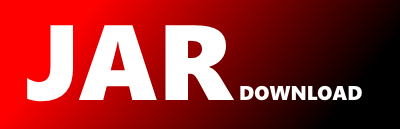
software.amazon.awssdk.services.elasticbeanstalk.model.UpdateEnvironmentRequest Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Request to update an environment.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateEnvironmentRequest extends ElasticBeanstalkRequest implements
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(UpdateEnvironmentRequest::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentId").getter(getter(UpdateEnvironmentRequest::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentId").build()).build();
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(UpdateEnvironmentRequest::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GroupName").getter(getter(UpdateEnvironmentRequest::groupName)).setter(setter(Builder::groupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GroupName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(UpdateEnvironmentRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField TIER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Tier").getter(getter(UpdateEnvironmentRequest::tier)).setter(setter(Builder::tier))
.constructor(EnvironmentTier::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tier").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(UpdateEnvironmentRequest::versionLabel))
.setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(UpdateEnvironmentRequest::templateName))
.setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField SOLUTION_STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SolutionStackName").getter(getter(UpdateEnvironmentRequest::solutionStackName))
.setter(setter(Builder::solutionStackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SolutionStackName").build()).build();
private static final SdkField PLATFORM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformArn").getter(getter(UpdateEnvironmentRequest::platformArn)).setter(setter(Builder::platformArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformArn").build()).build();
private static final SdkField> OPTION_SETTINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OptionSettings")
.getter(getter(UpdateEnvironmentRequest::optionSettings))
.setter(setter(Builder::optionSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionSettings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ConfigurationOptionSetting::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OPTIONS_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OptionsToRemove")
.getter(getter(UpdateEnvironmentRequest::optionsToRemove))
.setter(setter(Builder::optionsToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionsToRemove").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(OptionSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_NAME_FIELD,
ENVIRONMENT_ID_FIELD, ENVIRONMENT_NAME_FIELD, GROUP_NAME_FIELD, DESCRIPTION_FIELD, TIER_FIELD, VERSION_LABEL_FIELD,
TEMPLATE_NAME_FIELD, SOLUTION_STACK_NAME_FIELD, PLATFORM_ARN_FIELD, OPTION_SETTINGS_FIELD, OPTIONS_TO_REMOVE_FIELD));
private final String applicationName;
private final String environmentId;
private final String environmentName;
private final String groupName;
private final String description;
private final EnvironmentTier tier;
private final String versionLabel;
private final String templateName;
private final String solutionStackName;
private final String platformArn;
private final List optionSettings;
private final List optionsToRemove;
private UpdateEnvironmentRequest(BuilderImpl builder) {
super(builder);
this.applicationName = builder.applicationName;
this.environmentId = builder.environmentId;
this.environmentName = builder.environmentName;
this.groupName = builder.groupName;
this.description = builder.description;
this.tier = builder.tier;
this.versionLabel = builder.versionLabel;
this.templateName = builder.templateName;
this.solutionStackName = builder.solutionStackName;
this.platformArn = builder.platformArn;
this.optionSettings = builder.optionSettings;
this.optionsToRemove = builder.optionsToRemove;
}
/**
*
* The name of the application with which the environment is associated.
*
*
* @return The name of the application with which the environment is associated.
*/
public final String applicationName() {
return applicationName;
}
/**
*
* The ID of the environment to update.
*
*
* If no environment with this ID exists, AWS Elastic Beanstalk returns an InvalidParameterValue
error.
*
*
* Condition: You must specify either this or an EnvironmentName, or both. If you do not specify either, AWS Elastic
* Beanstalk returns MissingRequiredParameter
error.
*
*
* @return The ID of the environment to update.
*
* If no environment with this ID exists, AWS Elastic Beanstalk returns an
* InvalidParameterValue
error.
*
*
* Condition: You must specify either this or an EnvironmentName, or both. If you do not specify either, AWS
* Elastic Beanstalk returns MissingRequiredParameter
error.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* The name of the environment to update. If no environment with this name exists, AWS Elastic Beanstalk returns an
* InvalidParameterValue
error.
*
*
* Condition: You must specify either this or an EnvironmentId, or both. If you do not specify either, AWS Elastic
* Beanstalk returns MissingRequiredParameter
error.
*
*
* @return The name of the environment to update. If no environment with this name exists, AWS Elastic Beanstalk
* returns an InvalidParameterValue
error.
*
* Condition: You must specify either this or an EnvironmentId, or both. If you do not specify either, AWS
* Elastic Beanstalk returns MissingRequiredParameter
error.
*/
public final String environmentName() {
return environmentName;
}
/**
*
* The name of the group to which the target environment belongs. Specify a group name only if the environment's
* name is specified in an environment manifest and not with the environment name or environment ID parameters. See
* Environment
* Manifest (env.yaml) for details.
*
*
* @return The name of the group to which the target environment belongs. Specify a group name only if the
* environment's name is specified in an environment manifest and not with the environment name or
* environment ID parameters. See Environment
* Manifest (env.yaml) for details.
