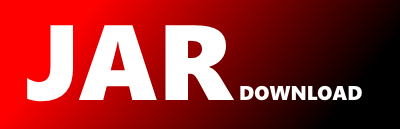
software.amazon.awssdk.services.elasticache.model.DecreaseReplicaCountRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DecreaseReplicaCountRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationGroupId").getter(getter(DecreaseReplicaCountRequest::replicationGroupId))
.setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField NEW_REPLICA_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NewReplicaCount").getter(getter(DecreaseReplicaCountRequest::newReplicaCount))
.setter(setter(Builder::newReplicaCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewReplicaCount").build()).build();
private static final SdkField> REPLICA_CONFIGURATION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReplicaConfiguration")
.getter(getter(DecreaseReplicaCountRequest::replicaConfiguration))
.setter(setter(Builder::replicaConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicaConfiguration").build(),
ListTrait
.builder()
.memberLocationName("ConfigureShard")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ConfigureShard::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ConfigureShard").build()).build()).build()).build();
private static final SdkField> REPLICAS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReplicasToRemove")
.getter(getter(DecreaseReplicaCountRequest::replicasToRemove))
.setter(setter(Builder::replicasToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicasToRemove").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(DecreaseReplicaCountRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
NEW_REPLICA_COUNT_FIELD, REPLICA_CONFIGURATION_FIELD, REPLICAS_TO_REMOVE_FIELD, APPLY_IMMEDIATELY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String replicationGroupId;
private final Integer newReplicaCount;
private final List replicaConfiguration;
private final List replicasToRemove;
private final Boolean applyImmediately;
private DecreaseReplicaCountRequest(BuilderImpl builder) {
super(builder);
this.replicationGroupId = builder.replicationGroupId;
this.newReplicaCount = builder.newReplicaCount;
this.replicaConfiguration = builder.replicaConfiguration;
this.replicasToRemove = builder.replicasToRemove;
this.applyImmediately = builder.applyImmediately;
}
/**
*
* The id of the replication group from which you want to remove replica nodes.
*
*
* @return The id of the replication group from which you want to remove replica nodes.
*/
public final String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The number of read replica nodes you want at the completion of this operation. For Valkey or Redis OSS (cluster
* mode disabled) replication groups, this is the number of replica nodes in the replication group. For Valkey or
* Redis OSS (cluster mode enabled) replication groups, this is the number of replica nodes in each of the
* replication group's node groups.
*
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ is enabled: 1
*
*
* -
*
* If Multi-AZ is not enabled: 0
*
*
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if your
* primary node fails)
*
*
*
*
* @return The number of read replica nodes you want at the completion of this operation. For Valkey or Redis OSS
* (cluster mode disabled) replication groups, this is the number of replica nodes in the replication group.
* For Valkey or Redis OSS (cluster mode enabled) replication groups, this is the number of replica nodes in
* each of the replication group's node groups.
*
* The minimum number of replicas in a shard or replication group is:
*
*
* -
*
* Valkey or Redis OSS (cluster mode disabled)
*
*
* -
*
* If Multi-AZ is enabled: 1
*
*
* -
*
* If Multi-AZ is not enabled: 0
*
*
*
*
* -
*
* Valkey or Redis OSS (cluster mode enabled): 0 (though you will not be able to failover to a replica if
* your primary node fails)
*
*
*/
public final Integer newReplicaCount() {
return newReplicaCount;
}
/**
* For responses, this returns true if the service returned a value for the ReplicaConfiguration property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReplicaConfiguration() {
return replicaConfiguration != null && !(replicaConfiguration instanceof SdkAutoConstructList);
}
/**
*
* A list of ConfigureShard
objects that can be used to configure each shard in a Valkey or Redis OSS
* (cluster mode enabled) replication group. The ConfigureShard
has three members:
* NewReplicaCount
, NodeGroupId
, and PreferredAvailabilityZones
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReplicaConfiguration} method.
*
*
* @return A list of ConfigureShard
objects that can be used to configure each shard in a Valkey or
* Redis OSS (cluster mode enabled) replication group. The ConfigureShard
has three members:
* NewReplicaCount
, NodeGroupId
, and PreferredAvailabilityZones
.
*/
public final List replicaConfiguration() {
return replicaConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the ReplicasToRemove property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReplicasToRemove() {
return replicasToRemove != null && !(replicasToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of the node ids to remove from the replication group or node group (shard).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReplicasToRemove} method.
*
*
* @return A list of the node ids to remove from the replication group or node group (shard).
*/
public final List replicasToRemove() {
return replicasToRemove;
}
/**
*
* If True
, the number of replica nodes is decreased immediately. ApplyImmediately=False
* is not currently supported.
*
*
* @return If True
, the number of replica nodes is decreased immediately.
* ApplyImmediately=False
is not currently supported.
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(newReplicaCount());
hashCode = 31 * hashCode + Objects.hashCode(hasReplicaConfiguration() ? replicaConfiguration() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasReplicasToRemove() ? replicasToRemove() : null);
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DecreaseReplicaCountRequest)) {
return false;
}
DecreaseReplicaCountRequest other = (DecreaseReplicaCountRequest) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(newReplicaCount(), other.newReplicaCount())
&& hasReplicaConfiguration() == other.hasReplicaConfiguration()
&& Objects.equals(replicaConfiguration(), other.replicaConfiguration())
&& hasReplicasToRemove() == other.hasReplicasToRemove()
&& Objects.equals(replicasToRemove(), other.replicasToRemove())
&& Objects.equals(applyImmediately(), other.applyImmediately());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DecreaseReplicaCountRequest").add("ReplicationGroupId", replicationGroupId())
.add("NewReplicaCount", newReplicaCount())
.add("ReplicaConfiguration", hasReplicaConfiguration() ? replicaConfiguration() : null)
.add("ReplicasToRemove", hasReplicasToRemove() ? replicasToRemove() : null)
.add("ApplyImmediately", applyImmediately()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "NewReplicaCount":
return Optional.ofNullable(clazz.cast(newReplicaCount()));
case "ReplicaConfiguration":
return Optional.ofNullable(clazz.cast(replicaConfiguration()));
case "ReplicasToRemove":
return Optional.ofNullable(clazz.cast(replicasToRemove()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ReplicationGroupId", REPLICATION_GROUP_ID_FIELD);
map.put("NewReplicaCount", NEW_REPLICA_COUNT_FIELD);
map.put("ReplicaConfiguration", REPLICA_CONFIGURATION_FIELD);
map.put("ReplicasToRemove", REPLICAS_TO_REMOVE_FIELD);
map.put("ApplyImmediately", APPLY_IMMEDIATELY_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function