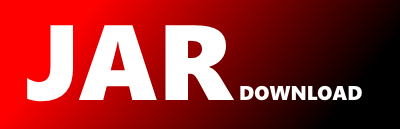
software.amazon.awssdk.services.elasticloadbalancingv2.DefaultElasticLoadBalancingV2Client Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AllocationIdNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AvailabilityZoneNotSupportedException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CertificateNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateListenerException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateLoadBalancerNameException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateTagKeysException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateTargetGroupNameException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ElasticLoadBalancingV2Exception;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ElasticLoadBalancingV2Request;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.HealthUnavailableException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.IncompatibleProtocolsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidConfigurationRequestException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidLoadBalancerActionException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSchemeException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSecurityGroupException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSubnetException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidTargetException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ListenerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.LoadBalancerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.OperationNotPermittedException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.PriorityInUseException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ResourceInUseException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RuleNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SslPolicyNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TargetGroupAssociationLimitException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TargetGroupNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyActionsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyCertificatesException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyListenersException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyLoadBalancersException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyRegistrationsForTargetIdException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyRulesException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTagsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTargetGroupsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTargetsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyUniqueTargetGroupsPerLoadBalancerException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.UnsupportedProtocolException;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersIterable;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersIterable;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsIterable;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.AddListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeregisterTargetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeAccountLimitsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeListenersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeLoadBalancersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeRulesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeSslPoliciesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetGroupAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetHealthRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyTargetGroupAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RegisterTargetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RemoveListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetIpAddressTypeRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetRulePrioritiesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetSecurityGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetSubnetsRequestMarshaller;
/**
* Internal implementation of {@link ElasticLoadBalancingV2Client}.
*
* @see ElasticLoadBalancingV2Client#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultElasticLoadBalancingV2Client implements ElasticLoadBalancingV2Client {
private final SyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultElasticLoadBalancingV2Client(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Adds the specified SSL server certificate to the certificate list for the specified HTTPS or TLS listener.
*
*
* If the certificate in already in the certificate list, the call is successful but the certificate is not added
* again.
*
*
* To get the certificate list for a listener, use DescribeListenerCertificates. To remove certificates from
* the certificate list for a listener, use RemoveListenerCertificates. To replace the default certificate
* for a listener, use ModifyListener.
*
*
* For more information, see SSL Certificates in the Application Load Balancers Guide.
*
*
* @param addListenerCertificatesRequest
* @return Result of the AddListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.AddListenerCertificates
* @see AWS API Documentation
*/
@Override
public AddListenerCertificatesResponse addListenerCertificates(AddListenerCertificatesRequest addListenerCertificatesRequest)
throws ListenerNotFoundException, TooManyCertificatesException, CertificateNotFoundException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddListenerCertificates").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(addListenerCertificatesRequest)
.withMarshaller(new AddListenerCertificatesRequestMarshaller(protocolFactory)));
}
/**
*
* Adds the specified tags to the specified Elastic Load Balancing resource. You can tag your Application Load
* Balancers, Network Load Balancers, and your target groups.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* To list the current tags for your resources, use DescribeTags. To remove tags from your resources, use
* RemoveTags.
*
*
* @param addTagsRequest
* @return Result of the AddTags operation returned by the service.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws TooManyTagsException
* You've reached the limit on the number of tags per load balancer.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.AddTags
* @see AWS
* API Documentation
*/
@Override
public AddTagsResponse addTags(AddTagsRequest addTagsRequest) throws DuplicateTagKeysException, TooManyTagsException,
LoadBalancerNotFoundException, TargetGroupNotFoundException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams().withOperationName("AddTags")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(addTagsRequest)
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)));
}
/**
*
* Creates a listener for the specified Application Load Balancer or Network Load Balancer.
*
*
* To update a listener, use ModifyListener. When you are finished with a listener, you can delete it using
* DeleteListener. If you are finished with both the listener and the load balancer, you can delete them both
* using DeleteLoadBalancer.
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* listeners with the same settings, each call succeeds.
*
*
* For more information, see Listeners
* for Your Application Load Balancers in the Application Load Balancers Guide and Listeners for
* Your Network Load Balancers in the Network Load Balancers Guide.
*
*
* @param createListenerRequest
* @return Result of the CreateListener operation returned by the service.
* @throws DuplicateListenerException
* A listener with the specified port already exists.
