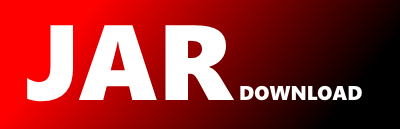
software.amazon.awssdk.services.elasticbeanstalk.model.CreateEnvironmentResponse Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the properties of an environment.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateEnvironmentResponse extends ElasticBeanstalkResponse implements
ToCopyableBuilder {
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(CreateEnvironmentResponse::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentId").getter(getter(CreateEnvironmentResponse::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentId").build()).build();
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(CreateEnvironmentResponse::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(CreateEnvironmentResponse::versionLabel))
.setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final SdkField SOLUTION_STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SolutionStackName").getter(getter(CreateEnvironmentResponse::solutionStackName))
.setter(setter(Builder::solutionStackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SolutionStackName").build()).build();
private static final SdkField PLATFORM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformArn").getter(getter(CreateEnvironmentResponse::platformArn))
.setter(setter(Builder::platformArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformArn").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(CreateEnvironmentResponse::templateName))
.setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateEnvironmentResponse::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField ENDPOINT_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointURL").getter(getter(CreateEnvironmentResponse::endpointURL))
.setter(setter(Builder::endpointURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointURL").build()).build();
private static final SdkField CNAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("CNAME")
.getter(getter(CreateEnvironmentResponse::cname)).setter(setter(Builder::cname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CNAME").build()).build();
private static final SdkField DATE_CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DateCreated").getter(getter(CreateEnvironmentResponse::dateCreated))
.setter(setter(Builder::dateCreated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateCreated").build()).build();
private static final SdkField DATE_UPDATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DateUpdated").getter(getter(CreateEnvironmentResponse::dateUpdated))
.setter(setter(Builder::dateUpdated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateUpdated").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(CreateEnvironmentResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField ABORTABLE_OPERATION_IN_PROGRESS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AbortableOperationInProgress")
.getter(getter(CreateEnvironmentResponse::abortableOperationInProgress))
.setter(setter(Builder::abortableOperationInProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AbortableOperationInProgress")
.build()).build();
private static final SdkField HEALTH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Health")
.getter(getter(CreateEnvironmentResponse::healthAsString)).setter(setter(Builder::health))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Health").build()).build();
private static final SdkField HEALTH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthStatus").getter(getter(CreateEnvironmentResponse::healthStatusAsString))
.setter(setter(Builder::healthStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthStatus").build()).build();
private static final SdkField RESOURCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Resources")
.getter(getter(CreateEnvironmentResponse::resources)).setter(setter(Builder::resources))
.constructor(EnvironmentResourcesDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Resources").build()).build();
private static final SdkField TIER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Tier").getter(getter(CreateEnvironmentResponse::tier)).setter(setter(Builder::tier))
.constructor(EnvironmentTier::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier").build()).build();
private static final SdkField> ENVIRONMENT_LINKS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EnvironmentLinks")
.getter(getter(CreateEnvironmentResponse::environmentLinks))
.setter(setter(Builder::environmentLinks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentLinks").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EnvironmentLink::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENVIRONMENT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentArn").getter(getter(CreateEnvironmentResponse::environmentArn))
.setter(setter(Builder::environmentArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentArn").build()).build();
