGroup(CreateUserGroupRequest createUserGroupRequest) throws UserNotFoundException,
DuplicateUserNameException, UserGroupAlreadyExistsException, ServiceLinkedRoleNotFoundException,
DefaultUserRequiredException, UserGroupQuotaExceededException, InvalidParameterValueException,
TagQuotaPerResourceExceededException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateUserGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUserGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUserGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createUserGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Decreases the number of node groups in a Global datastore
*
*
* @param decreaseNodeGroupsInGlobalReplicationGroupRequest
* @return Result of the DecreaseNodeGroupsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DecreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public DecreaseNodeGroupsInGlobalReplicationGroupResponse decreaseNodeGroupsInGlobalReplicationGroup(
DecreaseNodeGroupsInGlobalReplicationGroupRequest decreaseNodeGroupsInGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DecreaseNodeGroupsInGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
decreaseNodeGroupsInGlobalReplicationGroupRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
decreaseNodeGroupsInGlobalReplicationGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DecreaseNodeGroupsInGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DecreaseNodeGroupsInGlobalReplicationGroup")
.withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(decreaseNodeGroupsInGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DecreaseNodeGroupsInGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Dynamically decreases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
* @param decreaseReplicaCountRequest
* @return Result of the DecreaseReplicaCount operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws NoOperationException
* The operation was not performed because no changes were required.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DecreaseReplicaCount
* @see AWS API Documentation
*/
@Override
public DecreaseReplicaCountResponse decreaseReplicaCount(DecreaseReplicaCountRequest decreaseReplicaCountRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, InvalidCacheClusterStateException,
InvalidVpcNetworkStateException, InsufficientCacheClusterCapacityException, ClusterQuotaForCustomerExceededException,
NodeGroupsPerReplicationGroupQuotaExceededException, NodeQuotaForCustomerExceededException,
ServiceLinkedRoleNotFoundException, NoOperationException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DecreaseReplicaCountResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(decreaseReplicaCountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, decreaseReplicaCountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DecreaseReplicaCount");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DecreaseReplicaCount").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(decreaseReplicaCountRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DecreaseReplicaCountRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a previously provisioned cluster. DeleteCacheCluster
deletes all associated cache nodes,
* node endpoints and the cluster itself. When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cluster; you cannot cancel or revert this operation.
*
*
* This operation is not valid for:
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled) clusters
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled) clusters
*
*
* -
*
* A cluster that is the last read replica of a replication group
*
*
* -
*
* A cluster that is the primary node of a replication group
*
*
* -
*
* A node group (shard) that has Multi-AZ mode enabled
*
*
* -
*
* A cluster from a Valkey or Redis OSS (cluster mode enabled) replication group
*
*
* -
*
* A cluster that is not in the available
state
*
*
*
*
* @param deleteCacheClusterRequest
* Represents the input of a DeleteCacheCluster
operation.
* @return Result of the DeleteCacheCluster operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteCacheCluster
* @see AWS API Documentation
*/
@Override
public DeleteCacheClusterResponse deleteCacheCluster(DeleteCacheClusterRequest deleteCacheClusterRequest)
throws CacheClusterNotFoundException, InvalidCacheClusterStateException, SnapshotAlreadyExistsException,
SnapshotFeatureNotSupportedException, SnapshotQuotaExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCacheClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCacheClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCacheClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCacheCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteCacheCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteCacheClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCacheClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified cache parameter group. You cannot delete a cache parameter group if it is associated with
* any cache clusters. You cannot delete the default cache parameter groups in your account.
*
*
* @param deleteCacheParameterGroupRequest
* Represents the input of a DeleteCacheParameterGroup
operation.
* @return Result of the DeleteCacheParameterGroup operation returned by the service.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteCacheParameterGroup
* @see AWS API Documentation
*/
@Override
public DeleteCacheParameterGroupResponse deleteCacheParameterGroup(
DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest) throws InvalidCacheParameterGroupStateException,
CacheParameterGroupNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCacheParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCacheParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCacheParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCacheParameterGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCacheParameterGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteCacheParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCacheParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a cache security group.
*
*
*
* You cannot delete a cache security group if it is associated with any clusters.
*
*
*
* @param deleteCacheSecurityGroupRequest
* Represents the input of a DeleteCacheSecurityGroup
operation.
* @return Result of the DeleteCacheSecurityGroup operation returned by the service.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteCacheSecurityGroup
* @see AWS API Documentation
*/
@Override
public DeleteCacheSecurityGroupResponse deleteCacheSecurityGroup(
DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest) throws InvalidCacheSecurityGroupStateException,
CacheSecurityGroupNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCacheSecurityGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCacheSecurityGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCacheSecurityGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCacheSecurityGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCacheSecurityGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteCacheSecurityGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCacheSecurityGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a cache subnet group.
*
*
*
* You cannot delete a default cache subnet group or one that is associated with any clusters.
*
*
*
* @param deleteCacheSubnetGroupRequest
* Represents the input of a DeleteCacheSubnetGroup
operation.
* @return Result of the DeleteCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupInUseException
* The requested cache subnet group is currently in use.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteCacheSubnetGroup
* @see AWS API Documentation
*/
@Override
public DeleteCacheSubnetGroupResponse deleteCacheSubnetGroup(DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest)
throws CacheSubnetGroupInUseException, CacheSubnetGroupNotFoundException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCacheSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCacheSubnetGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCacheSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCacheSubnetGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCacheSubnetGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteCacheSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCacheSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deleting a Global datastore is a two-step process:
*
*
* -
*
* First, you must DisassociateGlobalReplicationGroup to remove the secondary clusters in the Global
* datastore.
