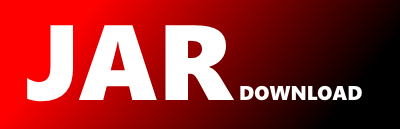
software.amazon.awssdk.services.elasticache.model.CacheCluster Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains all of the attributes of a specific cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CacheCluster implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::cacheClusterId)).setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField CONFIGURATION_ENDPOINT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CacheCluster::configurationEndpoint)).setter(setter(Builder::configurationEndpoint))
.constructor(Endpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationEndpoint").build())
.build();
private static final SdkField CLIENT_DOWNLOAD_LANDING_PAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::clientDownloadLandingPage)).setter(setter(Builder::clientDownloadLandingPage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientDownloadLandingPage").build())
.build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField CACHE_CLUSTER_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::cacheClusterStatus)).setter(setter(Builder::cacheClusterStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterStatus").build())
.build();
private static final SdkField NUM_CACHE_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CacheCluster::numCacheNodes)).setter(setter(Builder::numCacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheNodes").build()).build();
private static final SdkField PREFERRED_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::preferredAvailabilityZone)).setter(setter(Builder::preferredAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZone").build())
.build();
private static final SdkField CACHE_CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(CacheCluster::cacheClusterCreateTime)).setter(setter(Builder::cacheClusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterCreateTime").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField PENDING_MODIFIED_VALUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(CacheCluster::pendingModifiedValues))
.setter(setter(Builder::pendingModifiedValues)).constructor(PendingModifiedValues::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingModifiedValues").build())
.build();
private static final SdkField NOTIFICATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(CacheCluster::notificationConfiguration)).setter(setter(Builder::notificationConfiguration))
.constructor(NotificationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationConfiguration").build())
.build();
private static final SdkField> CACHE_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CacheCluster::cacheSecurityGroups))
.setter(setter(Builder::cacheSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CacheSecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroup").build()).build()).build()).build();
private static final SdkField CACHE_PARAMETER_GROUP_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(CacheCluster::cacheParameterGroup))
.setter(setter(Builder::cacheParameterGroup)).constructor(CacheParameterGroupStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroup").build())
.build();
private static final SdkField CACHE_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::cacheSubnetGroupName)).setter(setter(Builder::cacheSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSubnetGroupName").build())
.build();
private static final SdkField> CACHE_NODES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CacheCluster::cacheNodes))
.setter(setter(Builder::cacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodes").build(),
ListTrait
.builder()
.memberLocationName("CacheNode")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CacheNode::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheNode").build()).build()).build()).build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(CacheCluster::autoMinorVersionUpgrade)).setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CacheCluster::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::replicationGroupId)).setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CacheCluster::snapshotRetentionLimit)).setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CacheCluster::snapshotWindow)).setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField AUTH_TOKEN_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(CacheCluster::authTokenEnabled)).setter(setter(Builder::authTokenEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenEnabled").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(CacheCluster::transitEncryptionEnabled)).setter(setter(Builder::transitEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionEnabled").build())
.build();
private static final SdkField AT_REST_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(CacheCluster::atRestEncryptionEnabled)).setter(setter(Builder::atRestEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AtRestEncryptionEnabled").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CACHE_CLUSTER_ID_FIELD,
CONFIGURATION_ENDPOINT_FIELD, CLIENT_DOWNLOAD_LANDING_PAGE_FIELD, CACHE_NODE_TYPE_FIELD, ENGINE_FIELD,
ENGINE_VERSION_FIELD, CACHE_CLUSTER_STATUS_FIELD, NUM_CACHE_NODES_FIELD, PREFERRED_AVAILABILITY_ZONE_FIELD,
CACHE_CLUSTER_CREATE_TIME_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, PENDING_MODIFIED_VALUES_FIELD,
NOTIFICATION_CONFIGURATION_FIELD, CACHE_SECURITY_GROUPS_FIELD, CACHE_PARAMETER_GROUP_FIELD,
CACHE_SUBNET_GROUP_NAME_FIELD, CACHE_NODES_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, SECURITY_GROUPS_FIELD,
REPLICATION_GROUP_ID_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD, SNAPSHOT_WINDOW_FIELD, AUTH_TOKEN_ENABLED_FIELD,
TRANSIT_ENCRYPTION_ENABLED_FIELD, AT_REST_ENCRYPTION_ENABLED_FIELD));
private static final long serialVersionUID = 1L;
private final String cacheClusterId;
private final Endpoint configurationEndpoint;
private final String clientDownloadLandingPage;
