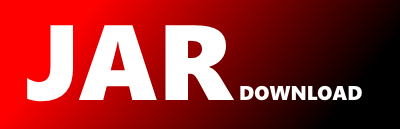
software.amazon.awssdk.services.elasticache.model.ReplicationGroup Maven / Gradle / Ivy
Show all versions of elasticache Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticache.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains all of the attributes of a specific Redis replication group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationGroup implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REPLICATION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::replicationGroupId)).setter(setter(Builder::replicationGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationGroupId").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PENDING_MODIFIED_VALUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(ReplicationGroup::pendingModifiedValues)).setter(setter(Builder::pendingModifiedValues))
.constructor(ReplicationGroupPendingModifiedValues::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingModifiedValues").build())
.build();
private static final SdkField> MEMBER_CLUSTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ReplicationGroup::memberClusters))
.setter(setter(Builder::memberClusters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemberClusters").build(),
ListTrait
.builder()
.memberLocationName("ClusterId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ClusterId").build()).build()).build()).build();
private static final SdkField> NODE_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ReplicationGroup::nodeGroups))
.setter(setter(Builder::nodeGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeGroups").build(),
ListTrait
.builder()
.memberLocationName("NodeGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NodeGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeGroup").build()).build()).build()).build();
private static final SdkField SNAPSHOTTING_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::snapshottingClusterId)).setter(setter(Builder::snapshottingClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshottingClusterId").build())
.build();
private static final SdkField AUTOMATIC_FAILOVER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::automaticFailoverAsString)).setter(setter(Builder::automaticFailover))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomaticFailover").build()).build();
private static final SdkField CONFIGURATION_ENDPOINT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(ReplicationGroup::configurationEndpoint)).setter(setter(Builder::configurationEndpoint))
.constructor(Endpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationEndpoint").build())
.build();
private static final SdkField SNAPSHOT_RETENTION_LIMIT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ReplicationGroup::snapshotRetentionLimit)).setter(setter(Builder::snapshotRetentionLimit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionLimit").build())
.build();
private static final SdkField SNAPSHOT_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::snapshotWindow)).setter(setter(Builder::snapshotWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWindow").build()).build();
private static final SdkField CLUSTER_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ReplicationGroup::clusterEnabled)).setter(setter(Builder::clusterEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterEnabled").build()).build();
private static final SdkField CACHE_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::cacheNodeType)).setter(setter(Builder::cacheNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CacheNodeType").build()).build();
private static final SdkField AUTH_TOKEN_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ReplicationGroup::authTokenEnabled)).setter(setter(Builder::authTokenEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthTokenEnabled").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ReplicationGroup::transitEncryptionEnabled)).setter(setter(Builder::transitEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitEncryptionEnabled").build())
.build();
private static final SdkField AT_REST_ENCRYPTION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ReplicationGroup::atRestEncryptionEnabled)).setter(setter(Builder::atRestEncryptionEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AtRestEncryptionEnabled").build())
.build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ReplicationGroup::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_GROUP_ID_FIELD,
DESCRIPTION_FIELD, STATUS_FIELD, PENDING_MODIFIED_VALUES_FIELD, MEMBER_CLUSTERS_FIELD, NODE_GROUPS_FIELD,
SNAPSHOTTING_CLUSTER_ID_FIELD, AUTOMATIC_FAILOVER_FIELD, CONFIGURATION_ENDPOINT_FIELD,
SNAPSHOT_RETENTION_LIMIT_FIELD, SNAPSHOT_WINDOW_FIELD, CLUSTER_ENABLED_FIELD, CACHE_NODE_TYPE_FIELD,
AUTH_TOKEN_ENABLED_FIELD, TRANSIT_ENCRYPTION_ENABLED_FIELD, AT_REST_ENCRYPTION_ENABLED_FIELD, KMS_KEY_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String replicationGroupId;
private final String description;
private final String status;
private final ReplicationGroupPendingModifiedValues pendingModifiedValues;
private final List memberClusters;
private final List nodeGroups;
private final String snapshottingClusterId;
private final String automaticFailover;
private final Endpoint configurationEndpoint;
private final Integer snapshotRetentionLimit;
private final String snapshotWindow;
private final Boolean clusterEnabled;
private final String cacheNodeType;
private final Boolean authTokenEnabled;
private final Boolean transitEncryptionEnabled;
private final Boolean atRestEncryptionEnabled;
private final String kmsKeyId;
private ReplicationGroup(BuilderImpl builder) {
this.replicationGroupId = builder.replicationGroupId;
this.description = builder.description;
this.status = builder.status;
this.pendingModifiedValues = builder.pendingModifiedValues;
this.memberClusters = builder.memberClusters;
this.nodeGroups = builder.nodeGroups;
this.snapshottingClusterId = builder.snapshottingClusterId;
this.automaticFailover = builder.automaticFailover;
this.configurationEndpoint = builder.configurationEndpoint;
this.snapshotRetentionLimit = builder.snapshotRetentionLimit;
this.snapshotWindow = builder.snapshotWindow;
this.clusterEnabled = builder.clusterEnabled;
this.cacheNodeType = builder.cacheNodeType;
this.authTokenEnabled = builder.authTokenEnabled;
this.transitEncryptionEnabled = builder.transitEncryptionEnabled;
this.atRestEncryptionEnabled = builder.atRestEncryptionEnabled;
this.kmsKeyId = builder.kmsKeyId;
}
/**
*
* The identifier for the replication group.
