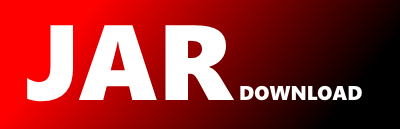
software.amazon.awssdk.services.elasticloadbalancingv2.ElasticLoadBalancingV2AsyncClient Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTrustStoreRevocationsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTrustStoreRevocationsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTrustStoreRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTrustStoreResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteSharedTrustStoreAssociationRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteSharedTrustStoreAssociationResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTrustStoreRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTrustStoreResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeCapacityReservationRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeCapacityReservationResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreCaCertificatesBundleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreCaCertificatesBundleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreRevocationContentRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreRevocationContentResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyCapacityReservationRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyCapacityReservationResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTrustStoreRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTrustStoreResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTrustStoreRevocationsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTrustStoreRevocationsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsRequest;
import software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsResponse;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenerCertificatesPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeRulesPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreAssociationsPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreRevocationsPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoresPublisher;
import software.amazon.awssdk.services.elasticloadbalancingv2.waiters.ElasticLoadBalancingV2AsyncWaiter;
/**
* Service client for accessing Elastic Load Balancing v2 asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
* Elastic Load Balancing
*
* A load balancer distributes incoming traffic across targets, such as your EC2 instances. This enables you to increase
* the availability of your application. The load balancer also monitors the health of its registered targets and
* ensures that it routes traffic only to healthy targets. You configure your load balancer to accept incoming traffic
* by specifying one or more listeners, which are configured with a protocol and port number for connections from
* clients to the load balancer. You configure a target group with a protocol and port number for connections from the
* load balancer to the targets, and with health check settings to be used when checking the health status of the
* targets.
*
*
* Elastic Load Balancing supports the following types of load balancers: Application Load Balancers, Network Load
* Balancers, Gateway Load Balancers, and Classic Load Balancers. This reference covers the following load balancer
* types:
*
*
* -
*
* Application Load Balancer - Operates at the application layer (layer 7) and supports HTTP and HTTPS.
*
*
* -
*
* Network Load Balancer - Operates at the transport layer (layer 4) and supports TCP, TLS, and UDP.
*
*
* -
*
* Gateway Load Balancer - Operates at the network layer (layer 3).
*
*
*
*
* For more information, see the Elastic
* Load Balancing User Guide.
*
*
* All Elastic Load Balancing operations are idempotent, which means that they complete at most one time. If you repeat
* an operation, it succeeds.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ElasticLoadBalancingV2AsyncClient extends AwsClient {
String SERVICE_NAME = "elasticloadbalancing";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "elasticloadbalancing";
/**
*
* Adds the specified SSL server certificate to the certificate list for the specified HTTPS or TLS listener.
*
*
* If the certificate in already in the certificate list, the call is successful but the certificate is not added
* again.
*
*
* For more information, see HTTPS
* listeners in the Application Load Balancers Guide or TLS listeners
* in the Network Load Balancers Guide.
*
*
* @param addListenerCertificatesRequest
* @return A Java Future containing the result of the AddListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - CertificateNotFoundException The specified certificate does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture addListenerCertificates(
AddListenerCertificatesRequest addListenerCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified SSL server certificate to the certificate list for the specified HTTPS or TLS listener.
*
*
* If the certificate in already in the certificate list, the call is successful but the certificate is not added
* again.
*
*
* For more information, see HTTPS
* listeners in the Application Load Balancers Guide or TLS listeners
* in the Network Load Balancers Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AddListenerCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link AddListenerCertificatesRequest#builder()}
*
*
* @param addListenerCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.AddListenerCertificatesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the AddListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - CertificateNotFoundException The specified certificate does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture addListenerCertificates(
Consumer addListenerCertificatesRequest) {
return addListenerCertificates(AddListenerCertificatesRequest.builder().applyMutation(addListenerCertificatesRequest)
.build());
}
/**
*
* Adds the specified tags to the specified Elastic Load Balancing resource. You can tag your Application Load
* Balancers, Network Load Balancers, Gateway Load Balancers, target groups, trust stores, listeners, and rules.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
* @param addTagsRequest
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTagKeysException A tag key was specified more than once.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddTags
* @see AWS API Documentation
*/
default CompletableFuture addTags(AddTagsRequest addTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified tags to the specified Elastic Load Balancing resource. You can tag your Application Load
* Balancers, Network Load Balancers, Gateway Load Balancers, target groups, trust stores, listeners, and rules.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key,
* AddTags
updates its value.
*
*
*
* This is a convenience which creates an instance of the {@link AddTagsRequest.Builder} avoiding the need to create
* one manually via {@link AddTagsRequest#builder()}
*
*
* @param addTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTagsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTagKeysException A tag key was specified more than once.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddTags
* @see AWS API Documentation
*/
default CompletableFuture addTags(Consumer addTagsRequest) {
return addTags(AddTagsRequest.builder().applyMutation(addTagsRequest).build());
}
/**
*
* Adds the specified revocation file to the specified trust store.
*
*
* @param addTrustStoreRevocationsRequest
* @return A Java Future containing the result of the AddTrustStoreRevocations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - InvalidRevocationContentException The provided revocation file is an invalid format, or uses an
* incorrect algorithm.
* - TooManyTrustStoreRevocationEntriesException The specified trust store has too many revocation
* entries.
* - RevocationContentNotFoundException The specified revocation file does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture addTrustStoreRevocations(
AddTrustStoreRevocationsRequest addTrustStoreRevocationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified revocation file to the specified trust store.
*
*
*
* This is a convenience which creates an instance of the {@link AddTrustStoreRevocationsRequest.Builder} avoiding
* the need to create one manually via {@link AddTrustStoreRevocationsRequest#builder()}
*
*
* @param addTrustStoreRevocationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.AddTrustStoreRevocationsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the AddTrustStoreRevocations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - InvalidRevocationContentException The provided revocation file is an invalid format, or uses an
* incorrect algorithm.
* - TooManyTrustStoreRevocationEntriesException The specified trust store has too many revocation
* entries.
* - RevocationContentNotFoundException The specified revocation file does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.AddTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture addTrustStoreRevocations(
Consumer addTrustStoreRevocationsRequest) {
return addTrustStoreRevocations(AddTrustStoreRevocationsRequest.builder().applyMutation(addTrustStoreRevocationsRequest)
.build());
}
/**
*
* Creates a listener for the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* listeners with the same settings, each call succeeds.
*
*
* @param createListenerRequest
* @return A Java Future containing the result of the CreateListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreNotReadyException The specified trust store is not active.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateListener
* @see AWS API Documentation
*/
default CompletableFuture createListener(CreateListenerRequest createListenerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a listener for the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* listeners with the same settings, each call succeeds.
*
*
*
* This is a convenience which creates an instance of the {@link CreateListenerRequest.Builder} avoiding the need to
* create one manually via {@link CreateListenerRequest#builder()}
*
*
* @param createListenerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateListenerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreNotReadyException The specified trust store is not active.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateListener
* @see AWS API Documentation
*/
default CompletableFuture createListener(Consumer createListenerRequest) {
return createListener(CreateListenerRequest.builder().applyMutation(createListenerRequest).build());
}
/**
*
* Creates an Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* load balancers with the same settings, each call succeeds.
*
*
* @param createLoadBalancerRequest
* @return A Java Future containing the result of the CreateLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateLoadBalancerNameException A load balancer with the specified name already exists.
* - TooManyLoadBalancersException You've reached the limit on the number of load balancers for your
* Amazon Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - InvalidSecurityGroupException The specified security group does not exist.
* - InvalidSchemeException The requested scheme is not valid.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - DuplicateTagKeysException A tag key was specified more than once.
* - ResourceInUseException A specified resource is in use.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateLoadBalancer
* @see AWS API Documentation
*/
default CompletableFuture createLoadBalancer(CreateLoadBalancerRequest createLoadBalancerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Application Load Balancer, Network Load Balancer, or Gateway Load Balancer.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* load balancers with the same settings, each call succeeds.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLoadBalancerRequest.Builder} avoiding the
* need to create one manually via {@link CreateLoadBalancerRequest#builder()}
*
*
* @param createLoadBalancerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateLoadBalancerNameException A load balancer with the specified name already exists.
* - TooManyLoadBalancersException You've reached the limit on the number of load balancers for your
* Amazon Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - InvalidSecurityGroupException The specified security group does not exist.