*
*
* -
*
* Once the Global datastore contains only the primary cluster, you can use the
* DeleteGlobalReplicationGroup
API to delete the Global datastore while retainining the primary
* cluster using RetainPrimaryReplicationGroup=true
.
*
*
*
*
* Since the Global Datastore has only a primary cluster, you can delete the Global Datastore while retaining the
* primary by setting RetainPrimaryReplicationGroup=true
. The primary cluster is never deleted when
* deleting a Global Datastore. It can only be deleted when it no longer is associated with any Global Datastore.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
* @param deleteGlobalReplicationGroupRequest
* @return Result of the DeleteGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public DeleteGlobalReplicationGroupResponse deleteGlobalReplicationGroup(
DeleteGlobalReplicationGroupRequest deleteGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGlobalReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an existing replication group. By default, this operation deletes the entire replication group, including
* the primary/primaries and all of the read replicas. If the replication group has only one primary, you can
* optionally delete only the read replicas, while retaining the primary by setting
* RetainPrimaryCluster=true
.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
*
* -
*
* CreateSnapshot
permission is required to create a final snapshot. Without this permission, the API
* call will fail with an Access Denied
exception.
*
*
* -
*
* This operation is valid for Redis OSS only.
*
*
*
*
*
* @param deleteReplicationGroupRequest
* Represents the input of a DeleteReplicationGroup
operation.
* @return Result of the DeleteReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws SnapshotAlreadyExistsException
* You already have a snapshot with the given name.
* @throws SnapshotFeatureNotSupportedException
* You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* @throws SnapshotQuotaExceededException
* The request cannot be processed because it would exceed the maximum number of snapshots.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteReplicationGroup
* @see AWS API Documentation
*/
@Override
public DeleteReplicationGroupResponse deleteReplicationGroup(DeleteReplicationGroupRequest deleteReplicationGroupRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, SnapshotAlreadyExistsException,
SnapshotFeatureNotSupportedException, SnapshotQuotaExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a specified existing serverless cache.
*
*
*
* CreateServerlessCacheSnapshot
permission is required to create a final snapshot. Without this
* permission, the API call will fail with an Access Denied
exception.
*
*
*
* @param deleteServerlessCacheRequest
* @return Result of the DeleteServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotAlreadyExistsException
* A serverless cache snapshot with this name already exists. Available for Valkey, Redis OSS and Serverless
* Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteServerlessCache
* @see AWS API Documentation
*/
@Override
public DeleteServerlessCacheResponse deleteServerlessCache(DeleteServerlessCacheRequest deleteServerlessCacheRequest)
throws ServerlessCacheNotFoundException, InvalidServerlessCacheStateException,
ServerlessCacheSnapshotAlreadyExistsException, InvalidParameterValueException, InvalidParameterCombinationException,
InvalidCredentialsException, ServiceLinkedRoleNotFoundException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteServerlessCacheResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteServerlessCacheRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServerlessCacheRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteServerlessCache");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteServerlessCache").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteServerlessCacheRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteServerlessCacheRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an existing serverless cache snapshot. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
* @param deleteServerlessCacheSnapshotRequest
* @return Result of the DeleteServerlessCacheSnapshot operation returned by the service.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteServerlessCacheSnapshot
* @see AWS API Documentation
*/
@Override
public DeleteServerlessCacheSnapshotResponse deleteServerlessCacheSnapshot(
DeleteServerlessCacheSnapshotRequest deleteServerlessCacheSnapshotRequest) throws ServiceLinkedRoleNotFoundException,
ServerlessCacheSnapshotNotFoundException, InvalidServerlessCacheSnapshotStateException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteServerlessCacheSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteServerlessCacheSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteServerlessCacheSnapshotRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteServerlessCacheSnapshot");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteServerlessCacheSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteServerlessCacheSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteServerlessCacheSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param deleteSnapshotRequest
* Represents the input of a DeleteSnapshot
operation.
* @return Result of the DeleteSnapshot operation returned by the service.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws InvalidSnapshotStateException
* The current state of the snapshot does not allow the requested operation to occur.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteSnapshot
* @see AWS
* API Documentation
*/
@Override
public DeleteSnapshotResponse deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest) throws SnapshotNotFoundException,
InvalidSnapshotStateException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSnapshotRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSnapshot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user. The user will be removed from
* all user groups and in turn removed from all replication groups. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws InvalidUserStateException
* The user is not in active state.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws DefaultUserAssociatedToUserGroupException
* The default user assigned to the user group.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws InvalidUserStateException,
UserNotFoundException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
DefaultUserAssociatedToUserGroupException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user group. The user group must first
* be disassociated from the replication group before it can be deleted. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param deleteUserGroupRequest
* @return Result of the DeleteUserGroup operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DeleteUserGroup
* @see AWS
* API Documentation
*/
@Override
public DeleteUserGroupResponse deleteUserGroup(DeleteUserGroupRequest deleteUserGroupRequest)
throws UserGroupNotFoundException, InvalidUserGroupStateException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteUserGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUserGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteUserGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteUserGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cache cluster if a cluster identifier is supplied.
*
*
* By default, abbreviated information about the clusters is returned. You can use the optional
* ShowCacheNodeInfo flag to retrieve detailed information about the cache nodes associated with the
* clusters. These details include the DNS address and port for the cache node endpoint.
*
*
* If the cluster is in the creating state, only cluster-level information is displayed until all of the
* nodes are successfully provisioned.