* @throws TooManyListenersException
* You've reached the limit on the number of listeners per load balancer.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws SslPolicyNotFoundException
* The specified SSL policy does not exist.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.CreateListener
* @see AWS API Documentation
*/
@Override
public CreateListenerResponse createListener(CreateListenerRequest createListenerRequest) throws DuplicateListenerException,
TooManyListenersException, TooManyCertificatesException, LoadBalancerNotFoundException, TargetGroupNotFoundException,
TargetGroupAssociationLimitException, InvalidConfigurationRequestException, IncompatibleProtocolsException,
SslPolicyNotFoundException, CertificateNotFoundException, UnsupportedProtocolException,
TooManyRegistrationsForTargetIdException, TooManyTargetsException, TooManyActionsException,
InvalidLoadBalancerActionException, TooManyUniqueTargetGroupsPerLoadBalancerException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateListener").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createListenerRequest)
.withMarshaller(new CreateListenerRequestMarshaller(protocolFactory)));
}
/**
*
* Creates an Application Load Balancer or a Network Load Balancer.
*
*
* When you create a load balancer, you can specify security groups, public subnets, IP address type, and tags.
* Otherwise, you could do so later using SetSecurityGroups, SetSubnets, SetIpAddressType, and
* AddTags.
*
*
* To create listeners for your load balancer, use CreateListener. To describe your current load balancers,
* see DescribeLoadBalancers. When you are finished with a load balancer, you can delete it using
* DeleteLoadBalancer.
*
*
* For limit information, see Limits for
* Your Application Load Balancer in the Application Load Balancers Guide and Limits for Your
* Network Load Balancer in the Network Load Balancers Guide.
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* load balancers with the same settings, each call succeeds.
*
*
* For more information, see Application Load Balancers in the Application Load Balancers Guide and Network Load
* Balancers in the Network Load Balancers Guide.
*
*
* @param createLoadBalancerRequest
* @return Result of the CreateLoadBalancer operation returned by the service.
* @throws DuplicateLoadBalancerNameException
* A load balancer with the specified name already exists.
* @throws TooManyLoadBalancersException
* You've reached the limit on the number of load balancers for your AWS account.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SubnetNotFoundException
* The specified subnet does not exist.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @throws InvalidSecurityGroupException
* The specified security group does not exist.
* @throws InvalidSchemeException
* The requested scheme is not valid.
* @throws TooManyTagsException
* You've reached the limit on the number of tags per load balancer.
* @throws DuplicateTagKeysException
* A tag key was specified more than once.
* @throws ResourceInUseException
* A specified resource is in use.
* @throws AllocationIdNotFoundException
* The specified allocation ID does not exist.
* @throws AvailabilityZoneNotSupportedException
* The specified Availability Zone is not supported.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.CreateLoadBalancer
* @see AWS API Documentation
*/
@Override
public CreateLoadBalancerResponse createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest)
throws DuplicateLoadBalancerNameException, TooManyLoadBalancersException, InvalidConfigurationRequestException,
SubnetNotFoundException, InvalidSubnetException, InvalidSecurityGroupException, InvalidSchemeException,
TooManyTagsException, DuplicateTagKeysException, ResourceInUseException, AllocationIdNotFoundException,
AvailabilityZoneNotSupportedException, OperationNotPermittedException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateLoadBalancer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createLoadBalancerRequest)
.withMarshaller(new CreateLoadBalancerRequestMarshaller(protocolFactory)));
}
/**
*
* Creates a rule for the specified listener. The listener must be associated with an Application Load Balancer.
*
*
* Rules are evaluated in priority order, from the lowest value to the highest value. When the conditions for a rule
* are met, its actions are performed. If the conditions for no rules are met, the actions for the default rule are
* performed. For more information, see Listener Rules in the Application Load Balancers Guide.
*
*
* To view your current rules, use DescribeRules. To update a rule, use ModifyRule. To set the
* priorities of your rules, use SetRulePriorities. To delete a rule, use DeleteRule.
*
*
* @param createRuleRequest
* @return Result of the CreateRule operation returned by the service.
* @throws PriorityInUseException
* The specified priority is in use.
* @throws TooManyTargetGroupsException
* You've reached the limit on the number of target groups for your AWS account.
* @throws TooManyRulesException
* You've reached the limit on the number of rules per load balancer.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.CreateRule
* @see AWS API Documentation
*/
@Override
public CreateRuleResponse createRule(CreateRuleRequest createRuleRequest) throws PriorityInUseException,
TooManyTargetGroupsException, TooManyRulesException, TargetGroupAssociationLimitException,
IncompatibleProtocolsException, ListenerNotFoundException, TargetGroupNotFoundException,
InvalidConfigurationRequestException, TooManyRegistrationsForTargetIdException, TooManyTargetsException,
UnsupportedProtocolException, TooManyActionsException, InvalidLoadBalancerActionException,
TooManyUniqueTargetGroupsPerLoadBalancerException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateRule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createRuleRequest)
.withMarshaller(new CreateRuleRequestMarshaller(protocolFactory)));
}
/**
*
* Creates a target group.
