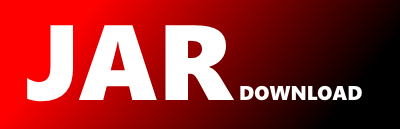
software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupRequest Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateTargetGroupRequest extends ElasticLoadBalancingV2Request implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(CreateTargetGroupRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Protocol").getter(getter(CreateTargetGroupRequest::protocolAsString)).setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Protocol").build()).build();
private static final SdkField PROTOCOL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProtocolVersion").getter(getter(CreateTargetGroupRequest::protocolVersion))
.setter(setter(Builder::protocolVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProtocolVersion").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(CreateTargetGroupRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(CreateTargetGroupRequest::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField HEALTH_CHECK_PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckProtocol").getter(getter(CreateTargetGroupRequest::healthCheckProtocolAsString))
.setter(setter(Builder::healthCheckProtocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckProtocol").build())
.build();
private static final SdkField HEALTH_CHECK_PORT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPort").getter(getter(CreateTargetGroupRequest::healthCheckPort))
.setter(setter(Builder::healthCheckPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPort").build()).build();
private static final SdkField HEALTH_CHECK_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HealthCheckEnabled").getter(getter(CreateTargetGroupRequest::healthCheckEnabled))
.setter(setter(Builder::healthCheckEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckEnabled").build())
.build();
private static final SdkField HEALTH_CHECK_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPath").getter(getter(CreateTargetGroupRequest::healthCheckPath))
.setter(setter(Builder::healthCheckPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPath").build()).build();
private static final SdkField HEALTH_CHECK_INTERVAL_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("HealthCheckIntervalSeconds")
.getter(getter(CreateTargetGroupRequest::healthCheckIntervalSeconds))
.setter(setter(Builder::healthCheckIntervalSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckIntervalSeconds").build())
.build();
private static final SdkField HEALTH_CHECK_TIMEOUT_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("HealthCheckTimeoutSeconds")
.getter(getter(CreateTargetGroupRequest::healthCheckTimeoutSeconds))
.setter(setter(Builder::healthCheckTimeoutSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckTimeoutSeconds").build())
.build();
private static final SdkField HEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("HealthyThresholdCount").getter(getter(CreateTargetGroupRequest::healthyThresholdCount))
.setter(setter(Builder::healthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthyThresholdCount").build())
.build();
private static final SdkField UNHEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("UnhealthyThresholdCount").getter(getter(CreateTargetGroupRequest::unhealthyThresholdCount))
.setter(setter(Builder::unhealthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnhealthyThresholdCount").build())
.build();
private static final SdkField MATCHER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Matcher").getter(getter(CreateTargetGroupRequest::matcher)).setter(setter(Builder::matcher))
.constructor(Matcher::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Matcher").build()).build();
private static final SdkField TARGET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetType").getter(getter(CreateTargetGroupRequest::targetTypeAsString))
.setter(setter(Builder::targetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetType").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateTargetGroupRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, PROTOCOL_FIELD,
PROTOCOL_VERSION_FIELD, PORT_FIELD, VPC_ID_FIELD, HEALTH_CHECK_PROTOCOL_FIELD, HEALTH_CHECK_PORT_FIELD,
HEALTH_CHECK_ENABLED_FIELD, HEALTH_CHECK_PATH_FIELD, HEALTH_CHECK_INTERVAL_SECONDS_FIELD,
HEALTH_CHECK_TIMEOUT_SECONDS_FIELD, HEALTHY_THRESHOLD_COUNT_FIELD, UNHEALTHY_THRESHOLD_COUNT_FIELD, MATCHER_FIELD,
TARGET_TYPE_FIELD, TAGS_FIELD));
private final String name;
private final String protocol;
private final String protocolVersion;
private final Integer port;
private final String vpcId;
private final String healthCheckProtocol;
private final String healthCheckPort;
private final Boolean healthCheckEnabled;
private final String healthCheckPath;
private final Integer healthCheckIntervalSeconds;
private final Integer healthCheckTimeoutSeconds;
private final Integer healthyThresholdCount;
private final Integer unhealthyThresholdCount;
private final Matcher matcher;
private final String targetType;
private final List tags;
private CreateTargetGroupRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.protocol = builder.protocol;
this.protocolVersion = builder.protocolVersion;
this.port = builder.port;
this.vpcId = builder.vpcId;
this.healthCheckProtocol = builder.healthCheckProtocol;
this.healthCheckPort = builder.healthCheckPort;
this.healthCheckEnabled = builder.healthCheckEnabled;
this.healthCheckPath = builder.healthCheckPath;
this.healthCheckIntervalSeconds = builder.healthCheckIntervalSeconds;
this.healthCheckTimeoutSeconds = builder.healthCheckTimeoutSeconds;
this.healthyThresholdCount = builder.healthyThresholdCount;
this.unhealthyThresholdCount = builder.unhealthyThresholdCount;
this.matcher = builder.matcher;
this.targetType = builder.targetType;
this.tags = builder.tags;
}
/**
*
* The name of the target group.