private final String cacheNodeType;
private final String engine;
private final String engineVersion;
private final String cacheClusterStatus;
private final Integer numCacheNodes;
private final String preferredAvailabilityZone;
private final Instant cacheClusterCreateTime;
private final String preferredMaintenanceWindow;
private final PendingModifiedValues pendingModifiedValues;
private final NotificationConfiguration notificationConfiguration;
private final List cacheSecurityGroups;
private final CacheParameterGroupStatus cacheParameterGroup;
private final String cacheSubnetGroupName;
private final List cacheNodes;
private final Boolean autoMinorVersionUpgrade;
private final List securityGroups;
private final String replicationGroupId;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final Boolean authTokenEnabled;
private final Boolean transitEncryptionEnabled;
private final Boolean atRestEncryptionEnabled;
private CacheCluster(BuilderImpl builder) {
this.cacheClusterId = builder.cacheClusterId;
this.configurationEndpoint = builder.configurationEndpoint;
this.clientDownloadLandingPage = builder.clientDownloadLandingPage;
this.cacheNodeType = builder.cacheNodeType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.cacheClusterStatus = builder.cacheClusterStatus;
this.numCacheNodes = builder.numCacheNodes;
this.preferredAvailabilityZone = builder.preferredAvailabilityZone;
this.cacheClusterCreateTime = builder.cacheClusterCreateTime;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.pendingModifiedValues = builder.pendingModifiedValues;
this.notificationConfiguration = builder.notificationConfiguration;
this.cacheSecurityGroups = builder.cacheSecurityGroups;
this.cacheParameterGroup = builder.cacheParameterGroup;
this.cacheSubnetGroupName = builder.cacheSubnetGroupName;
this.cacheNodes = builder.cacheNodes;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.securityGroups = builder.securityGroups;
this.replicationGroupId = builder.replicationGroupId;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.authTokenEnabled = builder.authTokenEnabled;
this.transitEncryptionEnabled = builder.transitEncryptionEnabled;
this.atRestEncryptionEnabled = builder.atRestEncryptionEnabled;
}
/**
*
* The user-supplied identifier of the cluster. This identifier is a unique key that identifies a cluster.
*
*
* @return The user-supplied identifier of the cluster. This identifier is a unique key that identifies a cluster.
*/
public String cacheClusterId() {
return cacheClusterId;
}
/**
*
* Represents a Memcached cluster endpoint which, if Automatic Discovery is enabled on the cluster, can be used by
* an application to connect to any node in the cluster. The configuration endpoint will always have
* .cfg
in it.
*
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
*
*
* @return Represents a Memcached cluster endpoint which, if Automatic Discovery is enabled on the cluster, can be
* used by an application to connect to any node in the cluster. The configuration endpoint will always have
* .cfg
in it.
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
*/
public Endpoint configurationEndpoint() {
return configurationEndpoint;
}
/**
*
* The URL of the web page where you can download the latest ElastiCache client library.
*
*
* @return The URL of the web page where you can download the latest ElastiCache client library.
*/
public String clientDownloadLandingPage() {
return clientDownloadLandingPage;
}
/**
*
* The name of the compute and memory capacity node type for the cluster.
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return The name of the compute and memory capacity node type for the cluster.
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The name of the cache engine (memcached
or redis
) to be used for this cluster.
*
*
* @return The name of the cache engine (memcached
or redis
) to be used for this cluster.
*/
public String engine() {
return engine;
}
/**
*
* The version of the cache engine that is used in this cluster.
*
*
* @return The version of the cache engine that is used in this cluster.
*/
public String engineVersion() {
return engineVersion;
}
/**
*
* The current state of this cluster, one of the following values: available
, creating
,
* deleted
, deleting
, incompatible-network
, modifying
,
* rebooting cluster nodes
, restore-failed
, or snapshotting
.
*
*
* @return The current state of this cluster, one of the following values: available
,
* creating
, deleted
, deleting
, incompatible-network
,
* modifying
, rebooting cluster nodes
, restore-failed
, or
* snapshotting
.
*/
public String cacheClusterStatus() {
return cacheClusterStatus;
}
/**
*
* The number of cache nodes in the cluster.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be between 1
* and 20.
*
*
* @return The number of cache nodes in the cluster.
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 20.
*/
public Integer numCacheNodes() {
return numCacheNodes;
}
/**
*
* The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are located in
* different Availability Zones.
*
*
* @return The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are
* located in different Availability Zones.
*/
public String preferredAvailabilityZone() {
return preferredAvailabilityZone;
}
/**
*
* The date and time when the cluster was created.
*
*
* @return The date and time when the cluster was created.
*/
public Instant cacheClusterCreateTime() {
return cacheClusterCreateTime;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
* Returns the value of the PendingModifiedValues property for this object.
*
* @return The value of the PendingModifiedValues property for this object.
*/
public PendingModifiedValues pendingModifiedValues() {
return pendingModifiedValues;
}
/**
*
* Describes a notification topic and its status. Notification topics are used for publishing ElastiCache events to
* subscribers using Amazon Simple Notification Service (SNS).
