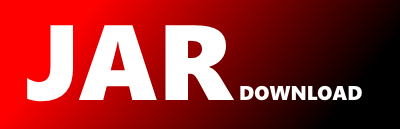
software.amazon.awssdk.services.elasticache.ElastiCacheAsyncClient Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.elasticache.model.AddTagsToResourceRequest;
import software.amazon.awssdk.services.elasticache.model.AddTagsToResourceResponse;
import software.amazon.awssdk.services.elasticache.model.AuthorizeCacheSecurityGroupIngressRequest;
import software.amazon.awssdk.services.elasticache.model.AuthorizeCacheSecurityGroupIngressResponse;
import software.amazon.awssdk.services.elasticache.model.BatchApplyUpdateActionRequest;
import software.amazon.awssdk.services.elasticache.model.BatchApplyUpdateActionResponse;
import software.amazon.awssdk.services.elasticache.model.BatchStopUpdateActionRequest;
import software.amazon.awssdk.services.elasticache.model.BatchStopUpdateActionResponse;
import software.amazon.awssdk.services.elasticache.model.CompleteMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.CompleteMigrationResponse;
import software.amazon.awssdk.services.elasticache.model.CopyServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CopyServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CopySnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CopySnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSecurityGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSecurityGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.CreateSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.CreateUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.CreateUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.CreateUserRequest;
import software.amazon.awssdk.services.elasticache.model.CreateUserResponse;
import software.amazon.awssdk.services.elasticache.model.DecreaseNodeGroupsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DecreaseNodeGroupsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountRequest;
import software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSecurityGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSecurityGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.DeleteUserRequest;
import software.amazon.awssdk.services.elasticache.model.DeleteUserResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeEventsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsResponse;
import software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest;
import software.amazon.awssdk.services.elasticache.model.DescribeUsersResponse;
import software.amazon.awssdk.services.elasticache.model.DisassociateGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.DisassociateGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ExportServerlessCacheSnapshotRequest;
import software.amazon.awssdk.services.elasticache.model.ExportServerlessCacheSnapshotResponse;
import software.amazon.awssdk.services.elasticache.model.FailoverGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.FailoverGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.IncreaseNodeGroupsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.IncreaseNodeGroupsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.IncreaseReplicaCountRequest;
import software.amazon.awssdk.services.elasticache.model.IncreaseReplicaCountResponse;
import software.amazon.awssdk.services.elasticache.model.ListAllowedNodeTypeModificationsRequest;
import software.amazon.awssdk.services.elasticache.model.ListAllowedNodeTypeModificationsResponse;
import software.amazon.awssdk.services.elasticache.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.elasticache.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheSubnetGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyCacheSubnetGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyServerlessCacheRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyServerlessCacheResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyUserGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyUserGroupResponse;
import software.amazon.awssdk.services.elasticache.model.ModifyUserRequest;
import software.amazon.awssdk.services.elasticache.model.ModifyUserResponse;
import software.amazon.awssdk.services.elasticache.model.PurchaseReservedCacheNodesOfferingRequest;
import software.amazon.awssdk.services.elasticache.model.PurchaseReservedCacheNodesOfferingResponse;
import software.amazon.awssdk.services.elasticache.model.RebalanceSlotsInGlobalReplicationGroupRequest;
import software.amazon.awssdk.services.elasticache.model.RebalanceSlotsInGlobalReplicationGroupResponse;
import software.amazon.awssdk.services.elasticache.model.RebootCacheClusterRequest;
import software.amazon.awssdk.services.elasticache.model.RebootCacheClusterResponse;
import software.amazon.awssdk.services.elasticache.model.RemoveTagsFromResourceRequest;
import software.amazon.awssdk.services.elasticache.model.RemoveTagsFromResourceResponse;
import software.amazon.awssdk.services.elasticache.model.ResetCacheParameterGroupRequest;
import software.amazon.awssdk.services.elasticache.model.ResetCacheParameterGroupResponse;
import software.amazon.awssdk.services.elasticache.model.RevokeCacheSecurityGroupIngressRequest;
import software.amazon.awssdk.services.elasticache.model.RevokeCacheSecurityGroupIngressResponse;
import software.amazon.awssdk.services.elasticache.model.StartMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.StartMigrationResponse;
import software.amazon.awssdk.services.elasticache.model.TestFailoverRequest;
import software.amazon.awssdk.services.elasticache.model.TestFailoverResponse;
import software.amazon.awssdk.services.elasticache.model.TestMigrationRequest;
import software.amazon.awssdk.services.elasticache.model.TestMigrationResponse;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParametersPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSecurityGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeEngineDefaultParametersPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeGlobalReplicationGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCacheSnapshotsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCachesPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeServiceUpdatesPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeUpdateActionsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeUserGroupsPublisher;
import software.amazon.awssdk.services.elasticache.paginators.DescribeUsersPublisher;
import software.amazon.awssdk.services.elasticache.waiters.ElastiCacheAsyncWaiter;
/**
* Service client for accessing Amazon ElastiCache asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
* Amazon ElastiCache
*
* Amazon ElastiCache is a web service that makes it easier to set up, operate, and scale a distributed cache in the
* cloud.
*
*
* With ElastiCache, customers get all of the benefits of a high-performance, in-memory cache with less of the
* administrative burden involved in launching and managing a distributed cache. The service makes setup, scaling, and
* cluster failure handling much simpler than in a self-managed cache deployment.
*
*
* In addition, through integration with Amazon CloudWatch, customers get enhanced visibility into the key performance
* statistics associated with their cache and can receive alarms if a part of their cache runs hot.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ElastiCacheAsyncClient extends AwsClient {
String SERVICE_NAME = "elasticache";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "elasticache";
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* For example, you can use cost-allocation tags to your ElastiCache resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services.
*
*
* For more information, see Using
* Cost Allocation Tags in Amazon ElastiCache in the ElastiCache User Guide.
*
*
* @param addTagsToResourceRequest
* Represents the input of an AddTagsToResource operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.AddTagsToResource
* @see AWS
* API Documentation
*/
default CompletableFuture addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* For example, you can use cost-allocation tags to your ElastiCache resources, Amazon generates a cost allocation
* report as a comma-separated value (CSV) file with your usage and costs aggregated by your tags. You can apply
* tags that represent business categories (such as cost centers, application names, or owners) to organize your
* costs across multiple services.
*
*
* For more information, see Using
* Cost Allocation Tags in Amazon ElastiCache in the ElastiCache User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AddTagsToResourceRequest.Builder} avoiding the need
* to create one manually via {@link AddTagsToResourceRequest#builder()}
*
*
* @param addTagsToResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.AddTagsToResourceRequest.Builder} to create a
* request. Represents the input of an AddTagsToResource operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.AddTagsToResource
* @see AWS
* API Documentation
*/
default CompletableFuture addTagsToResource(
Consumer addTagsToResourceRequest) {
return addTagsToResource(AddTagsToResourceRequest.builder().applyMutation(addTagsToResourceRequest).build());
}
/**
*
* Allows network ingress to a cache security group. Applications using ElastiCache must be running on Amazon EC2,
* and Amazon EC2 security groups are used as the authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one region to an ElastiCache cluster in another
* region.
*
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* Represents the input of an AuthorizeCacheSecurityGroupIngress operation.
* @return A Java Future containing the result of the AuthorizeCacheSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - AuthorizationAlreadyExistsException The specified Amazon EC2 security group is already authorized for
* the specified cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.AuthorizeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture authorizeCacheSecurityGroupIngress(
AuthorizeCacheSecurityGroupIngressRequest authorizeCacheSecurityGroupIngressRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows network ingress to a cache security group. Applications using ElastiCache must be running on Amazon EC2,
* and Amazon EC2 security groups are used as the authorization mechanism.
*
*
*
* You cannot authorize ingress from an Amazon EC2 security group in one region to an ElastiCache cluster in another
* region.
*
*
*
* This is a convenience which creates an instance of the {@link AuthorizeCacheSecurityGroupIngressRequest.Builder}
* avoiding the need to create one manually via {@link AuthorizeCacheSecurityGroupIngressRequest#builder()}
*
*
* @param authorizeCacheSecurityGroupIngressRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.AuthorizeCacheSecurityGroupIngressRequest.Builder}
* to create a request. Represents the input of an AuthorizeCacheSecurityGroupIngress operation.
* @return A Java Future containing the result of the AuthorizeCacheSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - AuthorizationAlreadyExistsException The specified Amazon EC2 security group is already authorized for
* the specified cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.AuthorizeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture authorizeCacheSecurityGroupIngress(
Consumer authorizeCacheSecurityGroupIngressRequest) {
return authorizeCacheSecurityGroupIngress(AuthorizeCacheSecurityGroupIngressRequest.builder()
.applyMutation(authorizeCacheSecurityGroupIngressRequest).build());
}
/**
*
* Apply the service update. For more information on service updates and applying them, see Applying Service
* Updates.
*
*
* @param batchApplyUpdateActionRequest
* @return A Java Future containing the result of the BatchApplyUpdateAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.BatchApplyUpdateAction
* @see AWS API Documentation
*/
default CompletableFuture batchApplyUpdateAction(
BatchApplyUpdateActionRequest batchApplyUpdateActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Apply the service update. For more information on service updates and applying them, see Applying Service
* Updates.
*
*
*
* This is a convenience which creates an instance of the {@link BatchApplyUpdateActionRequest.Builder} avoiding the
* need to create one manually via {@link BatchApplyUpdateActionRequest#builder()}
*
*
* @param batchApplyUpdateActionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.BatchApplyUpdateActionRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the BatchApplyUpdateAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.BatchApplyUpdateAction
* @see AWS API Documentation
*/
default CompletableFuture batchApplyUpdateAction(
Consumer batchApplyUpdateActionRequest) {
return batchApplyUpdateAction(BatchApplyUpdateActionRequest.builder().applyMutation(batchApplyUpdateActionRequest)
.build());
}
/**
*
* Stop the service update. For more information on service updates and stopping them, see Stopping
* Service Updates.
*
*
* @param batchStopUpdateActionRequest
* @return A Java Future containing the result of the BatchStopUpdateAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.BatchStopUpdateAction
* @see AWS API Documentation
*/
default CompletableFuture batchStopUpdateAction(
BatchStopUpdateActionRequest batchStopUpdateActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stop the service update. For more information on service updates and stopping them, see Stopping
* Service Updates.
*
*
*
* This is a convenience which creates an instance of the {@link BatchStopUpdateActionRequest.Builder} avoiding the
* need to create one manually via {@link BatchStopUpdateActionRequest#builder()}
*
*
* @param batchStopUpdateActionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.BatchStopUpdateActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the BatchStopUpdateAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.BatchStopUpdateAction
* @see AWS API Documentation
*/
default CompletableFuture batchStopUpdateAction(
Consumer batchStopUpdateActionRequest) {
return batchStopUpdateAction(BatchStopUpdateActionRequest.builder().applyMutation(batchStopUpdateActionRequest).build());
}
/**
*
* Complete the migration of data.
*
*
* @param completeMigrationRequest
* @return A Java Future containing the result of the CompleteMigration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotUnderMigrationException The designated replication group is not available for data
* migration.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CompleteMigration
* @see AWS
* API Documentation
*/
default CompletableFuture completeMigration(CompleteMigrationRequest completeMigrationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Complete the migration of data.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteMigrationRequest.Builder} avoiding the need
* to create one manually via {@link CompleteMigrationRequest#builder()}
*
*
* @param completeMigrationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CompleteMigrationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CompleteMigration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotUnderMigrationException The designated replication group is not available for data
* migration.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CompleteMigration
* @see AWS
* API Documentation
*/
default CompletableFuture completeMigration(
Consumer completeMigrationRequest) {
return completeMigration(CompleteMigrationRequest.builder().applyMutation(completeMigrationRequest).build());
}
/**
*
* Creates a copy of an existing serverless cache’s snapshot. Available for Valkey, Redis OSS and Serverless
* Memcached only.
*
*
* @param copyServerlessCacheSnapshotRequest
* @return A Java Future containing the result of the CopyServerlessCacheSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheSnapshotQuotaExceededException The number of serverless cache snapshots exceeds the
* customer snapshot quota. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CopyServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture copyServerlessCacheSnapshot(
CopyServerlessCacheSnapshotRequest copyServerlessCacheSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a copy of an existing serverless cache’s snapshot. Available for Valkey, Redis OSS and Serverless
* Memcached only.
*
*
*
* This is a convenience which creates an instance of the {@link CopyServerlessCacheSnapshotRequest.Builder}
* avoiding the need to create one manually via {@link CopyServerlessCacheSnapshotRequest#builder()}
*
*
* @param copyServerlessCacheSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CopyServerlessCacheSnapshotRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CopyServerlessCacheSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheSnapshotQuotaExceededException The number of serverless cache snapshots exceeds the
* customer snapshot quota. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CopyServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture copyServerlessCacheSnapshot(
Consumer copyServerlessCacheSnapshotRequest) {
return copyServerlessCacheSnapshot(CopyServerlessCacheSnapshotRequest.builder()
.applyMutation(copyServerlessCacheSnapshotRequest).build());
}
/**
*
* Makes a copy of an existing snapshot.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* Users or groups that have permissions to use the CopySnapshot
operation can create their own Amazon
* S3 buckets and copy snapshots to it. To control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot
operation. For more information about using IAM to control the
* use of ElastiCache operations, see Exporting Snapshots and
* Authentication & Access
* Control.
*
*
*
* You could receive the following error messages.
*
*
* Error Messages
*
*
* -
*
* Error Message: The S3 bucket %s is outside of the region.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s does not exist.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s is not owned by the authenticated user.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The authenticated user does not have sufficient permissions to perform the desired
* activity.
*
*
* Solution: Contact your system administrator to get the needed permissions.
*
*
* -
*
* Error Message: The S3 bucket %s already contains an object with key %s.
*
*
* Solution: Give the TargetSnapshotName
a new and unique value. If exporting a snapshot, you
* could alternatively create a new Amazon S3 bucket and use this same value for TargetSnapshotName
.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ permissions %s on the S3 Bucket.
*
*
* Solution: Add List and Read permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted WRITE permissions %s on the S3 Bucket.
*
*
* Solution: Add Upload/Delete permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ_ACP permissions %s on the S3 Bucket.
*
*
* Solution: Add View Permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
*
*
* @param copySnapshotRequest
* Represents the input of a CopySnapshotMessage
operation.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidSnapshotStateException The current state of the snapshot does not allow the requested
* operation to occur.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CopySnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copySnapshot(CopySnapshotRequest copySnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Makes a copy of an existing snapshot.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* Users or groups that have permissions to use the CopySnapshot
operation can create their own Amazon
* S3 buckets and copy snapshots to it. To control access to your snapshots, use an IAM policy to control who has
* the ability to use the CopySnapshot
operation. For more information about using IAM to control the
* use of ElastiCache operations, see Exporting Snapshots and
* Authentication & Access
* Control.
*
*
*
* You could receive the following error messages.
*
*
* Error Messages
*
*
* -
*
* Error Message: The S3 bucket %s is outside of the region.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s does not exist.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The S3 bucket %s is not owned by the authenticated user.
*
*
* Solution: Create an Amazon S3 bucket in the same region as your snapshot. For more information, see Step 1: Create an Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: The authenticated user does not have sufficient permissions to perform the desired
* activity.
*
*
* Solution: Contact your system administrator to get the needed permissions.
*
*
* -
*
* Error Message: The S3 bucket %s already contains an object with key %s.
*
*
* Solution: Give the TargetSnapshotName
a new and unique value. If exporting a snapshot, you
* could alternatively create a new Amazon S3 bucket and use this same value for TargetSnapshotName
.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ permissions %s on the S3 Bucket.