*
*
* If the cluster is in the deleting state, only cluster-level information is displayed.
*
*
* If cache nodes are currently being added to the cluster, node endpoint information and creation time for the
* additional nodes are not displayed until they are completely provisioned. When the cluster state is
* available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cluster, no endpoint information for the removed nodes is
* displayed.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters
operation.
* @return Result of the DescribeCacheClusters operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheClusters
* @see AWS API Documentation
*/
@Override
public DescribeCacheClustersResponse describeCacheClusters(DescribeCacheClustersRequest describeCacheClustersRequest)
throws CacheClusterNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheClustersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheClustersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheClusters");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheClusters").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheClustersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheClustersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of the available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
operation.
* @return Result of the DescribeCacheEngineVersions operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
@Override
public DescribeCacheEngineVersionsResponse describeCacheEngineVersions(
DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest) throws AwsServiceException,
SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheEngineVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheEngineVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheEngineVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheEngineVersions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheEngineVersions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheEngineVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheEngineVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of cache parameter group descriptions. If a cache parameter group name is specified, the list
* contains only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
operation.
* @return Result of the DescribeCacheParameterGroups operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
@Override
public DescribeCacheParameterGroupsResponse describeCacheParameterGroups(
DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest) throws CacheParameterGroupNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheParameterGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheParameterGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheParameterGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheParameterGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheParameterGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheParameterGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheParameterGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the detailed parameter list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters
operation.
* @return Result of the DescribeCacheParameters operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheParameters
* @see AWS API Documentation
*/
@Override
public DescribeCacheParametersResponse describeCacheParameters(DescribeCacheParametersRequest describeCacheParametersRequest)
throws CacheParameterGroupNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheParametersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheParametersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheParametersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheParameters");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheParameters").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheParametersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheParametersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of cache security group descriptions. If a cache security group name is specified, the list
* contains only the description of that group. This applicable only when you have ElastiCache in Classic setup
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
operation.
* @return Result of the DescribeCacheSecurityGroups operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
@Override
public DescribeCacheSecurityGroupsResponse describeCacheSecurityGroups(
DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest) throws CacheSecurityGroupNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheSecurityGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheSecurityGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheSecurityGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheSecurityGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheSecurityGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheSecurityGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheSecurityGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of cache subnet group descriptions. If a subnet group name is specified, the list contains only
* the description of that group. This is applicable only when you have ElastiCache in VPC setup. All ElastiCache
* clusters now launch in VPC by default.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups
operation.
* @return Result of the DescribeCacheSubnetGroups operation returned by the service.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
@Override
public DescribeCacheSubnetGroupsResponse describeCacheSubnetGroups(
DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest) throws CacheSubnetGroupNotFoundException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeCacheSubnetGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCacheSubnetGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCacheSubnetGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCacheSubnetGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCacheSubnetGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeCacheSubnetGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCacheSubnetGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the default engine and system parameter information for the specified cache engine.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
operation.
* @return Result of the DescribeEngineDefaultParameters operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
@Override
public DescribeEngineDefaultParametersResponse describeEngineDefaultParameters(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest) throws InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeEngineDefaultParametersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEngineDefaultParametersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeEngineDefaultParametersRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEngineDefaultParameters");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEngineDefaultParameters").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeEngineDefaultParametersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeEngineDefaultParametersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns events related to clusters, cache security groups, and cache parameter groups. You can obtain events
* specific to a particular cluster, cache security group, or cache parameter group by providing the name as a
* parameter.
*
*
* By default, only the events occurring within the last hour are returned; however, you can retrieve up to 14 days'
* worth of events if necessary.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents
operation.
* @return Result of the DescribeEvents operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeEvents
* @see AWS
* API Documentation
*/
@Override
public DescribeEventsResponse describeEvents(DescribeEventsRequest describeEventsRequest)
throws InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeEventsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEventsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEvents");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeEvents").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeEventsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeEventsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about a particular global replication group. If no identifier is specified, returns
* information about all Global datastores.
*
*
* @param describeGlobalReplicationGroupsRequest
* @return Result of the DescribeGlobalReplicationGroups operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
@Override
public DescribeGlobalReplicationGroupsResponse describeGlobalReplicationGroups(
DescribeGlobalReplicationGroupsRequest describeGlobalReplicationGroupsRequest)
throws GlobalReplicationGroupNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeGlobalReplicationGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeGlobalReplicationGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeGlobalReplicationGroupsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeGlobalReplicationGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeGlobalReplicationGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeGlobalReplicationGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeGlobalReplicationGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups
returns information about all replication groups.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups
operation.
* @return Result of the DescribeReplicationGroups operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
@Override
public DescribeReplicationGroupsResponse describeReplicationGroups(
DescribeReplicationGroupsRequest describeReplicationGroupsRequest) throws ReplicationGroupNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeReplicationGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReplicationGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReplicationGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReplicationGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReplicationGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeReplicationGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeReplicationGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about reserved cache nodes for this account, or about a specified reserved cache node.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
operation.
* @return Result of the DescribeReservedCacheNodes operation returned by the service.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
@Override
public DescribeReservedCacheNodesResponse describeReservedCacheNodes(
DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest) throws ReservedCacheNodeNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeReservedCacheNodesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReservedCacheNodesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReservedCacheNodesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReservedCacheNodes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReservedCacheNodes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeReservedCacheNodesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeReservedCacheNodesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists available reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return Result of the DescribeReservedCacheNodesOfferings operation returned by the service.
