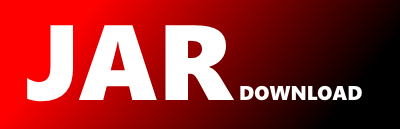
software.amazon.awssdk.services.elasticache.model.DescribeReservedCacheNodesOfferingsRequest Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a DescribeReservedCacheNodesOfferings
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeReservedCacheNodesOfferingsRequest extends ElastiCacheRequest implements
ToCopyableBuilder {
private static final SdkField RESERVED_CACHE_NODES_OFFERING_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::reservedCacheNodesOfferingId))
.setter(setter(Builder::reservedCacheNodesOfferingId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReservedCacheNodesOfferingId")
.build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField DURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::duration)).setter(setter(Builder::duration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Duration").build()).build();
private static final SdkField PRODUCT_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::productDescription))
.setter(setter(Builder::productDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProductDescription").build())
.build();
private static final SdkField OFFERING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::offeringType)).setter(setter(Builder::offeringType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OfferingType").build()).build();
private static final SdkField MAX_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::maxRecords)).setter(setter(Builder::maxRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxRecords").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeReservedCacheNodesOfferingsRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
RESERVED_CACHE_NODES_OFFERING_ID_FIELD, CACHE_NODE_TYPE_FIELD, DURATION_FIELD, PRODUCT_DESCRIPTION_FIELD,
OFFERING_TYPE_FIELD, MAX_RECORDS_FIELD, MARKER_FIELD));
private final String reservedCacheNodesOfferingId;
private final String cacheNodeType;
private final String duration;
private final String productDescription;
private final String offeringType;
private final Integer maxRecords;
private final String marker;
private DescribeReservedCacheNodesOfferingsRequest(BuilderImpl builder) {
super(builder);
this.reservedCacheNodesOfferingId = builder.reservedCacheNodesOfferingId;
this.cacheNodeType = builder.cacheNodeType;
this.duration = builder.duration;
this.productDescription = builder.productDescription;
this.offeringType = builder.offeringType;
this.maxRecords = builder.maxRecords;
this.marker = builder.marker;
}
/**
*
* The offering identifier filter value. Use this parameter to show only the available offering that matches the
* specified reservation identifier.
*
*
* Example: 438012d3-4052-4cc7-b2e3-8d3372e0e706
*
*
* @return The offering identifier filter value. Use this parameter to show only the available offering that matches
* the specified reservation identifier.
*
* Example: 438012d3-4052-4cc7-b2e3-8d3372e0e706
*/
public String reservedCacheNodesOfferingId() {
return reservedCacheNodesOfferingId;
}
/**
*
* The cache node type filter value. Use this parameter to show only the available offerings matching the specified
* cache node type.
*
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types provide
* more memory and computational power at lower cost when compared to their equivalent previous generation
* counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
, cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
, cache.m3.xlarge
,
* cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
, cache.m4.2xlarge
,
* cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
, cache.m1.large
,
* cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
, cache.r3.2xlarge
,
* cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
, cache.r4.2xlarge
,
* cache.r4.4xlarge
, cache.r4.8xlarge
, cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
, cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return The cache node type filter value. Use this parameter to show only the available offerings matching the
* specified cache node type.
*
* The following node types are supported by ElastiCache. Generally speaking, the current generation types
* provide more memory and computational power at lower cost when compared to their equivalent previous
* generation counterparts.
*
*
* -
*
* General purpose:
*
*
* -
*
* Current generation:
*
*
* T2 node types: cache.t2.micro
, cache.t2.small
,
* cache.t2.medium
*
*
* M3 node types: cache.m3.medium
, cache.m3.large
,
* cache.m3.xlarge
, cache.m3.2xlarge
*
*
* M4 node types: cache.m4.large
, cache.m4.xlarge
,
* cache.m4.2xlarge
, cache.m4.4xlarge
, cache.m4.10xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* T1 node types: cache.t1.micro
*
*
* M1 node types: cache.m1.small
, cache.m1.medium
,
* cache.m1.large
, cache.m1.xlarge
*
*
*
*
* -
*
* Compute optimized:
*
*
* -
*
* Previous generation: (not recommended)
*
*
* C1 node types: cache.c1.xlarge
*
*
*
*
* -
*
* Memory optimized:
*
*
* -
*
* Current generation:
*
*
* R3 node types: cache.r3.large
, cache.r3.xlarge
,
* cache.r3.2xlarge
, cache.r3.4xlarge
, cache.r3.8xlarge
*
*
* R4 node types; cache.r4.large
, cache.r4.xlarge
,
* cache.r4.2xlarge
, cache.r4.4xlarge
, cache.r4.8xlarge
,
* cache.r4.16xlarge
*
*
* -
*
* Previous generation: (not recommended)
*
*
* M2 node types: cache.m2.xlarge
, cache.m2.2xlarge
,
* cache.m2.4xlarge
*
*
*
*
*
*
* Notes:
*
*
* -
*
* All T2 instances are created in an Amazon Virtual Private Cloud (Amazon VPC).
