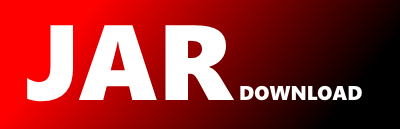
software.amazon.awssdk.services.elasticloadbalancingv2.model.RuleCondition Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a condition for a rule.
*
*
* Each rule can optionally include up to one of each of the following conditions: http-request-method
,
* host-header
, path-pattern
, and source-ip
. Each rule can also optionally
* include one or more of each of the following conditions: http-header
and query-string
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RuleCondition implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField FIELD_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Field")
.getter(getter(RuleCondition::field)).setter(setter(Builder::field))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Field").build()).build();
private static final SdkField> VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Values")
.getter(getter(RuleCondition::values))
.setter(setter(Builder::values))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Values").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField HOST_HEADER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HostHeaderConfig")
.getter(getter(RuleCondition::hostHeaderConfig)).setter(setter(Builder::hostHeaderConfig))
.constructor(HostHeaderConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HostHeaderConfig").build()).build();
private static final SdkField PATH_PATTERN_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PathPatternConfig")
.getter(getter(RuleCondition::pathPatternConfig)).setter(setter(Builder::pathPatternConfig))
.constructor(PathPatternConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PathPatternConfig").build()).build();
private static final SdkField HTTP_HEADER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HttpHeaderConfig")
.getter(getter(RuleCondition::httpHeaderConfig)).setter(setter(Builder::httpHeaderConfig))
.constructor(HttpHeaderConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpHeaderConfig").build()).build();
private static final SdkField QUERY_STRING_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("QueryStringConfig")
.getter(getter(RuleCondition::queryStringConfig)).setter(setter(Builder::queryStringConfig))
.constructor(QueryStringConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueryStringConfig").build()).build();
private static final SdkField HTTP_REQUEST_METHOD_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HttpRequestMethodConfig")
.getter(getter(RuleCondition::httpRequestMethodConfig)).setter(setter(Builder::httpRequestMethodConfig))
.constructor(HttpRequestMethodConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpRequestMethodConfig").build())
.build();
private static final SdkField SOURCE_IP_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SourceIpConfig")
.getter(getter(RuleCondition::sourceIpConfig)).setter(setter(Builder::sourceIpConfig))
.constructor(SourceIpConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceIpConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FIELD_FIELD, VALUES_FIELD,
HOST_HEADER_CONFIG_FIELD, PATH_PATTERN_CONFIG_FIELD, HTTP_HEADER_CONFIG_FIELD, QUERY_STRING_CONFIG_FIELD,
HTTP_REQUEST_METHOD_CONFIG_FIELD, SOURCE_IP_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String field;
private final List values;
private final HostHeaderConditionConfig hostHeaderConfig;
private final PathPatternConditionConfig pathPatternConfig;
private final HttpHeaderConditionConfig httpHeaderConfig;
private final QueryStringConditionConfig queryStringConfig;
private final HttpRequestMethodConditionConfig httpRequestMethodConfig;
private final SourceIpConditionConfig sourceIpConfig;
private RuleCondition(BuilderImpl builder) {
this.field = builder.field;
this.values = builder.values;
this.hostHeaderConfig = builder.hostHeaderConfig;
this.pathPatternConfig = builder.pathPatternConfig;
this.httpHeaderConfig = builder.httpHeaderConfig;
this.queryStringConfig = builder.queryStringConfig;
this.httpRequestMethodConfig = builder.httpRequestMethodConfig;
this.sourceIpConfig = builder.sourceIpConfig;
}
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*
* @return The field in the HTTP request. The following are the possible values:
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*/
public final String field() {
return field;
}
/**
* Returns true if the Values property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasValues() {
return values != null && !(values instanceof SdkAutoConstructList);
}
/**
*
* The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
*
* If Field
is host-header
and you are not using HostHeaderConfig
, you can
* specify a single host name (for example, my.example.com) in Values
. A host name is case insensitive,
* can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
, you can
* specify a single path pattern (for example, /img/*) in Values
. A path pattern is case-sensitive, can
* be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasValues()} to see if a value was sent in this field.
