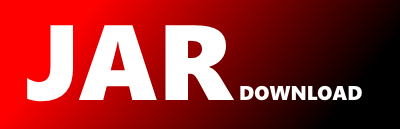
software.amazon.awssdk.services.elasticache.model.CreateCacheClusterRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a CreateCacheCluster operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateCacheClusterRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::cacheClusterId)).setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::replicationGroupId)).setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField AZ_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::azModeAsString)).setter(setter(Builder::azMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AZMode").build()).build();
private static final SdkField PREFERRED_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::preferredAvailabilityZone))
.setter(setter(Builder::preferredAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZone").build())
.build();
private static final SdkField> PREFERRED_AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateCacheClusterRequest::preferredAvailabilityZones))
.setter(setter(Builder::preferredAvailabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredAvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName("PreferredAvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PreferredAvailabilityZone").build()).build()).build()).build();
private static final SdkField NUM_CACHE_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CreateCacheClusterRequest::numCacheNodes)).setter(setter(Builder::numCacheNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumCacheNodes").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField CACHE_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::cacheParameterGroupName)).setter(setter(Builder::cacheParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheParameterGroupName").build())
.build();
private static final SdkField CACHE_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::cacheSubnetGroupName)).setter(setter(Builder::cacheSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSubnetGroupName").build())
.build();
private static final SdkField> CACHE_SECURITY_GROUP_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateCacheClusterRequest::cacheSecurityGroupNames))
.setter(setter(Builder::cacheSecurityGroupNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheSecurityGroupNames").build(),
ListTrait
.builder()
.memberLocationName("CacheSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CacheSecurityGroupName").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateCacheClusterRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("SecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SecurityGroupId").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateCacheClusterRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final SdkField> SNAPSHOT_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateCacheClusterRequest::snapshotArns))
.setter(setter(Builder::snapshotArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotArns").build(),
ListTrait
.builder()
.memberLocationName("SnapshotArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SnapshotArn").build()).build()).build()).build();
private static final SdkField SNAPSHOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::snapshotName)).setter(setter(Builder::snapshotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotName").build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CreateCacheClusterRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField NOTIFICATION_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::notificationTopicArn)).setter(setter(Builder::notificationTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicArn").build())
.build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(CreateCacheClusterRequest::autoMinorVersionUpgrade)).setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CreateCacheClusterRequest::snapshotRetentionLimit)).setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::snapshotWindow)).setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField AUTH_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateCacheClusterRequest::authToken)).setter(setter(Builder::authToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CACHE_CLUSTER_ID_FIELD,
REPLICATION_GROUP_ID_FIELD, AZ_MODE_FIELD, PREFERRED_AVAILABILITY_ZONE_FIELD, PREFERRED_AVAILABILITY_ZONES_FIELD,
NUM_CACHE_NODES_FIELD, CACHE_NODE_TYPE_FIELD, ENGINE_FIELD, ENGINE_VERSION_FIELD, CACHE_PARAMETER_GROUP_NAME_FIELD,
CACHE_SUBNET_GROUP_NAME_FIELD, CACHE_SECURITY_GROUP_NAMES_FIELD, SECURITY_GROUP_IDS_FIELD, TAGS_FIELD,
SNAPSHOT_ARNS_FIELD, SNAPSHOT_NAME_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, PORT_FIELD,
NOTIFICATION_TOPIC_ARN_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, SNAPSHOT_RETENTION_LIMIT_FIELD,
SNAPSHOT_WINDOW_FIELD, AUTH_TOKEN_FIELD));
private final String cacheClusterId;
private final String replicationGroupId;
private final String azMode;
private final String preferredAvailabilityZone;
private final List preferredAvailabilityZones;
private final Integer numCacheNodes;
private final String cacheNodeType;
private final String engine;
private final String engineVersion;
private final String cacheParameterGroupName;
private final String cacheSubnetGroupName;
private final List cacheSecurityGroupNames;
private final List securityGroupIds;
private final List tags;
private final List snapshotArns;
private final String snapshotName;
private final String preferredMaintenanceWindow;
private final Integer port;
private final String notificationTopicArn;
private final Boolean autoMinorVersionUpgrade;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final String authToken;
private CreateCacheClusterRequest(BuilderImpl builder) {
super(builder);
this.cacheClusterId = builder.cacheClusterId;
this.replicationGroupId = builder.replicationGroupId;
this.azMode = builder.azMode;