* - InvalidSchemeException The requested scheme is not valid.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - DuplicateTagKeysException A tag key was specified more than once.
* - ResourceInUseException A specified resource is in use.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateLoadBalancer
* @see AWS API Documentation
*/
default CompletableFuture createLoadBalancer(
Consumer createLoadBalancerRequest) {
return createLoadBalancer(CreateLoadBalancerRequest.builder().applyMutation(createLoadBalancerRequest).build());
}
/**
*
* Creates a rule for the specified listener. The listener must be associated with an Application Load Balancer.
*
*
* Each rule consists of a priority, one or more actions, and one or more conditions. Rules are evaluated in
* priority order, from the lowest value to the highest value. When the conditions for a rule are met, its actions
* are performed. If the conditions for no rules are met, the actions for the default rule are performed. For more
* information, see Listener rules in the Application Load Balancers Guide.
*
*
* @param createRuleRequest
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - PriorityInUseException The specified priority is in use.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - TooManyRulesException You've reached the limit on the number of rules per load balancer.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateRule
* @see AWS API Documentation
*/
default CompletableFuture createRule(CreateRuleRequest createRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a rule for the specified listener. The listener must be associated with an Application Load Balancer.
*
*
* Each rule consists of a priority, one or more actions, and one or more conditions. Rules are evaluated in
* priority order, from the lowest value to the highest value. When the conditions for a rule are met, its actions
* are performed. If the conditions for no rules are met, the actions for the default rule are performed. For more
* information, see Listener rules in the Application Load Balancers Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRuleRequest.Builder} avoiding the need to
* create one manually via {@link CreateRuleRequest#builder()}
*
*
* @param createRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateRuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - PriorityInUseException The specified priority is in use.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - TooManyRulesException You've reached the limit on the number of rules per load balancer.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateRule
* @see AWS API Documentation
*/
default CompletableFuture createRule(Consumer createRuleRequest) {
return createRule(CreateRuleRequest.builder().applyMutation(createRuleRequest).build());
}
/**
*
* Creates a target group.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* target groups with the same settings, each call succeeds.
*
*
* @param createTargetGroupRequest
* @return A Java Future containing the result of the CreateTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTargetGroupNameException A target group with the specified name already exists.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture createTargetGroup(CreateTargetGroupRequest createTargetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a target group.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* This operation is idempotent, which means that it completes at most one time. If you attempt to create multiple
* target groups with the same settings, each call succeeds.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTargetGroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateTargetGroupRequest#builder()}
*
*
* @param createTargetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTargetGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTargetGroupNameException A target group with the specified name already exists.
* - TooManyTargetGroupsException You've reached the limit on the number of target groups for your Amazon
* Web Services account.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture createTargetGroup(
Consumer createTargetGroupRequest) {
return createTargetGroup(CreateTargetGroupRequest.builder().applyMutation(createTargetGroupRequest).build());
}
/**
*
* Creates a trust store.
*
*
* @param createTrustStoreRequest
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTrustStoreNameException A trust store with the specified name already exists.
* - TooManyTrustStoresException You've reached the limit on the number of trust stores for your Amazon
* Web Services account.
* - InvalidCaCertificatesBundleException The specified ca certificate bundle is in an invalid format, or
* corrupt.
* - CaCertificatesBundleNotFoundException The specified ca certificate bundle does not exist.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - DuplicateTagKeysException A tag key was specified more than once.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture createTrustStore(CreateTrustStoreRequest createTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a trust store.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link CreateTrustStoreRequest#builder()}
*
*
* @param createTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateTrustStoreRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateTrustStoreNameException A trust store with the specified name already exists.
* - TooManyTrustStoresException You've reached the limit on the number of trust stores for your Amazon
* Web Services account.
* - InvalidCaCertificatesBundleException The specified ca certificate bundle is in an invalid format, or
* corrupt.
* - CaCertificatesBundleNotFoundException The specified ca certificate bundle does not exist.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - DuplicateTagKeysException A tag key was specified more than once.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.CreateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture createTrustStore(
Consumer createTrustStoreRequest) {
return createTrustStore(CreateTrustStoreRequest.builder().applyMutation(createTrustStoreRequest).build());
}
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer to which it is attached.
*
*
* @param deleteListenerRequest
* @return A Java Future containing the result of the DeleteListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteListener
* @see AWS API Documentation
*/
default CompletableFuture deleteListener(DeleteListenerRequest deleteListenerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified listener.
*
*
* Alternatively, your listener is deleted when you delete the load balancer to which it is attached.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteListenerRequest.Builder} avoiding the need to
* create one manually via {@link DeleteListenerRequest#builder()}
*
*
* @param deleteListenerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteListenerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteListener
* @see AWS API Documentation
*/
default CompletableFuture deleteListener(Consumer deleteListenerRequest) {
return deleteListener(DeleteListenerRequest.builder().applyMutation(deleteListenerRequest).build());
}
/**
*
* Deletes the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer. Deleting a load
* balancer also deletes its listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
* @param deleteLoadBalancerRequest
* @return A Java Future containing the result of the DeleteLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteLoadBalancer
* @see AWS API Documentation
*/
default CompletableFuture deleteLoadBalancer(DeleteLoadBalancerRequest deleteLoadBalancerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified Application Load Balancer, Network Load Balancer, or Gateway Load Balancer. Deleting a load
* balancer also deletes its listeners.
*
*
* You can't delete a load balancer if deletion protection is enabled. If the load balancer does not exist or has
* already been deleted, the call succeeds.
*
*
* Deleting a load balancer does not affect its registered targets. For example, your EC2 instances continue to run
* and are still registered to their target groups. If you no longer need these EC2 instances, you can stop or
* terminate them.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLoadBalancerRequest.Builder} avoiding the
* need to create one manually via {@link DeleteLoadBalancerRequest#builder()}
*
*
* @param deleteLoadBalancerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteLoadBalancerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteLoadBalancer
* @see AWS API Documentation
*/
default CompletableFuture deleteLoadBalancer(
Consumer deleteLoadBalancerRequest) {
return deleteLoadBalancer(DeleteLoadBalancerRequest.builder().applyMutation(deleteLoadBalancerRequest).build());
}
/**
*
* Deletes the specified rule.
*
*
* You can't delete the default rule.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteRule
* @see AWS API Documentation
*/
default CompletableFuture deleteRule(DeleteRuleRequest deleteRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified rule.
*
*
* You can't delete the default rule.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRuleRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRuleRequest#builder()}
*
*
* @param deleteRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteRuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteRule
* @see AWS API Documentation
*/
default CompletableFuture deleteRule(Consumer deleteRuleRequest) {
return deleteRule(DeleteRuleRequest.builder().applyMutation(deleteRuleRequest).build());
}
/**
*
* Deletes a shared trust store association.
*
*
* @param deleteSharedTrustStoreAssociationRequest
* @return A Java Future containing the result of the DeleteSharedTrustStoreAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - DeleteAssociationSameAccountException The specified association can't be within the same account.
* - TrustStoreAssociationNotFoundException The specified association does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteSharedTrustStoreAssociation
* @see AWS API Documentation
*/
default CompletableFuture deleteSharedTrustStoreAssociation(
DeleteSharedTrustStoreAssociationRequest deleteSharedTrustStoreAssociationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a shared trust store association.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSharedTrustStoreAssociationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteSharedTrustStoreAssociationRequest#builder()}
*
*
* @param deleteSharedTrustStoreAssociationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteSharedTrustStoreAssociationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DeleteSharedTrustStoreAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - DeleteAssociationSameAccountException The specified association can't be within the same account.
* - TrustStoreAssociationNotFoundException The specified association does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteSharedTrustStoreAssociation
* @see AWS API Documentation
*/
default CompletableFuture deleteSharedTrustStoreAssociation(
Consumer deleteSharedTrustStoreAssociationRequest) {
return deleteSharedTrustStoreAssociation(DeleteSharedTrustStoreAssociationRequest.builder()
.applyMutation(deleteSharedTrustStoreAssociationRequest).build());
}
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks. Deleting a target group does not affect its registered targets. For example, any EC2
* instances continue to run until you stop or terminate them.
*
*
* @param deleteTargetGroupRequest
* @return A Java Future containing the result of the DeleteTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteTargetGroup(DeleteTargetGroupRequest deleteTargetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified target group.
