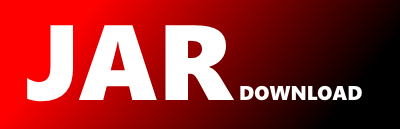
software.amazon.awssdk.services.elasticloadbalancingv2.DefaultElasticLoadBalancingV2AsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AllocationIdNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AlpnPolicyNotSupportedException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AvailabilityZoneNotSupportedException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CertificateNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateListenerException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateLoadBalancerNameException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateTagKeysException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DuplicateTargetGroupNameException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ElasticLoadBalancingV2Exception;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.HealthUnavailableException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.IncompatibleProtocolsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidConfigurationRequestException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidLoadBalancerActionException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSchemeException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSecurityGroupException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidSubnetException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.InvalidTargetException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ListenerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.LoadBalancerNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.OperationNotPermittedException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.PriorityInUseException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ResourceInUseException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RuleNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SslPolicyNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TargetGroupAssociationLimitException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TargetGroupNotFoundException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyActionsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyCertificatesException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyListenersException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyLoadBalancersException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyRegistrationsForTargetIdException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyRulesException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTagsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTargetGroupsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyTargetsException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.TooManyUniqueTargetGroupsPerLoadBalancerException;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.UnsupportedProtocolException;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.AddListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.CreateTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeleteTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DeregisterTargetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeAccountLimitsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeListenersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeLoadBalancersRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeRulesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeSslPoliciesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetGroupAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.DescribeTargetHealthRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyListenerRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyLoadBalancerAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyRuleRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyTargetGroupAttributesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.ModifyTargetGroupRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RegisterTargetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RemoveListenerCertificatesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetIpAddressTypeRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetRulePrioritiesRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetSecurityGroupsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.transform.SetSubnetsRequestMarshaller;
import software.amazon.awssdk.services.elasticloadbalancingv2.waiters.ElasticLoadBalancingV2AsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ElasticLoadBalancingV2AsyncClient}.
*
* @see ElasticLoadBalancingV2AsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultElasticLoadBalancingV2AsyncClient implements ElasticLoadBalancingV2AsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultElasticLoadBalancingV2AsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ElasticLoadBalancingV2ServiceClientConfiguration serviceClientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultElasticLoadBalancingV2AsyncClient(
ElasticLoadBalancingV2ServiceClientConfiguration serviceClientConfiguration,
SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.serviceClientConfiguration = serviceClientConfiguration;
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Adds the specified SSL server certificate to the certificate list for the specified HTTPS or TLS listener.
*
*
* If the certificate in already in the certificate list, the call is successful but the certificate is not added
* again.
*
*
* For more information, see HTTPS
* listeners in the Application Load Balancers Guide or TLS listeners
* in the Network Load Balancers Guide.
*
*
* @param addListenerCertificatesRequest
* @return A Java Future containing the result of the AddListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - CertificateNotFoundException The specified certificate does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddListenerCertificates
* @see AWS API Documentation
*/
@Override
public CompletableFuture addListenerCertificates(
AddListenerCertificatesRequest addListenerCertificatesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addListenerCertificatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddListenerCertificates");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddListenerCertificates")
.withMarshaller(new AddListenerCertificatesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(addListenerCertificatesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds the specified tags to the specified Elastic Load Balancing resource. You can tag your Application Load
* Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, and rules.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* @param addTagsRequest
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateTagKeysException A tag key was specified more than once.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddTags
* @see AWS API Documentation
*/
@Override
public CompletableFuture addTags(AddTagsRequest addTagsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTags");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("AddTags")
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(addTagsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a listener for the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* listeners with the same settings, each call succeeds.
*
*
* @param createListenerRequest
* @return A Java Future containing the result of the CreateListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateListener
* @see AWS API Documentation
*/
@Override
public CompletableFuture createListener(CreateListenerRequest createListenerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createListenerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateListener");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateListener")
.withMarshaller(new CreateListenerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createListenerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* load balancers with the same settings, each call succeeds.
*
*
* @param createLoadBalancerRequest
* @return A Java Future containing the result of the CreateLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateLoadBalancerNameException A load balancer with the specified name already exists.
* - TooManyLoadBalancersException You've reached the limit on the number of load balancers for your
* Amazon Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - InvalidSecurityGroupException The specified security group does not exist.