*
*
* Solution: Add List and Read permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted WRITE permissions %s on the S3 Bucket.
*
*
* Solution: Add Upload/Delete permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
* -
*
* Error Message: ElastiCache has not been granted READ_ACP permissions %s on the S3 Bucket.
*
*
* Solution: Add View Permissions on the bucket. For more information, see Step 2: Grant ElastiCache Access to Your Amazon S3 Bucket in the ElastiCache User Guide.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CopySnapshotRequest.Builder} avoiding the need to
* create one manually via {@link CopySnapshotRequest#builder()}
*
*
* @param copySnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CopySnapshotRequest.Builder} to create a request.
* Represents the input of a CopySnapshotMessage
operation.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidSnapshotStateException The current state of the snapshot does not allow the requested
* operation to occur.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CopySnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copySnapshot(Consumer copySnapshotRequest) {
return copySnapshot(CopySnapshotRequest.builder().applyMutation(copySnapshotRequest).build());
}
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant cache engine software, either
* Memcached, Valkey or Redis OSS.
*
*
* This operation is not supported for Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
* @param createCacheClusterRequest
* Represents the input of a CreateCacheCluster operation.
* @return A Java Future containing the result of the CreateCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - CacheClusterAlreadyExistsException You already have a cluster with the given identifier.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture createCacheCluster(CreateCacheClusterRequest createCacheClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a cluster. All nodes in the cluster run the same protocol-compliant cache engine software, either
* Memcached, Valkey or Redis OSS.
*
*
* This operation is not supported for Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCacheClusterRequest.Builder} avoiding the
* need to create one manually via {@link CreateCacheClusterRequest#builder()}
*
*
* @param createCacheClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateCacheClusterRequest.Builder} to create a
* request. Represents the input of a CreateCacheCluster operation.
* @return A Java Future containing the result of the CreateCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - CacheClusterAlreadyExistsException You already have a cluster with the given identifier.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture createCacheCluster(
Consumer createCacheClusterRequest) {
return createCacheCluster(CreateCacheClusterRequest.builder().applyMutation(createCacheClusterRequest).build());
}
/**
*
* Creates a new Amazon ElastiCache cache parameter group. An ElastiCache cache parameter group is a collection of
* parameters and their values that are applied to all of the nodes in any cluster or replication group using the
* CacheParameterGroup.
*
*
* A newly created CacheParameterGroup is an exact duplicate of the default parameter group for the
* CacheParameterGroupFamily. To customize the newly created CacheParameterGroup you can change the values of
* specific parameters. For more information, see:
*
*
* -
*
*
* ModifyCacheParameterGroup in the ElastiCache API Reference.
*
*
* -
*
* Parameters and Parameter
* Groups in the ElastiCache User Guide.
*
*
*
*
* @param createCacheParameterGroupRequest
* Represents the input of a CreateCacheParameterGroup
operation.
* @return A Java Future containing the result of the CreateCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupQuotaExceededException The request cannot be processed because it would exceed the
* maximum number of cache security groups.
* - CacheParameterGroupAlreadyExistsException A cache parameter group with the requested name already
* exists.
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheParameterGroup(
CreateCacheParameterGroupRequest createCacheParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon ElastiCache cache parameter group. An ElastiCache cache parameter group is a collection of
* parameters and their values that are applied to all of the nodes in any cluster or replication group using the
* CacheParameterGroup.
*
*
* A newly created CacheParameterGroup is an exact duplicate of the default parameter group for the
* CacheParameterGroupFamily. To customize the newly created CacheParameterGroup you can change the values of
* specific parameters. For more information, see:
*
*
* -
*
*
* ModifyCacheParameterGroup in the ElastiCache API Reference.
*
*
* -
*
* Parameters and Parameter
* Groups in the ElastiCache User Guide.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateCacheParameterGroupRequest.Builder} avoiding
* the need to create one manually via {@link CreateCacheParameterGroupRequest#builder()}
*
*
* @param createCacheParameterGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateCacheParameterGroupRequest.Builder} to
* create a request. Represents the input of a CreateCacheParameterGroup
operation.
* @return A Java Future containing the result of the CreateCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupQuotaExceededException The request cannot be processed because it would exceed the
* maximum number of cache security groups.
* - CacheParameterGroupAlreadyExistsException A cache parameter group with the requested name already
* exists.
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheParameterGroup(
Consumer createCacheParameterGroupRequest) {
return createCacheParameterGroup(CreateCacheParameterGroupRequest.builder()
.applyMutation(createCacheParameterGroupRequest).build());
}
/**
*
* Creates a new cache security group. Use a cache security group to control access to one or more clusters.
*
*
* Cache security groups are only used when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC). If you are creating a cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
* @param createCacheSecurityGroupRequest
* Represents the input of a CreateCacheSecurityGroup
operation.
* @return A Java Future containing the result of the CreateCacheSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupAlreadyExistsException A cache security group with the specified name already
* exists.
* - CacheSecurityGroupQuotaExceededException The request cannot be processed because it would exceed the
* allowed number of cache security groups.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheSecurityGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheSecurityGroup(
CreateCacheSecurityGroupRequest createCacheSecurityGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new cache security group. Use a cache security group to control access to one or more clusters.
*
*
* Cache security groups are only used when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC). If you are creating a cluster inside of a VPC, use a cache subnet group instead. For more
* information, see CreateCacheSubnetGroup.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCacheSecurityGroupRequest.Builder} avoiding
* the need to create one manually via {@link CreateCacheSecurityGroupRequest#builder()}
*
*
* @param createCacheSecurityGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateCacheSecurityGroupRequest.Builder} to
* create a request. Represents the input of a CreateCacheSecurityGroup
operation.
* @return A Java Future containing the result of the CreateCacheSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupAlreadyExistsException A cache security group with the specified name already
* exists.
* - CacheSecurityGroupQuotaExceededException The request cannot be processed because it would exceed the
* allowed number of cache security groups.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheSecurityGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheSecurityGroup(
Consumer createCacheSecurityGroupRequest) {
return createCacheSecurityGroup(CreateCacheSecurityGroupRequest.builder().applyMutation(createCacheSecurityGroupRequest)
.build());
}
/**
*
* Creates a new cache subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createCacheSubnetGroupRequest
* Represents the input of a CreateCacheSubnetGroup
operation.
* @return A Java Future containing the result of the CreateCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupAlreadyExistsException The requested cache subnet group name is already in use by an
* existing cache subnet group.
* - CacheSubnetGroupQuotaExceededException The request cannot be processed because it would exceed the
* allowed number of cache subnet groups.
* - CacheSubnetQuotaExceededException The request cannot be processed because it would exceed the allowed
* number of subnets in a cache subnet group.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidSubnetException An invalid subnet identifier was specified.
* - SubnetNotAllowedException At least one subnet ID does not match the other subnet IDs. This mismatch
* typically occurs when a user sets one subnet ID to a regional Availability Zone and a different one to an
* outpost. Or when a user sets the subnet ID to an Outpost when not subscribed on this service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheSubnetGroup(
CreateCacheSubnetGroupRequest createCacheSubnetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new cache subnet group.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* This is a convenience which creates an instance of the {@link CreateCacheSubnetGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateCacheSubnetGroupRequest#builder()}
*
*
* @param createCacheSubnetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateCacheSubnetGroupRequest.Builder} to create
* a request. Represents the input of a CreateCacheSubnetGroup
operation.
* @return A Java Future containing the result of the CreateCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupAlreadyExistsException The requested cache subnet group name is already in use by an
* existing cache subnet group.
* - CacheSubnetGroupQuotaExceededException The request cannot be processed because it would exceed the
* allowed number of cache subnet groups.
* - CacheSubnetQuotaExceededException The request cannot be processed because it would exceed the allowed
* number of subnets in a cache subnet group.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidSubnetException An invalid subnet identifier was specified.
* - SubnetNotAllowedException At least one subnet ID does not match the other subnet IDs. This mismatch
* typically occurs when a user sets one subnet ID to a regional Availability Zone and a different one to an
* outpost. Or when a user sets the subnet ID to an Outpost when not subscribed on this service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture createCacheSubnetGroup(
Consumer createCacheSubnetGroupRequest) {
return createCacheSubnetGroup(CreateCacheSubnetGroupRequest.builder().applyMutation(createCacheSubnetGroupRequest)
.build());
}
/**
*
* Global Datastore offers fully managed, fast, reliable and secure cross-region replication. Using Global Datastore
* with Valkey or Redis OSS, you can create cross-region read replica clusters for ElastiCache to enable low-latency
* reads and disaster recovery across regions. For more information, see Replication Across
* Regions Using Global Datastore.
*
*
* -
*
* The GlobalReplicationGroupIdSuffix is the name of the Global datastore.
*
*
* -
*
* The PrimaryReplicationGroupId represents the name of the primary cluster that accepts writes and will
* replicate updates to the secondary cluster.
*
*
*
*
* @param createGlobalReplicationGroupRequest
* @return A Java Future containing the result of the CreateGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - GlobalReplicationGroupAlreadyExistsException The Global datastore name already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture createGlobalReplicationGroup(
CreateGlobalReplicationGroupRequest createGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Global Datastore offers fully managed, fast, reliable and secure cross-region replication. Using Global Datastore
* with Valkey or Redis OSS, you can create cross-region read replica clusters for ElastiCache to enable low-latency
* reads and disaster recovery across regions. For more information, see Replication Across
* Regions Using Global Datastore.
*
*
* -
*
* The GlobalReplicationGroupIdSuffix is the name of the Global datastore.
*
*
* -
*
* The PrimaryReplicationGroupId represents the name of the primary cluster that accepts writes and will
* replicate updates to the secondary cluster.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateGlobalReplicationGroupRequest.Builder}
* avoiding the need to create one manually via {@link CreateGlobalReplicationGroupRequest#builder()}
*
*
* @param createGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateGlobalReplicationGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - GlobalReplicationGroupAlreadyExistsException The Global datastore name already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture createGlobalReplicationGroup(
Consumer createGlobalReplicationGroupRequest) {
return createGlobalReplicationGroup(CreateGlobalReplicationGroupRequest.builder()
.applyMutation(createGlobalReplicationGroupRequest).build());
}
/**
*
* Creates a Valkey or Redis OSS (cluster mode disabled) or a Valkey or Redis OSS (cluster mode enabled) replication
* group.
*
*
* This API can be used to create a standalone regional replication group or a secondary replication group
* associated with a Global datastore.
*
*
* A Valkey or Redis OSS (cluster mode disabled) replication group is a collection of nodes, where one of the nodes
* is a read/write primary and the others are read-only replicas. Writes to the primary are asynchronously
* propagated to the replicas.
*
*
* A Valkey or Redis OSS cluster-mode enabled cluster is comprised of from 1 to 90 shards (API/CLI: node groups).
* Each shard has a primary node and up to 5 read-only replica nodes. The configuration can range from 90 shards and
* 0 replicas to 15 shards and 5 replicas, which is the maximum number or replicas allowed.
*
*
* The node or shard limit can be increased to a maximum of 500 per cluster if the Valkey or Redis OSS engine
* version is 5.0.6 or higher. For example, you can choose to configure a 500 node cluster that ranges between 83
* shards (one primary and 5 replicas per shard) and 500 shards (single primary and no replicas). Make sure there
* are enough available IP addresses to accommodate the increase. Common pitfalls include the subnets in the subnet
* group have too small a CIDR range or the subnets are shared and heavily used by other clusters. For more
* information, see Creating a Subnet
* Group. For versions below 5.0.6, the limit is 250 per cluster.
*
*
* To request a limit increase, see Amazon Service Limits and choose
* the limit type Nodes per cluster per instance type.
*
*
* When a Valkey or Redis OSS (cluster mode disabled) replication group has been successfully created, you can add
* one or more read replicas to it, up to a total of 5 read replicas. If you need to increase or decrease the number
* of node groups (console: shards), you can use scaling. For more information, see Scaling self-designed clusters in
* the ElastiCache User Guide.
*
*
*
* This operation is valid for Valkey and Redis OSS only.
*
*
*
* @param createReplicationGroupRequest
* Represents the input of a CreateReplicationGroup
operation.
* @return A Java Future containing the result of the CreateReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - ReplicationGroupAlreadyExistsException The specified replication group already exists.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture createReplicationGroup(
CreateReplicationGroupRequest createReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Valkey or Redis OSS (cluster mode disabled) or a Valkey or Redis OSS (cluster mode enabled) replication
* group.
*
*
* This API can be used to create a standalone regional replication group or a secondary replication group
* associated with a Global datastore.
*
*
* A Valkey or Redis OSS (cluster mode disabled) replication group is a collection of nodes, where one of the nodes
* is a read/write primary and the others are read-only replicas. Writes to the primary are asynchronously
* propagated to the replicas.
*
*
* A Valkey or Redis OSS cluster-mode enabled cluster is comprised of from 1 to 90 shards (API/CLI: node groups).
* Each shard has a primary node and up to 5 read-only replica nodes. The configuration can range from 90 shards and
* 0 replicas to 15 shards and 5 replicas, which is the maximum number or replicas allowed.
*
*
* The node or shard limit can be increased to a maximum of 500 per cluster if the Valkey or Redis OSS engine
* version is 5.0.6 or higher. For example, you can choose to configure a 500 node cluster that ranges between 83
* shards (one primary and 5 replicas per shard) and 500 shards (single primary and no replicas). Make sure there
* are enough available IP addresses to accommodate the increase. Common pitfalls include the subnets in the subnet
* group have too small a CIDR range or the subnets are shared and heavily used by other clusters. For more
* information, see Creating a Subnet
* Group. For versions below 5.0.6, the limit is 250 per cluster.
*
*
* To request a limit increase, see Amazon Service Limits and choose
* the limit type Nodes per cluster per instance type.
*
*
* When a Valkey or Redis OSS (cluster mode disabled) replication group has been successfully created, you can add
* one or more read replicas to it, up to a total of 5 read replicas. If you need to increase or decrease the number
* of node groups (console: shards), you can use scaling. For more information, see Scaling self-designed clusters in
* the ElastiCache User Guide.
*
*
*
* This operation is valid for Valkey and Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link CreateReplicationGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateReplicationGroupRequest#builder()}
*
*
* @param createReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateReplicationGroupRequest.Builder} to create
* a request. Represents the input of a CreateReplicationGroup
operation.
* @return A Java Future containing the result of the CreateReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - ReplicationGroupAlreadyExistsException The specified replication group already exists.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture createReplicationGroup(
Consumer createReplicationGroupRequest) {
return createReplicationGroup(CreateReplicationGroupRequest.builder().applyMutation(createReplicationGroupRequest)
.build());
}
/**
*
* Creates a serverless cache.
*
*
* @param createServerlessCacheRequest
* @return A Java Future containing the result of the CreateServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheAlreadyExistsException A serverless cache with this name already exists.
* - ServerlessCacheQuotaForCustomerExceededException The number of serverless caches exceeds the customer
* quota.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture createServerlessCache(
CreateServerlessCacheRequest createServerlessCacheRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a serverless cache.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServerlessCacheRequest.Builder} avoiding the
* need to create one manually via {@link CreateServerlessCacheRequest#builder()}
*
*
* @param createServerlessCacheRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheAlreadyExistsException A serverless cache with this name already exists.
* - ServerlessCacheQuotaForCustomerExceededException The number of serverless caches exceeds the customer
* quota.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture createServerlessCache(
Consumer createServerlessCacheRequest) {
return createServerlessCache(CreateServerlessCacheRequest.builder().applyMutation(createServerlessCacheRequest).build());
}
/**
*
* This API creates a copy of an entire ServerlessCache at a specific moment in time. Available for Valkey, Redis
* OSS and Serverless Memcached only.
