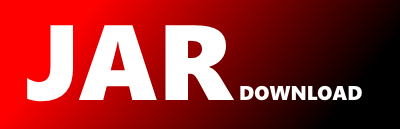
software.amazon.awssdk.services.elasticache.model.ServiceUpdate Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An update that you can apply to your Valkey or Redis OSS clusters.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceUpdate implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVICE_UPDATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUpdateName").getter(getter(ServiceUpdate::serviceUpdateName))
.setter(setter(Builder::serviceUpdateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateName").build()).build();
private static final SdkField SERVICE_UPDATE_RELEASE_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).memberName("ServiceUpdateReleaseDate")
.getter(getter(ServiceUpdate::serviceUpdateReleaseDate)).setter(setter(Builder::serviceUpdateReleaseDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateReleaseDate").build())
.build();
private static final SdkField SERVICE_UPDATE_END_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ServiceUpdateEndDate").getter(getter(ServiceUpdate::serviceUpdateEndDate))
.setter(setter(Builder::serviceUpdateEndDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateEndDate").build())
.build();
private static final SdkField SERVICE_UPDATE_SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUpdateSeverity").getter(getter(ServiceUpdate::serviceUpdateSeverityAsString))
.setter(setter(Builder::serviceUpdateSeverity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateSeverity").build())
.build();
private static final SdkField SERVICE_UPDATE_RECOMMENDED_APPLY_BY_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ServiceUpdateRecommendedApplyByDate")
.getter(getter(ServiceUpdate::serviceUpdateRecommendedApplyByDate))
.setter(setter(Builder::serviceUpdateRecommendedApplyByDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ServiceUpdateRecommendedApplyByDate").build()).build();
private static final SdkField SERVICE_UPDATE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUpdateStatus").getter(getter(ServiceUpdate::serviceUpdateStatusAsString))
.setter(setter(Builder::serviceUpdateStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateStatus").build())
.build();
private static final SdkField SERVICE_UPDATE_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUpdateDescription").getter(getter(ServiceUpdate::serviceUpdateDescription))
.setter(setter(Builder::serviceUpdateDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateDescription").build())
.build();
private static final SdkField SERVICE_UPDATE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceUpdateType").getter(getter(ServiceUpdate::serviceUpdateTypeAsString))
.setter(setter(Builder::serviceUpdateType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(ServiceUpdate::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ServiceUpdate::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField AUTO_UPDATE_AFTER_RECOMMENDED_APPLY_BY_DATE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AutoUpdateAfterRecommendedApplyByDate")
.getter(getter(ServiceUpdate::autoUpdateAfterRecommendedApplyByDate))
.setter(setter(Builder::autoUpdateAfterRecommendedApplyByDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AutoUpdateAfterRecommendedApplyByDate").build()).build();
private static final SdkField ESTIMATED_UPDATE_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EstimatedUpdateTime").getter(getter(ServiceUpdate::estimatedUpdateTime))
.setter(setter(Builder::estimatedUpdateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedUpdateTime").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_UPDATE_NAME_FIELD,
SERVICE_UPDATE_RELEASE_DATE_FIELD, SERVICE_UPDATE_END_DATE_FIELD, SERVICE_UPDATE_SEVERITY_FIELD,
SERVICE_UPDATE_RECOMMENDED_APPLY_BY_DATE_FIELD, SERVICE_UPDATE_STATUS_FIELD, SERVICE_UPDATE_DESCRIPTION_FIELD,
SERVICE_UPDATE_TYPE_FIELD, ENGINE_FIELD, ENGINE_VERSION_FIELD, AUTO_UPDATE_AFTER_RECOMMENDED_APPLY_BY_DATE_FIELD,
ESTIMATED_UPDATE_TIME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String serviceUpdateName;
private final Instant serviceUpdateReleaseDate;
private final Instant serviceUpdateEndDate;
private final String serviceUpdateSeverity;
private final Instant serviceUpdateRecommendedApplyByDate;
private final String serviceUpdateStatus;
private final String serviceUpdateDescription;
private final String serviceUpdateType;
private final String engine;
private final String engineVersion;
private final Boolean autoUpdateAfterRecommendedApplyByDate;
private final String estimatedUpdateTime;
private ServiceUpdate(BuilderImpl builder) {
this.serviceUpdateName = builder.serviceUpdateName;
this.serviceUpdateReleaseDate = builder.serviceUpdateReleaseDate;
this.serviceUpdateEndDate = builder.serviceUpdateEndDate;
this.serviceUpdateSeverity = builder.serviceUpdateSeverity;
this.serviceUpdateRecommendedApplyByDate = builder.serviceUpdateRecommendedApplyByDate;
this.serviceUpdateStatus = builder.serviceUpdateStatus;
this.serviceUpdateDescription = builder.serviceUpdateDescription;
this.serviceUpdateType = builder.serviceUpdateType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.autoUpdateAfterRecommendedApplyByDate = builder.autoUpdateAfterRecommendedApplyByDate;
this.estimatedUpdateTime = builder.estimatedUpdateTime;
}
/**
*
* The unique ID of the service update
*
*
* @return The unique ID of the service update
*/
public final String serviceUpdateName() {
return serviceUpdateName;
}
/**
*
* The date when the service update is initially available
*
*
* @return The date when the service update is initially available
*/
public final Instant serviceUpdateReleaseDate() {
return serviceUpdateReleaseDate;
}
/**
*
* The date after which the service update is no longer available
*
*
* @return The date after which the service update is no longer available
*/
public final Instant serviceUpdateEndDate() {
return serviceUpdateEndDate;
}
/**
*
* The severity of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateSeverity} will return {@link ServiceUpdateSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateSeverityAsString}.
