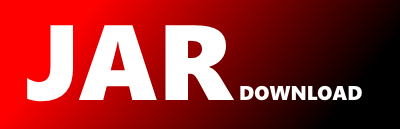
software.amazon.awssdk.services.elasticbeanstalk.model.SingleInstanceHealth Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticbeanstalk.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Detailed health information about an Amazon EC2 instance in your Elastic Beanstalk environment.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SingleInstanceHealth implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SingleInstanceHealth::instanceId)).setter(setter(Builder::instanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceId").build()).build();
private static final SdkField HEALTH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SingleInstanceHealth::healthStatus)).setter(setter(Builder::healthStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthStatus").build()).build();
private static final SdkField COLOR_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SingleInstanceHealth::color)).setter(setter(Builder::color))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Color").build()).build();
private static final SdkField> CAUSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(SingleInstanceHealth::causes))
.setter(setter(Builder::causes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Causes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAUNCHED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(SingleInstanceHealth::launchedAt)).setter(setter(Builder::launchedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchedAt").build()).build();
private static final SdkField APPLICATION_METRICS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(SingleInstanceHealth::applicationMetrics))
.setter(setter(Builder::applicationMetrics)).constructor(ApplicationMetrics::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationMetrics").build())
.build();
private static final SdkField SYSTEM_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(SingleInstanceHealth::system)).setter(setter(Builder::system)).constructor(SystemStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("System").build()).build();
private static final SdkField DEPLOYMENT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(SingleInstanceHealth::deployment)).setter(setter(Builder::deployment))
.constructor(Deployment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Deployment").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SingleInstanceHealth::availabilityZone)).setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SingleInstanceHealth::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INSTANCE_ID_FIELD,
HEALTH_STATUS_FIELD, COLOR_FIELD, CAUSES_FIELD, LAUNCHED_AT_FIELD, APPLICATION_METRICS_FIELD, SYSTEM_FIELD,
DEPLOYMENT_FIELD, AVAILABILITY_ZONE_FIELD, INSTANCE_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String instanceId;
private final String healthStatus;
private final String color;
private final List causes;
private final Instant launchedAt;
private final ApplicationMetrics applicationMetrics;
private final SystemStatus system;
private final Deployment deployment;
private final String availabilityZone;
private final String instanceType;
private SingleInstanceHealth(BuilderImpl builder) {
this.instanceId = builder.instanceId;
this.healthStatus = builder.healthStatus;
this.color = builder.color;
this.causes = builder.causes;
this.launchedAt = builder.launchedAt;
this.applicationMetrics = builder.applicationMetrics;
this.system = builder.system;
this.deployment = builder.deployment;
this.availabilityZone = builder.availabilityZone;
this.instanceType = builder.instanceType;
}
/**
*
* The ID of the Amazon EC2 instance.
*
*
* @return The ID of the Amazon EC2 instance.
*/
public String instanceId() {
return instanceId;
}
/**
*
* Returns the health status of the specified instance. For more information, see Health Colors and
* Statuses.
*
*
* @return Returns the health status of the specified instance. For more information, see Health Colors
* and Statuses.
*/
public String healthStatus() {
return healthStatus;
}
/**
*
* Represents the color indicator that gives you information about the health of the EC2 instance. For more
* information, see Health Colors and
* Statuses.
*
*
* @return Represents the color indicator that gives you information about the health of the EC2 instance. For more
* information, see Health Colors
* and Statuses.
*/
public String color() {
return color;
}
/**
*
* Represents the causes, which provide more information about the current health status.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Represents the causes, which provide more information about the current health status.
*/
public List causes() {
return causes;
}
/**
*
* The time at which the EC2 instance was launched.
*
*
* @return The time at which the EC2 instance was launched.
*/
public Instant launchedAt() {
return launchedAt;
}
/**
*
* Request metrics from your application.
*
*
* @return Request metrics from your application.
*/
public ApplicationMetrics applicationMetrics() {
return applicationMetrics;
}
/**
*
* Operating system metrics from the instance.
*
*
* @return Operating system metrics from the instance.
*/
public SystemStatus system() {
return system;
}
/**
*
* Information about the most recent deployment to an instance.
*
*
* @return Information about the most recent deployment to an instance.
*/
public Deployment deployment() {
return deployment;
}
/**
*
* The availability zone in which the instance runs.
*
*
* @return The availability zone in which the instance runs.
*/
public String availabilityZone() {
return availabilityZone;
}
/**
*
* The instance's type.
*
*
* @return The instance's type.
*/
public String instanceType() {
return instanceType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(instanceId());
hashCode = 31 * hashCode + Objects.hashCode(healthStatus());
hashCode = 31 * hashCode + Objects.hashCode(color());
hashCode = 31 * hashCode + Objects.hashCode(causes());
hashCode = 31 * hashCode + Objects.hashCode(launchedAt());
hashCode = 31 * hashCode + Objects.hashCode(applicationMetrics());
hashCode = 31 * hashCode + Objects.hashCode(system());
hashCode = 31 * hashCode + Objects.hashCode(deployment());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SingleInstanceHealth)) {
return false;
}
SingleInstanceHealth other = (SingleInstanceHealth) obj;
return Objects.equals(instanceId(), other.instanceId()) && Objects.equals(healthStatus(), other.healthStatus())
&& Objects.equals(color(), other.color()) && Objects.equals(causes(), other.causes())
&& Objects.equals(launchedAt(), other.launchedAt())
&& Objects.equals(applicationMetrics(), other.applicationMetrics()) && Objects.equals(system(), other.system())
&& Objects.equals(deployment(), other.deployment())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(instanceType(), other.instanceType());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SingleInstanceHealth").add("InstanceId", instanceId()).add("HealthStatus", healthStatus())
.add("Color", color()).add("Causes", causes()).add("LaunchedAt", launchedAt())
.add("ApplicationMetrics", applicationMetrics()).add("System", system()).add("Deployment", deployment())
.add("AvailabilityZone", availabilityZone()).add("InstanceType", instanceType()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InstanceId":
return Optional.ofNullable(clazz.cast(instanceId()));
case "HealthStatus":
return Optional.ofNullable(clazz.cast(healthStatus()));
case "Color":
return Optional.ofNullable(clazz.cast(color()));
case "Causes":
return Optional.ofNullable(clazz.cast(causes()));
case "LaunchedAt":
return Optional.ofNullable(clazz.cast(launchedAt()));
case "ApplicationMetrics":
return Optional.ofNullable(clazz.cast(applicationMetrics()));
case "System":
return Optional.ofNullable(clazz.cast(system()));
case "Deployment":
return Optional.ofNullable(clazz.cast(deployment()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function