*
*
* @param createServerlessCacheSnapshotRequest
* @return A Java Future containing the result of the CreateServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotQuotaExceededException The number of serverless cache snapshots exceeds the
* customer snapshot quota. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture createServerlessCacheSnapshot(
CreateServerlessCacheSnapshotRequest createServerlessCacheSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API creates a copy of an entire ServerlessCache at a specific moment in time. Available for Valkey, Redis
* OSS and Serverless Memcached only.
*
*
*
* This is a convenience which creates an instance of the {@link CreateServerlessCacheSnapshotRequest.Builder}
* avoiding the need to create one manually via {@link CreateServerlessCacheSnapshotRequest#builder()}
*
*
* @param createServerlessCacheSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateServerlessCacheSnapshotRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotQuotaExceededException The number of serverless cache snapshots exceeds the
* customer snapshot quota. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture createServerlessCacheSnapshot(
Consumer createServerlessCacheSnapshotRequest) {
return createServerlessCacheSnapshot(CreateServerlessCacheSnapshotRequest.builder()
.applyMutation(createServerlessCacheSnapshotRequest).build());
}
/**
*
* Creates a copy of an entire cluster or replication group at a specific moment in time.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param createSnapshotRequest
* Represents the input of a CreateSnapshot
operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture createSnapshot(CreateSnapshotRequest createSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a copy of an entire cluster or replication group at a specific moment in time.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSnapshotRequest.Builder} avoiding the need to
* create one manually via {@link CreateSnapshotRequest#builder()}
*
*
* @param createSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateSnapshotRequest.Builder} to create a
* request. Represents the input of a CreateSnapshot
operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture createSnapshot(Consumer createSnapshotRequest) {
return createSnapshot(CreateSnapshotRequest.builder().applyMutation(createSnapshotRequest).build());
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 and onwards: Creates a user. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserAlreadyExistsException A user with this ID already exists.
* - UserQuotaExceededException The quota of users has been exceeded.
* - DuplicateUserNameException A user with this username already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 and onwards: Creates a user. For more information, see Using Role Based Access Control
* (RBAC).
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserAlreadyExistsException A user with this ID already exists.
* - UserQuotaExceededException The quota of users has been exceeded.
* - DuplicateUserNameException A user with this username already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Creates a user group. For more information, see
* Using Role Based Access
* Control (RBAC)
*
*
* @param createUserGroupRequest
* @return A Java Future containing the result of the CreateUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - DuplicateUserNameException A user with this username already exists.
* - UserGroupAlreadyExistsException The user group with this ID already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - DefaultUserRequiredException You must add default user to a user group.
* - UserGroupQuotaExceededException The number of users exceeds the user group limit.
* - InvalidParameterValueException The value for a parameter is invalid.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createUserGroup(CreateUserGroupRequest createUserGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Creates a user group. For more information, see
* Using Role Based Access
* Control (RBAC)
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserGroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateUserGroupRequest#builder()}
*
*
* @param createUserGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.CreateUserGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - DuplicateUserNameException A user with this username already exists.
* - UserGroupAlreadyExistsException The user group with this ID already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - DefaultUserRequiredException You must add default user to a user group.
* - UserGroupQuotaExceededException The number of users exceeds the user group limit.
* - InvalidParameterValueException The value for a parameter is invalid.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.CreateUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createUserGroup(
Consumer createUserGroupRequest) {
return createUserGroup(CreateUserGroupRequest.builder().applyMutation(createUserGroupRequest).build());
}
/**
*
* Decreases the number of node groups in a Global datastore
*
*
* @param decreaseNodeGroupsInGlobalReplicationGroupRequest
* @return A Java Future containing the result of the DecreaseNodeGroupsInGlobalReplicationGroup operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DecreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture decreaseNodeGroupsInGlobalReplicationGroup(
DecreaseNodeGroupsInGlobalReplicationGroupRequest decreaseNodeGroupsInGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Decreases the number of node groups in a Global datastore
*
*
*
* This is a convenience which creates an instance of the
* {@link DecreaseNodeGroupsInGlobalReplicationGroupRequest.Builder} avoiding the need to create one manually via
* {@link DecreaseNodeGroupsInGlobalReplicationGroupRequest#builder()}
*
*
* @param decreaseNodeGroupsInGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DecreaseNodeGroupsInGlobalReplicationGroupRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DecreaseNodeGroupsInGlobalReplicationGroup operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DecreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture decreaseNodeGroupsInGlobalReplicationGroup(
Consumer decreaseNodeGroupsInGlobalReplicationGroupRequest) {
return decreaseNodeGroupsInGlobalReplicationGroup(DecreaseNodeGroupsInGlobalReplicationGroupRequest.builder()
.applyMutation(decreaseNodeGroupsInGlobalReplicationGroupRequest).build());
}
/**
*
* Dynamically decreases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
* @param decreaseReplicaCountRequest
* @return A Java Future containing the result of the DecreaseReplicaCount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - NoOperationException The operation was not performed because no changes were required.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DecreaseReplicaCount
* @see AWS API Documentation
*/
default CompletableFuture decreaseReplicaCount(
DecreaseReplicaCountRequest decreaseReplicaCountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Dynamically decreases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
*
* This is a convenience which creates an instance of the {@link DecreaseReplicaCountRequest.Builder} avoiding the
* need to create one manually via {@link DecreaseReplicaCountRequest#builder()}
*
*
* @param decreaseReplicaCountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DecreaseReplicaCount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - NoOperationException The operation was not performed because no changes were required.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DecreaseReplicaCount
* @see AWS API Documentation
*/
default CompletableFuture decreaseReplicaCount(
Consumer decreaseReplicaCountRequest) {
return decreaseReplicaCount(DecreaseReplicaCountRequest.builder().applyMutation(decreaseReplicaCountRequest).build());
}
/**
*
* Deletes a previously provisioned cluster. DeleteCacheCluster
deletes all associated cache nodes,
* node endpoints and the cluster itself. When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cluster; you cannot cancel or revert this operation.
*
*
* This operation is not valid for:
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled) clusters
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled) clusters
*
*
* -
*
* A cluster that is the last read replica of a replication group
*
*
* -
*
* A cluster that is the primary node of a replication group
*
*
* -
*
* A node group (shard) that has Multi-AZ mode enabled
*
*
* -
*
* A cluster from a Valkey or Redis OSS (cluster mode enabled) replication group
*
*
* -
*
* A cluster that is not in the available
state
*
*
*
*
* @param deleteCacheClusterRequest
* Represents the input of a DeleteCacheCluster
operation.
* @return A Java Future containing the result of the DeleteCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheCluster(DeleteCacheClusterRequest deleteCacheClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a previously provisioned cluster. DeleteCacheCluster
deletes all associated cache nodes,
* node endpoints and the cluster itself. When you receive a successful response from this operation, Amazon
* ElastiCache immediately begins deleting the cluster; you cannot cancel or revert this operation.
*
*
* This operation is not valid for:
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled) clusters
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled) clusters
*
*
* -
*
* A cluster that is the last read replica of a replication group
*
*
* -
*
* A cluster that is the primary node of a replication group
*
*
* -
*
* A node group (shard) that has Multi-AZ mode enabled
*
*
* -
*
* A cluster from a Valkey or Redis OSS (cluster mode enabled) replication group
*
*
* -
*
* A cluster that is not in the available
state
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCacheClusterRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCacheClusterRequest#builder()}
*
*
* @param deleteCacheClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteCacheClusterRequest.Builder} to create a
* request. Represents the input of a DeleteCacheCluster
operation.
* @return A Java Future containing the result of the DeleteCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheCluster(
Consumer deleteCacheClusterRequest) {
return deleteCacheCluster(DeleteCacheClusterRequest.builder().applyMutation(deleteCacheClusterRequest).build());
}
/**
*
* Deletes the specified cache parameter group. You cannot delete a cache parameter group if it is associated with
* any cache clusters. You cannot delete the default cache parameter groups in your account.
*
*
* @param deleteCacheParameterGroupRequest
* Represents the input of a DeleteCacheParameterGroup
operation.
* @return A Java Future containing the result of the DeleteCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheParameterGroup(
DeleteCacheParameterGroupRequest deleteCacheParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified cache parameter group. You cannot delete a cache parameter group if it is associated with
* any cache clusters. You cannot delete the default cache parameter groups in your account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCacheParameterGroupRequest.Builder} avoiding
* the need to create one manually via {@link DeleteCacheParameterGroupRequest#builder()}
*
*
* @param deleteCacheParameterGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteCacheParameterGroupRequest.Builder} to
* create a request. Represents the input of a DeleteCacheParameterGroup
operation.
* @return A Java Future containing the result of the DeleteCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheParameterGroup(
Consumer deleteCacheParameterGroupRequest) {
return deleteCacheParameterGroup(DeleteCacheParameterGroupRequest.builder()
.applyMutation(deleteCacheParameterGroupRequest).build());
}
/**
*
* Deletes a cache security group.
*
*
*
* You cannot delete a cache security group if it is associated with any clusters.
*
*
*
* @param deleteCacheSecurityGroupRequest
* Represents the input of a DeleteCacheSecurityGroup
operation.
* @return A Java Future containing the result of the DeleteCacheSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheSecurityGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheSecurityGroup(
DeleteCacheSecurityGroupRequest deleteCacheSecurityGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a cache security group.
*
*
*
* You cannot delete a cache security group if it is associated with any clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCacheSecurityGroupRequest.Builder} avoiding
* the need to create one manually via {@link DeleteCacheSecurityGroupRequest#builder()}
*
*
* @param deleteCacheSecurityGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteCacheSecurityGroupRequest.Builder} to
* create a request. Represents the input of a DeleteCacheSecurityGroup
operation.
* @return A Java Future containing the result of the DeleteCacheSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheSecurityGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheSecurityGroup(
Consumer deleteCacheSecurityGroupRequest) {
return deleteCacheSecurityGroup(DeleteCacheSecurityGroupRequest.builder().applyMutation(deleteCacheSecurityGroupRequest)
.build());
}
/**
*
* Deletes a cache subnet group.
*
*
*
* You cannot delete a default cache subnet group or one that is associated with any clusters.
*
*
*
* @param deleteCacheSubnetGroupRequest
* Represents the input of a DeleteCacheSubnetGroup
operation.
* @return A Java Future containing the result of the DeleteCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupInUseException The requested cache subnet group is currently in use.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheSubnetGroup(
DeleteCacheSubnetGroupRequest deleteCacheSubnetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a cache subnet group.
*
*
*
* You cannot delete a default cache subnet group or one that is associated with any clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCacheSubnetGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCacheSubnetGroupRequest#builder()}
*
*
* @param deleteCacheSubnetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteCacheSubnetGroupRequest.Builder} to create
* a request. Represents the input of a DeleteCacheSubnetGroup
operation.
* @return A Java Future containing the result of the DeleteCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupInUseException The requested cache subnet group is currently in use.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteCacheSubnetGroup(
Consumer deleteCacheSubnetGroupRequest) {
return deleteCacheSubnetGroup(DeleteCacheSubnetGroupRequest.builder().applyMutation(deleteCacheSubnetGroupRequest)
.build());
}
/**
*
* Deleting a Global datastore is a two-step process:
*
*
* -
*
* First, you must DisassociateGlobalReplicationGroup to remove the secondary clusters in the Global
* datastore.
*
*
* -
*
* Once the Global datastore contains only the primary cluster, you can use the
* DeleteGlobalReplicationGroup
API to delete the Global datastore while retainining the primary
* cluster using RetainPrimaryReplicationGroup=true
.
*
*
*
*
* Since the Global Datastore has only a primary cluster, you can delete the Global Datastore while retaining the
* primary by setting RetainPrimaryReplicationGroup=true
. The primary cluster is never deleted when
* deleting a Global Datastore. It can only be deleted when it no longer is associated with any Global Datastore.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
* @param deleteGlobalReplicationGroupRequest
* @return A Java Future containing the result of the DeleteGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalReplicationGroup(
DeleteGlobalReplicationGroupRequest deleteGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deleting a Global datastore is a two-step process:
*
*
* -
*
* First, you must DisassociateGlobalReplicationGroup to remove the secondary clusters in the Global
* datastore.
*
*
* -
*
* Once the Global datastore contains only the primary cluster, you can use the
* DeleteGlobalReplicationGroup
API to delete the Global datastore while retainining the primary
* cluster using RetainPrimaryReplicationGroup=true
.
*
*
*
*
* Since the Global Datastore has only a primary cluster, you can delete the Global Datastore while retaining the
* primary by setting RetainPrimaryReplicationGroup=true
. The primary cluster is never deleted when
* deleting a Global Datastore. It can only be deleted when it no longer is associated with any Global Datastore.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGlobalReplicationGroupRequest.Builder}
* avoiding the need to create one manually via {@link DeleteGlobalReplicationGroupRequest#builder()}
*
*
* @param deleteGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteGlobalReplicationGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalReplicationGroup(
Consumer deleteGlobalReplicationGroupRequest) {
return deleteGlobalReplicationGroup(DeleteGlobalReplicationGroupRequest.builder()
.applyMutation(deleteGlobalReplicationGroupRequest).build());
}
/**
*
* Deletes an existing replication group. By default, this operation deletes the entire replication group, including
* the primary/primaries and all of the read replicas. If the replication group has only one primary, you can
* optionally delete only the read replicas, while retaining the primary by setting
* RetainPrimaryCluster=true
.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
*
* -
*
* CreateSnapshot
permission is required to create a final snapshot. Without this permission, the API
* call will fail with an Access Denied
exception.
*
*
* -
*
* This operation is valid for Redis OSS only.
*
*
*
*
*
* @param deleteReplicationGroupRequest
* Represents the input of a DeleteReplicationGroup
operation.
* @return A Java Future containing the result of the DeleteReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteReplicationGroup(
DeleteReplicationGroupRequest deleteReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing replication group. By default, this operation deletes the entire replication group, including
* the primary/primaries and all of the read replicas. If the replication group has only one primary, you can
* optionally delete only the read replicas, while retaining the primary by setting
* RetainPrimaryCluster=true
.
*
*
* When you receive a successful response from this operation, Amazon ElastiCache immediately begins deleting the
* selected resources; you cannot cancel or revert this operation.
*
*
*
* -
*
* CreateSnapshot
permission is required to create a final snapshot. Without this permission, the API
* call will fail with an Access Denied
exception.
*
*
* -
*
* This operation is valid for Redis OSS only.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DeleteReplicationGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteReplicationGroupRequest#builder()}
*
*
* @param deleteReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteReplicationGroupRequest.Builder} to create
* a request. Represents the input of a DeleteReplicationGroup
operation.
* @return A Java Future containing the result of the DeleteReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - SnapshotAlreadyExistsException You already have a snapshot with the given name.
* - SnapshotFeatureNotSupportedException You attempted one of the following operations:
*
* -
*
* Creating a snapshot of a Valkey or Redis OSS cluster running on a cache.t1.micro
cache node.
*
*
* -
*
* Creating a snapshot of a cluster that is running Memcached rather than Valkey or Redis OSS.
*
*
*
*
* Neither of these are supported by ElastiCache.
* - SnapshotQuotaExceededException The request cannot be processed because it would exceed the maximum
* number of snapshots.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteReplicationGroup(
Consumer deleteReplicationGroupRequest) {
return deleteReplicationGroup(DeleteReplicationGroupRequest.builder().applyMutation(deleteReplicationGroupRequest)
.build());
}
/**
*
* Deletes a specified existing serverless cache.
*
*
*
* CreateServerlessCacheSnapshot
permission is required to create a final snapshot. Without this
* permission, the API call will fail with an Access Denied
exception.