*
*
* This name must be unique per region per account, can have a maximum of 32 characters, must contain only
* alphanumeric characters or hyphens, and must not begin or end with a hyphen.
*
*
* @return The name of the target group.
*
* This name must be unique per region per account, can have a maximum of 32 characters, must contain only
* alphanumeric characters or hyphens, and must not begin or end with a hyphen.
*/
public final String name() {
return name;
}
/**
*
* The protocol to use for routing traffic to the targets. For Application Load Balancers, the supported protocols
* are HTTP and HTTPS. For Network Load Balancers, the supported protocols are TCP, TLS, UDP, or TCP_UDP. For
* Gateway Load Balancers, the supported protocol is GENEVE. A TCP_UDP listener must be associated with a TCP_UDP
* target group. If the target is a Lambda function, this parameter does not apply.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol to use for routing traffic to the targets. For Application Load Balancers, the supported
* protocols are HTTP and HTTPS. For Network Load Balancers, the supported protocols are TCP, TLS, UDP, or
* TCP_UDP. For Gateway Load Balancers, the supported protocol is GENEVE. A TCP_UDP listener must be
* associated with a TCP_UDP target group. If the target is a Lambda function, this parameter does not
* apply.
* @see ProtocolEnum
*/
public final ProtocolEnum protocol() {
return ProtocolEnum.fromValue(protocol);
}
/**
*
* The protocol to use for routing traffic to the targets. For Application Load Balancers, the supported protocols
* are HTTP and HTTPS. For Network Load Balancers, the supported protocols are TCP, TLS, UDP, or TCP_UDP. For
* Gateway Load Balancers, the supported protocol is GENEVE. A TCP_UDP listener must be associated with a TCP_UDP
* target group. If the target is a Lambda function, this parameter does not apply.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol to use for routing traffic to the targets. For Application Load Balancers, the supported
* protocols are HTTP and HTTPS. For Network Load Balancers, the supported protocols are TCP, TLS, UDP, or
* TCP_UDP. For Gateway Load Balancers, the supported protocol is GENEVE. A TCP_UDP listener must be
* associated with a TCP_UDP target group. If the target is a Lambda function, this parameter does not
* apply.
* @see ProtocolEnum
*/
public final String protocolAsString() {
return protocol;
}
/**
*
* [HTTP/HTTPS protocol] The protocol version. Specify GRPC
to send requests to targets using gRPC.
* Specify HTTP2
to send requests to targets using HTTP/2. The default is HTTP1
, which
* sends requests to targets using HTTP/1.1.
*
*
* @return [HTTP/HTTPS protocol] The protocol version. Specify GRPC
to send requests to targets using
* gRPC. Specify HTTP2
to send requests to targets using HTTP/2. The default is
* HTTP1
, which sends requests to targets using HTTP/1.1.
*/
public final String protocolVersion() {
return protocolVersion;
}
/**
*
* The port on which the targets receive traffic. This port is used unless you specify a port override when
* registering the target. If the target is a Lambda function, this parameter does not apply. If the protocol is
* GENEVE, the supported port is 6081.
*
*
* @return The port on which the targets receive traffic. This port is used unless you specify a port override when
* registering the target. If the target is a Lambda function, this parameter does not apply. If the
* protocol is GENEVE, the supported port is 6081.
*/
public final Integer port() {
return port;
}
/**
*
* The identifier of the virtual private cloud (VPC). If the target is a Lambda function, this parameter does not
* apply. Otherwise, this parameter is required.
