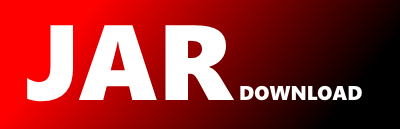
software.amazon.awssdk.services.elasticache.model.NodeGroupMemberUpdateStatus Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The status of the service update on the node group member
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NodeGroupMemberUpdateStatus implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CACHE_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(NodeGroupMemberUpdateStatus::cacheClusterId)).setter(setter(Builder::cacheClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheClusterId").build()).build();
private static final SdkField CACHE_NODE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(NodeGroupMemberUpdateStatus::cacheNodeId)).setter(setter(Builder::cacheNodeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeId").build()).build();
private static final SdkField NODE_UPDATE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateStatusAsString)).setter(setter(Builder::nodeUpdateStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateStatus").build()).build();
private static final SdkField NODE_DELETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(NodeGroupMemberUpdateStatus::nodeDeletionDate)).setter(setter(Builder::nodeDeletionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeDeletionDate").build()).build();
private static final SdkField NODE_UPDATE_START_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateStartDate)).setter(setter(Builder::nodeUpdateStartDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateStartDate").build())
.build();
private static final SdkField NODE_UPDATE_END_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateEndDate)).setter(setter(Builder::nodeUpdateEndDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateEndDate").build()).build();
private static final SdkField NODE_UPDATE_INITIATED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateInitiatedByAsString))
.setter(setter(Builder::nodeUpdateInitiatedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateInitiatedBy").build())
.build();
private static final SdkField NODE_UPDATE_INITIATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateInitiatedDate))
.setter(setter(Builder::nodeUpdateInitiatedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateInitiatedDate").build())
.build();
private static final SdkField NODE_UPDATE_STATUS_MODIFIED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.getter(getter(NodeGroupMemberUpdateStatus::nodeUpdateStatusModifiedDate))
.setter(setter(Builder::nodeUpdateStatusModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeUpdateStatusModifiedDate")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CACHE_CLUSTER_ID_FIELD,
CACHE_NODE_ID_FIELD, NODE_UPDATE_STATUS_FIELD, NODE_DELETION_DATE_FIELD, NODE_UPDATE_START_DATE_FIELD,
NODE_UPDATE_END_DATE_FIELD, NODE_UPDATE_INITIATED_BY_FIELD, NODE_UPDATE_INITIATED_DATE_FIELD,
NODE_UPDATE_STATUS_MODIFIED_DATE_FIELD));
private static final long serialVersionUID = 1L;
private final String cacheClusterId;
private final String cacheNodeId;
private final String nodeUpdateStatus;
private final Instant nodeDeletionDate;
private final Instant nodeUpdateStartDate;
private final Instant nodeUpdateEndDate;
private final String nodeUpdateInitiatedBy;
private final Instant nodeUpdateInitiatedDate;
private final Instant nodeUpdateStatusModifiedDate;
private NodeGroupMemberUpdateStatus(BuilderImpl builder) {
this.cacheClusterId = builder.cacheClusterId;
this.cacheNodeId = builder.cacheNodeId;
this.nodeUpdateStatus = builder.nodeUpdateStatus;
this.nodeDeletionDate = builder.nodeDeletionDate;
this.nodeUpdateStartDate = builder.nodeUpdateStartDate;
this.nodeUpdateEndDate = builder.nodeUpdateEndDate;
this.nodeUpdateInitiatedBy = builder.nodeUpdateInitiatedBy;
this.nodeUpdateInitiatedDate = builder.nodeUpdateInitiatedDate;
this.nodeUpdateStatusModifiedDate = builder.nodeUpdateStatusModifiedDate;
}
/**
*
* The cache cluster ID
*
*
* @return The cache cluster ID
*/
public String cacheClusterId() {
return cacheClusterId;
}
/**
*
* The node ID of the cache cluster
*
*
* @return The node ID of the cache cluster
*/
public String cacheNodeId() {
return cacheNodeId;
}
/**
*
* The update status of the node
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nodeUpdateStatus}
* will return {@link NodeUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #nodeUpdateStatusAsString}.
*
*
* @return The update status of the node
* @see NodeUpdateStatus
*/
public NodeUpdateStatus nodeUpdateStatus() {
return NodeUpdateStatus.fromValue(nodeUpdateStatus);
}
/**
*
* The update status of the node
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nodeUpdateStatus}
* will return {@link NodeUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #nodeUpdateStatusAsString}.
