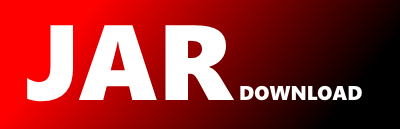
software.amazon.awssdk.services.elasticbeanstalk.ElasticBeanstalkAsyncClient Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.elasticbeanstalk.model.AbortEnvironmentUpdateRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.AbortEnvironmentUpdateResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ApplyEnvironmentManagedActionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ApplyEnvironmentManagedActionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.AssociateEnvironmentOperationsRoleRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.AssociateEnvironmentOperationsRoleResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CheckDnsAvailabilityRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CheckDnsAvailabilityResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ComposeEnvironmentsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ComposeEnvironmentsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateApplicationRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateApplicationResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateApplicationVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateApplicationVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateConfigurationTemplateRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateConfigurationTemplateResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateEnvironmentRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateEnvironmentResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreatePlatformVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreatePlatformVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateStorageLocationRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.CreateStorageLocationResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteApplicationVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteApplicationVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteConfigurationTemplateRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteConfigurationTemplateResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteEnvironmentConfigurationRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeleteEnvironmentConfigurationResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeletePlatformVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DeletePlatformVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeAccountAttributesRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeAccountAttributesResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeApplicationVersionsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeApplicationVersionsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeApplicationsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeApplicationsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeConfigurationOptionsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeConfigurationOptionsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeConfigurationSettingsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeConfigurationSettingsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentHealthRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentHealthResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentResourcesRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentResourcesResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeInstancesHealthRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribeInstancesHealthResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribePlatformVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DescribePlatformVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.DisassociateEnvironmentOperationsRoleRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.DisassociateEnvironmentOperationsRoleResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListAvailableSolutionStacksRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListAvailableSolutionStacksResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.RebuildEnvironmentRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.RebuildEnvironmentResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.RequestEnvironmentInfoRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.RequestEnvironmentInfoResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.RestartAppServerRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.RestartAppServerResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.RetrieveEnvironmentInfoRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.RetrieveEnvironmentInfoResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.SwapEnvironmentCnamEsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.SwapEnvironmentCnamEsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.TerminateEnvironmentRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.TerminateEnvironmentResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationResourceLifecycleRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationResourceLifecycleResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationVersionRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateApplicationVersionResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateConfigurationTemplateRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateConfigurationTemplateResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateEnvironmentRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateEnvironmentResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateTagsForResourceRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.UpdateTagsForResourceResponse;
import software.amazon.awssdk.services.elasticbeanstalk.model.ValidateConfigurationSettingsRequest;
import software.amazon.awssdk.services.elasticbeanstalk.model.ValidateConfigurationSettingsResponse;
import software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEnvironmentManagedActionHistoryPublisher;
import software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher;
import software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformBranchesPublisher;
import software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher;
import software.amazon.awssdk.services.elasticbeanstalk.waiters.ElasticBeanstalkAsyncWaiter;
/**
* Service client for accessing Elastic Beanstalk asynchronously. This can be created using the static
* {@link #builder()} method.
*
* AWS Elastic Beanstalk
*
* AWS Elastic Beanstalk makes it easy for you to create, deploy, and manage scalable, fault-tolerant applications
* running on the Amazon Web Services cloud.
*
*
* For more information about this product, go to the AWS Elastic
* Beanstalk details page. The location of the latest AWS Elastic Beanstalk WSDL is https://elasticbeanstalk.s3.amazonaws.com/doc/2010-12-01/AWSElasticBeanstalk.wsdl. To install the Software
* Development Kits (SDKs), Integrated Development Environment (IDE) Toolkits, and command line tools that enable you to
* access the API, go to Tools for Amazon Web Services.
*
*
* Endpoints
*
*
* For a list of region-specific endpoints that AWS Elastic Beanstalk supports, go to Regions and Endpoints in
* the Amazon Web Services Glossary.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface ElasticBeanstalkAsyncClient extends SdkClient {
String SERVICE_NAME = "elasticbeanstalk";
/**
* Create a {@link ElasticBeanstalkAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ElasticBeanstalkAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ElasticBeanstalkAsyncClient}.
*/
static ElasticBeanstalkAsyncClientBuilder builder() {
return new DefaultElasticBeanstalkAsyncClientBuilder();
}
/**
*
* Cancels in-progress environment configuration update or application version deployment.
*
*
* @param abortEnvironmentUpdateRequest
* @return A Java Future containing the result of the AbortEnvironmentUpdate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.AbortEnvironmentUpdate
* @see AWS API Documentation
*/
default CompletableFuture abortEnvironmentUpdate(
AbortEnvironmentUpdateRequest abortEnvironmentUpdateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels in-progress environment configuration update or application version deployment.
*
*
*
* This is a convenience which creates an instance of the {@link AbortEnvironmentUpdateRequest.Builder} avoiding the
* need to create one manually via {@link AbortEnvironmentUpdateRequest#builder()}
*
*
* @param abortEnvironmentUpdateRequest
* A {@link Consumer} that will call methods on {@link AbortEnvironmentUpdateMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the AbortEnvironmentUpdate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.AbortEnvironmentUpdate
* @see AWS API Documentation
*/
default CompletableFuture abortEnvironmentUpdate(
Consumer abortEnvironmentUpdateRequest) {
return abortEnvironmentUpdate(AbortEnvironmentUpdateRequest.builder().applyMutation(abortEnvironmentUpdateRequest)
.build());
}
/**
*
* Applies a scheduled managed action immediately. A managed action can be applied only if its status is
* Scheduled
. Get the status and action ID of a managed action with
* DescribeEnvironmentManagedActions.
*
*
* @param applyEnvironmentManagedActionRequest
* Request to execute a scheduled managed action immediately.
* @return A Java Future containing the result of the ApplyEnvironmentManagedAction operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - ManagedActionInvalidStateException Cannot modify the managed action in its current state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ApplyEnvironmentManagedAction
* @see AWS API Documentation
*/
default CompletableFuture applyEnvironmentManagedAction(
ApplyEnvironmentManagedActionRequest applyEnvironmentManagedActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Applies a scheduled managed action immediately. A managed action can be applied only if its status is
* Scheduled
. Get the status and action ID of a managed action with
* DescribeEnvironmentManagedActions.
*
*
*
* This is a convenience which creates an instance of the {@link ApplyEnvironmentManagedActionRequest.Builder}
* avoiding the need to create one manually via {@link ApplyEnvironmentManagedActionRequest#builder()}
*
*
* @param applyEnvironmentManagedActionRequest
* A {@link Consumer} that will call methods on {@link ApplyEnvironmentManagedActionRequest.Builder} to
* create a request. Request to execute a scheduled managed action immediately.
* @return A Java Future containing the result of the ApplyEnvironmentManagedAction operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - ManagedActionInvalidStateException Cannot modify the managed action in its current state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ApplyEnvironmentManagedAction
* @see AWS API Documentation
*/
default CompletableFuture applyEnvironmentManagedAction(
Consumer applyEnvironmentManagedActionRequest) {
return applyEnvironmentManagedAction(ApplyEnvironmentManagedActionRequest.builder()
.applyMutation(applyEnvironmentManagedActionRequest).build());
}
/**
*
* Add or change the operations role used by an environment. After this call is made, Elastic Beanstalk uses the
* associated operations role for permissions to downstream services during subsequent calls acting on this
* environment. For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @param associateEnvironmentOperationsRoleRequest
* Request to add or change the operations role used by an environment.
* @return A Java Future containing the result of the AssociateEnvironmentOperationsRole operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.AssociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
default CompletableFuture associateEnvironmentOperationsRole(
AssociateEnvironmentOperationsRoleRequest associateEnvironmentOperationsRoleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Add or change the operations role used by an environment. After this call is made, Elastic Beanstalk uses the
* associated operations role for permissions to downstream services during subsequent calls acting on this
* environment. For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateEnvironmentOperationsRoleRequest.Builder}
* avoiding the need to create one manually via {@link AssociateEnvironmentOperationsRoleRequest#builder()}
*
*
* @param associateEnvironmentOperationsRoleRequest
* A {@link Consumer} that will call methods on {@link AssociateEnvironmentOperationsRoleMessage.Builder} to
* create a request. Request to add or change the operations role used by an environment.
* @return A Java Future containing the result of the AssociateEnvironmentOperationsRole operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.AssociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
default CompletableFuture associateEnvironmentOperationsRole(
Consumer associateEnvironmentOperationsRoleRequest) {
return associateEnvironmentOperationsRole(AssociateEnvironmentOperationsRoleRequest.builder()
.applyMutation(associateEnvironmentOperationsRoleRequest).build());
}
/**
*
* Checks if the specified CNAME is available.
*
*
* @param checkDnsAvailabilityRequest
* Results message indicating whether a CNAME is available.
