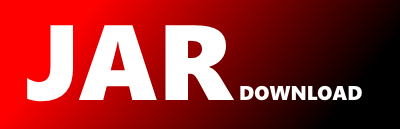
software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyTargetGroupRequest extends ElasticLoadBalancingV2Request implements
ToCopyableBuilder {
private static final SdkField TARGET_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetGroupArn").getter(getter(ModifyTargetGroupRequest::targetGroupArn))
.setter(setter(Builder::targetGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetGroupArn").build()).build();
private static final SdkField HEALTH_CHECK_PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckProtocol").getter(getter(ModifyTargetGroupRequest::healthCheckProtocolAsString))
.setter(setter(Builder::healthCheckProtocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckProtocol").build())
.build();
private static final SdkField HEALTH_CHECK_PORT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPort").getter(getter(ModifyTargetGroupRequest::healthCheckPort))
.setter(setter(Builder::healthCheckPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPort").build()).build();
private static final SdkField HEALTH_CHECK_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPath").getter(getter(ModifyTargetGroupRequest::healthCheckPath))
.setter(setter(Builder::healthCheckPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPath").build()).build();
private static final SdkField HEALTH_CHECK_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HealthCheckEnabled").getter(getter(ModifyTargetGroupRequest::healthCheckEnabled))
.setter(setter(Builder::healthCheckEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckEnabled").build())
.build();
private static final SdkField HEALTH_CHECK_INTERVAL_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("HealthCheckIntervalSeconds")
.getter(getter(ModifyTargetGroupRequest::healthCheckIntervalSeconds))
.setter(setter(Builder::healthCheckIntervalSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckIntervalSeconds").build())
.build();
private static final SdkField HEALTH_CHECK_TIMEOUT_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("HealthCheckTimeoutSeconds")
.getter(getter(ModifyTargetGroupRequest::healthCheckTimeoutSeconds))
.setter(setter(Builder::healthCheckTimeoutSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckTimeoutSeconds").build())
.build();
private static final SdkField HEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("HealthyThresholdCount").getter(getter(ModifyTargetGroupRequest::healthyThresholdCount))
.setter(setter(Builder::healthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthyThresholdCount").build())
.build();
private static final SdkField UNHEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("UnhealthyThresholdCount").getter(getter(ModifyTargetGroupRequest::unhealthyThresholdCount))
.setter(setter(Builder::unhealthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnhealthyThresholdCount").build())
.build();
private static final SdkField MATCHER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Matcher").getter(getter(ModifyTargetGroupRequest::matcher)).setter(setter(Builder::matcher))
.constructor(Matcher::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Matcher").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TARGET_GROUP_ARN_FIELD,
HEALTH_CHECK_PROTOCOL_FIELD, HEALTH_CHECK_PORT_FIELD, HEALTH_CHECK_PATH_FIELD, HEALTH_CHECK_ENABLED_FIELD,
HEALTH_CHECK_INTERVAL_SECONDS_FIELD, HEALTH_CHECK_TIMEOUT_SECONDS_FIELD, HEALTHY_THRESHOLD_COUNT_FIELD,
UNHEALTHY_THRESHOLD_COUNT_FIELD, MATCHER_FIELD));
private final String targetGroupArn;
private final String healthCheckProtocol;
private final String healthCheckPort;
private final String healthCheckPath;
private final Boolean healthCheckEnabled;
private final Integer healthCheckIntervalSeconds;
private final Integer healthCheckTimeoutSeconds;
private final Integer healthyThresholdCount;
private final Integer unhealthyThresholdCount;
private final Matcher matcher;
private ModifyTargetGroupRequest(BuilderImpl builder) {
super(builder);
this.targetGroupArn = builder.targetGroupArn;
this.healthCheckProtocol = builder.healthCheckProtocol;
this.healthCheckPort = builder.healthCheckPort;
this.healthCheckPath = builder.healthCheckPath;
this.healthCheckEnabled = builder.healthCheckEnabled;
this.healthCheckIntervalSeconds = builder.healthCheckIntervalSeconds;
this.healthCheckTimeoutSeconds = builder.healthCheckTimeoutSeconds;
this.healthyThresholdCount = builder.healthyThresholdCount;
this.unhealthyThresholdCount = builder.unhealthyThresholdCount;
this.matcher = builder.matcher;
}
/**
*
* The Amazon Resource Name (ARN) of the target group.
