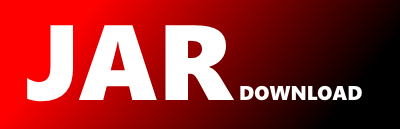
software.amazon.awssdk.services.elasticbeanstalk.model.SourceBuildInformation Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Location of the source code for an application version.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SourceBuildInformation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceType").getter(getter(SourceBuildInformation::sourceTypeAsString))
.setter(setter(Builder::sourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceType").build()).build();
private static final SdkField SOURCE_REPOSITORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceRepository").getter(getter(SourceBuildInformation::sourceRepositoryAsString))
.setter(setter(Builder::sourceRepository))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceRepository").build()).build();
private static final SdkField SOURCE_LOCATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceLocation").getter(getter(SourceBuildInformation::sourceLocation))
.setter(setter(Builder::sourceLocation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceLocation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_TYPE_FIELD,
SOURCE_REPOSITORY_FIELD, SOURCE_LOCATION_FIELD));
private static final long serialVersionUID = 1L;
private final String sourceType;
private final String sourceRepository;
private final String sourceLocation;
private SourceBuildInformation(BuilderImpl builder) {
this.sourceType = builder.sourceType;
this.sourceRepository = builder.sourceRepository;
this.sourceLocation = builder.sourceLocation;
}
/**
*
* The type of repository.
*
*
* -
*
* Git
*
*
* -
*
* Zip
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The type of repository.
*
* -
*
* Git
*
*
* -
*
* Zip
*
*
* @see SourceType
*/
public final SourceType sourceType() {
return SourceType.fromValue(sourceType);
}
/**
*
* The type of repository.
*
*
* -
*
* Git
*
*
* -
*
* Zip
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The type of repository.
*
* -
*
* Git
*
*
* -
*
* Zip
*
*
* @see SourceType
*/
public final String sourceTypeAsString() {
return sourceType;
}
/**
*
* Location where the repository is stored.
*
*
* -
*
* CodeCommit
*
*
* -
*
* S3
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceRepository}
* will return {@link SourceRepository#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #sourceRepositoryAsString}.
*
*
* @return Location where the repository is stored.
*
* -
*
* CodeCommit
*
*
* -
*
* S3
*
*
* @see SourceRepository
*/
public final SourceRepository sourceRepository() {
return SourceRepository.fromValue(sourceRepository);
}
/**
*
* Location where the repository is stored.
*
*
* -
*
* CodeCommit
*
*
* -
*
* S3
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceRepository}
* will return {@link SourceRepository#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #sourceRepositoryAsString}.
*
*
* @return Location where the repository is stored.
*
* -
*
* CodeCommit
*
*
* -
*
* S3
*
*
* @see SourceRepository
*/
public final String sourceRepositoryAsString() {
return sourceRepository;
}
/**
*
* The location of the source code, as a formatted string, depending on the value of SourceRepository
*
*
* -
*
* For CodeCommit
, the format is the repository name and commit ID, separated by a forward slash. For
* example, my-git-repo/265cfa0cf6af46153527f55d6503ec030551f57a
.
*
*
* -
*
* For S3
, the format is the S3 bucket name and object key, separated by a forward slash. For example,
* my-s3-bucket/Folders/my-source-file
.
*
*
*
*
* @return The location of the source code, as a formatted string, depending on the value of
* SourceRepository
*
* -
*
* For CodeCommit
, the format is the repository name and commit ID, separated by a forward
* slash. For example, my-git-repo/265cfa0cf6af46153527f55d6503ec030551f57a
.
*
*
* -
*
* For S3
, the format is the S3 bucket name and object key, separated by a forward slash. For
* example, my-s3-bucket/Folders/my-source-file
.
*
*
*/
public final String sourceLocation() {
return sourceLocation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(sourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceRepositoryAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceLocation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SourceBuildInformation)) {
return false;
}
SourceBuildInformation other = (SourceBuildInformation) obj;
return Objects.equals(sourceTypeAsString(), other.sourceTypeAsString())
&& Objects.equals(sourceRepositoryAsString(), other.sourceRepositoryAsString())
&& Objects.equals(sourceLocation(), other.sourceLocation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SourceBuildInformation").add("SourceType", sourceTypeAsString())
.add("SourceRepository", sourceRepositoryAsString()).add("SourceLocation", sourceLocation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SourceType":
return Optional.ofNullable(clazz.cast(sourceTypeAsString()));
case "SourceRepository":
return Optional.ofNullable(clazz.cast(sourceRepositoryAsString()));
case "SourceLocation":
return Optional.ofNullable(clazz.cast(sourceLocation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function