this.preferredAvailabilityZone = builder.preferredAvailabilityZone;
this.preferredAvailabilityZones = builder.preferredAvailabilityZones;
this.numCacheNodes = builder.numCacheNodes;
this.cacheNodeType = builder.cacheNodeType;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.cacheParameterGroupName = builder.cacheParameterGroupName;
this.cacheSubnetGroupName = builder.cacheSubnetGroupName;
this.cacheSecurityGroupNames = builder.cacheSecurityGroupNames;
this.securityGroupIds = builder.securityGroupIds;
this.tags = builder.tags;
this.snapshotArns = builder.snapshotArns;
this.snapshotName = builder.snapshotName;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.port = builder.port;
this.notificationTopicArn = builder.notificationTopicArn;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.authToken = builder.authToken;
}
/**
*
* The node group (shard) identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 20 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The node group (shard) identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 20 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public String cacheClusterId() {
return cacheClusterId;
}
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the cluster
* is added to the specified replication group as a read replica; otherwise, the cluster is a standalone primary
* that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the cluster is
* created in Availability Zones that provide the best spread of read replicas across Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The ID of the replication group to which this cluster should belong. If this parameter is specified, the
* cluster is added to the specified replication group as a read replica; otherwise, the cluster is a
* standalone primary that is not part of any replication group.
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across
* Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String replicationGroupId() {
return replicationGroupId;
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #azMode} will
* return {@link AZMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #azModeAsString}.
*
*
* @return Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or
* created across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
*/
public AZMode azMode() {
return AZMode.fromValue(azMode);
}
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created across
* multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #azMode} will
* return {@link AZMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #azModeAsString}.
*
*
* @return Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or
* created across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
*/
public String azModeAsString() {
return azMode;
}
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this Memcached cluster are placed in the preferred Availability Zone. If you want to
* create your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*
* @return The EC2 Availability Zone in which the cluster is created.
*
* All nodes belonging to this Memcached cluster are placed in the preferred Availability Zone. If you want
* to create your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*/
public String preferredAvailabilityZone() {
return preferredAvailabilityZone;
}
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability Zones
* that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead, or
* repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is
* not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*/
public List preferredAvailabilityZones() {
return preferredAvailabilityZones;
}
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be between 1
* and 20.
*
*
* If you need more than 20 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase Request
* form at http://aws.amazon.com/contact-us
* /elasticache-node-limit-request/.
*
*
* @return The initial number of cache nodes that the cluster has.
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 20.
*
*
* If you need more than 20 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon
* .com/contact-us/elasticache-node-limit-request/.
*/
public Integer numCacheNodes() {
return numCacheNodes;
}
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public String cacheNodeType() {
return cacheNodeType;
}
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*
* @return The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
*/
public String engine() {
return engine;
}
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine versions,
* use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting a
* Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you want to use an
* earlier engine version, you must delete the existing cluster or replication group and create it anew with the
* earlier engine version.
*
*
* @return The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster or replication group and
* create it anew with the earlier engine version.
*/
public String engineVersion() {
return engineVersion;
}
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*
* @return The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*/
public String cacheParameterGroupName() {
return cacheParameterGroupName;
}
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @return The name of the subnet group to be used for the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*/
public String cacheSubnetGroupName() {
return cacheSubnetGroupName;
}
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
*/
public List cacheSecurityGroupNames() {
return cacheSecurityGroupNames;
}
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*/
public List securityGroupIds() {
return securityGroupIds;
}
/**
*
* A list of cost allocation tags to be added to this resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of cost allocation tags to be added to this resource.