*
*
*
* @param deleteServerlessCacheRequest
* @return A Java Future containing the result of the DeleteServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture deleteServerlessCache(
DeleteServerlessCacheRequest deleteServerlessCacheRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified existing serverless cache.
*
*
*
* CreateServerlessCacheSnapshot
permission is required to create a final snapshot. Without this
* permission, the API call will fail with an Access Denied
exception.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerlessCacheRequest.Builder} avoiding the
* need to create one manually via {@link DeleteServerlessCacheRequest#builder()}
*
*
* @param deleteServerlessCacheRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotAlreadyExistsException A serverless cache snapshot with this name already
* exists. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture deleteServerlessCache(
Consumer deleteServerlessCacheRequest) {
return deleteServerlessCache(DeleteServerlessCacheRequest.builder().applyMutation(deleteServerlessCacheRequest).build());
}
/**
*
* Deletes an existing serverless cache snapshot. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
* @param deleteServerlessCacheSnapshotRequest
* @return A Java Future containing the result of the DeleteServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture deleteServerlessCacheSnapshot(
DeleteServerlessCacheSnapshotRequest deleteServerlessCacheSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing serverless cache snapshot. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerlessCacheSnapshotRequest.Builder}
* avoiding the need to create one manually via {@link DeleteServerlessCacheSnapshotRequest#builder()}
*
*
* @param deleteServerlessCacheSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteServerlessCacheSnapshotRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture deleteServerlessCacheSnapshot(
Consumer deleteServerlessCacheSnapshotRequest) {
return deleteServerlessCacheSnapshot(DeleteServerlessCacheSnapshotRequest.builder()
.applyMutation(deleteServerlessCacheSnapshotRequest).build());
}
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param deleteSnapshotRequest
* Represents the input of a DeleteSnapshot
operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidSnapshotStateException The current state of the snapshot does not allow the requested
* operation to occur.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing snapshot. When you receive a successful response from this operation, ElastiCache immediately
* begins deleting the snapshot; you cannot cancel or revert this operation.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSnapshotRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSnapshotRequest#builder()}
*
*
* @param deleteSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteSnapshotRequest.Builder} to create a
* request. Represents the input of a DeleteSnapshot
operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidSnapshotStateException The current state of the snapshot does not allow the requested
* operation to occur.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSnapshot(Consumer deleteSnapshotRequest) {
return deleteSnapshot(DeleteSnapshotRequest.builder().applyMutation(deleteSnapshotRequest).build());
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user. The user will be removed from
* all user groups and in turn removed from all replication groups. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidUserStateException The user is not in active state.
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - DefaultUserAssociatedToUserGroupException The default user assigned to the user group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user. The user will be removed from
* all user groups and in turn removed from all replication groups. For more information, see Using Role Based Access Control
* (RBAC).
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidUserStateException The user is not in active state.
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - DefaultUserAssociatedToUserGroupException The default user assigned to the user group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user group. The user group must first
* be disassociated from the replication group before it can be deleted. For more information, see Using Role Based Access Control
* (RBAC).
*
*
* @param deleteUserGroupRequest
* @return A Java Future containing the result of the DeleteUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - InvalidUserGroupStateException The user group is not in an active state.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUserGroup(DeleteUserGroupRequest deleteUserGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* For Valkey engine version 7.2 onwards and Redis OSS 6.0 onwards: Deletes a user group. The user group must first
* be disassociated from the replication group before it can be deleted. For more information, see Using Role Based Access Control
* (RBAC).
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserGroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteUserGroupRequest#builder()}
*
*
* @param deleteUserGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DeleteUserGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - InvalidUserGroupStateException The user group is not in an active state.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DeleteUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteUserGroup(
Consumer deleteUserGroupRequest) {
return deleteUserGroup(DeleteUserGroupRequest.builder().applyMutation(deleteUserGroupRequest).build());
}
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cache cluster if a cluster identifier is supplied.
*
*
* By default, abbreviated information about the clusters is returned. You can use the optional
* ShowCacheNodeInfo flag to retrieve detailed information about the cache nodes associated with the
* clusters. These details include the DNS address and port for the cache node endpoint.
*
*
* If the cluster is in the creating state, only cluster-level information is displayed until all of the
* nodes are successfully provisioned.
*
*
* If the cluster is in the deleting state, only cluster-level information is displayed.
*
*
* If cache nodes are currently being added to the cluster, node endpoint information and creation time for the
* additional nodes are not displayed until they are completely provisioned. When the cluster state is
* available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cluster, no endpoint information for the removed nodes is
* displayed.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters
operation.
* @return A Java Future containing the result of the DescribeCacheClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default CompletableFuture describeCacheClusters(
DescribeCacheClustersRequest describeCacheClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cache cluster if a cluster identifier is supplied.
*
*
* By default, abbreviated information about the clusters is returned. You can use the optional
* ShowCacheNodeInfo flag to retrieve detailed information about the cache nodes associated with the
* clusters. These details include the DNS address and port for the cache node endpoint.
*
*
* If the cluster is in the creating state, only cluster-level information is displayed until all of the
* nodes are successfully provisioned.
*
*
* If the cluster is in the deleting state, only cluster-level information is displayed.
*
*
* If cache nodes are currently being added to the cluster, node endpoint information and creation time for the
* additional nodes are not displayed until they are completely provisioned. When the cluster state is
* available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cluster, no endpoint information for the removed nodes is
* displayed.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCacheClustersRequest#builder()}
*
*
* @param describeCacheClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest.Builder} to create a
* request. Represents the input of a DescribeCacheClusters
operation.
* @return A Java Future containing the result of the DescribeCacheClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default CompletableFuture describeCacheClusters(
Consumer describeCacheClustersRequest) {
return describeCacheClusters(DescribeCacheClustersRequest.builder().applyMutation(describeCacheClustersRequest).build());
}
/**
*
* Returns information about all provisioned clusters if no cluster identifier is specified, or about a specific
* cache cluster if a cluster identifier is supplied.
*
*
* By default, abbreviated information about the clusters is returned. You can use the optional
* ShowCacheNodeInfo flag to retrieve detailed information about the cache nodes associated with the
* clusters. These details include the DNS address and port for the cache node endpoint.
*
*
* If the cluster is in the creating state, only cluster-level information is displayed until all of the
* nodes are successfully provisioned.
*
*
* If the cluster is in the deleting state, only cluster-level information is displayed.
*
*
* If cache nodes are currently being added to the cluster, node endpoint information and creation time for the
* additional nodes are not displayed until they are completely provisioned. When the cluster state is
* available, the cluster is ready for use.
*
*
* If cache nodes are currently being removed from the cluster, no endpoint information for the removed nodes is
* displayed.
*
*
* @return A Java Future containing the result of the DescribeCacheClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default CompletableFuture describeCacheClusters() {
return describeCacheClusters(DescribeCacheClustersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default DescribeCacheClustersPublisher describeCacheClustersPaginator() {
return describeCacheClustersPaginator(DescribeCacheClustersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation.
*
*
* @param describeCacheClustersRequest
* Represents the input of a DescribeCacheClusters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default DescribeCacheClustersPublisher describeCacheClustersPaginator(
DescribeCacheClustersRequest describeCacheClustersRequest) {
return new DescribeCacheClustersPublisher(this, describeCacheClustersRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheClustersPublisher publisher = client.describeCacheClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheClusters(software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCacheClustersRequest#builder()}
*
*
* @param describeCacheClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheClustersRequest.Builder} to create a
* request. Represents the input of a DescribeCacheClusters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheClusters
* @see AWS API Documentation
*/
default DescribeCacheClustersPublisher describeCacheClustersPaginator(
Consumer describeCacheClustersRequest) {
return describeCacheClustersPaginator(DescribeCacheClustersRequest.builder().applyMutation(describeCacheClustersRequest)
.build());
}
/**
*
* Returns a list of the available cache engines and their versions.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
operation.
* @return A Java Future containing the result of the DescribeCacheEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default CompletableFuture describeCacheEngineVersions(
DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the available cache engines and their versions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheEngineVersionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheEngineVersionsRequest#builder()}
*
*
* @param describeCacheEngineVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheEngineVersions
operation.
* @return A Java Future containing the result of the DescribeCacheEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default CompletableFuture describeCacheEngineVersions(
Consumer describeCacheEngineVersionsRequest) {
return describeCacheEngineVersions(DescribeCacheEngineVersionsRequest.builder()
.applyMutation(describeCacheEngineVersionsRequest).build());
}
/**
*
* Returns a list of the available cache engines and their versions.
*
*
* @return A Java Future containing the result of the DescribeCacheEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default CompletableFuture describeCacheEngineVersions() {
return describeCacheEngineVersions(DescribeCacheEngineVersionsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default DescribeCacheEngineVersionsPublisher describeCacheEngineVersionsPaginator() {
return describeCacheEngineVersionsPaginator(DescribeCacheEngineVersionsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation.
*
*
* @param describeCacheEngineVersionsRequest
* Represents the input of a DescribeCacheEngineVersions
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default DescribeCacheEngineVersionsPublisher describeCacheEngineVersionsPaginator(
DescribeCacheEngineVersionsRequest describeCacheEngineVersionsRequest) {
return new DescribeCacheEngineVersionsPublisher(this, describeCacheEngineVersionsRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheEngineVersionsPublisher publisher = client.describeCacheEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheEngineVersions(software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheEngineVersionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheEngineVersionsRequest#builder()}
*
*
* @param describeCacheEngineVersionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheEngineVersionsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheEngineVersions
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheEngineVersions
* @see AWS API Documentation
*/
default DescribeCacheEngineVersionsPublisher describeCacheEngineVersionsPaginator(
Consumer describeCacheEngineVersionsRequest) {
return describeCacheEngineVersionsPaginator(DescribeCacheEngineVersionsRequest.builder()
.applyMutation(describeCacheEngineVersionsRequest).build());
}
/**
*
* Returns a list of cache parameter group descriptions. If a cache parameter group name is specified, the list
* contains only the descriptions for that group.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
operation.
* @return A Java Future containing the result of the DescribeCacheParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheParameterGroups(
DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of cache parameter group descriptions. If a cache parameter group name is specified, the list
* contains only the descriptions for that group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheParameterGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheParameterGroupsRequest#builder()}
*
*
* @param describeCacheParameterGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheParameterGroups
operation.
* @return A Java Future containing the result of the DescribeCacheParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheParameterGroups(
Consumer describeCacheParameterGroupsRequest) {
return describeCacheParameterGroups(DescribeCacheParameterGroupsRequest.builder()
.applyMutation(describeCacheParameterGroupsRequest).build());
}
/**
*
* Returns a list of cache parameter group descriptions. If a cache parameter group name is specified, the list
* contains only the descriptions for that group.
*
*
* @return A Java Future containing the result of the DescribeCacheParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheParameterGroups() {
return describeCacheParameterGroups(DescribeCacheParameterGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default DescribeCacheParameterGroupsPublisher describeCacheParameterGroupsPaginator() {
return describeCacheParameterGroupsPaginator(DescribeCacheParameterGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation.
*
*
* @param describeCacheParameterGroupsRequest
* Represents the input of a DescribeCacheParameterGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default DescribeCacheParameterGroupsPublisher describeCacheParameterGroupsPaginator(
DescribeCacheParameterGroupsRequest describeCacheParameterGroupsRequest) {
return new DescribeCacheParameterGroupsPublisher(this, describeCacheParameterGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParameterGroupsPublisher publisher = client.describeCacheParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheParameterGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheParameterGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheParameterGroupsRequest#builder()}
*
*
* @param describeCacheParameterGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheParameterGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheParameterGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameterGroups
* @see AWS API Documentation
*/
default DescribeCacheParameterGroupsPublisher describeCacheParameterGroupsPaginator(
Consumer describeCacheParameterGroupsRequest) {
return describeCacheParameterGroupsPaginator(DescribeCacheParameterGroupsRequest.builder()
.applyMutation(describeCacheParameterGroupsRequest).build());
}
/**
*
* Returns the detailed parameter list for a particular cache parameter group.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters
operation.
* @return A Java Future containing the result of the DescribeCacheParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameters
* @see AWS API Documentation
*/
default CompletableFuture describeCacheParameters(
DescribeCacheParametersRequest describeCacheParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the detailed parameter list for a particular cache parameter group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheParametersRequest.Builder} avoiding
* the need to create one manually via {@link DescribeCacheParametersRequest#builder()}
*
*
* @param describeCacheParametersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest.Builder} to create
* a request. Represents the input of a DescribeCacheParameters
operation.
* @return A Java Future containing the result of the DescribeCacheParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameters
* @see AWS API Documentation
*/
default CompletableFuture describeCacheParameters(
Consumer describeCacheParametersRequest) {
return describeCacheParameters(DescribeCacheParametersRequest.builder().applyMutation(describeCacheParametersRequest)
.build());
}
/**
*
* This is a variant of
* {@link #describeCacheParameters(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParametersPublisher publisher = client.describeCacheParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParametersPublisher publisher = client.describeCacheParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheParameters(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest)}
* operation.
*
*
* @param describeCacheParametersRequest
* Represents the input of a DescribeCacheParameters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameters
* @see AWS API Documentation
*/
default DescribeCacheParametersPublisher describeCacheParametersPaginator(
DescribeCacheParametersRequest describeCacheParametersRequest) {
return new DescribeCacheParametersPublisher(this, describeCacheParametersRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheParameters(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParametersPublisher publisher = client.describeCacheParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheParametersPublisher publisher = client.describeCacheParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheParameters(software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheParametersRequest.Builder} avoiding
* the need to create one manually via {@link DescribeCacheParametersRequest#builder()}
*
*
* @param describeCacheParametersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheParametersRequest.Builder} to create
* a request. Represents the input of a DescribeCacheParameters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheParameters
* @see AWS API Documentation
*/
default DescribeCacheParametersPublisher describeCacheParametersPaginator(
Consumer describeCacheParametersRequest) {
return describeCacheParametersPaginator(DescribeCacheParametersRequest.builder()
.applyMutation(describeCacheParametersRequest).build());
}
/**
*
* Returns a list of cache security group descriptions. If a cache security group name is specified, the list
* contains only the description of that group. This applicable only when you have ElastiCache in Classic setup
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
operation.
* @return A Java Future containing the result of the DescribeCacheSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheSecurityGroups(
DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of cache security group descriptions. If a cache security group name is specified, the list
* contains only the description of that group. This applicable only when you have ElastiCache in Classic setup
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheSecurityGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheSecurityGroupsRequest#builder()}
*
*
* @param describeCacheSecurityGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheSecurityGroups
operation.
* @return A Java Future containing the result of the DescribeCacheSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheSecurityGroups(
Consumer describeCacheSecurityGroupsRequest) {
return describeCacheSecurityGroups(DescribeCacheSecurityGroupsRequest.builder()
.applyMutation(describeCacheSecurityGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeCacheSecurityGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSecurityGroupsPublisher publisher = client.describeCacheSecurityGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSecurityGroupsPublisher publisher = client.describeCacheSecurityGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheSecurityGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest)}
* operation.