* - InvalidSchemeException The requested scheme is not valid.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - DuplicateTagKeysException A tag key was specified more than once.
* - ResourceInUseException A specified resource is in use.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateLoadBalancer
* @see AWS API Documentation
*/
@Override
public CompletableFuture createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoadBalancer");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLoadBalancer")
.withMarshaller(new CreateLoadBalancerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createLoadBalancerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a rule for the specified listener. The listener must be associated with an Application Load Balancer.
*
*
* Each rule consists of a priority, one or more actions, and one or more conditions. Rules are evaluated in
* priority order, from the lowest value to the highest value. When the conditions for a rule are met, its actions
* are performed. If the conditions for no rules are met, the actions for the default rule are performed. For more
* information, see Listener rules in the Application Load Balancers Guide.
*
*
* @param createRuleRequest
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - PriorityInUseException The specified priority is in use.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - TooManyRulesException You've reached the limit on the number of rules per load balancer.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateRule
* @see AWS API Documentation
*/
@Override
public CompletableFuture createRule(CreateRuleRequest createRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateRule")
.withMarshaller(new CreateRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a target group.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* target groups with the same settings, each call succeeds.
*
*
* @param createTargetGroupRequest
* @return A Java Future containing the result of the CreateTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateTargetGroupNameException A target group with the specified name already exists.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateTargetGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture createTargetGroup(CreateTargetGroupRequest createTargetGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTargetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTargetGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateTargetGroup")
.withMarshaller(new CreateTargetGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createTargetGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer to which it is attached.
*
*
* @param deleteListenerRequest
* @return A Java Future containing the result of the DeleteListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteListener
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteListener(DeleteListenerRequest deleteListenerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteListenerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteListener");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteListener")
.withMarshaller(new DeleteListenerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteListenerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer. Deleting a load
* balancer also deletes its listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* @return A Java Future containing the result of the DeleteLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteLoadBalancer
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoadBalancer");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLoadBalancer")
.withMarshaller(new DeleteLoadBalancerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteLoadBalancerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified rule.
*
*
* You can't delete the default rule.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteRule
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteRule(DeleteRuleRequest deleteRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRule")
.withMarshaller(new DeleteRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks. Deleting a target group does not affect its registered targets. For example, any EC2
* instances continue to run until you stop or terminate them.
*
*
* @param deleteTargetGroupRequest
* @return A Java Future containing the result of the DeleteTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteTargetGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteTargetGroup(DeleteTargetGroupRequest deleteTargetGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTargetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTargetGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTargetGroup")
.withMarshaller(new DeleteTargetGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteTargetGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* @param deregisterTargetsRequest
* @return A Java Future containing the result of the DeregisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeregisterTargets
* @see AWS API Documentation
*/
@Override
public CompletableFuture deregisterTargets(DeregisterTargetsRequest deregisterTargetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterTargets");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeregisterTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterTargets")
.withMarshaller(new DeregisterTargetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deregisterTargetsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your Amazon Web Services account.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param describeAccountLimitsRequest
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeAccountLimits
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeAccountLimits(
DescribeAccountLimitsRequest describeAccountLimitsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAccountLimitsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAccountLimits");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeAccountLimitsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAccountLimits")
.withMarshaller(new DescribeAccountLimitsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeAccountLimitsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the default certificate and the certificate list for the specified HTTPS or TLS listener.
*
*
* If the default certificate is also in the certificate list, it appears twice in the results (once with
* IsDefault
set to true and once with IsDefault
set to false).
*
*
* For more information, see SSL certificates in the Application Load Balancers Guide or Server certificates in the Network Load Balancers Guide.
*
*
* @param describeListenerCertificatesRequest
* @return A Java Future containing the result of the DescribeListenerCertificates operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerCertificates
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeListenerCertificates(
DescribeListenerCertificatesRequest describeListenerCertificatesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeListenerCertificatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeListenerCertificates");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeListenerCertificates")
.withMarshaller(new DescribeListenerCertificatesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeListenerCertificatesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer, Network Load
* Balancer, or Gateway Load Balancer. You must specify either a load balancer or one or more listeners.
