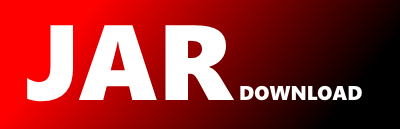
software.amazon.awssdk.services.elasticache.model.UpdateAction Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The status of the service update for a specific replication group
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateAction implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::replicationGroupId)).setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField SERVICE_UPDATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::serviceUpdateName)).setter(setter(Builder::serviceUpdateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateName").build()).build();
private static final SdkField SERVICE_UPDATE_RELEASE_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).getter(getter(UpdateAction::serviceUpdateReleaseDate))
.setter(setter(Builder::serviceUpdateReleaseDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateReleaseDate").build())
.build();
private static final SdkField SERVICE_UPDATE_SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::serviceUpdateSeverityAsString)).setter(setter(Builder::serviceUpdateSeverity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateSeverity").build())
.build();
private static final SdkField SERVICE_UPDATE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::serviceUpdateStatusAsString)).setter(setter(Builder::serviceUpdateStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateStatus").build())
.build();
private static final SdkField SERVICE_UPDATE_RECOMMENDED_APPLY_BY_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.getter(getter(UpdateAction::serviceUpdateRecommendedApplyByDate))
.setter(setter(Builder::serviceUpdateRecommendedApplyByDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ServiceUpdateRecommendedApplyByDate").build()).build();
private static final SdkField SERVICE_UPDATE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::serviceUpdateTypeAsString)).setter(setter(Builder::serviceUpdateType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceUpdateType").build()).build();
private static final SdkField UPDATE_ACTION_AVAILABLE_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).getter(getter(UpdateAction::updateActionAvailableDate))
.setter(setter(Builder::updateActionAvailableDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateActionAvailableDate").build())
.build();
private static final SdkField UPDATE_ACTION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::updateActionStatusAsString)).setter(setter(Builder::updateActionStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateActionStatus").build())
.build();
private static final SdkField NODES_UPDATED_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::nodesUpdated)).setter(setter(Builder::nodesUpdated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodesUpdated").build()).build();
private static final SdkField UPDATE_ACTION_STATUS_MODIFIED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.getter(getter(UpdateAction::updateActionStatusModifiedDate))
.setter(setter(Builder::updateActionStatusModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateActionStatusModifiedDate")
.build()).build();
private static final SdkField SLA_MET_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::slaMetAsString)).setter(setter(Builder::slaMet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SlaMet").build()).build();
private static final SdkField> NODE_GROUP_UPDATE_STATUS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateAction::nodeGroupUpdateStatus))
.setter(setter(Builder::nodeGroupUpdateStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroupUpdateStatus").build(),
ListTrait
.builder()
.memberLocationName("NodeGroupUpdateStatus")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NodeGroupUpdateStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroupUpdateStatus").build()).build()).build()).build();
private static final SdkField ESTIMATED_UPDATE_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateAction::estimatedUpdateTime)).setter(setter(Builder::estimatedUpdateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedUpdateTime").build())
.build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD, SERVICE_UPDATE_NAME_FIELD,
SERVICE_UPDATE_RELEASE_DATE_FIELD, SERVICE_UPDATE_SEVERITY_FIELD, SERVICE_UPDATE_STATUS_FIELD,
SERVICE_UPDATE_RECOMMENDED_APPLY_BY_DATE_FIELD, SERVICE_UPDATE_TYPE_FIELD,
UPDATE_ACTION_AVAILABLE_DATE_FIELD, UPDATE_ACTION_STATUS_FIELD, NODES_UPDATED_FIELD,
UPDATE_ACTION_STATUS_MODIFIED_DATE_FIELD, SLA_MET_FIELD, NODE_GROUP_UPDATE_STATUS_FIELD,
ESTIMATED_UPDATE_TIME_FIELD));
private static final long serialVersionUID = 1L;
private final String replicationGroupId;
private final String serviceUpdateName;
private final Instant serviceUpdateReleaseDate;
private final String serviceUpdateSeverity;
private final String serviceUpdateStatus;
private final Instant serviceUpdateRecommendedApplyByDate;
private final String serviceUpdateType;
private final Instant updateActionAvailableDate;
private final String updateActionStatus;
private final String nodesUpdated;
private final Instant updateActionStatusModifiedDate;
private final String slaMet;
private final List nodeGroupUpdateStatus;
private final String estimatedUpdateTime;
private UpdateAction(BuilderImpl builder) {
this.replicationGroupId = builder.replicationGroupId;
this.serviceUpdateName = builder.serviceUpdateName;
this.serviceUpdateReleaseDate = builder.serviceUpdateReleaseDate;
this.serviceUpdateSeverity = builder.serviceUpdateSeverity;
this.serviceUpdateStatus = builder.serviceUpdateStatus;
this.serviceUpdateRecommendedApplyByDate = builder.serviceUpdateRecommendedApplyByDate;
this.serviceUpdateType = builder.serviceUpdateType;
this.updateActionAvailableDate = builder.updateActionAvailableDate;
this.updateActionStatus = builder.updateActionStatus;
this.nodesUpdated = builder.nodesUpdated;
this.updateActionStatusModifiedDate = builder.updateActionStatusModifiedDate;
this.slaMet = builder.slaMet;
this.nodeGroupUpdateStatus = builder.nodeGroupUpdateStatus;
this.estimatedUpdateTime = builder.estimatedUpdateTime;
}
/**
*
* The ID of the replication group
*
*
* @return The ID of the replication group
*/
public String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The unique ID of the service update
*
*
* @return The unique ID of the service update
*/
public String serviceUpdateName() {
return serviceUpdateName;
}
/**
*
* The date the update is first available
*
*
* @return The date the update is first available
*/
public Instant serviceUpdateReleaseDate() {
return serviceUpdateReleaseDate;
}
/**
*
* The severity of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateSeverity} will return {@link ServiceUpdateSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateSeverityAsString}.
