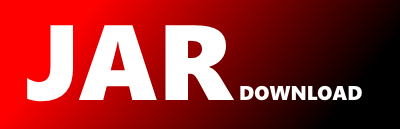
software.amazon.awssdk.services.elasticloadbalancingv2.model.Action Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about an action.
*
*
* Each rule must include exactly one of the following types of actions: forward
,
* fixed-response
, or redirect
, and it must be the last action to be performed.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Action implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(Action::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField TARGET_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetGroupArn").getter(getter(Action::targetGroupArn)).setter(setter(Builder::targetGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetGroupArn").build()).build();
private static final SdkField AUTHENTICATE_OIDC_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AuthenticateOidcConfig")
.getter(getter(Action::authenticateOidcConfig)).setter(setter(Builder::authenticateOidcConfig))
.constructor(AuthenticateOidcActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthenticateOidcConfig").build())
.build();
private static final SdkField AUTHENTICATE_COGNITO_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AuthenticateCognitoConfig")
.getter(getter(Action::authenticateCognitoConfig)).setter(setter(Builder::authenticateCognitoConfig))
.constructor(AuthenticateCognitoActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthenticateCognitoConfig").build())
.build();
private static final SdkField ORDER_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Order")
.getter(getter(Action::order)).setter(setter(Builder::order))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Order").build()).build();
private static final SdkField REDIRECT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RedirectConfig")
.getter(getter(Action::redirectConfig)).setter(setter(Builder::redirectConfig))
.constructor(RedirectActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedirectConfig").build()).build();
private static final SdkField FIXED_RESPONSE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("FixedResponseConfig")
.getter(getter(Action::fixedResponseConfig)).setter(setter(Builder::fixedResponseConfig))
.constructor(FixedResponseActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FixedResponseConfig").build())
.build();
private static final SdkField FORWARD_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ForwardConfig")
.getter(getter(Action::forwardConfig)).setter(setter(Builder::forwardConfig))
.constructor(ForwardActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForwardConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD,
TARGET_GROUP_ARN_FIELD, AUTHENTICATE_OIDC_CONFIG_FIELD, AUTHENTICATE_COGNITO_CONFIG_FIELD, ORDER_FIELD,
REDIRECT_CONFIG_FIELD, FIXED_RESPONSE_CONFIG_FIELD, FORWARD_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String targetGroupArn;
private final AuthenticateOidcActionConfig authenticateOidcConfig;
private final AuthenticateCognitoActionConfig authenticateCognitoConfig;
private final Integer order;
private final RedirectActionConfig redirectConfig;
private final FixedResponseActionConfig fixedResponseConfig;
private final ForwardActionConfig forwardConfig;
private Action(BuilderImpl builder) {
this.type = builder.type;
this.targetGroupArn = builder.targetGroupArn;
this.authenticateOidcConfig = builder.authenticateOidcConfig;
this.authenticateCognitoConfig = builder.authenticateCognitoConfig;
this.order = builder.order;
this.redirectConfig = builder.redirectConfig;
this.fixedResponseConfig = builder.fixedResponseConfig;
this.forwardConfig = builder.forwardConfig;
}
/**
*
* The type of action.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ActionTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of action.
* @see ActionTypeEnum
*/
public final ActionTypeEnum type() {
return ActionTypeEnum.fromValue(type);
}
/**
*
* The type of action.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ActionTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of action.
* @see ActionTypeEnum
*/
public final String typeAsString() {
return type;
}
/**
*
* The Amazon Resource Name (ARN) of the target group. Specify only when Type
is forward
* and you want to route to a single target group. To route to one or more target groups, use
* ForwardConfig
instead.
*
*
* @return The Amazon Resource Name (ARN) of the target group. Specify only when Type
is
* forward
and you want to route to a single target group. To route to one or more target
* groups, use ForwardConfig
instead.
*/
public final String targetGroupArn() {
return targetGroupArn;
}
/**
*
* [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC). Specify
* only when Type
is authenticate-oidc
.
*
*
* @return [HTTPS listeners] Information about an identity provider that is compliant with OpenID Connect (OIDC).
* Specify only when Type
is authenticate-oidc
.