private static final SdkField OPERATIONS_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationsRole").getter(getter(CreateEnvironmentResponse::operationsRole))
.setter(setter(Builder::operationsRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationsRole").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENVIRONMENT_NAME_FIELD,
ENVIRONMENT_ID_FIELD, APPLICATION_NAME_FIELD, VERSION_LABEL_FIELD, SOLUTION_STACK_NAME_FIELD, PLATFORM_ARN_FIELD,
TEMPLATE_NAME_FIELD, DESCRIPTION_FIELD, ENDPOINT_URL_FIELD, CNAME_FIELD, DATE_CREATED_FIELD, DATE_UPDATED_FIELD,
STATUS_FIELD, ABORTABLE_OPERATION_IN_PROGRESS_FIELD, HEALTH_FIELD, HEALTH_STATUS_FIELD, RESOURCES_FIELD, TIER_FIELD,
ENVIRONMENT_LINKS_FIELD, ENVIRONMENT_ARN_FIELD, OPERATIONS_ROLE_FIELD));
private final String environmentName;
private final String environmentId;
private final String applicationName;
private final String versionLabel;
private final String solutionStackName;
private final String platformArn;
private final String templateName;
private final String description;
private final String endpointURL;
private final String cname;
private final Instant dateCreated;
private final Instant dateUpdated;
private final String status;
private final Boolean abortableOperationInProgress;
private final String health;
private final String healthStatus;
private final EnvironmentResourcesDescription resources;
private final EnvironmentTier tier;
private final List environmentLinks;
private final String environmentArn;
private final String operationsRole;
private CreateEnvironmentResponse(BuilderImpl builder) {
super(builder);
this.environmentName = builder.environmentName;
this.environmentId = builder.environmentId;
this.applicationName = builder.applicationName;
this.versionLabel = builder.versionLabel;
this.solutionStackName = builder.solutionStackName;
this.platformArn = builder.platformArn;
this.templateName = builder.templateName;
this.description = builder.description;
this.endpointURL = builder.endpointURL;
this.cname = builder.cname;
this.dateCreated = builder.dateCreated;
this.dateUpdated = builder.dateUpdated;
this.status = builder.status;
this.abortableOperationInProgress = builder.abortableOperationInProgress;
this.health = builder.health;
this.healthStatus = builder.healthStatus;
this.resources = builder.resources;
this.tier = builder.tier;
this.environmentLinks = builder.environmentLinks;
this.environmentArn = builder.environmentArn;
this.operationsRole = builder.operationsRole;
}
/**
*
* The name of this environment.
*
*
* @return The name of this environment.
*/
public final String environmentName() {
return environmentName;
}
/**
*
* The ID of this environment.
*
*
* @return The ID of this environment.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* The name of the application associated with this environment.
*
*
* @return The name of the application associated with this environment.
*/
public final String applicationName() {
return applicationName;
}
/**
*
* The application version deployed in this environment.
*
*
* @return The application version deployed in this environment.
*/
public final String versionLabel() {
return versionLabel;
}
/**
*
* The name of the SolutionStack
deployed with this environment.
*
*
* @return The name of the SolutionStack
deployed with this environment.
*/
public final String solutionStackName() {
return solutionStackName;
}
/**
*
* The ARN of the platform version.
*
*
* @return The ARN of the platform version.
*/
public final String platformArn() {
return platformArn;
}
/**
*
* The name of the configuration template used to originally launch this environment.
*
*
* @return The name of the configuration template used to originally launch this environment.
*/
public final String templateName() {
return templateName;
}
/**
*
* Describes this environment.
*
*
* @return Describes this environment.
*/
public final String description() {
return description;
}
/**
*
* For load-balanced, autoscaling environments, the URL to the LoadBalancer. For single-instance environments, the
* IP address of the instance.
*
*
* @return For load-balanced, autoscaling environments, the URL to the LoadBalancer. For single-instance
* environments, the IP address of the instance.
*/
public final String endpointURL() {
return endpointURL;
}
/**
*
* The URL to the CNAME for this environment.
*
*
* @return The URL to the CNAME for this environment.
*/
public final String cname() {
return cname;
}
/**
*
* The creation date for this environment.
*
*
* @return The creation date for this environment.
*/
public final Instant dateCreated() {
return dateCreated;
}
/**
*
* The last modified date for this environment.
*
*
* @return The last modified date for this environment.
*/
public final Instant dateUpdated() {
return dateUpdated;
}
/**
*
* The current operational status of the environment:
*
*
* -
*
* Launching
: Environment is in the process of initial deployment.
