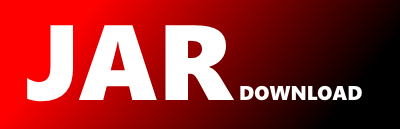
software.amazon.awssdk.services.elasticloadbalancing.DefaultElasticLoadBalancingClient Maven / Gradle / Ivy
Show all versions of elasticloadbalancing Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancing;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.elasticloadbalancing.internal.ElasticLoadBalancingServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.elasticloadbalancing.model.AddTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.AddTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.ApplySecurityGroupsToLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.ApplySecurityGroupsToLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.AttachLoadBalancerToSubnetsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.AttachLoadBalancerToSubnetsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CertificateNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.ConfigureHealthCheckRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.ConfigureHealthCheckResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateAppCookieStickinessPolicyRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateAppCookieStickinessPolicyResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLbCookieStickinessPolicyRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLbCookieStickinessPolicyResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerListenersRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerListenersResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerPolicyRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerPolicyResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.CreateLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerListenersRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerListenersResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerPolicyRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerPolicyResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeleteLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DependencyThrottleException;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeregisterInstancesFromLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DeregisterInstancesFromLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeInstanceHealthRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeInstanceHealthResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerPoliciesRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerPoliciesResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerPolicyTypesRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancerPolicyTypesResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancersRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeLoadBalancersResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DescribeTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DetachLoadBalancerFromSubnetsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DetachLoadBalancerFromSubnetsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DisableAvailabilityZonesForLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.DisableAvailabilityZonesForLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.DuplicateListenerException;
import software.amazon.awssdk.services.elasticloadbalancing.model.DuplicateLoadBalancerNameException;
import software.amazon.awssdk.services.elasticloadbalancing.model.DuplicatePolicyNameException;
import software.amazon.awssdk.services.elasticloadbalancing.model.DuplicateTagKeysException;
import software.amazon.awssdk.services.elasticloadbalancing.model.ElasticLoadBalancingException;
import software.amazon.awssdk.services.elasticloadbalancing.model.EnableAvailabilityZonesForLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.EnableAvailabilityZonesForLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.InvalidConfigurationRequestException;
import software.amazon.awssdk.services.elasticloadbalancing.model.InvalidInstanceException;
import software.amazon.awssdk.services.elasticloadbalancing.model.InvalidSchemeException;
import software.amazon.awssdk.services.elasticloadbalancing.model.InvalidSecurityGroupException;
import software.amazon.awssdk.services.elasticloadbalancing.model.InvalidSubnetException;
import software.amazon.awssdk.services.elasticloadbalancing.model.ListenerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.LoadBalancerAttributeNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.LoadBalancerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.ModifyLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.ModifyLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.OperationNotPermittedException;
import software.amazon.awssdk.services.elasticloadbalancing.model.PolicyNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.PolicyTypeNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.RegisterInstancesWithLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.RegisterInstancesWithLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.RemoveTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.RemoveTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerListenerSslCertificateRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerListenerSslCertificateResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerPoliciesForBackendServerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerPoliciesForBackendServerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerPoliciesOfListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancing.model.SetLoadBalancerPoliciesOfListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancing.model.SubnetNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancing.model.TooManyLoadBalancersException;
import software.amazon.awssdk.services.elasticloadbalancing.model.TooManyPoliciesException;
import software.amazon.awssdk.services.elasticloadbalancing.model.TooManyTagsException;
import software.amazon.awssdk.services.elasticloadbalancing.model.UnsupportedProtocolException;
import software.amazon.awssdk.services.elasticloadbalancing.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.ApplySecurityGroupsToLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.AttachLoadBalancerToSubnetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.ConfigureHealthCheckRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.CreateAppCookieStickinessPolicyRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.CreateLbCookieStickinessPolicyRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.CreateLoadBalancerListenersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.CreateLoadBalancerPolicyRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.CreateLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DeleteLoadBalancerListenersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DeleteLoadBalancerPolicyRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DeleteLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DeregisterInstancesFromLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeAccountLimitsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeInstanceHealthRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeLoadBalancerPoliciesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeLoadBalancerPolicyTypesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeLoadBalancersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DetachLoadBalancerFromSubnetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.DisableAvailabilityZonesForLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.EnableAvailabilityZonesForLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.ModifyLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.RegisterInstancesWithLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.SetLoadBalancerListenerSslCertificateRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.SetLoadBalancerPoliciesForBackendServerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.transform.SetLoadBalancerPoliciesOfListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancing.waiters.ElasticLoadBalancingWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link ElasticLoadBalancingClient}.
*
* @see ElasticLoadBalancingClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultElasticLoadBalancingClient implements ElasticLoadBalancingClient {
private static final Logger log = Logger.loggerFor(DefaultElasticLoadBalancingClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final SyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultElasticLoadBalancingClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
}
/**
*
* Adds the specified tags to the specified load balancer. Each load balancer can have a maximum of 10 tags.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the load
* balancer, AddTags
updates its value.