*
*
* To register targets with the target group, use RegisterTargets. To update the health check settings for
* the target group, use ModifyTargetGroup. To monitor the health of targets in the target group, use
* DescribeTargetHealth.
*
*
* To route traffic to the targets in a target group, specify the target group in an action using
* CreateListener or CreateRule.
*
*
* To delete a target group, use DeleteTargetGroup.
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* target groups with the same settings, each call succeeds.
*
*
* For more information, see Target Groups for Your Application Load Balancers in the Application Load Balancers Guide or Target
* Groups for Your Network Load Balancers in the Network Load Balancers Guide.
*
*
* @param createTargetGroupRequest
* @return Result of the CreateTargetGroup operation returned by the service.
* @throws DuplicateTargetGroupNameException
* A target group with the specified name already exists.
* @throws TooManyTargetGroupsException
* You've reached the limit on the number of target groups for your AWS account.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.CreateTargetGroup
* @see AWS API Documentation
*/
@Override
public CreateTargetGroupResponse createTargetGroup(CreateTargetGroupRequest createTargetGroupRequest)
throws DuplicateTargetGroupNameException, TooManyTargetGroupsException, InvalidConfigurationRequestException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateTargetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createTargetGroupRequest)
.withMarshaller(new CreateTargetGroupRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer to which it is attached, using
* DeleteLoadBalancer.
*
*
* @param deleteListenerRequest
* @return Result of the DeleteListener operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DeleteListener
* @see AWS API Documentation
*/
@Override
public DeleteListenerResponse deleteListener(DeleteListenerRequest deleteListenerRequest) throws ListenerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteListener").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteListenerRequest)
.withMarshaller(new DeleteListenerRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes the specified Application Load Balancer or Network Load Balancer and its attached listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* @return Result of the DeleteLoadBalancer operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ResourceInUseException
* A specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DeleteLoadBalancer
* @see AWS API Documentation
*/
@Override
public DeleteLoadBalancerResponse deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest)
throws LoadBalancerNotFoundException, OperationNotPermittedException, ResourceInUseException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteLoadBalancer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteLoadBalancerRequest)
.withMarshaller(new DeleteLoadBalancerRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes the specified rule.
*
*
* @param deleteRuleRequest
* @return Result of the DeleteRule operation returned by the service.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DeleteRule
* @see AWS API Documentation
*/
@Override
public DeleteRuleResponse deleteRule(DeleteRuleRequest deleteRuleRequest) throws RuleNotFoundException,
OperationNotPermittedException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteRule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteRuleRequest)
.withMarshaller(new DeleteRuleRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks.
*
*
* @param deleteTargetGroupRequest
* @return Result of the DeleteTargetGroup operation returned by the service.
* @throws ResourceInUseException
* A specified resource is in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DeleteTargetGroup
* @see AWS API Documentation
*/
@Override
public DeleteTargetGroupResponse deleteTargetGroup(DeleteTargetGroupRequest deleteTargetGroupRequest)
throws ResourceInUseException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteTargetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteTargetGroupRequest)
.withMarshaller(new DeleteTargetGroupRequestMarshaller(protocolFactory)));
}
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* @param deregisterTargetsRequest
* @return Result of the DeregisterTargets operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DeregisterTargets
* @see AWS API Documentation
*/
@Override
public DeregisterTargetsResponse deregisterTargets(DeregisterTargetsRequest deregisterTargetsRequest)
throws TargetGroupNotFoundException, InvalidTargetException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeregisterTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeregisterTargets").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterTargetsRequest)
.withMarshaller(new DeregisterTargetsRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your AWS account.
*
*
* For more information, see Limits for
* Your Application Load Balancers in the Application Load Balancer Guide or Limits for Your
* Network Load Balancers in the Network Load Balancers Guide.
*
*
* @param describeAccountLimitsRequest
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeAccountLimits
* @see AWS API Documentation
*/
@Override
public DescribeAccountLimitsResponse describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest)
throws AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeAccountLimitsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeAccountLimits").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeAccountLimitsRequest)
.withMarshaller(new DescribeAccountLimitsRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the default certificate and the certificate list for the specified HTTPS or TLS listener.
*
*
* If the default certificate is also in the certificate list, it appears twice in the results (once with
* IsDefault
set to true and once with IsDefault
set to false).
*
*
* For more information, see SSL Certificates in the Application Load Balancers Guide.
