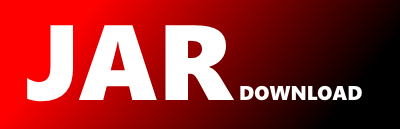
software.amazon.awssdk.services.elasticbeanstalk.model.EventDescription Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an event.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EventDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField EVENT_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EventDate").getter(getter(EventDescription::eventDate)).setter(setter(Builder::eventDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventDate").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Message")
.getter(getter(EventDescription::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(EventDescription::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(EventDescription::versionLabel)).setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(EventDescription::templateName)).setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(EventDescription::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField PLATFORM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformArn").getter(getter(EventDescription::platformArn)).setter(setter(Builder::platformArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformArn").build()).build();
private static final SdkField REQUEST_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RequestId").getter(getter(EventDescription::requestId)).setter(setter(Builder::requestId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestId").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Severity").getter(getter(EventDescription::severityAsString)).setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Severity").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EVENT_DATE_FIELD,
MESSAGE_FIELD, APPLICATION_NAME_FIELD, VERSION_LABEL_FIELD, TEMPLATE_NAME_FIELD, ENVIRONMENT_NAME_FIELD,
PLATFORM_ARN_FIELD, REQUEST_ID_FIELD, SEVERITY_FIELD));
private static final long serialVersionUID = 1L;
private final Instant eventDate;
private final String message;
private final String applicationName;
private final String versionLabel;
private final String templateName;
private final String environmentName;
private final String platformArn;
private final String requestId;
private final String severity;
private EventDescription(BuilderImpl builder) {
this.eventDate = builder.eventDate;
this.message = builder.message;
this.applicationName = builder.applicationName;
this.versionLabel = builder.versionLabel;
this.templateName = builder.templateName;
this.environmentName = builder.environmentName;
this.platformArn = builder.platformArn;
this.requestId = builder.requestId;
this.severity = builder.severity;
}
/**
*
* The date when the event occurred.
*
*
* @return The date when the event occurred.
*/
public Instant eventDate() {
return eventDate;
}
/**
*
* The event message.
*
*
* @return The event message.
*/
public String message() {
return message;
}
/**
*
* The application associated with the event.
*
*
* @return The application associated with the event.
*/
public String applicationName() {
return applicationName;
}
/**
*
* The release label for the application version associated with this event.
*
*
* @return The release label for the application version associated with this event.
*/
public String versionLabel() {
return versionLabel;
}
/**
*
* The name of the configuration associated with this event.
*
*
* @return The name of the configuration associated with this event.
*/
public String templateName() {
return templateName;
}
/**
*
* The name of the environment associated with this event.
*
*
* @return The name of the environment associated with this event.
*/
public String environmentName() {
return environmentName;
}
/**
*
* The ARN of the platform version.
*
*
* @return The ARN of the platform version.
*/
public String platformArn() {
return platformArn;
}
/**
*
* The web service request ID for the activity of this event.
*
*
* @return The web service request ID for the activity of this event.
*/
public String requestId() {
return requestId;
}
/**
*
* The severity level of this event.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link EventSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return The severity level of this event.
* @see EventSeverity
*/
public EventSeverity severity() {
return EventSeverity.fromValue(severity);
}
/**
*
* The severity level of this event.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link EventSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return The severity level of this event.
* @see EventSeverity
*/
public String severityAsString() {
return severity;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eventDate());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(platformArn());
hashCode = 31 * hashCode + Objects.hashCode(requestId());
hashCode = 31 * hashCode + Objects.hashCode(severityAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EventDescription)) {
return false;
}
EventDescription other = (EventDescription) obj;
return Objects.equals(eventDate(), other.eventDate()) && Objects.equals(message(), other.message())
&& Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(versionLabel(), other.versionLabel()) && Objects.equals(templateName(), other.templateName())
&& Objects.equals(environmentName(), other.environmentName())
&& Objects.equals(platformArn(), other.platformArn()) && Objects.equals(requestId(), other.requestId())
&& Objects.equals(severityAsString(), other.severityAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("EventDescription").add("EventDate", eventDate()).add("Message", message())
.add("ApplicationName", applicationName()).add("VersionLabel", versionLabel())
.add("TemplateName", templateName()).add("EnvironmentName", environmentName()).add("PlatformArn", platformArn())
.add("RequestId", requestId()).add("Severity", severityAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EventDate":
return Optional.ofNullable(clazz.cast(eventDate()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "PlatformArn":
return Optional.ofNullable(clazz.cast(platformArn()));
case "RequestId":
return Optional.ofNullable(clazz.cast(requestId()));
case "Severity":
return Optional.ofNullable(clazz.cast(severityAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function