* @throws ReservedCacheNodesOfferingNotFoundException
* The requested cache node offering does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
@Override
public DescribeReservedCacheNodesOfferingsResponse describeReservedCacheNodesOfferings(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest)
throws ReservedCacheNodesOfferingNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeReservedCacheNodesOfferingsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReservedCacheNodesOfferingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeReservedCacheNodesOfferingsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReservedCacheNodesOfferings");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReservedCacheNodesOfferings").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeReservedCacheNodesOfferingsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeReservedCacheNodesOfferingsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about serverless cache snapshots. By default, this API lists all of the customer’s serverless
* cache snapshots. It can also describe a single serverless cache snapshot, or the snapshots associated with a
* particular serverless cache. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
* @param describeServerlessCacheSnapshotsRequest
* @return Result of the DescribeServerlessCacheSnapshots operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
@Override
public DescribeServerlessCacheSnapshotsResponse describeServerlessCacheSnapshots(
DescribeServerlessCacheSnapshotsRequest describeServerlessCacheSnapshotsRequest)
throws ServerlessCacheNotFoundException, ServerlessCacheSnapshotNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeServerlessCacheSnapshotsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServerlessCacheSnapshotsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeServerlessCacheSnapshotsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServerlessCacheSnapshots");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServerlessCacheSnapshots").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeServerlessCacheSnapshotsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeServerlessCacheSnapshotsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about a specific serverless cache. If no identifier is specified, then the API returns
* information on all the serverless caches belonging to this Amazon Web Services account.
*
*
* @param describeServerlessCachesRequest
* @return Result of the DescribeServerlessCaches operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeServerlessCaches
* @see AWS API Documentation
*/
@Override
public DescribeServerlessCachesResponse describeServerlessCaches(
DescribeServerlessCachesRequest describeServerlessCachesRequest) throws ServerlessCacheNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeServerlessCachesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServerlessCachesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServerlessCachesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServerlessCaches");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServerlessCaches").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeServerlessCachesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeServerlessCachesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns details of the service updates
*
*
* @param describeServiceUpdatesRequest
* @return Result of the DescribeServiceUpdates operation returned by the service.
* @throws ServiceUpdateNotFoundException
* The service update doesn't exist
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeServiceUpdates
* @see AWS API Documentation
*/
@Override
public DescribeServiceUpdatesResponse describeServiceUpdates(DescribeServiceUpdatesRequest describeServiceUpdatesRequest)
throws ServiceUpdateNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeServiceUpdatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServiceUpdatesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServiceUpdatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServiceUpdates");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServiceUpdates").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeServiceUpdatesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeServiceUpdatesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about cluster or replication group snapshots. By default, DescribeSnapshots
* lists all of your snapshots; it can optionally describe a single snapshot, or just the snapshots associated with
* a particular cache cluster.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage
operation.
* @return Result of the DescribeSnapshots operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
@Override
public DescribeSnapshotsResponse describeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest)
throws CacheClusterNotFoundException, SnapshotNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeSnapshotsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSnapshotsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSnapshotsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSnapshots");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeSnapshots").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeSnapshotsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSnapshotsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns details of the update actions
*
*
* @param describeUpdateActionsRequest
* @return Result of the DescribeUpdateActions operation returned by the service.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeUpdateActions
* @see AWS API Documentation
*/
@Override
public DescribeUpdateActionsResponse describeUpdateActions(DescribeUpdateActionsRequest describeUpdateActionsRequest)
throws InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeUpdateActionsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUpdateActionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUpdateActionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUpdateActions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUpdateActions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUpdateActionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUpdateActionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of user groups.
*
*
* @param describeUserGroupsRequest
* @return Result of the DescribeUserGroups operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeUserGroups
* @see AWS API Documentation
*/
@Override
public DescribeUserGroupsResponse describeUserGroups(DescribeUserGroupsRequest describeUserGroupsRequest)
throws UserGroupNotFoundException, ServiceLinkedRoleNotFoundException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeUserGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUserGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUserGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUserGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of users.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DescribeUsers
* @see AWS API
* Documentation
*/
@Override
public DescribeUsersResponse describeUsers(DescribeUsersRequest describeUsersRequest) throws UserNotFoundException,
ServiceLinkedRoleNotFoundException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeUsersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUsersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUsersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUsers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUsers").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUsersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUsersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Remove a secondary cluster from the Global datastore using the Global datastore name. The secondary cluster will
* no longer receive updates from the primary cluster, but will remain as a standalone cluster in that Amazon
* region.
*
*
* @param disassociateGlobalReplicationGroupRequest
* @return Result of the DisassociateGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.DisassociateGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public DisassociateGlobalReplicationGroupResponse disassociateGlobalReplicationGroup(
DisassociateGlobalReplicationGroupRequest disassociateGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DisassociateGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateGlobalReplicationGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides the functionality to export the serverless cache snapshot data to Amazon S3. Available for Valkey and
* Redis OSS only.
*
*
* @param exportServerlessCacheSnapshotRequest
* @return Result of the ExportServerlessCacheSnapshot operation returned by the service.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ExportServerlessCacheSnapshot
* @see AWS API Documentation
*/
@Override
public ExportServerlessCacheSnapshotResponse exportServerlessCacheSnapshot(
ExportServerlessCacheSnapshotRequest exportServerlessCacheSnapshotRequest)
throws ServerlessCacheSnapshotNotFoundException, InvalidServerlessCacheSnapshotStateException,
ServiceLinkedRoleNotFoundException, InvalidParameterValueException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ExportServerlessCacheSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(exportServerlessCacheSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
exportServerlessCacheSnapshotRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ExportServerlessCacheSnapshot");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ExportServerlessCacheSnapshot").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(exportServerlessCacheSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ExportServerlessCacheSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Used to failover the primary region to a secondary region. The secondary region will become primary, and all
* other clusters will become secondary.
