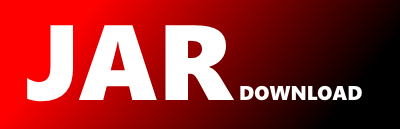
software.amazon.awssdk.services.elasticloadbalancingv2.model.TargetGroup Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a target group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TargetGroup implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TARGET_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetGroupArn").getter(getter(TargetGroup::targetGroupArn)).setter(setter(Builder::targetGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetGroupArn").build()).build();
private static final SdkField TARGET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetGroupName").getter(getter(TargetGroup::targetGroupName)).setter(setter(Builder::targetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetGroupName").build()).build();
private static final SdkField PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Protocol").getter(getter(TargetGroup::protocolAsString)).setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Protocol").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(TargetGroup::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(TargetGroup::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField HEALTH_CHECK_PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckProtocol").getter(getter(TargetGroup::healthCheckProtocolAsString))
.setter(setter(Builder::healthCheckProtocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckProtocol").build())
.build();
private static final SdkField HEALTH_CHECK_PORT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPort").getter(getter(TargetGroup::healthCheckPort)).setter(setter(Builder::healthCheckPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPort").build()).build();
private static final SdkField HEALTH_CHECK_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HealthCheckEnabled").getter(getter(TargetGroup::healthCheckEnabled))
.setter(setter(Builder::healthCheckEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckEnabled").build())
.build();
private static final SdkField HEALTH_CHECK_INTERVAL_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("HealthCheckIntervalSeconds")
.getter(getter(TargetGroup::healthCheckIntervalSeconds))
.setter(setter(Builder::healthCheckIntervalSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckIntervalSeconds").build())
.build();
private static final SdkField HEALTH_CHECK_TIMEOUT_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("HealthCheckTimeoutSeconds")
.getter(getter(TargetGroup::healthCheckTimeoutSeconds)).setter(setter(Builder::healthCheckTimeoutSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckTimeoutSeconds").build())
.build();
private static final SdkField HEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("HealthyThresholdCount").getter(getter(TargetGroup::healthyThresholdCount))
.setter(setter(Builder::healthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthyThresholdCount").build())
.build();
private static final SdkField UNHEALTHY_THRESHOLD_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("UnhealthyThresholdCount").getter(getter(TargetGroup::unhealthyThresholdCount))
.setter(setter(Builder::unhealthyThresholdCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnhealthyThresholdCount").build())
.build();
private static final SdkField HEALTH_CHECK_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HealthCheckPath").getter(getter(TargetGroup::healthCheckPath)).setter(setter(Builder::healthCheckPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckPath").build()).build();
private static final SdkField MATCHER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Matcher").getter(getter(TargetGroup::matcher)).setter(setter(Builder::matcher))
.constructor(Matcher::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Matcher").build()).build();
private static final SdkField> LOAD_BALANCER_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LoadBalancerArns")
.getter(getter(TargetGroup::loadBalancerArns))
.setter(setter(Builder::loadBalancerArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadBalancerArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TARGET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetType").getter(getter(TargetGroup::targetTypeAsString)).setter(setter(Builder::targetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetType").build()).build();
private static final SdkField PROTOCOL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProtocolVersion").getter(getter(TargetGroup::protocolVersion)).setter(setter(Builder::protocolVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProtocolVersion").build()).build();
private static final SdkField IP_ADDRESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpAddressType").getter(getter(TargetGroup::ipAddressTypeAsString))
.setter(setter(Builder::ipAddressType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpAddressType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TARGET_GROUP_ARN_FIELD,
TARGET_GROUP_NAME_FIELD, PROTOCOL_FIELD, PORT_FIELD, VPC_ID_FIELD, HEALTH_CHECK_PROTOCOL_FIELD,