*
*
* You can delete a target group if it is not referenced by any actions. Deleting a target group also deletes any
* associated health checks. Deleting a target group does not affect its registered targets. For example, any EC2
* instances continue to run until you stop or terminate them.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTargetGroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteTargetGroupRequest#builder()}
*
*
* @param deleteTargetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTargetGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceInUseException A specified resource is in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteTargetGroup(
Consumer deleteTargetGroupRequest) {
return deleteTargetGroup(DeleteTargetGroupRequest.builder().applyMutation(deleteTargetGroupRequest).build());
}
/**
*
* Deletes a trust store.
*
*
* @param deleteTrustStoreRequest
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreInUseException The specified trust store is currently in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteTrustStore
* @see AWS API Documentation
*/
default CompletableFuture deleteTrustStore(DeleteTrustStoreRequest deleteTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a trust store.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link DeleteTrustStoreRequest#builder()}
*
*
* @param deleteTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeleteTrustStoreRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreInUseException The specified trust store is currently in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeleteTrustStore
* @see AWS API Documentation
*/
default CompletableFuture deleteTrustStore(
Consumer deleteTrustStoreRequest) {
return deleteTrustStore(DeleteTrustStoreRequest.builder().applyMutation(deleteTrustStoreRequest).build());
}
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* The load balancer stops sending requests to targets that are deregistering, but uses connection draining to
* ensure that in-flight traffic completes on the existing connections. This deregistration delay is configured by
* default but can be updated for each target group.
*
*
* For more information, see the following:
*
*
* -
*
* Deregistration delay in the Application Load Balancers User Guide
*
*
* -
*
* Deregistration delay in the Network Load Balancers User Guide
*
*
* -
*
*
* Deregistration delay in the Gateway Load Balancers User Guide
*
*
*
*
* Note: If the specified target does not exist, the action returns successfully.
*
*
* @param deregisterTargetsRequest
* @return A Java Future containing the result of the DeregisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeregisterTargets
* @see AWS API Documentation
*/
default CompletableFuture deregisterTargets(DeregisterTargetsRequest deregisterTargetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters the specified targets from the specified target group. After the targets are deregistered, they no
* longer receive traffic from the load balancer.
*
*
* The load balancer stops sending requests to targets that are deregistering, but uses connection draining to
* ensure that in-flight traffic completes on the existing connections. This deregistration delay is configured by
* default but can be updated for each target group.
*
*
* For more information, see the following:
*
*
* -
*
* Deregistration delay in the Application Load Balancers User Guide
*
*
* -
*
* Deregistration delay in the Network Load Balancers User Guide
*
*
* -
*
*
* Deregistration delay in the Gateway Load Balancers User Guide
*
*
*
*
* Note: If the specified target does not exist, the action returns successfully.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterTargetsRequest.Builder} avoiding the need
* to create one manually via {@link DeregisterTargetsRequest#builder()}
*
*
* @param deregisterTargetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DeregisterTargetsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeregisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DeregisterTargets
* @see AWS API Documentation
*/
default CompletableFuture deregisterTargets(
Consumer deregisterTargetsRequest) {
return deregisterTargets(DeregisterTargetsRequest.builder().applyMutation(deregisterTargetsRequest).build());
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your Amazon Web Services account.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param describeAccountLimitsRequest
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default CompletableFuture describeAccountLimits(
DescribeAccountLimitsRequest describeAccountLimitsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your Amazon Web Services account.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccountLimitsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAccountLimitsRequest#builder()}
*
*
* @param describeAccountLimitsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeAccountLimitsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default CompletableFuture describeAccountLimits(
Consumer describeAccountLimitsRequest) {
return describeAccountLimits(DescribeAccountLimitsRequest.builder().applyMutation(describeAccountLimitsRequest).build());
}
/**
*
* Describes the current Elastic Load Balancing resource limits for your Amazon Web Services account.
*
*
* For more information, see the following:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default CompletableFuture describeAccountLimits() {
return describeAccountLimits(DescribeAccountLimitsRequest.builder().build());
}
/**
*
* Describes the capacity reservation status for the specified load balancer.
*
*
* @param describeCapacityReservationRequest
* @return A Java Future containing the result of the DescribeCapacityReservation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeCapacityReservation
* @see AWS API Documentation
*/
default CompletableFuture describeCapacityReservation(
DescribeCapacityReservationRequest describeCapacityReservationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the capacity reservation status for the specified load balancer.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCapacityReservationRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCapacityReservationRequest#builder()}
*
*
* @param describeCapacityReservationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeCapacityReservationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeCapacityReservation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeCapacityReservation
* @see AWS API Documentation
*/
default CompletableFuture describeCapacityReservation(
Consumer describeCapacityReservationRequest) {
return describeCapacityReservation(DescribeCapacityReservationRequest.builder()
.applyMutation(describeCapacityReservationRequest).build());
}
/**
*
* Describes the attributes for the specified listener.
*
*
* @param describeListenerAttributesRequest
* @return A Java Future containing the result of the DescribeListenerAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeListenerAttributes(
DescribeListenerAttributesRequest describeListenerAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the attributes for the specified listener.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenerAttributesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeListenerAttributesRequest#builder()}
*
*
* @param describeListenerAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeListenerAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeListenerAttributes(
Consumer describeListenerAttributesRequest) {
return describeListenerAttributes(DescribeListenerAttributesRequest.builder()
.applyMutation(describeListenerAttributesRequest).build());
}
/**
*
* Describes the default certificate and the certificate list for the specified HTTPS or TLS listener.
*
*
* If the default certificate is also in the certificate list, it appears twice in the results (once with
* IsDefault
set to true and once with IsDefault
set to false).
*
*
* For more information, see SSL certificates in the Application Load Balancers Guide or Server certificates in the Network Load Balancers Guide.
*
*
* @param describeListenerCertificatesRequest
* @return A Java Future containing the result of the DescribeListenerCertificates operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture describeListenerCertificates(
DescribeListenerCertificatesRequest describeListenerCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the default certificate and the certificate list for the specified HTTPS or TLS listener.
*
*
* If the default certificate is also in the certificate list, it appears twice in the results (once with
* IsDefault
set to true and once with IsDefault
set to false).
*
*
* For more information, see SSL certificates in the Application Load Balancers Guide or Server certificates in the Network Load Balancers Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenerCertificatesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeListenerCertificatesRequest#builder()}
*
*
* @param describeListenerCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeListenerCertificates operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture describeListenerCertificates(
Consumer describeListenerCertificatesRequest) {
return describeListenerCertificates(DescribeListenerCertificatesRequest.builder()
.applyMutation(describeListenerCertificatesRequest).build());
}
/**
*
* This is a variant of
* {@link #describeListenerCertificates(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenerCertificatesPublisher publisher = client.describeListenerCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenerCertificatesPublisher publisher = client.describeListenerCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeListenerCertificates(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest)}
* operation.
*
*
* @param describeListenerCertificatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerCertificates
* @see AWS API Documentation
*/
default DescribeListenerCertificatesPublisher describeListenerCertificatesPaginator(
DescribeListenerCertificatesRequest describeListenerCertificatesRequest) {
return new DescribeListenerCertificatesPublisher(this, describeListenerCertificatesRequest);
}
/**
*
* This is a variant of
* {@link #describeListenerCertificates(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenerCertificatesPublisher publisher = client.describeListenerCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenerCertificatesPublisher publisher = client.describeListenerCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeListenerCertificates(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenerCertificatesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeListenerCertificatesRequest#builder()}
*
*
* @param describeListenerCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenerCertificatesRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListenerCertificates
* @see AWS API Documentation
*/
default DescribeListenerCertificatesPublisher describeListenerCertificatesPaginator(
Consumer describeListenerCertificatesRequest) {
return describeListenerCertificatesPaginator(DescribeListenerCertificatesRequest.builder()
.applyMutation(describeListenerCertificatesRequest).build());
}
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer, Network Load
* Balancer, or Gateway Load Balancer. You must specify either a load balancer or one or more listeners.