*
*
* @return The severity of the service update
* @see ServiceUpdateSeverity
*/
public final ServiceUpdateSeverity serviceUpdateSeverity() {
return ServiceUpdateSeverity.fromValue(serviceUpdateSeverity);
}
/**
*
* The severity of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateSeverity} will return {@link ServiceUpdateSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateSeverityAsString}.
*
*
* @return The severity of the service update
* @see ServiceUpdateSeverity
*/
public final String serviceUpdateSeverityAsString() {
return serviceUpdateSeverity;
}
/**
*
* The recommendend date to apply the service update in order to ensure compliance. For information on compliance,
* see Self-Service Security Updates for Compliance.
*
*
* @return The recommendend date to apply the service update in order to ensure compliance. For information on
* compliance, see Self-Service Security Updates for Compliance.
*/
public final Instant serviceUpdateRecommendedApplyByDate() {
return serviceUpdateRecommendedApplyByDate;
}
/**
*
* The status of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateStatus} will return {@link ServiceUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateStatusAsString}.
*
*
* @return The status of the service update
* @see ServiceUpdateStatus
*/
public final ServiceUpdateStatus serviceUpdateStatus() {
return ServiceUpdateStatus.fromValue(serviceUpdateStatus);
}
/**
*
* The status of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateStatus} will return {@link ServiceUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateStatusAsString}.
*
*
* @return The status of the service update
* @see ServiceUpdateStatus
*/
public final String serviceUpdateStatusAsString() {
return serviceUpdateStatus;
}
/**
*
* Provides details of the service update
*
*
* @return Provides details of the service update
*/
public final String serviceUpdateDescription() {
return serviceUpdateDescription;
}
/**
*
* Reflects the nature of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceUpdateType}
* will return {@link ServiceUpdateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceUpdateTypeAsString}.
*
*
* @return Reflects the nature of the service update
* @see ServiceUpdateType
*/
public final ServiceUpdateType serviceUpdateType() {
return ServiceUpdateType.fromValue(serviceUpdateType);
}
/**
*
* Reflects the nature of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceUpdateType}
* will return {@link ServiceUpdateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceUpdateTypeAsString}.
*
*
* @return Reflects the nature of the service update
* @see ServiceUpdateType
*/
public final String serviceUpdateTypeAsString() {
return serviceUpdateType;
}
/**
*
* The Elasticache engine to which the update applies. Either Valkey, Redis OSS or Memcached.
*
*
* @return The Elasticache engine to which the update applies. Either Valkey, Redis OSS or Memcached.
*/
public final String engine() {
return engine;
}
/**
*
* The Elasticache engine version to which the update applies. Either Valkey, Redis OSS or Memcached engine version.
*
*
* @return The Elasticache engine version to which the update applies. Either Valkey, Redis OSS or Memcached engine
* version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*
*
* @return Indicates whether the service update will be automatically applied once the recommended apply-by date has
* expired.