*/
public List tags() {
return tags;
}
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon S3
* object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The
* Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*/
public List snapshotArns() {
return snapshotArns;
}
/**
*
* The name of a Redis snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The name of a Redis snapshot from which to restore data into the new node group (shard). The snapshot
* status changes to restoring
while the new node group (shard) is being created.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String snapshotName() {
return snapshotName;
}
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
* Valid values for ddd
are:
*
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range
* in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @return Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period. Valid values for ddd
are:
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as
* a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*/
public String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*
* @return The port number on which each of the cache nodes accepts connections.
*/
public Integer port() {
return port;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are
* sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*/
public String notificationTopicArn() {
return notificationTopicArn;
}
/**
*
* This parameter is currently disabled.
*
*
* @return This parameter is currently disabled.
*/
public Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if you
* set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*
* @return The number of days for which ElastiCache retains automatic snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before
* being deleted.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*/
public Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String snapshotWindow() {
return snapshotWindow;
}
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@'.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @return Reserved parameter. The password used to access a password protected server.
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@'.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*/
public String authToken() {
return authToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(azModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(preferredAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(preferredAvailabilityZones());
hashCode = 31 * hashCode + Objects.hashCode(numCacheNodes());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(cacheParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cacheSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(cacheSecurityGroupNames());
hashCode = 31 * hashCode + Objects.hashCode(securityGroupIds());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(snapshotArns());
hashCode = 31 * hashCode + Objects.hashCode(snapshotName());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(authToken());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateCacheClusterRequest)) {
return false;
}
CreateCacheClusterRequest other = (CreateCacheClusterRequest) obj;
return Objects.equals(cacheClusterId(), other.cacheClusterId())
&& Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(azModeAsString(), other.azModeAsString())
&& Objects.equals(preferredAvailabilityZone(), other.preferredAvailabilityZone())
&& Objects.equals(preferredAvailabilityZones(), other.preferredAvailabilityZones())
&& Objects.equals(numCacheNodes(), other.numCacheNodes())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(cacheParameterGroupName(), other.cacheParameterGroupName())
&& Objects.equals(cacheSubnetGroupName(), other.cacheSubnetGroupName())
&& Objects.equals(cacheSecurityGroupNames(), other.cacheSecurityGroupNames())
&& Objects.equals(securityGroupIds(), other.securityGroupIds()) && Objects.equals(tags(), other.tags())
&& Objects.equals(snapshotArns(), other.snapshotArns()) && Objects.equals(snapshotName(), other.snapshotName())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(port(), other.port()) && Objects.equals(notificationTopicArn(), other.notificationTopicArn())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow()) && Objects.equals(authToken(), other.authToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateCacheClusterRequest").add("CacheClusterId", cacheClusterId())
.add("ReplicationGroupId", replicationGroupId()).add("AZMode", azModeAsString())
.add("PreferredAvailabilityZone", preferredAvailabilityZone())
.add("PreferredAvailabilityZones", preferredAvailabilityZones()).add("NumCacheNodes", numCacheNodes())
.add("CacheNodeType", cacheNodeType()).add("Engine", engine()).add("EngineVersion", engineVersion())
.add("CacheParameterGroupName", cacheParameterGroupName()).add("CacheSubnetGroupName", cacheSubnetGroupName())
.add("CacheSecurityGroupNames", cacheSecurityGroupNames()).add("SecurityGroupIds", securityGroupIds())
.add("Tags", tags()).add("SnapshotArns", snapshotArns()).add("SnapshotName", snapshotName())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("Port", port())
.add("NotificationTopicArn", notificationTopicArn()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("SnapshotRetentionLimit", snapshotRetentionLimit()).add("SnapshotWindow", snapshotWindow())