*
*
* @param describeListenersRequest
* @return A Java Future containing the result of the DescribeListeners operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListeners
* @see AWS API Documentation
*/
default CompletableFuture describeListeners(DescribeListenersRequest describeListenersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified listeners or the listeners for the specified Application Load Balancer, Network Load
* Balancer, or Gateway Load Balancer. You must specify either a load balancer or one or more listeners.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenersRequest.Builder} avoiding the need
* to create one manually via {@link DescribeListenersRequest#builder()}
*
*
* @param describeListenersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeListeners operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListeners
* @see AWS API Documentation
*/
default CompletableFuture describeListeners(
Consumer describeListenersRequest) {
return describeListeners(DescribeListenersRequest.builder().applyMutation(describeListenersRequest).build());
}
/**
*
* This is a variant of
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersPublisher publisher = client.describeListenersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersPublisher publisher = client.describeListenersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation.
*
*
* @param describeListenersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListeners
* @see AWS API Documentation
*/
default DescribeListenersPublisher describeListenersPaginator(DescribeListenersRequest describeListenersRequest) {
return new DescribeListenersPublisher(this, describeListenersRequest);
}
/**
*
* This is a variant of
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersPublisher publisher = client.describeListenersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeListenersPublisher publisher = client.describeListenersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeListeners(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeListenersRequest.Builder} avoiding the need
* to create one manually via {@link DescribeListenersRequest#builder()}
*
*
* @param describeListenersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeListenersRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeListeners
* @see AWS API Documentation
*/
default DescribeListenersPublisher describeListenersPaginator(
Consumer describeListenersRequest) {
return describeListenersPaginator(DescribeListenersRequest.builder().applyMutation(describeListenersRequest).build());
}
/**
*
* Describes the attributes for the specified Application Load Balancer, Network Load Balancer, or Gateway Load
* Balancer.
*
*
* For more information, see the following:
*
*
* -
*
* Load balancer attributes in the Application Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Network Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeLoadBalancerAttributesRequest
* @return A Java Future containing the result of the DescribeLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeLoadBalancerAttributes(
DescribeLoadBalancerAttributesRequest describeLoadBalancerAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the attributes for the specified Application Load Balancer, Network Load Balancer, or Gateway Load
* Balancer.
*
*
* For more information, see the following:
*
*
* -
*
* Load balancer attributes in the Application Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Network Load Balancers Guide
*
*
* -
*
* Load balancer attributes in the Gateway Load Balancers Guide
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLoadBalancerAttributesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeLoadBalancerAttributesRequest#builder()}
*
*
* @param describeLoadBalancerAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancerAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancerAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeLoadBalancerAttributes(
Consumer describeLoadBalancerAttributesRequest) {
return describeLoadBalancerAttributes(DescribeLoadBalancerAttributesRequest.builder()
.applyMutation(describeLoadBalancerAttributesRequest).build());
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* @param describeLoadBalancersRequest
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default CompletableFuture describeLoadBalancers(
DescribeLoadBalancersRequest describeLoadBalancersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLoadBalancersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeLoadBalancersRequest#builder()}
*
*
* @param describeLoadBalancersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default CompletableFuture describeLoadBalancers(
Consumer describeLoadBalancersRequest) {
return describeLoadBalancers(DescribeLoadBalancersRequest.builder().applyMutation(describeLoadBalancersRequest).build());
}
/**
*
* Describes the specified load balancers or all of your load balancers.
*
*
* @return A Java Future containing the result of the DescribeLoadBalancers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default CompletableFuture describeLoadBalancers() {
return describeLoadBalancers(DescribeLoadBalancersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default DescribeLoadBalancersPublisher describeLoadBalancersPaginator() {
return describeLoadBalancersPaginator(DescribeLoadBalancersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation.
*
*
* @param describeLoadBalancersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default DescribeLoadBalancersPublisher describeLoadBalancersPaginator(
DescribeLoadBalancersRequest describeLoadBalancersRequest) {
return new DescribeLoadBalancersPublisher(this, describeLoadBalancersRequest);
}
/**
*
* This is a variant of
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeLoadBalancersPublisher publisher = client.describeLoadBalancersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeLoadBalancers(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLoadBalancersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeLoadBalancersRequest#builder()}
*
*
* @param describeLoadBalancersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeLoadBalancersRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeLoadBalancers
* @see AWS API Documentation
*/
default DescribeLoadBalancersPublisher describeLoadBalancersPaginator(
Consumer describeLoadBalancersRequest) {
return describeLoadBalancersPaginator(DescribeLoadBalancersRequest.builder().applyMutation(describeLoadBalancersRequest)
.build());
}
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
* @param describeRulesRequest
* @return A Java Future containing the result of the DescribeRules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeRules
* @see AWS API Documentation
*/
default CompletableFuture describeRules(DescribeRulesRequest describeRulesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified rules or the rules for the specified listener. You must specify either a listener or one
* or more rules.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRulesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeRulesRequest#builder()}
*
*
* @param describeRulesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeRules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeRules
* @see AWS API Documentation
*/
default CompletableFuture describeRules(Consumer describeRulesRequest) {
return describeRules(DescribeRulesRequest.builder().applyMutation(describeRulesRequest).build());
}
/**
*
* This is a variant of
* {@link #describeRules(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeRulesPublisher publisher = client.describeRulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeRulesPublisher publisher = client.describeRulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRules(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest)}
* operation.
*
*
* @param describeRulesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeRules
* @see AWS API Documentation
*/
default DescribeRulesPublisher describeRulesPaginator(DescribeRulesRequest describeRulesRequest) {
return new DescribeRulesPublisher(this, describeRulesRequest);
}
/**
*
* This is a variant of
* {@link #describeRules(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeRulesPublisher publisher = client.describeRulesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeRulesPublisher publisher = client.describeRulesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeRules(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRulesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeRulesRequest#builder()}
*
*
* @param describeRulesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeRulesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeRules
* @see AWS API Documentation
*/
default DescribeRulesPublisher describeRulesPaginator(Consumer describeRulesRequest) {
return describeRulesPaginator(DescribeRulesRequest.builder().applyMutation(describeRulesRequest).build());
}
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security policies in the Application Load Balancers Guide or Security policies in the Network Load Balancers Guide.
*
*
* @param describeSslPoliciesRequest
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeSSLPolicies
* @see AWS API Documentation
*/
default CompletableFuture describeSSLPolicies(
DescribeSslPoliciesRequest describeSslPoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security policies in the Application Load Balancers Guide or Security policies in the Network Load Balancers Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSslPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeSslPoliciesRequest#builder()}
*
*
* @param describeSslPoliciesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeSslPoliciesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeSSLPolicies
* @see AWS API Documentation
*/
default CompletableFuture describeSSLPolicies(
Consumer describeSslPoliciesRequest) {
return describeSSLPolicies(DescribeSslPoliciesRequest.builder().applyMutation(describeSslPoliciesRequest).build());
}
/**
*
* Describes the specified policies or all policies used for SSL negotiation.
*
*
* For more information, see Security policies in the Application Load Balancers Guide or Security policies in the Network Load Balancers Guide.
*
*
* @return A Java Future containing the result of the DescribeSSLPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeSSLPolicies
* @see AWS API Documentation
*/
default CompletableFuture describeSSLPolicies() {
return describeSSLPolicies(DescribeSslPoliciesRequest.builder().build());
}
/**
*
* Describes the tags for the specified Elastic Load Balancing resources. You can describe the tags for one or more
* Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or rules.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTags
* @see AWS API Documentation
*/
default CompletableFuture describeTags(DescribeTagsRequest describeTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the tags for the specified Elastic Load Balancing resources. You can describe the tags for one or more
* Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or rules.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTagsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTagsRequest#builder()}
*
*
* @param describeTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTagsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTags
* @see AWS API Documentation
*/
default CompletableFuture describeTags(Consumer describeTagsRequest) {
return describeTags(DescribeTagsRequest.builder().applyMutation(describeTagsRequest).build());
}
/**
*
* Describes the attributes for the specified target group.
*
*
* For more information, see the following:
*
*
* -
*
* Target group attributes in the Application Load Balancers Guide
*
*
* -
*
* Target group attributes in the Network Load Balancers Guide
*
*
* -
*
* Target group attributes in the Gateway Load Balancers Guide
*
*
*
*
* @param describeTargetGroupAttributesRequest
* @return A Java Future containing the result of the DescribeTargetGroupAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroupAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeTargetGroupAttributes(
DescribeTargetGroupAttributesRequest describeTargetGroupAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the attributes for the specified target group.