*
*
* @param describeListenerCertificatesRequest
* @return Result of the DescribeListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeListenerCertificates
* @see AWS API Documentation
*/
@Override
public DescribeListenerCertificatesResponse describeListenerCertificates(
DescribeListenerCertificatesRequest describeListenerCertificatesRequest) throws ListenerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeListenerCertificates").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeListenerCertificatesRequest)
.withMarshaller(new DescribeListenerCertificatesRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer or Network Load
* Balancer. You must specify either a load balancer or one or more listeners.
*
*
* For an HTTPS or TLS listener, the output includes the default certificate for the listener. To describe the
* certificate list for the listener, use DescribeListenerCertificates.
*
*
* @param describeListenersRequest
* @return Result of the DescribeListeners operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeListeners
* @see AWS API Documentation
*/
@Override
public DescribeListenersResponse describeListeners(DescribeListenersRequest describeListenersRequest)
throws ListenerNotFoundException, LoadBalancerNotFoundException, UnsupportedProtocolException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeListenersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeListeners").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeListenersRequest)
.withMarshaller(new DescribeListenersRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer or Network Load
* Balancer. You must specify either a load balancer or one or more listeners.
*
*
* For an HTTPS or TLS listener, the output includes the default certificate for the listener. To describe the
* certificate list for the listener, use DescribeListenerCertificates.
*
*
*
* This is a variant of
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersIterable responses = client.describeListenersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersIterable responses = client
* .describeListenersPaginator(request);
* for (software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersIterable responses = client.describeListenersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation.
*
*
* @param describeListenersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeListeners
* @see AWS API Documentation
*/
@Override
public DescribeListenersIterable describeListenersPaginator(DescribeListenersRequest describeListenersRequest)
throws ListenerNotFoundException, LoadBalancerNotFoundException, UnsupportedProtocolException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
return new DescribeListenersIterable(this, applyPaginatorUserAgent(describeListenersRequest));
}
/**
*
* Describes the attributes for the specified Application Load Balancer or Network Load Balancer.
*
*
* For more information, see Load Balancer Attributes in the Application Load Balancers Guide or Load Balancer Attributes in the Network Load Balancers Guide.
*
*
* @param describeLoadBalancerAttributesRequest
* @return Result of the DescribeLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancerAttributesResponse describeLoadBalancerAttributes(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest) throws LoadBalancerNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancerAttributes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeLoadBalancerAttributesRequest)
.withMarshaller(new DescribeLoadBalancerAttributesRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* To describe the listeners for a load balancer, use DescribeListeners. To describe the attributes for a
* load balancer, use DescribeLoadBalancerAttributes.
*
*
* @param describeLoadBalancersRequest
* @return Result of the DescribeLoadBalancers operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeLoadBalancers
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancersResponse describeLoadBalancers(DescribeLoadBalancersRequest describeLoadBalancersRequest)
throws LoadBalancerNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeLoadBalancersRequest)
.withMarshaller(new DescribeLoadBalancersRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* To describe the listeners for a load balancer, use DescribeListeners. To describe the attributes for a
* load balancer, use DescribeLoadBalancerAttributes.
*
*
*
* This is a variant of
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersIterable responses = client.describeLoadBalancersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersIterable responses = client
* .describeLoadBalancersPaginator(request);
* for (software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersIterable responses = client.describeLoadBalancersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation.
*
*
* @param describeLoadBalancersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeLoadBalancers
* @see AWS API Documentation
*/
@Override
public DescribeLoadBalancersIterable describeLoadBalancersPaginator(DescribeLoadBalancersRequest describeLoadBalancersRequest)
throws LoadBalancerNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
return new DescribeLoadBalancersIterable(this, applyPaginatorUserAgent(describeLoadBalancersRequest));
}
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* @return Result of the DescribeRules operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeRules
* @see AWS API Documentation
*/
@Override
public DescribeRulesResponse describeRules(DescribeRulesRequest describeRulesRequest) throws ListenerNotFoundException,
RuleNotFoundException, UnsupportedProtocolException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeRulesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeRules").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRulesRequest)
.withMarshaller(new DescribeRulesRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security Policies in the Application Load Balancers Guide.
*
*
* @param describeSslPoliciesRequest
* @return Result of the DescribeSSLPolicies operation returned by the service.