*
*
* For more information, see Tag Your Classic Load
* Balancer in the Classic Load Balancers Guide.
*
*
* @param addTagsRequest
* Contains the parameters for AddTags.
* @return Result of the AddTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TooManyTagsException
* The quota for the number of tags that can be assigned to a load balancer has been reached.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.AddTags
* @see AWS
* API Documentation
*/
@Override
public AddTagsResponse addTags(AddTagsRequest addTagsRequest) throws LoadBalancerNotFoundException, TooManyTagsException,
DuplicateTagKeysException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddTags").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(addTagsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates one or more security groups with your load balancer in a virtual private cloud (VPC). The specified
* security groups override the previously associated security groups.
*
*
* For more information, see Security Groups for Load Balancers in a VPC in the Classic Load Balancers Guide.
*
*
* @param applySecurityGroupsToLoadBalancerRequest
* Contains the parameters for ApplySecurityGroupsToLoadBalancer.
* @return Result of the ApplySecurityGroupsToLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws InvalidSecurityGroupException
* One or more of the specified security groups do not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.ApplySecurityGroupsToLoadBalancer
* @see AWS API Documentation
*/
@Override
public ApplySecurityGroupsToLoadBalancerResponse applySecurityGroupsToLoadBalancer(
ApplySecurityGroupsToLoadBalancerRequest applySecurityGroupsToLoadBalancerRequest)
throws LoadBalancerNotFoundException, InvalidConfigurationRequestException, InvalidSecurityGroupException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ApplySecurityGroupsToLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(applySecurityGroupsToLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
applySecurityGroupsToLoadBalancerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ApplySecurityGroupsToLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ApplySecurityGroupsToLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(applySecurityGroupsToLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ApplySecurityGroupsToLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds one or more subnets to the set of configured subnets for the specified load balancer.
*
*
* The load balancer evenly distributes requests across all registered subnets. For more information, see Add or Remove
* Subnets for Your Load Balancer in a VPC in the Classic Load Balancers Guide.
*
*
* @param attachLoadBalancerToSubnetsRequest
* Contains the parameters for AttachLoaBalancerToSubnets.
* @return Result of the AttachLoadBalancerToSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SubnetNotFoundException
* One or more of the specified subnets do not exist.
* @throws InvalidSubnetException
* The specified VPC has no associated Internet gateway.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.AttachLoadBalancerToSubnets
* @see AWS API Documentation
*/
@Override
public AttachLoadBalancerToSubnetsResponse attachLoadBalancerToSubnets(
AttachLoadBalancerToSubnetsRequest attachLoadBalancerToSubnetsRequest) throws LoadBalancerNotFoundException,
InvalidConfigurationRequestException, SubnetNotFoundException, InvalidSubnetException, AwsServiceException,
SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AttachLoadBalancerToSubnetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(attachLoadBalancerToSubnetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachLoadBalancerToSubnetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachLoadBalancerToSubnets");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachLoadBalancerToSubnets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(attachLoadBalancerToSubnetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AttachLoadBalancerToSubnetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Specifies the health check settings to use when evaluating the health state of your EC2 instances.
*
*
* For more information, see Configure Health
* Checks for Your Load Balancer in the Classic Load Balancers Guide.
*
*
* @param configureHealthCheckRequest
* Contains the parameters for ConfigureHealthCheck.
* @return Result of the ConfigureHealthCheck operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.ConfigureHealthCheck
* @see AWS API Documentation
*/
@Override
public ConfigureHealthCheckResponse configureHealthCheck(ConfigureHealthCheckRequest configureHealthCheckRequest)
throws LoadBalancerNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ConfigureHealthCheckResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(configureHealthCheckRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, configureHealthCheckRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ConfigureHealthCheck");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ConfigureHealthCheck").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(configureHealthCheckRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ConfigureHealthCheckRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates a stickiness policy with sticky session lifetimes that follow that of an application-generated cookie.
* This policy can be associated only with HTTP/HTTPS listeners.
*
*
* This policy is similar to the policy created by CreateLBCookieStickinessPolicy, except that the lifetime
* of the special Elastic Load Balancing cookie, AWSELB
, follows the lifetime of the
* application-generated cookie specified in the policy configuration. The load balancer only inserts a new
* stickiness cookie when the application response includes a new application cookie.
*
*
* If the application cookie is explicitly removed or expires, the session stops being sticky until a new
* application cookie is issued.
*
*
* For more information, see Application-Controlled Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param createAppCookieStickinessPolicyRequest
* Contains the parameters for CreateAppCookieStickinessPolicy.
* @return Result of the CreateAppCookieStickinessPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.CreateAppCookieStickinessPolicy
* @see AWS API Documentation
*/
@Override
public CreateAppCookieStickinessPolicyResponse createAppCookieStickinessPolicy(
CreateAppCookieStickinessPolicyRequest createAppCookieStickinessPolicyRequest) throws LoadBalancerNotFoundException,
DuplicatePolicyNameException, TooManyPoliciesException, InvalidConfigurationRequestException, AwsServiceException,
SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateAppCookieStickinessPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAppCookieStickinessPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createAppCookieStickinessPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAppCookieStickinessPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAppCookieStickinessPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createAppCookieStickinessPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAppCookieStickinessPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates a stickiness policy with sticky session lifetimes controlled by the lifetime of the browser
* (user-agent) or a specified expiration period. This policy can be associated only with HTTP/HTTPS listeners.
*
*
* When a load balancer implements this policy, the load balancer uses a special cookie to track the instance for
* each request. When the load balancer receives a request, it first checks to see if this cookie is present in the
* request. If so, the load balancer sends the request to the application server specified in the cookie. If not,
* the load balancer sends the request to a server that is chosen based on the existing load-balancing algorithm.
*
*
* A cookie is inserted into the response for binding subsequent requests from the same user to that server. The
* validity of the cookie is based on the cookie expiration time, which is specified in the policy configuration.
*
*
* For more information, see Duration-Based Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param createLbCookieStickinessPolicyRequest
* Contains the parameters for CreateLBCookieStickinessPolicy.
* @return Result of the CreateLBCookieStickinessPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.CreateLBCookieStickinessPolicy
* @see AWS API Documentation
*/
@Override
public CreateLbCookieStickinessPolicyResponse createLBCookieStickinessPolicy(
CreateLbCookieStickinessPolicyRequest createLbCookieStickinessPolicyRequest) throws LoadBalancerNotFoundException,
DuplicatePolicyNameException, TooManyPoliciesException, InvalidConfigurationRequestException, AwsServiceException,
SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLbCookieStickinessPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLbCookieStickinessPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createLbCookieStickinessPolicyRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLBCookieStickinessPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLBCookieStickinessPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createLbCookieStickinessPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateLbCookieStickinessPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a Classic Load Balancer.
*
*
* You can add listeners, security groups, subnets, and tags when you create your load balancer, or you can add them
* later using CreateLoadBalancerListeners, ApplySecurityGroupsToLoadBalancer,
* AttachLoadBalancerToSubnets, and AddTags.
*
*
* To describe your current load balancers, see DescribeLoadBalancers. When you are finished with a load
* balancer, you can delete it using DeleteLoadBalancer.
*
*
* You can create up to 20 load balancers per region per account. You can request an increase for the number of load
* balancers for your account. For more information, see Limits for Your Classic
* Load Balancer in the Classic Load Balancers Guide.
*
*
* @param createLoadBalancerRequest
* Contains the parameters for CreateLoadBalancer.
* @return Result of the CreateLoadBalancer operation returned by the service.
* @throws DuplicateLoadBalancerNameException
* The specified load balancer name already exists for this account.
* @throws TooManyLoadBalancersException
* The quota for the number of load balancers has been reached.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SubnetNotFoundException
* One or more of the specified subnets do not exist.
* @throws InvalidSubnetException
* The specified VPC has no associated Internet gateway.
* @throws InvalidSecurityGroupException
* One or more of the specified security groups do not exist.
* @throws InvalidSchemeException
* The specified value for the schema is not valid. You can only specify a scheme for load balancers in a
* VPC.
* @throws TooManyTagsException
* The quota for the number of tags that can be assigned to a load balancer has been reached.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws UnsupportedProtocolException
* The specified protocol or signature version is not supported.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.CreateLoadBalancer
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerResponse createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest)
throws DuplicateLoadBalancerNameException, TooManyLoadBalancersException, CertificateNotFoundException,
InvalidConfigurationRequestException, SubnetNotFoundException, InvalidSubnetException, InvalidSecurityGroupException,
InvalidSchemeException, TooManyTagsException, DuplicateTagKeysException, UnsupportedProtocolException,
OperationNotPermittedException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoadBalancer");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates one or more listeners for the specified load balancer. If a listener with the specified port does not
* already exist, it is created; otherwise, the properties of the new listener must match the properties of the
* existing listener.
*
*
* For more information, see Listeners for
* Your Classic Load Balancer in the Classic Load Balancers Guide.
*
*
* @param createLoadBalancerListenersRequest
* Contains the parameters for CreateLoadBalancerListeners.