*
*
* -
*
* Updating
: Environment is in the process of updating its configuration settings or application
* version.
*
*
* -
*
* Ready
: Environment is available to have an action performed on it, such as update or terminate.
*
*
* -
*
* Terminating
: Environment is in the shut-down process.
*
*
* -
*
* Terminated
: Environment is not running.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EnvironmentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current operational status of the environment:
*
* -
*
* Launching
: Environment is in the process of initial deployment.
*
*
* -
*
* Updating
: Environment is in the process of updating its configuration settings or
* application version.
*
*
* -
*
* Ready
: Environment is available to have an action performed on it, such as update or
* terminate.
*
*
* -
*
* Terminating
: Environment is in the shut-down process.
*
*
* -
*
* Terminated
: Environment is not running.
*
*
* @see EnvironmentStatus
*/
public final EnvironmentStatus status() {
return EnvironmentStatus.fromValue(status);
}
/**
*
* The current operational status of the environment:
*
*
* -
*
* Launching
: Environment is in the process of initial deployment.
*
*
* -
*
* Updating
: Environment is in the process of updating its configuration settings or application
* version.
*
*
* -
*
* Ready
: Environment is available to have an action performed on it, such as update or terminate.
*
*
* -
*
* Terminating
: Environment is in the shut-down process.
*
*
* -
*
* Terminated
: Environment is not running.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link EnvironmentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current operational status of the environment:
*
* -
*
* Launching
: Environment is in the process of initial deployment.
*
*
* -
*
* Updating
: Environment is in the process of updating its configuration settings or
* application version.
*
*
* -
*
* Ready
: Environment is available to have an action performed on it, such as update or
* terminate.
*
*
* -
*
* Terminating
: Environment is in the shut-down process.
*
*
* -
*
* Terminated
: Environment is not running.
*
*
* @see EnvironmentStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* Indicates if there is an in-progress environment configuration update or application version deployment that you
* can cancel.
*
*
* true:
There is an update in progress.
*
*
* false:
There are no updates currently in progress.
*
*
* @return Indicates if there is an in-progress environment configuration update or application version deployment
* that you can cancel.
*
* true:
There is an update in progress.
*
*
* false:
There are no updates currently in progress.
*/
public final Boolean abortableOperationInProgress() {
return abortableOperationInProgress;
}
/**
*
* Describes the health status of the environment. AWS Elastic Beanstalk indicates the failure levels for a running
* environment:
*
*
* -
*
* Red
: Indicates the environment is not responsive. Occurs when three or more consecutive failures
* occur for an environment.
*
*
* -
*
* Yellow
: Indicates that something is wrong. Occurs when two consecutive failures occur for an
* environment.
*
*
* -
*
* Green
: Indicates the environment is healthy and fully functional.
*
*
* -
*
* Grey
: Default health for a new environment. The environment is not fully launched and health checks
* have not started or health checks are suspended during an UpdateEnvironment
or
* RestartEnvironment
request.
*
*
*
*
* Default: Grey
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #health} will
* return {@link EnvironmentHealth#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthAsString}.
*
*
* @return Describes the health status of the environment. AWS Elastic Beanstalk indicates the failure levels for a
* running environment:
*
* -
*
* Red
: Indicates the environment is not responsive. Occurs when three or more consecutive
* failures occur for an environment.
*
*
* -
*
* Yellow
: Indicates that something is wrong. Occurs when two consecutive failures occur for an
* environment.
*
*
* -
*
* Green
: Indicates the environment is healthy and fully functional.
*
*
* -
*
* Grey
: Default health for a new environment. The environment is not fully launched and health
* checks have not started or health checks are suspended during an UpdateEnvironment
or
* RestartEnvironment
request.
*
*
*
*
* Default: Grey
* @see EnvironmentHealth
*/
public final EnvironmentHealth health() {
return EnvironmentHealth.fromValue(health);
}
/**
*
* Describes the health status of the environment. AWS Elastic Beanstalk indicates the failure levels for a running
* environment:
*
*
* -
*
* Red
: Indicates the environment is not responsive. Occurs when three or more consecutive failures
* occur for an environment.