HEALTH_CHECK_PORT_FIELD, HEALTH_CHECK_ENABLED_FIELD, HEALTH_CHECK_INTERVAL_SECONDS_FIELD,
HEALTH_CHECK_TIMEOUT_SECONDS_FIELD, HEALTHY_THRESHOLD_COUNT_FIELD, UNHEALTHY_THRESHOLD_COUNT_FIELD,
HEALTH_CHECK_PATH_FIELD, MATCHER_FIELD, LOAD_BALANCER_ARNS_FIELD, TARGET_TYPE_FIELD, PROTOCOL_VERSION_FIELD,
IP_ADDRESS_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String targetGroupArn;
private final String targetGroupName;
private final String protocol;
private final Integer port;
private final String vpcId;
private final String healthCheckProtocol;
private final String healthCheckPort;
private final Boolean healthCheckEnabled;
private final Integer healthCheckIntervalSeconds;
private final Integer healthCheckTimeoutSeconds;
private final Integer healthyThresholdCount;
private final Integer unhealthyThresholdCount;
private final String healthCheckPath;
private final Matcher matcher;
private final List loadBalancerArns;
private final String targetType;
private final String protocolVersion;
private final String ipAddressType;
private TargetGroup(BuilderImpl builder) {
this.targetGroupArn = builder.targetGroupArn;
this.targetGroupName = builder.targetGroupName;
this.protocol = builder.protocol;
this.port = builder.port;
this.vpcId = builder.vpcId;
this.healthCheckProtocol = builder.healthCheckProtocol;
this.healthCheckPort = builder.healthCheckPort;
this.healthCheckEnabled = builder.healthCheckEnabled;
this.healthCheckIntervalSeconds = builder.healthCheckIntervalSeconds;
this.healthCheckTimeoutSeconds = builder.healthCheckTimeoutSeconds;
this.healthyThresholdCount = builder.healthyThresholdCount;
this.unhealthyThresholdCount = builder.unhealthyThresholdCount;
this.healthCheckPath = builder.healthCheckPath;
this.matcher = builder.matcher;
this.loadBalancerArns = builder.loadBalancerArns;
this.targetType = builder.targetType;
this.protocolVersion = builder.protocolVersion;
this.ipAddressType = builder.ipAddressType;
}
/**
*
* The Amazon Resource Name (ARN) of the target group.
*
*
* @return The Amazon Resource Name (ARN) of the target group.
*/
public final String targetGroupArn() {
return targetGroupArn;
}
/**
*
* The name of the target group.
*
*
* @return The name of the target group.
*/
public final String targetGroupName() {
return targetGroupName;
}
/**
*
* The protocol to use for routing traffic to the targets.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol to use for routing traffic to the targets.
* @see ProtocolEnum
*/
public final ProtocolEnum protocol() {
return ProtocolEnum.fromValue(protocol);
}
/**
*
* The protocol to use for routing traffic to the targets.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol to use for routing traffic to the targets.
* @see ProtocolEnum
*/
public final String protocolAsString() {
return protocol;
}
/**
*
* The port on which the targets are listening. This parameter is not used if the target is a Lambda function.
*
*
* @return The port on which the targets are listening. This parameter is not used if the target is a Lambda
* function.
*/
public final Integer port() {
return port;
}
/**
*
* The ID of the VPC for the targets.
*
*
* @return The ID of the VPC for the targets.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The protocol to use to connect with the target. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for
* health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol to use to connect with the target. The GENEVE, TLS, UDP, and TCP_UDP protocols are not
* supported for health checks.
* @see ProtocolEnum
*/
public final ProtocolEnum healthCheckProtocol() {
return ProtocolEnum.fromValue(healthCheckProtocol);
}
/**
*
* The protocol to use to connect with the target. The GENEVE, TLS, UDP, and TCP_UDP protocols are not supported for
* health checks.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #healthCheckProtocol} will return {@link ProtocolEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #healthCheckProtocolAsString}.
*
*
* @return The protocol to use to connect with the target. The GENEVE, TLS, UDP, and TCP_UDP protocols are not
* supported for health checks.
* @see ProtocolEnum
*/
public final String healthCheckProtocolAsString() {
return healthCheckProtocol;
}
/**
*
* The port to use to connect with the target.
*
*
* @return The port to use to connect with the target.
*/
public final String healthCheckPort() {
return healthCheckPort;
}
/**
*
* Indicates whether health checks are enabled.
*
*
* @return Indicates whether health checks are enabled.
*/
public final Boolean healthCheckEnabled() {
return healthCheckEnabled;
}
/**
*
* The approximate amount of time, in seconds, between health checks of an individual target.
*
*
* @return The approximate amount of time, in seconds, between health checks of an individual target.
*/
public final Integer healthCheckIntervalSeconds() {
return healthCheckIntervalSeconds;
}
/**
*
* The amount of time, in seconds, during which no response means a failed health check.
*
*
* @return The amount of time, in seconds, during which no response means a failed health check.