* @return Result of the CreateLoadBalancerListeners operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DuplicateListenerException
* A listener already exists for the specified load balancer name and port, but with a different instance
* port, protocol, or SSL certificate.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws UnsupportedProtocolException
* The specified protocol or signature version is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.CreateLoadBalancerListeners
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerListenersResponse createLoadBalancerListeners(
CreateLoadBalancerListenersRequest createLoadBalancerListenersRequest) throws LoadBalancerNotFoundException,
DuplicateListenerException, CertificateNotFoundException, InvalidConfigurationRequestException,
UnsupportedProtocolException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoadBalancerListenersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLoadBalancerListenersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoadBalancerListenersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoadBalancerListeners");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLoadBalancerListeners").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createLoadBalancerListenersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateLoadBalancerListenersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a policy with the specified attributes for the specified load balancer.
*
*
* Policies are settings that are saved for your load balancer and that can be applied to the listener or the
* application server, depending on the policy type.
*
*
* @param createLoadBalancerPolicyRequest
* Contains the parameters for CreateLoadBalancerPolicy.
* @return Result of the CreateLoadBalancerPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyTypeNotFoundException
* One or more of the specified policy types do not exist.
* @throws DuplicatePolicyNameException
* A policy with the specified name already exists for this load balancer.
* @throws TooManyPoliciesException
* The quota for the number of policies for this load balancer has been reached.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.CreateLoadBalancerPolicy
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerPolicyResponse createLoadBalancerPolicy(
CreateLoadBalancerPolicyRequest createLoadBalancerPolicyRequest) throws LoadBalancerNotFoundException,
PolicyTypeNotFoundException, DuplicatePolicyNameException, TooManyPoliciesException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoadBalancerPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLoadBalancerPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoadBalancerPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoadBalancerPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLoadBalancerPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createLoadBalancerPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateLoadBalancerPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified load balancer.
*
*
* If you are attempting to recreate a load balancer, you must reconfigure all settings. The DNS name associated
* with a deleted load balancer are no longer usable. The name and associated DNS record of the deleted load
* balancer no longer exist and traffic sent to any of its IP addresses is no longer delivered to your instances.
*
*
* If the load balancer does not exist or has already been deleted, the call to DeleteLoadBalancer
* still succeeds.
*
*
* @param deleteLoadBalancerRequest
* Contains the parameters for DeleteLoadBalancer.
* @return Result of the DeleteLoadBalancer operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DeleteLoadBalancer
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerResponse deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest)
throws AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoadBalancer");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified listeners from the specified load balancer.
*
*
* @param deleteLoadBalancerListenersRequest
* Contains the parameters for DeleteLoadBalancerListeners.
* @return Result of the DeleteLoadBalancerListeners operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DeleteLoadBalancerListeners
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerListenersResponse deleteLoadBalancerListeners(
DeleteLoadBalancerListenersRequest deleteLoadBalancerListenersRequest) throws LoadBalancerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoadBalancerListenersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLoadBalancerListenersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoadBalancerListenersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoadBalancerListeners");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLoadBalancerListeners").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteLoadBalancerListenersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteLoadBalancerListenersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified policy from the specified load balancer. This policy must not be enabled for any listeners.
*
*
* @param deleteLoadBalancerPolicyRequest
* Contains the parameters for DeleteLoadBalancerPolicy.
* @return Result of the DeleteLoadBalancerPolicy operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DeleteLoadBalancerPolicy
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerPolicyResponse deleteLoadBalancerPolicy(
DeleteLoadBalancerPolicyRequest deleteLoadBalancerPolicyRequest) throws LoadBalancerNotFoundException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoadBalancerPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLoadBalancerPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoadBalancerPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoadBalancerPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLoadBalancerPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteLoadBalancerPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteLoadBalancerPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters the specified instances from the specified load balancer. After the instance is deregistered, it no
* longer receives traffic from the load balancer.
*
*
* You can use DescribeLoadBalancers to verify that the instance is deregistered from the load balancer.
*
*
* For more information, see Register or De-Register EC2 Instances in the Classic Load Balancers Guide.
*
*
* @param deregisterInstancesFromLoadBalancerRequest
* Contains the parameters for DeregisterInstancesFromLoadBalancer.
* @return Result of the DeregisterInstancesFromLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DeregisterInstancesFromLoadBalancer
* @see AWS API Documentation
*/
@Override
public DeregisterInstancesFromLoadBalancerResponse deregisterInstancesFromLoadBalancer(
DeregisterInstancesFromLoadBalancerRequest deregisterInstancesFromLoadBalancerRequest)
throws LoadBalancerNotFoundException, InvalidInstanceException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeregisterInstancesFromLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterInstancesFromLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deregisterInstancesFromLoadBalancerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterInstancesFromLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterInstancesFromLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deregisterInstancesFromLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterInstancesFromLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your AWS account.
*
*
* For more information, see Limits for Your Classic
* Load Balancer in the Classic Load Balancers Guide.