*
*
* @param failoverGlobalReplicationGroupRequest
* @return Result of the FailoverGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.FailoverGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public FailoverGlobalReplicationGroupResponse failoverGlobalReplicationGroup(
FailoverGlobalReplicationGroupRequest failoverGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(FailoverGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(failoverGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
failoverGlobalReplicationGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "FailoverGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("FailoverGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(failoverGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new FailoverGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Increase the number of node groups in the Global datastore
*
*
* @param increaseNodeGroupsInGlobalReplicationGroupRequest
* @return Result of the IncreaseNodeGroupsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.IncreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public IncreaseNodeGroupsInGlobalReplicationGroupResponse increaseNodeGroupsInGlobalReplicationGroup(
IncreaseNodeGroupsInGlobalReplicationGroupRequest increaseNodeGroupsInGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(IncreaseNodeGroupsInGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
increaseNodeGroupsInGlobalReplicationGroupRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
increaseNodeGroupsInGlobalReplicationGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "IncreaseNodeGroupsInGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("IncreaseNodeGroupsInGlobalReplicationGroup")
.withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(increaseNodeGroupsInGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new IncreaseNodeGroupsInGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Dynamically increases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
* @param increaseReplicaCountRequest
* @return Result of the IncreaseReplicaCount operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws ClusterQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of clusters per customer.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws NoOperationException
* The operation was not performed because no changes were required.
* @throws InvalidKmsKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.IncreaseReplicaCount
* @see AWS API Documentation
*/
@Override
public IncreaseReplicaCountResponse increaseReplicaCount(IncreaseReplicaCountRequest increaseReplicaCountRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, InvalidCacheClusterStateException,
InvalidVpcNetworkStateException, InsufficientCacheClusterCapacityException, ClusterQuotaForCustomerExceededException,
NodeGroupsPerReplicationGroupQuotaExceededException, NodeQuotaForCustomerExceededException, NoOperationException,
InvalidKmsKeyException, InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException,
SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(IncreaseReplicaCountResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(increaseReplicaCountRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, increaseReplicaCountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "IncreaseReplicaCount");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("IncreaseReplicaCount").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(increaseReplicaCountRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new IncreaseReplicaCountRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all available node types that you can scale with your cluster's replication group's current node type.
*
*
* When you use the ModifyCacheCluster
or ModifyReplicationGroup
operations to scale your
* cluster or replication group, the value of the CacheNodeType
parameter must be one of the node types
* returned by this operation.
*
*
* @param listAllowedNodeTypeModificationsRequest
* The input parameters for the ListAllowedNodeTypeModifications
operation.
* @return Result of the ListAllowedNodeTypeModifications operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ListAllowedNodeTypeModifications
* @see AWS API Documentation
*/
@Override
public ListAllowedNodeTypeModificationsResponse listAllowedNodeTypeModifications(
ListAllowedNodeTypeModificationsRequest listAllowedNodeTypeModificationsRequest)
throws CacheClusterNotFoundException, ReplicationGroupNotFoundException, InvalidParameterCombinationException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListAllowedNodeTypeModificationsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAllowedNodeTypeModificationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listAllowedNodeTypeModificationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAllowedNodeTypeModifications");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAllowedNodeTypeModifications").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listAllowedNodeTypeModificationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListAllowedNodeTypeModificationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all tags currently on a named resource.
*
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* If the cluster is not in the available state, ListTagsForResource
returns an error.
*
*
* @param listTagsForResourceRequest
* The input parameters for the ListTagsForResource
operation.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidArnException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws CacheClusterNotFoundException, CacheParameterGroupNotFoundException, CacheSecurityGroupNotFoundException,
CacheSubnetGroupNotFoundException, InvalidReplicationGroupStateException, ReplicationGroupNotFoundException,
ReservedCacheNodeNotFoundException, SnapshotNotFoundException, UserNotFoundException, UserGroupNotFoundException,
ServerlessCacheNotFoundException, InvalidServerlessCacheStateException, ServerlessCacheSnapshotNotFoundException,
InvalidServerlessCacheSnapshotStateException, InvalidArnException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* parameters by specifying the parameters and the new values.
*
*
* @param modifyCacheClusterRequest
* Represents the input of a ModifyCacheCluster
operation.
* @return Result of the ModifyCacheCluster operation returned by the service.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyCacheCluster
* @see AWS API Documentation
*/
@Override
public ModifyCacheClusterResponse modifyCacheCluster(ModifyCacheClusterRequest modifyCacheClusterRequest)
throws InvalidCacheClusterStateException, InvalidCacheSecurityGroupStateException,
InsufficientCacheClusterCapacityException, CacheClusterNotFoundException, NodeQuotaForClusterExceededException,
NodeQuotaForCustomerExceededException, CacheSecurityGroupNotFoundException, CacheParameterGroupNotFoundException,
InvalidVpcNetworkStateException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyCacheClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyCacheClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyCacheClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyCacheCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyCacheCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyCacheClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyCacheClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the parameters of a cache parameter group. You can modify up to 20 parameters in a single request by
* submitting a list parameter name and value pairs.
*
*
* @param modifyCacheParameterGroupRequest
* Represents the input of a ModifyCacheParameterGroup
operation.