* @throws SslPolicyNotFoundException
* The specified SSL policy does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeSSLPolicies
* @see AWS API Documentation
*/
@Override
public DescribeSslPoliciesResponse describeSSLPolicies(DescribeSslPoliciesRequest describeSslPoliciesRequest)
throws SslPolicyNotFoundException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeSslPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeSSLPolicies").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeSslPoliciesRequest)
.withMarshaller(new DescribeSslPoliciesRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the tags for the specified resources. You can describe the tags for one or more Application Load
* Balancers, Network Load Balancers, and target groups.
*
*
* @param describeTagsRequest
* @return Result of the DescribeTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeTags
* @see AWS API Documentation
*/
@Override
public DescribeTagsResponse describeTags(DescribeTagsRequest describeTagsRequest) throws LoadBalancerNotFoundException,
TargetGroupNotFoundException, ListenerNotFoundException, RuleNotFoundException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeTags").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTagsRequest)
.withMarshaller(new DescribeTagsRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the attributes for the specified target group.
*
*
* For more information, see Target Group Attributes in the Application Load Balancers Guide or Target Group Attributes in the Network Load Balancers Guide.
*
*
* @param describeTargetGroupAttributesRequest
* @return Result of the DescribeTargetGroupAttributes operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeTargetGroupAttributes
* @see AWS API Documentation
*/
@Override
public DescribeTargetGroupAttributesResponse describeTargetGroupAttributes(
DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest) throws TargetGroupNotFoundException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetGroupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetGroupAttributes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTargetGroupAttributesRequest)
.withMarshaller(new DescribeTargetGroupAttributesRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* To describe the targets for a target group, use DescribeTargetHealth. To describe the attributes of a
* target group, use DescribeTargetGroupAttributes.
*
*
* @param describeTargetGroupsRequest
* @return Result of the DescribeTargetGroups operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeTargetGroups
* @see AWS API Documentation
*/
@Override
public DescribeTargetGroupsResponse describeTargetGroups(DescribeTargetGroupsRequest describeTargetGroupsRequest)
throws LoadBalancerNotFoundException, TargetGroupNotFoundException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetGroups").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTargetGroupsRequest)
.withMarshaller(new DescribeTargetGroupsRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* To describe the targets for a target group, use DescribeTargetHealth. To describe the attributes of a
* target group, use DescribeTargetGroupAttributes.
*
*
*
* This is a variant of
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsIterable responses = client.describeTargetGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsIterable responses = client
* .describeTargetGroupsPaginator(request);
* for (software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsIterable responses = client.describeTargetGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation.
*
*
* @param describeTargetGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeTargetGroups
* @see AWS API Documentation
*/
@Override
public DescribeTargetGroupsIterable describeTargetGroupsPaginator(DescribeTargetGroupsRequest describeTargetGroupsRequest)
throws LoadBalancerNotFoundException, TargetGroupNotFoundException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
return new DescribeTargetGroupsIterable(this, applyPaginatorUserAgent(describeTargetGroupsRequest));
}
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* @return Result of the DescribeTargetHealth operation returned by the service.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws HealthUnavailableException
* The health of the specified targets could not be retrieved due to an internal error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.DescribeTargetHealth
* @see AWS API Documentation
*/
@Override
public DescribeTargetHealthResponse describeTargetHealth(DescribeTargetHealthRequest describeTargetHealthRequest)
throws InvalidTargetException, TargetGroupNotFoundException, HealthUnavailableException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetHealthResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetHealth").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTargetHealthRequest)
.withMarshaller(new DescribeTargetHealthRequestMarshaller(protocolFactory)));
}
/**
*
* Replaces the specified properties of the specified listener. Any properties that you do not specify remain
* unchanged.
*
*
* Changing the protocol from HTTPS to HTTP, or from TLS to TCP, removes the security policy and default certificate
* properties. If you change the protocol from HTTP to HTTPS, or from TCP to TLS, you must add the security policy
* and default certificate properties.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyListenerRequest
* @return Result of the ModifyListener operation returned by the service.
* @throws DuplicateListenerException
* A listener with the specified port already exists.
* @throws TooManyListenersException
* You've reached the limit on the number of listeners per load balancer.
* @throws TooManyCertificatesException
* You've reached the limit on the number of certificates per load balancer.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws SslPolicyNotFoundException
* The specified SSL policy does not exist.