*
*
* @return The identifier for the replication group.
*/
public String replicationGroupId() {
return replicationGroupId;
}
/**
*
* The user supplied description of the replication group.
*
*
* @return The user supplied description of the replication group.
*/
public String description() {
return description;
}
/**
*
* The current state of this replication group - creating
, available
,
* modifying
, deleting
, create-failed
, snapshotting
.
*
*
* @return The current state of this replication group - creating
, available
,
* modifying
, deleting
, create-failed
, snapshotting
.
*/
public String status() {
return status;
}
/**
*
* A group of settings to be applied to the replication group, either immediately or during the next maintenance
* window.
*
*
* @return A group of settings to be applied to the replication group, either immediately or during the next
* maintenance window.
*/
public ReplicationGroupPendingModifiedValues pendingModifiedValues() {
return pendingModifiedValues;
}
/**
*
* The names of all the cache clusters that are part of this replication group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The names of all the cache clusters that are part of this replication group.
*/
public List memberClusters() {
return memberClusters;
}
/**
*
* A list of node groups in this replication group. For Redis (cluster mode disabled) replication groups, this is a
* single-element list. For Redis (cluster mode enabled) replication groups, the list contains an entry for each
* node group (shard).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of node groups in this replication group. For Redis (cluster mode disabled) replication groups,
* this is a single-element list. For Redis (cluster mode enabled) replication groups, the list contains an
* entry for each node group (shard).
*/
public List nodeGroups() {
return nodeGroups;
}
/**
*
* The cluster ID that is used as the daily snapshot source for the replication group.
*
*
* @return The cluster ID that is used as the daily snapshot source for the replication group.
*/
public String snapshottingClusterId() {
return snapshottingClusterId;
}
/**
*
* Indicates the status of Multi-AZ with automatic failover for this Redis replication group.
*
*
* Amazon ElastiCache for Redis does not support Multi-AZ with automatic failover on:
*
*
* -
*
* Redis versions earlier than 2.8.6.
*
*
* -
*
* Redis (cluster mode disabled): T1 node types.
*
*
* -
*
* Redis (cluster mode enabled): T1 node types.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of Multi-AZ with automatic failover for this Redis replication group.
*
* Amazon ElastiCache for Redis does not support Multi-AZ with automatic failover on:
*
*
* -
*
* Redis versions earlier than 2.8.6.
*
*
* -
*
* Redis (cluster mode disabled): T1 node types.
*
*
* -
*
* Redis (cluster mode enabled): T1 node types.
*
*
* @see AutomaticFailoverStatus
*/
public AutomaticFailoverStatus automaticFailover() {
return AutomaticFailoverStatus.fromValue(automaticFailover);
}
/**
*
* Indicates the status of Multi-AZ with automatic failover for this Redis replication group.
*
*
* Amazon ElastiCache for Redis does not support Multi-AZ with automatic failover on:
*
*
* -
*
* Redis versions earlier than 2.8.6.
*
*
* -
*
* Redis (cluster mode disabled): T1 node types.
*
*
* -
*
* Redis (cluster mode enabled): T1 node types.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #automaticFailover}
* will return {@link AutomaticFailoverStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #automaticFailoverAsString}.