*
*
* @return Describes a notification topic and its status. Notification topics are used for publishing ElastiCache
* events to subscribers using Amazon Simple Notification Service (SNS).
*/
public NotificationConfiguration notificationConfiguration() {
return notificationConfiguration;
}
/**
*
* A list of cache security group elements, composed of name and status sub-elements.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of cache security group elements, composed of name and status sub-elements.
*/
public List cacheSecurityGroups() {
return cacheSecurityGroups;
}
/**
*
* Status of the cache parameter group.
*
*
* @return Status of the cache parameter group.
*/
public CacheParameterGroupStatus cacheParameterGroup() {
return cacheParameterGroup;
}
/**
*
* The name of the cache subnet group associated with the cluster.
*
*
* @return The name of the cache subnet group associated with the cluster.
*/
public String cacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
*
* A list of cache nodes that are members of the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of cache nodes that are members of the cluster.
*/
public List cacheNodes() {
return cacheNodes;
}
/**
*
* This parameter is currently disabled.
*
*
* @return This parameter is currently disabled.
*/
public Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* A list of VPC Security Groups associated with the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of VPC Security Groups associated with the cluster.
*/
public List securityGroups() {
return securityGroups;
}
/**
*
* The replication group to which this cluster belongs. If this field is empty, the cluster is not associated with
* any replication group.
*
*
* @return The replication group to which this cluster belongs. If this field is empty, the cluster is not
* associated with any replication group.
*/
public String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*/
public Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* Example: 05:00-09:00
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your
* cluster.
*
* Example: 05:00-09:00
*/
public String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
*
* Default: false
*
*
* @return A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
* Default: false
*/
public Boolean authTokenEnabled() {
return authTokenEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To enable
* in-transit encryption on a cluster you must set TransitEncryptionEnabled
to true
when
* you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*
*
* @return A flag that enables in-transit encryption when set to true
.
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To
* enable in-transit encryption on a cluster you must set TransitEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*/
public Boolean transitEncryptionEnabled() {
return transitEncryptionEnabled;
}
/**
*
* A flag that enables encryption at-rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To enable
* at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to true
when you
* create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*
*
* @return A flag that enables encryption at-rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To
* enable at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*/
public Boolean atRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(configurationEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(clientDownloadLandingPage());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterStatus());
hashCode = 31 * hashCode + Objects.hashCode(numCacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(preferredAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(pendingModifiedValues());
hashCode = 31 * hashCode + Objects.hashCode(notificationConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(cacheSecurityGroups());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroup());
hashCode = 31 * hashCode + Objects.hashCode(cacheSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(securityGroups());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(authTokenEnabled());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(atRestEncryptionEnabled());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CacheCluster)) {
return false;
}
CacheCluster other = (CacheCluster) obj;
return Objects.equals(cacheClusterId(), other.cacheClusterId())
&& Objects.equals(configurationEndpoint(), other.configurationEndpoint())
&& Objects.equals(clientDownloadLandingPage(), other.clientDownloadLandingPage())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(cacheClusterStatus(), other.cacheClusterStatus())
&& Objects.equals(numCacheNodes(), other.numCacheNodes())
&& Objects.equals(preferredAvailabilityZone(), other.preferredAvailabilityZone())
&& Objects.equals(cacheClusterCreateTime(), other.cacheClusterCreateTime())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(pendingModifiedValues(), other.pendingModifiedValues())
&& Objects.equals(notificationConfiguration(), other.notificationConfiguration())
&& Objects.equals(cacheSecurityGroups(), other.cacheSecurityGroups())
&& Objects.equals(cacheParameterGroup(), other.cacheParameterGroup())
&& Objects.equals(cacheSubnetGroupName(), other.cacheSubnetGroupName())
&& Objects.equals(cacheNodes(), other.cacheNodes())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(securityGroups(), other.securityGroups())
&& Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(authTokenEnabled(), other.authTokenEnabled())
&& Objects.equals(transitEncryptionEnabled(), other.transitEncryptionEnabled())
&& Objects.equals(atRestEncryptionEnabled(), other.atRestEncryptionEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CacheCluster").add("CacheClusterId", cacheClusterId())