*
*
* For more information, see the following:
*
*
* -
*
* Target group attributes in the Application Load Balancers Guide
*
*
* -
*
* Target group attributes in the Network Load Balancers Guide
*
*
* -
*
* Target group attributes in the Gateway Load Balancers Guide
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTargetGroupAttributesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTargetGroupAttributesRequest#builder()}
*
*
* @param describeTargetGroupAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeTargetGroupAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroupAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeTargetGroupAttributes(
Consumer describeTargetGroupAttributesRequest) {
return describeTargetGroupAttributes(DescribeTargetGroupAttributesRequest.builder()
.applyMutation(describeTargetGroupAttributesRequest).build());
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* @param describeTargetGroupsRequest
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeTargetGroups(
DescribeTargetGroupsRequest describeTargetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTargetGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTargetGroupsRequest#builder()}
*
*
* @param describeTargetGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeTargetGroups(
Consumer describeTargetGroupsRequest) {
return describeTargetGroups(DescribeTargetGroupsRequest.builder().applyMutation(describeTargetGroupsRequest).build());
}
/**
*
* Describes the specified target groups or all of your target groups. By default, all target groups are described.
* Alternatively, you can specify one of the following to filter the results: the ARN of the load balancer, the
* names of one or more target groups, or the ARNs of one or more target groups.
*
*
* @return A Java Future containing the result of the DescribeTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeTargetGroups() {
return describeTargetGroups(DescribeTargetGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default DescribeTargetGroupsPublisher describeTargetGroupsPaginator() {
return describeTargetGroupsPaginator(DescribeTargetGroupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation.
*
*
* @param describeTargetGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default DescribeTargetGroupsPublisher describeTargetGroupsPaginator(DescribeTargetGroupsRequest describeTargetGroupsRequest) {
return new DescribeTargetGroupsPublisher(this, describeTargetGroupsRequest);
}
/**
*
* This is a variant of
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTargetGroupsPublisher publisher = client.describeTargetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTargetGroups(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTargetGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTargetGroupsRequest#builder()}
*
*
* @param describeTargetGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetGroupsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetGroups
* @see AWS API Documentation
*/
default DescribeTargetGroupsPublisher describeTargetGroupsPaginator(
Consumer describeTargetGroupsRequest) {
return describeTargetGroupsPaginator(DescribeTargetGroupsRequest.builder().applyMutation(describeTargetGroupsRequest)
.build());
}
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
* @param describeTargetHealthRequest
* @return A Java Future containing the result of the DescribeTargetHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TargetGroupNotFoundException The specified target group does not exist.
* - HealthUnavailableException The health of the specified targets could not be retrieved due to an
* internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetHealth
* @see AWS API Documentation
*/
default CompletableFuture describeTargetHealth(
DescribeTargetHealthRequest describeTargetHealthRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the health of the specified targets or all of your targets.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTargetHealthRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTargetHealthRequest#builder()}
*
*
* @param describeTargetHealthRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTargetHealthRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeTargetHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TargetGroupNotFoundException The specified target group does not exist.
* - HealthUnavailableException The health of the specified targets could not be retrieved due to an
* internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTargetHealth
* @see AWS API Documentation
*/
default CompletableFuture describeTargetHealth(
Consumer describeTargetHealthRequest) {
return describeTargetHealth(DescribeTargetHealthRequest.builder().applyMutation(describeTargetHealthRequest).build());
}
/**
*
* Describes all resources associated with the specified trust store.
*
*
* @param describeTrustStoreAssociationsRequest
* @return A Java Future containing the result of the DescribeTrustStoreAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreAssociations
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStoreAssociations(
DescribeTrustStoreAssociationsRequest describeTrustStoreAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all resources associated with the specified trust store.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoreAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTrustStoreAssociationsRequest#builder()}
*
*
* @param describeTrustStoreAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeTrustStoreAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreAssociations
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStoreAssociations(
Consumer describeTrustStoreAssociationsRequest) {
return describeTrustStoreAssociations(DescribeTrustStoreAssociationsRequest.builder()
.applyMutation(describeTrustStoreAssociationsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeTrustStoreAssociations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreAssociationsPublisher publisher = client.describeTrustStoreAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreAssociationsPublisher publisher = client.describeTrustStoreAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStoreAssociations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest)}
* operation.
*
*
* @param describeTrustStoreAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreAssociations
* @see AWS API Documentation
*/
default DescribeTrustStoreAssociationsPublisher describeTrustStoreAssociationsPaginator(
DescribeTrustStoreAssociationsRequest describeTrustStoreAssociationsRequest) {
return new DescribeTrustStoreAssociationsPublisher(this, describeTrustStoreAssociationsRequest);
}
/**
*
* This is a variant of
* {@link #describeTrustStoreAssociations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreAssociationsPublisher publisher = client.describeTrustStoreAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreAssociationsPublisher publisher = client.describeTrustStoreAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStoreAssociations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoreAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTrustStoreAssociationsRequest#builder()}
*
*
* @param describeTrustStoreAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreAssociationsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreAssociations
* @see AWS API Documentation
*/
default DescribeTrustStoreAssociationsPublisher describeTrustStoreAssociationsPaginator(
Consumer describeTrustStoreAssociationsRequest) {
return describeTrustStoreAssociationsPaginator(DescribeTrustStoreAssociationsRequest.builder()
.applyMutation(describeTrustStoreAssociationsRequest).build());
}
/**
*
* Describes the revocation files in use by the specified trust store or revocation files.
*
*
* @param describeTrustStoreRevocationsRequest
* @return A Java Future containing the result of the DescribeTrustStoreRevocations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStoreRevocations(
DescribeTrustStoreRevocationsRequest describeTrustStoreRevocationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the revocation files in use by the specified trust store or revocation files.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoreRevocationsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTrustStoreRevocationsRequest#builder()}
*
*
* @param describeTrustStoreRevocationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeTrustStoreRevocations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStoreRevocations(
Consumer describeTrustStoreRevocationsRequest) {
return describeTrustStoreRevocations(DescribeTrustStoreRevocationsRequest.builder()
.applyMutation(describeTrustStoreRevocationsRequest).build());
}
/**
*
* This is a variant of
* {@link #describeTrustStoreRevocations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreRevocationsPublisher publisher = client.describeTrustStoreRevocationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreRevocationsPublisher publisher = client.describeTrustStoreRevocationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStoreRevocations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest)}
* operation.
*
*
* @param describeTrustStoreRevocationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreRevocations
* @see AWS API Documentation
*/
default DescribeTrustStoreRevocationsPublisher describeTrustStoreRevocationsPaginator(
DescribeTrustStoreRevocationsRequest describeTrustStoreRevocationsRequest) {
return new DescribeTrustStoreRevocationsPublisher(this, describeTrustStoreRevocationsRequest);
}
/**
*
* This is a variant of
* {@link #describeTrustStoreRevocations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreRevocationsPublisher publisher = client.describeTrustStoreRevocationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoreRevocationsPublisher publisher = client.describeTrustStoreRevocationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStoreRevocations(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoreRevocationsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTrustStoreRevocationsRequest#builder()}
*
*
* @param describeTrustStoreRevocationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoreRevocationsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStoreRevocations
* @see AWS API Documentation
*/
default DescribeTrustStoreRevocationsPublisher describeTrustStoreRevocationsPaginator(
Consumer describeTrustStoreRevocationsRequest) {
return describeTrustStoreRevocationsPaginator(DescribeTrustStoreRevocationsRequest.builder()
.applyMutation(describeTrustStoreRevocationsRequest).build());
}
/**
*
* Describes all trust stores for the specified account.
*
*
* @param describeTrustStoresRequest
* @return A Java Future containing the result of the DescribeTrustStores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStores
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStores(
DescribeTrustStoresRequest describeTrustStoresRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all trust stores for the specified account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoresRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTrustStoresRequest#builder()}
*
*
* @param describeTrustStoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeTrustStores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStores
* @see AWS API Documentation
*/
default CompletableFuture describeTrustStores(
Consumer describeTrustStoresRequest) {
return describeTrustStores(DescribeTrustStoresRequest.builder().applyMutation(describeTrustStoresRequest).build());
}
/**
*
* This is a variant of
* {@link #describeTrustStores(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoresPublisher publisher = client.describeTrustStoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoresPublisher publisher = client.describeTrustStoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStores(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest)}
* operation.