*
*
* @param describeAccountLimitsRequest
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeAccountLimits
* @see AWS API Documentation
*/
@Override
public DescribeAccountLimitsResponse describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest)
throws AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeAccountLimitsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAccountLimitsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAccountLimitsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAccountLimits");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeAccountLimits").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeAccountLimitsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAccountLimitsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the state of the specified instances with respect to the specified load balancer. If no instances are
* specified, the call describes the state of all instances that are currently registered with the load balancer. If
* instances are specified, their state is returned even if they are no longer registered with the load balancer.
* The state of terminated instances is not returned.
*
*
* @param describeInstanceHealthRequest
* Contains the parameters for DescribeInstanceHealth.
* @return Result of the DescribeInstanceHealth operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeInstanceHealth
* @see AWS API Documentation
*/
@Override
public DescribeInstanceHealthResponse describeInstanceHealth(DescribeInstanceHealthRequest describeInstanceHealthRequest)
throws LoadBalancerNotFoundException, InvalidInstanceException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeInstanceHealthResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeInstanceHealthRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceHealthRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceHealth");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceHealth").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeInstanceHealthRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeInstanceHealthRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the attributes for the specified load balancer.
*
*
* @param describeLoadBalancerAttributesRequest
* Contains the parameters for DescribeLoadBalancerAttributes.
* @return Result of the DescribeLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws LoadBalancerAttributeNotFoundException
* The specified load balancer attribute does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerAttributesResponse describeLoadBalancerAttributes(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest) throws LoadBalancerNotFoundException,
LoadBalancerAttributeNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLoadBalancerAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeLoadBalancerAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancerAttributes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancerAttributes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeLoadBalancerAttributesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLoadBalancerAttributesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified policies.
*
*
* If you specify a load balancer name, the action returns the descriptions of all policies created for the load
* balancer. If you specify a policy name associated with your load balancer, the action returns the description of
* that policy. If you don't specify a load balancer name, the action returns descriptions of the specified sample
* policies, or descriptions of all sample policies. The names of the sample policies have the
* ELBSample-
prefix.
*
*
* @param describeLoadBalancerPoliciesRequest
* Contains the parameters for DescribeLoadBalancerPolicies.
* @return Result of the DescribeLoadBalancerPolicies operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeLoadBalancerPolicies
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerPoliciesResponse describeLoadBalancerPolicies(
DescribeLoadBalancerPoliciesRequest describeLoadBalancerPoliciesRequest) throws LoadBalancerNotFoundException,
PolicyNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancerPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLoadBalancerPoliciesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLoadBalancerPoliciesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancerPolicies");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancerPolicies").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeLoadBalancerPoliciesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLoadBalancerPoliciesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified load balancer policy types or all load balancer policy types.
*
*
* The description of each type indicates how it can be used. For example, some policies can be used only with layer
* 7 listeners, some policies can be used only with layer 4 listeners, and some policies can be used only with your
* EC2 instances.
*
*
* You can use CreateLoadBalancerPolicy to create a policy configuration for any of these policy types. Then,
* depending on the policy type, use either SetLoadBalancerPoliciesOfListener or
* SetLoadBalancerPoliciesForBackendServer to set the policy.
*
*
* @param describeLoadBalancerPolicyTypesRequest
* Contains the parameters for DescribeLoadBalancerPolicyTypes.
* @return Result of the DescribeLoadBalancerPolicyTypes operation returned by the service.
* @throws PolicyTypeNotFoundException
* One or more of the specified policy types do not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeLoadBalancerPolicyTypes
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerPolicyTypesResponse describeLoadBalancerPolicyTypes(
DescribeLoadBalancerPolicyTypesRequest describeLoadBalancerPolicyTypesRequest) throws PolicyTypeNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancerPolicyTypesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLoadBalancerPolicyTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeLoadBalancerPolicyTypesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancerPolicyTypes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancerPolicyTypes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeLoadBalancerPolicyTypesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLoadBalancerPolicyTypesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified the load balancers. If no load balancers are specified, the call describes all of your
* load balancers.
*
*
* @param describeLoadBalancersRequest
* Contains the parameters for DescribeLoadBalancers.
* @return Result of the DescribeLoadBalancers operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws DependencyThrottleException
* A request made by Elastic Load Balancing to another service exceeds the maximum request rate permitted
* for your account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancersResponse describeLoadBalancers(DescribeLoadBalancersRequest describeLoadBalancersRequest)
throws LoadBalancerNotFoundException, DependencyThrottleException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLoadBalancersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLoadBalancersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancers").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeLoadBalancersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLoadBalancersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the tags associated with the specified load balancers.
*
*
* @param describeTagsRequest
* Contains the parameters for DescribeTags.
* @return Result of the DescribeTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DescribeTags
* @see AWS API Documentation
*/
@Override
public DescribeTagsResponse describeTags(DescribeTagsRequest describeTagsRequest) throws LoadBalancerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeTags").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeTagsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the specified subnets from the set of configured subnets for the load balancer.
*
*
* After a subnet is removed, all EC2 instances registered with the load balancer in the removed subnet go into the
* OutOfService
state. Then, the load balancer balances the traffic among the remaining routable
* subnets.