*
*
* @param describeCacheSecurityGroupsRequest
* Represents the input of a DescribeCacheSecurityGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
default DescribeCacheSecurityGroupsPublisher describeCacheSecurityGroupsPaginator(
DescribeCacheSecurityGroupsRequest describeCacheSecurityGroupsRequest) {
return new DescribeCacheSecurityGroupsPublisher(this, describeCacheSecurityGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheSecurityGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSecurityGroupsPublisher publisher = client.describeCacheSecurityGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSecurityGroupsPublisher publisher = client.describeCacheSecurityGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheSecurityGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheSecurityGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCacheSecurityGroupsRequest#builder()}
*
*
* @param describeCacheSecurityGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheSecurityGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheSecurityGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSecurityGroups
* @see AWS API Documentation
*/
default DescribeCacheSecurityGroupsPublisher describeCacheSecurityGroupsPaginator(
Consumer describeCacheSecurityGroupsRequest) {
return describeCacheSecurityGroupsPaginator(DescribeCacheSecurityGroupsRequest.builder()
.applyMutation(describeCacheSecurityGroupsRequest).build());
}
/**
*
* Returns a list of cache subnet group descriptions. If a subnet group name is specified, the list contains only
* the description of that group. This is applicable only when you have ElastiCache in VPC setup. All ElastiCache
* clusters now launch in VPC by default.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups
operation.
* @return A Java Future containing the result of the DescribeCacheSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheSubnetGroups(
DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of cache subnet group descriptions. If a subnet group name is specified, the list contains only
* the description of that group. This is applicable only when you have ElastiCache in VPC setup. All ElastiCache
* clusters now launch in VPC by default.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheSubnetGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeCacheSubnetGroupsRequest#builder()}
*
*
* @param describeCacheSubnetGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheSubnetGroups
operation.
* @return A Java Future containing the result of the DescribeCacheSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheSubnetGroups(
Consumer describeCacheSubnetGroupsRequest) {
return describeCacheSubnetGroups(DescribeCacheSubnetGroupsRequest.builder()
.applyMutation(describeCacheSubnetGroupsRequest).build());
}
/**
*
* Returns a list of cache subnet group descriptions. If a subnet group name is specified, the list contains only
* the description of that group. This is applicable only when you have ElastiCache in VPC setup. All ElastiCache
* clusters now launch in VPC by default.
*
*
* @return A Java Future containing the result of the DescribeCacheSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeCacheSubnetGroups() {
return describeCacheSubnetGroups(DescribeCacheSubnetGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default DescribeCacheSubnetGroupsPublisher describeCacheSubnetGroupsPaginator() {
return describeCacheSubnetGroupsPaginator(DescribeCacheSubnetGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation.
*
*
* @param describeCacheSubnetGroupsRequest
* Represents the input of a DescribeCacheSubnetGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default DescribeCacheSubnetGroupsPublisher describeCacheSubnetGroupsPaginator(
DescribeCacheSubnetGroupsRequest describeCacheSubnetGroupsRequest) {
return new DescribeCacheSubnetGroupsPublisher(this, describeCacheSubnetGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeCacheSubnetGroupsPublisher publisher = client.describeCacheSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCacheSubnetGroups(software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCacheSubnetGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeCacheSubnetGroupsRequest#builder()}
*
*
* @param describeCacheSubnetGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeCacheSubnetGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeCacheSubnetGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeCacheSubnetGroups
* @see AWS API Documentation
*/
default DescribeCacheSubnetGroupsPublisher describeCacheSubnetGroupsPaginator(
Consumer describeCacheSubnetGroupsRequest) {
return describeCacheSubnetGroupsPaginator(DescribeCacheSubnetGroupsRequest.builder()
.applyMutation(describeCacheSubnetGroupsRequest).build());
}
/**
*
* Returns the default engine and system parameter information for the specified cache engine.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
operation.
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultParameters(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the default engine and system parameter information for the specified cache engine.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEngineDefaultParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEngineDefaultParametersRequest#builder()}
*
*
* @param describeEngineDefaultParametersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest.Builder}
* to create a request. Represents the input of a DescribeEngineDefaultParameters
operation.
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultParameters(
Consumer describeEngineDefaultParametersRequest) {
return describeEngineDefaultParameters(DescribeEngineDefaultParametersRequest.builder()
.applyMutation(describeEngineDefaultParametersRequest).build());
}
/**
*
* This is a variant of
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest)}
* operation.
*
*
* @param describeEngineDefaultParametersRequest
* Represents the input of a DescribeEngineDefaultParameters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default DescribeEngineDefaultParametersPublisher describeEngineDefaultParametersPaginator(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest) {
return new DescribeEngineDefaultParametersPublisher(this, describeEngineDefaultParametersRequest);
}
/**
*
* This is a variant of
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEngineDefaultParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEngineDefaultParametersRequest#builder()}
*
*
* @param describeEngineDefaultParametersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeEngineDefaultParametersRequest.Builder}
* to create a request. Represents the input of a DescribeEngineDefaultParameters
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default DescribeEngineDefaultParametersPublisher describeEngineDefaultParametersPaginator(
Consumer describeEngineDefaultParametersRequest) {
return describeEngineDefaultParametersPaginator(DescribeEngineDefaultParametersRequest.builder()
.applyMutation(describeEngineDefaultParametersRequest).build());
}
/**
*
* Returns events related to clusters, cache security groups, and cache parameter groups. You can obtain events
* specific to a particular cluster, cache security group, or cache parameter group by providing the name as a
* parameter.
*
*
* By default, only the events occurring within the last hour are returned; however, you can retrieve up to 14 days'
* worth of events if necessary.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents
operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default CompletableFuture describeEvents(DescribeEventsRequest describeEventsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns events related to clusters, cache security groups, and cache parameter groups. You can obtain events
* specific to a particular cluster, cache security group, or cache parameter group by providing the name as a
* parameter.
*
*
* By default, only the events occurring within the last hour are returned; however, you can retrieve up to 14 days'
* worth of events if necessary.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEventsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeEventsRequest#builder()}
*
*
* @param describeEventsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest.Builder} to create a
* request. Represents the input of a DescribeEvents
operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default CompletableFuture describeEvents(Consumer describeEventsRequest) {
return describeEvents(DescribeEventsRequest.builder().applyMutation(describeEventsRequest).build());
}
/**
*
* Returns events related to clusters, cache security groups, and cache parameter groups. You can obtain events
* specific to a particular cluster, cache security group, or cache parameter group by providing the name as a
* parameter.
*
*
* By default, only the events occurring within the last hour are returned; however, you can retrieve up to 14 days'
* worth of events if necessary.
*
*
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default CompletableFuture describeEvents() {
return describeEvents(DescribeEventsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator() {
return describeEventsPaginator(DescribeEventsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation.
*
*
* @param describeEventsRequest
* Represents the input of a DescribeEvents
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator(DescribeEventsRequest describeEventsRequest) {
return new DescribeEventsPublisher(this, describeEventsRequest);
}
/**
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEventsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeEventsRequest#builder()}
*
*
* @param describeEventsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeEventsRequest.Builder} to create a
* request. Represents the input of a DescribeEvents
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeEvents
* @see AWS
* API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator(Consumer describeEventsRequest) {
return describeEventsPaginator(DescribeEventsRequest.builder().applyMutation(describeEventsRequest).build());
}
/**
*
* Returns information about a particular global replication group. If no identifier is specified, returns
* information about all Global datastores.
*
*
* @param describeGlobalReplicationGroupsRequest
* @return A Java Future containing the result of the DescribeGlobalReplicationGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalReplicationGroups(
DescribeGlobalReplicationGroupsRequest describeGlobalReplicationGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a particular global replication group. If no identifier is specified, returns
* information about all Global datastores.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalReplicationGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeGlobalReplicationGroupsRequest#builder()}
*
*
* @param describeGlobalReplicationGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeGlobalReplicationGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalReplicationGroups(
Consumer describeGlobalReplicationGroupsRequest) {
return describeGlobalReplicationGroups(DescribeGlobalReplicationGroupsRequest.builder()
.applyMutation(describeGlobalReplicationGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeGlobalReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeGlobalReplicationGroupsPublisher publisher = client.describeGlobalReplicationGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeGlobalReplicationGroupsPublisher publisher = client.describeGlobalReplicationGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest)}
* operation.
*
*
* @param describeGlobalReplicationGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
default DescribeGlobalReplicationGroupsPublisher describeGlobalReplicationGroupsPaginator(
DescribeGlobalReplicationGroupsRequest describeGlobalReplicationGroupsRequest) {
return new DescribeGlobalReplicationGroupsPublisher(this, describeGlobalReplicationGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeGlobalReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeGlobalReplicationGroupsPublisher publisher = client.describeGlobalReplicationGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeGlobalReplicationGroupsPublisher publisher = client.describeGlobalReplicationGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalReplicationGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeGlobalReplicationGroupsRequest#builder()}
*
*
* @param describeGlobalReplicationGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeGlobalReplicationGroupsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeGlobalReplicationGroups
* @see AWS API Documentation
*/
default DescribeGlobalReplicationGroupsPublisher describeGlobalReplicationGroupsPaginator(
Consumer describeGlobalReplicationGroupsRequest) {
return describeGlobalReplicationGroupsPaginator(DescribeGlobalReplicationGroupsRequest.builder()
.applyMutation(describeGlobalReplicationGroupsRequest).build());
}
/**
*
* Returns information about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups
returns information about all replication groups.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups
operation.
* @return A Java Future containing the result of the DescribeReplicationGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default CompletableFuture describeReplicationGroups(
DescribeReplicationGroupsRequest describeReplicationGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups
returns information about all replication groups.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReplicationGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReplicationGroupsRequest#builder()}
*
*
* @param describeReplicationGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeReplicationGroups
operation.
* @return A Java Future containing the result of the DescribeReplicationGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default CompletableFuture describeReplicationGroups(
Consumer describeReplicationGroupsRequest) {
return describeReplicationGroups(DescribeReplicationGroupsRequest.builder()
.applyMutation(describeReplicationGroupsRequest).build());
}
/**
*
* Returns information about a particular replication group. If no identifier is specified,
* DescribeReplicationGroups
returns information about all replication groups.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @return A Java Future containing the result of the DescribeReplicationGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default CompletableFuture describeReplicationGroups() {
return describeReplicationGroups(DescribeReplicationGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default DescribeReplicationGroupsPublisher describeReplicationGroupsPaginator() {
return describeReplicationGroupsPaginator(DescribeReplicationGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation.
*
*
* @param describeReplicationGroupsRequest
* Represents the input of a DescribeReplicationGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default DescribeReplicationGroupsPublisher describeReplicationGroupsPaginator(
DescribeReplicationGroupsRequest describeReplicationGroupsRequest) {
return new DescribeReplicationGroupsPublisher(this, describeReplicationGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReplicationGroupsPublisher publisher = client.describeReplicationGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReplicationGroups(software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReplicationGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReplicationGroupsRequest#builder()}
*
*
* @param describeReplicationGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReplicationGroupsRequest.Builder} to
* create a request. Represents the input of a DescribeReplicationGroups
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReplicationGroups
* @see AWS API Documentation
*/
default DescribeReplicationGroupsPublisher describeReplicationGroupsPaginator(
Consumer describeReplicationGroupsRequest) {
return describeReplicationGroupsPaginator(DescribeReplicationGroupsRequest.builder()
.applyMutation(describeReplicationGroupsRequest).build());
}
/**
*
* Returns information about reserved cache nodes for this account, or about a specified reserved cache node.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
operation.
* @return A Java Future containing the result of the DescribeReservedCacheNodes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodes(
DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about reserved cache nodes for this account, or about a specified reserved cache node.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedCacheNodesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReservedCacheNodesRequest#builder()}
*
*
* @param describeReservedCacheNodesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest.Builder} to
* create a request. Represents the input of a DescribeReservedCacheNodes
operation.
* @return A Java Future containing the result of the DescribeReservedCacheNodes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodes(
Consumer describeReservedCacheNodesRequest) {
return describeReservedCacheNodes(DescribeReservedCacheNodesRequest.builder()
.applyMutation(describeReservedCacheNodesRequest).build());
}
/**
*
* Returns information about reserved cache nodes for this account, or about a specified reserved cache node.
*
*
* @return A Java Future containing the result of the DescribeReservedCacheNodes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodes() {
return describeReservedCacheNodes(DescribeReservedCacheNodesRequest.builder().build());
}
/**
*
* Lists available reserved cache node offerings.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return A Java Future containing the result of the DescribeReservedCacheNodesOfferings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodesOfferings(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists available reserved cache node offerings.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedCacheNodesOfferingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeReservedCacheNodesOfferingsRequest#builder()}
*
*
* @param describeReservedCacheNodesOfferingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest.Builder}
* to create a request. Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return A Java Future containing the result of the DescribeReservedCacheNodesOfferings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodesOfferings(
Consumer describeReservedCacheNodesOfferingsRequest) {
return describeReservedCacheNodesOfferings(DescribeReservedCacheNodesOfferingsRequest.builder()
.applyMutation(describeReservedCacheNodesOfferingsRequest).build());
}
/**
*
* Lists available reserved cache node offerings.
*
*
* @return A Java Future containing the result of the DescribeReservedCacheNodesOfferings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default CompletableFuture describeReservedCacheNodesOfferings() {
return describeReservedCacheNodesOfferings(DescribeReservedCacheNodesOfferingsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesOfferingsPublisher describeReservedCacheNodesOfferingsPaginator() {
return describeReservedCacheNodesOfferingsPaginator(DescribeReservedCacheNodesOfferingsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation.
*
*
* @param describeReservedCacheNodesOfferingsRequest
* Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesOfferingsPublisher describeReservedCacheNodesOfferingsPaginator(
DescribeReservedCacheNodesOfferingsRequest describeReservedCacheNodesOfferingsRequest) {
return new DescribeReservedCacheNodesOfferingsPublisher(this, describeReservedCacheNodesOfferingsRequest);
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesOfferingsPublisher publisher = client.describeReservedCacheNodesOfferingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodesOfferings(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedCacheNodesOfferingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeReservedCacheNodesOfferingsRequest#builder()}
*
*
* @param describeReservedCacheNodesOfferingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest.Builder}
* to create a request. Represents the input of a DescribeReservedCacheNodesOfferings
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodesOfferings
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesOfferingsPublisher describeReservedCacheNodesOfferingsPaginator(
Consumer describeReservedCacheNodesOfferingsRequest) {
return describeReservedCacheNodesOfferingsPaginator(DescribeReservedCacheNodesOfferingsRequest.builder()
.applyMutation(describeReservedCacheNodesOfferingsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesPublisher describeReservedCacheNodesPaginator() {
return describeReservedCacheNodesPaginator(DescribeReservedCacheNodesRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation.
*
*
* @param describeReservedCacheNodesRequest
* Represents the input of a DescribeReservedCacheNodes
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesPublisher describeReservedCacheNodesPaginator(
DescribeReservedCacheNodesRequest describeReservedCacheNodesRequest) {
return new DescribeReservedCacheNodesPublisher(this, describeReservedCacheNodesRequest);
}
/**
*
* This is a variant of
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeReservedCacheNodesPublisher publisher = client.describeReservedCacheNodesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedCacheNodes(software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedCacheNodesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReservedCacheNodesRequest#builder()}
*
*
* @param describeReservedCacheNodesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesRequest.Builder} to
* create a request. Represents the input of a DescribeReservedCacheNodes
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeReservedCacheNodes
* @see AWS API Documentation
*/
default DescribeReservedCacheNodesPublisher describeReservedCacheNodesPaginator(
Consumer describeReservedCacheNodesRequest) {
return describeReservedCacheNodesPaginator(DescribeReservedCacheNodesRequest.builder()
.applyMutation(describeReservedCacheNodesRequest).build());
}
/**
*
* Returns information about serverless cache snapshots. By default, this API lists all of the customer’s serverless
* cache snapshots. It can also describe a single serverless cache snapshot, or the snapshots associated with a
* particular serverless cache. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
* @param describeServerlessCacheSnapshotsRequest
* @return A Java Future containing the result of the DescribeServerlessCacheSnapshots operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
default CompletableFuture describeServerlessCacheSnapshots(
DescribeServerlessCacheSnapshotsRequest describeServerlessCacheSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about serverless cache snapshots. By default, this API lists all of the customer’s serverless
* cache snapshots. It can also describe a single serverless cache snapshot, or the snapshots associated with a
* particular serverless cache. Available for Valkey, Redis OSS and Serverless Memcached only.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerlessCacheSnapshotsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeServerlessCacheSnapshotsRequest#builder()}
*
*
* @param describeServerlessCacheSnapshotsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeServerlessCacheSnapshots operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
default CompletableFuture describeServerlessCacheSnapshots(
Consumer describeServerlessCacheSnapshotsRequest) {
return describeServerlessCacheSnapshots(DescribeServerlessCacheSnapshotsRequest.builder()
.applyMutation(describeServerlessCacheSnapshotsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeServerlessCacheSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCacheSnapshotsPublisher publisher = client.describeServerlessCacheSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCacheSnapshotsPublisher publisher = client.describeServerlessCacheSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServerlessCacheSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest)}
* operation.