*
*
* @param describeTrustStoresRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStores
* @see AWS API Documentation
*/
default DescribeTrustStoresPublisher describeTrustStoresPaginator(DescribeTrustStoresRequest describeTrustStoresRequest) {
return new DescribeTrustStoresPublisher(this, describeTrustStoresRequest);
}
/**
*
* This is a variant of
* {@link #describeTrustStores(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoresPublisher publisher = client.describeTrustStoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.elasticloadbalancingv2.paginators.DescribeTrustStoresPublisher publisher = client.describeTrustStoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of PageSize won't limit the number of results you get with the paginator.
* It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeTrustStores(software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrustStoresRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTrustStoresRequest#builder()}
*
*
* @param describeTrustStoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.DescribeTrustStoresRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.DescribeTrustStores
* @see AWS API Documentation
*/
default DescribeTrustStoresPublisher describeTrustStoresPaginator(
Consumer describeTrustStoresRequest) {
return describeTrustStoresPaginator(DescribeTrustStoresRequest.builder().applyMutation(describeTrustStoresRequest)
.build());
}
/**
*
* Retrieves the resource policy for a specified resource.
*
*
* @param getResourcePolicyRequest
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource policy for a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetResourcePolicyRequest#builder()}
*
*
* @param getResourcePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.GetResourcePolicyRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException The specified resource does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture getResourcePolicy(
Consumer getResourcePolicyRequest) {
return getResourcePolicy(GetResourcePolicyRequest.builder().applyMutation(getResourcePolicyRequest).build());
}
/**
*
* Retrieves the ca certificate bundle.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
* @param getTrustStoreCaCertificatesBundleRequest
* @return A Java Future containing the result of the GetTrustStoreCaCertificatesBundle operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetTrustStoreCaCertificatesBundle
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreCaCertificatesBundle(
GetTrustStoreCaCertificatesBundleRequest getTrustStoreCaCertificatesBundleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the ca certificate bundle.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
*
* This is a convenience which creates an instance of the {@link GetTrustStoreCaCertificatesBundleRequest.Builder}
* avoiding the need to create one manually via {@link GetTrustStoreCaCertificatesBundleRequest#builder()}
*
*
* @param getTrustStoreCaCertificatesBundleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreCaCertificatesBundleRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetTrustStoreCaCertificatesBundle operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetTrustStoreCaCertificatesBundle
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreCaCertificatesBundle(
Consumer getTrustStoreCaCertificatesBundleRequest) {
return getTrustStoreCaCertificatesBundle(GetTrustStoreCaCertificatesBundleRequest.builder()
.applyMutation(getTrustStoreCaCertificatesBundleRequest).build());
}
/**
*
* Retrieves the specified revocation file.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
* @param getTrustStoreRevocationContentRequest
* @return A Java Future containing the result of the GetTrustStoreRevocationContent operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetTrustStoreRevocationContent
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreRevocationContent(
GetTrustStoreRevocationContentRequest getTrustStoreRevocationContentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the specified revocation file.
*
*
* This action returns a pre-signed S3 URI which is active for ten minutes.
*
*
*
* This is a convenience which creates an instance of the {@link GetTrustStoreRevocationContentRequest.Builder}
* avoiding the need to create one manually via {@link GetTrustStoreRevocationContentRequest#builder()}
*
*
* @param getTrustStoreRevocationContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.GetTrustStoreRevocationContentRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetTrustStoreRevocationContent operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.GetTrustStoreRevocationContent
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreRevocationContent(
Consumer getTrustStoreRevocationContentRequest) {
return getTrustStoreRevocationContent(GetTrustStoreRevocationContentRequest.builder()
.applyMutation(getTrustStoreRevocationContentRequest).build());
}
/**
*
* Modifies the capacity reservation of the specified load balancer.
*
*
* When modifying capacity reservation, you must include at least one MinimumLoadBalancerCapacity
or
* ResetCapacityReservation
.
*
*
* @param modifyCapacityReservationRequest
* @return A Java Future containing the result of the ModifyCapacityReservation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - CapacityUnitsLimitExceededException You've exceeded the capacity units limit.
* - CapacityReservationPendingException There is a pending capacity reservation.
* - InsufficientCapacityException There is insufficient capacity to reserve.
* - CapacityDecreaseRequestsLimitExceededException You've exceeded the daily capacity decrease limit for
* this reservation.
* - PriorRequestNotCompleteException This operation is not allowed while a prior request has not been
* completed.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyCapacityReservation
* @see AWS API Documentation
*/
default CompletableFuture modifyCapacityReservation(
ModifyCapacityReservationRequest modifyCapacityReservationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the capacity reservation of the specified load balancer.
*
*
* When modifying capacity reservation, you must include at least one MinimumLoadBalancerCapacity
or
* ResetCapacityReservation
.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyCapacityReservationRequest.Builder} avoiding
* the need to create one manually via {@link ModifyCapacityReservationRequest#builder()}
*
*
* @param modifyCapacityReservationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyCapacityReservationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ModifyCapacityReservation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - CapacityUnitsLimitExceededException You've exceeded the capacity units limit.
* - CapacityReservationPendingException There is a pending capacity reservation.
* - InsufficientCapacityException There is insufficient capacity to reserve.
* - CapacityDecreaseRequestsLimitExceededException You've exceeded the daily capacity decrease limit for
* this reservation.
* - PriorRequestNotCompleteException This operation is not allowed while a prior request has not been
* completed.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyCapacityReservation
* @see AWS API Documentation
*/
default CompletableFuture modifyCapacityReservation(
Consumer modifyCapacityReservationRequest) {
return modifyCapacityReservation(ModifyCapacityReservationRequest.builder()
.applyMutation(modifyCapacityReservationRequest).build());
}
/**
*
* Replaces the specified properties of the specified listener. Any properties that you do not specify remain
* unchanged.
*
*
* Changing the protocol from HTTPS to HTTP, or from TLS to TCP, removes the security policy and default certificate
* properties. If you change the protocol from HTTP to HTTPS, or from TCP to TLS, you must add the security policy
* and default certificate properties.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyListenerRequest
* @return A Java Future containing the result of the ModifyListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreNotReadyException The specified trust store is not active.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyListener
* @see AWS API Documentation
*/
default CompletableFuture modifyListener(ModifyListenerRequest modifyListenerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Replaces the specified properties of the specified listener. Any properties that you do not specify remain
* unchanged.
*
*
* Changing the protocol from HTTPS to HTTP, or from TLS to TCP, removes the security policy and default certificate
* properties. If you change the protocol from HTTP to HTTPS, or from TCP to TLS, you must add the security policy
* and default certificate properties.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyListenerRequest.Builder} avoiding the need to
* create one manually via {@link ModifyListenerRequest#builder()}
*
*
* @param modifyListenerRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ModifyListener operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - DuplicateListenerException A listener with the specified port already exists.
* - TooManyListenersException You've reached the limit on the number of listeners per load balancer.
* - TooManyCertificatesException You've reached the limit on the number of certificates per load
* balancer.
* - ListenerNotFoundException The specified listener does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - SslPolicyNotFoundException The specified SSL policy does not exist.
* - CertificateNotFoundException The specified certificate does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - AlpnPolicyNotSupportedException The specified ALPN policy is not supported.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - TrustStoreNotReadyException The specified trust store is not active.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyListener
* @see AWS API Documentation
*/
default CompletableFuture modifyListener(Consumer modifyListenerRequest) {
return modifyListener(ModifyListenerRequest.builder().applyMutation(modifyListenerRequest).build());
}
/**
*
* Modifies the specified attributes of the specified listener.
*
*
* @param modifyListenerAttributesRequest
* @return A Java Future containing the result of the ModifyListenerAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyListenerAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyListenerAttributes(
ModifyListenerAttributesRequest modifyListenerAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the specified attributes of the specified listener.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyListenerAttributesRequest.Builder} avoiding
* the need to create one manually via {@link ModifyListenerAttributesRequest#builder()}
*
*
* @param modifyListenerAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyListenerAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ModifyListenerAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyListenerAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyListenerAttributes(
Consumer modifyListenerAttributesRequest) {
return modifyListenerAttributes(ModifyListenerAttributesRequest.builder().applyMutation(modifyListenerAttributesRequest)
.build());
}
/**
*
* Modifies the specified attributes of the specified Application Load Balancer, Network Load Balancer, or Gateway
* Load Balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
* @param modifyLoadBalancerAttributesRequest
* @return A Java Future containing the result of the ModifyLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyLoadBalancerAttributes(
ModifyLoadBalancerAttributesRequest modifyLoadBalancerAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the specified attributes of the specified Application Load Balancer, Network Load Balancer, or Gateway
* Load Balancer.