*
*
* @param detachLoadBalancerFromSubnetsRequest
* Contains the parameters for DetachLoadBalancerFromSubnets.
* @return Result of the DetachLoadBalancerFromSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DetachLoadBalancerFromSubnets
* @see AWS API Documentation
*/
@Override
public DetachLoadBalancerFromSubnetsResponse detachLoadBalancerFromSubnets(
DetachLoadBalancerFromSubnetsRequest detachLoadBalancerFromSubnetsRequest) throws LoadBalancerNotFoundException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DetachLoadBalancerFromSubnetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(detachLoadBalancerFromSubnetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
detachLoadBalancerFromSubnetsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DetachLoadBalancerFromSubnets");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DetachLoadBalancerFromSubnets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(detachLoadBalancerFromSubnetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DetachLoadBalancerFromSubnetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the specified Availability Zones from the set of Availability Zones for the specified load balancer in
* EC2-Classic or a default VPC.
*
*
* For load balancers in a non-default VPC, use DetachLoadBalancerFromSubnets.
*
*
* There must be at least one Availability Zone registered with a load balancer at all times. After an Availability
* Zone is removed, all instances registered with the load balancer that are in the removed Availability Zone go
* into the OutOfService
state. Then, the load balancer attempts to equally balance the traffic among
* its remaining Availability Zones.
*
*
* For more information, see Add or Remove
* Availability Zones in the Classic Load Balancers Guide.
*
*
* @param disableAvailabilityZonesForLoadBalancerRequest
* Contains the parameters for DisableAvailabilityZonesForLoadBalancer.
* @return Result of the DisableAvailabilityZonesForLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.DisableAvailabilityZonesForLoadBalancer
* @see AWS API Documentation
*/
@Override
public DisableAvailabilityZonesForLoadBalancerResponse disableAvailabilityZonesForLoadBalancer(
DisableAvailabilityZonesForLoadBalancerRequest disableAvailabilityZonesForLoadBalancerRequest)
throws LoadBalancerNotFoundException, InvalidConfigurationRequestException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DisableAvailabilityZonesForLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disableAvailabilityZonesForLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disableAvailabilityZonesForLoadBalancerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableAvailabilityZonesForLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableAvailabilityZonesForLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(disableAvailabilityZonesForLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisableAvailabilityZonesForLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds the specified Availability Zones to the set of Availability Zones for the specified load balancer in
* EC2-Classic or a default VPC.
*
*
* For load balancers in a non-default VPC, use AttachLoadBalancerToSubnets.
*
*
* The load balancer evenly distributes requests across all its registered Availability Zones that contain
* instances. For more information, see Add or Remove
* Availability Zones in the Classic Load Balancers Guide.
*
*
* @param enableAvailabilityZonesForLoadBalancerRequest
* Contains the parameters for EnableAvailabilityZonesForLoadBalancer.
* @return Result of the EnableAvailabilityZonesForLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.EnableAvailabilityZonesForLoadBalancer
* @see AWS API Documentation
*/
@Override
public EnableAvailabilityZonesForLoadBalancerResponse enableAvailabilityZonesForLoadBalancer(
EnableAvailabilityZonesForLoadBalancerRequest enableAvailabilityZonesForLoadBalancerRequest)
throws LoadBalancerNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(EnableAvailabilityZonesForLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(enableAvailabilityZonesForLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
enableAvailabilityZonesForLoadBalancerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableAvailabilityZonesForLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableAvailabilityZonesForLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(enableAvailabilityZonesForLoadBalancerRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new EnableAvailabilityZonesForLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the attributes of the specified load balancer.
*
*
* You can modify the load balancer attributes, such as AccessLogs
, ConnectionDraining
,
* and CrossZoneLoadBalancing
by either enabling or disabling them. Or, you can modify the load
* balancer attribute ConnectionSettings
by specifying an idle connection timeout value for your load
* balancer.
*
*
* For more information, see the following in the Classic Load Balancers Guide:
*
*
* -
*
*
* -
*
*
* -
*
* Access
* Logs
*
*
* -
*
*
*
*
* @param modifyLoadBalancerAttributesRequest
* Contains the parameters for ModifyLoadBalancerAttributes.
* @return Result of the ModifyLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws LoadBalancerAttributeNotFoundException
* The specified load balancer attribute does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public ModifyLoadBalancerAttributesResponse modifyLoadBalancerAttributes(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest) throws LoadBalancerNotFoundException,
LoadBalancerAttributeNotFoundException, InvalidConfigurationRequestException, AwsServiceException,
SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyLoadBalancerAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyLoadBalancerAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyLoadBalancerAttributes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyLoadBalancerAttributes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyLoadBalancerAttributesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyLoadBalancerAttributesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds the specified instances to the specified load balancer.