*
*
* @return The update status of the node
* @see NodeUpdateStatus
*/
public String nodeUpdateStatusAsString() {
return nodeUpdateStatus;
}
/**
*
* The deletion date of the node
*
*
* @return The deletion date of the node
*/
public Instant nodeDeletionDate() {
return nodeDeletionDate;
}
/**
*
* The start date of the update for a node
*
*
* @return The start date of the update for a node
*/
public Instant nodeUpdateStartDate() {
return nodeUpdateStartDate;
}
/**
*
* The end date of the update for a node
*
*
* @return The end date of the update for a node
*/
public Instant nodeUpdateEndDate() {
return nodeUpdateEndDate;
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #nodeUpdateInitiatedBy} will return {@link NodeUpdateInitiatedBy#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #nodeUpdateInitiatedByAsString}.
*
*
* @return Reflects whether the update was initiated by the customer or automatically applied
* @see NodeUpdateInitiatedBy
*/
public NodeUpdateInitiatedBy nodeUpdateInitiatedBy() {
return NodeUpdateInitiatedBy.fromValue(nodeUpdateInitiatedBy);
}
/**
*
* Reflects whether the update was initiated by the customer or automatically applied
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #nodeUpdateInitiatedBy} will return {@link NodeUpdateInitiatedBy#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #nodeUpdateInitiatedByAsString}.
*
*
* @return Reflects whether the update was initiated by the customer or automatically applied
* @see NodeUpdateInitiatedBy
*/
public String nodeUpdateInitiatedByAsString() {
return nodeUpdateInitiatedBy;
}
/**
*
* The date when the update is triggered
*
*
* @return The date when the update is triggered
*/
public Instant nodeUpdateInitiatedDate() {
return nodeUpdateInitiatedDate;
}
/**
*
* The date when the NodeUpdateStatus was last modified
*
*
* @return The date when the NodeUpdateStatus was last modified
*/
public Instant nodeUpdateStatusModifiedDate() {
return nodeUpdateStatusModifiedDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cacheClusterId());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeId());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(nodeDeletionDate());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateStartDate());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateEndDate());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateInitiatedByAsString());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateInitiatedDate());
hashCode = 31 * hashCode + Objects.hashCode(nodeUpdateStatusModifiedDate());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NodeGroupMemberUpdateStatus)) {
return false;
}
NodeGroupMemberUpdateStatus other = (NodeGroupMemberUpdateStatus) obj;
return Objects.equals(cacheClusterId(), other.cacheClusterId()) && Objects.equals(cacheNodeId(), other.cacheNodeId())
&& Objects.equals(nodeUpdateStatusAsString(), other.nodeUpdateStatusAsString())
&& Objects.equals(nodeDeletionDate(), other.nodeDeletionDate())
&& Objects.equals(nodeUpdateStartDate(), other.nodeUpdateStartDate())
&& Objects.equals(nodeUpdateEndDate(), other.nodeUpdateEndDate())
&& Objects.equals(nodeUpdateInitiatedByAsString(), other.nodeUpdateInitiatedByAsString())
&& Objects.equals(nodeUpdateInitiatedDate(), other.nodeUpdateInitiatedDate())
&& Objects.equals(nodeUpdateStatusModifiedDate(), other.nodeUpdateStatusModifiedDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("NodeGroupMemberUpdateStatus").add("CacheClusterId", cacheClusterId())
.add("CacheNodeId", cacheNodeId()).add("NodeUpdateStatus", nodeUpdateStatusAsString())
.add("NodeDeletionDate", nodeDeletionDate()).add("NodeUpdateStartDate", nodeUpdateStartDate())
.add("NodeUpdateEndDate", nodeUpdateEndDate()).add("NodeUpdateInitiatedBy", nodeUpdateInitiatedByAsString())
.add("NodeUpdateInitiatedDate", nodeUpdateInitiatedDate())
.add("NodeUpdateStatusModifiedDate", nodeUpdateStatusModifiedDate()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CacheClusterId":
return Optional.ofNullable(clazz.cast(cacheClusterId()));
case "CacheNodeId":
return Optional.ofNullable(clazz.cast(cacheNodeId()));
case "NodeUpdateStatus":
return Optional.ofNullable(clazz.cast(nodeUpdateStatusAsString()));
case "NodeDeletionDate":
return Optional.ofNullable(clazz.cast(nodeDeletionDate()));
case "NodeUpdateStartDate":
return Optional.ofNullable(clazz.cast(nodeUpdateStartDate()));
case "NodeUpdateEndDate":
return Optional.ofNullable(clazz.cast(nodeUpdateEndDate()));
case "NodeUpdateInitiatedBy":
return Optional.ofNullable(clazz.cast(nodeUpdateInitiatedByAsString()));
case "NodeUpdateInitiatedDate":
return Optional.ofNullable(clazz.cast(nodeUpdateInitiatedDate()));
case "NodeUpdateStatusModifiedDate":
return Optional.ofNullable(clazz.cast(nodeUpdateStatusModifiedDate()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function