*/
public final String groupName() {
return groupName;
}
/**
*
* If this parameter is specified, AWS Elastic Beanstalk updates the description of this environment.
*
*
* @return If this parameter is specified, AWS Elastic Beanstalk updates the description of this environment.
*/
public final String description() {
return description;
}
/**
*
* This specifies the tier to use to update the environment.
*
*
* Condition: At this time, if you change the tier version, name, or type, AWS Elastic Beanstalk returns
* InvalidParameterValue
error.
*
*
* @return This specifies the tier to use to update the environment.
*
* Condition: At this time, if you change the tier version, name, or type, AWS Elastic Beanstalk returns
* InvalidParameterValue
error.
*/
public final EnvironmentTier tier() {
return tier;
}
/**
*
* If this parameter is specified, AWS Elastic Beanstalk deploys the named application version to the environment.
* If no such application version is found, returns an InvalidParameterValue
error.
*
*
* @return If this parameter is specified, AWS Elastic Beanstalk deploys the named application version to the
* environment. If no such application version is found, returns an InvalidParameterValue
* error.
*/
public final String versionLabel() {
return versionLabel;
}
/**
*
* If this parameter is specified, AWS Elastic Beanstalk deploys this configuration template to the environment. If
* no such configuration template is found, AWS Elastic Beanstalk returns an InvalidParameterValue
* error.
*
*
* @return If this parameter is specified, AWS Elastic Beanstalk deploys this configuration template to the
* environment. If no such configuration template is found, AWS Elastic Beanstalk returns an
* InvalidParameterValue
error.
*/
public final String templateName() {
return templateName;
}
/**
*
* This specifies the platform version that the environment will run after the environment is updated.
*
*
* @return This specifies the platform version that the environment will run after the environment is updated.
*/
public final String solutionStackName() {
return solutionStackName;
}
/**
*
* The ARN of the platform, if used.
*
*
* @return The ARN of the platform, if used.
*/
public final String platformArn() {
return platformArn;
}
/**
* For responses, this returns true if the service returned a value for the OptionSettings property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOptionSettings() {
return optionSettings != null && !(optionSettings instanceof SdkAutoConstructList);
}
/**
*
* If specified, AWS Elastic Beanstalk updates the configuration set associated with the running environment and
* sets the specified configuration options to the requested value.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOptionSettings} method.
*
*
* @return If specified, AWS Elastic Beanstalk updates the configuration set associated with the running environment
* and sets the specified configuration options to the requested value.
*/
public final List optionSettings() {
return optionSettings;
}
/**
* For responses, this returns true if the service returned a value for the OptionsToRemove property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOptionsToRemove() {
return optionsToRemove != null && !(optionsToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of custom user-defined configuration options to remove from the configuration set for this environment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOptionsToRemove} method.
*
*
* @return A list of custom user-defined configuration options to remove from the configuration set for this
* environment.
*/
public final List optionsToRemove() {
return optionsToRemove;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(groupName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(tier());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(solutionStackName());
hashCode = 31 * hashCode + Objects.hashCode(platformArn());
hashCode = 31 * hashCode + Objects.hashCode(hasOptionSettings() ? optionSettings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOptionsToRemove() ? optionsToRemove() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateEnvironmentRequest)) {
return false;
}
UpdateEnvironmentRequest other = (UpdateEnvironmentRequest) obj;
return Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(environmentId(), other.environmentId())
&& Objects.equals(environmentName(), other.environmentName()) && Objects.equals(groupName(), other.groupName())
&& Objects.equals(description(), other.description()) && Objects.equals(tier(), other.tier())
&& Objects.equals(versionLabel(), other.versionLabel()) && Objects.equals(templateName(), other.templateName())
&& Objects.equals(solutionStackName(), other.solutionStackName())
&& Objects.equals(platformArn(), other.platformArn()) && hasOptionSettings() == other.hasOptionSettings()
&& Objects.equals(optionSettings(), other.optionSettings()) && hasOptionsToRemove() == other.hasOptionsToRemove()
&& Objects.equals(optionsToRemove(), other.optionsToRemove());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateEnvironmentRequest").add("ApplicationName", applicationName())
.add("EnvironmentId", environmentId()).add("EnvironmentName", environmentName()).add("GroupName", groupName())
.add("Description", description()).add("Tier", tier()).add("VersionLabel", versionLabel())
.add("TemplateName", templateName()).add("SolutionStackName", solutionStackName())
.add("PlatformArn", platformArn()).add("OptionSettings", hasOptionSettings() ? optionSettings() : null)
.add("OptionsToRemove", hasOptionsToRemove() ? optionsToRemove() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "EnvironmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "GroupName":
return Optional.ofNullable(clazz.cast(groupName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Tier":
return Optional.ofNullable(clazz.cast(tier()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "SolutionStackName":
return Optional.ofNullable(clazz.cast(solutionStackName()));
case "PlatformArn":
return Optional.ofNullable(clazz.cast(platformArn()));
case "OptionSettings":
return Optional.ofNullable(clazz.cast(optionSettings()));
case "OptionsToRemove":
return Optional.ofNullable(clazz.cast(optionsToRemove()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function