.add("ConfigurationEndpoint", configurationEndpoint())
.add("ClientDownloadLandingPage", clientDownloadLandingPage()).add("CacheNodeType", cacheNodeType())
.add("Engine", engine()).add("EngineVersion", engineVersion()).add("CacheClusterStatus", cacheClusterStatus())
.add("NumCacheNodes", numCacheNodes()).add("PreferredAvailabilityZone", preferredAvailabilityZone())
.add("CacheClusterCreateTime", cacheClusterCreateTime())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("PendingModifiedValues", pendingModifiedValues())
.add("NotificationConfiguration", notificationConfiguration()).add("CacheSecurityGroups", cacheSecurityGroups())
.add("CacheParameterGroup", cacheParameterGroup()).add("CacheSubnetGroupName", cacheSubnetGroupName())
.add("CacheNodes", cacheNodes()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SecurityGroups", securityGroups()).add("ReplicationGroupId", replicationGroupId())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("AuthTokenEnabled", authTokenEnabled()).add("TransitEncryptionEnabled", transitEncryptionEnabled())
.add("AtRestEncryptionEnabled", atRestEncryptionEnabled()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "ConfigurationEndpoint":
return Optional.ofNullable(clazz.cast(configurationEndpoint()));
case "ClientDownloadLandingPage":
return Optional.ofNullable(clazz.cast(clientDownloadLandingPage()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "CacheClusterStatus":
return Optional.ofNullable(clazz.cast(cacheClusterStatus()));
case "NumCacheNodes":
return Optional.ofNullable(clazz.cast(numCacheNodes()));
case "PreferredAvailabilityZone":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZone()));
case "CacheClusterCreateTime":
return Optional.ofNullable(clazz.cast(cacheClusterCreateTime()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "PendingModifiedValues":
return Optional.ofNullable(clazz.cast(pendingModifiedValues()));
case "NotificationConfiguration":
return Optional.ofNullable(clazz.cast(notificationConfiguration()));
case "CacheSecurityGroups":
return Optional.ofNullable(clazz.cast(cacheSecurityGroups()));
case "CacheParameterGroup":
return Optional.ofNullable(clazz.cast(cacheParameterGroup()));
case "CacheSubnetGroupName":
return Optional.ofNullable(clazz.cast(cacheSubnetGroupName()));
case "CacheNodes":
return Optional.ofNullable(clazz.cast(cacheNodes()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "AuthTokenEnabled":
return Optional.ofNullable(clazz.cast(authTokenEnabled()));
case "TransitEncryptionEnabled":
return Optional.ofNullable(clazz.cast(transitEncryptionEnabled()));
case "AtRestEncryptionEnabled":
return Optional.ofNullable(clazz.cast(atRestEncryptionEnabled()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder configurationEndpoint(Endpoint configurationEndpoint);
/**
*
* Represents a Memcached cluster endpoint which, if Automatic Discovery is enabled on the cluster, can be used
* by an application to connect to any node in the cluster. The configuration endpoint will always have
* .cfg
in it.
*
*
* Example: mem-3.9dvc4r.cfg.usw2.cache.amazonaws.com:11211
*
* This is a convenience that creates an instance of the {@link Endpoint.Builder} avoiding the need to create
* one manually via {@link Endpoint#builder()}.
*
* When the {@link Consumer} completes, {@link Endpoint.Builder#build()} is called immediately and its result is
* passed to {@link #configurationEndpoint(Endpoint)}.
*
* @param configurationEndpoint
* a consumer that will call methods on {@link Endpoint.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #configurationEndpoint(Endpoint)
*/
default Builder configurationEndpoint(Consumer configurationEndpoint) {
return configurationEndpoint(Endpoint.builder().applyMutation(configurationEndpoint).build());
}
/**
*
* The URL of the web page where you can download the latest ElastiCache client library.
*
*
* @param clientDownloadLandingPage
* The URL of the web page where you can download the latest ElastiCache client library.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientDownloadLandingPage(String clientDownloadLandingPage);
/**
*
* The name of the compute and memory capacity node type for the cluster.
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param cacheNodeType
* The name of the compute and memory capacity node type for the cluster.
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation
* types provide more memory and computational power at lower cost when compared to their equivalent
* previous generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodeType(String cacheNodeType);
/**
*
* The name of the cache engine (memcached
or redis
) to be used for this cluster.
*
*
* @param engine
* The name of the cache engine (memcached
or redis
) to be used for this
* cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder engine(String engine);
/**
*
* The version of the cache engine that is used in this cluster.
*
*
* @param engineVersion
* The version of the cache engine that is used in this cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder engineVersion(String engineVersion);
/**
*
* The current state of this cluster, one of the following values: available
, creating
, deleted
, deleting
, incompatible-network
, modifying
,
* rebooting cluster nodes
, restore-failed
, or snapshotting
.
*
*
* @param cacheClusterStatus
* The current state of this cluster, one of the following values: available
,
* creating
, deleted
, deleting
, incompatible-network
,
* modifying
, rebooting cluster nodes
, restore-failed
, or
* snapshotting
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheClusterStatus(String cacheClusterStatus);
/**
*
* The number of cache nodes in the cluster.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 20.
*
*
* @param numCacheNodes
* The number of cache nodes in the cluster.