* @return A Java Future containing the result of the CheckDNSAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CheckDNSAvailability
* @see AWS API Documentation
*/
default CompletableFuture checkDNSAvailability(
CheckDnsAvailabilityRequest checkDnsAvailabilityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Checks if the specified CNAME is available.
*
*
*
* This is a convenience which creates an instance of the {@link CheckDnsAvailabilityRequest.Builder} avoiding the
* need to create one manually via {@link CheckDnsAvailabilityRequest#builder()}
*
*
* @param checkDnsAvailabilityRequest
* A {@link Consumer} that will call methods on {@link CheckDNSAvailabilityMessage.Builder} to create a
* request. Results message indicating whether a CNAME is available.
* @return A Java Future containing the result of the CheckDNSAvailability operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CheckDNSAvailability
* @see AWS API Documentation
*/
default CompletableFuture checkDNSAvailability(
Consumer checkDnsAvailabilityRequest) {
return checkDNSAvailability(CheckDnsAvailabilityRequest.builder().applyMutation(checkDnsAvailabilityRequest).build());
}
/**
*
* Create or update a group of environments that each run a separate component of a single application. Takes a list
* of version labels that specify application source bundles for each of the environments to create or update. The
* name of each environment and other required information must be included in the source bundles in an environment
* manifest named env.yaml
. See Compose
* Environments for details.
*
*
* @param composeEnvironmentsRequest
* Request to create or update a group of environments.
* @return A Java Future containing the result of the ComposeEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyEnvironmentsException The specified account has reached its limit of environments.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ComposeEnvironments
* @see AWS API Documentation
*/
default CompletableFuture composeEnvironments(
ComposeEnvironmentsRequest composeEnvironmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create or update a group of environments that each run a separate component of a single application. Takes a list
* of version labels that specify application source bundles for each of the environments to create or update. The
* name of each environment and other required information must be included in the source bundles in an environment
* manifest named env.yaml
. See Compose
* Environments for details.
*
*
*
* This is a convenience which creates an instance of the {@link ComposeEnvironmentsRequest.Builder} avoiding the
* need to create one manually via {@link ComposeEnvironmentsRequest#builder()}
*
*
* @param composeEnvironmentsRequest
* A {@link Consumer} that will call methods on {@link ComposeEnvironmentsMessage.Builder} to create a
* request. Request to create or update a group of environments.
* @return A Java Future containing the result of the ComposeEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyEnvironmentsException The specified account has reached its limit of environments.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ComposeEnvironments
* @see AWS API Documentation
*/
default CompletableFuture composeEnvironments(
Consumer composeEnvironmentsRequest) {
return composeEnvironments(ComposeEnvironmentsRequest.builder().applyMutation(composeEnvironmentsRequest).build());
}
/**
*
* Creates an application that has one configuration template named default
and no application
* versions.
*
*
* @param createApplicationRequest
* Request to create an application.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyApplicationsException The specified account has reached its limit of applications.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an application that has one configuration template named default
and no application
* versions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationMessage.Builder} to create a request.
* Request to create an application.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyApplicationsException The specified account has reached its limit of applications.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(
Consumer createApplicationRequest) {
return createApplication(CreateApplicationRequest.builder().applyMutation(createApplicationRequest).build());
}
/**
*
* Creates an application version for the specified application. You can create an application version from a source
* bundle in Amazon S3, a commit in AWS CodeCommit, or the output of an AWS CodeBuild build as follows:
*
*
* Specify a commit in an AWS CodeCommit repository with SourceBuildInformation
.
*
*
* Specify a build in an AWS CodeBuild with SourceBuildInformation
and BuildConfiguration
.
*
*
* Specify a source bundle in S3 with SourceBundle
*
*
* Omit both SourceBuildInformation
and SourceBundle
to use the default sample
* application.
*
*
*
* After you create an application version with a specified Amazon S3 bucket and key location, you can't change that
* Amazon S3 location. If you change the Amazon S3 location, you receive an exception when you attempt to launch an
* environment from the application version.
*
*
*
* @param createApplicationVersionRequest
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyApplicationsException The specified account has reached its limit of applications.
* - TooManyApplicationVersionsException The specified account has reached its limit of application
* versions.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - S3LocationNotInServiceRegionException The specified S3 bucket does not belong to the S3 region in
* which the service is running. The following regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* - CodeBuildNotInServiceRegionException AWS CodeBuild is not available in the specified region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture createApplicationVersion(
CreateApplicationVersionRequest createApplicationVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an application version for the specified application. You can create an application version from a source
* bundle in Amazon S3, a commit in AWS CodeCommit, or the output of an AWS CodeBuild build as follows:
*
*
* Specify a commit in an AWS CodeCommit repository with SourceBuildInformation
.
*
*
* Specify a build in an AWS CodeBuild with SourceBuildInformation
and BuildConfiguration
.
*
*
* Specify a source bundle in S3 with SourceBundle
*
*
* Omit both SourceBuildInformation
and SourceBundle
to use the default sample
* application.
*
*
*
* After you create an application version with a specified Amazon S3 bucket and key location, you can't change that
* Amazon S3 location. If you change the Amazon S3 location, you receive an exception when you attempt to launch an
* environment from the application version.
*
*
*
* This is a convenience which creates an instance of the {@link CreateApplicationVersionRequest.Builder} avoiding
* the need to create one manually via {@link CreateApplicationVersionRequest#builder()}
*
*
* @param createApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationVersionMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyApplicationsException The specified account has reached its limit of applications.
* - TooManyApplicationVersionsException The specified account has reached its limit of application
* versions.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - S3LocationNotInServiceRegionException The specified S3 bucket does not belong to the S3 region in
* which the service is running. The following regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* - CodeBuildNotInServiceRegionException AWS CodeBuild is not available in the specified region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture createApplicationVersion(
Consumer createApplicationVersionRequest) {
return createApplicationVersion(CreateApplicationVersionRequest.builder().applyMutation(createApplicationVersionRequest)
.build());
}
/**
*
* Creates an AWS Elastic Beanstalk configuration template, associated with a specific Elastic Beanstalk
* application. You define application configuration settings in a configuration template. You can then use the
* configuration template to deploy different versions of the application with the same configuration settings.
*
*
* Templates aren't associated with any environment. The EnvironmentName
response element is always
* null
.
*
*
* Related Topics
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createConfigurationTemplateRequest
* Request to create a configuration template.
* @return A Java Future containing the result of the CreateConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - TooManyConfigurationTemplatesException The specified account has reached its limit of configuration
* templates.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture createConfigurationTemplate(
CreateConfigurationTemplateRequest createConfigurationTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an AWS Elastic Beanstalk configuration template, associated with a specific Elastic Beanstalk
* application. You define application configuration settings in a configuration template. You can then use the
* configuration template to deploy different versions of the application with the same configuration settings.
*
*
* Templates aren't associated with any environment. The EnvironmentName
response element is always
* null
.
*
*
* Related Topics
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateConfigurationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link CreateConfigurationTemplateRequest#builder()}
*
*
* @param createConfigurationTemplateRequest
* A {@link Consumer} that will call methods on {@link CreateConfigurationTemplateMessage.Builder} to create
* a request. Request to create a configuration template.
* @return A Java Future containing the result of the CreateConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - TooManyConfigurationTemplatesException The specified account has reached its limit of configuration
* templates.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture createConfigurationTemplate(
Consumer createConfigurationTemplateRequest) {
return createConfigurationTemplate(CreateConfigurationTemplateRequest.builder()
.applyMutation(createConfigurationTemplateRequest).build());
}
/**
*
* Launches an AWS Elastic Beanstalk environment for the specified application using the specified configuration.
*
*
* @param createEnvironmentRequest
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyEnvironmentsException The specified account has reached its limit of environments.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture createEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Launches an AWS Elastic Beanstalk environment for the specified application using the specified configuration.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link CreateEnvironmentRequest#builder()}
*
*
* @param createEnvironmentRequest
* A {@link Consumer} that will call methods on {@link CreateEnvironmentMessage.Builder} to create a request.
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyEnvironmentsException The specified account has reached its limit of environments.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture createEnvironment(
Consumer createEnvironmentRequest) {
return createEnvironment(CreateEnvironmentRequest.builder().applyMutation(createEnvironmentRequest).build());
}
/**
*
* Create a new version of your custom platform.
*
*
* @param createPlatformVersionRequest
* Request to create a new platform version.
* @return A Java Future containing the result of the CreatePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - TooManyPlatformsException You have exceeded the maximum number of allowed platforms associated with
* the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreatePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture createPlatformVersion(
CreatePlatformVersionRequest createPlatformVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a new version of your custom platform.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePlatformVersionRequest.Builder} avoiding the
* need to create one manually via {@link CreatePlatformVersionRequest#builder()}
*
*
* @param createPlatformVersionRequest
* A {@link Consumer} that will call methods on {@link CreatePlatformVersionRequest.Builder} to create a
* request. Request to create a new platform version.