* @throws CertificateNotFoundException
* The specified certificate does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.ModifyListener
* @see AWS API Documentation
*/
@Override
public ModifyListenerResponse modifyListener(ModifyListenerRequest modifyListenerRequest) throws DuplicateListenerException,
TooManyListenersException, TooManyCertificatesException, ListenerNotFoundException, TargetGroupNotFoundException,
TargetGroupAssociationLimitException, IncompatibleProtocolsException, SslPolicyNotFoundException,
CertificateNotFoundException, InvalidConfigurationRequestException, UnsupportedProtocolException,
TooManyRegistrationsForTargetIdException, TooManyTargetsException, TooManyActionsException,
InvalidLoadBalancerActionException, TooManyUniqueTargetGroupsPerLoadBalancerException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyListener").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(modifyListenerRequest)
.withMarshaller(new ModifyListenerRequestMarshaller(protocolFactory)));
}
/**
*
* Modifies the specified attributes of the specified Application Load Balancer or Network Load Balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* @return Result of the ModifyLoadBalancerAttributes operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public ModifyLoadBalancerAttributesResponse modifyLoadBalancerAttributes(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest) throws LoadBalancerNotFoundException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyLoadBalancerAttributes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(modifyLoadBalancerAttributesRequest)
.withMarshaller(new ModifyLoadBalancerAttributesRequestMarshaller(protocolFactory)));
}
/**
*
* Replaces the specified properties of the specified rule. Any properties that you do not specify are unchanged.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* To modify the actions for the default rule, use ModifyListener.
*
*
* @param modifyRuleRequest
* @return Result of the ModifyRule operation returned by the service.
* @throws TargetGroupAssociationLimitException
* You've reached the limit on the number of load balancers per target group.
* @throws IncompatibleProtocolsException
* The specified configuration is not valid with this protocol.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws UnsupportedProtocolException
* The specified protocol is not supported.
* @throws TooManyActionsException
* You've reached the limit on the number of actions per rule.
* @throws InvalidLoadBalancerActionException
* The requested action is not valid.
* @throws TooManyUniqueTargetGroupsPerLoadBalancerException
* You've reached the limit on the number of unique target groups per load balancer across all listeners. If
* a target group is used by multiple actions for a load balancer, it is counted as only one use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.ModifyRule
* @see AWS API Documentation
*/
@Override
public ModifyRuleResponse modifyRule(ModifyRuleRequest modifyRuleRequest) throws TargetGroupAssociationLimitException,
IncompatibleProtocolsException, RuleNotFoundException, OperationNotPermittedException,
TooManyRegistrationsForTargetIdException, TooManyTargetsException, TargetGroupNotFoundException,
UnsupportedProtocolException, TooManyActionsException, InvalidLoadBalancerActionException,
TooManyUniqueTargetGroupsPerLoadBalancerException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyRule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(modifyRuleRequest)
.withMarshaller(new ModifyRuleRequestMarshaller(protocolFactory)));
}
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* To monitor the health of the targets, use DescribeTargetHealth.
*
*
* @param modifyTargetGroupRequest
* @return Result of the ModifyTargetGroup operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.ModifyTargetGroup
* @see AWS API Documentation
*/
@Override
public ModifyTargetGroupResponse modifyTargetGroup(ModifyTargetGroupRequest modifyTargetGroupRequest)
throws TargetGroupNotFoundException, InvalidConfigurationRequestException, AwsServiceException, SdkClientException,
ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyTargetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(modifyTargetGroupRequest)
.withMarshaller(new ModifyTargetGroupRequestMarshaller(protocolFactory)));
}
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* @return Result of the ModifyTargetGroupAttributes operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.ModifyTargetGroupAttributes
* @see AWS API Documentation
*/
@Override
public ModifyTargetGroupAttributesResponse modifyTargetGroupAttributes(
ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest) throws TargetGroupNotFoundException,
InvalidConfigurationRequestException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyTargetGroupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyTargetGroupAttributes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(modifyTargetGroupAttributesRequest)
.withMarshaller(new ModifyTargetGroupAttributesRequestMarshaller(protocolFactory)));
}
/**
*
* Registers the specified targets with the specified target group.
*
*
* If the target is an EC2 instance, it must be in the running
state when you register it.
*
*
* By default, the load balancer routes requests to registered targets using the protocol and port for the target
* group. Alternatively, you can override the port for a target when you register it. You can register each EC2
* instance or IP address with the same target group multiple times using different ports.
*
*
* With a Network Load Balancer, you cannot register instances by instance ID if they have the following instance
* types: C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1. You can register instances of
* these types by IP address.
*
*
* To remove a target from a target group, use DeregisterTargets.
*
*
* @param registerTargetsRequest
* @return Result of the RegisterTargets operation returned by the service.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws TooManyTargetsException
* You've reached the limit on the number of targets.
* @throws InvalidTargetException
* The specified target does not exist, is not in the same VPC as the target group, or has an unsupported
* instance type.
