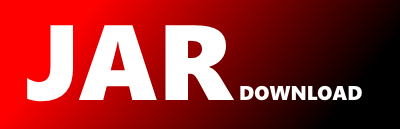
software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsRequest Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class SetSubnetsRequest extends ElasticLoadBalancingV2Request implements
ToCopyableBuilder {
private static final SdkField LOAD_BALANCER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LoadBalancerArn").getter(getter(SetSubnetsRequest::loadBalancerArn))
.setter(setter(Builder::loadBalancerArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadBalancerArn").build()).build();
private static final SdkField> SUBNETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Subnets")
.getter(getter(SetSubnetsRequest::subnets))
.setter(setter(Builder::subnets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Subnets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SUBNET_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SubnetMappings")
.getter(getter(SetSubnetsRequest::subnetMappings))
.setter(setter(Builder::subnetMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetMappings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SubnetMapping::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IP_ADDRESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpAddressType").getter(getter(SetSubnetsRequest::ipAddressTypeAsString))
.setter(setter(Builder::ipAddressType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpAddressType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOAD_BALANCER_ARN_FIELD,
SUBNETS_FIELD, SUBNET_MAPPINGS_FIELD, IP_ADDRESS_TYPE_FIELD));
private final String loadBalancerArn;
private final List subnets;
private final List subnetMappings;
private final String ipAddressType;
private SetSubnetsRequest(BuilderImpl builder) {
super(builder);
this.loadBalancerArn = builder.loadBalancerArn;
this.subnets = builder.subnets;
this.subnetMappings = builder.subnetMappings;
this.ipAddressType = builder.ipAddressType;
}
/**
*
* The Amazon Resource Name (ARN) of the load balancer.
*
*
* @return The Amazon Resource Name (ARN) of the load balancer.
*/
public final String loadBalancerArn() {
return loadBalancerArn;
}
/**
* For responses, this returns true if the service returned a value for the Subnets property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSubnets() {
return subnets != null && !(subnets instanceof SdkAutoConstructList);
}
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSubnets} method.
*
*
* @return The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*/
public final List subnets() {
return subnets;
}
/**
* For responses, this returns true if the service returned a value for the SubnetMappings property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSubnetMappings() {
return subnetMappings != null && !(subnetMappings instanceof SdkAutoConstructList);
}
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot specify
* Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one Elastic
* IP address per subnet if you need static IP addresses for your internet-facing load balancer. For internal load
* balancers, you can specify one private IP address per subnet from the IPv4 range of the subnet. For
* internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSubnetMappings} method.
*
*
* @return The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one
* Elastic IP address per subnet if you need static IP addresses for your internet-facing load balancer. For
* internal load balancers, you can specify one private IP address per subnet from the IPv4 range of the
* subnet. For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*/
public final List subnetMappings() {
return subnetMappings;
}
/**
*
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible values
* are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses). You can’t
* specify dualstack
for a load balancer with a UDP or TCP_UDP listener.
*
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible values
* are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link IpAddressType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipAddressTypeAsString}.
*
*
* @return [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses). You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP
* listener.
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses).
* @see IpAddressType
*/
public final IpAddressType ipAddressType() {
return IpAddressType.fromValue(ipAddressType);
}
/**
*
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible values
* are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses). You can’t
* specify dualstack
for a load balancer with a UDP or TCP_UDP listener.
*
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible values
* are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link IpAddressType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipAddressTypeAsString}.
*
*
* @return [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses). You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP
* listener.
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses).
* @see IpAddressType
*/
public final String ipAddressTypeAsString() {
return ipAddressType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(loadBalancerArn());
hashCode = 31 * hashCode + Objects.hashCode(hasSubnets() ? subnets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSubnetMappings() ? subnetMappings() : null);
hashCode = 31 * hashCode + Objects.hashCode(ipAddressTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SetSubnetsRequest)) {
return false;
}
SetSubnetsRequest other = (SetSubnetsRequest) obj;
return Objects.equals(loadBalancerArn(), other.loadBalancerArn()) && hasSubnets() == other.hasSubnets()
&& Objects.equals(subnets(), other.subnets()) && hasSubnetMappings() == other.hasSubnetMappings()
&& Objects.equals(subnetMappings(), other.subnetMappings())
&& Objects.equals(ipAddressTypeAsString(), other.ipAddressTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SetSubnetsRequest").add("LoadBalancerArn", loadBalancerArn())
.add("Subnets", hasSubnets() ? subnets() : null)
.add("SubnetMappings", hasSubnetMappings() ? subnetMappings() : null)
.add("IpAddressType", ipAddressTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LoadBalancerArn":
return Optional.ofNullable(clazz.cast(loadBalancerArn()));
case "Subnets":
return Optional.ofNullable(clazz.cast(subnets()));
case "SubnetMappings":
return Optional.ofNullable(clazz.cast(subnetMappings()));
case "IpAddressType":
return Optional.ofNullable(clazz.cast(ipAddressTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder subnets(Collection subnets);
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* @param subnets
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder subnets(String... subnets);
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one
* Elastic IP address per subnet if you need static IP addresses for your internet-facing load balancer. For
* internal load balancers, you can specify one private IP address per subnet from the IPv4 range of the subnet.