*/
public final Integer healthCheckTimeoutSeconds() {
return healthCheckTimeoutSeconds;
}
/**
*
* The number of consecutive health checks successes required before considering an unhealthy target healthy.
*
*
* @return The number of consecutive health checks successes required before considering an unhealthy target
* healthy.
*/
public final Integer healthyThresholdCount() {
return healthyThresholdCount;
}
/**
*
* The number of consecutive health check failures required before considering the target unhealthy.
*
*
* @return The number of consecutive health check failures required before considering the target unhealthy.
*/
public final Integer unhealthyThresholdCount() {
return unhealthyThresholdCount;
}
/**
*
* The destination for health checks on the targets.
*
*
* @return The destination for health checks on the targets.
*/
public final String healthCheckPath() {
return healthCheckPath;
}
/**
*
* The HTTP or gRPC codes to use when checking for a successful response from a target.
*
*
* @return The HTTP or gRPC codes to use when checking for a successful response from a target.
*/
public final Matcher matcher() {
return matcher;
}
/**
* For responses, this returns true if the service returned a value for the LoadBalancerArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLoadBalancerArns() {
return loadBalancerArns != null && !(loadBalancerArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Name (ARN) of the load balancer that routes traffic to this target group. You can use each
* target group with only one load balancer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLoadBalancerArns} method.
*
*
* @return The Amazon Resource Name (ARN) of the load balancer that routes traffic to this target group. You can use
* each target group with only one load balancer.
*/
public final List loadBalancerArns() {
return loadBalancerArns;
}
/**
*
* The type of target that you must specify when registering targets with this target group. The possible values are
* instance
(register targets by instance ID), ip
(register targets by IP address),
* lambda
(register a single Lambda function as a target), or alb
(register a single
* Application Load Balancer as a target).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of target that you must specify when registering targets with this target group. The possible
* values are instance
(register targets by instance ID), ip
(register targets by
* IP address), lambda
(register a single Lambda function as a target), or alb
* (register a single Application Load Balancer as a target).
* @see TargetTypeEnum
*/
public final TargetTypeEnum targetType() {
return TargetTypeEnum.fromValue(targetType);
}
/**
*
* The type of target that you must specify when registering targets with this target group. The possible values are
* instance
(register targets by instance ID), ip
(register targets by IP address),
* lambda
(register a single Lambda function as a target), or alb
(register a single
* Application Load Balancer as a target).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of target that you must specify when registering targets with this target group. The possible
* values are instance
(register targets by instance ID), ip
(register targets by
* IP address), lambda
(register a single Lambda function as a target), or alb
* (register a single Application Load Balancer as a target).
* @see TargetTypeEnum
*/
public final String targetTypeAsString() {
return targetType;
}
/**
*
* [HTTP/HTTPS protocol] The protocol version. The possible values are GRPC
, HTTP1
, and
* HTTP2
.
*
*
* @return [HTTP/HTTPS protocol] The protocol version. The possible values are GRPC
, HTTP1
* , and HTTP2
.
*/
public final String protocolVersion() {
return protocolVersion;
}
/**
*
* The type of IP address used for this target group. The possible values are ipv4
and
* ipv6
. This is an optional parameter. If not specified, the IP address type defaults to
* ipv4
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link TargetGroupIpAddressTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #ipAddressTypeAsString}.
*
*
* @return The type of IP address used for this target group. The possible values are ipv4
and
* ipv6
. This is an optional parameter. If not specified, the IP address type defaults to
* ipv4
.
* @see TargetGroupIpAddressTypeEnum
*/
public final TargetGroupIpAddressTypeEnum ipAddressType() {
return TargetGroupIpAddressTypeEnum.fromValue(ipAddressType);
}
/**
*
* The type of IP address used for this target group. The possible values are ipv4
and
* ipv6
. This is an optional parameter. If not specified, the IP address type defaults to
* ipv4
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link TargetGroupIpAddressTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #ipAddressTypeAsString}.
*
*
* @return The type of IP address used for this target group. The possible values are ipv4
and
* ipv6
. This is an optional parameter. If not specified, the IP address type defaults to
* ipv4
.