*
*
* @return The Amazon Resource Name (ARN) of the target group.
*/
public final String targetGroupArn() {
return targetGroupArn;
}
/**
*
* The protocol the load balancer uses when performing health checks on targets. For Application Load Balancers, the
* default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is TCP. The TCP protocol is
* not supported for health checks if the protocol of the target group is HTTP or HTTPS. It is supported for health
* checks only if the protocol of the target group is TCP, TLS, UDP, or TCP_UDP. The GENEVE, TLS, UDP, and TCP_UDP
* protocols are not supported for health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol the load balancer uses when performing health checks on targets. For Application Load
* Balancers, the default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is
* TCP. The TCP protocol is not supported for health checks if the protocol of the target group is HTTP or
* HTTPS. It is supported for health checks only if the protocol of the target group is TCP, TLS, UDP, or
* TCP_UDP. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for health checks.
* @see ProtocolEnum
*/
public final ProtocolEnum healthCheckProtocol() {
return ProtocolEnum.fromValue(healthCheckProtocol);
}
/**
*
* The protocol the load balancer uses when performing health checks on targets. For Application Load Balancers, the
* default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is TCP. The TCP protocol is
* not supported for health checks if the protocol of the target group is HTTP or HTTPS. It is supported for health
* checks only if the protocol of the target group is TCP, TLS, UDP, or TCP_UDP. The GENEVE, TLS, UDP, and TCP_UDP
* protocols are not supported for health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol the load balancer uses when performing health checks on targets. For Application Load
* Balancers, the default is HTTP. For Network Load Balancers and Gateway Load Balancers, the default is
* TCP. The TCP protocol is not supported for health checks if the protocol of the target group is HTTP or
* HTTPS. It is supported for health checks only if the protocol of the target group is TCP, TLS, UDP, or
* TCP_UDP. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for health checks.
* @see ProtocolEnum
*/
public final String healthCheckProtocolAsString() {
return healthCheckProtocol;
}
/**
*
* The port the load balancer uses when performing health checks on targets.
*
*
* @return The port the load balancer uses when performing health checks on targets.
*/
public final String healthCheckPort() {
return healthCheckPort;
}
/**
*
* [HTTP/HTTPS health checks] The destination for health checks on the targets.
*
*
* [HTTP1 or HTTP2 protocol version] The ping path. The default is /.
*
*
* [GRPC protocol version] The path of a custom health check method with the format /package.service/method. The
* default is /Amazon Web Services.ALB/healthcheck.
*
*
* @return [HTTP/HTTPS health checks] The destination for health checks on the targets.
*
* [HTTP1 or HTTP2 protocol version] The ping path. The default is /.
*
*
* [GRPC protocol version] The path of a custom health check method with the format /package.service/method.
* The default is /Amazon Web Services.ALB/healthcheck.
*/
public final String healthCheckPath() {
return healthCheckPath;
}
/**
*
* Indicates whether health checks are enabled.
*
*
* @return Indicates whether health checks are enabled.
*/
public final Boolean healthCheckEnabled() {
return healthCheckEnabled;
}
/**
*
* The approximate amount of time, in seconds, between health checks of an individual target. For TCP health checks,
* the supported values are 10 or 30 seconds.
*
*
* @return The approximate amount of time, in seconds, between health checks of an individual target. For TCP health
* checks, the supported values are 10 or 30 seconds.
*/
public final Integer healthCheckIntervalSeconds() {
return healthCheckIntervalSeconds;
}
/**
*
* [HTTP/HTTPS health checks] The amount of time, in seconds, during which no response means a failed health check.
*
*
* @return [HTTP/HTTPS health checks] The amount of time, in seconds, during which no response means a failed health
* check.
