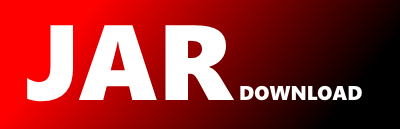
software.amazon.awssdk.services.elasticbeanstalk.model.CreateEnvironmentRequest Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateEnvironmentRequest extends ElasticBeanstalkRequest implements
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(CreateEnvironmentRequest::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(CreateEnvironmentRequest::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GroupName").getter(getter(CreateEnvironmentRequest::groupName)).setter(setter(Builder::groupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateEnvironmentRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField CNAME_PREFIX_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CNAMEPrefix").getter(getter(CreateEnvironmentRequest::cnamePrefix)).setter(setter(Builder::cnamePrefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CNAMEPrefix").build()).build();
private static final SdkField TIER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Tier").getter(getter(CreateEnvironmentRequest::tier)).setter(setter(Builder::tier))
.constructor(EnvironmentTier::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateEnvironmentRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(CreateEnvironmentRequest::versionLabel))
.setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(CreateEnvironmentRequest::templateName))
.setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField SOLUTION_STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SolutionStackName").getter(getter(CreateEnvironmentRequest::solutionStackName))
.setter(setter(Builder::solutionStackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SolutionStackName").build()).build();
private static final SdkField PLATFORM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformArn").getter(getter(CreateEnvironmentRequest::platformArn)).setter(setter(Builder::platformArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformArn").build()).build();
private static final SdkField> OPTION_SETTINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OptionSettings")
.getter(getter(CreateEnvironmentRequest::optionSettings))
.setter(setter(Builder::optionSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionSettings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ConfigurationOptionSetting::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OPTIONS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OptionsToRemove")
.getter(getter(CreateEnvironmentRequest::optionsToRemove))
.setter(setter(Builder::optionsToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionsToRemove").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(OptionSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OPERATIONS_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationsRole").getter(getter(CreateEnvironmentRequest::operationsRole))
.setter(setter(Builder::operationsRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationsRole").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_NAME_FIELD,
ENVIRONMENT_NAME_FIELD, GROUP_NAME_FIELD, DESCRIPTION_FIELD, CNAME_PREFIX_FIELD, TIER_FIELD, TAGS_FIELD,
VERSION_LABEL_FIELD, TEMPLATE_NAME_FIELD, SOLUTION_STACK_NAME_FIELD, PLATFORM_ARN_FIELD, OPTION_SETTINGS_FIELD,
OPTIONS_TO_REMOVE_FIELD, OPERATIONS_ROLE_FIELD));
private final String applicationName;
private final String environmentName;
private final String groupName;
private final String description;
private final String cnamePrefix;
private final EnvironmentTier tier;
private final List tags;
private final String versionLabel;
private final String templateName;
private final String solutionStackName;
private final String platformArn;
private final List optionSettings;
private final List optionsToRemove;
private final String operationsRole;
private CreateEnvironmentRequest(BuilderImpl builder) {
super(builder);
this.applicationName = builder.applicationName;
this.environmentName = builder.environmentName;
this.groupName = builder.groupName;
this.description = builder.description;
this.cnamePrefix = builder.cnamePrefix;
this.tier = builder.tier;
this.tags = builder.tags;
this.versionLabel = builder.versionLabel;
this.templateName = builder.templateName;
this.solutionStackName = builder.solutionStackName;
this.platformArn = builder.platformArn;
this.optionSettings = builder.optionSettings;
this.optionsToRemove = builder.optionsToRemove;
this.operationsRole = builder.operationsRole;
}
/**
*
* The name of the application that is associated with this environment.
*
*
* @return The name of the application that is associated with this environment.
*/
public final String applicationName() {
return applicationName;
}
/**
*
* A unique name for the environment.
*
*
* Constraint: Must be from 4 to 40 characters in length. The name can contain only letters, numbers, and hyphens.
* It can't start or end with a hyphen. This name must be unique within a region in your account. If the specified
* name already exists in the region, Elastic Beanstalk returns an InvalidParameterValue
error.
*
*
* If you don't specify the CNAMEPrefix
parameter, the environment name becomes part of the CNAME, and
* therefore part of the visible URL for your application.
*
*
* @return A unique name for the environment.
*
* Constraint: Must be from 4 to 40 characters in length. The name can contain only letters, numbers, and
* hyphens. It can't start or end with a hyphen. This name must be unique within a region in your account.