*
*
* @param describeServerlessCacheSnapshotsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
default DescribeServerlessCacheSnapshotsPublisher describeServerlessCacheSnapshotsPaginator(
DescribeServerlessCacheSnapshotsRequest describeServerlessCacheSnapshotsRequest) {
return new DescribeServerlessCacheSnapshotsPublisher(this, describeServerlessCacheSnapshotsRequest);
}
/**
*
* This is a variant of
* {@link #describeServerlessCacheSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCacheSnapshotsPublisher publisher = client.describeServerlessCacheSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCacheSnapshotsPublisher publisher = client.describeServerlessCacheSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServerlessCacheSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerlessCacheSnapshotsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeServerlessCacheSnapshotsRequest#builder()}
*
*
* @param describeServerlessCacheSnapshotsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServerlessCacheSnapshotsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCacheSnapshots
* @see AWS API Documentation
*/
default DescribeServerlessCacheSnapshotsPublisher describeServerlessCacheSnapshotsPaginator(
Consumer describeServerlessCacheSnapshotsRequest) {
return describeServerlessCacheSnapshotsPaginator(DescribeServerlessCacheSnapshotsRequest.builder()
.applyMutation(describeServerlessCacheSnapshotsRequest).build());
}
/**
*
* Returns information about a specific serverless cache. If no identifier is specified, then the API returns
* information on all the serverless caches belonging to this Amazon Web Services account.
*
*
* @param describeServerlessCachesRequest
* @return A Java Future containing the result of the DescribeServerlessCaches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCaches
* @see AWS API Documentation
*/
default CompletableFuture describeServerlessCaches(
DescribeServerlessCachesRequest describeServerlessCachesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specific serverless cache. If no identifier is specified, then the API returns
* information on all the serverless caches belonging to this Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerlessCachesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeServerlessCachesRequest#builder()}
*
*
* @param describeServerlessCachesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeServerlessCaches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCaches
* @see AWS API Documentation
*/
default CompletableFuture describeServerlessCaches(
Consumer describeServerlessCachesRequest) {
return describeServerlessCaches(DescribeServerlessCachesRequest.builder().applyMutation(describeServerlessCachesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #describeServerlessCaches(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCachesPublisher publisher = client.describeServerlessCachesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCachesPublisher publisher = client.describeServerlessCachesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServerlessCaches(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest)}
* operation.
*
*
* @param describeServerlessCachesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCaches
* @see AWS API Documentation
*/
default DescribeServerlessCachesPublisher describeServerlessCachesPaginator(
DescribeServerlessCachesRequest describeServerlessCachesRequest) {
return new DescribeServerlessCachesPublisher(this, describeServerlessCachesRequest);
}
/**
*
* This is a variant of
* {@link #describeServerlessCaches(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCachesPublisher publisher = client.describeServerlessCachesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServerlessCachesPublisher publisher = client.describeServerlessCachesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServerlessCaches(software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServerlessCachesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeServerlessCachesRequest#builder()}
*
*
* @param describeServerlessCachesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServerlessCachesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServerlessCaches
* @see AWS API Documentation
*/
default DescribeServerlessCachesPublisher describeServerlessCachesPaginator(
Consumer describeServerlessCachesRequest) {
return describeServerlessCachesPaginator(DescribeServerlessCachesRequest.builder()
.applyMutation(describeServerlessCachesRequest).build());
}
/**
*
* Returns details of the service updates
*
*
* @param describeServiceUpdatesRequest
* @return A Java Future containing the result of the DescribeServiceUpdates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServiceUpdates
* @see AWS API Documentation
*/
default CompletableFuture describeServiceUpdates(
DescribeServiceUpdatesRequest describeServiceUpdatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns details of the service updates
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServiceUpdatesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeServiceUpdatesRequest#builder()}
*
*
* @param describeServiceUpdatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeServiceUpdates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServiceUpdates
* @see AWS API Documentation
*/
default CompletableFuture describeServiceUpdates(
Consumer describeServiceUpdatesRequest) {
return describeServiceUpdates(DescribeServiceUpdatesRequest.builder().applyMutation(describeServiceUpdatesRequest)
.build());
}
/**
*
* This is a variant of
* {@link #describeServiceUpdates(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServiceUpdatesPublisher publisher = client.describeServiceUpdatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServiceUpdatesPublisher publisher = client.describeServiceUpdatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServiceUpdates(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest)}
* operation.
*
*
* @param describeServiceUpdatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServiceUpdates
* @see AWS API Documentation
*/
default DescribeServiceUpdatesPublisher describeServiceUpdatesPaginator(
DescribeServiceUpdatesRequest describeServiceUpdatesRequest) {
return new DescribeServiceUpdatesPublisher(this, describeServiceUpdatesRequest);
}
/**
*
* This is a variant of
* {@link #describeServiceUpdates(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServiceUpdatesPublisher publisher = client.describeServiceUpdatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeServiceUpdatesPublisher publisher = client.describeServiceUpdatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeServiceUpdates(software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServiceUpdatesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeServiceUpdatesRequest#builder()}
*
*
* @param describeServiceUpdatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeServiceUpdatesRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceUpdateNotFoundException The service update doesn't exist
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeServiceUpdates
* @see AWS API Documentation
*/
default DescribeServiceUpdatesPublisher describeServiceUpdatesPaginator(
Consumer describeServiceUpdatesRequest) {
return describeServiceUpdatesPaginator(DescribeServiceUpdatesRequest.builder()
.applyMutation(describeServiceUpdatesRequest).build());
}
/**
*
* Returns information about cluster or replication group snapshots. By default, DescribeSnapshots
* lists all of your snapshots; it can optionally describe a single snapshot, or just the snapshots associated with
* a particular cache cluster.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage
operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about cluster or replication group snapshots. By default, DescribeSnapshots
* lists all of your snapshots; it can optionally describe a single snapshot, or just the snapshots associated with
* a particular cache cluster.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSnapshotsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeSnapshotsRequest#builder()}
*
*
* @param describeSnapshotsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest.Builder} to create a
* request. Represents the input of a DescribeSnapshotsMessage
operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeSnapshots(
Consumer describeSnapshotsRequest) {
return describeSnapshots(DescribeSnapshotsRequest.builder().applyMutation(describeSnapshotsRequest).build());
}
/**
*
* Returns information about cluster or replication group snapshots. By default, DescribeSnapshots
* lists all of your snapshots; it can optionally describe a single snapshot, or just the snapshots associated with
* a particular cache cluster.
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeSnapshots() {
return describeSnapshots(DescribeSnapshotsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default DescribeSnapshotsPublisher describeSnapshotsPaginator() {
return describeSnapshotsPaginator(DescribeSnapshotsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)}
* operation.
*
*
* @param describeSnapshotsRequest
* Represents the input of a DescribeSnapshotsMessage
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default DescribeSnapshotsPublisher describeSnapshotsPaginator(DescribeSnapshotsRequest describeSnapshotsRequest) {
return new DescribeSnapshotsPublisher(this, describeSnapshotsRequest);
}
/**
*
* This is a variant of
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeSnapshotsPublisher publisher = client.describeSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeSnapshots(software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSnapshotsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeSnapshotsRequest#builder()}
*
*
* @param describeSnapshotsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeSnapshotsRequest.Builder} to create a
* request. Represents the input of a DescribeSnapshotsMessage
operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeSnapshots
* @see AWS
* API Documentation
*/
default DescribeSnapshotsPublisher describeSnapshotsPaginator(
Consumer describeSnapshotsRequest) {
return describeSnapshotsPaginator(DescribeSnapshotsRequest.builder().applyMutation(describeSnapshotsRequest).build());
}
/**
*
* Returns details of the update actions
*
*
* @param describeUpdateActionsRequest
* @return A Java Future containing the result of the DescribeUpdateActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUpdateActions
* @see AWS API Documentation
*/
default CompletableFuture describeUpdateActions(
DescribeUpdateActionsRequest describeUpdateActionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns details of the update actions
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUpdateActionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUpdateActionsRequest#builder()}
*
*
* @param describeUpdateActionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeUpdateActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUpdateActions
* @see AWS API Documentation
*/
default CompletableFuture describeUpdateActions(
Consumer describeUpdateActionsRequest) {
return describeUpdateActions(DescribeUpdateActionsRequest.builder().applyMutation(describeUpdateActionsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeUpdateActions(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUpdateActionsPublisher publisher = client.describeUpdateActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUpdateActionsPublisher publisher = client.describeUpdateActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUpdateActions(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest)}
* operation.
*
*
* @param describeUpdateActionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUpdateActions
* @see AWS API Documentation
*/
default DescribeUpdateActionsPublisher describeUpdateActionsPaginator(
DescribeUpdateActionsRequest describeUpdateActionsRequest) {
return new DescribeUpdateActionsPublisher(this, describeUpdateActionsRequest);
}
/**
*
* This is a variant of
* {@link #describeUpdateActions(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUpdateActionsPublisher publisher = client.describeUpdateActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUpdateActionsPublisher publisher = client.describeUpdateActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUpdateActions(software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUpdateActionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUpdateActionsRequest#builder()}
*
*
* @param describeUpdateActionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUpdateActionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUpdateActions
* @see AWS API Documentation
*/
default DescribeUpdateActionsPublisher describeUpdateActionsPaginator(
Consumer describeUpdateActionsRequest) {
return describeUpdateActionsPaginator(DescribeUpdateActionsRequest.builder().applyMutation(describeUpdateActionsRequest)
.build());
}
/**
*
* Returns a list of user groups.
*
*
* @param describeUserGroupsRequest
* @return A Java Future containing the result of the DescribeUserGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUserGroups
* @see AWS API Documentation
*/
default CompletableFuture describeUserGroups(DescribeUserGroupsRequest describeUserGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of user groups.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUserGroupsRequest#builder()}
*
*
* @param describeUserGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeUserGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUserGroups
* @see AWS API Documentation
*/
default CompletableFuture describeUserGroups(
Consumer describeUserGroupsRequest) {
return describeUserGroups(DescribeUserGroupsRequest.builder().applyMutation(describeUserGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeUserGroups(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUserGroupsPublisher publisher = client.describeUserGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUserGroupsPublisher publisher = client.describeUserGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUserGroups(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest)}
* operation.
*
*
* @param describeUserGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUserGroups
* @see AWS API Documentation
*/
default DescribeUserGroupsPublisher describeUserGroupsPaginator(DescribeUserGroupsRequest describeUserGroupsRequest) {
return new DescribeUserGroupsPublisher(this, describeUserGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeUserGroups(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUserGroupsPublisher publisher = client.describeUserGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUserGroupsPublisher publisher = client.describeUserGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUserGroups(software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUserGroupsRequest#builder()}
*
*
* @param describeUserGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUserGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUserGroups
* @see AWS API Documentation
*/
default DescribeUserGroupsPublisher describeUserGroupsPaginator(
Consumer describeUserGroupsRequest) {
return describeUserGroupsPaginator(DescribeUserGroupsRequest.builder().applyMutation(describeUserGroupsRequest).build());
}
/**
*
* Returns a list of users.
*
*
* @param describeUsersRequest
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(DescribeUsersRequest describeUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of users.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(Consumer describeUsersRequest) {
return describeUsers(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* This is a variant of
* {@link #describeUsers(software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest)} operation.
*
*
* @param describeUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersPublisher describeUsersPaginator(DescribeUsersRequest describeUsersRequest) {
return new DescribeUsersPublisher(this, describeUsersRequest);
}
/**
*
* This is a variant of
* {@link #describeUsers(software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticache.paginators.DescribeUsersPublisher publisher = client.describeUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticache.model.DescribeUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeUsers(software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DescribeUsersRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default DescribeUsersPublisher describeUsersPaginator(Consumer describeUsersRequest) {
return describeUsersPaginator(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Remove a secondary cluster from the Global datastore using the Global datastore name. The secondary cluster will
* no longer receive updates from the primary cluster, but will remain as a standalone cluster in that Amazon
* region.
*
*
* @param disassociateGlobalReplicationGroupRequest
* @return A Java Future containing the result of the DisassociateGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DisassociateGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateGlobalReplicationGroup(
DisassociateGlobalReplicationGroupRequest disassociateGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Remove a secondary cluster from the Global datastore using the Global datastore name. The secondary cluster will
* no longer receive updates from the primary cluster, but will remain as a standalone cluster in that Amazon
* region.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateGlobalReplicationGroupRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateGlobalReplicationGroupRequest#builder()}
*
*
* @param disassociateGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.DisassociateGlobalReplicationGroupRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DisassociateGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.DisassociateGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateGlobalReplicationGroup(
Consumer disassociateGlobalReplicationGroupRequest) {
return disassociateGlobalReplicationGroup(DisassociateGlobalReplicationGroupRequest.builder()
.applyMutation(disassociateGlobalReplicationGroupRequest).build());
}
/**
*
* Provides the functionality to export the serverless cache snapshot data to Amazon S3. Available for Valkey and
* Redis OSS only.
*
*
* @param exportServerlessCacheSnapshotRequest
* @return A Java Future containing the result of the ExportServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ExportServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture exportServerlessCacheSnapshot(
ExportServerlessCacheSnapshotRequest exportServerlessCacheSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides the functionality to export the serverless cache snapshot data to Amazon S3. Available for Valkey and
* Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link ExportServerlessCacheSnapshotRequest.Builder}
* avoiding the need to create one manually via {@link ExportServerlessCacheSnapshotRequest#builder()}
*
*
* @param exportServerlessCacheSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ExportServerlessCacheSnapshotRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ExportServerlessCacheSnapshot operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ExportServerlessCacheSnapshot
* @see AWS API Documentation
*/
default CompletableFuture exportServerlessCacheSnapshot(
Consumer exportServerlessCacheSnapshotRequest) {
return exportServerlessCacheSnapshot(ExportServerlessCacheSnapshotRequest.builder()
.applyMutation(exportServerlessCacheSnapshotRequest).build());
}
/**
*
* Used to failover the primary region to a secondary region. The secondary region will become primary, and all
* other clusters will become secondary.
*
*
* @param failoverGlobalReplicationGroupRequest
* @return A Java Future containing the result of the FailoverGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.FailoverGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture failoverGlobalReplicationGroup(
FailoverGlobalReplicationGroupRequest failoverGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Used to failover the primary region to a secondary region. The secondary region will become primary, and all
* other clusters will become secondary.