*
*
* @param describeListenersRequest
* @return A Java Future containing the result of the DescribeListeners operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListeners
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeListeners(DescribeListenersRequest describeListenersRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeListenersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeListeners");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeListenersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeListeners")
.withMarshaller(new DescribeListenersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeListenersRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the attributes for the specified Application Load Balancer, Network Load Balancer, or Gateway Load
* Balancer.
*
*
* For more information, see the following:
*
*
* -
*
* Load balancer attributes in the Application Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Network Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeLoadBalancerAttributesRequest
* @return A Java Future containing the result of the DescribeLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeLoadBalancerAttributes(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeLoadBalancerAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancerAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancerAttributes")
.withMarshaller(new DescribeLoadBalancerAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeLoadBalancerAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* @param describeLoadBalancersRequest
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeLoadBalancers(
DescribeLoadBalancersRequest describeLoadBalancersRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLoadBalancersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBalancers");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeLoadBalancersResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBalancers")
.withMarshaller(new DescribeLoadBalancersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeLoadBalancersRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* @return A Java Future containing the result of the DescribeRules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeRules
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeRules(DescribeRulesRequest describeRulesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRulesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRules");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeRulesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRules")
.withMarshaller(new DescribeRulesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeRulesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security policies in the Application Load Balancers Guide or Security policies in the Network Load Balancers Guide.
*
*
* @param describeSslPoliciesRequest
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeSSLPolicies
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeSSLPolicies(
DescribeSslPoliciesRequest describeSslPoliciesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSslPoliciesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSSLPolicies");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeSslPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSSLPolicies")
.withMarshaller(new DescribeSslPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeSslPoliciesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the tags for the specified Elastic Load Balancing resources. You can describe the tags for one or more
* Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or rules.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTags
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeTags(DescribeTagsRequest describeTagsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTags");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTags").withMarshaller(new DescribeTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeTagsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the attributes for the specified target group.
*
*
* For more information, see the following:
*
*
* -
*
* Target group attributes in the Application Load Balancers Guide
*
*
* -
*
* Target group attributes in the Network Load Balancers Guide
*
*
* -
*
* Target group attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeTargetGroupAttributesRequest
* @return A Java Future containing the result of the DescribeTargetGroupAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroupAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeTargetGroupAttributes(
DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeTargetGroupAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTargetGroupAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetGroupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetGroupAttributes")
.withMarshaller(new DescribeTargetGroupAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeTargetGroupAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* @param describeTargetGroupsRequest
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeTargetGroups(
DescribeTargetGroupsRequest describeTargetGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTargetGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTargetGroups");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetGroups")
.withMarshaller(new DescribeTargetGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeTargetGroupsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* @return A Java Future containing the result of the DescribeTargetHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TargetGroupNotFoundException The specified target group does not exist.
* - HealthUnavailableException The health of the specified targets could not be retrieved due to an
* internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetHealth
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeTargetHealth(
DescribeTargetHealthRequest describeTargetHealthRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTargetHealthRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTargetHealth");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTargetHealthResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTargetHealth")
.withMarshaller(new DescribeTargetHealthRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeTargetHealthRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Replaces the specified properties of the specified listener. Any properties that you do not specify remain
* unchanged.
*
*
* Changing the protocol from HTTPS to HTTP, or from TLS to TCP, removes the security policy and default certificate
* properties. If you change the protocol from HTTP to HTTPS, or from TCP to TLS, you must add the security policy
* and default certificate properties.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyListenerRequest
* @return A Java Future containing the result of the ModifyListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyListener
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyListener(ModifyListenerRequest modifyListenerRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyListenerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyListener");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyListenerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyListener")
.withMarshaller(new ModifyListenerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyListenerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies the specified attributes of the specified Application Load Balancer, Network Load Balancer, or Gateway
* Load Balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* @return A Java Future containing the result of the ModifyLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyLoadBalancerAttributes(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyLoadBalancerAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyLoadBalancerAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyLoadBalancerAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyLoadBalancerAttributes")
.withMarshaller(new ModifyLoadBalancerAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyLoadBalancerAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Replaces the specified properties of the specified rule. Any properties that you do not specify are unchanged.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyRuleRequest
* @return A Java Future containing the result of the ModifyRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TargetGroupNotFoundException The specified target group does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyRule
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyRule(ModifyRuleRequest modifyRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ModifyRule")
.withMarshaller(new ModifyRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* @param modifyTargetGroupRequest
* @return A Java Future containing the result of the ModifyTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyTargetGroup(ModifyTargetGroupRequest modifyTargetGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyTargetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyTargetGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyTargetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyTargetGroup")
.withMarshaller(new ModifyTargetGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyTargetGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* @return A Java Future containing the result of the ModifyTargetGroupAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroupAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyTargetGroupAttributes(
ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyTargetGroupAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyTargetGroupAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ModifyTargetGroupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyTargetGroupAttributes")
.withMarshaller(new ModifyTargetGroupAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyTargetGroupAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Registers the specified targets with the specified target group.