*
*
* -
*
* Yellow
: Indicates that something is wrong. Occurs when two consecutive failures occur for an
* environment.
*
*
* -
*
* Green
: Indicates the environment is healthy and fully functional.
*
*
* -
*
* Grey
: Default health for a new environment. The environment is not fully launched and health checks
* have not started or health checks are suspended during an UpdateEnvironment
or
* RestartEnvironment
request.
*
*
*
*
* Default: Grey
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #health} will
* return {@link EnvironmentHealth#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthAsString}.
*
*
* @return Describes the health status of the environment. AWS Elastic Beanstalk indicates the failure levels for a
* running environment:
*
* -
*
* Red
: Indicates the environment is not responsive. Occurs when three or more consecutive
* failures occur for an environment.
*
*
* -
*
* Yellow
: Indicates that something is wrong. Occurs when two consecutive failures occur for an
* environment.
*
*
* -
*
* Green
: Indicates the environment is healthy and fully functional.
*
*
* -
*
* Grey
: Default health for a new environment. The environment is not fully launched and health
* checks have not started or health checks are suspended during an UpdateEnvironment
or
* RestartEnvironment
request.
*
*
*
*
* Default: Grey
* @see EnvironmentHealth
*/
public final String healthAsString() {
return health;
}
/**
*
* Returns the health status of the application running in your environment. For more information, see Health Colors and
* Statuses.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link EnvironmentHealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #healthStatusAsString}.
*
*
* @return Returns the health status of the application running in your environment. For more information, see Health Colors
* and Statuses.
* @see EnvironmentHealthStatus
*/
public final EnvironmentHealthStatus healthStatus() {
return EnvironmentHealthStatus.fromValue(healthStatus);
}
/**
*
* Returns the health status of the application running in your environment. For more information, see Health Colors and
* Statuses.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link EnvironmentHealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #healthStatusAsString}.
*
*
* @return Returns the health status of the application running in your environment. For more information, see Health Colors
* and Statuses.
* @see EnvironmentHealthStatus
*/
public final String healthStatusAsString() {
return healthStatus;
}
/**
*
* The description of the AWS resources used by this environment.
*
*
* @return The description of the AWS resources used by this environment.
*/
public final EnvironmentResourcesDescription resources() {
return resources;
}
/**
*
* Describes the current tier of this environment.
*
*
* @return Describes the current tier of this environment.
*/
public final EnvironmentTier tier() {
return tier;
}
/**
* Returns true if the EnvironmentLinks property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasEnvironmentLinks() {
return environmentLinks != null && !(environmentLinks instanceof SdkAutoConstructList);
}
/**
*
* A list of links to other environments in the same group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasEnvironmentLinks()} to see if a value was sent in this field.
*
*
* @return A list of links to other environments in the same group.
*/
public final List environmentLinks() {
return environmentLinks;
}
/**
*
* The environment's Amazon Resource Name (ARN), which can be used in other API requests that require an ARN.
*
*
* @return The environment's Amazon Resource Name (ARN), which can be used in other API requests that require an
* ARN.
*/
public final String environmentArn() {
return environmentArn;
}
/**
*
* The Amazon Resource Name (ARN) of the environment's operations role. For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the environment's operations role. For more information, see Operations
* roles in the AWS Elastic Beanstalk Developer Guide.