* @return A Java Future containing the result of the CreatePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - TooManyPlatformsException You have exceeded the maximum number of allowed platforms associated with
* the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreatePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture createPlatformVersion(
Consumer createPlatformVersionRequest) {
return createPlatformVersion(CreatePlatformVersionRequest.builder().applyMutation(createPlatformVersionRequest).build());
}
/**
*
* Creates a bucket in Amazon S3 to store application versions, logs, and other files used by Elastic Beanstalk
* environments. The Elastic Beanstalk console and EB CLI call this API the first time you create an environment in
* a region. If the storage location already exists, CreateStorageLocation
still returns the bucket
* name but does not create a new bucket.
*
*
* @param createStorageLocationRequest
* @return A Java Future containing the result of the CreateStorageLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - S3SubscriptionRequiredException The specified account does not have a subscription to Amazon S3.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateStorageLocation
* @see AWS API Documentation
*/
default CompletableFuture createStorageLocation(
CreateStorageLocationRequest createStorageLocationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a bucket in Amazon S3 to store application versions, logs, and other files used by Elastic Beanstalk
* environments. The Elastic Beanstalk console and EB CLI call this API the first time you create an environment in
* a region. If the storage location already exists, CreateStorageLocation
still returns the bucket
* name but does not create a new bucket.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStorageLocationRequest.Builder} avoiding the
* need to create one manually via {@link CreateStorageLocationRequest#builder()}
*
*
* @param createStorageLocationRequest
* A {@link Consumer} that will call methods on {@link CreateStorageLocationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateStorageLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - S3SubscriptionRequiredException The specified account does not have a subscription to Amazon S3.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateStorageLocation
* @see AWS API Documentation
*/
default CompletableFuture createStorageLocation(
Consumer createStorageLocationRequest) {
return createStorageLocation(CreateStorageLocationRequest.builder().applyMutation(createStorageLocationRequest).build());
}
/**
*
* Creates a bucket in Amazon S3 to store application versions, logs, and other files used by Elastic Beanstalk
* environments. The Elastic Beanstalk console and EB CLI call this API the first time you create an environment in
* a region. If the storage location already exists, CreateStorageLocation
still returns the bucket
* name but does not create a new bucket.
*
*
* @return A Java Future containing the result of the CreateStorageLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - S3SubscriptionRequiredException The specified account does not have a subscription to Amazon S3.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.CreateStorageLocation
* @see AWS API Documentation
*/
default CompletableFuture createStorageLocation() {
return createStorageLocation(CreateStorageLocationRequest.builder().build());
}
/**
*
* Deletes the specified application along with all associated versions and configurations. The application versions
* will not be deleted from your Amazon S3 bucket.
*
*
*
* You cannot delete an application that has a running environment.
*
*
*
* @param deleteApplicationRequest
* Request to delete an application.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteApplication
* @see AWS API Documentation
*/
default CompletableFuture deleteApplication(DeleteApplicationRequest deleteApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified application along with all associated versions and configurations. The application versions
* will not be deleted from your Amazon S3 bucket.
*
*
*
* You cannot delete an application that has a running environment.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationRequest.Builder} avoiding the need
* to create one manually via {@link DeleteApplicationRequest#builder()}
*
*
* @param deleteApplicationRequest
* A {@link Consumer} that will call methods on {@link DeleteApplicationMessage.Builder} to create a request.
* Request to delete an application.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteApplication
* @see AWS API Documentation
*/
default CompletableFuture deleteApplication(
Consumer deleteApplicationRequest) {
return deleteApplication(DeleteApplicationRequest.builder().applyMutation(deleteApplicationRequest).build());
}
/**
*
* Deletes the specified version from the specified application.
*
*
*
* You cannot delete an application version that is associated with a running environment.
*
*
*
* @param deleteApplicationVersionRequest
* Request to delete an application version.
* @return A Java Future containing the result of the DeleteApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SourceBundleDeletionException Unable to delete the Amazon S3 source bundle associated with the
* application version. The application version was deleted successfully.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - S3LocationNotInServiceRegionException The specified S3 bucket does not belong to the S3 region in
* which the service is running. The following regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture deleteApplicationVersion(
DeleteApplicationVersionRequest deleteApplicationVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified version from the specified application.
*
*
*
* You cannot delete an application version that is associated with a running environment.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationVersionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteApplicationVersionRequest#builder()}
*
*
* @param deleteApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link DeleteApplicationVersionMessage.Builder} to create a
* request. Request to delete an application version.
* @return A Java Future containing the result of the DeleteApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SourceBundleDeletionException Unable to delete the Amazon S3 source bundle associated with the
* application version. The application version was deleted successfully.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - S3LocationNotInServiceRegionException The specified S3 bucket does not belong to the S3 region in
* which the service is running. The following regions are supported:
*
* -
*
* IAD/us-east-1
*
*
* -
*
* PDX/us-west-2
*
*
* -
*
* DUB/eu-west-1
*
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture deleteApplicationVersion(
Consumer deleteApplicationVersionRequest) {
return deleteApplicationVersion(DeleteApplicationVersionRequest.builder().applyMutation(deleteApplicationVersionRequest)
.build());
}
/**
*
* Deletes the specified configuration template.
*
*
*
* When you launch an environment using a configuration template, the environment gets a copy of the template. You
* can delete or modify the environment's copy of the template without affecting the running environment.
*
*
*
* @param deleteConfigurationTemplateRequest
* Request to delete a configuration template.
* @return A Java Future containing the result of the DeleteConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture deleteConfigurationTemplate(
DeleteConfigurationTemplateRequest deleteConfigurationTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified configuration template.
*
*
*
* When you launch an environment using a configuration template, the environment gets a copy of the template. You
* can delete or modify the environment's copy of the template without affecting the running environment.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConfigurationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link DeleteConfigurationTemplateRequest#builder()}
*
*
* @param deleteConfigurationTemplateRequest
* A {@link Consumer} that will call methods on {@link DeleteConfigurationTemplateMessage.Builder} to create
* a request. Request to delete a configuration template.
* @return A Java Future containing the result of the DeleteConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture deleteConfigurationTemplate(
Consumer deleteConfigurationTemplateRequest) {
return deleteConfigurationTemplate(DeleteConfigurationTemplateRequest.builder()
.applyMutation(deleteConfigurationTemplateRequest).build());
}
/**
*
* Deletes the draft configuration associated with the running environment.
*
*
* Updating a running environment with any configuration changes creates a draft configuration set. You can get the
* draft configuration using DescribeConfigurationSettings while the update is in progress or if the update
* fails. The DeploymentStatus
for the draft configuration indicates whether the deployment is in
* process or has failed. The draft configuration remains in existence until it is deleted with this action.
*
*
* @param deleteEnvironmentConfigurationRequest
* Request to delete a draft environment configuration.
* @return A Java Future containing the result of the DeleteEnvironmentConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteEnvironmentConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteEnvironmentConfiguration(
DeleteEnvironmentConfigurationRequest deleteEnvironmentConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the draft configuration associated with the running environment.
*
*
* Updating a running environment with any configuration changes creates a draft configuration set. You can get the
* draft configuration using DescribeConfigurationSettings while the update is in progress or if the update
* fails. The DeploymentStatus
for the draft configuration indicates whether the deployment is in
* process or has failed. The draft configuration remains in existence until it is deleted with this action.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEnvironmentConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteEnvironmentConfigurationRequest#builder()}
*
*
* @param deleteEnvironmentConfigurationRequest
* A {@link Consumer} that will call methods on {@link DeleteEnvironmentConfigurationMessage.Builder} to
* create a request. Request to delete a draft environment configuration.
* @return A Java Future containing the result of the DeleteEnvironmentConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeleteEnvironmentConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteEnvironmentConfiguration(
Consumer deleteEnvironmentConfigurationRequest) {
return deleteEnvironmentConfiguration(DeleteEnvironmentConfigurationRequest.builder()
.applyMutation(deleteEnvironmentConfigurationRequest).build());
}
/**
*
* Deletes the specified version of a custom platform.
*
*
* @param deletePlatformVersionRequest
* @return A Java Future containing the result of the DeletePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - PlatformVersionStillReferencedException You cannot delete the platform version because there are
* still environments running on it.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeletePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture deletePlatformVersion(
DeletePlatformVersionRequest deletePlatformVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified version of a custom platform.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePlatformVersionRequest.Builder} avoiding the
* need to create one manually via {@link DeletePlatformVersionRequest#builder()}
*
*
* @param deletePlatformVersionRequest
* A {@link Consumer} that will call methods on {@link DeletePlatformVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - PlatformVersionStillReferencedException You cannot delete the platform version because there are
* still environments running on it.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DeletePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture deletePlatformVersion(
Consumer deletePlatformVersionRequest) {
return deletePlatformVersion(DeletePlatformVersionRequest.builder().applyMutation(deletePlatformVersionRequest).build());
}
/**
*
* Returns attributes related to AWS Elastic Beanstalk that are associated with the calling AWS account.