*
*
* If the target is an EC2 instance, it must be in the running
state when you register it.
*
*
* By default, the load balancer routes requests to registered targets using the protocol and port for the target
* group. Alternatively, you can override the port for a target when you register it. You can register each EC2
* instance or IP address with the same target group multiple times using different ports.
*
*
* With a Network Load Balancer, you cannot register instances by instance ID if they have the following instance
* types: C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1. You can register instances of
* these types by IP address.
*
*
* @param registerTargetsRequest
* @return A Java Future containing the result of the RegisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RegisterTargets
* @see AWS API Documentation
*/
@Override
public CompletableFuture registerTargets(RegisterTargetsRequest registerTargetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterTargets");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RegisterTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RegisterTargets")
.withMarshaller(new RegisterTargetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(registerTargetsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified certificate from the certificate list for the specified HTTPS or TLS listener.
*
*
* @param removeListenerCertificatesRequest
* @return A Java Future containing the result of the RemoveListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ListenerNotFoundException The specified listener does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveListenerCertificates
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeListenerCertificates(
RemoveListenerCertificatesRequest removeListenerCertificatesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeListenerCertificatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveListenerCertificates");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveListenerCertificatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveListenerCertificates")
.withMarshaller(new RemoveListenerCertificatesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(removeListenerCertificatesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified tags from the specified Elastic Load Balancing resources. You can remove the tags for one
* or more Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or
* rules.
*
*
* @param removeTagsRequest
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TooManyTagsException You've reached the limit on the number of tags per load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveTags
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeTags(RemoveTagsRequest removeTagsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTags");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(RemoveTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("RemoveTags")
.withMarshaller(new RemoveTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(removeTagsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the type of IP addresses used by the subnets of the specified load balancer.
*
*
* @param setIpAddressTypeRequest
* @return A Java Future containing the result of the SetIpAddressType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetIpAddressType
* @see AWS API Documentation
*/
@Override
public CompletableFuture setIpAddressType(SetIpAddressTypeRequest setIpAddressTypeRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, setIpAddressTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetIpAddressType");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetIpAddressTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetIpAddressType")
.withMarshaller(new SetIpAddressTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(setIpAddressTypeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* @return A Java Future containing the result of the SetRulePriorities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - RuleNotFoundException The specified rule does not exist.
* - PriorityInUseException The specified priority is in use.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetRulePriorities
* @see AWS API Documentation
*/
@Override
public CompletableFuture setRulePriorities(SetRulePrioritiesRequest setRulePrioritiesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, setRulePrioritiesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetRulePriorities");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetRulePrioritiesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetRulePriorities")
.withMarshaller(new SetRulePrioritiesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(setRulePrioritiesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associates the specified security groups with the specified Application Load Balancer. The specified security
* groups override the previously associated security groups.
*
*
* You can't specify a security group for a Network Load Balancer or Gateway Load Balancer.
*
*
* @param setSecurityGroupsRequest
* @return A Java Future containing the result of the SetSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSecurityGroupException The specified security group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSecurityGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture setSecurityGroups(SetSecurityGroupsRequest setSecurityGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, setSecurityGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetSecurityGroups");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetSecurityGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetSecurityGroups")
.withMarshaller(new SetSecurityGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(setSecurityGroupsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables the Availability Zones for the specified public subnets for the specified Application Load Balancer or
* Network Load Balancer. The specified subnets replace the previously enabled subnets.
*
*
* When you specify subnets for a Network Load Balancer, you must include all subnets that were enabled previously,
* with their existing configurations, plus any additional subnets.