*
*
* If any of the specified attributes can't be modified as requested, the call fails. Any existing attributes that
* you do not modify retain their current values.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyLoadBalancerAttributesRequest.Builder}
* avoiding the need to create one manually via {@link ModifyLoadBalancerAttributesRequest#builder()}
*
*
* @param modifyLoadBalancerAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyLoadBalancerAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ModifyLoadBalancerAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyLoadBalancerAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyLoadBalancerAttributes(
Consumer modifyLoadBalancerAttributesRequest) {
return modifyLoadBalancerAttributes(ModifyLoadBalancerAttributesRequest.builder()
.applyMutation(modifyLoadBalancerAttributesRequest).build());
}
/**
*
* Replaces the specified properties of the specified rule. Any properties that you do not specify are unchanged.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
* @param modifyRuleRequest
* @return A Java Future containing the result of the ModifyRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TargetGroupNotFoundException The specified target group does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyRule
* @see AWS API Documentation
*/
default CompletableFuture modifyRule(ModifyRuleRequest modifyRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Replaces the specified properties of the specified rule. Any properties that you do not specify are unchanged.
*
*
* To add an item to a list, remove an item from a list, or update an item in a list, you must provide the entire
* list. For example, to add an action, specify a list with the current actions plus the new action.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyRuleRequest.Builder} avoiding the need to
* create one manually via {@link ModifyRuleRequest#builder()}
*
*
* @param modifyRuleRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyRuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ModifyRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupAssociationLimitException You've reached the limit on the number of load balancers per
* target group.
* - IncompatibleProtocolsException The specified configuration is not valid with this protocol.
* - RuleNotFoundException The specified rule does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - TargetGroupNotFoundException The specified target group does not exist.
* - UnsupportedProtocolException The specified protocol is not supported.
* - TooManyActionsException You've reached the limit on the number of actions per rule.
* - InvalidLoadBalancerActionException The requested action is not valid.
* - TooManyUniqueTargetGroupsPerLoadBalancerException You've reached the limit on the number of unique
* target groups per load balancer across all listeners. If a target group is used by multiple actions for a
* load balancer, it is counted as only one use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyRule
* @see AWS API Documentation
*/
default CompletableFuture modifyRule(Consumer modifyRuleRequest) {
return modifyRule(ModifyRuleRequest.builder().applyMutation(modifyRuleRequest).build());
}
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
* @param modifyTargetGroupRequest
* @return A Java Future containing the result of the ModifyTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyTargetGroup(ModifyTargetGroupRequest modifyTargetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the health checks used when evaluating the health state of the targets in the specified target group.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyTargetGroupRequest.Builder} avoiding the need
* to create one manually via {@link ModifyTargetGroupRequest#builder()}
*
*
* @param modifyTargetGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ModifyTargetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroup
* @see AWS API Documentation
*/
default CompletableFuture modifyTargetGroup(
Consumer modifyTargetGroupRequest) {
return modifyTargetGroup(ModifyTargetGroupRequest.builder().applyMutation(modifyTargetGroupRequest).build());
}
/**
*
* Modifies the specified attributes of the specified target group.
*
*
* @param modifyTargetGroupAttributesRequest
* @return A Java Future containing the result of the ModifyTargetGroupAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroupAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyTargetGroupAttributes(
ModifyTargetGroupAttributesRequest modifyTargetGroupAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the specified attributes of the specified target group.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyTargetGroupAttributesRequest.Builder}
* avoiding the need to create one manually via {@link ModifyTargetGroupAttributesRequest#builder()}
*
*
* @param modifyTargetGroupAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTargetGroupAttributesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ModifyTargetGroupAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTargetGroupAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyTargetGroupAttributes(
Consumer modifyTargetGroupAttributesRequest) {
return modifyTargetGroupAttributes(ModifyTargetGroupAttributesRequest.builder()
.applyMutation(modifyTargetGroupAttributesRequest).build());
}
/**
*
* Update the ca certificate bundle for the specified trust store.
*
*
* @param modifyTrustStoreRequest
* @return A Java Future containing the result of the ModifyTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - InvalidCaCertificatesBundleException The specified ca certificate bundle is in an invalid format, or
* corrupt.
* - CaCertificatesBundleNotFoundException The specified ca certificate bundle does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTrustStore
* @see AWS API Documentation
*/
default CompletableFuture modifyTrustStore(ModifyTrustStoreRequest modifyTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Update the ca certificate bundle for the specified trust store.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link ModifyTrustStoreRequest#builder()}
*
*
* @param modifyTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.ModifyTrustStoreRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ModifyTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - InvalidCaCertificatesBundleException The specified ca certificate bundle is in an invalid format, or
* corrupt.
* - CaCertificatesBundleNotFoundException The specified ca certificate bundle does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.ModifyTrustStore
* @see AWS API Documentation
*/
default CompletableFuture modifyTrustStore(
Consumer modifyTrustStoreRequest) {
return modifyTrustStore(ModifyTrustStoreRequest.builder().applyMutation(modifyTrustStoreRequest).build());
}
/**
*
* Registers the specified targets with the specified target group.
*
*
* If the target is an EC2 instance, it must be in the running
state when you register it.
*
*
* By default, the load balancer routes requests to registered targets using the protocol and port for the target
* group. Alternatively, you can override the port for a target when you register it. You can register each EC2
* instance or IP address with the same target group multiple times using different ports.
*
*
* With a Network Load Balancer, you can't register instances by instance ID if they have the following instance
* types: C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1. You can register instances of
* these types by IP address.
*
*
* @param registerTargetsRequest
* @return A Java Future containing the result of the RegisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RegisterTargets
* @see AWS API Documentation
*/
default CompletableFuture registerTargets(RegisterTargetsRequest registerTargetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Registers the specified targets with the specified target group.
*
*
* If the target is an EC2 instance, it must be in the running
state when you register it.
*
*
* By default, the load balancer routes requests to registered targets using the protocol and port for the target
* group. Alternatively, you can override the port for a target when you register it. You can register each EC2
* instance or IP address with the same target group multiple times using different ports.
*
*
* With a Network Load Balancer, you can't register instances by instance ID if they have the following instance
* types: C1, CC1, CC2, CG1, CG2, CR1, CS1, G1, G2, HI1, HS1, M1, M2, M3, and T1. You can register instances of
* these types by IP address.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterTargetsRequest.Builder} avoiding the need
* to create one manually via {@link RegisterTargetsRequest#builder()}
*
*
* @param registerTargetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.RegisterTargetsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the RegisterTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TargetGroupNotFoundException The specified target group does not exist.
* - TooManyTargetsException You've reached the limit on the number of targets.
* - InvalidTargetException The specified target does not exist, is not in the same VPC as the target
* group, or has an unsupported instance type.
* - TooManyRegistrationsForTargetIdException You've reached the limit on the number of times a target can
* be registered with a load balancer.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RegisterTargets
* @see AWS API Documentation
*/
default CompletableFuture registerTargets(
Consumer registerTargetsRequest) {
return registerTargets(RegisterTargetsRequest.builder().applyMutation(registerTargetsRequest).build());
}
/**
*
* Removes the specified certificate from the certificate list for the specified HTTPS or TLS listener.
*
*
* @param removeListenerCertificatesRequest
* @return A Java Future containing the result of the RemoveListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture removeListenerCertificates(
RemoveListenerCertificatesRequest removeListenerCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified certificate from the certificate list for the specified HTTPS or TLS listener.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveListenerCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link RemoveListenerCertificatesRequest#builder()}
*
*
* @param removeListenerCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveListenerCertificatesRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the RemoveListenerCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ListenerNotFoundException The specified listener does not exist.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveListenerCertificates
* @see AWS API Documentation
*/
default CompletableFuture removeListenerCertificates(
Consumer removeListenerCertificatesRequest) {
return removeListenerCertificates(RemoveListenerCertificatesRequest.builder()
.applyMutation(removeListenerCertificatesRequest).build());
}
/**
*
* Removes the specified tags from the specified Elastic Load Balancing resources. You can remove the tags for one
* or more Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or
* rules.
