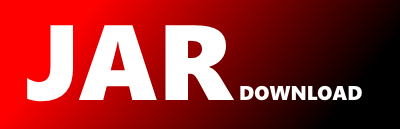
software.amazon.awssdk.services.elasticbeanstalk.model.Latency Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the average latency for the slowest X percent of requests over the last 10 seconds.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Latency implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField P999_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P999")
.getter(getter(Latency::p999)).setter(setter(Builder::p999))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P999").build()).build();
private static final SdkField P99_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P99")
.getter(getter(Latency::p99)).setter(setter(Builder::p99))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P99").build()).build();
private static final SdkField P95_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P95")
.getter(getter(Latency::p95)).setter(setter(Builder::p95))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P95").build()).build();
private static final SdkField P90_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P90")
.getter(getter(Latency::p90)).setter(setter(Builder::p90))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P90").build()).build();
private static final SdkField P85_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P85")
.getter(getter(Latency::p85)).setter(setter(Builder::p85))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P85").build()).build();
private static final SdkField P75_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P75")
.getter(getter(Latency::p75)).setter(setter(Builder::p75))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P75").build()).build();
private static final SdkField P50_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P50")
.getter(getter(Latency::p50)).setter(setter(Builder::p50))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P50").build()).build();
private static final SdkField P10_FIELD = SdkField. builder(MarshallingType.DOUBLE).memberName("P10")
.getter(getter(Latency::p10)).setter(setter(Builder::p10))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("P10").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(P999_FIELD, P99_FIELD,
P95_FIELD, P90_FIELD, P85_FIELD, P75_FIELD, P50_FIELD, P10_FIELD));
private static final long serialVersionUID = 1L;
private final Double p999;
private final Double p99;
private final Double p95;
private final Double p90;
private final Double p85;
private final Double p75;
private final Double p50;
private final Double p10;
private Latency(BuilderImpl builder) {
this.p999 = builder.p999;
this.p99 = builder.p99;
this.p95 = builder.p95;
this.p90 = builder.p90;
this.p85 = builder.p85;
this.p75 = builder.p75;
this.p50 = builder.p50;
this.p10 = builder.p10;
}
/**
*
* The average latency for the slowest 0.1 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 0.1 percent of requests over the last 10 seconds.
*/
public final Double p999() {
return p999;
}
/**
*
* The average latency for the slowest 1 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 1 percent of requests over the last 10 seconds.
*/
public final Double p99() {
return p99;
}
/**
*
* The average latency for the slowest 5 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 5 percent of requests over the last 10 seconds.
*/
public final Double p95() {
return p95;
}
/**
*
* The average latency for the slowest 10 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 10 percent of requests over the last 10 seconds.
*/
public final Double p90() {
return p90;
}
/**
*
* The average latency for the slowest 15 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 15 percent of requests over the last 10 seconds.
*/
public final Double p85() {
return p85;
}
/**
*
* The average latency for the slowest 25 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 25 percent of requests over the last 10 seconds.
*/
public final Double p75() {
return p75;
}
/**
*
* The average latency for the slowest 50 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 50 percent of requests over the last 10 seconds.
*/
public final Double p50() {
return p50;
}
/**
*
* The average latency for the slowest 90 percent of requests over the last 10 seconds.
*
*
* @return The average latency for the slowest 90 percent of requests over the last 10 seconds.
*/
public final Double p10() {
return p10;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(p999());
hashCode = 31 * hashCode + Objects.hashCode(p99());
hashCode = 31 * hashCode + Objects.hashCode(p95());
hashCode = 31 * hashCode + Objects.hashCode(p90());
hashCode = 31 * hashCode + Objects.hashCode(p85());
hashCode = 31 * hashCode + Objects.hashCode(p75());
hashCode = 31 * hashCode + Objects.hashCode(p50());
hashCode = 31 * hashCode + Objects.hashCode(p10());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Latency)) {
return false;
}
Latency other = (Latency) obj;
return Objects.equals(p999(), other.p999()) && Objects.equals(p99(), other.p99()) && Objects.equals(p95(), other.p95())
&& Objects.equals(p90(), other.p90()) && Objects.equals(p85(), other.p85()) && Objects.equals(p75(), other.p75())
&& Objects.equals(p50(), other.p50()) && Objects.equals(p10(), other.p10());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Latency").add("P999", p999()).add("P99", p99()).add("P95", p95()).add("P90", p90())
.add("P85", p85()).add("P75", p75()).add("P50", p50()).add("P10", p10()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "P999":
return Optional.ofNullable(clazz.cast(p999()));
case "P99":
return Optional.ofNullable(clazz.cast(p99()));
case "P95":
return Optional.ofNullable(clazz.cast(p95()));
case "P90":
return Optional.ofNullable(clazz.cast(p90()));
case "P85":
return Optional.ofNullable(clazz.cast(p85()));
case "P75":
return Optional.ofNullable(clazz.cast(p75()));
case "P50":
return Optional.ofNullable(clazz.cast(p50()));
case "P10":
return Optional.ofNullable(clazz.cast(p10()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function