*
*
* @param setSubnetsRequest
* @return A Java Future containing the result of the SetSubnets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSubnets
* @see AWS API Documentation
*/
@Override
public CompletableFuture setSubnets(SetSubnetsRequest setSubnetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, setSubnetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Elastic Load Balancing v2");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetSubnets");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(SetSubnetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("SetSubnets")
.withMarshaller(new SetSubnetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(setSubnetsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public ElasticLoadBalancingV2AsyncWaiter waiter() {
return ElasticLoadBalancingV2AsyncWaiter.builder().client(this).scheduledExecutorService(executorService).build();
}
@Override
public final ElasticLoadBalancingV2ServiceClientConfiguration serviceClientConfiguration() {
return this.serviceClientConfiguration;
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private AwsQueryProtocolFactory init() {
return AwsQueryProtocolFactory
.builder()
.registerModeledException(
ExceptionMetadata.builder().errorCode("ALPNPolicyNotFound")
.exceptionBuilderSupplier(AlpnPolicyNotSupportedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedProtocol")
.exceptionBuilderSupplier(UnsupportedProtocolException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyUniqueTargetGroupsPerLoadBalancer")
.exceptionBuilderSupplier(TooManyUniqueTargetGroupsPerLoadBalancerException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AllocationIdNotFound")
.exceptionBuilderSupplier(AllocationIdNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceInUse")
.exceptionBuilderSupplier(ResourceInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateTargetGroupName")
.exceptionBuilderSupplier(DuplicateTargetGroupNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRules")
.exceptionBuilderSupplier(TooManyRulesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyCertificates")
.exceptionBuilderSupplier(TooManyCertificatesException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LoadBalancerNotFound")
.exceptionBuilderSupplier(LoadBalancerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTargetGroups")
.exceptionBuilderSupplier(TooManyTargetGroupsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyLoadBalancers")
.exceptionBuilderSupplier(TooManyLoadBalancersException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidConfigurationRequest")
.exceptionBuilderSupplier(InvalidConfigurationRequestException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TargetGroupAssociationLimit")
.exceptionBuilderSupplier(TargetGroupAssociationLimitException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSecurityGroup")
.exceptionBuilderSupplier(InvalidSecurityGroupException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("IncompatibleProtocols")
.exceptionBuilderSupplier(IncompatibleProtocolsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyActions")
.exceptionBuilderSupplier(TooManyActionsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyListeners")
.exceptionBuilderSupplier(TooManyListenersException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateLoadBalancerName")
.exceptionBuilderSupplier(DuplicateLoadBalancerNameException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotPermitted")
.exceptionBuilderSupplier(OperationNotPermittedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidLoadBalancerAction")
.exceptionBuilderSupplier(InvalidLoadBalancerActionException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("CertificateNotFound")
.exceptionBuilderSupplier(CertificateNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateTagKeys")
.exceptionBuilderSupplier(DuplicateTagKeysException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTags")
.exceptionBuilderSupplier(TooManyTagsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TargetGroupNotFound")
.exceptionBuilderSupplier(TargetGroupNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRegistrationsForTargetId")
.exceptionBuilderSupplier(TooManyRegistrationsForTargetIdException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ListenerNotFound")
.exceptionBuilderSupplier(ListenerNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PriorityInUse")
.exceptionBuilderSupplier(PriorityInUseException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidTarget")
.exceptionBuilderSupplier(InvalidTargetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetNotFound")
.exceptionBuilderSupplier(SubnetNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AvailabilityZoneNotSupported")
.exceptionBuilderSupplier(AvailabilityZoneNotSupportedException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RuleNotFound")
.exceptionBuilderSupplier(RuleNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SSLPolicyNotFound")
.exceptionBuilderSupplier(SslPolicyNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateListener")
.exceptionBuilderSupplier(DuplicateListenerException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidScheme")
.exceptionBuilderSupplier(InvalidSchemeException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("HealthUnavailable")
.exceptionBuilderSupplier(HealthUnavailableException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSubnet")
.exceptionBuilderSupplier(InvalidSubnetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyTargets")
.exceptionBuilderSupplier(TooManyTargetsException::builder).httpStatusCode(400).build())
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(ElasticLoadBalancingV2Exception::builder).build();
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
@Override
public void close() {
clientHandler.close();
}
}