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 20.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder numCacheNodes(Integer numCacheNodes);
/**
*
* The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are
* located in different Availability Zones.
*
*
* @param preferredAvailabilityZone
* The name of the Availability Zone in which the cluster is located or "Multiple" if the cache nodes are
* located in different Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredAvailabilityZone(String preferredAvailabilityZone);
/**
*
* The date and time when the cluster was created.
*
*
* @param cacheClusterCreateTime
* The date and time when the cluster was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheClusterCreateTime(Instant cacheClusterCreateTime);
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified
* as a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a
* 60 minute period.
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredMaintenanceWindow(String preferredMaintenanceWindow);
/**
* Sets the value of the PendingModifiedValues property for this object.
*
* @param pendingModifiedValues
* The new value for the PendingModifiedValues property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder pendingModifiedValues(PendingModifiedValues pendingModifiedValues);
/**
* Sets the value of the PendingModifiedValues property for this object.
*
* This is a convenience that creates an instance of the {@link PendingModifiedValues.Builder} avoiding the need
* to create one manually via {@link PendingModifiedValues#builder()}.
*
* When the {@link Consumer} completes, {@link PendingModifiedValues.Builder#build()} is called immediately and
* its result is passed to {@link #pendingModifiedValues(PendingModifiedValues)}.
*
* @param pendingModifiedValues
* a consumer that will call methods on {@link PendingModifiedValues.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #pendingModifiedValues(PendingModifiedValues)
*/
default Builder pendingModifiedValues(Consumer pendingModifiedValues) {
return pendingModifiedValues(PendingModifiedValues.builder().applyMutation(pendingModifiedValues).build());
}
/**
*
* Describes a notification topic and its status. Notification topics are used for publishing ElastiCache events
* to subscribers using Amazon Simple Notification Service (SNS).
*
*
* @param notificationConfiguration
* Describes a notification topic and its status. Notification topics are used for publishing ElastiCache
* events to subscribers using Amazon Simple Notification Service (SNS).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationConfiguration(NotificationConfiguration notificationConfiguration);
/**
*
* Describes a notification topic and its status. Notification topics are used for publishing ElastiCache events
* to subscribers using Amazon Simple Notification Service (SNS).
*
* This is a convenience that creates an instance of the {@link NotificationConfiguration.Builder} avoiding the
* need to create one manually via {@link NotificationConfiguration#builder()}.
*
* When the {@link Consumer} completes, {@link NotificationConfiguration.Builder#build()} is called immediately
* and its result is passed to {@link #notificationConfiguration(NotificationConfiguration)}.
*
* @param notificationConfiguration
* a consumer that will call methods on {@link NotificationConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #notificationConfiguration(NotificationConfiguration)
*/
default Builder notificationConfiguration(Consumer notificationConfiguration) {
return notificationConfiguration(NotificationConfiguration.builder().applyMutation(notificationConfiguration).build());
}
/**
*
* A list of cache security group elements, composed of name and status sub-elements.
*
*
* @param cacheSecurityGroups
* A list of cache security group elements, composed of name and status sub-elements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSecurityGroups(Collection cacheSecurityGroups);
/**
*
* A list of cache security group elements, composed of name and status sub-elements.
*
*
* @param cacheSecurityGroups
* A list of cache security group elements, composed of name and status sub-elements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSecurityGroups(CacheSecurityGroupMembership... cacheSecurityGroups);
/**
*
* A list of cache security group elements, composed of name and status sub-elements.
*
* This is a convenience that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #cacheSecurityGroups(List)}.
*
* @param cacheSecurityGroups
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cacheSecurityGroups(List)
*/
Builder cacheSecurityGroups(Consumer... cacheSecurityGroups);
/**
*
* Status of the cache parameter group.
*
*
* @param cacheParameterGroup
* Status of the cache parameter group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheParameterGroup(CacheParameterGroupStatus cacheParameterGroup);
/**
*
* Status of the cache parameter group.
*
* This is a convenience that creates an instance of the {@link CacheParameterGroupStatus.Builder} avoiding the
* need to create one manually via {@link CacheParameterGroupStatus#builder()}.
*
* When the {@link Consumer} completes, {@link CacheParameterGroupStatus.Builder#build()} is called immediately
* and its result is passed to {@link #cacheParameterGroup(CacheParameterGroupStatus)}.
*
* @param cacheParameterGroup
* a consumer that will call methods on {@link CacheParameterGroupStatus.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cacheParameterGroup(CacheParameterGroupStatus)
*/
default Builder cacheParameterGroup(Consumer cacheParameterGroup) {
return cacheParameterGroup(CacheParameterGroupStatus.builder().applyMutation(cacheParameterGroup).build());
}
/**
*
* The name of the cache subnet group associated with the cluster.