.add("AuthToken", authToken()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "AZMode":
return Optional.ofNullable(clazz.cast(azModeAsString()));
case "PreferredAvailabilityZone":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZone()));
case "PreferredAvailabilityZones":
return Optional.ofNullable(clazz.cast(preferredAvailabilityZones()));
case "NumCacheNodes":
return Optional.ofNullable(clazz.cast(numCacheNodes()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "CacheParameterGroupName":
return Optional.ofNullable(clazz.cast(cacheParameterGroupName()));
case "CacheSubnetGroupName":
return Optional.ofNullable(clazz.cast(cacheSubnetGroupName()));
case "CacheSecurityGroupNames":
return Optional.ofNullable(clazz.cast(cacheSecurityGroupNames()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "SnapshotArns":
return Optional.ofNullable(clazz.cast(snapshotArns()));
case "SnapshotName":
return Optional.ofNullable(clazz.cast(snapshotName()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "NotificationTopicArn":
return Optional.ofNullable(clazz.cast(notificationTopicArn()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "AuthToken":
return Optional.ofNullable(clazz.cast(authToken()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 20 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheClusterId(String cacheClusterId);
/**
*
* The ID of the replication group to which this cluster should belong. If this parameter is specified, the
* cluster is added to the specified replication group as a read replica; otherwise, the cluster is a standalone
* primary that is not part of any replication group.
*
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across Availability
* Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param replicationGroupId
* The ID of the replication group to which this cluster should belong. If this parameter is specified,
* the cluster is added to the specified replication group as a read replica; otherwise, the cluster is a
* standalone primary that is not part of any replication group.
*
* If the specified replication group is Multi-AZ enabled and the Availability Zone is not specified, the
* cluster is created in Availability Zones that provide the best spread of read replicas across
* Availability Zones.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationGroupId(String replicationGroupId);
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param azMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or
* created across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
Builder azMode(String azMode);
/**
*
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or created
* across multiple Availability Zones in the cluster's region.
*
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache assumes
* single-az
mode.
*
*
* @param azMode
* Specifies whether the nodes in this Memcached cluster are created in a single Availability Zone or
* created across multiple Availability Zones in the cluster's region.
*
* This parameter is only supported for Memcached clusters.
*
*
* If the AZMode
and PreferredAvailabilityZones
are not specified, ElastiCache
* assumes single-az
mode.
* @see AZMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see AZMode
*/
Builder azMode(AZMode azMode);
/**
*
* The EC2 Availability Zone in which the cluster is created.
*
*
* All nodes belonging to this Memcached cluster are placed in the preferred Availability Zone. If you want to
* create your nodes across multiple Availability Zones, use PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
*
*
* @param preferredAvailabilityZone
* The EC2 Availability Zone in which the cluster is created.
*
* All nodes belonging to this Memcached cluster are placed in the preferred Availability Zone. If you
* want to create your nodes across multiple Availability Zones, use
* PreferredAvailabilityZones
.
*
*
* Default: System chosen Availability Zone.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredAvailabilityZone(String preferredAvailabilityZone);
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead,
* or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* @param preferredAvailabilityZones
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list
* is not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in
* Availability Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredAvailabilityZones(Collection preferredAvailabilityZones);
/**
*
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list is not
* important.
*
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in Availability
* Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
instead,
* or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
*
*
* @param preferredAvailabilityZones
* A list of the Availability Zones in which cache nodes are created. The order of the zones in the list
* is not important.
*
* This option is only supported on Memcached.
*
*
*
* If you are creating your cluster in an Amazon VPC (recommended) you can only locate nodes in
* Availability Zones that are associated with the subnets in the selected subnet group.
*
*
* The number of Availability Zones listed must equal the value of NumCacheNodes
.
*
*
*
* If you want all the nodes in the same Availability Zone, use PreferredAvailabilityZone
* instead, or repeat the Availability Zone multiple times in the list.