*
*
* The result currently has one set of attributes—resource quotas.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeAccountAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeAccountAttributes(
DescribeAccountAttributesRequest describeAccountAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns attributes related to AWS Elastic Beanstalk that are associated with the calling AWS account.
*
*
* The result currently has one set of attributes—resource quotas.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccountAttributesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeAccountAttributesRequest#builder()}
*
*
* @param describeAccountAttributesRequest
* A {@link Consumer} that will call methods on {@link DescribeAccountAttributesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeAccountAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeAccountAttributes(
Consumer describeAccountAttributesRequest) {
return describeAccountAttributes(DescribeAccountAttributesRequest.builder()
.applyMutation(describeAccountAttributesRequest).build());
}
/**
*
* Returns attributes related to AWS Elastic Beanstalk that are associated with the calling AWS account.
*
*
* The result currently has one set of attributes—resource quotas.
*
*
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeAccountAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeAccountAttributes() {
return describeAccountAttributes(DescribeAccountAttributesRequest.builder().build());
}
/**
*
* Retrieve a list of application versions.
*
*
* @param describeApplicationVersionsRequest
* Request to describe application versions.
* @return A Java Future containing the result of the DescribeApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplicationVersions
* @see AWS API Documentation
*/
default CompletableFuture describeApplicationVersions(
DescribeApplicationVersionsRequest describeApplicationVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve a list of application versions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeApplicationVersionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeApplicationVersionsRequest#builder()}
*
*
* @param describeApplicationVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeApplicationVersionsMessage.Builder} to create
* a request. Request to describe application versions.
* @return A Java Future containing the result of the DescribeApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplicationVersions
* @see AWS API Documentation
*/
default CompletableFuture describeApplicationVersions(
Consumer describeApplicationVersionsRequest) {
return describeApplicationVersions(DescribeApplicationVersionsRequest.builder()
.applyMutation(describeApplicationVersionsRequest).build());
}
/**
*
* Retrieve a list of application versions.
*
*
* @return A Java Future containing the result of the DescribeApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplicationVersions
* @see AWS API Documentation
*/
default CompletableFuture describeApplicationVersions() {
return describeApplicationVersions(DescribeApplicationVersionsRequest.builder().build());
}
/**
*
* Returns the descriptions of existing applications.
*
*
* @param describeApplicationsRequest
* Request to describe one or more applications.
* @return A Java Future containing the result of the DescribeApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplications
* @see AWS API Documentation
*/
default CompletableFuture describeApplications(
DescribeApplicationsRequest describeApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the descriptions of existing applications.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeApplicationsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeApplicationsRequest#builder()}
*
*
* @param describeApplicationsRequest
* A {@link Consumer} that will call methods on {@link DescribeApplicationsMessage.Builder} to create a
* request. Request to describe one or more applications.
* @return A Java Future containing the result of the DescribeApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplications
* @see AWS API Documentation
*/
default CompletableFuture describeApplications(
Consumer describeApplicationsRequest) {
return describeApplications(DescribeApplicationsRequest.builder().applyMutation(describeApplicationsRequest).build());
}
/**
*
* Returns the descriptions of existing applications.
*
*
* @return A Java Future containing the result of the DescribeApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeApplications
* @see AWS API Documentation
*/
default CompletableFuture describeApplications() {
return describeApplications(DescribeApplicationsRequest.builder().build());
}
/**
*
* Describes the configuration options that are used in a particular configuration template or environment, or that
* a specified solution stack defines. The description includes the values the options, their default values, and an
* indication of the required action on a running environment if an option value is changed.
*
*
* @param describeConfigurationOptionsRequest
* Result message containing a list of application version descriptions.
* @return A Java Future containing the result of the DescribeConfigurationOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeConfigurationOptions
* @see AWS API Documentation
*/
default CompletableFuture describeConfigurationOptions(
DescribeConfigurationOptionsRequest describeConfigurationOptionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the configuration options that are used in a particular configuration template or environment, or that
* a specified solution stack defines. The description includes the values the options, their default values, and an
* indication of the required action on a running environment if an option value is changed.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeConfigurationOptionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeConfigurationOptionsRequest#builder()}
*
*
* @param describeConfigurationOptionsRequest
* A {@link Consumer} that will call methods on {@link DescribeConfigurationOptionsMessage.Builder} to create
* a request. Result message containing a list of application version descriptions.
* @return A Java Future containing the result of the DescribeConfigurationOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeConfigurationOptions
* @see AWS API Documentation
*/
default CompletableFuture describeConfigurationOptions(
Consumer describeConfigurationOptionsRequest) {
return describeConfigurationOptions(DescribeConfigurationOptionsRequest.builder()
.applyMutation(describeConfigurationOptionsRequest).build());
}
/**
*
* Returns a description of the settings for the specified configuration set, that is, either a configuration
* template or the configuration set associated with a running environment.
*
*
* When describing the settings for the configuration set associated with a running environment, it is possible to
* receive two sets of setting descriptions. One is the deployed configuration set, and the other is a draft
* configuration of an environment that is either in the process of deployment or that failed to deploy.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param describeConfigurationSettingsRequest
* Result message containing all of the configuration settings for a specified solution stack or
* configuration template.
* @return A Java Future containing the result of the DescribeConfigurationSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeConfigurationSettings
* @see AWS API Documentation
*/
default CompletableFuture describeConfigurationSettings(
DescribeConfigurationSettingsRequest describeConfigurationSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a description of the settings for the specified configuration set, that is, either a configuration
* template or the configuration set associated with a running environment.
*
*
* When describing the settings for the configuration set associated with a running environment, it is possible to
* receive two sets of setting descriptions. One is the deployed configuration set, and the other is a draft
* configuration of an environment that is either in the process of deployment or that failed to deploy.
*
*
* Related Topics
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeConfigurationSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeConfigurationSettingsRequest#builder()}
*
*
* @param describeConfigurationSettingsRequest
* A {@link Consumer} that will call methods on {@link DescribeConfigurationSettingsMessage.Builder} to
* create a request. Result message containing all of the configuration settings for a specified solution
* stack or configuration template.
* @return A Java Future containing the result of the DescribeConfigurationSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeConfigurationSettings
* @see AWS API Documentation
*/
default CompletableFuture describeConfigurationSettings(
Consumer describeConfigurationSettingsRequest) {
return describeConfigurationSettings(DescribeConfigurationSettingsRequest.builder()
.applyMutation(describeConfigurationSettingsRequest).build());
}
/**
*
* Returns information about the overall health of the specified environment. The DescribeEnvironmentHealth
* operation is only available with AWS Elastic Beanstalk Enhanced Health.
*
*
* @param describeEnvironmentHealthRequest
* See the example below to learn how to create a request body.
* @return A Java Future containing the result of the DescribeEnvironmentHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException One or more input parameters is not valid. Please correct the input
* parameters and try the operation again.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentHealth
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentHealth(
DescribeEnvironmentHealthRequest describeEnvironmentHealthRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the overall health of the specified environment. The DescribeEnvironmentHealth
* operation is only available with AWS Elastic Beanstalk Enhanced Health.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEnvironmentHealthRequest.Builder} avoiding
* the need to create one manually via {@link DescribeEnvironmentHealthRequest#builder()}
*
*
* @param describeEnvironmentHealthRequest
* A {@link Consumer} that will call methods on {@link DescribeEnvironmentHealthRequest.Builder} to create a
* request. See the example below to learn how to create a request body.
* @return A Java Future containing the result of the DescribeEnvironmentHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException One or more input parameters is not valid. Please correct the input
* parameters and try the operation again.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentHealth
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentHealth(
Consumer describeEnvironmentHealthRequest) {
return describeEnvironmentHealth(DescribeEnvironmentHealthRequest.builder()
.applyMutation(describeEnvironmentHealthRequest).build());
}
/**
*
* Lists an environment's completed and failed managed actions.
*
*
* @param describeEnvironmentManagedActionHistoryRequest
* Request to list completed and failed managed actions.
* @return A Java Future containing the result of the DescribeEnvironmentManagedActionHistory operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActionHistory
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentManagedActionHistory(
DescribeEnvironmentManagedActionHistoryRequest describeEnvironmentManagedActionHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists an environment's completed and failed managed actions.
*
*
*
* This is a convenience which creates an instance of the
* {@link DescribeEnvironmentManagedActionHistoryRequest.Builder} avoiding the need to create one manually via
* {@link DescribeEnvironmentManagedActionHistoryRequest#builder()}
*
*
* @param describeEnvironmentManagedActionHistoryRequest
* A {@link Consumer} that will call methods on
* {@link DescribeEnvironmentManagedActionHistoryRequest.Builder} to create a request. Request to list
* completed and failed managed actions.