* @see TargetGroupIpAddressTypeEnum
*/
public final String ipAddressTypeAsString() {
return ipAddressType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(targetGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(targetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(protocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckProtocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPort());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckEnabled());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckIntervalSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckTimeoutSeconds());
hashCode = 31 * hashCode + Objects.hashCode(healthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(unhealthyThresholdCount());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckPath());
hashCode = 31 * hashCode + Objects.hashCode(matcher());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancerArns() ? loadBalancerArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(targetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(protocolVersion());
hashCode = 31 * hashCode + Objects.hashCode(ipAddressTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TargetGroup)) {
return false;
}
TargetGroup other = (TargetGroup) obj;
return Objects.equals(targetGroupArn(), other.targetGroupArn())
&& Objects.equals(targetGroupName(), other.targetGroupName())
&& Objects.equals(protocolAsString(), other.protocolAsString()) && Objects.equals(port(), other.port())
&& Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(healthCheckProtocolAsString(), other.healthCheckProtocolAsString())
&& Objects.equals(healthCheckPort(), other.healthCheckPort())
&& Objects.equals(healthCheckEnabled(), other.healthCheckEnabled())
&& Objects.equals(healthCheckIntervalSeconds(), other.healthCheckIntervalSeconds())
&& Objects.equals(healthCheckTimeoutSeconds(), other.healthCheckTimeoutSeconds())
&& Objects.equals(healthyThresholdCount(), other.healthyThresholdCount())
&& Objects.equals(unhealthyThresholdCount(), other.unhealthyThresholdCount())
&& Objects.equals(healthCheckPath(), other.healthCheckPath()) && Objects.equals(matcher(), other.matcher())
&& hasLoadBalancerArns() == other.hasLoadBalancerArns()
&& Objects.equals(loadBalancerArns(), other.loadBalancerArns())
&& Objects.equals(targetTypeAsString(), other.targetTypeAsString())
&& Objects.equals(protocolVersion(), other.protocolVersion())
&& Objects.equals(ipAddressTypeAsString(), other.ipAddressTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TargetGroup").add("TargetGroupArn", targetGroupArn()).add("TargetGroupName", targetGroupName())
.add("Protocol", protocolAsString()).add("Port", port()).add("VpcId", vpcId())
.add("HealthCheckProtocol", healthCheckProtocolAsString()).add("HealthCheckPort", healthCheckPort())
.add("HealthCheckEnabled", healthCheckEnabled()).add("HealthCheckIntervalSeconds", healthCheckIntervalSeconds())
.add("HealthCheckTimeoutSeconds", healthCheckTimeoutSeconds())
.add("HealthyThresholdCount", healthyThresholdCount()).add("UnhealthyThresholdCount", unhealthyThresholdCount())
.add("HealthCheckPath", healthCheckPath()).add("Matcher", matcher())
.add("LoadBalancerArns", hasLoadBalancerArns() ? loadBalancerArns() : null)
.add("TargetType", targetTypeAsString()).add("ProtocolVersion", protocolVersion())
.add("IpAddressType", ipAddressTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TargetGroupArn":
return Optional.ofNullable(clazz.cast(targetGroupArn()));
case "TargetGroupName":
return Optional.ofNullable(clazz.cast(targetGroupName()));
case "Protocol":
return Optional.ofNullable(clazz.cast(protocolAsString()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "HealthCheckProtocol":
return Optional.ofNullable(clazz.cast(healthCheckProtocolAsString()));
case "HealthCheckPort":
return Optional.ofNullable(clazz.cast(healthCheckPort()));
case "HealthCheckEnabled":
return Optional.ofNullable(clazz.cast(healthCheckEnabled()));
case "HealthCheckIntervalSeconds":
return Optional.ofNullable(clazz.cast(healthCheckIntervalSeconds()));
case "HealthCheckTimeoutSeconds":
return Optional.ofNullable(clazz.cast(healthCheckTimeoutSeconds()));
case "HealthyThresholdCount":
return Optional.ofNullable(clazz.cast(healthyThresholdCount()));
case "UnhealthyThresholdCount":
return Optional.ofNullable(clazz.cast(unhealthyThresholdCount()));
case "HealthCheckPath":
return Optional.ofNullable(clazz.cast(healthCheckPath()));
case "Matcher":
return Optional.ofNullable(clazz.cast(matcher()));
case "LoadBalancerArns":
return Optional.ofNullable(clazz.cast(loadBalancerArns()));
case "TargetType":
return Optional.ofNullable(clazz.cast(targetTypeAsString()));
case "ProtocolVersion":
return Optional.ofNullable(clazz.cast(protocolVersion()));
case "IpAddressType":
return Optional.ofNullable(clazz.cast(ipAddressTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function