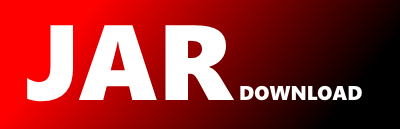
software.amazon.awssdk.services.elasticbeanstalk.model.PlatformBranchSummary Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Summary information about a platform branch.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PlatformBranchSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PLATFORM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformName").getter(getter(PlatformBranchSummary::platformName)).setter(setter(Builder::platformName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformName").build()).build();
private static final SdkField BRANCH_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BranchName").getter(getter(PlatformBranchSummary::branchName)).setter(setter(Builder::branchName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BranchName").build()).build();
private static final SdkField LIFECYCLE_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LifecycleState").getter(getter(PlatformBranchSummary::lifecycleState))
.setter(setter(Builder::lifecycleState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LifecycleState").build()).build();
private static final SdkField BRANCH_ORDER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BranchOrder").getter(getter(PlatformBranchSummary::branchOrder)).setter(setter(Builder::branchOrder))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BranchOrder").build()).build();
private static final SdkField> SUPPORTED_TIER_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedTierList")
.getter(getter(PlatformBranchSummary::supportedTierList))
.setter(setter(Builder::supportedTierList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedTierList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PLATFORM_NAME_FIELD,
BRANCH_NAME_FIELD, LIFECYCLE_STATE_FIELD, BRANCH_ORDER_FIELD, SUPPORTED_TIER_LIST_FIELD));
private static final long serialVersionUID = 1L;
private final String platformName;
private final String branchName;
private final String lifecycleState;
private final Integer branchOrder;
private final List supportedTierList;
private PlatformBranchSummary(BuilderImpl builder) {
this.platformName = builder.platformName;
this.branchName = builder.branchName;
this.lifecycleState = builder.lifecycleState;
this.branchOrder = builder.branchOrder;
this.supportedTierList = builder.supportedTierList;
}
/**
*
* The name of the platform to which this platform branch belongs.
*
*
* @return The name of the platform to which this platform branch belongs.
*/
public final String platformName() {
return platformName;
}
/**
*
* The name of the platform branch.
*
*
* @return The name of the platform branch.
*/
public final String branchName() {
return branchName;
}
/**
*
* The support life cycle state of the platform branch.
*
*
* Possible values: beta
| supported
| deprecated
| retired
*
*
* @return The support life cycle state of the platform branch.
*
* Possible values: beta
| supported
| deprecated
|
* retired
*/
public final String lifecycleState() {
return lifecycleState;
}
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This can be
* helpful, for example, if your code calls the ListPlatformBranches
action and then displays a list of
* platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*
* @return An ordinal number that designates the order in which platform branches have been added to a platform.
* This can be helpful, for example, if your code calls the ListPlatformBranches
action and
* then displays a list of platform branches.
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*/
public final Integer branchOrder() {
return branchOrder;
}
/**
* Returns true if the SupportedTierList property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasSupportedTierList() {
return supportedTierList != null && !(supportedTierList instanceof SdkAutoConstructList);
}
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSupportedTierList()} to see if a value was sent in this field.
*
*
* @return The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*/
public final List supportedTierList() {
return supportedTierList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(platformName());
hashCode = 31 * hashCode + Objects.hashCode(branchName());
hashCode = 31 * hashCode + Objects.hashCode(lifecycleState());
hashCode = 31 * hashCode + Objects.hashCode(branchOrder());
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedTierList() ? supportedTierList() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PlatformBranchSummary)) {
return false;
}
PlatformBranchSummary other = (PlatformBranchSummary) obj;
return Objects.equals(platformName(), other.platformName()) && Objects.equals(branchName(), other.branchName())
&& Objects.equals(lifecycleState(), other.lifecycleState()) && Objects.equals(branchOrder(), other.branchOrder())
&& hasSupportedTierList() == other.hasSupportedTierList()
&& Objects.equals(supportedTierList(), other.supportedTierList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PlatformBranchSummary").add("PlatformName", platformName()).add("BranchName", branchName())
.add("LifecycleState", lifecycleState()).add("BranchOrder", branchOrder())
.add("SupportedTierList", hasSupportedTierList() ? supportedTierList() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PlatformName":
return Optional.ofNullable(clazz.cast(platformName()));
case "BranchName":
return Optional.ofNullable(clazz.cast(branchName()));
case "LifecycleState":
return Optional.ofNullable(clazz.cast(lifecycleState()));
case "BranchOrder":
return Optional.ofNullable(clazz.cast(branchOrder()));
case "SupportedTierList":
return Optional.ofNullable(clazz.cast(supportedTierList()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Possible values: beta
| supported
| deprecated
|
* retired
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lifecycleState(String lifecycleState);
/**
*
* An ordinal number that designates the order in which platform branches have been added to a platform. This
* can be helpful, for example, if your code calls the ListPlatformBranches
action and then
* displays a list of platform branches.
*
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
*
*
* @param branchOrder
* An ordinal number that designates the order in which platform branches have been added to a platform.
* This can be helpful, for example, if your code calls the ListPlatformBranches
action and
* then displays a list of platform branches.
*
* A larger BranchOrder
value designates a newer platform branch within the platform.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder branchOrder(Integer branchOrder);
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* @param supportedTierList
* The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder supportedTierList(Collection supportedTierList);
/**
*
* The environment tiers that platform versions in this branch support.
*
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
*
*
* @param supportedTierList
* The environment tiers that platform versions in this branch support.
*
* Possible values: WebServer/Standard
| Worker/SQS/HTTP
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder supportedTierList(String... supportedTierList);
}
static final class BuilderImpl implements Builder {
private String platformName;
private String branchName;
private String lifecycleState;
private Integer branchOrder;
private List supportedTierList = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(PlatformBranchSummary model) {
platformName(model.platformName);
branchName(model.branchName);
lifecycleState(model.lifecycleState);
branchOrder(model.branchOrder);
supportedTierList(model.supportedTierList);
}
public final String getPlatformName() {
return platformName;
}
@Override
public final Builder platformName(String platformName) {
this.platformName = platformName;
return this;
}
public final void setPlatformName(String platformName) {
this.platformName = platformName;
}
public final String getBranchName() {
return branchName;
}
@Override
public final Builder branchName(String branchName) {
this.branchName = branchName;
return this;
}
public final void setBranchName(String branchName) {
this.branchName = branchName;
}
public final String getLifecycleState() {
return lifecycleState;
}
@Override
public final Builder lifecycleState(String lifecycleState) {
this.lifecycleState = lifecycleState;
return this;
}
public final void setLifecycleState(String lifecycleState) {
this.lifecycleState = lifecycleState;
}
public final Integer getBranchOrder() {
return branchOrder;
}
@Override
public final Builder branchOrder(Integer branchOrder) {
this.branchOrder = branchOrder;
return this;
}
public final void setBranchOrder(Integer branchOrder) {
this.branchOrder = branchOrder;
}
public final Collection getSupportedTierList() {
if (supportedTierList instanceof SdkAutoConstructList) {
return null;
}
return supportedTierList;
}
@Override
public final Builder supportedTierList(Collection supportedTierList) {
this.supportedTierList = SupportedTierListCopier.copy(supportedTierList);
return this;
}
@Override
@SafeVarargs
public final Builder supportedTierList(String... supportedTierList) {
supportedTierList(Arrays.asList(supportedTierList));
return this;
}
public final void setSupportedTierList(Collection supportedTierList) {
this.supportedTierList = SupportedTierListCopier.copy(supportedTierList);
}
@Override
public PlatformBranchSummary build() {
return new PlatformBranchSummary(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}