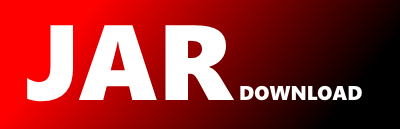
software.amazon.awssdk.services.elasticbeanstalk.model.BuildConfiguration Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Settings for an AWS CodeBuild build.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BuildConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ARTIFACT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ArtifactName").getter(getter(BuildConfiguration::artifactName)).setter(setter(Builder::artifactName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ArtifactName").build()).build();
private static final SdkField CODE_BUILD_SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CodeBuildServiceRole").getter(getter(BuildConfiguration::codeBuildServiceRole))
.setter(setter(Builder::codeBuildServiceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeBuildServiceRole").build())
.build();
private static final SdkField COMPUTE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ComputeType").getter(getter(BuildConfiguration::computeTypeAsString))
.setter(setter(Builder::computeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeType").build()).build();
private static final SdkField IMAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Image")
.getter(getter(BuildConfiguration::image)).setter(setter(Builder::image))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Image").build()).build();
private static final SdkField TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TimeoutInMinutes").getter(getter(BuildConfiguration::timeoutInMinutes))
.setter(setter(Builder::timeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutInMinutes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARTIFACT_NAME_FIELD,
CODE_BUILD_SERVICE_ROLE_FIELD, COMPUTE_TYPE_FIELD, IMAGE_FIELD, TIMEOUT_IN_MINUTES_FIELD));
private static final long serialVersionUID = 1L;
private final String artifactName;
private final String codeBuildServiceRole;
private final String computeType;
private final String image;
private final Integer timeoutInMinutes;
private BuildConfiguration(BuilderImpl builder) {
this.artifactName = builder.artifactName;
this.codeBuildServiceRole = builder.codeBuildServiceRole;
this.computeType = builder.computeType;
this.image = builder.image;
this.timeoutInMinutes = builder.timeoutInMinutes;
}
/**
*
* The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact in the
* S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label-artifact
* -name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*
*
* @return The name of the artifact of the CodeBuild build. If provided, Elastic Beanstalk stores the build artifact
* in the S3 location
* S3-bucket/resources/application-name/codebuild/codebuild-version-label
* -artifact-name.zip. If not provided, Elastic Beanstalk stores the build artifact in the S3
* location S3-bucket/resources/application-name/codebuild/codebuild-version-label.zip.
*/
public String artifactName() {
return artifactName;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS CodeBuild to
* interact with dependent AWS services on behalf of the AWS account.
*
*
* @return The Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that enables AWS
* CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*/
public String codeBuildServiceRole() {
return codeBuildServiceRole;
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computeType} will
* return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #computeTypeAsString}.
*
*
* @return Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @see ComputeType
*/
public ComputeType computeType() {
return ComputeType.fromValue(computeType);
}
/**
*
* Information about the compute resources the build project will use.
*
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #computeType} will
* return {@link ComputeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #computeTypeAsString}.
*
*
* @return Information about the compute resources the build project will use.
*
* -
*
* BUILD_GENERAL1_SMALL: Use up to 3 GB memory and 2 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_MEDIUM: Use up to 7 GB memory and 4 vCPUs for builds
*
*
* -
*
* BUILD_GENERAL1_LARGE: Use up to 15 GB memory and 8 vCPUs for builds
*
*
* @see ComputeType
*/
public String computeTypeAsString() {
return computeType;
}
/**
*
* The ID of the Docker image to use for this build project.
*
*
* @return The ID of the Docker image to use for this build project.
*/
public String image() {
return image;
}
/**
*
* How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related build that
* does not get marked as completed. The default is 60 minutes.
*
*
* @return How long in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait until timing out any related
* build that does not get marked as completed. The default is 60 minutes.
*/
public Integer timeoutInMinutes() {
return timeoutInMinutes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(artifactName());
hashCode = 31 * hashCode + Objects.hashCode(codeBuildServiceRole());
hashCode = 31 * hashCode + Objects.hashCode(computeTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(image());
hashCode = 31 * hashCode + Objects.hashCode(timeoutInMinutes());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BuildConfiguration)) {
return false;
}
BuildConfiguration other = (BuildConfiguration) obj;
return Objects.equals(artifactName(), other.artifactName())
&& Objects.equals(codeBuildServiceRole(), other.codeBuildServiceRole())
&& Objects.equals(computeTypeAsString(), other.computeTypeAsString()) && Objects.equals(image(), other.image())
&& Objects.equals(timeoutInMinutes(), other.timeoutInMinutes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("BuildConfiguration").add("ArtifactName", artifactName())
.add("CodeBuildServiceRole", codeBuildServiceRole()).add("ComputeType", computeTypeAsString())
.add("Image", image()).add("TimeoutInMinutes", timeoutInMinutes()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ArtifactName":
return Optional.ofNullable(clazz.cast(artifactName()));
case "CodeBuildServiceRole":
return Optional.ofNullable(clazz.cast(codeBuildServiceRole()));
case "ComputeType":
return Optional.ofNullable(clazz.cast(computeTypeAsString()));
case "Image":
return Optional.ofNullable(clazz.cast(image()));
case "TimeoutInMinutes":
return Optional.ofNullable(clazz.cast(timeoutInMinutes()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function