*
*
* @return The identifier of the virtual private cloud (VPC). If the target is a Lambda function, this parameter
* does not apply. Otherwise, this parameter is required.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The protocol the load balancer uses when performing health checks on targets. For Application Load Balancers, the
* default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is TCP. The TCP protocol is
* not supported for health checks if the protocol of the target group is HTTP or HTTPS. The GENEVE, TLS, UDP, and
* TCP_UDP protocols are not supported for health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol the load balancer uses when performing health checks on targets. For Application Load
* Balancers, the default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is
* TCP. The TCP protocol is not supported for health checks if the protocol of the target group is HTTP or
* HTTPS. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for health checks.
* @see ProtocolEnum
*/
public final ProtocolEnum healthCheckProtocol() {
return ProtocolEnum.fromValue(healthCheckProtocol);
}
/**
*
* The protocol the load balancer uses when performing health checks on targets. For Application Load Balancers, the
* default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is TCP. The TCP protocol is
* not supported for health checks if the protocol of the target group is HTTP or HTTPS. The GENEVE, TLS, UDP, and
* TCP_UDP protocols are not supported for health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol the load balancer uses when performing health checks on targets. For Application Load
* Balancers, the default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is
* TCP. The TCP protocol is not supported for health checks if the protocol of the target group is HTTP or
* HTTPS. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for health checks.
* @see ProtocolEnum
*/
public final String healthCheckProtocolAsString() {
return healthCheckProtocol;
}
/**
*
* The port the load balancer uses when performing health checks on targets. If the protocol is HTTP, HTTPS, TCP,
* TLS, UDP, or TCP_UDP, the default is traffic-port
, which is the port on which each target receives
* traffic from the load balancer. If the protocol is GENEVE, the default is port 80.
*
*
* @return The port the load balancer uses when performing health checks on targets. If the protocol is HTTP, HTTPS,
* TCP, TLS, UDP, or TCP_UDP, the default is traffic-port
, which is the port on which each
* target receives traffic from the load balancer. If the protocol is GENEVE, the default is port 80.
*/
public final String healthCheckPort() {
return healthCheckPort;
}
/**
*
* Indicates whether health checks are enabled. If the target type is lambda
, health checks are
* disabled by default but can be enabled. If the target type is instance
or ip
, health
* checks are always enabled and cannot be disabled.
*
*
* @return Indicates whether health checks are enabled. If the target type is lambda
, health checks are
* disabled by default but can be enabled. If the target type is instance
or ip
,
* health checks are always enabled and cannot be disabled.
*/
public final Boolean healthCheckEnabled() {
return healthCheckEnabled;
}
/**
*
* [HTTP/HTTPS health checks] The destination for health checks on the targets.
*
*
* [HTTP1 or HTTP2 protocol version] The ping path. The default is /.
*
*
* [GRPC protocol version] The path of a custom health check method with the format /package.service/method. The
* default is /AWS.ALB/healthcheck.
*
*
* @return [HTTP/HTTPS health checks] The destination for health checks on the targets.
*
* [HTTP1 or HTTP2 protocol version] The ping path. The default is /.
*
*
* [GRPC protocol version] The path of a custom health check method with the format /package.service/method.
* The default is /AWS.ALB/healthcheck.
*/
public final String healthCheckPath() {
return healthCheckPath;
}
/**
*
* The approximate amount of time, in seconds, between health checks of an individual target. If the target group
* protocol is TCP, TLS, UDP, or TCP_UDP, the supported values are 10 and 30 seconds. If the target group protocol
* is HTTP or HTTPS, the default is 30 seconds. If the target group protocol is GENEVE, the default is 10 seconds.
* If the target type is lambda
, the default is 35 seconds.
*
*
* @return The approximate amount of time, in seconds, between health checks of an individual target. If the target
* group protocol is TCP, TLS, UDP, or TCP_UDP, the supported values are 10 and 30 seconds. If the target
* group protocol is HTTP or HTTPS, the default is 30 seconds. If the target group protocol is GENEVE, the
* default is 10 seconds. If the target type is lambda
, the default is 35 seconds.
*/
public final Integer healthCheckIntervalSeconds() {
return healthCheckIntervalSeconds;
}
/**
*
* The amount of time, in seconds, during which no response from a target means a failed health check. For target
* groups with a protocol of HTTP, HTTPS, or GENEVE, the default is 5 seconds. For target groups with a protocol of
* TCP or TLS, this value must be 6 seconds for HTTP health checks and 10 seconds for TCP and HTTPS health checks.