*
*
* @return The severity of the service update
* @see ServiceUpdateSeverity
*/
public ServiceUpdateSeverity serviceUpdateSeverity() {
return ServiceUpdateSeverity.fromValue(serviceUpdateSeverity);
}
/**
*
* The severity of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateSeverity} will return {@link ServiceUpdateSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateSeverityAsString}.
*
*
* @return The severity of the service update
* @see ServiceUpdateSeverity
*/
public String serviceUpdateSeverityAsString() {
return serviceUpdateSeverity;
}
/**
*
* The status of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateStatus} will return {@link ServiceUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateStatusAsString}.
*
*
* @return The status of the service update
* @see ServiceUpdateStatus
*/
public ServiceUpdateStatus serviceUpdateStatus() {
return ServiceUpdateStatus.fromValue(serviceUpdateStatus);
}
/**
*
* The status of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serviceUpdateStatus} will return {@link ServiceUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serviceUpdateStatusAsString}.
*
*
* @return The status of the service update
* @see ServiceUpdateStatus
*/
public String serviceUpdateStatusAsString() {
return serviceUpdateStatus;
}
/**
*
* The recommended date to apply the service update to ensure compliance. For information on compliance, see Self-Service Security Updates for Compliance.
*
*
* @return The recommended date to apply the service update to ensure compliance. For information on compliance, see
* Self-Service Security Updates for Compliance.
*/
public Instant serviceUpdateRecommendedApplyByDate() {
return serviceUpdateRecommendedApplyByDate;
}
/**
*
* Reflects the nature of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceUpdateType}
* will return {@link ServiceUpdateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceUpdateTypeAsString}.
*
*
* @return Reflects the nature of the service update
* @see ServiceUpdateType
*/
public ServiceUpdateType serviceUpdateType() {
return ServiceUpdateType.fromValue(serviceUpdateType);
}
/**
*
* Reflects the nature of the service update
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #serviceUpdateType}
* will return {@link ServiceUpdateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #serviceUpdateTypeAsString}.
*
*
* @return Reflects the nature of the service update
* @see ServiceUpdateType
*/
public String serviceUpdateTypeAsString() {
return serviceUpdateType;
}
/**
*
* The date that the service update is available to a replication group
*
*
* @return The date that the service update is available to a replication group
*/
public Instant updateActionAvailableDate() {
return updateActionAvailableDate;
}
/**
*
* The status of the update action
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #updateActionStatus} will return {@link UpdateActionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #updateActionStatusAsString}.
*
*
* @return The status of the update action
* @see UpdateActionStatus
*/
public UpdateActionStatus updateActionStatus() {
return UpdateActionStatus.fromValue(updateActionStatus);
}
/**
*
* The status of the update action
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #updateActionStatus} will return {@link UpdateActionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #updateActionStatusAsString}.
*
*
* @return The status of the update action
* @see UpdateActionStatus
*/
public String updateActionStatusAsString() {
return updateActionStatus;
}
/**
*
* The progress of the service update on the replication group
*
*
* @return The progress of the service update on the replication group
*/
public String nodesUpdated() {
return nodesUpdated;
}
/**
*
* The date when the UpdateActionStatus was last modified
*
*
* @return The date when the UpdateActionStatus was last modified
*/
public Instant updateActionStatusModifiedDate() {
return updateActionStatusModifiedDate;
}
/**
*
* If yes, all nodes in the replication group have been updated by the recommended apply-by date. If no, at least
* one node in the replication group have not been updated by the recommended apply-by date. If N/A, the replication
* group was created after the recommended apply-by date.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #slaMet} will
* return {@link SlaMet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #slaMetAsString}.
*
*
* @return If yes, all nodes in the replication group have been updated by the recommended apply-by date. If no, at
* least one node in the replication group have not been updated by the recommended apply-by date. If N/A,
* the replication group was created after the recommended apply-by date.
* @see SlaMet
*/
public SlaMet slaMet() {
return SlaMet.fromValue(slaMet);
}
/**
*
* If yes, all nodes in the replication group have been updated by the recommended apply-by date. If no, at least
* one node in the replication group have not been updated by the recommended apply-by date. If N/A, the replication
* group was created after the recommended apply-by date.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #slaMet} will
* return {@link SlaMet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #slaMetAsString}.