*
*
* @param removeTagsRequest
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveTags
* @see AWS API Documentation
*/
default CompletableFuture removeTags(RemoveTagsRequest removeTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the specified Elastic Load Balancing resources. You can remove the tags for one
* or more Application Load Balancers, Network Load Balancers, Gateway Load Balancers, target groups, listeners, or
* rules.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveTagsRequest.Builder} avoiding the need to
* create one manually via {@link RemoveTagsRequest#builder()}
*
*
* @param removeTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTagsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - TargetGroupNotFoundException The specified target group does not exist.
* - ListenerNotFoundException The specified listener does not exist.
* - RuleNotFoundException The specified rule does not exist.
* - TooManyTagsException You've reached the limit on the number of tags for this resource.
* - TrustStoreNotFoundException The specified trust store does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveTags
* @see AWS API Documentation
*/
default CompletableFuture removeTags(Consumer removeTagsRequest) {
return removeTags(RemoveTagsRequest.builder().applyMutation(removeTagsRequest).build());
}
/**
*
* Removes the specified revocation file from the specified trust store.
*
*
* @param removeTrustStoreRevocationsRequest
* @return A Java Future containing the result of the RemoveTrustStoreRevocations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture removeTrustStoreRevocations(
RemoveTrustStoreRevocationsRequest removeTrustStoreRevocationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified revocation file from the specified trust store.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveTrustStoreRevocationsRequest.Builder}
* avoiding the need to create one manually via {@link RemoveTrustStoreRevocationsRequest#builder()}
*
*
* @param removeTrustStoreRevocationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.RemoveTrustStoreRevocationsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the RemoveTrustStoreRevocations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TrustStoreNotFoundException The specified trust store does not exist.
* - RevocationIdNotFoundException The specified revocation ID does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.RemoveTrustStoreRevocations
* @see AWS API Documentation
*/
default CompletableFuture removeTrustStoreRevocations(
Consumer removeTrustStoreRevocationsRequest) {
return removeTrustStoreRevocations(RemoveTrustStoreRevocationsRequest.builder()
.applyMutation(removeTrustStoreRevocationsRequest).build());
}
/**
*
* Sets the type of IP addresses used by the subnets of the specified load balancer.
*
*
* @param setIpAddressTypeRequest
* @return A Java Future containing the result of the SetIpAddressType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetIpAddressType
* @see AWS API Documentation
*/
default CompletableFuture setIpAddressType(SetIpAddressTypeRequest setIpAddressTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the type of IP addresses used by the subnets of the specified load balancer.
*
*
*
* This is a convenience which creates an instance of the {@link SetIpAddressTypeRequest.Builder} avoiding the need
* to create one manually via {@link SetIpAddressTypeRequest#builder()}
*
*
* @param setIpAddressTypeRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SetIpAddressTypeRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the SetIpAddressType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetIpAddressType
* @see AWS API Documentation
*/
default CompletableFuture setIpAddressType(
Consumer setIpAddressTypeRequest) {
return setIpAddressType(SetIpAddressTypeRequest.builder().applyMutation(setIpAddressTypeRequest).build());
}
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
* @param setRulePrioritiesRequest
* @return A Java Future containing the result of the SetRulePriorities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleNotFoundException The specified rule does not exist.
* - PriorityInUseException The specified priority is in use.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetRulePriorities
* @see AWS API Documentation
*/
default CompletableFuture setRulePriorities(SetRulePrioritiesRequest setRulePrioritiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the priorities of the specified rules.
*
*
* You can reorder the rules as long as there are no priority conflicts in the new order. Any existing rules that
* you do not specify retain their current priority.
*
*
*
* This is a convenience which creates an instance of the {@link SetRulePrioritiesRequest.Builder} avoiding the need
* to create one manually via {@link SetRulePrioritiesRequest#builder()}
*
*
* @param setRulePrioritiesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SetRulePrioritiesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the SetRulePriorities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleNotFoundException The specified rule does not exist.
* - PriorityInUseException The specified priority is in use.
* - OperationNotPermittedException This operation is not allowed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetRulePriorities
* @see AWS API Documentation
*/
default CompletableFuture setRulePriorities(
Consumer setRulePrioritiesRequest) {
return setRulePriorities(SetRulePrioritiesRequest.builder().applyMutation(setRulePrioritiesRequest).build());
}
/**
*
* Associates the specified security groups with the specified Application Load Balancer or Network Load Balancer.
* The specified security groups override the previously associated security groups.
*
*
* You can't perform this operation on a Network Load Balancer unless you specified a security group for the load
* balancer when you created it.
*
*
* You can't associate a security group with a Gateway Load Balancer.
*
*
* @param setSecurityGroupsRequest
* @return A Java Future containing the result of the SetSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSecurityGroupException The specified security group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSecurityGroups
* @see AWS API Documentation
*/
default CompletableFuture setSecurityGroups(SetSecurityGroupsRequest setSecurityGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified security groups with the specified Application Load Balancer or Network Load Balancer.
* The specified security groups override the previously associated security groups.
*
*
* You can't perform this operation on a Network Load Balancer unless you specified a security group for the load
* balancer when you created it.
*
*
* You can't associate a security group with a Gateway Load Balancer.
*
*
*
* This is a convenience which creates an instance of the {@link SetSecurityGroupsRequest.Builder} avoiding the need
* to create one manually via {@link SetSecurityGroupsRequest#builder()}
*
*
* @param setSecurityGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSecurityGroupsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the SetSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - InvalidSecurityGroupException The specified security group does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSecurityGroups
* @see AWS API Documentation
*/
default CompletableFuture setSecurityGroups(
Consumer setSecurityGroupsRequest) {
return setSecurityGroups(SetSecurityGroupsRequest.builder().applyMutation(setSecurityGroupsRequest).build());
}
/**
*
* Enables the Availability Zones for the specified public subnets for the specified Application Load Balancer,
* Network Load Balancer or Gateway Load Balancer. The specified subnets replace the previously enabled subnets.
*
*
* When you specify subnets for a Network Load Balancer, or Gateway Load Balancer you must include all subnets that
* were enabled previously, with their existing configurations, plus any additional subnets.
*
*
* @param setSubnetsRequest
* @return A Java Future containing the result of the SetSubnets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - CapacityReservationPendingException There is a pending capacity reservation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSubnets
* @see AWS API Documentation
*/
default CompletableFuture setSubnets(SetSubnetsRequest setSubnetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enables the Availability Zones for the specified public subnets for the specified Application Load Balancer,
* Network Load Balancer or Gateway Load Balancer. The specified subnets replace the previously enabled subnets.
*
*
* When you specify subnets for a Network Load Balancer, or Gateway Load Balancer you must include all subnets that
* were enabled previously, with their existing configurations, plus any additional subnets.
*
*
*
* This is a convenience which creates an instance of the {@link SetSubnetsRequest.Builder} avoiding the need to
* create one manually via {@link SetSubnetsRequest#builder()}
*
*
* @param setSubnetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.elasticloadbalancingv2.model.SetSubnetsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SetSubnets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LoadBalancerNotFoundException The specified load balancer does not exist.
* - InvalidConfigurationRequestException The requested configuration is not valid.
* - SubnetNotFoundException The specified subnet does not exist.
* - InvalidSubnetException The specified subnet is out of available addresses.
* - AllocationIdNotFoundException The specified allocation ID does not exist.
* - AvailabilityZoneNotSupportedException The specified Availability Zone is not supported.
* - CapacityReservationPendingException There is a pending capacity reservation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ElasticLoadBalancingV2Exception Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ElasticLoadBalancingV2AsyncClient.SetSubnets
* @see AWS API Documentation
*/
default CompletableFuture setSubnets(Consumer setSubnetsRequest) {
return setSubnets(SetSubnetsRequest.builder().applyMutation(setSubnetsRequest).build());
}
/**
* Create an instance of {@link ElasticLoadBalancingV2AsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link ElasticLoadBalancingV2AsyncWaiter}
*/
default ElasticLoadBalancingV2AsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
@Override
default ElasticLoadBalancingV2ServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link ElasticLoadBalancingV2AsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ElasticLoadBalancingV2AsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ElasticLoadBalancingV2AsyncClient}.
*/
static ElasticLoadBalancingV2AsyncClientBuilder builder() {
return new DefaultElasticLoadBalancingV2AsyncClientBuilder();
}
}