* @return A Java Future containing the result of the DescribeEnvironmentManagedActionHistory operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActionHistory
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentManagedActionHistory(
Consumer describeEnvironmentManagedActionHistoryRequest) {
return describeEnvironmentManagedActionHistory(DescribeEnvironmentManagedActionHistoryRequest.builder()
.applyMutation(describeEnvironmentManagedActionHistoryRequest).build());
}
/**
*
* Lists an environment's completed and failed managed actions.
*
*
*
* This is a variant of
* {@link #describeEnvironmentManagedActionHistory(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEnvironmentManagedActionHistoryPublisher publisher = client.describeEnvironmentManagedActionHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEnvironmentManagedActionHistoryPublisher publisher = client.describeEnvironmentManagedActionHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEnvironmentManagedActionHistory(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryRequest)}
* operation.
*
*
* @param describeEnvironmentManagedActionHistoryRequest
* Request to list completed and failed managed actions.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActionHistory
* @see AWS API Documentation
*/
default DescribeEnvironmentManagedActionHistoryPublisher describeEnvironmentManagedActionHistoryPaginator(
DescribeEnvironmentManagedActionHistoryRequest describeEnvironmentManagedActionHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists an environment's completed and failed managed actions.
*
*
*
* This is a variant of
* {@link #describeEnvironmentManagedActionHistory(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEnvironmentManagedActionHistoryPublisher publisher = client.describeEnvironmentManagedActionHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEnvironmentManagedActionHistoryPublisher publisher = client.describeEnvironmentManagedActionHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxItems won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEnvironmentManagedActionHistory(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentManagedActionHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the
* {@link DescribeEnvironmentManagedActionHistoryRequest.Builder} avoiding the need to create one manually via
* {@link DescribeEnvironmentManagedActionHistoryRequest#builder()}
*
*
* @param describeEnvironmentManagedActionHistoryRequest
* A {@link Consumer} that will call methods on
* {@link DescribeEnvironmentManagedActionHistoryRequest.Builder} to create a request. Request to list
* completed and failed managed actions.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActionHistory
* @see AWS API Documentation
*/
default DescribeEnvironmentManagedActionHistoryPublisher describeEnvironmentManagedActionHistoryPaginator(
Consumer describeEnvironmentManagedActionHistoryRequest) {
return describeEnvironmentManagedActionHistoryPaginator(DescribeEnvironmentManagedActionHistoryRequest.builder()
.applyMutation(describeEnvironmentManagedActionHistoryRequest).build());
}
/**
*
* Lists an environment's upcoming and in-progress managed actions.
*
*
* @param describeEnvironmentManagedActionsRequest
* Request to list an environment's upcoming and in-progress managed actions.
* @return A Java Future containing the result of the DescribeEnvironmentManagedActions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActions
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentManagedActions(
DescribeEnvironmentManagedActionsRequest describeEnvironmentManagedActionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists an environment's upcoming and in-progress managed actions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEnvironmentManagedActionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEnvironmentManagedActionsRequest#builder()}
*
*
* @param describeEnvironmentManagedActionsRequest
* A {@link Consumer} that will call methods on {@link DescribeEnvironmentManagedActionsRequest.Builder} to
* create a request. Request to list an environment's upcoming and in-progress managed actions.
* @return A Java Future containing the result of the DescribeEnvironmentManagedActions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentManagedActions
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentManagedActions(
Consumer describeEnvironmentManagedActionsRequest) {
return describeEnvironmentManagedActions(DescribeEnvironmentManagedActionsRequest.builder()
.applyMutation(describeEnvironmentManagedActionsRequest).build());
}
/**
*
* Returns AWS resources for this environment.
*
*
* @param describeEnvironmentResourcesRequest
* Request to describe the resources in an environment.
* @return A Java Future containing the result of the DescribeEnvironmentResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentResources
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentResources(
DescribeEnvironmentResourcesRequest describeEnvironmentResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns AWS resources for this environment.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEnvironmentResourcesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEnvironmentResourcesRequest#builder()}
*
*
* @param describeEnvironmentResourcesRequest
* A {@link Consumer} that will call methods on {@link DescribeEnvironmentResourcesMessage.Builder} to create
* a request. Request to describe the resources in an environment.
* @return A Java Future containing the result of the DescribeEnvironmentResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironmentResources
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironmentResources(
Consumer describeEnvironmentResourcesRequest) {
return describeEnvironmentResources(DescribeEnvironmentResourcesRequest.builder()
.applyMutation(describeEnvironmentResourcesRequest).build());
}
/**
*
* Returns descriptions for existing environments.
*
*
* @param describeEnvironmentsRequest
* Request to describe one or more environments.
* @return A Java Future containing the result of the DescribeEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironments
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironments(
DescribeEnvironmentsRequest describeEnvironmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns descriptions for existing environments.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEnvironmentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeEnvironmentsRequest#builder()}
*
*
* @param describeEnvironmentsRequest
* A {@link Consumer} that will call methods on {@link DescribeEnvironmentsMessage.Builder} to create a
* request. Request to describe one or more environments.
* @return A Java Future containing the result of the DescribeEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironments
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironments(
Consumer describeEnvironmentsRequest) {
return describeEnvironments(DescribeEnvironmentsRequest.builder().applyMutation(describeEnvironmentsRequest).build());
}
/**
*
* Returns descriptions for existing environments.
*
*
* @return A Java Future containing the result of the DescribeEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEnvironments
* @see AWS API Documentation
*/
default CompletableFuture describeEnvironments() {
return describeEnvironments(DescribeEnvironmentsRequest.builder().build());
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* @param describeEventsRequest
* Request to retrieve a list of events for an environment.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default CompletableFuture describeEvents(DescribeEventsRequest describeEventsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEventsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeEventsRequest#builder()}
*
*
* @param describeEventsRequest
* A {@link Consumer} that will call methods on {@link DescribeEventsMessage.Builder} to create a request.
* Request to retrieve a list of events for an environment.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default CompletableFuture describeEvents(Consumer describeEventsRequest) {
return describeEvents(DescribeEventsRequest.builder().applyMutation(describeEventsRequest).build());
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default CompletableFuture describeEvents() {
return describeEvents(DescribeEventsRequest.builder().build());
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator() {
return describeEventsPaginator(DescribeEventsRequest.builder().build());
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)}
* operation.
*
*
* @param describeEventsRequest
* Request to retrieve a list of events for an environment.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator(DescribeEventsRequest describeEventsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns list of event descriptions matching criteria up to the last 6 weeks.
*
*
*
* This action returns the most recent 1,000 events from the specified NextToken
.
*
*
*
* This is a variant of
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.DescribeEventsPublisher publisher = client.describeEventsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEvents(software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeEventsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeEventsRequest#builder()}
*
*
* @param describeEventsRequest
* A {@link Consumer} that will call methods on {@link DescribeEventsMessage.Builder} to create a request.
* Request to retrieve a list of events for an environment.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeEvents
* @see AWS API Documentation
*/
default DescribeEventsPublisher describeEventsPaginator(Consumer describeEventsRequest) {
return describeEventsPaginator(DescribeEventsRequest.builder().applyMutation(describeEventsRequest).build());
}
/**
*
* Retrieves detailed information about the health of instances in your AWS Elastic Beanstalk. This operation
* requires enhanced health
* reporting.
*
*
* @param describeInstancesHealthRequest
* Parameters for a call to DescribeInstancesHealth
.
* @return A Java Future containing the result of the DescribeInstancesHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException One or more input parameters is not valid. Please correct the input
* parameters and try the operation again.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeInstancesHealth
* @see AWS API Documentation
*/
default CompletableFuture describeInstancesHealth(
DescribeInstancesHealthRequest describeInstancesHealthRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves detailed information about the health of instances in your AWS Elastic Beanstalk. This operation
* requires enhanced health
* reporting.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstancesHealthRequest.Builder} avoiding
* the need to create one manually via {@link DescribeInstancesHealthRequest#builder()}
*
*
* @param describeInstancesHealthRequest
* A {@link Consumer} that will call methods on {@link DescribeInstancesHealthRequest.Builder} to create a
* request. Parameters for a call to DescribeInstancesHealth
.
* @return A Java Future containing the result of the DescribeInstancesHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException One or more input parameters is not valid. Please correct the input
* parameters and try the operation again.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribeInstancesHealth
* @see AWS API Documentation
*/
default CompletableFuture describeInstancesHealth(
Consumer describeInstancesHealthRequest) {
return describeInstancesHealth(DescribeInstancesHealthRequest.builder().applyMutation(describeInstancesHealthRequest)
.build());
}
/**
*
* Describes a platform version. Provides full details. Compare to ListPlatformVersions, which provides
* summary information about a list of platform versions.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param describePlatformVersionRequest
* @return A Java Future containing the result of the DescribePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture describePlatformVersion(
DescribePlatformVersionRequest describePlatformVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a platform version. Provides full details. Compare to ListPlatformVersions, which provides
* summary information about a list of platform versions.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePlatformVersionRequest.Builder} avoiding
* the need to create one manually via {@link DescribePlatformVersionRequest#builder()}
*
*
* @param describePlatformVersionRequest
* A {@link Consumer} that will call methods on {@link DescribePlatformVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribePlatformVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DescribePlatformVersion
* @see AWS API Documentation
*/
default CompletableFuture describePlatformVersion(
Consumer describePlatformVersionRequest) {
return describePlatformVersion(DescribePlatformVersionRequest.builder().applyMutation(describePlatformVersionRequest)
.build());
}
/**
*
* Disassociate the operations role from an environment. After this call is made, Elastic Beanstalk uses the
* caller's permissions for permissions to downstream services during subsequent calls acting on this environment.