*
*
* Default: System chosen Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredAvailabilityZones(String... preferredAvailabilityZones);
/**
*
* The initial number of cache nodes that the cluster has.
*
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be between
* 1 and 20.
*
*
* If you need more than 20 nodes for your Memcached cluster, please fill out the ElastiCache Limit Increase
* Request form at http://aws.amazon.com
* /contact-us/elasticache-node-limit-request/.
*
*
* @param numCacheNodes
* The initial number of cache nodes that the cluster has.
*
* For clusters running Redis, this value must be 1. For clusters running Memcached, this value must be
* between 1 and 20.
*
*
* If you need more than 20 nodes for your Memcached cluster, please fill out the ElastiCache Limit
* Increase Request form at http
* ://aws.amazon.com/contact-us/elasticache-node-limit-request/.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder numCacheNodes(Integer numCacheNodes);
/**
*
* The compute and memory capacity of the nodes in the node group (shard).
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param cacheNodeType
* The compute and memory capacity of the nodes in the node group (shard).
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation
* types provide more memory and computational power at lower cost when compared to their equivalent
* previous generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheNodeType(String cacheNodeType);
/**
*
* The name of the cache engine to be used for this cluster.
*
*
* Valid values for this parameter are: memcached
| redis
*
*
* @param engine
* The name of the cache engine to be used for this cluster.
*
* Valid values for this parameter are: memcached
| redis
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder engine(String engine);
/**
*
* The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If you
* want to use an earlier engine version, you must delete the existing cluster or replication group and create
* it anew with the earlier engine version.
*
*
* @param engineVersion
* The version number of the cache engine to be used for this cluster. To view the supported cache engine
* versions, use the DescribeCacheEngineVersions operation.
*
* Important: You can upgrade to a newer engine version (see Selecting a Cache Engine and Version), but you cannot downgrade to an earlier engine version. If
* you want to use an earlier engine version, you must delete the existing cluster or replication group
* and create it anew with the earlier engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder engineVersion(String engineVersion);
/**
*
* The name of the parameter group to associate with this cluster. If this argument is omitted, the default
* parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
*
*
* @param cacheParameterGroupName
* The name of the parameter group to associate with this cluster. If this argument is omitted, the
* default parameter group for the specified engine is used. You cannot use any parameter group which has
* cluster-enabled='yes'
when creating a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheParameterGroupName(String cacheParameterGroupName);
/**
*
* The name of the subnet group to be used for the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you start
* creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
*
*
* @param cacheSubnetGroupName
* The name of the subnet group to be used for the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
*
* If you're going to launch your cluster in an Amazon VPC, you need to create a subnet group before you
* start creating a cluster. For more information, see Subnets and Subnet
* Groups.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSubnetGroupName(String cacheSubnetGroupName);
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSecurityGroupNames(Collection cacheSecurityGroupNames);
/**
*
* A list of security group names to associate with this cluster.
*
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud (Amazon
* VPC).
*
*
* @param cacheSecurityGroupNames
* A list of security group names to associate with this cluster.
*
* Use this parameter only when you are creating a cluster outside of an Amazon Virtual Private Cloud
* (Amazon VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cacheSecurityGroupNames(String... cacheSecurityGroupNames);
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroupIds(Collection securityGroupIds);
/**
*
* One or more VPC security groups associated with the cluster.
*
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param securityGroupIds
* One or more VPC security groups associated with the cluster.
*
* Use this parameter only when you are creating a cluster in an Amazon Virtual Private Cloud (Amazon
* VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder securityGroupIds(String... securityGroupIds);
/**
*
* A list of cost allocation tags to be added to this resource.
*
*
* @param tags
* A list of cost allocation tags to be added to this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* A list of cost allocation tags to be added to this resource.
*
*
* @param tags
* A list of cost allocation tags to be added to this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A list of cost allocation tags to be added to this resource.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon
* S3 object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* @param snapshotArns
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard).