* If the target type is lambda
, the default is 30 seconds.
*
*
* @return The amount of time, in seconds, during which no response from a target means a failed health check. For
* target groups with a protocol of HTTP, HTTPS, or GENEVE, the default is 5 seconds. For target groups with
* a protocol of TCP or TLS, this value must be 6 seconds for HTTP health checks and 10 seconds for TCP and
* HTTPS health checks. If the target type is lambda
, the default is 30 seconds.
*/
public final Integer healthCheckTimeoutSeconds() {
return healthCheckTimeoutSeconds;
}
/**
*
* The number of consecutive health checks successes required before considering an unhealthy target healthy. For
* target groups with a protocol of HTTP or HTTPS, the default is 5. For target groups with a protocol of TCP, TLS,
* or GENEVE, the default is 3. If the target type is lambda
, the default is 5.
*
*
* @return The number of consecutive health checks successes required before considering an unhealthy target
* healthy. For target groups with a protocol of HTTP or HTTPS, the default is 5. For target groups with a
* protocol of TCP, TLS, or GENEVE, the default is 3. If the target type is lambda
, the default
* is 5.
*/
public final Integer healthyThresholdCount() {
return healthyThresholdCount;
}
/**
*
* The number of consecutive health check failures required before considering a target unhealthy. If the target
* group protocol is HTTP or HTTPS, the default is 2. If the target group protocol is TCP or TLS, this value must be
* the same as the healthy threshold count. If the target group protocol is GENEVE, the default is 3. If the target
* type is lambda
, the default is 2.
*
*
* @return The number of consecutive health check failures required before considering a target unhealthy. If the
* target group protocol is HTTP or HTTPS, the default is 2. If the target group protocol is TCP or TLS,
* this value must be the same as the healthy threshold count. If the target group protocol is GENEVE, the
* default is 3. If the target type is lambda
, the default is 2.
*/
public final Integer unhealthyThresholdCount() {
return unhealthyThresholdCount;
}
/**
*
* [HTTP/HTTPS health checks] The HTTP or gRPC codes to use when checking for a successful response from a target.
*
*
* @return [HTTP/HTTPS health checks] The HTTP or gRPC codes to use when checking for a successful response from a
* target.
*/
public final Matcher matcher() {
return matcher;
}
/**
*
* The type of target that you must specify when registering targets with this target group. You can't specify
* targets for a target group using more than one target type.
*
*
* -
*
* instance
- Register targets by instance ID. This is the default value.
*
*
* -
*
* ip
- Register targets by IP address. You can specify IP addresses from the subnets of the virtual
* private cloud (VPC) for the target group, the RFC 1918 range (10.0.0.0/8, 172.16.0.0/12, and 192.168.0.0/16), and
* the RFC 6598 range (100.64.0.0/10). You can't specify publicly routable IP addresses.
*
*
* -
*
* lambda
- Register a single Lambda function as a target.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of target that you must specify when registering targets with this target group. You can't
* specify targets for a target group using more than one target type.
*
* -
*
* instance
- Register targets by instance ID. This is the default value.
*
*
* -
*
* ip
- Register targets by IP address. You can specify IP addresses from the subnets of the
* virtual private cloud (VPC) for the target group, the RFC 1918 range (10.0.0.0/8, 172.16.0.0/12, and
* 192.168.0.0/16), and the RFC 6598 range (100.64.0.0/10). You can't specify publicly routable IP
* addresses.
*
*
* -
*
* lambda
- Register a single Lambda function as a target.
*
*
* @see TargetTypeEnum
*/
public final TargetTypeEnum targetType() {
return TargetTypeEnum.fromValue(targetType);
}
/**
*
* The type of target that you must specify when registering targets with this target group. You can't specify
* targets for a target group using more than one target type.
*
*
* -
*
* instance
- Register targets by instance ID. This is the default value.
*
*
* -
*
* ip
- Register targets by IP address. You can specify IP addresses from the subnets of the virtual
* private cloud (VPC) for the target group, the RFC 1918 range (10.0.0.0/8, 172.16.0.0/12, and 192.168.0.0/16), and
* the RFC 6598 range (100.64.0.0/10). You can't specify publicly routable IP addresses.