* @return Result of the ModifyCacheParameterGroup operation returned by the service.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyCacheParameterGroup
* @see AWS API Documentation
*/
@Override
public ModifyCacheParameterGroupResponse modifyCacheParameterGroup(
ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest) throws CacheParameterGroupNotFoundException,
InvalidCacheParameterGroupStateException, InvalidParameterValueException, InvalidParameterCombinationException,
InvalidGlobalReplicationGroupStateException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyCacheParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyCacheParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyCacheParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyCacheParameterGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyCacheParameterGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyCacheParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyCacheParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies an existing cache subnet group.
*
*
* @param modifyCacheSubnetGroupRequest
* Represents the input of a ModifyCacheSubnetGroup
operation.
* @return Result of the ModifyCacheSubnetGroup operation returned by the service.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws CacheSubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a cache subnet
* group.
* @throws SubnetInUseException
* The requested subnet is being used by another cache subnet group.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws SubnetNotAllowedException
* At least one subnet ID does not match the other subnet IDs. This mismatch typically occurs when a user
* sets one subnet ID to a regional Availability Zone and a different one to an outpost. Or when a user sets
* the subnet ID to an Outpost when not subscribed on this service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyCacheSubnetGroup
* @see AWS API Documentation
*/
@Override
public ModifyCacheSubnetGroupResponse modifyCacheSubnetGroup(ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest)
throws CacheSubnetGroupNotFoundException, CacheSubnetQuotaExceededException, SubnetInUseException,
InvalidSubnetException, SubnetNotAllowedException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyCacheSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyCacheSubnetGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyCacheSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyCacheSubnetGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyCacheSubnetGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyCacheSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyCacheSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the settings for a Global datastore.
*
*
* @param modifyGlobalReplicationGroupRequest
* @return Result of the ModifyGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public ModifyGlobalReplicationGroupResponse modifyGlobalReplicationGroup(
ModifyGlobalReplicationGroupRequest modifyGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyGlobalReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyGlobalReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the settings for a replication group. This is limited to Valkey and Redis OSS 7 and above.
*
*
* -
*
* Scaling
* for Valkey or Redis OSS (cluster mode enabled) in the ElastiCache User Guide
*
*
* -
*
* ModifyReplicationGroupShardConfiguration in the ElastiCache API Reference
*
*
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param modifyReplicationGroupRequest
* Represents the input of a ModifyReplicationGroups
operation.
* @return Result of the ModifyReplicationGroup operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws NodeQuotaForClusterExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes in a single
* cluster.
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws InvalidKmsKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyReplicationGroup
* @see AWS API Documentation
*/
@Override
public ModifyReplicationGroupResponse modifyReplicationGroup(ModifyReplicationGroupRequest modifyReplicationGroupRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, InvalidUserGroupStateException,
UserGroupNotFoundException, InvalidCacheClusterStateException, InvalidCacheSecurityGroupStateException,
InsufficientCacheClusterCapacityException, CacheClusterNotFoundException, NodeQuotaForClusterExceededException,
NodeQuotaForCustomerExceededException, CacheSecurityGroupNotFoundException, CacheParameterGroupNotFoundException,
InvalidVpcNetworkStateException, InvalidKmsKeyException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyReplicationGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyReplicationGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies a replication group's shards (node groups) by allowing you to add shards, remove shards, or rebalance
* the keyspaces among existing shards.
*
*
* @param modifyReplicationGroupShardConfigurationRequest
* Represents the input for a ModifyReplicationGroupShardConfiguration
operation.
* @return Result of the ModifyReplicationGroupShardConfiguration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws InsufficientCacheClusterCapacityException
* The requested cache node type is not available in the specified Availability Zone. For more information,
* see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* @throws NodeGroupsPerReplicationGroupQuotaExceededException
* The request cannot be processed because it would exceed the maximum allowed number of node groups
* (shards) in a single replication group. The default maximum is 90
* @throws NodeQuotaForCustomerExceededException
* The request cannot be processed because it would exceed the allowed number of cache nodes per customer.
* @throws InvalidKmsKeyException
* The KMS key supplied is not valid.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyReplicationGroupShardConfiguration
* @see AWS API Documentation
*/
@Override
public ModifyReplicationGroupShardConfigurationResponse modifyReplicationGroupShardConfiguration(
ModifyReplicationGroupShardConfigurationRequest modifyReplicationGroupShardConfigurationRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException, InvalidCacheClusterStateException,
InvalidVpcNetworkStateException, InsufficientCacheClusterCapacityException,
NodeGroupsPerReplicationGroupQuotaExceededException, NodeQuotaForCustomerExceededException, InvalidKmsKeyException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyReplicationGroupShardConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
modifyReplicationGroupShardConfigurationRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
modifyReplicationGroupShardConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyReplicationGroupShardConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyReplicationGroupShardConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(modifyReplicationGroupShardConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyReplicationGroupShardConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API modifies the attributes of a serverless cache.
*
*
* @param modifyServerlessCacheRequest
* @return Result of the ModifyServerlessCache operation returned by the service.
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidCredentialsException
* You must enter valid credentials.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyServerlessCache
* @see AWS API Documentation
*/
@Override
public ModifyServerlessCacheResponse modifyServerlessCache(ModifyServerlessCacheRequest modifyServerlessCacheRequest)
throws ServerlessCacheNotFoundException, InvalidServerlessCacheStateException, InvalidParameterValueException,
InvalidParameterCombinationException, InvalidCredentialsException, InvalidUserGroupStateException,
UserGroupNotFoundException, ServiceLinkedRoleNotFoundException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyServerlessCacheResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyServerlessCacheRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyServerlessCacheRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyServerlessCache");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyServerlessCache").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyServerlessCacheRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyServerlessCacheRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes user password(s) and/or access string.