*
*
* @param cacheSubnetGroupName
* The name of the cache subnet group associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSubnetGroupName(String cacheSubnetGroupName);
/**
*
* A list of cache nodes that are members of the cluster.
*
*
* @param cacheNodes
* A list of cache nodes that are members of the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodes(Collection cacheNodes);
/**
*
* A list of cache nodes that are members of the cluster.
*
*
* @param cacheNodes
* A list of cache nodes that are members of the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodes(CacheNode... cacheNodes);
/**
*
* A list of cache nodes that are members of the cluster.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #cacheNodes(List)}.
*
* @param cacheNodes
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cacheNodes(List)
*/
Builder cacheNodes(Consumer... cacheNodes);
/**
*
* This parameter is currently disabled.
*
*
* @param autoMinorVersionUpgrade
* This parameter is currently disabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade);
/**
*
* A list of VPC Security Groups associated with the cluster.
*
*
* @param securityGroups
* A list of VPC Security Groups associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroups(Collection securityGroups);
/**
*
* A list of VPC Security Groups associated with the cluster.
*
*
* @param securityGroups
* A list of VPC Security Groups associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroups(SecurityGroupMembership... securityGroups);
/**
*
* A list of VPC Security Groups associated with the cluster.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding
* the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called
* immediately and its result is passed to {@link #securityGroups(List)}.
*
* @param securityGroups
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #securityGroups(List)
*/
Builder securityGroups(Consumer... securityGroups);
/**
*
* The replication group to which this cluster belongs. If this field is empty, the cluster is not associated
* with any replication group.
*
*
* @param replicationGroupId
* The replication group to which this cluster belongs. If this field is empty, the cluster is not
* associated with any replication group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationGroupId(String replicationGroupId);
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for
* 5 days before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is
* retained for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit is set to zero (0), backups are turned off.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotRetentionLimit(Integer snapshotRetentionLimit);
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your cluster.
*
*
* Example: 05:00-09:00
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your
* cluster.
*
* Example: 05:00-09:00
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotWindow(String snapshotWindow);
/**
*
* A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
*
* Default: false
*
*
* @param authTokenEnabled
* A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder authTokenEnabled(Boolean authTokenEnabled);
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To enable
* in-transit encryption on a cluster you must set TransitEncryptionEnabled
to true
* when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*
*
* @param transitEncryptionEnabled
* A flag that enables in-transit encryption when set to true
.
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To
* enable in-transit encryption on a cluster you must set TransitEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder transitEncryptionEnabled(Boolean transitEncryptionEnabled);
/**
*
* A flag that enables encryption at-rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To enable
* at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to true
when
* you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
*
*
* @param atRestEncryptionEnabled
* A flag that enables encryption at-rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To
* enable at-rest encryption on a cluster you must set AtRestEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
or 4.x
.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder atRestEncryptionEnabled(Boolean atRestEncryptionEnabled);
}
static final class BuilderImpl implements Builder {
private String cacheClusterId;
private Endpoint configurationEndpoint;
private String clientDownloadLandingPage;
private String cacheNodeType;
private String engine;
private String engineVersion;
private String cacheClusterStatus;
private Integer numCacheNodes;
private String preferredAvailabilityZone;
private Instant cacheClusterCreateTime;
private String preferredMaintenanceWindow;
private PendingModifiedValues pendingModifiedValues;
private NotificationConfiguration notificationConfiguration;
private List cacheSecurityGroups = DefaultSdkAutoConstructList.getInstance();
private CacheParameterGroupStatus cacheParameterGroup;
private String cacheSubnetGroupName;
private List cacheNodes = DefaultSdkAutoConstructList.getInstance();
private Boolean autoMinorVersionUpgrade;
private List securityGroups = DefaultSdkAutoConstructList.getInstance();
private String replicationGroupId;
private Integer snapshotRetentionLimit;
private String snapshotWindow;
private Boolean authTokenEnabled;
private Boolean transitEncryptionEnabled;
private Boolean atRestEncryptionEnabled;
private BuilderImpl() {
}
private BuilderImpl(CacheCluster model) {