*/
public final Integer healthCheckTimeoutSeconds() {
return healthCheckTimeoutSeconds;
}
/**
*
* The number of consecutive health checks successes required before considering an unhealthy target healthy.
*
*
* @return The number of consecutive health checks successes required before considering an unhealthy target
* healthy.
*/
public final Integer healthyThresholdCount() {
return healthyThresholdCount;
}
/**
*
* The number of consecutive health check failures required before considering the target unhealthy. For target
* groups with a protocol of TCP or TLS, this value must be the same as the healthy threshold count.
*
*
* @return The number of consecutive health check failures required before considering the target unhealthy. For
* target groups with a protocol of TCP or TLS, this value must be the same as the healthy threshold count.
*/
public final Integer unhealthyThresholdCount() {
return unhealthyThresholdCount;
}
/**
*
* [HTTP/HTTPS health checks] The HTTP or gRPC codes to use when checking for a successful response from a target.
*
*
* @return [HTTP/HTTPS health checks] The HTTP or gRPC codes to use when checking for a successful response from a
* target.
*/
public final Matcher matcher() {
return matcher;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(targetGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckProtocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPort());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPath());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckEnabled());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckIntervalSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckTimeoutSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(unhealthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(matcher());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyTargetGroupRequest)) {
return false;
}
ModifyTargetGroupRequest other = (ModifyTargetGroupRequest) obj;
return Objects.equals(targetGroupArn(), other.targetGroupArn())
&& Objects.equals(healthCheckProtocolAsString(), other.healthCheckProtocolAsString())
&& Objects.equals(healthCheckPort(), other.healthCheckPort())
&& Objects.equals(healthCheckPath(), other.healthCheckPath())
&& Objects.equals(healthCheckEnabled(), other.healthCheckEnabled())
&& Objects.equals(healthCheckIntervalSeconds(), other.healthCheckIntervalSeconds())
&& Objects.equals(healthCheckTimeoutSeconds(), other.healthCheckTimeoutSeconds())
&& Objects.equals(healthyThresholdCount(), other.healthyThresholdCount())
&& Objects.equals(unhealthyThresholdCount(), other.unhealthyThresholdCount())
&& Objects.equals(matcher(), other.matcher());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyTargetGroupRequest").add("TargetGroupArn", targetGroupArn())
.add("HealthCheckProtocol", healthCheckProtocolAsString()).add("HealthCheckPort", healthCheckPort())
.add("HealthCheckPath", healthCheckPath()).add("HealthCheckEnabled", healthCheckEnabled())
.add("HealthCheckIntervalSeconds", healthCheckIntervalSeconds())
.add("HealthCheckTimeoutSeconds", healthCheckTimeoutSeconds())
.add("HealthyThresholdCount", healthyThresholdCount()).add("UnhealthyThresholdCount", unhealthyThresholdCount())
.add("Matcher", matcher()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TargetGroupArn":
return Optional.ofNullable(clazz.cast(targetGroupArn()));
case "HealthCheckProtocol":
return Optional.ofNullable(clazz.cast(healthCheckProtocolAsString()));
case "HealthCheckPort":
return Optional.ofNullable(clazz.cast(healthCheckPort()));
case "HealthCheckPath":
return Optional.ofNullable(clazz.cast(healthCheckPath()));
case "HealthCheckEnabled":
return Optional.ofNullable(clazz.cast(healthCheckEnabled()));
case "HealthCheckIntervalSeconds":
return Optional.ofNullable(clazz.cast(healthCheckIntervalSeconds()));
case "HealthCheckTimeoutSeconds":
return Optional.ofNullable(clazz.cast(healthCheckTimeoutSeconds()));
case "HealthyThresholdCount":
return Optional.ofNullable(clazz.cast(healthyThresholdCount()));
case "UnhealthyThresholdCount":
return Optional.ofNullable(clazz.cast(unhealthyThresholdCount()));
case "Matcher":
return Optional.ofNullable(clazz.cast(matcher()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function