*
*
* @param modifyUserRequest
* @return Result of the ModifyUser operation returned by the service.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws InvalidUserStateException
* The user is not in active state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyUser
* @see AWS API
* Documentation
*/
@Override
public ModifyUserResponse modifyUser(ModifyUserRequest modifyUserRequest) throws UserNotFoundException,
InvalidUserStateException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyUserResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(modifyUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes the list of users that belong to the user group.
*
*
* @param modifyUserGroupRequest
* @return Result of the ModifyUserGroup operation returned by the service.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws DuplicateUserNameException
* A user with this username already exists.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws DefaultUserRequiredException
* You must add default user to a user group.
* @throws InvalidUserGroupStateException
* The user group is not in an active state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ModifyUserGroup
* @see AWS
* API Documentation
*/
@Override
public ModifyUserGroupResponse modifyUserGroup(ModifyUserGroupRequest modifyUserGroupRequest)
throws UserGroupNotFoundException, UserNotFoundException, DuplicateUserNameException,
ServiceLinkedRoleNotFoundException, DefaultUserRequiredException, InvalidUserGroupStateException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyUserGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyUserGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyUserGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyUserGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyUserGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyUserGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyUserGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Allows you to purchase a reserved cache node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable. For more information, see Managing Costs with Reserved
* Nodes.
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* Represents the input of a PurchaseReservedCacheNodesOffering
operation.
* @return Result of the PurchaseReservedCacheNodesOffering operation returned by the service.
* @throws ReservedCacheNodesOfferingNotFoundException
* The requested cache node offering does not exist.
* @throws ReservedCacheNodeAlreadyExistsException
* You already have a reservation with the given identifier.
* @throws ReservedCacheNodeQuotaExceededException
* The request cannot be processed because it would exceed the user's cache node quota.
* @throws TagQuotaPerResourceExceededException
* The request cannot be processed because it would cause the resource to have more than the allowed number
* of tags. The maximum number of tags permitted on a resource is 50.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.PurchaseReservedCacheNodesOffering
* @see AWS API Documentation
*/
@Override
public PurchaseReservedCacheNodesOfferingResponse purchaseReservedCacheNodesOffering(
PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest)
throws ReservedCacheNodesOfferingNotFoundException, ReservedCacheNodeAlreadyExistsException,
ReservedCacheNodeQuotaExceededException, TagQuotaPerResourceExceededException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(PurchaseReservedCacheNodesOfferingResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(purchaseReservedCacheNodesOfferingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
purchaseReservedCacheNodesOfferingRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PurchaseReservedCacheNodesOffering");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PurchaseReservedCacheNodesOffering").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(purchaseReservedCacheNodesOfferingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PurchaseReservedCacheNodesOfferingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Redistribute slots to ensure uniform distribution across existing shards in the cluster.
*
*
* @param rebalanceSlotsInGlobalReplicationGroupRequest
* @return Result of the RebalanceSlotsInGlobalReplicationGroup operation returned by the service.
* @throws GlobalReplicationGroupNotFoundException
* The Global datastore does not exist
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.RebalanceSlotsInGlobalReplicationGroup
* @see AWS API Documentation
*/
@Override
public RebalanceSlotsInGlobalReplicationGroupResponse rebalanceSlotsInGlobalReplicationGroup(
RebalanceSlotsInGlobalReplicationGroupRequest rebalanceSlotsInGlobalReplicationGroupRequest)
throws GlobalReplicationGroupNotFoundException, InvalidGlobalReplicationGroupStateException,
InvalidParameterValueException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RebalanceSlotsInGlobalReplicationGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(rebalanceSlotsInGlobalReplicationGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
rebalanceSlotsInGlobalReplicationGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RebalanceSlotsInGlobalReplicationGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RebalanceSlotsInGlobalReplicationGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(rebalanceSlotsInGlobalReplicationGroupRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RebalanceSlotsInGlobalReplicationGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Reboots some, or all, of the cache nodes within a provisioned cluster. This operation applies any modified cache
* parameter groups to the cluster. The reboot operation takes place as soon as possible, and results in a momentary
* outage to the cluster. During the reboot, the cluster status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being rebooted) to be lost.
*
*
* When the reboot is complete, a cluster event is created.
*
*
* Rebooting a cluster is currently supported on Memcached, Valkey and Redis OSS (cluster mode disabled) clusters.
* Rebooting is not supported on Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
* If you make changes to parameters that require a Valkey or Redis OSS (cluster mode enabled) cluster reboot for
* the changes to be applied, see Rebooting a Cluster for an
* alternate process.
*
*
* @param rebootCacheClusterRequest
* Represents the input of a RebootCacheCluster
operation.
* @return Result of the RebootCacheCluster operation returned by the service.
* @throws InvalidCacheClusterStateException
* The requested cluster is not in the available
state.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.RebootCacheCluster
* @see AWS API Documentation
*/
@Override
public RebootCacheClusterResponse rebootCacheCluster(RebootCacheClusterRequest rebootCacheClusterRequest)
throws InvalidCacheClusterStateException, CacheClusterNotFoundException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RebootCacheClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(rebootCacheClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rebootCacheClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RebootCacheCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RebootCacheCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(rebootCacheClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RebootCacheClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the tags identified by the TagKeys
list from the named resource. A tag is a key-value pair
* where the key and value are case-sensitive. You can use tags to categorize and track all your ElastiCache
* resources, with the exception of global replication group. When you add or remove tags on replication groups,
* those actions will be replicated to all nodes in the replication group. For more information, see Resource-level
* permissions.