* For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* @param subnetMappings
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify
* one Elastic IP address per subnet if you need static IP addresses for your internet-facing load
* balancer. For internal load balancers, you can specify one private IP address per subnet from the IPv4
* range of the subnet. For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder subnetMappings(Collection subnetMappings);
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one
* Elastic IP address per subnet if you need static IP addresses for your internet-facing load balancer. For
* internal load balancers, you can specify one private IP address per subnet from the IPv4 range of the subnet.
* For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* @param subnetMappings
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify
* one Elastic IP address per subnet if you need static IP addresses for your internet-facing load
* balancer. For internal load balancers, you can specify one private IP address per subnet from the IPv4
* range of the subnet. For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder subnetMappings(SubnetMapping... subnetMappings);
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Application Load Balancers on Outposts] You must specify one Outpost subnet.
*
*
* [Application Load Balancers on Local Zones] You can specify subnets from one or more Local Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one
* Elastic IP address per subnet if you need static IP addresses for your internet-facing load balancer. For
* internal load balancers, you can specify one private IP address per subnet from the IPv4 range of the subnet.
* For internet-facing load balancer, you can specify one IPv6 address per subnet.
*
*
* [Gateway Load Balancers] You can specify subnets from one or more Availability Zones.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetMapping.Builder} avoiding the need
* to create one manually via
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetMapping#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetMapping.Builder#build()} is called
* immediately and its result is passed to {@link #subnetMappings(List)}.
*
* @param subnetMappings
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SubnetMapping.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #subnetMappings(java.util.Collection)
*/
Builder subnetMappings(Consumer... subnetMappings);
/**
*
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
* You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP listener.
*
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
*
*
* @param ipAddressType
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and
* IPv6 addresses). You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP
* listener.
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and
* IPv6 addresses).
* @see IpAddressType
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpAddressType
*/
Builder ipAddressType(String ipAddressType);
/**
*
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
* You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP listener.
*
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
*
*
* @param ipAddressType
* [Network Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and
* IPv6 addresses). You can’t specify dualstack
for a load balancer with a UDP or TCP_UDP
* listener.
*
* [Gateway Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and
* IPv6 addresses).
* @see IpAddressType
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpAddressType
*/
Builder ipAddressType(IpAddressType ipAddressType);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ElasticLoadBalancingV2Request.BuilderImpl implements Builder {
private String loadBalancerArn;
private List subnets = DefaultSdkAutoConstructList.getInstance();
private List subnetMappings = DefaultSdkAutoConstructList.getInstance();
private String ipAddressType;
private BuilderImpl() {
}
private BuilderImpl(SetSubnetsRequest model) {
super(model);
loadBalancerArn(model.loadBalancerArn);
subnets(model.subnets);
subnetMappings(model.subnetMappings);
ipAddressType(model.ipAddressType);
}
public final String getLoadBalancerArn() {
return loadBalancerArn;
}
public final void setLoadBalancerArn(String loadBalancerArn) {
this.loadBalancerArn = loadBalancerArn;
}
@Override
public final Builder loadBalancerArn(String loadBalancerArn) {
this.loadBalancerArn = loadBalancerArn;
return this;
}
public final Collection getSubnets() {
if (subnets instanceof SdkAutoConstructList) {
return null;
}
return subnets;
}
public final void setSubnets(Collection subnets) {
this.subnets = SubnetsCopier.copy(subnets);
}
@Override
public final Builder subnets(Collection subnets) {
this.subnets = SubnetsCopier.copy(subnets);
return this;
}
@Override
@SafeVarargs
public final Builder subnets(String... subnets) {
subnets(Arrays.asList(subnets));
return this;
}
public final List getSubnetMappings() {
List result = SubnetMappingsCopier.copyToBuilder(this.subnetMappings);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSubnetMappings(Collection subnetMappings) {
this.subnetMappings = SubnetMappingsCopier.copyFromBuilder(subnetMappings);
}
@Override
public final Builder subnetMappings(Collection subnetMappings) {
this.subnetMappings = SubnetMappingsCopier.copy(subnetMappings);
return this;
}
@Override
@SafeVarargs
public final Builder subnetMappings(SubnetMapping... subnetMappings) {
subnetMappings(Arrays.asList(subnetMappings));
return this;
}
@Override
@SafeVarargs
public final Builder subnetMappings(Consumer... subnetMappings) {
subnetMappings(Stream.of(subnetMappings).map(c -> SubnetMapping.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getIpAddressType() {
return ipAddressType;
}
public final void setIpAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
}
@Override
public final Builder ipAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
return this;
}
@Override
public final Builder ipAddressType(IpAddressType ipAddressType) {
this.ipAddressType(ipAddressType == null ? null : ipAddressType.toString());
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public SetSubnetsRequest build() {
return new SetSubnetsRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}