*
*
* @return Indicates the status of Multi-AZ with automatic failover for this Redis replication group.
*
* Amazon ElastiCache for Redis does not support Multi-AZ with automatic failover on:
*
*
* -
*
* Redis versions earlier than 2.8.6.
*
*
* -
*
* Redis (cluster mode disabled): T1 node types.
*
*
* -
*
* Redis (cluster mode enabled): T1 node types.
*
*
* @see AutomaticFailoverStatus
*/
public String automaticFailoverAsString() {
return automaticFailover;
}
/**
*
* The configuration endpoint for this replication group. Use the configuration endpoint to connect to this
* replication group.
*
*
* @return The configuration endpoint for this replication group. Use the configuration endpoint to connect to this
* replication group.
*/
public Endpoint configurationEndpoint() {
return configurationEndpoint;
}
/**
*
* The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For example,
* if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained for 5 days
* before being deleted.
*
*
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*
*
* @return The number of days for which ElastiCache retains automatic cluster snapshots before deleting them. For
* example, if you set SnapshotRetentionLimit
to 5, a snapshot that was taken today is retained
* for 5 days before being deleted.
*
* If the value of SnapshotRetentionLimit
is set to zero (0), backups are turned off.
*
*/
public Integer snapshotRetentionLimit() {
return snapshotRetentionLimit;
}
/**
*
* The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group (shard).
*
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*
*
* @return The daily time range (in UTC) during which ElastiCache begins taking a daily snapshot of your node group
* (shard).
*
* Example: 05:00-09:00
*
*
* If you do not specify this parameter, ElastiCache automatically chooses an appropriate time range.
*
*
*
* This parameter is only valid if the Engine
parameter is redis
.
*
*/
public String snapshotWindow() {
return snapshotWindow;
}
/**
*
* A flag indicating whether or not this replication group is cluster enabled; i.e., whether its data can be
* partitioned across multiple shards (API/CLI: node groups).
*
*
* Valid values: true
| false
*
*
* @return A flag indicating whether or not this replication group is cluster enabled; i.e., whether its data can be
* partitioned across multiple shards (API/CLI: node groups).
*
* Valid values: true
| false
*/
public Boolean clusterEnabled() {
return clusterEnabled;
}
/**
*
* The name of the compute and memory capacity node type for each node in the replication group.
*
*
* @return The name of the compute and memory capacity node type for each node in the replication group.
*/
public String cacheNodeType() {
return cacheNodeType;
}
/**
*
* A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
*
* Default: false
*
*
* @return A flag that enables using an AuthToken
(password) when issuing Redis commands.
*
* Default: false
*/
public Boolean authTokenEnabled() {
return authTokenEnabled;
}
/**
*
* A flag that enables in-transit encryption when set to true
.
*
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To enable
* in-transit encryption on a cluster you must set TransitEncryptionEnabled
to true
when
* you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables in-transit encryption when set to true
.
*
* You cannot modify the value of TransitEncryptionEnabled
after the cluster is created. To
* enable in-transit encryption on a cluster you must set TransitEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public Boolean transitEncryptionEnabled() {
return transitEncryptionEnabled;
}
/**
*
* A flag that enables encryption at-rest when set to true
.
*
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To enable
* encryption at-rest on a cluster you must set AtRestEncryptionEnabled
to true
when you
* create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*
*
* @return A flag that enables encryption at-rest when set to true
.
*
* You cannot modify the value of AtRestEncryptionEnabled
after the cluster is created. To
* enable encryption at-rest on a cluster you must set AtRestEncryptionEnabled
to
* true
when you create a cluster.
*
*
* Required: Only available when creating a replication group in an Amazon VPC using redis version
* 3.2.6
, 4.x
or later.
*
*
* Default: false
*/
public Boolean atRestEncryptionEnabled() {
return atRestEncryptionEnabled;
}
/**
*
* The ID of the KMS key used to encrypt the disk in the cluster.
*
*
* @return The ID of the KMS key used to encrypt the disk in the cluster.