* The Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotArns(Collection snapshotArns);
/**
*
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis RDB
* snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard). The Amazon
* S3 object name in the ARN cannot contain any commas.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
*
*
* @param snapshotArns
* A single-element string list containing an Amazon Resource Name (ARN) that uniquely identifies a Redis
* RDB snapshot file stored in Amazon S3. The snapshot file is used to populate the node group (shard).
* The Amazon S3 object name in the ARN cannot contain any commas.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Example of an Amazon S3 ARN: arn:aws:s3:::my_bucket/snapshot1.rdb
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotArns(String... snapshotArns);
/**
*
* The name of a Redis snapshot from which to restore data into the new node group (shard). The snapshot status
* changes to restoring
while the new node group (shard) is being created.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotName
* The name of a Redis snapshot from which to restore data into the new node group (shard). The snapshot
* status changes to restoring
while the new node group (shard) is being created.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotName(String snapshotName);
/**
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period. Valid values for ddd
are:
*
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
*
*
* @param preferredMaintenanceWindow
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified
* as a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a
* 60 minute period. Valid values for ddd
are:
*
* Specifies the weekly time range during which maintenance on the cluster is performed. It is specified
* as a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a
* 60 minute period.
*
*
* Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:23:00-mon:01:30
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredMaintenanceWindow(String preferredMaintenanceWindow);
/**
*
* The port number on which each of the cache nodes accepts connections.
*
*
* @param port
* The port number on which each of the cache nodes accepts connections.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder port(Integer port);
/**
*
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications
* are sent.
*
*
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
*
*
* @param notificationTopicArn
* The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which
* notifications are sent.
*
* The Amazon SNS topic owner must be the same as the cluster owner.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationTopicArn(String notificationTopicArn);
/**
*
* This parameter is currently disabled.
*
*
* @param autoMinorVersionUpgrade
* This parameter is currently disabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade);
/**
*
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For example, if
* you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5 days before being
* deleted.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
*
*
* @param snapshotRetentionLimit
* The number of days for which ElastiCache retains automatic snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot taken today is retained for 5
* days before being deleted.
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* Default: 0 (i.e., automatic backups are disabled for this cache cluster).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotRetentionLimit(Integer snapshotRetentionLimit);
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @param snapshotWindow
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node
* group (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotWindow(String snapshotWindow);
/**
*
* Reserved parameter. The password used to access a password protected server.
*
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@'.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
*
*
* @param authToken
* Reserved parameter. The password used to access a password protected server.
*
* Password constraints:
*
*
* -
*
* Must be only printable ASCII characters.
*
*
* -
*
* Must be at least 16 characters and no more than 128 characters in length.
*
*
* -
*
* Cannot contain any of the following characters: '/', '"', or '@'.
*
*
*
*
* For more information, see AUTH password at
* http://redis.io/commands/AUTH.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder authToken(String authToken);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ElastiCacheRequest.BuilderImpl implements Builder {
private String cacheClusterId;
private String replicationGroupId;
private String azMode;