cacheClusterId(model.cacheClusterId);
configurationEndpoint(model.configurationEndpoint);
clientDownloadLandingPage(model.clientDownloadLandingPage);
cacheNodeType(model.cacheNodeType);
engine(model.engine);
engineVersion(model.engineVersion);
cacheClusterStatus(model.cacheClusterStatus);
numCacheNodes(model.numCacheNodes);
preferredAvailabilityZone(model.preferredAvailabilityZone);
cacheClusterCreateTime(model.cacheClusterCreateTime);
preferredMaintenanceWindow(model.preferredMaintenanceWindow);
pendingModifiedValues(model.pendingModifiedValues);
notificationConfiguration(model.notificationConfiguration);
cacheSecurityGroups(model.cacheSecurityGroups);
cacheParameterGroup(model.cacheParameterGroup);
cacheSubnetGroupName(model.cacheSubnetGroupName);
cacheNodes(model.cacheNodes);
autoMinorVersionUpgrade(model.autoMinorVersionUpgrade);
securityGroups(model.securityGroups);
replicationGroupId(model.replicationGroupId);
snapshotRetentionLimit(model.snapshotRetentionLimit);
snapshotWindow(model.snapshotWindow);
authTokenEnabled(model.authTokenEnabled);
transitEncryptionEnabled(model.transitEncryptionEnabled);
atRestEncryptionEnabled(model.atRestEncryptionEnabled);
}
public final String getCacheClusterId() {
return cacheClusterId;
}
@Override
public final Builder cacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
return this;
}
public final void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
public final Endpoint.Builder getConfigurationEndpoint() {
return configurationEndpoint != null ? configurationEndpoint.toBuilder() : null;
}
@Override
public final Builder configurationEndpoint(Endpoint configurationEndpoint) {
this.configurationEndpoint = configurationEndpoint;
return this;
}
public final void setConfigurationEndpoint(Endpoint.BuilderImpl configurationEndpoint) {
this.configurationEndpoint = configurationEndpoint != null ? configurationEndpoint.build() : null;
}
public final String getClientDownloadLandingPage() {
return clientDownloadLandingPage;
}
@Override
public final Builder clientDownloadLandingPage(String clientDownloadLandingPage) {
this.clientDownloadLandingPage = clientDownloadLandingPage;
return this;
}
public final void setClientDownloadLandingPage(String clientDownloadLandingPage) {
this.clientDownloadLandingPage = clientDownloadLandingPage;
}
public final String getCacheNodeType() {
return cacheNodeType;
}
@Override
public final Builder cacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
return this;
}
public final void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
public final String getEngine() {
return engine;
}
@Override
public final Builder engine(String engine) {
this.engine = engine;
return this;
}
public final void setEngine(String engine) {
this.engine = engine;
}
public final String getEngineVersion() {
return engineVersion;
}
@Override
public final Builder engineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public final void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
public final String getCacheClusterStatus() {
return cacheClusterStatus;
}
@Override
public final Builder cacheClusterStatus(String cacheClusterStatus) {
this.cacheClusterStatus = cacheClusterStatus;
return this;
}
public final void setCacheClusterStatus(String cacheClusterStatus) {
this.cacheClusterStatus = cacheClusterStatus;
}
public final Integer getNumCacheNodes() {
return numCacheNodes;
}
@Override
public final Builder numCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
return this;
}
public final void setNumCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
}
public final String getPreferredAvailabilityZone() {
return preferredAvailabilityZone;
}
@Override
public final Builder preferredAvailabilityZone(String preferredAvailabilityZone) {
this.preferredAvailabilityZone = preferredAvailabilityZone;
return this;
}
public final void setPreferredAvailabilityZone(String preferredAvailabilityZone) {
this.preferredAvailabilityZone = preferredAvailabilityZone;
}
public final Instant getCacheClusterCreateTime() {
return cacheClusterCreateTime;
}
@Override
public final Builder cacheClusterCreateTime(Instant cacheClusterCreateTime) {
this.cacheClusterCreateTime = cacheClusterCreateTime;
return this;
}
public final void setCacheClusterCreateTime(Instant cacheClusterCreateTime) {
this.cacheClusterCreateTime = cacheClusterCreateTime;
}
public final String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
@Override
public final Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
public final void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
public final PendingModifiedValues.Builder getPendingModifiedValues() {
return pendingModifiedValues != null ? pendingModifiedValues.toBuilder() : null;
}
@Override
public final Builder pendingModifiedValues(PendingModifiedValues pendingModifiedValues) {
this.pendingModifiedValues = pendingModifiedValues;
return this;
}
public final void setPendingModifiedValues(PendingModifiedValues.BuilderImpl pendingModifiedValues) {
this.pendingModifiedValues = pendingModifiedValues != null ? pendingModifiedValues.build() : null;
}
public final NotificationConfiguration.Builder getNotificationConfiguration() {
return notificationConfiguration != null ? notificationConfiguration.toBuilder() : null;
}
@Override
public final Builder notificationConfiguration(NotificationConfiguration notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration;
return this;
}
public final void setNotificationConfiguration(NotificationConfiguration.BuilderImpl notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration != null ? notificationConfiguration.build() : null;