*
*
* -
*
* lambda
- Register a single Lambda function as a target.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of target that you must specify when registering targets with this target group. You can't
* specify targets for a target group using more than one target type.
*
* -
*
* instance
- Register targets by instance ID. This is the default value.
*
*
* -
*
* ip
- Register targets by IP address. You can specify IP addresses from the subnets of the
* virtual private cloud (VPC) for the target group, the RFC 1918 range (10.0.0.0/8, 172.16.0.0/12, and
* 192.168.0.0/16), and the RFC 6598 range (100.64.0.0/10). You can't specify publicly routable IP
* addresses.
*
*
* -
*
* lambda
- Register a single Lambda function as a target.
*
*
* @see TargetTypeEnum
*/
public final String targetTypeAsString() {
return targetType;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags to assign to the target group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return The tags to assign to the target group.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(protocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(protocolVersion());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckProtocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPort());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckEnabled());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPath());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckIntervalSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckTimeoutSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(unhealthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(matcher());
hashCode = 31 * hashCode + Objects.hashCode(targetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateTargetGroupRequest)) {
return false;
}
CreateTargetGroupRequest other = (CreateTargetGroupRequest) obj;
return Objects.equals(name(), other.name()) && Objects.equals(protocolAsString(), other.protocolAsString())
&& Objects.equals(protocolVersion(), other.protocolVersion()) && Objects.equals(port(), other.port())
&& Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(healthCheckProtocolAsString(), other.healthCheckProtocolAsString())
&& Objects.equals(healthCheckPort(), other.healthCheckPort())
&& Objects.equals(healthCheckEnabled(), other.healthCheckEnabled())
&& Objects.equals(healthCheckPath(), other.healthCheckPath())
&& Objects.equals(healthCheckIntervalSeconds(), other.healthCheckIntervalSeconds())
&& Objects.equals(healthCheckTimeoutSeconds(), other.healthCheckTimeoutSeconds())
&& Objects.equals(healthyThresholdCount(), other.healthyThresholdCount())
&& Objects.equals(unhealthyThresholdCount(), other.unhealthyThresholdCount())
&& Objects.equals(matcher(), other.matcher()) && Objects.equals(targetTypeAsString(), other.targetTypeAsString())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateTargetGroupRequest").add("Name", name()).add("Protocol", protocolAsString())
.add("ProtocolVersion", protocolVersion()).add("Port", port()).add("VpcId", vpcId())
.add("HealthCheckProtocol", healthCheckProtocolAsString()).add("HealthCheckPort", healthCheckPort())
.add("HealthCheckEnabled", healthCheckEnabled()).add("HealthCheckPath", healthCheckPath())
.add("HealthCheckIntervalSeconds", healthCheckIntervalSeconds())
.add("HealthCheckTimeoutSeconds", healthCheckTimeoutSeconds())
.add("HealthyThresholdCount", healthyThresholdCount()).add("UnhealthyThresholdCount", unhealthyThresholdCount())
.add("Matcher", matcher()).add("TargetType", targetTypeAsString()).add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Protocol":
return Optional.ofNullable(clazz.cast(protocolAsString()));
case "ProtocolVersion":
return Optional.ofNullable(clazz.cast(protocolVersion()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "HealthCheckProtocol":
return Optional.ofNullable(clazz.cast(healthCheckProtocolAsString()));
case "HealthCheckPort":
return Optional.ofNullable(clazz.cast(healthCheckPort()));
case "HealthCheckEnabled":
return Optional.ofNullable(clazz.cast(healthCheckEnabled()));
case "HealthCheckPath":
return Optional.ofNullable(clazz.cast(healthCheckPath()));
case "HealthCheckIntervalSeconds":
return Optional.ofNullable(clazz.cast(healthCheckIntervalSeconds()));
case "HealthCheckTimeoutSeconds":
return Optional.ofNullable(clazz.cast(healthCheckTimeoutSeconds()));
case "HealthyThresholdCount":
return Optional.ofNullable(clazz.cast(healthyThresholdCount()));
case "UnhealthyThresholdCount":
return Optional.ofNullable(clazz.cast(unhealthyThresholdCount()));
case "Matcher":
return Optional.ofNullable(clazz.cast(matcher()));
case "TargetType":
return Optional.ofNullable(clazz.cast(targetTypeAsString()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function