*/
public String kmsKeyId() {
return kmsKeyId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationGroupId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(pendingModifiedValues());
hashCode = 31 * hashCode + Objects.hashCode(memberClusters());
hashCode = 31 * hashCode + Objects.hashCode(nodeGroups());
hashCode = 31 * hashCode + Objects.hashCode(snapshottingClusterId());
hashCode = 31 * hashCode + Objects.hashCode(automaticFailoverAsString());
hashCode = 31 * hashCode + Objects.hashCode(configurationEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionLimit());
hashCode = 31 * hashCode + Objects.hashCode(snapshotWindow());
hashCode = 31 * hashCode + Objects.hashCode(clusterEnabled());
hashCode = 31 * hashCode + Objects.hashCode(cacheNodeType());
hashCode = 31 * hashCode + Objects.hashCode(authTokenEnabled());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(atRestEncryptionEnabled());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationGroup)) {
return false;
}
ReplicationGroup other = (ReplicationGroup) obj;
return Objects.equals(replicationGroupId(), other.replicationGroupId())
&& Objects.equals(description(), other.description()) && Objects.equals(status(), other.status())
&& Objects.equals(pendingModifiedValues(), other.pendingModifiedValues())
&& Objects.equals(memberClusters(), other.memberClusters()) && Objects.equals(nodeGroups(), other.nodeGroups())
&& Objects.equals(snapshottingClusterId(), other.snapshottingClusterId())
&& Objects.equals(automaticFailoverAsString(), other.automaticFailoverAsString())
&& Objects.equals(configurationEndpoint(), other.configurationEndpoint())
&& Objects.equals(snapshotRetentionLimit(), other.snapshotRetentionLimit())
&& Objects.equals(snapshotWindow(), other.snapshotWindow())
&& Objects.equals(clusterEnabled(), other.clusterEnabled())
&& Objects.equals(cacheNodeType(), other.cacheNodeType())
&& Objects.equals(authTokenEnabled(), other.authTokenEnabled())
&& Objects.equals(transitEncryptionEnabled(), other.transitEncryptionEnabled())
&& Objects.equals(atRestEncryptionEnabled(), other.atRestEncryptionEnabled())
&& Objects.equals(kmsKeyId(), other.kmsKeyId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ReplicationGroup").add("ReplicationGroupId", replicationGroupId())
.add("Description", description()).add("Status", status()).add("PendingModifiedValues", pendingModifiedValues())
.add("MemberClusters", memberClusters()).add("NodeGroups", nodeGroups())
.add("SnapshottingClusterId", snapshottingClusterId()).add("AutomaticFailover", automaticFailoverAsString())
.add("ConfigurationEndpoint", configurationEndpoint()).add("SnapshotRetentionLimit", snapshotRetentionLimit())
.add("SnapshotWindow", snapshotWindow()).add("ClusterEnabled", clusterEnabled())
.add("CacheNodeType", cacheNodeType()).add("AuthTokenEnabled", authTokenEnabled())
.add("TransitEncryptionEnabled", transitEncryptionEnabled())
.add("AtRestEncryptionEnabled", atRestEncryptionEnabled()).add("KmsKeyId", kmsKeyId()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationGroupId":
return Optional.ofNullable(clazz.cast(replicationGroupId()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "PendingModifiedValues":
return Optional.ofNullable(clazz.cast(pendingModifiedValues()));
case "MemberClusters":
return Optional.ofNullable(clazz.cast(memberClusters()));
case "NodeGroups":
return Optional.ofNullable(clazz.cast(nodeGroups()));
case "SnapshottingClusterId":
return Optional.ofNullable(clazz.cast(snapshottingClusterId()));
case "AutomaticFailover":
return Optional.ofNullable(clazz.cast(automaticFailoverAsString()));
case "ConfigurationEndpoint":
return Optional.ofNullable(clazz.cast(configurationEndpoint()));
case "SnapshotRetentionLimit":
return Optional.ofNullable(clazz.cast(snapshotRetentionLimit()));
case "SnapshotWindow":
return Optional.ofNullable(clazz.cast(snapshotWindow()));
case "ClusterEnabled":
return Optional.ofNullable(clazz.cast(clusterEnabled()));
case "CacheNodeType":
return Optional.ofNullable(clazz.cast(cacheNodeType()));
case "AuthTokenEnabled":
return Optional.ofNullable(clazz.cast(authTokenEnabled()));
case "TransitEncryptionEnabled":
return Optional.ofNullable(clazz.cast(transitEncryptionEnabled()));
case "AtRestEncryptionEnabled":
return Optional.ofNullable(clazz.cast(atRestEncryptionEnabled()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function