}
public final Collection getCacheSecurityGroups() {
return cacheSecurityGroups != null ? cacheSecurityGroups.stream().map(CacheSecurityGroupMembership::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder cacheSecurityGroups(Collection cacheSecurityGroups) {
this.cacheSecurityGroups = CacheSecurityGroupMembershipListCopier.copy(cacheSecurityGroups);
return this;
}
@Override
@SafeVarargs
public final Builder cacheSecurityGroups(CacheSecurityGroupMembership... cacheSecurityGroups) {
cacheSecurityGroups(Arrays.asList(cacheSecurityGroups));
return this;
}
@Override
@SafeVarargs
public final Builder cacheSecurityGroups(Consumer... cacheSecurityGroups) {
cacheSecurityGroups(Stream.of(cacheSecurityGroups)
.map(c -> CacheSecurityGroupMembership.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setCacheSecurityGroups(Collection cacheSecurityGroups) {
this.cacheSecurityGroups = CacheSecurityGroupMembershipListCopier.copyFromBuilder(cacheSecurityGroups);
}
public final CacheParameterGroupStatus.Builder getCacheParameterGroup() {
return cacheParameterGroup != null ? cacheParameterGroup.toBuilder() : null;
}
@Override
public final Builder cacheParameterGroup(CacheParameterGroupStatus cacheParameterGroup) {
this.cacheParameterGroup = cacheParameterGroup;
return this;
}
public final void setCacheParameterGroup(CacheParameterGroupStatus.BuilderImpl cacheParameterGroup) {
this.cacheParameterGroup = cacheParameterGroup != null ? cacheParameterGroup.build() : null;
}
public final String getCacheSubnetGroupName() {
return cacheSubnetGroupName;
}
@Override
public final Builder cacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
return this;
}
public final void setCacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
}
public final Collection getCacheNodes() {
return cacheNodes != null ? cacheNodes.stream().map(CacheNode::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder cacheNodes(Collection cacheNodes) {
this.cacheNodes = CacheNodeListCopier.copy(cacheNodes);
return this;
}
@Override
@SafeVarargs
public final Builder cacheNodes(CacheNode... cacheNodes) {
cacheNodes(Arrays.asList(cacheNodes));
return this;
}
@Override
@SafeVarargs
public final Builder cacheNodes(Consumer... cacheNodes) {
cacheNodes(Stream.of(cacheNodes).map(c -> CacheNode.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setCacheNodes(Collection cacheNodes) {
this.cacheNodes = CacheNodeListCopier.copyFromBuilder(cacheNodes);
}
public final Boolean getAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
@Override
public final Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
public final void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
public final Collection getSecurityGroups() {
return securityGroups != null ? securityGroups.stream().map(SecurityGroupMembership::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder securityGroups(Collection securityGroups) {
this.securityGroups = SecurityGroupMembershipListCopier.copy(securityGroups);
return this;
}
@Override
@SafeVarargs
public final Builder securityGroups(SecurityGroupMembership... securityGroups) {
securityGroups(Arrays.asList(securityGroups));
return this;
}
@Override
@SafeVarargs
public final Builder securityGroups(Consumer... securityGroups) {
securityGroups(Stream.of(securityGroups).map(c -> SecurityGroupMembership.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setSecurityGroups(Collection securityGroups) {
this.securityGroups = SecurityGroupMembershipListCopier.copyFromBuilder(securityGroups);
}
public final String getReplicationGroupId() {
return replicationGroupId;
}
@Override
public final Builder replicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
return this;
}
public final void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
public final Integer getSnapshotRetentionLimit() {
return snapshotRetentionLimit;
}
@Override
public final Builder snapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
public final void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
public final String getSnapshotWindow() {
return snapshotWindow;
}
@Override
public final Builder snapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
return this;
}
public final void setSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
}
public final Boolean getAuthTokenEnabled() {
return authTokenEnabled;
}
@Override
public final Builder authTokenEnabled(Boolean authTokenEnabled) {
this.authTokenEnabled = authTokenEnabled;
return this;
}
public final void setAuthTokenEnabled(Boolean authTokenEnabled) {
this.authTokenEnabled = authTokenEnabled;
}
public final Boolean getTransitEncryptionEnabled() {
return transitEncryptionEnabled;
}
@Override
public final Builder transitEncryptionEnabled(Boolean transitEncryptionEnabled) {
this.transitEncryptionEnabled = transitEncryptionEnabled;
return this;
}
public final void setTransitEncryptionEnabled(Boolean transitEncryptionEnabled) {
this.transitEncryptionEnabled = transitEncryptionEnabled;
}
public final Boolean getAtRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
@Override
public final Builder atRestEncryptionEnabled(Boolean atRestEncryptionEnabled) {
this.atRestEncryptionEnabled = atRestEncryptionEnabled;
return this;
}
public final void setAtRestEncryptionEnabled(Boolean atRestEncryptionEnabled) {
this.atRestEncryptionEnabled = atRestEncryptionEnabled;
}
@Override
public CacheCluster build() {
return new CacheCluster(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}