*
*
* -
*
* Redis (cluster mode disabled): Redis backup/restore is not supported on T1 and T2 instances.
*
*
* -
*
* Redis (cluster mode enabled): Backup/restore is not supported on T1 instances.
*
*
* -
*
* Redis Append-only files (AOF) functionality is not supported for T1 or T2 instances.
*
*
*
*
* For a complete listing of node types and specifications, see:
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public String cacheNodeType() {
return cacheNodeType;
}
/**
*
* Duration filter value, specified in years or seconds. Use this parameter to show only reservations for a given
* duration.
*
*
* Valid Values: 1 | 3 | 31536000 | 94608000
*
*
* @return Duration filter value, specified in years or seconds. Use this parameter to show only reservations for a
* given duration.
*
* Valid Values: 1 | 3 | 31536000 | 94608000
*/
public String duration() {
return duration;
}
/**
*
* The product description filter value. Use this parameter to show only the available offerings matching the
* specified product description.
*
*
* @return The product description filter value. Use this parameter to show only the available offerings matching
* the specified product description.
*/
public String productDescription() {
return productDescription;
}
/**
*
* The offering type filter value. Use this parameter to show only the available offerings matching the specified
* offering type.
*
*
* Valid Values: "Light Utilization"|"Medium Utilization"|"Heavy Utilization"
*
*
* @return The offering type filter value. Use this parameter to show only the available offerings matching the
* specified offering type.
*
* Valid Values: "Light Utilization"|"Medium Utilization"|"Heavy Utilization"
*/
public String offeringType() {
return offeringType;
}
/**
*
* The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can be
* retrieved.
*
*
* Default: 100
*
*
* Constraints: minimum 20; maximum 100.
*
*
* @return The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a marker is included in the response so that the remaining results can be
* retrieved.
*
* Default: 100
*
*
* Constraints: minimum 20; maximum 100.
*/
public Integer maxRecords() {
return maxRecords;
}
/**
*
* An optional marker returned from a prior request. Use this marker for pagination of results from this operation.
* If this parameter is specified, the response includes only records beyond the marker, up to the value specified
* by MaxRecords
.
*
*
* @return An optional marker returned from a prior request. Use this marker for pagination of results from this
* operation. If this parameter is specified, the response includes only records beyond the marker, up to
* the value specified by MaxRecords
.
*/
public String marker() {
return marker;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(reservedCacheNodesOfferingId());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(duration());
hashCode = 31 * hashCode + Objects.hashCode(productDescription());
hashCode = 31 * hashCode + Objects.hashCode(offeringType());
hashCode = 31 * hashCode + Objects.hashCode(maxRecords());
hashCode = 31 * hashCode + Objects.hashCode(marker());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeReservedCacheNodesOfferingsRequest)) {
return false;
}
DescribeReservedCacheNodesOfferingsRequest other = (DescribeReservedCacheNodesOfferingsRequest) obj;
return Objects.equals(reservedCacheNodesOfferingId(), other.reservedCacheNodesOfferingId())
&& Objects.equals(cacheNodeType(), other.cacheNodeType()) && Objects.equals(duration(), other.duration())
&& Objects.equals(productDescription(), other.productDescription())
&& Objects.equals(offeringType(), other.offeringType()) && Objects.equals(maxRecords(), other.maxRecords())
&& Objects.equals(marker(), other.marker());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DescribeReservedCacheNodesOfferingsRequest")
.add("ReservedCacheNodesOfferingId", reservedCacheNodesOfferingId()).add("CacheNodeType", cacheNodeType())
.add("Duration", duration()).add("ProductDescription", productDescription()).add("OfferingType", offeringType())
.add("MaxRecords", maxRecords()).add("Marker", marker()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReservedCacheNodesOfferingId":
return Optional.ofNullable(clazz.cast(reservedCacheNodesOfferingId()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "Duration":
return Optional.ofNullable(clazz.cast(duration()));
case "ProductDescription":
return Optional.ofNullable(clazz.cast(productDescription()));
case "OfferingType":
return Optional.ofNullable(clazz.cast(offeringType()));
case "MaxRecords":
return Optional.ofNullable(clazz.cast(maxRecords()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function