*
*
* @return If yes, all nodes in the replication group have been updated by the recommended apply-by date. If no, at
* least one node in the replication group have not been updated by the recommended apply-by date. If N/A,
* the replication group was created after the recommended apply-by date.
* @see SlaMet
*/
public String slaMetAsString() {
return slaMet;
}
/**
*
* The status of the service update on the node group
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The status of the service update on the node group
*/
public List nodeGroupUpdateStatus() {
return nodeGroupUpdateStatus;
}
/**
*
* The estimated length of time for the update to complete
*
*
* @return The estimated length of time for the update to complete
*/
public String estimatedUpdateTime() {
return estimatedUpdateTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateName());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateReleaseDate());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateSeverityAsString());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateRecommendedApplyByDate());
hashCode = 31 * hashCode + Objects.hashCode(serviceUpdateTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(updateActionAvailableDate());
hashCode = 31 * hashCode + Objects.hashCode(updateActionStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(nodesUpdated());
hashCode = 31 * hashCode + Objects.hashCode(updateActionStatusModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(slaMetAsString());
hashCode = 31 * hashCode + Objects.hashCode(nodeGroupUpdateStatus());
hashCode = 31 * hashCode + Objects.hashCode(estimatedUpdateTime());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateAction)) {
return false;
}
UpdateAction other = (UpdateAction) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(serviceUpdateName(), other.serviceUpdateName())
&& Objects.equals(serviceUpdateReleaseDate(), other.serviceUpdateReleaseDate())
&& Objects.equals(serviceUpdateSeverityAsString(), other.serviceUpdateSeverityAsString())
&& Objects.equals(serviceUpdateStatusAsString(), other.serviceUpdateStatusAsString())
&& Objects.equals(serviceUpdateRecommendedApplyByDate(), other.serviceUpdateRecommendedApplyByDate())
&& Objects.equals(serviceUpdateTypeAsString(), other.serviceUpdateTypeAsString())
&& Objects.equals(updateActionAvailableDate(), other.updateActionAvailableDate())
&& Objects.equals(updateActionStatusAsString(), other.updateActionStatusAsString())
&& Objects.equals(nodesUpdated(), other.nodesUpdated())
&& Objects.equals(updateActionStatusModifiedDate(), other.updateActionStatusModifiedDate())
&& Objects.equals(slaMetAsString(), other.slaMetAsString())
&& Objects.equals(nodeGroupUpdateStatus(), other.nodeGroupUpdateStatus())
&& Objects.equals(estimatedUpdateTime(), other.estimatedUpdateTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UpdateAction").add("ReplicationGroupId", replicationGroupId())
.add("ServiceUpdateName", serviceUpdateName()).add("ServiceUpdateReleaseDate", serviceUpdateReleaseDate())
.add("ServiceUpdateSeverity", serviceUpdateSeverityAsString())
.add("ServiceUpdateStatus", serviceUpdateStatusAsString())
.add("ServiceUpdateRecommendedApplyByDate", serviceUpdateRecommendedApplyByDate())
.add("ServiceUpdateType", serviceUpdateTypeAsString())
.add("UpdateActionAvailableDate", updateActionAvailableDate())
.add("UpdateActionStatus", updateActionStatusAsString()).add("NodesUpdated", nodesUpdated())
.add("UpdateActionStatusModifiedDate", updateActionStatusModifiedDate()).add("SlaMet", slaMetAsString())
.add("NodeGroupUpdateStatus", nodeGroupUpdateStatus()).add("EstimatedUpdateTime", estimatedUpdateTime()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "ServiceUpdateName":
return Optional.ofNullable(clazz.cast(serviceUpdateName()));
case "ServiceUpdateReleaseDate":
return Optional.ofNullable(clazz.cast(serviceUpdateReleaseDate()));
case "ServiceUpdateSeverity":
return Optional.ofNullable(clazz.cast(serviceUpdateSeverityAsString()));
case "ServiceUpdateStatus":
return Optional.ofNullable(clazz.cast(serviceUpdateStatusAsString()));
case "ServiceUpdateRecommendedApplyByDate":
return Optional.ofNullable(clazz.cast(serviceUpdateRecommendedApplyByDate()));
case "ServiceUpdateType":
return Optional.ofNullable(clazz.cast(serviceUpdateTypeAsString()));
case "UpdateActionAvailableDate":
return Optional.ofNullable(clazz.cast(updateActionAvailableDate()));
case "UpdateActionStatus":
return Optional.ofNullable(clazz.cast(updateActionStatusAsString()));
case "NodesUpdated":
return Optional.ofNullable(clazz.cast(nodesUpdated()));
case "UpdateActionStatusModifiedDate":
return Optional.ofNullable(clazz.cast(updateActionStatusModifiedDate()));
case "SlaMet":
return Optional.ofNullable(clazz.cast(slaMetAsString()));
case "NodeGroupUpdateStatus":
return Optional.ofNullable(clazz.cast(nodeGroupUpdateStatus()));
case "EstimatedUpdateTime":
return Optional.ofNullable(clazz.cast(estimatedUpdateTime()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function