* If the specified name already exists in the region, Elastic Beanstalk returns an
* InvalidParameterValue
error.
*
*
* If you don't specify the CNAMEPrefix
parameter, the environment name becomes part of the
* CNAME, and therefore part of the visible URL for your application.
*/
public final String environmentName() {
return environmentName;
}
/**
*
* The name of the group to which the target environment belongs. Specify a group name only if the environment's
* name is specified in an environment manifest and not with the environment name parameter. See Environment Manifest
* (env.yaml) for details.
*
*
* @return The name of the group to which the target environment belongs. Specify a group name only if the
* environment's name is specified in an environment manifest and not with the environment name parameter.
* See Environment
* Manifest (env.yaml) for details.
*/
public final String groupName() {
return groupName;
}
/**
*
* Your description for this environment.
*
*
* @return Your description for this environment.
*/
public final String description() {
return description;
}
/**
*
* If specified, the environment attempts to use this value as the prefix for the CNAME in your Elastic Beanstalk
* environment URL. If not specified, the CNAME is generated automatically by appending a random alphanumeric string
* to the environment name.
*
*
* @return If specified, the environment attempts to use this value as the prefix for the CNAME in your Elastic
* Beanstalk environment URL. If not specified, the CNAME is generated automatically by appending a random
* alphanumeric string to the environment name.
*/
public final String cnamePrefix() {
return cnamePrefix;
}
/**
*
* Specifies the tier to use in creating this environment. The environment tier that you choose determines whether
* Elastic Beanstalk provisions resources to support a web application that handles HTTP(S) requests or a web
* application that handles background-processing tasks.
*
*
* @return Specifies the tier to use in creating this environment. The environment tier that you choose determines
* whether Elastic Beanstalk provisions resources to support a web application that handles HTTP(S) requests
* or a web application that handles background-processing tasks.
*/
public final EnvironmentTier tier() {
return tier;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Specifies the tags applied to resources in the environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return Specifies the tags applied to resources in the environment.
*/
public final List tags() {
return tags;
}
/**
*
* The name of the application version to deploy.
*
*
* Default: If not specified, Elastic Beanstalk attempts to deploy the sample application.
*
*
* @return The name of the application version to deploy.
*
* Default: If not specified, Elastic Beanstalk attempts to deploy the sample application.
*/
public final String versionLabel() {
return versionLabel;
}
/**
*
* The name of the Elastic Beanstalk configuration template to use with the environment.
*
*
*
* If you specify TemplateName
, then don't specify SolutionStackName
.
*
*
*
* @return The name of the Elastic Beanstalk configuration template to use with the environment.
*
* If you specify TemplateName
, then don't specify SolutionStackName
.
*
*/
public final String templateName() {
return templateName;
}
/**
*
* The name of an Elastic Beanstalk solution stack (platform version) to use with the environment. If specified,
* Elastic Beanstalk sets the configuration values to the default values associated with the specified solution
* stack. For a list of current solution stacks, see Elastic Beanstalk
* Supported Platforms in the AWS Elastic Beanstalk Platforms guide.
*
*
*
* If you specify SolutionStackName
, don't specify PlatformArn
or
* TemplateName
.
*
*
*
* @return The name of an Elastic Beanstalk solution stack (platform version) to use with the environment. If
* specified, Elastic Beanstalk sets the configuration values to the default values associated with the
* specified solution stack. For a list of current solution stacks, see Elastic
* Beanstalk Supported Platforms in the AWS Elastic Beanstalk Platforms guide.
*
* If you specify SolutionStackName
, don't specify PlatformArn
or
* TemplateName
.
*
*/
public final String solutionStackName() {
return solutionStackName;
}
/**
*
* The Amazon Resource Name (ARN) of the custom platform to use with the environment. For more information, see Custom Platforms in the
* AWS Elastic Beanstalk Developer Guide.
*
*
*
* If you specify PlatformArn
, don't specify SolutionStackName
.
*
*
*
* @return The Amazon Resource Name (ARN) of the custom platform to use with the environment. For more information,
* see Custom
* Platforms in the AWS Elastic Beanstalk Developer Guide.
*
* If you specify PlatformArn
, don't specify SolutionStackName
.