* For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @param disassociateEnvironmentOperationsRoleRequest
* Request to disassociate the operations role from an environment.
* @return A Java Future containing the result of the DisassociateEnvironmentOperationsRole operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DisassociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
default CompletableFuture disassociateEnvironmentOperationsRole(
DisassociateEnvironmentOperationsRoleRequest disassociateEnvironmentOperationsRoleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociate the operations role from an environment. After this call is made, Elastic Beanstalk uses the
* caller's permissions for permissions to downstream services during subsequent calls acting on this environment.
* For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
*
* This is a convenience which creates an instance of the
* {@link DisassociateEnvironmentOperationsRoleRequest.Builder} avoiding the need to create one manually via
* {@link DisassociateEnvironmentOperationsRoleRequest#builder()}
*
*
* @param disassociateEnvironmentOperationsRoleRequest
* A {@link Consumer} that will call methods on {@link DisassociateEnvironmentOperationsRoleMessage.Builder}
* to create a request. Request to disassociate the operations role from an environment.
* @return A Java Future containing the result of the DisassociateEnvironmentOperationsRole operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.DisassociateEnvironmentOperationsRole
* @see AWS API Documentation
*/
default CompletableFuture disassociateEnvironmentOperationsRole(
Consumer disassociateEnvironmentOperationsRoleRequest) {
return disassociateEnvironmentOperationsRole(DisassociateEnvironmentOperationsRoleRequest.builder()
.applyMutation(disassociateEnvironmentOperationsRoleRequest).build());
}
/**
*
* Returns a list of the available solution stack names, with the public version first and then in reverse
* chronological order.
*
*
* @param listAvailableSolutionStacksRequest
* @return A Java Future containing the result of the ListAvailableSolutionStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListAvailableSolutionStacks
* @see AWS API Documentation
*/
default CompletableFuture listAvailableSolutionStacks(
ListAvailableSolutionStacksRequest listAvailableSolutionStacksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the available solution stack names, with the public version first and then in reverse
* chronological order.
*
*
*
* This is a convenience which creates an instance of the {@link ListAvailableSolutionStacksRequest.Builder}
* avoiding the need to create one manually via {@link ListAvailableSolutionStacksRequest#builder()}
*
*
* @param listAvailableSolutionStacksRequest
* A {@link Consumer} that will call methods on {@link ListAvailableSolutionStacksRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListAvailableSolutionStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListAvailableSolutionStacks
* @see AWS API Documentation
*/
default CompletableFuture listAvailableSolutionStacks(
Consumer listAvailableSolutionStacksRequest) {
return listAvailableSolutionStacks(ListAvailableSolutionStacksRequest.builder()
.applyMutation(listAvailableSolutionStacksRequest).build());
}
/**
*
* Returns a list of the available solution stack names, with the public version first and then in reverse
* chronological order.
*
*
* @return A Java Future containing the result of the ListAvailableSolutionStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListAvailableSolutionStacks
* @see AWS API Documentation
*/
default CompletableFuture listAvailableSolutionStacks() {
return listAvailableSolutionStacks(ListAvailableSolutionStacksRequest.builder().build());
}
/**
*
* Lists the platform branches available for your account in an AWS Region. Provides summary information about each
* platform branch.
*
*
* For definitions of platform branch and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param listPlatformBranchesRequest
* @return A Java Future containing the result of the ListPlatformBranches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformBranches
* @see AWS API Documentation
*/
default CompletableFuture listPlatformBranches(
ListPlatformBranchesRequest listPlatformBranchesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the platform branches available for your account in an AWS Region. Provides summary information about each
* platform branch.
*
*
* For definitions of platform branch and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a convenience which creates an instance of the {@link ListPlatformBranchesRequest.Builder} avoiding the
* need to create one manually via {@link ListPlatformBranchesRequest#builder()}
*
*
* @param listPlatformBranchesRequest
* A {@link Consumer} that will call methods on {@link ListPlatformBranchesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPlatformBranches operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformBranches
* @see AWS API Documentation
*/
default CompletableFuture listPlatformBranches(
Consumer listPlatformBranchesRequest) {
return listPlatformBranches(ListPlatformBranchesRequest.builder().applyMutation(listPlatformBranchesRequest).build());
}
/**
*
* Lists the platform branches available for your account in an AWS Region. Provides summary information about each
* platform branch.
*
*
* For definitions of platform branch and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a variant of
* {@link #listPlatformBranches(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformBranchesPublisher publisher = client.listPlatformBranchesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformBranchesPublisher publisher = client.listPlatformBranchesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlatformBranches(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesRequest)}
* operation.
*
*
* @param listPlatformBranchesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformBranches
* @see AWS API Documentation
*/
default ListPlatformBranchesPublisher listPlatformBranchesPaginator(ListPlatformBranchesRequest listPlatformBranchesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the platform branches available for your account in an AWS Region. Provides summary information about each
* platform branch.
*
*
* For definitions of platform branch and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a variant of
* {@link #listPlatformBranches(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformBranchesPublisher publisher = client.listPlatformBranchesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformBranchesPublisher publisher = client.listPlatformBranchesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlatformBranches(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformBranchesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPlatformBranchesRequest.Builder} avoiding the
* need to create one manually via {@link ListPlatformBranchesRequest#builder()}
*
*
* @param listPlatformBranchesRequest
* A {@link Consumer} that will call methods on {@link ListPlatformBranchesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformBranches
* @see AWS API Documentation
*/
default ListPlatformBranchesPublisher listPlatformBranchesPaginator(
Consumer listPlatformBranchesRequest) {
return listPlatformBranchesPaginator(ListPlatformBranchesRequest.builder().applyMutation(listPlatformBranchesRequest)
.build());
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @param listPlatformVersionsRequest
* @return A Java Future containing the result of the ListPlatformVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default CompletableFuture listPlatformVersions(
ListPlatformVersionsRequest listPlatformVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a convenience which creates an instance of the {@link ListPlatformVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPlatformVersionsRequest#builder()}
*
*
* @param listPlatformVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPlatformVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPlatformVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default CompletableFuture listPlatformVersions(
Consumer listPlatformVersionsRequest) {
return listPlatformVersions(ListPlatformVersionsRequest.builder().applyMutation(listPlatformVersionsRequest).build());
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
* @return A Java Future containing the result of the ListPlatformVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default CompletableFuture listPlatformVersions() {
return listPlatformVersions(ListPlatformVersionsRequest.builder().build());
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a variant of
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default ListPlatformVersionsPublisher listPlatformVersionsPaginator() {
return listPlatformVersionsPaginator(ListPlatformVersionsRequest.builder().build());
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a variant of
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation.
*
*
* @param listPlatformVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default ListPlatformVersionsPublisher listPlatformVersionsPaginator(ListPlatformVersionsRequest listPlatformVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the platform versions available for your account in an AWS Region. Provides summary information about each
* platform version. Compare to DescribePlatformVersion, which provides full details about a single platform
* version.
*
*
* For definitions of platform version and other platform-related terms, see AWS Elastic Beanstalk
* Platforms Glossary.
*
*
*
* This is a variant of
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticbeanstalk.paginators.ListPlatformVersionsPublisher publisher = client.listPlatformVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlatformVersions(software.amazon.awssdk.services.elasticbeanstalk.model.ListPlatformVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPlatformVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPlatformVersionsRequest#builder()}
*
*
* @param listPlatformVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPlatformVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ElasticBeanstalkServiceException A generic service exception has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListPlatformVersions
* @see AWS API Documentation
*/
default ListPlatformVersionsPublisher listPlatformVersionsPaginator(
Consumer listPlatformVersionsRequest) {
return listPlatformVersionsPaginator(ListPlatformVersionsRequest.builder().applyMutation(listPlatformVersionsRequest)
.build());
}
/**
*
* Return the tags applied to an AWS Elastic Beanstalk resource. The response contains a list of tag key-value
* pairs.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ResourceNotFoundException A resource doesn't exist for the specified Amazon Resource Name (ARN).
* - ResourceTypeNotSupportedException The type of the specified Amazon Resource Name (ARN) isn't
* supported for this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Return the tags applied to an AWS Elastic Beanstalk resource. The response contains a list of tag key-value
* pairs.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - ResourceNotFoundException A resource doesn't exist for the specified Amazon Resource Name (ARN).