* @throws TooManyRegistrationsForTargetIdException
* You've reached the limit on the number of times a target can be registered with a load balancer.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.RegisterTargets
* @see AWS API Documentation
*/
@Override
public RegisterTargetsResponse registerTargets(RegisterTargetsRequest registerTargetsRequest)
throws TargetGroupNotFoundException, TooManyTargetsException, InvalidTargetException,
TooManyRegistrationsForTargetIdException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RegisterTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterTargets").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerTargetsRequest)
.withMarshaller(new RegisterTargetsRequestMarshaller(protocolFactory)));
}
/**
*
* Removes the specified certificate from the certificate list for the specified HTTPS or TLS listener.
*
*
* You can't remove the default certificate for a listener. To replace the default certificate, call
* ModifyListener.
*
*
* To list the certificates for your listener, use DescribeListenerCertificates.
*
*
* @param removeListenerCertificatesRequest
* @return Result of the RemoveListenerCertificates operation returned by the service.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.RemoveListenerCertificates
* @see AWS API Documentation
*/
@Override
public RemoveListenerCertificatesResponse removeListenerCertificates(
RemoveListenerCertificatesRequest removeListenerCertificatesRequest) throws ListenerNotFoundException,
OperationNotPermittedException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveListenerCertificates").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(removeListenerCertificatesRequest)
.withMarshaller(new RemoveListenerCertificatesRequestMarshaller(protocolFactory)));
}
/**
*
* Removes the specified tags from the specified Elastic Load Balancing resource.
*
*
* To list the current tags for your resources, use DescribeTags.
*
*
* @param removeTagsRequest
* @return Result of the RemoveTags operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws TargetGroupNotFoundException
* The specified target group does not exist.
* @throws ListenerNotFoundException
* The specified listener does not exist.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws TooManyTagsException
* You've reached the limit on the number of tags per load balancer.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.RemoveTags
* @see AWS API Documentation
*/
@Override
public RemoveTagsResponse removeTags(RemoveTagsRequest removeTagsRequest) throws LoadBalancerNotFoundException,
TargetGroupNotFoundException, ListenerNotFoundException, RuleNotFoundException, TooManyTagsException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RemoveTags").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(removeTagsRequest)
.withMarshaller(new RemoveTagsRequestMarshaller(protocolFactory)));
}
/**
*
* Sets the type of IP addresses used by the subnets of the specified Application Load Balancer or Network Load
* Balancer.
*
*
* @param setIpAddressTypeRequest
* @return Result of the SetIpAddressType operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.SetIpAddressType
* @see AWS API Documentation
*/
@Override
public SetIpAddressTypeResponse setIpAddressType(SetIpAddressTypeRequest setIpAddressTypeRequest)
throws LoadBalancerNotFoundException, InvalidConfigurationRequestException, InvalidSubnetException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetIpAddressTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetIpAddressType").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setIpAddressTypeRequest)
.withMarshaller(new SetIpAddressTypeRequestMarshaller(protocolFactory)));
}
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* @return Result of the SetRulePriorities operation returned by the service.
* @throws RuleNotFoundException
* The specified rule does not exist.
* @throws PriorityInUseException
* The specified priority is in use.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.SetRulePriorities
* @see AWS API Documentation
*/
@Override
public SetRulePrioritiesResponse setRulePriorities(SetRulePrioritiesRequest setRulePrioritiesRequest)
throws RuleNotFoundException, PriorityInUseException, OperationNotPermittedException, AwsServiceException,
SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetRulePrioritiesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetRulePriorities").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setRulePrioritiesRequest)
.withMarshaller(new SetRulePrioritiesRequestMarshaller(protocolFactory)));
}
/**
*
* Associates the specified security groups with the specified Application Load Balancer. The specified security
* groups override the previously associated security groups.
*
*
* You can't specify a security group for a Network Load Balancer.
*
*
* @param setSecurityGroupsRequest
* @return Result of the SetSecurityGroups operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws InvalidSecurityGroupException
* The specified security group does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.SetSecurityGroups
* @see AWS API Documentation
*/
@Override
public SetSecurityGroupsResponse setSecurityGroups(SetSecurityGroupsRequest setSecurityGroupsRequest)
throws LoadBalancerNotFoundException, InvalidConfigurationRequestException, InvalidSecurityGroupException,
AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetSecurityGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetSecurityGroups").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setSecurityGroupsRequest)
.withMarshaller(new SetSecurityGroupsRequestMarshaller(protocolFactory)));
}
/**
*
* Enables the Availability Zones for the specified public subnets for the specified load balancer. The specified
* subnets replace the previously enabled subnets.
*
*
* When you specify subnets for a Network Load Balancer, you must include all subnets that were enabled previously,
* with their existing configurations, plus any additional subnets.