*
*
* @return The condition value. Specify only when Field
is host-header
or
* path-pattern
. Alternatively, to specify multiple host names or multiple path patterns, use
* HostHeaderConfig
or PathPatternConfig
.
*
* If Field
is host-header
and you are not using HostHeaderConfig
,
* you can specify a single host name (for example, my.example.com) in Values
. A host name is
* case insensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
and you are not using PathPatternConfig
,
* you can specify a single path pattern (for example, /img/*) in Values
. A path pattern is
* case-sensitive, can be up to 128 characters in length, and can contain any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*/
public final List values() {
return values;
}
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*
* @return Information for a host header condition. Specify only when Field
is host-header
* .
*/
public final HostHeaderConditionConfig hostHeaderConfig() {
return hostHeaderConfig;
}
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*
* @return Information for a path pattern condition. Specify only when Field
is
* path-pattern
.
*/
public final PathPatternConditionConfig pathPatternConfig() {
return pathPatternConfig;
}
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*
* @return Information for an HTTP header condition. Specify only when Field
is
* http-header
.
*/
public final HttpHeaderConditionConfig httpHeaderConfig() {
return httpHeaderConfig;
}
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*
* @return Information for a query string condition. Specify only when Field
is
* query-string
.
*/
public final QueryStringConditionConfig queryStringConfig() {
return queryStringConfig;
}
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*
* @return Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*/
public final HttpRequestMethodConditionConfig httpRequestMethodConfig() {
return httpRequestMethodConfig;
}
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*
* @return Information for a source IP condition. Specify only when Field
is source-ip
.
*/
public final SourceIpConditionConfig sourceIpConfig() {
return sourceIpConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(field());
hashCode = 31 * hashCode + Objects.hashCode(hasValues() ? values() : null);
hashCode = 31 * hashCode + Objects.hashCode(hostHeaderConfig());
hashCode = 31 * hashCode + Objects.hashCode(pathPatternConfig());
hashCode = 31 * hashCode + Objects.hashCode(httpHeaderConfig());
hashCode = 31 * hashCode + Objects.hashCode(queryStringConfig());
hashCode = 31 * hashCode + Objects.hashCode(httpRequestMethodConfig());
hashCode = 31 * hashCode + Objects.hashCode(sourceIpConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RuleCondition)) {
return false;
}
RuleCondition other = (RuleCondition) obj;
return Objects.equals(field(), other.field()) && hasValues() == other.hasValues()
&& Objects.equals(values(), other.values()) && Objects.equals(hostHeaderConfig(), other.hostHeaderConfig())
&& Objects.equals(pathPatternConfig(), other.pathPatternConfig())
&& Objects.equals(httpHeaderConfig(), other.httpHeaderConfig())
&& Objects.equals(queryStringConfig(), other.queryStringConfig())
&& Objects.equals(httpRequestMethodConfig(), other.httpRequestMethodConfig())
&& Objects.equals(sourceIpConfig(), other.sourceIpConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RuleCondition").add("Field", field()).add("Values", hasValues() ? values() : null)
.add("HostHeaderConfig", hostHeaderConfig()).add("PathPatternConfig", pathPatternConfig())
.add("HttpHeaderConfig", httpHeaderConfig()).add("QueryStringConfig", queryStringConfig())
.add("HttpRequestMethodConfig", httpRequestMethodConfig()).add("SourceIpConfig", sourceIpConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Field":
return Optional.ofNullable(clazz.cast(field()));
case "Values":
return Optional.ofNullable(clazz.cast(values()));
case "HostHeaderConfig":
return Optional.ofNullable(clazz.cast(hostHeaderConfig()));
case "PathPatternConfig":
return Optional.ofNullable(clazz.cast(pathPatternConfig()));
case "HttpHeaderConfig":
return Optional.ofNullable(clazz.cast(httpHeaderConfig()));
case "QueryStringConfig":
return Optional.ofNullable(clazz.cast(queryStringConfig()));
case "HttpRequestMethodConfig":
return Optional.ofNullable(clazz.cast(httpRequestMethodConfig()));
case "SourceIpConfig":
return Optional.ofNullable(clazz.cast(sourceIpConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function