* - ResourceTypeNotSupportedException The type of the specified Amazon Resource Name (ARN) isn't
* supported for this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Deletes and recreates all of the AWS resources (for example: the Auto Scaling group, load balancer, etc.) for a
* specified environment and forces a restart.
*
*
* @param rebuildEnvironmentRequest
* @return A Java Future containing the result of the RebuildEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RebuildEnvironment
* @see AWS API Documentation
*/
default CompletableFuture rebuildEnvironment(RebuildEnvironmentRequest rebuildEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes and recreates all of the AWS resources (for example: the Auto Scaling group, load balancer, etc.) for a
* specified environment and forces a restart.
*
*
*
* This is a convenience which creates an instance of the {@link RebuildEnvironmentRequest.Builder} avoiding the
* need to create one manually via {@link RebuildEnvironmentRequest#builder()}
*
*
* @param rebuildEnvironmentRequest
* A {@link Consumer} that will call methods on {@link RebuildEnvironmentMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the RebuildEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RebuildEnvironment
* @see AWS API Documentation
*/
default CompletableFuture rebuildEnvironment(
Consumer rebuildEnvironmentRequest) {
return rebuildEnvironment(RebuildEnvironmentRequest.builder().applyMutation(rebuildEnvironmentRequest).build());
}
/**
*
* Initiates a request to compile the specified type of information of the deployed environment.
*
*
* Setting the InfoType
to tail
compiles the last lines from the application server log
* files of every Amazon EC2 instance in your environment.
*
*
* Setting the InfoType
to bundle
compresses the application server log files for every
* Amazon EC2 instance into a .zip
file. Legacy and .NET containers do not support bundle logs.
*
*
* Use RetrieveEnvironmentInfo to obtain the set of logs.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param requestEnvironmentInfoRequest
* Request to retrieve logs from an environment and store them in your Elastic Beanstalk storage bucket.
* @return A Java Future containing the result of the RequestEnvironmentInfo operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RequestEnvironmentInfo
* @see AWS API Documentation
*/
default CompletableFuture requestEnvironmentInfo(
RequestEnvironmentInfoRequest requestEnvironmentInfoRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Initiates a request to compile the specified type of information of the deployed environment.
*
*
* Setting the InfoType
to tail
compiles the last lines from the application server log
* files of every Amazon EC2 instance in your environment.
*
*
* Setting the InfoType
to bundle
compresses the application server log files for every
* Amazon EC2 instance into a .zip
file. Legacy and .NET containers do not support bundle logs.
*
*
* Use RetrieveEnvironmentInfo to obtain the set of logs.
*
*
* Related Topics
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link RequestEnvironmentInfoRequest.Builder} avoiding the
* need to create one manually via {@link RequestEnvironmentInfoRequest#builder()}
*
*
* @param requestEnvironmentInfoRequest
* A {@link Consumer} that will call methods on {@link RequestEnvironmentInfoMessage.Builder} to create a
* request. Request to retrieve logs from an environment and store them in your Elastic Beanstalk storage
* bucket.
* @return A Java Future containing the result of the RequestEnvironmentInfo operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RequestEnvironmentInfo
* @see AWS API Documentation
*/
default CompletableFuture requestEnvironmentInfo(
Consumer requestEnvironmentInfoRequest) {
return requestEnvironmentInfo(RequestEnvironmentInfoRequest.builder().applyMutation(requestEnvironmentInfoRequest)
.build());
}
/**
*
* Causes the environment to restart the application container server running on each Amazon EC2 instance.
*
*
* @param restartAppServerRequest
* @return A Java Future containing the result of the RestartAppServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RestartAppServer
* @see AWS API Documentation
*/
default CompletableFuture restartAppServer(RestartAppServerRequest restartAppServerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Causes the environment to restart the application container server running on each Amazon EC2 instance.
*
*
*
* This is a convenience which creates an instance of the {@link RestartAppServerRequest.Builder} avoiding the need
* to create one manually via {@link RestartAppServerRequest#builder()}
*
*
* @param restartAppServerRequest
* A {@link Consumer} that will call methods on {@link RestartAppServerMessage.Builder} to create a request.
* @return A Java Future containing the result of the RestartAppServer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RestartAppServer
* @see AWS API Documentation
*/
default CompletableFuture restartAppServer(
Consumer restartAppServerRequest) {
return restartAppServer(RestartAppServerRequest.builder().applyMutation(restartAppServerRequest).build());
}
/**
*
* Retrieves the compiled information from a RequestEnvironmentInfo request.
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param retrieveEnvironmentInfoRequest
* Request to download logs retrieved with RequestEnvironmentInfo.
* @return A Java Future containing the result of the RetrieveEnvironmentInfo operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RetrieveEnvironmentInfo
* @see AWS API Documentation
*/
default CompletableFuture retrieveEnvironmentInfo(
RetrieveEnvironmentInfoRequest retrieveEnvironmentInfoRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the compiled information from a RequestEnvironmentInfo request.
*
*
* Related Topics
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link RetrieveEnvironmentInfoRequest.Builder} avoiding
* the need to create one manually via {@link RetrieveEnvironmentInfoRequest#builder()}
*
*
* @param retrieveEnvironmentInfoRequest
* A {@link Consumer} that will call methods on {@link RetrieveEnvironmentInfoMessage.Builder} to create a
* request. Request to download logs retrieved with RequestEnvironmentInfo.
* @return A Java Future containing the result of the RetrieveEnvironmentInfo operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.RetrieveEnvironmentInfo
* @see AWS API Documentation
*/
default CompletableFuture retrieveEnvironmentInfo(
Consumer retrieveEnvironmentInfoRequest) {
return retrieveEnvironmentInfo(RetrieveEnvironmentInfoRequest.builder().applyMutation(retrieveEnvironmentInfoRequest)
.build());
}
/**
*
* Swaps the CNAMEs of two environments.
*
*
* @param swapEnvironmentCnamEsRequest
* Swaps the CNAMEs of two environments.
* @return A Java Future containing the result of the SwapEnvironmentCNAMEs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.SwapEnvironmentCNAMEs
* @see AWS API Documentation
*/
default CompletableFuture swapEnvironmentCNAMEs(
SwapEnvironmentCnamEsRequest swapEnvironmentCnamEsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Swaps the CNAMEs of two environments.
*
*
*
* This is a convenience which creates an instance of the {@link SwapEnvironmentCnamEsRequest.Builder} avoiding the
* need to create one manually via {@link SwapEnvironmentCnamEsRequest#builder()}
*
*
* @param swapEnvironmentCnamEsRequest
* A {@link Consumer} that will call methods on {@link SwapEnvironmentCNAMEsMessage.Builder} to create a
* request. Swaps the CNAMEs of two environments.
* @return A Java Future containing the result of the SwapEnvironmentCNAMEs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.SwapEnvironmentCNAMEs
* @see AWS API Documentation
*/
default CompletableFuture swapEnvironmentCNAMEs(
Consumer swapEnvironmentCnamEsRequest) {
return swapEnvironmentCNAMEs(SwapEnvironmentCnamEsRequest.builder().applyMutation(swapEnvironmentCnamEsRequest).build());
}
/**
*
* Terminates the specified environment.
*
*
* @param terminateEnvironmentRequest
* Request to terminate an environment.
* @return A Java Future containing the result of the TerminateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.TerminateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture terminateEnvironment(
TerminateEnvironmentRequest terminateEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Terminates the specified environment.
*
*
*
* This is a convenience which creates an instance of the {@link TerminateEnvironmentRequest.Builder} avoiding the
* need to create one manually via {@link TerminateEnvironmentRequest#builder()}
*
*
* @param terminateEnvironmentRequest
* A {@link Consumer} that will call methods on {@link TerminateEnvironmentMessage.Builder} to create a
* request. Request to terminate an environment.
* @return A Java Future containing the result of the TerminateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.TerminateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture terminateEnvironment(
Consumer terminateEnvironmentRequest) {
return terminateEnvironment(TerminateEnvironmentRequest.builder().applyMutation(terminateEnvironmentRequest).build());
}
/**
*
* Updates the specified application to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* these properties, specify an empty string.
*
*
*
* @param updateApplicationRequest
* Request to update an application.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified application to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* these properties, specify an empty string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationMessage.Builder} to create a request.
* Request to update an application.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(
Consumer updateApplicationRequest) {
return updateApplication(UpdateApplicationRequest.builder().applyMutation(updateApplicationRequest).build());
}
/**
*
* Modifies lifecycle settings for an application.