*
*
* @param setSubnetsRequest
* @return Result of the SetSubnets operation returned by the service.
* @throws LoadBalancerNotFoundException
* The specified load balancer does not exist.
* @throws InvalidConfigurationRequestException
* The requested configuration is not valid.
* @throws SubnetNotFoundException
* The specified subnet does not exist.
* @throws InvalidSubnetException
* The specified subnet is out of available addresses.
* @throws AllocationIdNotFoundException
* The specified allocation ID does not exist.
* @throws AvailabilityZoneNotSupportedException
* The specified Availability Zone is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ElasticLoadBalancingV2Exception
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ElasticLoadBalancingV2Client.SetSubnets
* @see AWS API Documentation
*/
@Override
public SetSubnetsResponse setSubnets(SetSubnetsRequest setSubnetsRequest) throws LoadBalancerNotFoundException,
InvalidConfigurationRequestException, SubnetNotFoundException, InvalidSubnetException, AllocationIdNotFoundException,
AvailabilityZoneNotSupportedException, AwsServiceException, SdkClientException, ElasticLoadBalancingV2Exception {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetSubnetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetSubnets").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setSubnetsRequest)
.withMarshaller(new SetSubnetsRequestMarshaller(protocolFactory)));
}
private AwsQueryProtocolFactory init() {
return AwsQueryProtocolFactory
.builder()
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedProtocol")
.exceptionBuilderSupplier(UnsupportedProtocolException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyUniqueTargetGroupsPerLoadBalancer")
.exceptionBuilderSupplier(TooManyUniqueTargetGroupsPerLoadBalancerException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AllocationIdNotFound")
.exceptionBuilderSupplier(AllocationIdNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceInUse")
.exceptionBuilderSupplier(ResourceInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateTargetGroupName")
.exceptionBuilderSupplier(DuplicateTargetGroupNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRules")
.exceptionBuilderSupplier(TooManyRulesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyCertificates")
.exceptionBuilderSupplier(TooManyCertificatesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LoadBalancerNotFound")
.exceptionBuilderSupplier(LoadBalancerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTargetGroups")
.exceptionBuilderSupplier(TooManyTargetGroupsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyLoadBalancers")
.exceptionBuilderSupplier(TooManyLoadBalancersException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidConfigurationRequest")
.exceptionBuilderSupplier(InvalidConfigurationRequestException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TargetGroupAssociationLimit")
.exceptionBuilderSupplier(TargetGroupAssociationLimitException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSecurityGroup")
.exceptionBuilderSupplier(InvalidSecurityGroupException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IncompatibleProtocols")
.exceptionBuilderSupplier(IncompatibleProtocolsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyActions")
.exceptionBuilderSupplier(TooManyActionsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyListeners")
.exceptionBuilderSupplier(TooManyListenersException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateLoadBalancerName")
.exceptionBuilderSupplier(DuplicateLoadBalancerNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotPermitted")
.exceptionBuilderSupplier(OperationNotPermittedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLoadBalancerAction")
.exceptionBuilderSupplier(InvalidLoadBalancerActionException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("CertificateNotFound")
.exceptionBuilderSupplier(CertificateNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateTagKeys")
.exceptionBuilderSupplier(DuplicateTagKeysException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTags")
.exceptionBuilderSupplier(TooManyTagsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TargetGroupNotFound")
.exceptionBuilderSupplier(TargetGroupNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRegistrationsForTargetId")
.exceptionBuilderSupplier(TooManyRegistrationsForTargetIdException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ListenerNotFound")
.exceptionBuilderSupplier(ListenerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PriorityInUse")
.exceptionBuilderSupplier(PriorityInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTarget")
.exceptionBuilderSupplier(InvalidTargetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetNotFound")
.exceptionBuilderSupplier(SubnetNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AvailabilityZoneNotSupported")
.exceptionBuilderSupplier(AvailabilityZoneNotSupportedException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RuleNotFound")
.exceptionBuilderSupplier(RuleNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SSLPolicyNotFound")
.exceptionBuilderSupplier(SslPolicyNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateListener")
.exceptionBuilderSupplier(DuplicateListenerException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidScheme")
.exceptionBuilderSupplier(InvalidSchemeException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("HealthUnavailable")
.exceptionBuilderSupplier(HealthUnavailableException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSubnet")
.exceptionBuilderSupplier(InvalidSubnetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTargets")
.exceptionBuilderSupplier(TooManyTargetsException::builder).httpStatusCode(400).build())
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(ElasticLoadBalancingV2Exception::builder).build();
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}