*
*
* @param removeTagsFromResourceRequest
* Represents the input of a RemoveTagsFromResource
operation.
* @return Result of the RemoveTagsFromResource operation returned by the service.
* @throws CacheClusterNotFoundException
* The requested cluster ID does not refer to an existing cluster.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws CacheSubnetGroupNotFoundException
* The requested cache subnet group name does not refer to an existing cache subnet group.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws ReservedCacheNodeNotFoundException
* The requested reserved cache node was not found.
* @throws SnapshotNotFoundException
* The requested snapshot name does not refer to an existing snapshot.
* @throws UserNotFoundException
* The user does not exist or could not be found.
* @throws UserGroupNotFoundException
* The user group was not found or does not exist
* @throws ServerlessCacheNotFoundException
* The serverless cache was not found or does not exist.
* @throws InvalidServerlessCacheStateException
* The account for these credentials is not currently active.
* @throws ServerlessCacheSnapshotNotFoundException
* This serverless cache snapshot could not be found or does not exist. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidServerlessCacheSnapshotStateException
* The state of the serverless cache snapshot was not received. Available for Valkey, Redis OSS and
* Serverless Memcached only.
* @throws InvalidArnException
* The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* @throws TagNotFoundException
* The requested tag was not found on this resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.RemoveTagsFromResource
* @see AWS API Documentation
*/
@Override
public RemoveTagsFromResourceResponse removeTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest)
throws CacheClusterNotFoundException, CacheParameterGroupNotFoundException, CacheSecurityGroupNotFoundException,
CacheSubnetGroupNotFoundException, InvalidReplicationGroupStateException, ReplicationGroupNotFoundException,
ReservedCacheNodeNotFoundException, SnapshotNotFoundException, UserNotFoundException, UserGroupNotFoundException,
ServerlessCacheNotFoundException, InvalidServerlessCacheStateException, ServerlessCacheSnapshotNotFoundException,
InvalidServerlessCacheSnapshotStateException, InvalidArnException, TagNotFoundException, AwsServiceException,
SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveTagsFromResourceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeTagsFromResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeTagsFromResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTagsFromResource");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveTagsFromResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(removeTagsFromResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveTagsFromResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the parameters of a cache parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire cache parameter group, specify the
* ResetAllParameters
and CacheParameterGroupName
parameters.
*
*
* @param resetCacheParameterGroupRequest
* Represents the input of a ResetCacheParameterGroup
operation.
* @return Result of the ResetCacheParameterGroup operation returned by the service.
* @throws InvalidCacheParameterGroupStateException
* The current state of the cache parameter group does not allow the requested operation to occur.
* @throws CacheParameterGroupNotFoundException
* The requested cache parameter group name does not refer to an existing cache parameter group.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws InvalidGlobalReplicationGroupStateException
* The Global datastore is not available or in primary-only state.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.ResetCacheParameterGroup
* @see AWS API Documentation
*/
@Override
public ResetCacheParameterGroupResponse resetCacheParameterGroup(
ResetCacheParameterGroupRequest resetCacheParameterGroupRequest) throws InvalidCacheParameterGroupStateException,
CacheParameterGroupNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
InvalidGlobalReplicationGroupStateException, AwsServiceException, SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ResetCacheParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(resetCacheParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, resetCacheParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ResetCacheParameterGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ResetCacheParameterGroup").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(resetCacheParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ResetCacheParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Revokes ingress from a cache security group. Use this operation to disallow access from an Amazon EC2 security
* group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest
* Represents the input of a RevokeCacheSecurityGroupIngress
operation.
* @return Result of the RevokeCacheSecurityGroupIngress operation returned by the service.
* @throws CacheSecurityGroupNotFoundException
* The requested cache security group name does not refer to an existing cache security group.
* @throws AuthorizationNotFoundException
* The specified Amazon EC2 security group is not authorized for the specified cache security group.
* @throws InvalidCacheSecurityGroupStateException
* The current state of the cache security group does not allow deletion.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.RevokeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
@Override
public RevokeCacheSecurityGroupIngressResponse revokeCacheSecurityGroupIngress(
RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest)
throws CacheSecurityGroupNotFoundException, AuthorizationNotFoundException, InvalidCacheSecurityGroupStateException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RevokeCacheSecurityGroupIngressResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(revokeCacheSecurityGroupIngressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
revokeCacheSecurityGroupIngressRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ElastiCache");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RevokeCacheSecurityGroupIngress");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RevokeCacheSecurityGroupIngress").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(revokeCacheSecurityGroupIngressRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RevokeCacheSecurityGroupIngressRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Start the migration of data.
*
*
* @param startMigrationRequest
* @return Result of the StartMigration operation returned by the service.
* @throws ReplicationGroupNotFoundException
* The specified replication group does not exist.
* @throws InvalidReplicationGroupStateException
* The requested replication group is not in the available
state.
* @throws ReplicationGroupAlreadyUnderMigrationException
* The targeted replication group is not available.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElastiCacheException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElastiCacheClient.StartMigration
* @see AWS
* API Documentation
*/
@Override
public StartMigrationResponse startMigration(StartMigrationRequest startMigrationRequest)
throws ReplicationGroupNotFoundException, InvalidReplicationGroupStateException,
ReplicationGroupAlreadyUnderMigrationException, InvalidParameterValueException, AwsServiceException,
SdkClientException, ElastiCacheException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(StartMigrationResponse::builder);
HttpResponseHandler