*
*
* The instance must be a running instance in the same network as the load balancer (EC2-Classic or the same VPC).
* If you have EC2-Classic instances and a load balancer in a VPC with ClassicLink enabled, you can link the
* EC2-Classic instances to that VPC and then register the linked EC2-Classic instances with the load balancer in
* the VPC.
*
*
* Note that RegisterInstanceWithLoadBalancer
completes when the request has been registered. Instance
* registration takes a little time to complete. To check the state of the registered instances, use
* DescribeLoadBalancers or DescribeInstanceHealth.
*
*
* After the instance is registered, it starts receiving traffic and requests from the load balancer. Any instance
* that is not in one of the Availability Zones registered for the load balancer is moved to the
* OutOfService
state. If an Availability Zone is added to the load balancer later, any instances
* registered with the load balancer move to the InService
state.
*
*
* To deregister instances from a load balancer, use DeregisterInstancesFromLoadBalancer.
*
*
* For more information, see Register or De-Register EC2 Instances in the Classic Load Balancers Guide.
*
*
* @param registerInstancesWithLoadBalancerRequest
* Contains the parameters for RegisterInstancesWithLoadBalancer.
* @return Result of the RegisterInstancesWithLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidInstanceException
* The specified endpoint is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.RegisterInstancesWithLoadBalancer
* @see AWS API Documentation
*/
@Override
public RegisterInstancesWithLoadBalancerResponse registerInstancesWithLoadBalancer(
RegisterInstancesWithLoadBalancerRequest registerInstancesWithLoadBalancerRequest)
throws LoadBalancerNotFoundException, InvalidInstanceException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RegisterInstancesWithLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(registerInstancesWithLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
registerInstancesWithLoadBalancerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterInstancesWithLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RegisterInstancesWithLoadBalancer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(registerInstancesWithLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterInstancesWithLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more tags from the specified load balancer.
*
*
* @param removeTagsRequest
* Contains the parameters for RemoveTags.
* @return Result of the RemoveTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.RemoveTags
* @see AWS API Documentation
*/
@Override
public RemoveTagsResponse removeTags(RemoveTagsRequest removeTagsRequest) throws LoadBalancerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RemoveTags").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(removeTagsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the certificate that terminates the specified listener's SSL connections. The specified certificate replaces
* any prior certificate that was used on the same load balancer and port.
*
*
* For more information about updating your SSL certificate, see Replace the SSL
* Certificate for Your Load Balancer in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerListenerSslCertificateRequest
* Contains the parameters for SetLoadBalancerListenerSSLCertificate.
* @return Result of the SetLoadBalancerListenerSSLCertificate operation returned by the service.
* @throws CertificateNotFoundException
* The specified ARN does not refer to a valid SSL certificate in AWS Identity and Access Management (IAM)
* or AWS Certificate Manager (ACM). Note that if you recently uploaded the certificate to IAM, this error
* might indicate that the certificate is not fully available yet.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws ListenerNotFoundException
* The load balancer does not have a listener configured at the specified port.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws UnsupportedProtocolException
* The specified protocol or signature version is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.SetLoadBalancerListenerSSLCertificate
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerListenerSslCertificateResponse setLoadBalancerListenerSSLCertificate(
SetLoadBalancerListenerSslCertificateRequest setLoadBalancerListenerSslCertificateRequest)
throws CertificateNotFoundException, LoadBalancerNotFoundException, ListenerNotFoundException,
InvalidConfigurationRequestException, UnsupportedProtocolException, AwsServiceException, SdkClientException,
ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetLoadBalancerListenerSslCertificateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setLoadBalancerListenerSslCertificateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
setLoadBalancerListenerSslCertificateRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetLoadBalancerListenerSSLCertificate");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetLoadBalancerListenerSSLCertificate").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(setLoadBalancerListenerSslCertificateRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetLoadBalancerListenerSslCertificateRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Replaces the set of policies associated with the specified port on which the EC2 instance is listening with a new
* set of policies. At this time, only the back-end server authentication policy type can be applied to the instance
* ports; this policy type is composed of multiple public key policies.
*
*
* Each time you use SetLoadBalancerPoliciesForBackendServer
to enable the policies, use the
* PolicyNames
parameter to list the policies that you want to enable.
*
*
* You can use DescribeLoadBalancers or DescribeLoadBalancerPolicies to verify that the policy is
* associated with the EC2 instance.
*
*
* For more information about enabling back-end instance authentication, see Configure Back-end Instance Authentication in the Classic Load Balancers Guide. For more information
* about Proxy Protocol, see Configure Proxy
* Protocol Support in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerPoliciesForBackendServerRequest
* Contains the parameters for SetLoadBalancerPoliciesForBackendServer.