*/
public final Boolean autoUpdateAfterRecommendedApplyByDate() {
return autoUpdateAfterRecommendedApplyByDate;
}
/**
*
* The estimated length of time the service update will take
*
*
* @return The estimated length of time the service update will take
*/
public final String estimatedUpdateTime() {
return estimatedUpdateTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateName());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateReleaseDate());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateEndDate());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateSeverityAsString());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateRecommendedApplyByDate());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateDescription());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(autoUpdateAfterRecommendedApplyByDate());
hashCode = 31 * hashCode + Objects.hashCode(estimatedUpdateTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceUpdate)) {
return false;
}
ServiceUpdate other = (ServiceUpdate) obj;
return Objects.equals(serviceUpdateName(), other.serviceUpdateName())
&& Objects.equals(serviceUpdateReleaseDate(), other.serviceUpdateReleaseDate())
&& Objects.equals(serviceUpdateEndDate(), other.serviceUpdateEndDate())
&& Objects.equals(serviceUpdateSeverityAsString(), other.serviceUpdateSeverityAsString())
&& Objects.equals(serviceUpdateRecommendedApplyByDate(), other.serviceUpdateRecommendedApplyByDate())
&& Objects.equals(serviceUpdateStatusAsString(), other.serviceUpdateStatusAsString())
&& Objects.equals(serviceUpdateDescription(), other.serviceUpdateDescription())
&& Objects.equals(serviceUpdateTypeAsString(), other.serviceUpdateTypeAsString())
&& Objects.equals(engine(), other.engine()) && Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(autoUpdateAfterRecommendedApplyByDate(), other.autoUpdateAfterRecommendedApplyByDate())
&& Objects.equals(estimatedUpdateTime(), other.estimatedUpdateTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceUpdate").add("ServiceUpdateName", serviceUpdateName())
.add("ServiceUpdateReleaseDate", serviceUpdateReleaseDate()).add("ServiceUpdateEndDate", serviceUpdateEndDate())
.add("ServiceUpdateSeverity", serviceUpdateSeverityAsString())
.add("ServiceUpdateRecommendedApplyByDate", serviceUpdateRecommendedApplyByDate())
.add("ServiceUpdateStatus", serviceUpdateStatusAsString())
.add("ServiceUpdateDescription", serviceUpdateDescription())
.add("ServiceUpdateType", serviceUpdateTypeAsString()).add("Engine", engine())
.add("EngineVersion", engineVersion())
.add("AutoUpdateAfterRecommendedApplyByDate", autoUpdateAfterRecommendedApplyByDate())
.add("EstimatedUpdateTime", estimatedUpdateTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServiceUpdateName":
return Optional.ofNullable(clazz.cast(serviceUpdateName()));
case "ServiceUpdateReleaseDate":
return Optional.ofNullable(clazz.cast(serviceUpdateReleaseDate()));
case "ServiceUpdateEndDate":
return Optional.ofNullable(clazz.cast(serviceUpdateEndDate()));
case "ServiceUpdateSeverity":
return Optional.ofNullable(clazz.cast(serviceUpdateSeverityAsString()));
case "ServiceUpdateRecommendedApplyByDate":
return Optional.ofNullable(clazz.cast(serviceUpdateRecommendedApplyByDate()));
case "ServiceUpdateStatus":
return Optional.ofNullable(clazz.cast(serviceUpdateStatusAsString()));
case "ServiceUpdateDescription":
return Optional.ofNullable(clazz.cast(serviceUpdateDescription()));
case "ServiceUpdateType":
return Optional.ofNullable(clazz.cast(serviceUpdateTypeAsString()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AutoUpdateAfterRecommendedApplyByDate":
return Optional.ofNullable(clazz.cast(autoUpdateAfterRecommendedApplyByDate()));
case "EstimatedUpdateTime":
return Optional.ofNullable(clazz.cast(estimatedUpdateTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ServiceUpdateName", SERVICE_UPDATE_NAME_FIELD);
map.put("ServiceUpdateReleaseDate", SERVICE_UPDATE_RELEASE_DATE_FIELD);
map.put("ServiceUpdateEndDate", SERVICE_UPDATE_END_DATE_FIELD);
map.put("ServiceUpdateSeverity", SERVICE_UPDATE_SEVERITY_FIELD);
map.put("ServiceUpdateRecommendedApplyByDate", SERVICE_UPDATE_RECOMMENDED_APPLY_BY_DATE_FIELD);
map.put("ServiceUpdateStatus", SERVICE_UPDATE_STATUS_FIELD);
map.put("ServiceUpdateDescription", SERVICE_UPDATE_DESCRIPTION_FIELD);
map.put("ServiceUpdateType", SERVICE_UPDATE_TYPE_FIELD);
map.put("Engine", ENGINE_FIELD);
map.put("EngineVersion", ENGINE_VERSION_FIELD);
map.put("AutoUpdateAfterRecommendedApplyByDate", AUTO_UPDATE_AFTER_RECOMMENDED_APPLY_BY_DATE_FIELD);
map.put("EstimatedUpdateTime", ESTIMATED_UPDATE_TIME_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function