*
*
* @param updateApplicationResourceLifecycleRequest
* @return A Java Future containing the result of the UpdateApplicationResourceLifecycle operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplicationResourceLifecycle
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationResourceLifecycle(
UpdateApplicationResourceLifecycleRequest updateApplicationResourceLifecycleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies lifecycle settings for an application.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationResourceLifecycleRequest.Builder}
* avoiding the need to create one manually via {@link UpdateApplicationResourceLifecycleRequest#builder()}
*
*
* @param updateApplicationResourceLifecycleRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationResourceLifecycleMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateApplicationResourceLifecycle operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplicationResourceLifecycle
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationResourceLifecycle(
Consumer updateApplicationResourceLifecycleRequest) {
return updateApplicationResourceLifecycle(UpdateApplicationResourceLifecycleRequest.builder()
.applyMutation(updateApplicationResourceLifecycleRequest).build());
}
/**
*
* Updates the specified application version to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* properties, specify an empty string.
*
*
*
* @param updateApplicationVersionRequest
* @return A Java Future containing the result of the UpdateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationVersion(
UpdateApplicationVersionRequest updateApplicationVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified application version to have the specified properties.
*
*
*
* If a property (for example, description
) is not provided, the value remains unchanged. To clear
* properties, specify an empty string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationVersionRequest.Builder} avoiding
* the need to create one manually via {@link UpdateApplicationVersionRequest#builder()}
*
*
* @param updateApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationVersionMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationVersion(
Consumer updateApplicationVersionRequest) {
return updateApplicationVersion(UpdateApplicationVersionRequest.builder().applyMutation(updateApplicationVersionRequest)
.build());
}
/**
*
* Updates the specified configuration template to have the specified properties or configuration option values.
*
*
*
* If a property (for example, ApplicationName
) is not provided, its value remains unchanged. To clear
* such properties, specify an empty string.
*
*
*
* Related Topics
*
*
* -
*
*
*
*
* @param updateConfigurationTemplateRequest
* The result message containing the options for the specified solution stack.
* @return A Java Future containing the result of the UpdateConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture updateConfigurationTemplate(
UpdateConfigurationTemplateRequest updateConfigurationTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified configuration template to have the specified properties or configuration option values.
*
*
*
* If a property (for example, ApplicationName
) is not provided, its value remains unchanged. To clear
* such properties, specify an empty string.
*
*
*
* Related Topics
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateConfigurationTemplateRequest.Builder}
* avoiding the need to create one manually via {@link UpdateConfigurationTemplateRequest#builder()}
*
*
* @param updateConfigurationTemplateRequest
* A {@link Consumer} that will call methods on {@link UpdateConfigurationTemplateMessage.Builder} to create
* a request. The result message containing the options for the specified solution stack.
* @return A Java Future containing the result of the UpdateConfigurationTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateConfigurationTemplate
* @see AWS API Documentation
*/
default CompletableFuture updateConfigurationTemplate(
Consumer updateConfigurationTemplateRequest) {
return updateConfigurationTemplate(UpdateConfigurationTemplateRequest.builder()
.applyMutation(updateConfigurationTemplateRequest).build());
}
/**
*
* Updates the environment description, deploys a new application version, updates the configuration settings to an
* entirely new configuration template, or updates select configuration option values in the running environment.
*
*
* Attempting to update both the release and configuration is not allowed and AWS Elastic Beanstalk returns an
* InvalidParameterCombination
error.
*
*
* When updating the configuration settings to a new template or individual settings, a draft configuration is
* created and DescribeConfigurationSettings for this environment returns two setting descriptions with
* different DeploymentStatus
values.
*
*
* @param updateEnvironmentRequest
* Request to update an environment.
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the environment description, deploys a new application version, updates the configuration settings to an
* entirely new configuration template, or updates select configuration option values in the running environment.
*
*
* Attempting to update both the release and configuration is not allowed and AWS Elastic Beanstalk returns an
* InvalidParameterCombination
error.
*
*
* When updating the configuration settings to a new template or individual settings, a draft configuration is
* created and DescribeConfigurationSettings for this environment returns two setting descriptions with
* different DeploymentStatus
values.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link UpdateEnvironmentRequest#builder()}
*
*
* @param updateEnvironmentRequest
* A {@link Consumer} that will call methods on {@link UpdateEnvironmentMessage.Builder} to create a request.
* Request to update an environment.
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture updateEnvironment(
Consumer updateEnvironmentRequest) {
return updateEnvironment(UpdateEnvironmentRequest.builder().applyMutation(updateEnvironmentRequest).build());
}
/**
*
* Update the list of tags applied to an AWS Elastic Beanstalk resource. Two lists can be passed:
* TagsToAdd
for tags to add or update, and TagsToRemove
.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
* If you create a custom IAM user policy to control permission to this operation, specify one of the following two
* virtual actions (or both) instead of the API operation name:
*
*
* - elasticbeanstalk:AddTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tags to add in the
* TagsToAdd
parameter.
*
*
* - elasticbeanstalk:RemoveTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tag keys to remove in the
* TagsToRemove
parameter.
*
*
*
*
* For details about creating a custom user policy, see Creating a Custom User Policy.
*
*
* @param updateTagsForResourceRequest
* @return A Java Future containing the result of the UpdateTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - TooManyTagsException The number of tags in the resource would exceed the number of tags that each
* resource can have.
*
* To calculate this, the operation considers both the number of tags the resource already has and the tags
* this operation would add if it succeeded.
* - ResourceNotFoundException A resource doesn't exist for the specified Amazon Resource Name (ARN).
* - ResourceTypeNotSupportedException The type of the specified Amazon Resource Name (ARN) isn't
* supported for this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture updateTagsForResource(
UpdateTagsForResourceRequest updateTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Update the list of tags applied to an AWS Elastic Beanstalk resource. Two lists can be passed:
* TagsToAdd
for tags to add or update, and TagsToRemove
.
*
*
* Elastic Beanstalk supports tagging of all of its resources. For details about resource tagging, see Tagging
* Application Resources.
*
*
* If you create a custom IAM user policy to control permission to this operation, specify one of the following two
* virtual actions (or both) instead of the API operation name:
*
*
* - elasticbeanstalk:AddTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tags to add in the
* TagsToAdd
parameter.
*
*
* - elasticbeanstalk:RemoveTags
* -
*
* Controls permission to call UpdateTagsForResource
and pass a list of tag keys to remove in the
* TagsToRemove
parameter.
*
*
*
*
* For details about creating a custom user policy, see Creating a Custom User Policy.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link UpdateTagsForResourceRequest#builder()}
*
*
* @param updateTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link UpdateTagsForResourceMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - OperationInProgressException Unable to perform the specified operation because another operation that
* effects an element in this activity is already in progress.
* - TooManyTagsException The number of tags in the resource would exceed the number of tags that each
* resource can have.
*
* To calculate this, the operation considers both the number of tags the resource already has and the tags
* this operation would add if it succeeded.
* - ResourceNotFoundException A resource doesn't exist for the specified Amazon Resource Name (ARN).
* - ResourceTypeNotSupportedException The type of the specified Amazon Resource Name (ARN) isn't
* supported for this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.UpdateTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture updateTagsForResource(
Consumer updateTagsForResourceRequest) {
return updateTagsForResource(UpdateTagsForResourceRequest.builder().applyMutation(updateTagsForResourceRequest).build());
}
/**
*
* Takes a set of configuration settings and either a configuration template or environment, and determines whether
* those values are valid.
*
*
* This action returns a list of messages indicating any errors or warnings associated with the selection of option
* values.
*
*
* @param validateConfigurationSettingsRequest
* A list of validation messages for a specified configuration template.
* @return A Java Future containing the result of the ValidateConfigurationSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ValidateConfigurationSettings
* @see AWS API Documentation
*/
default CompletableFuture validateConfigurationSettings(
ValidateConfigurationSettingsRequest validateConfigurationSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Takes a set of configuration settings and either a configuration template or environment, and determines whether
* those values are valid.
*
*
* This action returns a list of messages indicating any errors or warnings associated with the selection of option
* values.
*
*
*
* This is a convenience which creates an instance of the {@link ValidateConfigurationSettingsRequest.Builder}
* avoiding the need to create one manually via {@link ValidateConfigurationSettingsRequest#builder()}
*
*
* @param validateConfigurationSettingsRequest
* A {@link Consumer} that will call methods on {@link ValidateConfigurationSettingsMessage.Builder} to
* create a request. A list of validation messages for a specified configuration template.
* @return A Java Future containing the result of the ValidateConfigurationSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InsufficientPrivilegesException The specified account does not have sufficient privileges for one or
* more AWS services.
* - TooManyBucketsException The specified account has reached its limit of Amazon S3 buckets.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticBeanstalkException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ElasticBeanstalkAsyncClient.ValidateConfigurationSettings
* @see AWS API Documentation
*/
default CompletableFuture validateConfigurationSettings(
Consumer validateConfigurationSettingsRequest) {
return validateConfigurationSettings(ValidateConfigurationSettingsRequest.builder()
.applyMutation(validateConfigurationSettingsRequest).build());
}
/**
* Create an instance of {@link ElasticBeanstalkAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link ElasticBeanstalkAsyncWaiter}
*/
default ElasticBeanstalkAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
}