*
*
*
* This is a convenience which creates an instance of the {@link FailoverGlobalReplicationGroupRequest.Builder}
* avoiding the need to create one manually via {@link FailoverGlobalReplicationGroupRequest#builder()}
*
*
* @param failoverGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.FailoverGlobalReplicationGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the FailoverGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.FailoverGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture failoverGlobalReplicationGroup(
Consumer failoverGlobalReplicationGroupRequest) {
return failoverGlobalReplicationGroup(FailoverGlobalReplicationGroupRequest.builder()
.applyMutation(failoverGlobalReplicationGroupRequest).build());
}
/**
*
* Increase the number of node groups in the Global datastore
*
*
* @param increaseNodeGroupsInGlobalReplicationGroupRequest
* @return A Java Future containing the result of the IncreaseNodeGroupsInGlobalReplicationGroup operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.IncreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture increaseNodeGroupsInGlobalReplicationGroup(
IncreaseNodeGroupsInGlobalReplicationGroupRequest increaseNodeGroupsInGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Increase the number of node groups in the Global datastore
*
*
*
* This is a convenience which creates an instance of the
* {@link IncreaseNodeGroupsInGlobalReplicationGroupRequest.Builder} avoiding the need to create one manually via
* {@link IncreaseNodeGroupsInGlobalReplicationGroupRequest#builder()}
*
*
* @param increaseNodeGroupsInGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.IncreaseNodeGroupsInGlobalReplicationGroupRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the IncreaseNodeGroupsInGlobalReplicationGroup operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.IncreaseNodeGroupsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture increaseNodeGroupsInGlobalReplicationGroup(
Consumer increaseNodeGroupsInGlobalReplicationGroupRequest) {
return increaseNodeGroupsInGlobalReplicationGroup(IncreaseNodeGroupsInGlobalReplicationGroupRequest.builder()
.applyMutation(increaseNodeGroupsInGlobalReplicationGroupRequest).build());
}
/**
*
* Dynamically increases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
* @param increaseReplicaCountRequest
* @return A Java Future containing the result of the IncreaseReplicaCount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - NoOperationException The operation was not performed because no changes were required.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.IncreaseReplicaCount
* @see AWS API Documentation
*/
default CompletableFuture increaseReplicaCount(
IncreaseReplicaCountRequest increaseReplicaCountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Dynamically increases the number of replicas in a Valkey or Redis OSS (cluster mode disabled) replication group
* or the number of replica nodes in one or more node groups (shards) of a Valkey or Redis OSS (cluster mode
* enabled) replication group. This operation is performed with no cluster down time.
*
*
*
* This is a convenience which creates an instance of the {@link IncreaseReplicaCountRequest.Builder} avoiding the
* need to create one manually via {@link IncreaseReplicaCountRequest#builder()}
*
*
* @param increaseReplicaCountRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.IncreaseReplicaCountRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the IncreaseReplicaCount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - ClusterQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of clusters per customer.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - NoOperationException The operation was not performed because no changes were required.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.IncreaseReplicaCount
* @see AWS API Documentation
*/
default CompletableFuture increaseReplicaCount(
Consumer increaseReplicaCountRequest) {
return increaseReplicaCount(IncreaseReplicaCountRequest.builder().applyMutation(increaseReplicaCountRequest).build());
}
/**
*
* Lists all available node types that you can scale with your cluster's replication group's current node type.
*
*
* When you use the ModifyCacheCluster
or ModifyReplicationGroup
operations to scale your
* cluster or replication group, the value of the CacheNodeType
parameter must be one of the node types
* returned by this operation.
*
*
* @param listAllowedNodeTypeModificationsRequest
* The input parameters for the ListAllowedNodeTypeModifications
operation.
* @return A Java Future containing the result of the ListAllowedNodeTypeModifications operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ListAllowedNodeTypeModifications
* @see AWS API Documentation
*/
default CompletableFuture listAllowedNodeTypeModifications(
ListAllowedNodeTypeModificationsRequest listAllowedNodeTypeModificationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available node types that you can scale with your cluster's replication group's current node type.
*
*
* When you use the ModifyCacheCluster
or ModifyReplicationGroup
operations to scale your
* cluster or replication group, the value of the CacheNodeType
parameter must be one of the node types
* returned by this operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAllowedNodeTypeModificationsRequest.Builder}
* avoiding the need to create one manually via {@link ListAllowedNodeTypeModificationsRequest#builder()}
*
*
* @param listAllowedNodeTypeModificationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ListAllowedNodeTypeModificationsRequest.Builder}
* to create a request. The input parameters for the ListAllowedNodeTypeModifications
operation.
* @return A Java Future containing the result of the ListAllowedNodeTypeModifications operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ListAllowedNodeTypeModifications
* @see AWS API Documentation
*/
default CompletableFuture listAllowedNodeTypeModifications(
Consumer listAllowedNodeTypeModificationsRequest) {
return listAllowedNodeTypeModifications(ListAllowedNodeTypeModificationsRequest.builder()
.applyMutation(listAllowedNodeTypeModificationsRequest).build());
}
/**
*
* Lists all tags currently on a named resource.
*
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* If the cluster is not in the available state, ListTagsForResource
returns an error.
*
*
* @param listTagsForResourceRequest
* The input parameters for the ListTagsForResource
operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags currently on a named resource.
*
*
* A tag is a key-value pair where the key and value are case-sensitive. You can use tags to categorize and track
* all your ElastiCache resources, with the exception of global replication group. When you add or remove tags on
* replication groups, those actions will be replicated to all nodes in the replication group. For more information,
* see Resource-level
* permissions.
*
*
* If the cluster is not in the available state, ListTagsForResource
returns an error.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ListTagsForResourceRequest.Builder} to create a
* request. The input parameters for the ListTagsForResource
operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* parameters by specifying the parameters and the new values.
*
*
* @param modifyCacheClusterRequest
* Represents the input of a ModifyCacheCluster
operation.
* @return A Java Future containing the result of the ModifyCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheCluster(ModifyCacheClusterRequest modifyCacheClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the settings for a cluster. You can use this operation to change one or more cluster configuration
* parameters by specifying the parameters and the new values.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyCacheClusterRequest.Builder} avoiding the
* need to create one manually via {@link ModifyCacheClusterRequest#builder()}
*
*
* @param modifyCacheClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyCacheClusterRequest.Builder} to create a
* request. Represents the input of a ModifyCacheCluster
operation.
* @return A Java Future containing the result of the ModifyCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheCluster(
Consumer modifyCacheClusterRequest) {
return modifyCacheCluster(ModifyCacheClusterRequest.builder().applyMutation(modifyCacheClusterRequest).build());
}
/**
*
* Modifies the parameters of a cache parameter group. You can modify up to 20 parameters in a single request by
* submitting a list parameter name and value pairs.
*
*
* @param modifyCacheParameterGroupRequest
* Represents the input of a ModifyCacheParameterGroup
operation.
* @return A Java Future containing the result of the ModifyCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheParameterGroup(
ModifyCacheParameterGroupRequest modifyCacheParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the parameters of a cache parameter group. You can modify up to 20 parameters in a single request by
* submitting a list parameter name and value pairs.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyCacheParameterGroupRequest.Builder} avoiding
* the need to create one manually via {@link ModifyCacheParameterGroupRequest#builder()}
*
*
* @param modifyCacheParameterGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyCacheParameterGroupRequest.Builder} to
* create a request. Represents the input of a ModifyCacheParameterGroup
operation.
* @return A Java Future containing the result of the ModifyCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheParameterGroup(
Consumer modifyCacheParameterGroupRequest) {
return modifyCacheParameterGroup(ModifyCacheParameterGroupRequest.builder()
.applyMutation(modifyCacheParameterGroupRequest).build());
}
/**
*
* Modifies an existing cache subnet group.
*
*
* @param modifyCacheSubnetGroupRequest
* Represents the input of a ModifyCacheSubnetGroup
operation.
* @return A Java Future containing the result of the ModifyCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - CacheSubnetQuotaExceededException The request cannot be processed because it would exceed the allowed
* number of subnets in a cache subnet group.
* - SubnetInUseException The requested subnet is being used by another cache subnet group.
* - InvalidSubnetException An invalid subnet identifier was specified.
* - SubnetNotAllowedException At least one subnet ID does not match the other subnet IDs. This mismatch
* typically occurs when a user sets one subnet ID to a regional Availability Zone and a different one to an
* outpost. Or when a user sets the subnet ID to an Outpost when not subscribed on this service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheSubnetGroup(
ModifyCacheSubnetGroupRequest modifyCacheSubnetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies an existing cache subnet group.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyCacheSubnetGroupRequest.Builder} avoiding the
* need to create one manually via {@link ModifyCacheSubnetGroupRequest#builder()}
*
*
* @param modifyCacheSubnetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyCacheSubnetGroupRequest.Builder} to create
* a request. Represents the input of a ModifyCacheSubnetGroup
operation.
* @return A Java Future containing the result of the ModifyCacheSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - CacheSubnetQuotaExceededException The request cannot be processed because it would exceed the allowed
* number of subnets in a cache subnet group.
* - SubnetInUseException The requested subnet is being used by another cache subnet group.
* - InvalidSubnetException An invalid subnet identifier was specified.
* - SubnetNotAllowedException At least one subnet ID does not match the other subnet IDs. This mismatch
* typically occurs when a user sets one subnet ID to a regional Availability Zone and a different one to an
* outpost. Or when a user sets the subnet ID to an Outpost when not subscribed on this service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyCacheSubnetGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyCacheSubnetGroup(
Consumer modifyCacheSubnetGroupRequest) {
return modifyCacheSubnetGroup(ModifyCacheSubnetGroupRequest.builder().applyMutation(modifyCacheSubnetGroupRequest)
.build());
}
/**
*
* Modifies the settings for a Global datastore.
*
*
* @param modifyGlobalReplicationGroupRequest
* @return A Java Future containing the result of the ModifyGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyGlobalReplicationGroup(
ModifyGlobalReplicationGroupRequest modifyGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the settings for a Global datastore.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyGlobalReplicationGroupRequest.Builder}
* avoiding the need to create one manually via {@link ModifyGlobalReplicationGroupRequest#builder()}
*
*
* @param modifyGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyGlobalReplicationGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ModifyGlobalReplicationGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyGlobalReplicationGroup(
Consumer modifyGlobalReplicationGroupRequest) {
return modifyGlobalReplicationGroup(ModifyGlobalReplicationGroupRequest.builder()
.applyMutation(modifyGlobalReplicationGroupRequest).build());
}
/**
*
* Modifies the settings for a replication group. This is limited to Valkey and Redis OSS 7 and above.
*
*
* -
*
* Scaling
* for Valkey or Redis OSS (cluster mode enabled) in the ElastiCache User Guide
*
*
* -
*
* ModifyReplicationGroupShardConfiguration in the ElastiCache API Reference
*
*
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* @param modifyReplicationGroupRequest
* Represents the input of a ModifyReplicationGroups
operation.
* @return A Java Future containing the result of the ModifyReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyReplicationGroup(
ModifyReplicationGroupRequest modifyReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the settings for a replication group. This is limited to Valkey and Redis OSS 7 and above.
*
*
* -
*
* Scaling
* for Valkey or Redis OSS (cluster mode enabled) in the ElastiCache User Guide
*
*
* -
*
* ModifyReplicationGroupShardConfiguration in the ElastiCache API Reference
*
*
*
*
*
* This operation is valid for Valkey or Redis OSS only.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyReplicationGroupRequest.Builder} avoiding the
* need to create one manually via {@link ModifyReplicationGroupRequest#builder()}
*
*
* @param modifyReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupRequest.Builder} to create
* a request. Represents the input of a ModifyReplicationGroups
operation.
* @return A Java Future containing the result of the ModifyReplicationGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - NodeQuotaForClusterExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes in a single cluster.
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyReplicationGroup(
Consumer modifyReplicationGroupRequest) {
return modifyReplicationGroup(ModifyReplicationGroupRequest.builder().applyMutation(modifyReplicationGroupRequest)
.build());
}
/**
*
* Modifies a replication group's shards (node groups) by allowing you to add shards, remove shards, or rebalance
* the keyspaces among existing shards.
*
*
* @param modifyReplicationGroupShardConfigurationRequest
* Represents the input for a ModifyReplicationGroupShardConfiguration
operation.
* @return A Java Future containing the result of the ModifyReplicationGroupShardConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyReplicationGroupShardConfiguration
* @see AWS API Documentation
*/
default CompletableFuture modifyReplicationGroupShardConfiguration(
ModifyReplicationGroupShardConfigurationRequest modifyReplicationGroupShardConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies a replication group's shards (node groups) by allowing you to add shards, remove shards, or rebalance
* the keyspaces among existing shards.
*
*
*
* This is a convenience which creates an instance of the
* {@link ModifyReplicationGroupShardConfigurationRequest.Builder} avoiding the need to create one manually via
* {@link ModifyReplicationGroupShardConfigurationRequest#builder()}
*
*
* @param modifyReplicationGroupShardConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyReplicationGroupShardConfigurationRequest.Builder}
* to create a request. Represents the input for a ModifyReplicationGroupShardConfiguration
* operation.
* @return A Java Future containing the result of the ModifyReplicationGroupShardConfiguration operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - InvalidVpcNetworkStateException The VPC network is in an invalid state.
* - InsufficientCacheClusterCapacityException The requested cache node type is not available in the
* specified Availability Zone. For more information, see InsufficientCacheClusterCapacity in the ElastiCache User Guide.
* - NodeGroupsPerReplicationGroupQuotaExceededException The request cannot be processed because it would
* exceed the maximum allowed number of node groups (shards) in a single replication group. The default
* maximum is 90
* - NodeQuotaForCustomerExceededException The request cannot be processed because it would exceed the
* allowed number of cache nodes per customer.
* - InvalidKmsKeyException The KMS key supplied is not valid.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyReplicationGroupShardConfiguration
* @see AWS API Documentation
*/
default CompletableFuture modifyReplicationGroupShardConfiguration(
Consumer modifyReplicationGroupShardConfigurationRequest) {
return modifyReplicationGroupShardConfiguration(ModifyReplicationGroupShardConfigurationRequest.builder()
.applyMutation(modifyReplicationGroupShardConfigurationRequest).build());
}
/**
*
* This API modifies the attributes of a serverless cache.
*
*
* @param modifyServerlessCacheRequest
* @return A Java Future containing the result of the ModifyServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture modifyServerlessCache(
ModifyServerlessCacheRequest modifyServerlessCacheRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API modifies the attributes of a serverless cache.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyServerlessCacheRequest.Builder} avoiding the
* need to create one manually via {@link ModifyServerlessCacheRequest#builder()}
*
*
* @param modifyServerlessCacheRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyServerlessCacheRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ModifyServerlessCache operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidCredentialsException You must enter valid credentials.
* - InvalidUserGroupStateException The user group is not in an active state.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyServerlessCache
* @see AWS API Documentation
*/
default CompletableFuture modifyServerlessCache(
Consumer modifyServerlessCacheRequest) {
return modifyServerlessCache(ModifyServerlessCacheRequest.builder().applyMutation(modifyServerlessCacheRequest).build());
}
/**
*
* Changes user password(s) and/or access string.
*
*
* @param modifyUserRequest
* @return A Java Future containing the result of the ModifyUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - InvalidUserStateException The user is not in active state.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyUser
* @see AWS API
* Documentation
*/
default CompletableFuture modifyUser(ModifyUserRequest modifyUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes user password(s) and/or access string.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyUserRequest.Builder} avoiding the need to
* create one manually via {@link ModifyUserRequest#builder()}
*
*
* @param modifyUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the ModifyUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserNotFoundException The user does not exist or could not be found.
* - InvalidUserStateException The user is not in active state.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyUser
* @see AWS API
* Documentation
*/
default CompletableFuture modifyUser(Consumer modifyUserRequest) {
return modifyUser(ModifyUserRequest.builder().applyMutation(modifyUserRequest).build());
}
/**
*
* Changes the list of users that belong to the user group.
*
*
* @param modifyUserGroupRequest
* @return A Java Future containing the result of the ModifyUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - UserNotFoundException The user does not exist or could not be found.
