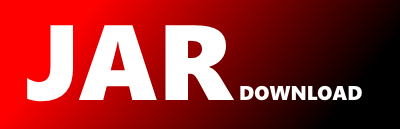
software.amazon.awssdk.services.elasticache.model.ConfigureShard Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Node group (shard) configuration options when adding or removing replicas. Each node group (shard) configuration has
* the following members: NodeGroupId, NewReplicaCount, and PreferredAvailabilityZones.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ConfigureShard implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NODE_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeGroupId").getter(getter(ConfigureShard::nodeGroupId)).setter(setter(Builder::nodeGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupId").build()).build();
private static final SdkField NEW_REPLICA_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NewReplicaCount").getter(getter(ConfigureShard::newReplicaCount))
.setter(setter(Builder::newReplicaCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewReplicaCount").build()).build();
private static final SdkField> PREFERRED_AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PreferredAvailabilityZones")
.getter(getter(ConfigureShard::preferredAvailabilityZones))
.setter(setter(Builder::preferredAvailabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName("PreferredAvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PreferredAvailabilityZone").build()).build()).build()).build();
private static final SdkField> PREFERRED_OUTPOST_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PreferredOutpostArns")
.getter(getter(ConfigureShard::preferredOutpostArns))
.setter(setter(Builder::preferredOutpostArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredOutpostArns").build(),
ListTrait
.builder()
.memberLocationName("PreferredOutpostArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PreferredOutpostArn").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NODE_GROUP_ID_FIELD,
NEW_REPLICA_COUNT_FIELD, PREFERRED_AVAILABILITY_ZONES_FIELD, PREFERRED_OUTPOST_ARNS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String nodeGroupId;
private final Integer newReplicaCount;
private final List preferredAvailabilityZones;
private final List preferredOutpostArns;
private ConfigureShard(BuilderImpl builder) {
this.nodeGroupId = builder.nodeGroupId;
this.newReplicaCount = builder.newReplicaCount;
this.preferredAvailabilityZones = builder.preferredAvailabilityZones;
this.preferredOutpostArns = builder.preferredOutpostArns;
}
/**
*
* The 4-digit id for the node group you are configuring. For Valkey or Redis OSS (cluster mode disabled)
* replication groups, the node group id is always 0001. To find a Valkey or Redis OSS (cluster mode enabled)'s node
* group's (shard's) id, see Finding a Shard's Id.
*
*
* @return The 4-digit id for the node group you are configuring. For Valkey or Redis OSS (cluster mode disabled)
* replication groups, the node group id is always 0001. To find a Valkey or Redis OSS (cluster mode
* enabled)'s node group's (shard's) id, see Finding a Shard's
* Id.
*/
public final String nodeGroupId() {
return nodeGroupId;
}
/**
*
* The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Valkey or Redis OSS replication
* group you are working with.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your
* primary node fails)
*
*
*
*
* @return The number of replicas you want in this node group at the end of this operation. The maximum value for
* NewReplicaCount
is 5. The minimum value depends upon the type of Valkey or Redis OSS
* replication group you are working with.
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ: 1
*
*
* -
*
* If Multi-AZ: 0
*
*
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if
* your primary node fails)
*
*
*/
public final Integer newReplicaCount() {
return newReplicaCount;
}
/**
* For responses, this returns true if the service returned a value for the PreferredAvailabilityZones property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasPreferredAvailabilityZones() {
return preferredAvailabilityZones != null && !(preferredAvailabilityZones instanceof SdkAutoConstructList);
}
/**
*
* A list of PreferredAvailabilityZone
strings that specify which availability zones the replication
* group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must equal the value of
* NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache selects the availability zone for each of the replicas.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPreferredAvailabilityZones} method.
*
*
* @return A list of PreferredAvailabilityZone
strings that specify which availability zones the
* replication group's nodes are to be in. The nummber of PreferredAvailabilityZone
values must
* equal the value of NewReplicaCount
plus 1 to account for the primary node. If this member of
* ReplicaConfiguration
is omitted, ElastiCache selects the availability zone for each of the
* replicas.
*/
public final List preferredAvailabilityZones() {
return preferredAvailabilityZones;
}
/**
* For responses, this returns true if the service returned a value for the PreferredOutpostArns property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPreferredOutpostArns() {
return preferredOutpostArns != null && !(preferredOutpostArns instanceof SdkAutoConstructList);
}
/**
*
* The outpost ARNs in which the cache cluster is created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPreferredOutpostArns} method.
*
*
* @return The outpost ARNs in which the cache cluster is created.
*/
public final List preferredOutpostArns() {
return preferredOutpostArns;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(nodeGroupId());
hashCode = 31 * hashCode + Objects.hashCode(newReplicaCount());
hashCode = 31 * hashCode + Objects.hashCode(hasPreferredAvailabilityZones() ? preferredAvailabilityZones() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPreferredOutpostArns() ? preferredOutpostArns() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ConfigureShard)) {
return false;
}
ConfigureShard other = (ConfigureShard) obj;
return Objects.equals(nodeGroupId(), other.nodeGroupId()) && Objects.equals(newReplicaCount(), other.newReplicaCount())
&& hasPreferredAvailabilityZones() == other.hasPreferredAvailabilityZones()
&& Objects.equals(preferredAvailabilityZones(), other.preferredAvailabilityZones())
&& hasPreferredOutpostArns() == other.hasPreferredOutpostArns()
&& Objects.equals(preferredOutpostArns(), other.preferredOutpostArns());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ConfigureShard").add("NodeGroupId", nodeGroupId()).add("NewReplicaCount", newReplicaCount())
.add("PreferredAvailabilityZones", hasPreferredAvailabilityZones() ? preferredAvailabilityZones() : null)
.add("PreferredOutpostArns", hasPreferredOutpostArns() ? preferredOutpostArns() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NodeGroupId":
return Optional.ofNullable(clazz.cast(nodeGroupId()));
case "NewReplicaCount":
return Optional.ofNullable(clazz.cast(newReplicaCount()));
case "PreferredAvailabilityZones":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZones()));
case "PreferredOutpostArns":
return Optional.ofNullable(clazz.cast(preferredOutpostArns()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("NodeGroupId", NODE_GROUP_ID_FIELD);
map.put("NewReplicaCount", NEW_REPLICA_COUNT_FIELD);
map.put("PreferredAvailabilityZones", PREFERRED_AVAILABILITY_ZONES_FIELD);
map.put("PreferredOutpostArns", PREFERRED_OUTPOST_ARNS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function