private String preferredAvailabilityZone;
private List preferredAvailabilityZones = DefaultSdkAutoConstructList.getInstance();
private Integer numCacheNodes;
private String cacheNodeType;
private String engine;
private String engineVersion;
private String cacheParameterGroupName;
private String cacheSubnetGroupName;
private List cacheSecurityGroupNames = DefaultSdkAutoConstructList.getInstance();
private List securityGroupIds = DefaultSdkAutoConstructList.getInstance();
private List tags = DefaultSdkAutoConstructList.getInstance();
private List snapshotArns = DefaultSdkAutoConstructList.getInstance();
private String snapshotName;
private String preferredMaintenanceWindow;
private Integer port;
private String notificationTopicArn;
private Boolean autoMinorVersionUpgrade;
private Integer snapshotRetentionLimit;
private String snapshotWindow;
private String authToken;
private BuilderImpl() {
}
private BuilderImpl(CreateCacheClusterRequest model) {
super(model);
cacheClusterId(model.cacheClusterId);
replicationGroupId(model.replicationGroupId);
azMode(model.azMode);
preferredAvailabilityZone(model.preferredAvailabilityZone);
preferredAvailabilityZones(model.preferredAvailabilityZones);
numCacheNodes(model.numCacheNodes);
cacheNodeType(model.cacheNodeType);
engine(model.engine);
engineVersion(model.engineVersion);
cacheParameterGroupName(model.cacheParameterGroupName);
cacheSubnetGroupName(model.cacheSubnetGroupName);
cacheSecurityGroupNames(model.cacheSecurityGroupNames);
securityGroupIds(model.securityGroupIds);
tags(model.tags);
snapshotArns(model.snapshotArns);
snapshotName(model.snapshotName);
preferredMaintenanceWindow(model.preferredMaintenanceWindow);
port(model.port);
notificationTopicArn(model.notificationTopicArn);
autoMinorVersionUpgrade(model.autoMinorVersionUpgrade);
snapshotRetentionLimit(model.snapshotRetentionLimit);
snapshotWindow(model.snapshotWindow);
authToken(model.authToken);
}
public final String getCacheClusterId() {
return cacheClusterId;
}
@Override
public final Builder cacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
return this;
}
public final void setCacheClusterId(String cacheClusterId) {
this.cacheClusterId = cacheClusterId;
}
public final String getReplicationGroupId() {
return replicationGroupId;
}
@Override
public final Builder replicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
return this;
}
public final void setReplicationGroupId(String replicationGroupId) {
this.replicationGroupId = replicationGroupId;
}
public final String getAzModeAsString() {
return azMode;
}
@Override
public final Builder azMode(String azMode) {
this.azMode = azMode;
return this;
}
@Override
public final Builder azMode(AZMode azMode) {
this.azMode(azMode.toString());
return this;
}
public final void setAzMode(String azMode) {
this.azMode = azMode;
}
public final String getPreferredAvailabilityZone() {
return preferredAvailabilityZone;
}
@Override
public final Builder preferredAvailabilityZone(String preferredAvailabilityZone) {
this.preferredAvailabilityZone = preferredAvailabilityZone;
return this;
}
public final void setPreferredAvailabilityZone(String preferredAvailabilityZone) {
this.preferredAvailabilityZone = preferredAvailabilityZone;
}
public final Collection getPreferredAvailabilityZones() {
return preferredAvailabilityZones;
}
@Override
public final Builder preferredAvailabilityZones(Collection preferredAvailabilityZones) {
this.preferredAvailabilityZones = PreferredAvailabilityZoneListCopier.copy(preferredAvailabilityZones);
return this;
}
@Override
@SafeVarargs
public final Builder preferredAvailabilityZones(String... preferredAvailabilityZones) {
preferredAvailabilityZones(Arrays.asList(preferredAvailabilityZones));
return this;
}
public final void setPreferredAvailabilityZones(Collection preferredAvailabilityZones) {
this.preferredAvailabilityZones = PreferredAvailabilityZoneListCopier.copy(preferredAvailabilityZones);
}
public final Integer getNumCacheNodes() {
return numCacheNodes;
}
@Override
public final Builder numCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
return this;
}
public final void setNumCacheNodes(Integer numCacheNodes) {
this.numCacheNodes = numCacheNodes;
}
public final String getCacheNodeType() {
return cacheNodeType;
}
@Override
public final Builder cacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
return this;
}
public final void setCacheNodeType(String cacheNodeType) {
this.cacheNodeType = cacheNodeType;
}
public final String getEngine() {
return engine;
}
@Override
public final Builder engine(String engine) {
this.engine = engine;
return this;
}
public final void setEngine(String engine) {
this.engine = engine;
}
public final String getEngineVersion() {
return engineVersion;