* @return Result of the SetLoadBalancerPoliciesForBackendServer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.SetLoadBalancerPoliciesForBackendServer
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerPoliciesForBackendServerResponse setLoadBalancerPoliciesForBackendServer(
SetLoadBalancerPoliciesForBackendServerRequest setLoadBalancerPoliciesForBackendServerRequest)
throws LoadBalancerNotFoundException, PolicyNotFoundException, InvalidConfigurationRequestException,
AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetLoadBalancerPoliciesForBackendServerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setLoadBalancerPoliciesForBackendServerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
setLoadBalancerPoliciesForBackendServerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetLoadBalancerPoliciesForBackendServer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetLoadBalancerPoliciesForBackendServer").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(setLoadBalancerPoliciesForBackendServerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetLoadBalancerPoliciesForBackendServerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Replaces the current set of policies for the specified load balancer port with the specified set of policies.
*
*
* To enable back-end server authentication, use SetLoadBalancerPoliciesForBackendServer.
*
*
* For more information about setting policies, see Update the SSL
* Negotiation Configuration, Duration-Based Session Stickiness, and Application-Controlled Session Stickiness in the Classic Load Balancers Guide.
*
*
* @param setLoadBalancerPoliciesOfListenerRequest
* Contains the parameters for SetLoadBalancePoliciesOfListener.
* @return Result of the SetLoadBalancerPoliciesOfListener operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws PolicyNotFoundException
* One or more of the specified policies do not exist.
* @throws ListenerNotFoundException
* The load balancer does not have a listener configured at the specified port.
* @throws InvalidConfigurationRequestException
* The requested configuration change is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingClient.SetLoadBalancerPoliciesOfListener
* @see AWS API Documentation
*/
@Override
public SetLoadBalancerPoliciesOfListenerResponse setLoadBalancerPoliciesOfListener(
SetLoadBalancerPoliciesOfListenerRequest setLoadBalancerPoliciesOfListenerRequest)
throws LoadBalancerNotFoundException, PolicyNotFoundException, ListenerNotFoundException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingException {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetLoadBalancerPoliciesOfListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setLoadBalancerPoliciesOfListenerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
setLoadBalancerPoliciesOfListenerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetLoadBalancerPoliciesOfListener");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetLoadBalancerPoliciesOfListener").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(setLoadBalancerPoliciesOfListenerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetLoadBalancerPoliciesOfListenerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
* Create an instance of {@link ElasticLoadBalancingWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link ElasticLoadBalancingWaiter}
*/
@Override
public ElasticLoadBalancingWaiter waiter() {
return ElasticLoadBalancingWaiter.builder().client(this).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
ElasticLoadBalancingServiceClientConfigurationBuilder serviceConfigBuilder = new ElasticLoadBalancingServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private AwsQueryProtocolFactory init() {
return AwsQueryProtocolFactory
.builder()
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedProtocol")
.exceptionBuilderSupplier(UnsupportedProtocolException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LoadBalancerAttributeNotFound")
.exceptionBuilderSupplier(LoadBalancerAttributeNotFoundException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LoadBalancerNotFound")
.exceptionBuilderSupplier(LoadBalancerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyLoadBalancers")
.exceptionBuilderSupplier(TooManyLoadBalancersException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidConfigurationRequest")
.exceptionBuilderSupplier(InvalidConfigurationRequestException::builder).httpStatusCode(409)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSecurityGroup")
.exceptionBuilderSupplier(InvalidSecurityGroupException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateLoadBalancerName")
.exceptionBuilderSupplier(DuplicateLoadBalancerNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DependencyThrottle")
.exceptionBuilderSupplier(DependencyThrottleException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotPermitted")
.exceptionBuilderSupplier(OperationNotPermittedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PolicyNotFound")
.exceptionBuilderSupplier(PolicyNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("CertificateNotFound")
.exceptionBuilderSupplier(CertificateNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateTagKeys")
.exceptionBuilderSupplier(DuplicateTagKeysException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTags")
.exceptionBuilderSupplier(TooManyTagsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ListenerNotFound")
.exceptionBuilderSupplier(ListenerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyPolicies")
.exceptionBuilderSupplier(TooManyPoliciesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicatePolicyName")
.exceptionBuilderSupplier(DuplicatePolicyNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetNotFound")
.exceptionBuilderSupplier(SubnetNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateListener")
.exceptionBuilderSupplier(DuplicateListenerException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidScheme")
.exceptionBuilderSupplier(InvalidSchemeException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PolicyTypeNotFound")
.exceptionBuilderSupplier(PolicyTypeNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSubnet")
.exceptionBuilderSupplier(InvalidSubnetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInstance")
.exceptionBuilderSupplier(InvalidInstanceException::builder).httpStatusCode(400).build())
.clientConfiguration(clientConfiguration).defaultServiceExceptionSupplier(ElasticLoadBalancingException::builder)
.build();
}
@Override
public final ElasticLoadBalancingServiceClientConfiguration serviceClientConfiguration() {
return new ElasticLoadBalancingServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}