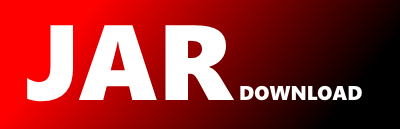
software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEnvironmentHealthRequest Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* See the example below to learn how to create a request body.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeEnvironmentHealthRequest extends ElasticBeanstalkRequest implements
ToCopyableBuilder {
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(DescribeEnvironmentHealthRequest::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentId").getter(getter(DescribeEnvironmentHealthRequest::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentId").build()).build();
private static final SdkField> ATTRIBUTE_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AttributeNames")
.getter(getter(DescribeEnvironmentHealthRequest::attributeNamesAsStrings))
.setter(setter(Builder::attributeNamesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeNames").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENVIRONMENT_NAME_FIELD,
ENVIRONMENT_ID_FIELD, ATTRIBUTE_NAMES_FIELD));
private final String environmentName;
private final String environmentId;
private final List attributeNames;
private DescribeEnvironmentHealthRequest(BuilderImpl builder) {
super(builder);
this.environmentName = builder.environmentName;
this.environmentId = builder.environmentId;
this.attributeNames = builder.attributeNames;
}
/**
*
* Specify the environment by name.
*
*
* You must specify either this or an EnvironmentName, or both.
*
*
* @return Specify the environment by name.
*
* You must specify either this or an EnvironmentName, or both.
*/
public final String environmentName() {
return environmentName;
}
/**
*
* Specify the environment by ID.
*
*
* You must specify either this or an EnvironmentName, or both.
*
*
* @return Specify the environment by ID.
*
* You must specify either this or an EnvironmentName, or both.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* Specify the response elements to return. To retrieve all attributes, set to All
. If no attribute
* names are specified, returns the name of the environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAttributeNames()} to see if a value was sent in this field.
*
*
* @return Specify the response elements to return. To retrieve all attributes, set to All
. If no
* attribute names are specified, returns the name of the environment.
*/
public final List attributeNames() {
return EnvironmentHealthAttributesCopier.copyStringToEnum(attributeNames);
}
/**
* Returns true if the AttributeNames property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasAttributeNames() {
return attributeNames != null && !(attributeNames instanceof SdkAutoConstructList);
}
/**
*
* Specify the response elements to return. To retrieve all attributes, set to All
. If no attribute
* names are specified, returns the name of the environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAttributeNames()} to see if a value was sent in this field.
*
*
* @return Specify the response elements to return. To retrieve all attributes, set to All
. If no
* attribute names are specified, returns the name of the environment.
*/
public final List attributeNamesAsStrings() {
return attributeNames;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(hasAttributeNames() ? attributeNamesAsStrings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeEnvironmentHealthRequest)) {
return false;
}
DescribeEnvironmentHealthRequest other = (DescribeEnvironmentHealthRequest) obj;
return Objects.equals(environmentName(), other.environmentName())
&& Objects.equals(environmentId(), other.environmentId()) && hasAttributeNames() == other.hasAttributeNames()
&& Objects.equals(attributeNamesAsStrings(), other.attributeNamesAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeEnvironmentHealthRequest").add("EnvironmentName", environmentName())
.add("EnvironmentId", environmentId())
.add("AttributeNames", hasAttributeNames() ? attributeNamesAsStrings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "EnvironmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "AttributeNames":
return Optional.ofNullable(clazz.cast(attributeNamesAsStrings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function