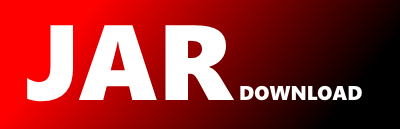
software.amazon.awssdk.services.elasticbeanstalk.model.DescribeEventsRequest Maven / Gradle / Ivy
Show all versions of elasticbeanstalk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Request to retrieve a list of events for an environment.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeEventsRequest extends ElasticBeanstalkRequest implements
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(DescribeEventsRequest::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField VERSION_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionLabel").getter(getter(DescribeEventsRequest::versionLabel)).setter(setter(Builder::versionLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionLabel").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(DescribeEventsRequest::templateName)).setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField ENVIRONMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentId").getter(getter(DescribeEventsRequest::environmentId))
.setter(setter(Builder::environmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentId").build()).build();
private static final SdkField ENVIRONMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EnvironmentName").getter(getter(DescribeEventsRequest::environmentName))
.setter(setter(Builder::environmentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnvironmentName").build()).build();
private static final SdkField PLATFORM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformArn").getter(getter(DescribeEventsRequest::platformArn)).setter(setter(Builder::platformArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformArn").build()).build();
private static final SdkField REQUEST_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RequestId").getter(getter(DescribeEventsRequest::requestId)).setter(setter(Builder::requestId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestId").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Severity").getter(getter(DescribeEventsRequest::severityAsString)).setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Severity").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(DescribeEventsRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(DescribeEventsRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField MAX_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxRecords").getter(getter(DescribeEventsRequest::maxRecords)).setter(setter(Builder::maxRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxRecords").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(DescribeEventsRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_NAME_FIELD,
VERSION_LABEL_FIELD, TEMPLATE_NAME_FIELD, ENVIRONMENT_ID_FIELD, ENVIRONMENT_NAME_FIELD, PLATFORM_ARN_FIELD,
REQUEST_ID_FIELD, SEVERITY_FIELD, START_TIME_FIELD, END_TIME_FIELD, MAX_RECORDS_FIELD, NEXT_TOKEN_FIELD));
private final String applicationName;
private final String versionLabel;
private final String templateName;
private final String environmentId;
private final String environmentName;
private final String platformArn;
private final String requestId;
private final String severity;
private final Instant startTime;
private final Instant endTime;
private final Integer maxRecords;
private final String nextToken;
private DescribeEventsRequest(BuilderImpl builder) {
super(builder);
this.applicationName = builder.applicationName;
this.versionLabel = builder.versionLabel;
this.templateName = builder.templateName;
this.environmentId = builder.environmentId;
this.environmentName = builder.environmentName;
this.platformArn = builder.platformArn;
this.requestId = builder.requestId;
this.severity = builder.severity;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.maxRecords = builder.maxRecords;
this.nextToken = builder.nextToken;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to include only those associated with
* this application.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to include only those associated
* with this application.
*/
public final String applicationName() {
return applicationName;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this application
* version.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this
* application version.
*/
public final String versionLabel() {
return versionLabel;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that are associated with this
* environment configuration.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that are associated with
* this environment configuration.
*/
public final String templateName() {
return templateName;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this
* environment.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this
* environment.
*/
public final String environmentId() {
return environmentId;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this
* environment.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those associated with this
* environment.
*/
public final String environmentName() {
return environmentName;
}
/**
*
* The ARN of a custom platform version. If specified, AWS Elastic Beanstalk restricts the returned descriptions to
* those associated with this custom platform version.
*
*
* @return The ARN of a custom platform version. If specified, AWS Elastic Beanstalk restricts the returned
* descriptions to those associated with this custom platform version.
*/
public final String platformArn() {
return platformArn;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the described events to include only those associated with this
* request ID.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the described events to include only those associated with
* this request ID.
*/
public final String requestId() {
return requestId;
}
/**
*
* If specified, limits the events returned from this call to include only those with the specified severity or
* higher.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link EventSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return If specified, limits the events returned from this call to include only those with the specified severity
* or higher.
* @see EventSeverity
*/
public final EventSeverity severity() {
return EventSeverity.fromValue(severity);
}
/**
*
* If specified, limits the events returned from this call to include only those with the specified severity or
* higher.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link EventSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return If specified, limits the events returned from this call to include only those with the specified severity
* or higher.
* @see EventSeverity
*/
public final String severityAsString() {
return severity;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that occur on or after this
* time.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that occur on or after
* this time.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that occur up to, but not
* including, the EndTime
.
*
*
* @return If specified, AWS Elastic Beanstalk restricts the returned descriptions to those that occur up to, but
* not including, the EndTime
.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* Specifies the maximum number of events that can be returned, beginning with the most recent event.
*
*
* @return Specifies the maximum number of events that can be returned, beginning with the most recent event.
*/
public final Integer maxRecords() {
return maxRecords;
}
/**
*
* Pagination token. If specified, the events return the next batch of results.
*
*
* @return Pagination token. If specified, the events return the next batch of results.
*/
public final String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(versionLabel());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(environmentId());
hashCode = 31 * hashCode + Objects.hashCode(environmentName());
hashCode = 31 * hashCode + Objects.hashCode(platformArn());
hashCode = 31 * hashCode + Objects.hashCode(requestId());
hashCode = 31 * hashCode + Objects.hashCode(severityAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(maxRecords());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeEventsRequest)) {
return false;
}
DescribeEventsRequest other = (DescribeEventsRequest) obj;
return Objects.equals(applicationName(), other.applicationName()) && Objects.equals(versionLabel(), other.versionLabel())
&& Objects.equals(templateName(), other.templateName()) && Objects.equals(environmentId(), other.environmentId())
&& Objects.equals(environmentName(), other.environmentName())
&& Objects.equals(platformArn(), other.platformArn()) && Objects.equals(requestId(), other.requestId())
&& Objects.equals(severityAsString(), other.severityAsString()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(maxRecords(), other.maxRecords())
&& Objects.equals(nextToken(), other.nextToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeEventsRequest").add("ApplicationName", applicationName())
.add("VersionLabel", versionLabel()).add("TemplateName", templateName()).add("EnvironmentId", environmentId())
.add("EnvironmentName", environmentName()).add("PlatformArn", platformArn()).add("RequestId", requestId())
.add("Severity", severityAsString()).add("StartTime", startTime()).add("EndTime", endTime())
.add("MaxRecords", maxRecords()).add("NextToken", nextToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "VersionLabel":
return Optional.ofNullable(clazz.cast(versionLabel()));
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "EnvironmentId":
return Optional.ofNullable(clazz.cast(environmentId()));
case "EnvironmentName":
return Optional.ofNullable(clazz.cast(environmentName()));
case "PlatformArn":
return Optional.ofNullable(clazz.cast(platformArn()));
case "RequestId":
return Optional.ofNullable(clazz.cast(requestId()));
case "Severity":
return Optional.ofNullable(clazz.cast(severityAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "MaxRecords":
return Optional.ofNullable(clazz.cast(maxRecords()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function