*/
public final String operationsRole() {
return operationsRole;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(solutionStackName());
hashCode = 31 * hashCode + Objects.hashCode(platformArn());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(endpointURL());
hashCode = 31 * hashCode + Objects.hashCode(cname());
hashCode = 31 * hashCode + Objects.hashCode(dateCreated());
hashCode = 31 * hashCode + Objects.hashCode(dateUpdated());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(abortableOperationInProgress());
hashCode = 31 * hashCode + Objects.hashCode(healthAsString());
hashCode = 31 * hashCode + Objects.hashCode(healthStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resources());
hashCode = 31 * hashCode + Objects.hashCode(tier());
hashCode = 31 * hashCode + Objects.hashCode(hasEnvironmentLinks() ? environmentLinks() : null);
hashCode = 31 * hashCode + Objects.hashCode(environmentArn());
hashCode = 31 * hashCode + Objects.hashCode(operationsRole());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateEnvironmentResponse)) {
return false;
}
CreateEnvironmentResponse other = (CreateEnvironmentResponse) obj;
return Objects.equals(environmentName(), other.environmentName())
&& Objects.equals(environmentId(), other.environmentId())
&& Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(versionLabel(), other.versionLabel())
&& Objects.equals(solutionStackName(), other.solutionStackName())
&& Objects.equals(platformArn(), other.platformArn()) && Objects.equals(templateName(), other.templateName())
&& Objects.equals(description(), other.description()) && Objects.equals(endpointURL(), other.endpointURL())
&& Objects.equals(cname(), other.cname()) && Objects.equals(dateCreated(), other.dateCreated())
&& Objects.equals(dateUpdated(), other.dateUpdated()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(abortableOperationInProgress(), other.abortableOperationInProgress())
&& Objects.equals(healthAsString(), other.healthAsString())
&& Objects.equals(healthStatusAsString(), other.healthStatusAsString())
&& Objects.equals(resources(), other.resources()) && Objects.equals(tier(), other.tier())
&& hasEnvironmentLinks() == other.hasEnvironmentLinks()
&& Objects.equals(environmentLinks(), other.environmentLinks())
&& Objects.equals(environmentArn(), other.environmentArn())
&& Objects.equals(operationsRole(), other.operationsRole());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateEnvironmentResponse").add("EnvironmentName", environmentName())
.add("EnvironmentId", environmentId()).add("ApplicationName", applicationName())
.add("VersionLabel", versionLabel()).add("SolutionStackName", solutionStackName())
.add("PlatformArn", platformArn()).add("TemplateName", templateName()).add("Description", description())
.add("EndpointURL", endpointURL()).add("CNAME", cname()).add("DateCreated", dateCreated())
.add("DateUpdated", dateUpdated()).add("Status", statusAsString())
.add("AbortableOperationInProgress", abortableOperationInProgress()).add("Health", healthAsString())
.add("HealthStatus", healthStatusAsString()).add("Resources", resources()).add("Tier", tier())
.add("EnvironmentLinks", hasEnvironmentLinks() ? environmentLinks() : null)
.add("EnvironmentArn", environmentArn()).add("OperationsRole", operationsRole()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "EnvironmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "SolutionStackName":
return Optional.ofNullable(clazz.cast(solutionStackName()));
case "PlatformArn":
return Optional.ofNullable(clazz.cast(platformArn()));
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "EndpointURL":
return Optional.ofNullable(clazz.cast(endpointURL()));
case "CNAME":
return Optional.ofNullable(clazz.cast(cname()));
case "DateCreated":
return Optional.ofNullable(clazz.cast(dateCreated()));
case "DateUpdated":
return Optional.ofNullable(clazz.cast(dateUpdated()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "AbortableOperationInProgress":
return Optional.ofNullable(clazz.cast(abortableOperationInProgress()));
case "Health":
return Optional.ofNullable(clazz.cast(healthAsString()));
case "HealthStatus":
return Optional.ofNullable(clazz.cast(healthStatusAsString()));
case "Resources":
return Optional.ofNullable(clazz.cast(resources()));
case "Tier":
return Optional.ofNullable(clazz.cast(tier()));
case "EnvironmentLinks":
return Optional.ofNullable(clazz.cast(environmentLinks()));
case "EnvironmentArn":
return Optional.ofNullable(clazz.cast(environmentArn()));
case "OperationsRole":
return Optional.ofNullable(clazz.cast(operationsRole()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function