*/
public final AuthenticateOidcActionConfig authenticateOidcConfig() {
return authenticateOidcConfig;
}
/**
*
* [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when Type
* is authenticate-cognito
.
*
*
* @return [HTTPS listeners] Information for using Amazon Cognito to authenticate users. Specify only when
* Type
is authenticate-cognito
.
*/
public final AuthenticateCognitoActionConfig authenticateCognitoConfig() {
return authenticateCognitoConfig;
}
/**
*
* The order for the action. This value is required for rules with multiple actions. The action with the lowest
* value for order is performed first.
*
*
* @return The order for the action. This value is required for rules with multiple actions. The action with the
* lowest value for order is performed first.
*/
public final Integer order() {
return order;
}
/**
*
* [Application Load Balancer] Information for creating a redirect action. Specify only when Type
is
* redirect
.
*
*
* @return [Application Load Balancer] Information for creating a redirect action. Specify only when
* Type
is redirect
.
*/
public final RedirectActionConfig redirectConfig() {
return redirectConfig;
}
/**
*
* [Application Load Balancer] Information for creating an action that returns a custom HTTP response. Specify only
* when Type
is fixed-response
.
*
*
* @return [Application Load Balancer] Information for creating an action that returns a custom HTTP response.
* Specify only when Type
is fixed-response
.
*/
public final FixedResponseActionConfig fixedResponseConfig() {
return fixedResponseConfig;
}
/**
*
* Information for creating an action that distributes requests among one or more target groups. For Network Load
* Balancers, you can specify a single target group. Specify only when Type
is forward
. If
* you specify both ForwardConfig
and TargetGroupArn
, you can specify only one target
* group using ForwardConfig
and it must be the same target group specified in
* TargetGroupArn
.
*
*
* @return Information for creating an action that distributes requests among one or more target groups. For Network
* Load Balancers, you can specify a single target group. Specify only when Type
is
* forward
. If you specify both ForwardConfig
and TargetGroupArn
, you
* can specify only one target group using ForwardConfig
and it must be the same target group
* specified in TargetGroupArn
.
*/
public final ForwardActionConfig forwardConfig() {
return forwardConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(targetGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(authenticateOidcConfig());
hashCode = 31 * hashCode + Objects.hashCode(authenticateCognitoConfig());
hashCode = 31 * hashCode + Objects.hashCode(order());
hashCode = 31 * hashCode + Objects.hashCode(redirectConfig());
hashCode = 31 * hashCode + Objects.hashCode(fixedResponseConfig());
hashCode = 31 * hashCode + Objects.hashCode(forwardConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Action)) {
return false;
}
Action other = (Action) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(targetGroupArn(), other.targetGroupArn())
&& Objects.equals(authenticateOidcConfig(), other.authenticateOidcConfig())
&& Objects.equals(authenticateCognitoConfig(), other.authenticateCognitoConfig())
&& Objects.equals(order(), other.order()) && Objects.equals(redirectConfig(), other.redirectConfig())
&& Objects.equals(fixedResponseConfig(), other.fixedResponseConfig())
&& Objects.equals(forwardConfig(), other.forwardConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Action").add("Type", typeAsString()).add("TargetGroupArn", targetGroupArn())
.add("AuthenticateOidcConfig", authenticateOidcConfig())
.add("AuthenticateCognitoConfig", authenticateCognitoConfig()).add("Order", order())
.add("RedirectConfig", redirectConfig()).add("FixedResponseConfig", fixedResponseConfig())
.add("ForwardConfig", forwardConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "TargetGroupArn":
return Optional.ofNullable(clazz.cast(targetGroupArn()));
case "AuthenticateOidcConfig":
return Optional.ofNullable(clazz.cast(authenticateOidcConfig()));
case "AuthenticateCognitoConfig":
return Optional.ofNullable(clazz.cast(authenticateCognitoConfig()));
case "Order":
return Optional.ofNullable(clazz.cast(order()));
case "RedirectConfig":
return Optional.ofNullable(clazz.cast(redirectConfig()));
case "FixedResponseConfig":
return Optional.ofNullable(clazz.cast(fixedResponseConfig()));
case "ForwardConfig":
return Optional.ofNullable(clazz.cast(forwardConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function