}
@Override
public final Builder engineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public final void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
public final String getCacheParameterGroupName() {
return cacheParameterGroupName;
}
@Override
public final Builder cacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
return this;
}
public final void setCacheParameterGroupName(String cacheParameterGroupName) {
this.cacheParameterGroupName = cacheParameterGroupName;
}
public final String getCacheSubnetGroupName() {
return cacheSubnetGroupName;
}
@Override
public final Builder cacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
return this;
}
public final void setCacheSubnetGroupName(String cacheSubnetGroupName) {
this.cacheSubnetGroupName = cacheSubnetGroupName;
}
public final Collection getCacheSecurityGroupNames() {
return cacheSecurityGroupNames;
}
@Override
public final Builder cacheSecurityGroupNames(Collection cacheSecurityGroupNames) {
this.cacheSecurityGroupNames = CacheSecurityGroupNameListCopier.copy(cacheSecurityGroupNames);
return this;
}
@Override
@SafeVarargs
public final Builder cacheSecurityGroupNames(String... cacheSecurityGroupNames) {
cacheSecurityGroupNames(Arrays.asList(cacheSecurityGroupNames));
return this;
}
public final void setCacheSecurityGroupNames(Collection cacheSecurityGroupNames) {
this.cacheSecurityGroupNames = CacheSecurityGroupNameListCopier.copy(cacheSecurityGroupNames);
}
public final Collection getSecurityGroupIds() {
return securityGroupIds;
}
@Override
public final Builder securityGroupIds(Collection securityGroupIds) {
this.securityGroupIds = SecurityGroupIdsListCopier.copy(securityGroupIds);
return this;
}
@Override
@SafeVarargs
public final Builder securityGroupIds(String... securityGroupIds) {
securityGroupIds(Arrays.asList(securityGroupIds));
return this;
}
public final void setSecurityGroupIds(Collection securityGroupIds) {
this.securityGroupIds = SecurityGroupIdsListCopier.copy(securityGroupIds);
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
public final Collection getSnapshotArns() {
return snapshotArns;
}
@Override
public final Builder snapshotArns(Collection snapshotArns) {
this.snapshotArns = SnapshotArnsListCopier.copy(snapshotArns);
return this;
}
@Override
@SafeVarargs
public final Builder snapshotArns(String... snapshotArns) {
snapshotArns(Arrays.asList(snapshotArns));
return this;
}
public final void setSnapshotArns(Collection snapshotArns) {
this.snapshotArns = SnapshotArnsListCopier.copy(snapshotArns);
}
public final String getSnapshotName() {
return snapshotName;
}
@Override
public final Builder snapshotName(String snapshotName) {
this.snapshotName = snapshotName;
return this;
}
public final void setSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
}
public final String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
@Override
public final Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
public final void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
public final Integer getPort() {
return port;
}
@Override
public final Builder port(Integer port) {
this.port = port;
return this;
}
public final void setPort(Integer port) {
this.port = port;
}
public final String getNotificationTopicArn() {
return notificationTopicArn;
}
@Override
public final Builder notificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
return this;
}
public final void setNotificationTopicArn(String notificationTopicArn) {
this.notificationTopicArn = notificationTopicArn;
}
public final Boolean getAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
@Override
public final Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
public final void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
public final Integer getSnapshotRetentionLimit() {
return snapshotRetentionLimit;
}
@Override
public final Builder snapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
public final void setSnapshotRetentionLimit(Integer snapshotRetentionLimit) {
this.snapshotRetentionLimit = snapshotRetentionLimit;
}
public final String getSnapshotWindow() {
return snapshotWindow;
}
@Override
public final Builder snapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
return this;
}
public final void setSnapshotWindow(String snapshotWindow) {
this.snapshotWindow = snapshotWindow;
}
public final String getAuthToken() {
return authToken;
}
@Override
public final Builder authToken(String authToken) {
this.authToken = authToken;
return this;
}
public final void setAuthToken(String authToken) {
this.authToken = authToken;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateCacheClusterRequest build() {
return new CreateCacheClusterRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}