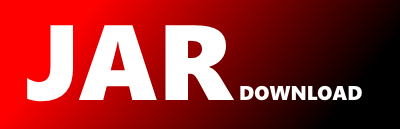
software.amazon.awssdk.services.elasticbeanstalk.model.EnvironmentResourceDescription Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the AWS resources in use by this environment. This data is live.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EnvironmentResourceDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(EnvironmentResourceDescription::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField> AUTO_SCALING_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AutoScalingGroups")
.getter(getter(EnvironmentResourceDescription::autoScalingGroups))
.setter(setter(Builder::autoScalingGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AutoScalingGroup::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> INSTANCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Instances")
.getter(getter(EnvironmentResourceDescription::instances))
.setter(setter(Builder::instances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Instances").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Instance::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LAUNCH_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LaunchConfigurations")
.getter(getter(EnvironmentResourceDescription::launchConfigurations))
.setter(setter(Builder::launchConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LaunchConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LAUNCH_TEMPLATES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LaunchTemplates")
.getter(getter(EnvironmentResourceDescription::launchTemplates))
.setter(setter(Builder::launchTemplates))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplates").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LaunchTemplate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LoadBalancers")
.getter(getter(EnvironmentResourceDescription::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TRIGGERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Triggers")
.getter(getter(EnvironmentResourceDescription::triggers))
.setter(setter(Builder::triggers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Triggers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Trigger::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> QUEUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Queues")
.getter(getter(EnvironmentResourceDescription::queues))
.setter(setter(Builder::queues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Queues").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Queue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENVIRONMENT_NAME_FIELD,
AUTO_SCALING_GROUPS_FIELD, INSTANCES_FIELD, LAUNCH_CONFIGURATIONS_FIELD, LAUNCH_TEMPLATES_FIELD,
LOAD_BALANCERS_FIELD, TRIGGERS_FIELD, QUEUES_FIELD));
private static final long serialVersionUID = 1L;
private final String environmentName;
private final List autoScalingGroups;
private final List instances;
private final List launchConfigurations;
private final List launchTemplates;
private final List loadBalancers;
private final List triggers;
private final List queues;
private EnvironmentResourceDescription(BuilderImpl builder) {
this.environmentName = builder.environmentName;
this.autoScalingGroups = builder.autoScalingGroups;
this.instances = builder.instances;
this.launchConfigurations = builder.launchConfigurations;
this.launchTemplates = builder.launchTemplates;
this.loadBalancers = builder.loadBalancers;
this.triggers = builder.triggers;
this.queues = builder.queues;
}
/**
*
* The name of the environment.
*
*
* @return The name of the environment.
*/
public final String environmentName() {
return environmentName;
}
/**
* Returns true if the AutoScalingGroups property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasAutoScalingGroups() {
return autoScalingGroups != null && !(autoScalingGroups instanceof SdkAutoConstructList);
}
/**
*
* The AutoScalingGroups
used by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAutoScalingGroups()} to see if a value was sent in this field.
*
*
* @return The AutoScalingGroups
used by this environment.
*/
public final List autoScalingGroups() {
return autoScalingGroups;
}
/**
* Returns true if the Instances property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasInstances() {
return instances != null && !(instances instanceof SdkAutoConstructList);
}
/**
*
* The Amazon EC2 instances used by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasInstances()} to see if a value was sent in this field.
*
*
* @return The Amazon EC2 instances used by this environment.
*/
public final List instances() {
return instances;
}
/**
* Returns true if the LaunchConfigurations property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasLaunchConfigurations() {
return launchConfigurations != null && !(launchConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The Auto Scaling launch configurations in use by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLaunchConfigurations()} to see if a value was sent in this field.
*
*
* @return The Auto Scaling launch configurations in use by this environment.
*/
public final List launchConfigurations() {
return launchConfigurations;
}
/**
* Returns true if the LaunchTemplates property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasLaunchTemplates() {
return launchTemplates != null && !(launchTemplates instanceof SdkAutoConstructList);
}
/**
*
* The Amazon EC2 launch templates in use by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLaunchTemplates()} to see if a value was sent in this field.
*
*
* @return The Amazon EC2 launch templates in use by this environment.
*/
public final List launchTemplates() {
return launchTemplates;
}
/**
* Returns true if the LoadBalancers property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* The LoadBalancers in use by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLoadBalancers()} to see if a value was sent in this field.
*
*
* @return The LoadBalancers in use by this environment.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* Returns true if the Triggers property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTriggers() {
return triggers != null && !(triggers instanceof SdkAutoConstructList);
}
/**
*
* The AutoScaling
triggers in use by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTriggers()} to see if a value was sent in this field.
*
*
* @return The AutoScaling
triggers in use by this environment.
*/
public final List triggers() {
return triggers;
}
/**
* Returns true if the Queues property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasQueues() {
return queues != null && !(queues instanceof SdkAutoConstructList);
}
/**
*
* The queues used by this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasQueues()} to see if a value was sent in this field.
*
*
* @return The queues used by this environment.
*/
public final List queues() {
return queues;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(hasAutoScalingGroups() ? autoScalingGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasInstances() ? instances() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLaunchConfigurations() ? launchConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLaunchTemplates() ? launchTemplates() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTriggers() ? triggers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasQueues() ? queues() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EnvironmentResourceDescription)) {
return false;
}
EnvironmentResourceDescription other = (EnvironmentResourceDescription) obj;
return Objects.equals(environmentName(), other.environmentName())
&& hasAutoScalingGroups() == other.hasAutoScalingGroups()
&& Objects.equals(autoScalingGroups(), other.autoScalingGroups()) && hasInstances() == other.hasInstances()
&& Objects.equals(instances(), other.instances()) && hasLaunchConfigurations() == other.hasLaunchConfigurations()
&& Objects.equals(launchConfigurations(), other.launchConfigurations())
&& hasLaunchTemplates() == other.hasLaunchTemplates()
&& Objects.equals(launchTemplates(), other.launchTemplates()) && hasLoadBalancers() == other.hasLoadBalancers()
&& Objects.equals(loadBalancers(), other.loadBalancers()) && hasTriggers() == other.hasTriggers()
&& Objects.equals(triggers(), other.triggers()) && hasQueues() == other.hasQueues()
&& Objects.equals(queues(), other.queues());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EnvironmentResourceDescription").add("EnvironmentName", environmentName())
.add("AutoScalingGroups", hasAutoScalingGroups() ? autoScalingGroups() : null)
.add("Instances", hasInstances() ? instances() : null)
.add("LaunchConfigurations", hasLaunchConfigurations() ? launchConfigurations() : null)
.add("LaunchTemplates", hasLaunchTemplates() ? launchTemplates() : null)
.add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("Triggers", hasTriggers() ? triggers() : null).add("Queues", hasQueues() ? queues() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "AutoScalingGroups":
return Optional.ofNullable(clazz.cast(autoScalingGroups()));
case "Instances":
return Optional.ofNullable(clazz.cast(instances()));
case "LaunchConfigurations":
return Optional.ofNullable(clazz.cast(launchConfigurations()));
case "LaunchTemplates":
return Optional.ofNullable(clazz.cast(launchTemplates()));
case "LoadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "Triggers":
return Optional.ofNullable(clazz.cast(triggers()));
case "Queues":
return Optional.ofNullable(clazz.cast(queues()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function