*
*/
public final String platformArn() {
return platformArn;
}
/**
* Returns true if the OptionSettings property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasOptionSettings() {
return optionSettings != null && !(optionSettings instanceof SdkAutoConstructList);
}
/**
*
* If specified, AWS Elastic Beanstalk sets the specified configuration options to the requested value in the
* configuration set for the new environment. These override the values obtained from the solution stack or the
* configuration template.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasOptionSettings()} to see if a value was sent in this field.
*
*
* @return If specified, AWS Elastic Beanstalk sets the specified configuration options to the requested value in
* the configuration set for the new environment. These override the values obtained from the solution stack
* or the configuration template.
*/
public final List optionSettings() {
return optionSettings;
}
/**
* Returns true if the OptionsToRemove property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasOptionsToRemove() {
return optionsToRemove != null && !(optionsToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of custom user-defined configuration options to remove from the configuration set for this new
* environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasOptionsToRemove()} to see if a value was sent in this field.
*
*
* @return A list of custom user-defined configuration options to remove from the configuration set for this new
* environment.
*/
public final List optionsToRemove() {
return optionsToRemove;
}
/**
*
* The Amazon Resource Name (ARN) of an existing IAM role to be used as the environment's operations role. If
* specified, Elastic Beanstalk uses the operations role for permissions to downstream services during this call and
* during subsequent calls acting on this environment. To specify an operations role, you must have the
* iam:PassRole
permission for the role. For more information, see Operations roles in the
* AWS Elastic Beanstalk Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of an existing IAM role to be used as the environment's operations role.
* If specified, Elastic Beanstalk uses the operations role for permissions to downstream services during
* this call and during subsequent calls acting on this environment. To specify an operations role, you must
* have the iam:PassRole
permission for the role. For more information, see Operations
* roles in the AWS Elastic Beanstalk Developer Guide.
*/
public final String operationsRole() {
return operationsRole;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(groupName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(cnamePrefix());
hashCode = 31 * hashCode + Objects.hashCode(tier());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(solutionStackName());
hashCode = 31 * hashCode + Objects.hashCode(platformArn());
hashCode = 31 * hashCode + Objects.hashCode(hasOptionSettings() ? optionSettings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOptionsToRemove() ? optionsToRemove() : null);
hashCode = 31 * hashCode + Objects.hashCode(operationsRole());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateEnvironmentRequest)) {
return false;
}
CreateEnvironmentRequest other = (CreateEnvironmentRequest) obj;
return Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(environmentName(), other.environmentName()) && Objects.equals(groupName(), other.groupName())
&& Objects.equals(description(), other.description()) && Objects.equals(cnamePrefix(), other.cnamePrefix())
&& Objects.equals(tier(), other.tier()) && hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(versionLabel(), other.versionLabel()) && Objects.equals(templateName(), other.templateName())
&& Objects.equals(solutionStackName(), other.solutionStackName())
&& Objects.equals(platformArn(), other.platformArn()) && hasOptionSettings() == other.hasOptionSettings()
&& Objects.equals(optionSettings(), other.optionSettings()) && hasOptionsToRemove() == other.hasOptionsToRemove()
&& Objects.equals(optionsToRemove(), other.optionsToRemove())
&& Objects.equals(operationsRole(), other.operationsRole());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateEnvironmentRequest").add("ApplicationName", applicationName())
.add("EnvironmentName", environmentName()).add("GroupName", groupName()).add("Description", description())
.add("CNAMEPrefix", cnamePrefix()).add("Tier", tier()).add("Tags", hasTags() ? tags() : null)
.add("VersionLabel", versionLabel()).add("TemplateName", templateName())
.add("SolutionStackName", solutionStackName()).add("PlatformArn", platformArn())
.add("OptionSettings", hasOptionSettings() ? optionSettings() : null)
.add("OptionsToRemove", hasOptionsToRemove() ? optionsToRemove() : null).add("OperationsRole", operationsRole())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "GroupName":
return Optional.ofNullable(clazz.cast(groupName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "CNAMEPrefix":
return Optional.ofNullable(clazz.cast(cnamePrefix()));
case "Tier":
return Optional.ofNullable(clazz.cast(tier()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "SolutionStackName":
return Optional.ofNullable(clazz.cast(solutionStackName()));
case "PlatformArn":
return Optional.ofNullable(clazz.cast(platformArn()));
case "OptionSettings":
return Optional.ofNullable(clazz.cast(optionSettings()));
case "OptionsToRemove":
return Optional.ofNullable(clazz.cast(optionsToRemove()));
case "OperationsRole":
return Optional.ofNullable(clazz.cast(operationsRole()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function