* - DuplicateUserNameException A user with this username already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - DefaultUserRequiredException You must add default user to a user group.
* - InvalidUserGroupStateException The user group is not in an active state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture modifyUserGroup(ModifyUserGroupRequest modifyUserGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Changes the list of users that belong to the user group.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyUserGroupRequest.Builder} avoiding the need
* to create one manually via {@link ModifyUserGroupRequest#builder()}
*
*
* @param modifyUserGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ModifyUserGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ModifyUserGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UserGroupNotFoundException The user group was not found or does not exist
* - UserNotFoundException The user does not exist or could not be found.
* - DuplicateUserNameException A user with this username already exists.
* - ServiceLinkedRoleNotFoundException The specified service linked role (SLR) was not found.
* - DefaultUserRequiredException You must add default user to a user group.
* - InvalidUserGroupStateException The user group is not in an active state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ModifyUserGroup
* @see AWS
* API Documentation
*/
default CompletableFuture modifyUserGroup(
Consumer modifyUserGroupRequest) {
return modifyUserGroup(ModifyUserGroupRequest.builder().applyMutation(modifyUserGroupRequest).build());
}
/**
*
* Allows you to purchase a reserved cache node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable. For more information, see Managing Costs with Reserved
* Nodes.
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* Represents the input of a PurchaseReservedCacheNodesOffering
operation.
* @return A Java Future containing the result of the PurchaseReservedCacheNodesOffering operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - ReservedCacheNodeAlreadyExistsException You already have a reservation with the given identifier.
* - ReservedCacheNodeQuotaExceededException The request cannot be processed because it would exceed the
* user's cache node quota.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.PurchaseReservedCacheNodesOffering
* @see AWS API Documentation
*/
default CompletableFuture purchaseReservedCacheNodesOffering(
PurchaseReservedCacheNodesOfferingRequest purchaseReservedCacheNodesOfferingRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to purchase a reserved cache node offering. Reserved nodes are not eligible for cancellation and are
* non-refundable. For more information, see Managing Costs with Reserved
* Nodes.
*
*
*
* This is a convenience which creates an instance of the {@link PurchaseReservedCacheNodesOfferingRequest.Builder}
* avoiding the need to create one manually via {@link PurchaseReservedCacheNodesOfferingRequest#builder()}
*
*
* @param purchaseReservedCacheNodesOfferingRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.PurchaseReservedCacheNodesOfferingRequest.Builder}
* to create a request. Represents the input of a PurchaseReservedCacheNodesOffering
operation.
* @return A Java Future containing the result of the PurchaseReservedCacheNodesOffering operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedCacheNodesOfferingNotFoundException The requested cache node offering does not exist.
* - ReservedCacheNodeAlreadyExistsException You already have a reservation with the given identifier.
* - ReservedCacheNodeQuotaExceededException The request cannot be processed because it would exceed the
* user's cache node quota.
* - TagQuotaPerResourceExceededException The request cannot be processed because it would cause the
* resource to have more than the allowed number of tags. The maximum number of tags permitted on a resource
* is 50.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.PurchaseReservedCacheNodesOffering
* @see AWS API Documentation
*/
default CompletableFuture purchaseReservedCacheNodesOffering(
Consumer purchaseReservedCacheNodesOfferingRequest) {
return purchaseReservedCacheNodesOffering(PurchaseReservedCacheNodesOfferingRequest.builder()
.applyMutation(purchaseReservedCacheNodesOfferingRequest).build());
}
/**
*
* Redistribute slots to ensure uniform distribution across existing shards in the cluster.
*
*
* @param rebalanceSlotsInGlobalReplicationGroupRequest
* @return A Java Future containing the result of the RebalanceSlotsInGlobalReplicationGroup operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RebalanceSlotsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture rebalanceSlotsInGlobalReplicationGroup(
RebalanceSlotsInGlobalReplicationGroupRequest rebalanceSlotsInGlobalReplicationGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Redistribute slots to ensure uniform distribution across existing shards in the cluster.
*
*
*
* This is a convenience which creates an instance of the
* {@link RebalanceSlotsInGlobalReplicationGroupRequest.Builder} avoiding the need to create one manually via
* {@link RebalanceSlotsInGlobalReplicationGroupRequest#builder()}
*
*
* @param rebalanceSlotsInGlobalReplicationGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.RebalanceSlotsInGlobalReplicationGroupRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the RebalanceSlotsInGlobalReplicationGroup operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GlobalReplicationGroupNotFoundException The Global datastore does not exist
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RebalanceSlotsInGlobalReplicationGroup
* @see AWS API Documentation
*/
default CompletableFuture rebalanceSlotsInGlobalReplicationGroup(
Consumer rebalanceSlotsInGlobalReplicationGroupRequest) {
return rebalanceSlotsInGlobalReplicationGroup(RebalanceSlotsInGlobalReplicationGroupRequest.builder()
.applyMutation(rebalanceSlotsInGlobalReplicationGroupRequest).build());
}
/**
*
* Reboots some, or all, of the cache nodes within a provisioned cluster. This operation applies any modified cache
* parameter groups to the cluster. The reboot operation takes place as soon as possible, and results in a momentary
* outage to the cluster. During the reboot, the cluster status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being rebooted) to be lost.
*
*
* When the reboot is complete, a cluster event is created.
*
*
* Rebooting a cluster is currently supported on Memcached, Valkey and Redis OSS (cluster mode disabled) clusters.
* Rebooting is not supported on Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
* If you make changes to parameters that require a Valkey or Redis OSS (cluster mode enabled) cluster reboot for
* the changes to be applied, see Rebooting a Cluster for an
* alternate process.
*
*
* @param rebootCacheClusterRequest
* Represents the input of a RebootCacheCluster
operation.
* @return A Java Future containing the result of the RebootCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RebootCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture rebootCacheCluster(RebootCacheClusterRequest rebootCacheClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Reboots some, or all, of the cache nodes within a provisioned cluster. This operation applies any modified cache
* parameter groups to the cluster. The reboot operation takes place as soon as possible, and results in a momentary
* outage to the cluster. During the reboot, the cluster status is set to REBOOTING.
*
*
* The reboot causes the contents of the cache (for each cache node being rebooted) to be lost.
*
*
* When the reboot is complete, a cluster event is created.
*
*
* Rebooting a cluster is currently supported on Memcached, Valkey and Redis OSS (cluster mode disabled) clusters.
* Rebooting is not supported on Valkey or Redis OSS (cluster mode enabled) clusters.
*
*
* If you make changes to parameters that require a Valkey or Redis OSS (cluster mode enabled) cluster reboot for
* the changes to be applied, see Rebooting a Cluster for an
* alternate process.
*
*
*
* This is a convenience which creates an instance of the {@link RebootCacheClusterRequest.Builder} avoiding the
* need to create one manually via {@link RebootCacheClusterRequest#builder()}
*
*
* @param rebootCacheClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.RebootCacheClusterRequest.Builder} to create a
* request. Represents the input of a RebootCacheCluster
operation.
* @return A Java Future containing the result of the RebootCacheCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheClusterStateException The requested cluster is not in the
available
state.
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RebootCacheCluster
* @see AWS API Documentation
*/
default CompletableFuture rebootCacheCluster(
Consumer rebootCacheClusterRequest) {
return rebootCacheCluster(RebootCacheClusterRequest.builder().applyMutation(rebootCacheClusterRequest).build());
}
/**
*
* Removes the tags identified by the TagKeys
list from the named resource. A tag is a key-value pair
* where the key and value are case-sensitive. You can use tags to categorize and track all your ElastiCache
* resources, with the exception of global replication group. When you add or remove tags on replication groups,
* those actions will be replicated to all nodes in the replication group. For more information, see Resource-level
* permissions.
*
*
* @param removeTagsFromResourceRequest
* Represents the input of a RemoveTagsFromResource
operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - TagNotFoundException The requested tag was not found on this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RemoveTagsFromResource
* @see AWS API Documentation
*/
default CompletableFuture removeTagsFromResource(
RemoveTagsFromResourceRequest removeTagsFromResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the tags identified by the TagKeys
list from the named resource. A tag is a key-value pair
* where the key and value are case-sensitive. You can use tags to categorize and track all your ElastiCache
* resources, with the exception of global replication group. When you add or remove tags on replication groups,
* those actions will be replicated to all nodes in the replication group. For more information, see Resource-level
* permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveTagsFromResourceRequest.Builder} avoiding the
* need to create one manually via {@link RemoveTagsFromResourceRequest#builder()}
*
*
* @param removeTagsFromResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.RemoveTagsFromResourceRequest.Builder} to create
* a request. Represents the input of a RemoveTagsFromResource
operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheClusterNotFoundException The requested cluster ID does not refer to an existing cluster.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - CacheSubnetGroupNotFoundException The requested cache subnet group name does not refer to an existing
* cache subnet group.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - ReservedCacheNodeNotFoundException The requested reserved cache node was not found.
* - SnapshotNotFoundException The requested snapshot name does not refer to an existing snapshot.
* - UserNotFoundException The user does not exist or could not be found.
* - UserGroupNotFoundException The user group was not found or does not exist
* - ServerlessCacheNotFoundException The serverless cache was not found or does not exist.
* - InvalidServerlessCacheStateException The account for these credentials is not currently active.
* - ServerlessCacheSnapshotNotFoundException This serverless cache snapshot could not be found or does
* not exist. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidServerlessCacheSnapshotStateException The state of the serverless cache snapshot was not
* received. Available for Valkey, Redis OSS and Serverless Memcached only.
* - InvalidArnException The requested Amazon Resource Name (ARN) does not refer to an existing resource.
* - TagNotFoundException The requested tag was not found on this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RemoveTagsFromResource
* @see AWS API Documentation
*/
default CompletableFuture removeTagsFromResource(
Consumer removeTagsFromResourceRequest) {
return removeTagsFromResource(RemoveTagsFromResourceRequest.builder().applyMutation(removeTagsFromResourceRequest)
.build());
}
/**
*
* Modifies the parameters of a cache parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire cache parameter group, specify the
* ResetAllParameters
and CacheParameterGroupName
parameters.
*
*
* @param resetCacheParameterGroupRequest
* Represents the input of a ResetCacheParameterGroup
operation.
* @return A Java Future containing the result of the ResetCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ResetCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture resetCacheParameterGroup(
ResetCacheParameterGroupRequest resetCacheParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the parameters of a cache parameter group to the engine or system default value. You can reset specific
* parameters by submitting a list of parameter names. To reset the entire cache parameter group, specify the
* ResetAllParameters
and CacheParameterGroupName
parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ResetCacheParameterGroupRequest.Builder} avoiding
* the need to create one manually via {@link ResetCacheParameterGroupRequest#builder()}
*
*
* @param resetCacheParameterGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.ResetCacheParameterGroupRequest.Builder} to
* create a request. Represents the input of a ResetCacheParameterGroup
operation.
* @return A Java Future containing the result of the ResetCacheParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidCacheParameterGroupStateException The current state of the cache parameter group does not
* allow the requested operation to occur.
* - CacheParameterGroupNotFoundException The requested cache parameter group name does not refer to an
* existing cache parameter group.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - InvalidGlobalReplicationGroupStateException The Global datastore is not available or in primary-only
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.ResetCacheParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture resetCacheParameterGroup(
Consumer resetCacheParameterGroupRequest) {
return resetCacheParameterGroup(ResetCacheParameterGroupRequest.builder().applyMutation(resetCacheParameterGroupRequest)
.build());
}
/**
*
* Revokes ingress from a cache security group. Use this operation to disallow access from an Amazon EC2 security
* group that had been previously authorized.
*
*
* @param revokeCacheSecurityGroupIngressRequest
* Represents the input of a RevokeCacheSecurityGroupIngress
operation.
* @return A Java Future containing the result of the RevokeCacheSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - AuthorizationNotFoundException The specified Amazon EC2 security group is not authorized for the
* specified cache security group.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RevokeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture revokeCacheSecurityGroupIngress(
RevokeCacheSecurityGroupIngressRequest revokeCacheSecurityGroupIngressRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Revokes ingress from a cache security group. Use this operation to disallow access from an Amazon EC2 security
* group that had been previously authorized.
*
*
*
* This is a convenience which creates an instance of the {@link RevokeCacheSecurityGroupIngressRequest.Builder}
* avoiding the need to create one manually via {@link RevokeCacheSecurityGroupIngressRequest#builder()}
*
*
* @param revokeCacheSecurityGroupIngressRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.RevokeCacheSecurityGroupIngressRequest.Builder}
* to create a request. Represents the input of a RevokeCacheSecurityGroupIngress
operation.
* @return A Java Future containing the result of the RevokeCacheSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CacheSecurityGroupNotFoundException The requested cache security group name does not refer to an
* existing cache security group.
* - AuthorizationNotFoundException The specified Amazon EC2 security group is not authorized for the
* specified cache security group.
* - InvalidCacheSecurityGroupStateException The current state of the cache security group does not allow
* deletion.
* - InvalidParameterValueException The value for a parameter is invalid.
* - InvalidParameterCombinationException Two or more incompatible parameters were specified.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.RevokeCacheSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture revokeCacheSecurityGroupIngress(
Consumer revokeCacheSecurityGroupIngressRequest) {
return revokeCacheSecurityGroupIngress(RevokeCacheSecurityGroupIngressRequest.builder()
.applyMutation(revokeCacheSecurityGroupIngressRequest).build());
}
/**
*
* Start the migration of data.
*
*
* @param startMigrationRequest
* @return A Java Future containing the result of the StartMigration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupAlreadyUnderMigrationException The targeted replication group is not available.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.StartMigration
* @see AWS
* API Documentation
*/
default CompletableFuture startMigration(StartMigrationRequest startMigrationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Start the migration of data.
*
*
*
* This is a convenience which creates an instance of the {@link StartMigrationRequest.Builder} avoiding the need to
* create one manually via {@link StartMigrationRequest#builder()}
*
*
* @param startMigrationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticache.model.StartMigrationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartMigration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReplicationGroupNotFoundException The specified replication group does not exist.
* - InvalidReplicationGroupStateException The requested replication group is not in the
*
available
state.
* - ReplicationGroupAlreadyUnderMigrationException The targeted replication group is not available.
* - InvalidParameterValueException The value for a parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElastiCacheException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ElastiCacheAsyncClient.StartMigration
* @see AWS
* API Documentation
*/
default CompletableFuture startMigration(Consumer startMigrationRequest) {
return startMigration(StartMigrationRequest.builder().applyMutation(startMigrationRequest).build());
}
/**
*
* Represents the input of a TestFailover
operation which tests automatic failover on a specified node
* group (called shard in the console) in a replication group (called cluster in the console).
*
*
* This API is designed for testing the behavior of your application in case of ElastiCache failover. It is not
* designed to be an operational tool for initiating a failover to overcome a problem you may have with the cluster.
* Moreover, in certain conditions such as large-scale operational events, Amazon may block this API.
*
*
* Note the following
*
*
* -
*
* A customer can use this operation to test automatic failover on up to 15 shards (called node groups in the
* ElastiCache API and Amazon CLI) in any rolling 24-hour period.
*
*
* -
*
* If calling this operation on shards in different clusters (called replication groups in the API and CLI), the
* calls can be made concurrently.
*
*
*
* -
*
* If calling this operation multiple times on different shards in the same Valkey or Redis OSS (cluster mode
* enabled) replication group, the first node replacement must complete before a subsequent call can be made.
*
*
* -
*
* To determine whether the node replacement is complete you can check Events using the Amazon ElastiCache console,
* the Amazon CLI, or the ElastiCache API. Look for the following automatic failover related events, listed here in
* order of occurrance:
*
*
*